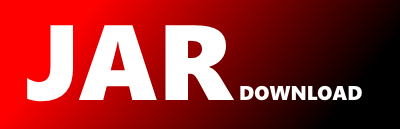
com.amazonaws.services.dlm.model.Schedule Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.dlm.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* [Custom snapshot and AMI policies only] Specifies a schedule for a snapshot or AMI lifecycle policy.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Schedule implements Serializable, Cloneable, StructuredPojo {
/**
*
* The name of the schedule.
*
*/
private String name;
/**
*
* Copy all user-defined tags on a source volume to snapshots of the volume created by this policy.
*
*/
private Boolean copyTags;
/**
*
* The tags to apply to policy-created resources. These user-defined tags are in addition to the Amazon Web
* Services-added lifecycle tags.
*
*/
private java.util.List tagsToAdd;
/**
*
* [AMI policies and snapshot policies that target instances only] A collection of key/value pairs with
* values determined dynamically when the policy is executed. Keys may be any valid Amazon EC2 tag key. Values must
* be in one of the two following formats: $(instance-id)
or $(timestamp)
. Variable tags
* are only valid for EBS Snapshot Management – Instance policies.
*
*/
private java.util.List variableTags;
/**
*
* The creation rule.
*
*/
private CreateRule createRule;
/**
*
* The retention rule for snapshots or AMIs created by the policy.
*
*/
private RetainRule retainRule;
/**
*
* [Custom snapshot policies only] The rule for enabling fast snapshot restore.
*
*/
private FastRestoreRule fastRestoreRule;
/**
*
* Specifies a rule for copying snapshots or AMIs across regions.
*
*
*
* You can't specify cross-Region copy rules for policies that create snapshots on an Outpost. If the policy creates
* snapshots in a Region, then snapshots can be copied to up to three Regions or Outposts.
*
*
*/
private java.util.List crossRegionCopyRules;
/**
*
* [Custom snapshot policies only] The rule for sharing snapshots with other Amazon Web Services accounts.
*
*/
private java.util.List shareRules;
/**
*
* [Custom AMI policies only] The AMI deprecation rule for the schedule.
*
*/
private DeprecateRule deprecateRule;
/**
*
* [Custom snapshot policies that target volumes only] The snapshot archiving rule for the schedule. When you
* specify an archiving rule, snapshots are automatically moved from the standard tier to the archive tier once the
* schedule's retention threshold is met. Snapshots are then retained in the archive tier for the archive retention
* period that you specify.
*
*
* For more information about using snapshot archiving, see Considerations
* for snapshot lifecycle policies.
*
*/
private ArchiveRule archiveRule;
/**
*
* The name of the schedule.
*
*
* @param name
* The name of the schedule.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the schedule.
*
*
* @return The name of the schedule.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the schedule.
*
*
* @param name
* The name of the schedule.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Schedule withName(String name) {
setName(name);
return this;
}
/**
*
* Copy all user-defined tags on a source volume to snapshots of the volume created by this policy.
*
*
* @param copyTags
* Copy all user-defined tags on a source volume to snapshots of the volume created by this policy.
*/
public void setCopyTags(Boolean copyTags) {
this.copyTags = copyTags;
}
/**
*
* Copy all user-defined tags on a source volume to snapshots of the volume created by this policy.
*
*
* @return Copy all user-defined tags on a source volume to snapshots of the volume created by this policy.
*/
public Boolean getCopyTags() {
return this.copyTags;
}
/**
*
* Copy all user-defined tags on a source volume to snapshots of the volume created by this policy.
*
*
* @param copyTags
* Copy all user-defined tags on a source volume to snapshots of the volume created by this policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Schedule withCopyTags(Boolean copyTags) {
setCopyTags(copyTags);
return this;
}
/**
*
* Copy all user-defined tags on a source volume to snapshots of the volume created by this policy.
*
*
* @return Copy all user-defined tags on a source volume to snapshots of the volume created by this policy.
*/
public Boolean isCopyTags() {
return this.copyTags;
}
/**
*
* The tags to apply to policy-created resources. These user-defined tags are in addition to the Amazon Web
* Services-added lifecycle tags.
