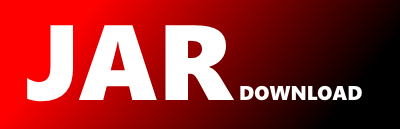
com.amazonaws.services.dlm.model.GetLifecyclePoliciesRequest Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.dlm.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetLifecyclePoliciesRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The identifiers of the data lifecycle policies.
*
*/
private java.util.List policyIds;
/**
*
* The activation state.
*
*/
private String state;
/**
*
* The resource type.
*
*/
private java.util.List resourceTypes;
/**
*
* The target tag for a policy.
*
*
* Tags are strings in the format key=value
.
*
*/
private java.util.List targetTags;
/**
*
* The tags to add to objects created by the policy.
*
*
* Tags are strings in the format key=value
.
*
*
* These user-defined tags are added in addition to the Amazon Web Services-added lifecycle tags.
*
*/
private java.util.List tagsToAdd;
/**
*
* [Default policies only] Specifies the type of default policy to get. Specify one of the following:
*
*
* -
*
* VOLUME
- To get only the default policy for EBS snapshots
*
*
* -
*
* INSTANCE
- To get only the default policy for EBS-backed AMIs
*
*
* -
*
* ALL
- To get all default policies
*
*
*
*/
private String defaultPolicyType;
/**
*
* The identifiers of the data lifecycle policies.
*
*
* @return The identifiers of the data lifecycle policies.
*/
public java.util.List getPolicyIds() {
return policyIds;
}
/**
*
* The identifiers of the data lifecycle policies.
*
*
* @param policyIds
* The identifiers of the data lifecycle policies.
*/
public void setPolicyIds(java.util.Collection policyIds) {
if (policyIds == null) {
this.policyIds = null;
return;
}
this.policyIds = new java.util.ArrayList(policyIds);
}
/**
*
* The identifiers of the data lifecycle policies.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPolicyIds(java.util.Collection)} or {@link #withPolicyIds(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param policyIds
* The identifiers of the data lifecycle policies.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetLifecyclePoliciesRequest withPolicyIds(String... policyIds) {
if (this.policyIds == null) {
setPolicyIds(new java.util.ArrayList(policyIds.length));
}
for (String ele : policyIds) {
this.policyIds.add(ele);
}
return this;
}
/**
*
* The identifiers of the data lifecycle policies.
*
*
* @param policyIds
* The identifiers of the data lifecycle policies.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetLifecyclePoliciesRequest withPolicyIds(java.util.Collection policyIds) {
setPolicyIds(policyIds);
return this;
}
/**
*
* The activation state.
*
*
* @param state
* The activation state.
* @see GettablePolicyStateValues
*/
public void setState(String state) {
this.state = state;
}
/**
*
* The activation state.
*
*
* @return The activation state.
* @see GettablePolicyStateValues
*/
public String getState() {
return this.state;
}
/**
*
* The activation state.
*
*
* @param state
* The activation state.
* @return Returns a reference to this object so that method calls can be chained together.
* @see GettablePolicyStateValues
*/
public GetLifecyclePoliciesRequest withState(String state) {
setState(state);
return this;
}
/**
*
* The activation state.
*
*
* @param state
* The activation state.
* @return Returns a reference to this object so that method calls can be chained together.
* @see GettablePolicyStateValues
*/
public GetLifecyclePoliciesRequest withState(GettablePolicyStateValues state) {
this.state = state.toString();
return this;
}
/**
*
* The resource type.
*
*
* @return The resource type.
* @see ResourceTypeValues
*/
public java.util.List getResourceTypes() {
return resourceTypes;
}
/**
*
* The resource type.
*
*
* @param resourceTypes
* The resource type.