*
*
* @return The tags to apply to policy-created resources. These user-defined tags are in addition to the Amazon Web
* Services-added lifecycle tags.
*/
public java.util.List getTagsToAdd() {
return tagsToAdd;
}
/**
*
* The tags to apply to policy-created resources. These user-defined tags are in addition to the Amazon Web
* Services-added lifecycle tags.
*
*
* @param tagsToAdd
* The tags to apply to policy-created resources. These user-defined tags are in addition to the Amazon Web
* Services-added lifecycle tags.
*/
public void setTagsToAdd(java.util.Collection tagsToAdd) {
if (tagsToAdd == null) {
this.tagsToAdd = null;
return;
}
this.tagsToAdd = new java.util.ArrayList(tagsToAdd);
}
/**
*
* The tags to apply to policy-created resources. These user-defined tags are in addition to the Amazon Web
* Services-added lifecycle tags.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTagsToAdd(java.util.Collection)} or {@link #withTagsToAdd(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param tagsToAdd
* The tags to apply to policy-created resources. These user-defined tags are in addition to the Amazon Web
* Services-added lifecycle tags.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Schedule withTagsToAdd(Tag... tagsToAdd) {
if (this.tagsToAdd == null) {
setTagsToAdd(new java.util.ArrayList(tagsToAdd.length));
}
for (Tag ele : tagsToAdd) {
this.tagsToAdd.add(ele);
}
return this;
}
/**
*
* The tags to apply to policy-created resources. These user-defined tags are in addition to the Amazon Web
* Services-added lifecycle tags.
*
*
* @param tagsToAdd
* The tags to apply to policy-created resources. These user-defined tags are in addition to the Amazon Web
* Services-added lifecycle tags.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Schedule withTagsToAdd(java.util.Collection tagsToAdd) {
setTagsToAdd(tagsToAdd);
return this;
}
/**
*
* [AMI policies and snapshot policies that target instances only] A collection of key/value pairs with
* values determined dynamically when the policy is executed. Keys may be any valid Amazon EC2 tag key. Values must
* be in one of the two following formats: $(instance-id)
or $(timestamp)
. Variable tags
* are only valid for EBS Snapshot Management – Instance policies.
*
*
* @return [AMI policies and snapshot policies that target instances only] A collection of key/value pairs
* with values determined dynamically when the policy is executed. Keys may be any valid Amazon EC2 tag key.
* Values must be in one of the two following formats: $(instance-id)
or
* $(timestamp)
. Variable tags are only valid for EBS Snapshot Management – Instance policies.
*/
public java.util.List getVariableTags() {
return variableTags;
}
/**
*
* [AMI policies and snapshot policies that target instances only] A collection of key/value pairs with
* values determined dynamically when the policy is executed. Keys may be any valid Amazon EC2 tag key. Values must
* be in one of the two following formats: $(instance-id)
or $(timestamp)
. Variable tags
* are only valid for EBS Snapshot Management – Instance policies.
*
*
* @param variableTags
* [AMI policies and snapshot policies that target instances only] A collection of key/value pairs
* with values determined dynamically when the policy is executed. Keys may be any valid Amazon EC2 tag key.
* Values must be in one of the two following formats: $(instance-id)
or
* $(timestamp)
. Variable tags are only valid for EBS Snapshot Management – Instance policies.
*/
public void setVariableTags(java.util.Collection variableTags) {
if (variableTags == null) {
this.variableTags = null;
return;
}
this.variableTags = new java.util.ArrayList(variableTags);
}
/**
*
* [AMI policies and snapshot policies that target instances only] A collection of key/value pairs with
* values determined dynamically when the policy is executed. Keys may be any valid Amazon EC2 tag key. Values must
* be in one of the two following formats: $(instance-id)
or $(timestamp)
. Variable tags
* are only valid for EBS Snapshot Management – Instance policies.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setVariableTags(java.util.Collection)} or {@link #withVariableTags(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param variableTags
* [AMI policies and snapshot policies that target instances only] A collection of key/value pairs
* with values determined dynamically when the policy is executed. Keys may be any valid Amazon EC2 tag key.
* Values must be in one of the two following formats: $(instance-id)
or
* $(timestamp)
. Variable tags are only valid for EBS Snapshot Management – Instance policies.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Schedule withVariableTags(Tag... variableTags) {
if (this.variableTags == null) {
setVariableTags(new java.util.ArrayList(variableTags.length));
}
for (Tag ele : variableTags) {
this.variableTags.add(ele);
}
return this;
}
/**
*
* [AMI policies and snapshot policies that target instances only] A collection of key/value pairs with
* values determined dynamically when the policy is executed. Keys may be any valid Amazon EC2 tag key. Values must
* be in one of the two following formats: $(instance-id)
or $(timestamp)
. Variable tags
* are only valid for EBS Snapshot Management – Instance policies.
*
*
* @param variableTags
* [AMI policies and snapshot policies that target instances only] A collection of key/value pairs
* with values determined dynamically when the policy is executed. Keys may be any valid Amazon EC2 tag key.
* Values must be in one of the two following formats: $(instance-id)
or
* $(timestamp)
. Variable tags are only valid for EBS Snapshot Management – Instance policies.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Schedule withVariableTags(java.util.Collection variableTags) {
setVariableTags(variableTags);
return this;
}
/**
*
* The creation rule.
*
*
* @param createRule
* The creation rule.
*/
public void setCreateRule(CreateRule createRule) {
this.createRule = createRule;
}
/**
*
* The creation rule.
*
*
* @return The creation rule.
*/
public CreateRule getCreateRule() {
return this.createRule;
}
/**
*
* The creation rule.
*
*
* @param createRule
* The creation rule.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Schedule withCreateRule(CreateRule createRule) {
setCreateRule(createRule);
return this;
}
/**
*
* The retention rule for snapshots or AMIs created by the policy.
*
*
* @param retainRule
* The retention rule for snapshots or AMIs created by the policy.
*/
public void setRetainRule(RetainRule retainRule) {
this.retainRule = retainRule;
}
/**
*
* The retention rule for snapshots or AMIs created by the policy.
*
*
* @return The retention rule for snapshots or AMIs created by the policy.
*/
public RetainRule getRetainRule() {
return this.retainRule;
}
/**
*
* The retention rule for snapshots or AMIs created by the policy.
*
*
* @param retainRule
* The retention rule for snapshots or AMIs created by the policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Schedule withRetainRule(RetainRule retainRule) {
setRetainRule(retainRule);
return this;
}
/**
*
* [Custom snapshot policies only] The rule for enabling fast snapshot restore.
*
*
* @param fastRestoreRule
* [Custom snapshot policies only] The rule for enabling fast snapshot restore.
*/
public void setFastRestoreRule(FastRestoreRule fastRestoreRule) {
this.fastRestoreRule = fastRestoreRule;
}
/**
*
* [Custom snapshot policies only] The rule for enabling fast snapshot restore.
*
*
* @return [Custom snapshot policies only] The rule for enabling fast snapshot restore.
*/
public FastRestoreRule getFastRestoreRule() {
return this.fastRestoreRule;
}
/**
*
* [Custom snapshot policies only] The rule for enabling fast snapshot restore.
*
*
* @param fastRestoreRule
* [Custom snapshot policies only] The rule for enabling fast snapshot restore.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Schedule withFastRestoreRule(FastRestoreRule fastRestoreRule) {
setFastRestoreRule(fastRestoreRule);
return this;
}
/**
*
* Specifies a rule for copying snapshots or AMIs across regions.
*
*
*
* You can't specify cross-Region copy rules for policies that create snapshots on an Outpost. If the policy creates
* snapshots in a Region, then snapshots can be copied to up to three Regions or Outposts.
*
*
*
* @return Specifies a rule for copying snapshots or AMIs across regions.
*
* You can't specify cross-Region copy rules for policies that create snapshots on an Outpost. If the policy
* creates snapshots in a Region, then snapshots can be copied to up to three Regions or Outposts.
*
*/
public java.util.List getCrossRegionCopyRules() {
return crossRegionCopyRules;
}
/**
*
* Specifies a rule for copying snapshots or AMIs across regions.