* @see ResourceTypeValues
*/
public void setResourceTypes(java.util.Collection resourceTypes) {
if (resourceTypes == null) {
this.resourceTypes = null;
return;
}
this.resourceTypes = new java.util.ArrayList(resourceTypes);
}
/**
*
* The resource type.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setResourceTypes(java.util.Collection)} or {@link #withResourceTypes(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param resourceTypes
* The resource type.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResourceTypeValues
*/
public GetLifecyclePoliciesRequest withResourceTypes(String... resourceTypes) {
if (this.resourceTypes == null) {
setResourceTypes(new java.util.ArrayList(resourceTypes.length));
}
for (String ele : resourceTypes) {
this.resourceTypes.add(ele);
}
return this;
}
/**
*
* The resource type.
*
*
* @param resourceTypes
* The resource type.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResourceTypeValues
*/
public GetLifecyclePoliciesRequest withResourceTypes(java.util.Collection resourceTypes) {
setResourceTypes(resourceTypes);
return this;
}
/**
*
* The resource type.
*
*
* @param resourceTypes
* The resource type.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResourceTypeValues
*/
public GetLifecyclePoliciesRequest withResourceTypes(ResourceTypeValues... resourceTypes) {
java.util.ArrayList resourceTypesCopy = new java.util.ArrayList(resourceTypes.length);
for (ResourceTypeValues value : resourceTypes) {
resourceTypesCopy.add(value.toString());
}
if (getResourceTypes() == null) {
setResourceTypes(resourceTypesCopy);
} else {
getResourceTypes().addAll(resourceTypesCopy);
}
return this;
}
/**
*
* The target tag for a policy.
*
*
* Tags are strings in the format key=value
.
*
*
* @return The target tag for a policy.
*
* Tags are strings in the format key=value
.
*/
public java.util.List getTargetTags() {
return targetTags;
}
/**
*
* The target tag for a policy.
*
*
* Tags are strings in the format key=value
.
*
*
* @param targetTags
* The target tag for a policy.
*
* Tags are strings in the format key=value
.
*/
public void setTargetTags(java.util.Collection targetTags) {
if (targetTags == null) {
this.targetTags = null;
return;
}
this.targetTags = new java.util.ArrayList(targetTags);
}
/**
*
* The target tag for a policy.
*
*
* Tags are strings in the format key=value
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTargetTags(java.util.Collection)} or {@link #withTargetTags(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param targetTags
* The target tag for a policy.
*
* Tags are strings in the format key=value
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetLifecyclePoliciesRequest withTargetTags(String... targetTags) {
if (this.targetTags == null) {
setTargetTags(new java.util.ArrayList(targetTags.length));
}
for (String ele : targetTags) {
this.targetTags.add(ele);
}
return this;
}
/**
*
* The target tag for a policy.
*
*
* Tags are strings in the format key=value
.
*
*
* @param targetTags
* The target tag for a policy.
*
* Tags are strings in the format key=value
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetLifecyclePoliciesRequest withTargetTags(java.util.Collection targetTags) {
setTargetTags(targetTags);
return this;
}
/**
*
* The tags to add to objects created by the policy.
*
*
* Tags are strings in the format key=value
.
*
*
* These user-defined tags are added in addition to the Amazon Web Services-added lifecycle tags.
*
*
* @return The tags to add to objects created by the policy.
*
* Tags are strings in the format key=value
.
*
*
* These user-defined tags are added in addition to the Amazon Web Services-added lifecycle tags.
*/
public java.util.List getTagsToAdd() {
return tagsToAdd;
}
/**
*
* The tags to add to objects created by the policy.
*
*
* Tags are strings in the format key=value
.
*
*
* These user-defined tags are added in addition to the Amazon Web Services-added lifecycle tags.
*
*
* @param tagsToAdd
* The tags to add to objects created by the policy.
*
* Tags are strings in the format key=value
.
*
*
* These user-defined tags are added in addition to the Amazon Web Services-added lifecycle tags.