*
*
*
* You can't specify cross-Region copy rules for policies that create snapshots on an Outpost. If the policy creates
* snapshots in a Region, then snapshots can be copied to up to three Regions or Outposts.
*
*
*
* @param crossRegionCopyRules
* Specifies a rule for copying snapshots or AMIs across regions.
*
* You can't specify cross-Region copy rules for policies that create snapshots on an Outpost. If the policy
* creates snapshots in a Region, then snapshots can be copied to up to three Regions or Outposts.
*
*/
public void setCrossRegionCopyRules(java.util.Collection crossRegionCopyRules) {
if (crossRegionCopyRules == null) {
this.crossRegionCopyRules = null;
return;
}
this.crossRegionCopyRules = new java.util.ArrayList(crossRegionCopyRules);
}
/**
*
* Specifies a rule for copying snapshots or AMIs across regions.
*
*
*
* You can't specify cross-Region copy rules for policies that create snapshots on an Outpost. If the policy creates
* snapshots in a Region, then snapshots can be copied to up to three Regions or Outposts.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCrossRegionCopyRules(java.util.Collection)} or {@link #withCrossRegionCopyRules(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param crossRegionCopyRules
* Specifies a rule for copying snapshots or AMIs across regions.
*
* You can't specify cross-Region copy rules for policies that create snapshots on an Outpost. If the policy
* creates snapshots in a Region, then snapshots can be copied to up to three Regions or Outposts.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Schedule withCrossRegionCopyRules(CrossRegionCopyRule... crossRegionCopyRules) {
if (this.crossRegionCopyRules == null) {
setCrossRegionCopyRules(new java.util.ArrayList(crossRegionCopyRules.length));
}
for (CrossRegionCopyRule ele : crossRegionCopyRules) {
this.crossRegionCopyRules.add(ele);
}
return this;
}
/**
*
* Specifies a rule for copying snapshots or AMIs across regions.
*
*
*
* You can't specify cross-Region copy rules for policies that create snapshots on an Outpost. If the policy creates
* snapshots in a Region, then snapshots can be copied to up to three Regions or Outposts.
*
*
*
* @param crossRegionCopyRules
* Specifies a rule for copying snapshots or AMIs across regions.
*
* You can't specify cross-Region copy rules for policies that create snapshots on an Outpost. If the policy
* creates snapshots in a Region, then snapshots can be copied to up to three Regions or Outposts.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Schedule withCrossRegionCopyRules(java.util.Collection crossRegionCopyRules) {
setCrossRegionCopyRules(crossRegionCopyRules);
return this;
}
/**
*
* [Custom snapshot policies only] The rule for sharing snapshots with other Amazon Web Services accounts.
*
*
* @return [Custom snapshot policies only] The rule for sharing snapshots with other Amazon Web Services
* accounts.
*/
public java.util.List getShareRules() {
return shareRules;
}
/**
*
* [Custom snapshot policies only] The rule for sharing snapshots with other Amazon Web Services accounts.
*
*
* @param shareRules
* [Custom snapshot policies only] The rule for sharing snapshots with other Amazon Web Services
* accounts.
*/
public void setShareRules(java.util.Collection shareRules) {
if (shareRules == null) {
this.shareRules = null;
return;
}
this.shareRules = new java.util.ArrayList(shareRules);
}
/**
*
* [Custom snapshot policies only] The rule for sharing snapshots with other Amazon Web Services accounts.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setShareRules(java.util.Collection)} or {@link #withShareRules(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param shareRules
* [Custom snapshot policies only] The rule for sharing snapshots with other Amazon Web Services
* accounts.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Schedule withShareRules(ShareRule... shareRules) {
if (this.shareRules == null) {
setShareRules(new java.util.ArrayList(shareRules.length));
}
for (ShareRule ele : shareRules) {
this.shareRules.add(ele);
}
return this;
}
/**
*
* [Custom snapshot policies only] The rule for sharing snapshots with other Amazon Web Services accounts.