*/
public void setTagsToAdd(java.util.Collection tagsToAdd) {
if (tagsToAdd == null) {
this.tagsToAdd = null;
return;
}
this.tagsToAdd = new java.util.ArrayList(tagsToAdd);
}
/**
*
* The tags to add to objects created by the policy.
*
*
* Tags are strings in the format key=value
.
*
*
* These user-defined tags are added in addition to the Amazon Web Services-added lifecycle tags.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTagsToAdd(java.util.Collection)} or {@link #withTagsToAdd(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param tagsToAdd
* The tags to add to objects created by the policy.
*
* Tags are strings in the format key=value
.
*
*
* These user-defined tags are added in addition to the Amazon Web Services-added lifecycle tags.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetLifecyclePoliciesRequest withTagsToAdd(String... tagsToAdd) {
if (this.tagsToAdd == null) {
setTagsToAdd(new java.util.ArrayList(tagsToAdd.length));
}
for (String ele : tagsToAdd) {
this.tagsToAdd.add(ele);
}
return this;
}
/**
*
* The tags to add to objects created by the policy.
*
*
* Tags are strings in the format key=value
.
*
*
* These user-defined tags are added in addition to the Amazon Web Services-added lifecycle tags.
*
*
* @param tagsToAdd
* The tags to add to objects created by the policy.
*
* Tags are strings in the format key=value
.
*
*
* These user-defined tags are added in addition to the Amazon Web Services-added lifecycle tags.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetLifecyclePoliciesRequest withTagsToAdd(java.util.Collection tagsToAdd) {
setTagsToAdd(tagsToAdd);
return this;
}
/**
*
* [Default policies only] Specifies the type of default policy to get. Specify one of the following:
*
*
* -
*
* VOLUME
- To get only the default policy for EBS snapshots
*
*
* -
*
* INSTANCE
- To get only the default policy for EBS-backed AMIs
*
*
* -
*
* ALL
- To get all default policies
*
*
*
*
* @param defaultPolicyType
* [Default policies only] Specifies the type of default policy to get. Specify one of the
* following:
*
* -
*
* VOLUME
- To get only the default policy for EBS snapshots
*
*
* -
*
* INSTANCE
- To get only the default policy for EBS-backed AMIs
*
*
* -
*
* ALL
- To get all default policies
*
*
* @see DefaultPoliciesTypeValues
*/
public void setDefaultPolicyType(String defaultPolicyType) {
this.defaultPolicyType = defaultPolicyType;
}
/**
*
* [Default policies only] Specifies the type of default policy to get. Specify one of the following:
*
*
* -
*
* VOLUME
- To get only the default policy for EBS snapshots
*
*
* -
*
* INSTANCE
- To get only the default policy for EBS-backed AMIs
*
*
* -
*
* ALL
- To get all default policies
*
*
*
*
* @return [Default policies only] Specifies the type of default policy to get. Specify one of the
* following:
*
* -
*
* VOLUME
- To get only the default policy for EBS snapshots
*
*
* -
*
* INSTANCE
- To get only the default policy for EBS-backed AMIs
*
*
* -
*
* ALL
- To get all default policies
*
*
* @see DefaultPoliciesTypeValues
*/
public String getDefaultPolicyType() {
return this.defaultPolicyType;
}
/**
*
* [Default policies only] Specifies the type of default policy to get. Specify one of the following:
*
*
* -
*
* VOLUME
- To get only the default policy for EBS snapshots
*
*
* -
*
* INSTANCE
- To get only the default policy for EBS-backed AMIs
*
*
* -
*
* ALL
- To get all default policies
*
*
*
*
* @param defaultPolicyType
* [Default policies only] Specifies the type of default policy to get. Specify one of the
* following:
*
* -
*
* VOLUME
- To get only the default policy for EBS snapshots
*
*
* -
*
* INSTANCE
- To get only the default policy for EBS-backed AMIs
*
*
* -
*
* ALL