*
*
* @param shareRules
* [Custom snapshot policies only] The rule for sharing snapshots with other Amazon Web Services
* accounts.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Schedule withShareRules(java.util.Collection shareRules) {
setShareRules(shareRules);
return this;
}
/**
*
* [Custom AMI policies only] The AMI deprecation rule for the schedule.
*
*
* @param deprecateRule
* [Custom AMI policies only] The AMI deprecation rule for the schedule.
*/
public void setDeprecateRule(DeprecateRule deprecateRule) {
this.deprecateRule = deprecateRule;
}
/**
*
* [Custom AMI policies only] The AMI deprecation rule for the schedule.
*
*
* @return [Custom AMI policies only] The AMI deprecation rule for the schedule.
*/
public DeprecateRule getDeprecateRule() {
return this.deprecateRule;
}
/**
*
* [Custom AMI policies only] The AMI deprecation rule for the schedule.
*
*
* @param deprecateRule
* [Custom AMI policies only] The AMI deprecation rule for the schedule.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Schedule withDeprecateRule(DeprecateRule deprecateRule) {
setDeprecateRule(deprecateRule);
return this;
}
/**
*
* [Custom snapshot policies that target volumes only] The snapshot archiving rule for the schedule. When you
* specify an archiving rule, snapshots are automatically moved from the standard tier to the archive tier once the
* schedule's retention threshold is met. Snapshots are then retained in the archive tier for the archive retention
* period that you specify.
*
*
* For more information about using snapshot archiving, see Considerations
* for snapshot lifecycle policies.
*
*
* @param archiveRule
* [Custom snapshot policies that target volumes only] The snapshot archiving rule for the schedule.
* When you specify an archiving rule, snapshots are automatically moved from the standard tier to the
* archive tier once the schedule's retention threshold is met. Snapshots are then retained in the archive
* tier for the archive retention period that you specify.
*
* For more information about using snapshot archiving, see Considerations for snapshot lifecycle policies.
*/
public void setArchiveRule(ArchiveRule archiveRule) {
this.archiveRule = archiveRule;
}
/**
*
* [Custom snapshot policies that target volumes only] The snapshot archiving rule for the schedule. When you
* specify an archiving rule, snapshots are automatically moved from the standard tier to the archive tier once the
* schedule's retention threshold is met. Snapshots are then retained in the archive tier for the archive retention
* period that you specify.
*
*
* For more information about using snapshot archiving, see Considerations
* for snapshot lifecycle policies.
*
*
* @return [Custom snapshot policies that target volumes only] The snapshot archiving rule for the schedule.
* When you specify an archiving rule, snapshots are automatically moved from the standard tier to the
* archive tier once the schedule's retention threshold is met. Snapshots are then retained in the archive
* tier for the archive retention period that you specify.
*
* For more information about using snapshot archiving, see Considerations for snapshot lifecycle policies.
*/
public ArchiveRule getArchiveRule() {
return this.archiveRule;
}
/**
*
* [Custom snapshot policies that target volumes only] The snapshot archiving rule for the schedule. When you
* specify an archiving rule, snapshots are automatically moved from the standard tier to the archive tier once the
* schedule's retention threshold is met. Snapshots are then retained in the archive tier for the archive retention
* period that you specify.
*
*
* For more information about using snapshot archiving, see Considerations
* for snapshot lifecycle policies.
*
*
* @param archiveRule
* [Custom snapshot policies that target volumes only] The snapshot archiving rule for the schedule.
* When you specify an archiving rule, snapshots are automatically moved from the standard tier to the
* archive tier once the schedule's retention threshold is met. Snapshots are then retained in the archive
* tier for the archive retention period that you specify.