- To get all default policies
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see DefaultPoliciesTypeValues
*/
public GetLifecyclePoliciesRequest withDefaultPolicyType(String defaultPolicyType) {
setDefaultPolicyType(defaultPolicyType);
return this;
}
/**
*
* [Default policies only] Specifies the type of default policy to get. Specify one of the following:
*
*
* -
*
* VOLUME
- To get only the default policy for EBS snapshots
*
*
* -
*
* INSTANCE
- To get only the default policy for EBS-backed AMIs
*
*
* -
*
* ALL
- To get all default policies
*
*
*
*
* @param defaultPolicyType
* [Default policies only] Specifies the type of default policy to get. Specify one of the
* following:
*
* -
*
* VOLUME
- To get only the default policy for EBS snapshots
*
*
* -
*
* INSTANCE
- To get only the default policy for EBS-backed AMIs
*
*
* -
*
* ALL
- To get all default policies
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see DefaultPoliciesTypeValues
*/
public GetLifecyclePoliciesRequest withDefaultPolicyType(DefaultPoliciesTypeValues defaultPolicyType) {
this.defaultPolicyType = defaultPolicyType.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getPolicyIds() != null)
sb.append("PolicyIds: ").append(getPolicyIds()).append(",");
if (getState() != null)
sb.append("State: ").append(getState()).append(",");
if (getResourceTypes() != null)
sb.append("ResourceTypes: ").append(getResourceTypes()).append(",");
if (getTargetTags() != null)
sb.append("TargetTags: ").append(getTargetTags()).append(",");
if (getTagsToAdd() != null)
sb.append("TagsToAdd: ").append(getTagsToAdd()).append(",");
if (getDefaultPolicyType() != null)
sb.append("DefaultPolicyType: ").append(getDefaultPolicyType());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetLifecyclePoliciesRequest == false)
return false;
GetLifecyclePoliciesRequest other = (GetLifecyclePoliciesRequest) obj;
if (other.getPolicyIds() == null ^ this.getPolicyIds() == null)
return false;
if (other.getPolicyIds() != null && other.getPolicyIds().equals(this.getPolicyIds()) == false)
return false;
if (other.getState() == null ^ this.getState() == null)
return false;
if (other.getState() != null && other.getState().equals(this.getState()) == false)
return false;
if (other.getResourceTypes() == null ^ this.getResourceTypes() == null)
return false;
if (other.getResourceTypes() != null && other.getResourceTypes().equals(this.getResourceTypes()) == false)
return false;
if (other.getTargetTags() == null ^ this.getTargetTags() == null)
return false;
if (other.getTargetTags() != null && other.getTargetTags().equals(this.getTargetTags()) == false)
return false;
if (other.getTagsToAdd() == null ^ this.getTagsToAdd() == null)
return false;
if (other.getTagsToAdd() != null && other.getTagsToAdd().equals(this.getTagsToAdd()) == false)
return false;
if (other.getDefaultPolicyType() == null ^ this.getDefaultPolicyType() == null)
return false;
if (other.getDefaultPolicyType() != null && other.getDefaultPolicyType().equals(this.getDefaultPolicyType()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getPolicyIds() == null) ? 0 : getPolicyIds().hashCode());
hashCode = prime * hashCode + ((getState() == null) ? 0 : getState().hashCode());
hashCode = prime * hashCode + ((getResourceTypes() == null) ? 0 : getResourceTypes().hashCode());
hashCode = prime * hashCode + ((getTargetTags() == null) ? 0 : getTargetTags().hashCode());
hashCode = prime * hashCode + ((getTagsToAdd() == null) ? 0 : getTagsToAdd().hashCode());
hashCode = prime * hashCode + ((getDefaultPolicyType() == null) ? 0 : getDefaultPolicyType().hashCode());
return hashCode;
}
@Override
public GetLifecyclePoliciesRequest clone() {
return (GetLifecyclePoliciesRequest) super.clone();
}
}