*
* For more information about using snapshot archiving, see Considerations for snapshot lifecycle policies.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Schedule withArchiveRule(ArchiveRule archiveRule) {
setArchiveRule(archiveRule);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getCopyTags() != null)
sb.append("CopyTags: ").append(getCopyTags()).append(",");
if (getTagsToAdd() != null)
sb.append("TagsToAdd: ").append(getTagsToAdd()).append(",");
if (getVariableTags() != null)
sb.append("VariableTags: ").append(getVariableTags()).append(",");
if (getCreateRule() != null)
sb.append("CreateRule: ").append(getCreateRule()).append(",");
if (getRetainRule() != null)
sb.append("RetainRule: ").append(getRetainRule()).append(",");
if (getFastRestoreRule() != null)
sb.append("FastRestoreRule: ").append(getFastRestoreRule()).append(",");
if (getCrossRegionCopyRules() != null)
sb.append("CrossRegionCopyRules: ").append(getCrossRegionCopyRules()).append(",");
if (getShareRules() != null)
sb.append("ShareRules: ").append(getShareRules()).append(",");
if (getDeprecateRule() != null)
sb.append("DeprecateRule: ").append(getDeprecateRule()).append(",");
if (getArchiveRule() != null)
sb.append("ArchiveRule: ").append(getArchiveRule());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Schedule == false)
return false;
Schedule other = (Schedule) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getCopyTags() == null ^ this.getCopyTags() == null)
return false;
if (other.getCopyTags() != null && other.getCopyTags().equals(this.getCopyTags()) == false)
return false;
if (other.getTagsToAdd() == null ^ this.getTagsToAdd() == null)
return false;
if (other.getTagsToAdd() != null && other.getTagsToAdd().equals(this.getTagsToAdd()) == false)
return false;
if (other.getVariableTags() == null ^ this.getVariableTags() == null)
return false;
if (other.getVariableTags() != null && other.getVariableTags().equals(this.getVariableTags()) == false)
return false;
if (other.getCreateRule() == null ^ this.getCreateRule() == null)
return false;
if (other.getCreateRule() != null && other.getCreateRule().equals(this.getCreateRule()) == false)
return false;
if (other.getRetainRule() == null ^ this.getRetainRule() == null)
return false;
if (other.getRetainRule() != null && other.getRetainRule().equals(this.getRetainRule()) == false)
return false;
if (other.getFastRestoreRule() == null ^ this.getFastRestoreRule() == null)
return false;
if (other.getFastRestoreRule() != null && other.getFastRestoreRule().equals(this.getFastRestoreRule()) == false)
return false;
if (other.getCrossRegionCopyRules() == null ^ this.getCrossRegionCopyRules() == null)
return false;
if (other.getCrossRegionCopyRules() != null && other.getCrossRegionCopyRules().equals(this.getCrossRegionCopyRules()) == false)
return false;
if (other.getShareRules() == null ^ this.getShareRules() == null)
return false;
if (other.getShareRules() != null && other.getShareRules().equals(this.getShareRules()) == false)
return false;
if (other.getDeprecateRule() == null ^ this.getDeprecateRule() == null)
return false;
if (other.getDeprecateRule() != null && other.getDeprecateRule().equals(this.getDeprecateRule()) == false)
return false;
if (other.getArchiveRule() == null ^ this.getArchiveRule() == null)
return false;
if (other.getArchiveRule() != null && other.getArchiveRule().equals(this.getArchiveRule()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getCopyTags() == null) ? 0 : getCopyTags().hashCode());
hashCode = prime * hashCode + ((getTagsToAdd() == null) ? 0 : getTagsToAdd().hashCode());
hashCode = prime * hashCode + ((getVariableTags() == null) ? 0 : getVariableTags().hashCode());
hashCode = prime * hashCode + ((getCreateRule() == null) ? 0 : getCreateRule().hashCode());
hashCode = prime * hashCode + ((getRetainRule() == null) ? 0 : getRetainRule().hashCode());
hashCode = prime * hashCode + ((getFastRestoreRule() == null) ? 0 : getFastRestoreRule().hashCode());
hashCode = prime * hashCode + ((getCrossRegionCopyRules() == null) ? 0 : getCrossRegionCopyRules().hashCode());
hashCode = prime * hashCode + ((getShareRules() == null) ? 0 : getShareRules().hashCode());
hashCode = prime * hashCode + ((getDeprecateRule() == null) ? 0 : getDeprecateRule().hashCode());
hashCode = prime * hashCode + ((getArchiveRule() == null) ? 0 : getArchiveRule().hashCode());
return hashCode;
}
@Override
public Schedule clone() {
try {
return (Schedule) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.dlm.model.transform.ScheduleMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}