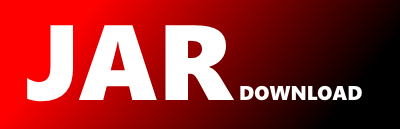
com.amazonaws.services.dlm.model.CreateLifecyclePolicyRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-dlm Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.dlm.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateLifecyclePolicyRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The Amazon Resource Name (ARN) of the IAM role used to run the operations specified by the lifecycle policy.
*
*/
private String executionRoleArn;
/**
*
* A description of the lifecycle policy. The characters ^[0-9A-Za-z _-]+$ are supported.
*
*/
private String description;
/**
*
* The activation state of the lifecycle policy after creation.
*
*/
private String state;
/**
*
* The configuration details of the lifecycle policy.
*
*
*
* If you create a default policy, you can specify the request parameters either in the request body, or in the
* PolicyDetails request structure, but not both.
*
*
*/
private PolicyDetails policyDetails;
/**
*
* The tags to apply to the lifecycle policy during creation.
*
*/
private java.util.Map tags;
/**
*
* [Default policies only] Specify the type of default policy to create.
*
*
* -
*
* To create a default policy for EBS snapshots, that creates snapshots of all volumes in the Region that do not
* have recent backups, specify VOLUME
.
*
*
* -
*
* To create a default policy for EBS-backed AMIs, that creates EBS-backed AMIs from all instances in the Region
* that do not have recent backups, specify INSTANCE
.
*
*
*
*/
private String defaultPolicy;
/**
*
* [Default policies only] Specifies how often the policy should run and create snapshots or AMIs. The
* creation frequency can range from 1 to 7 days. If you do not specify a value, the default is 1.
*
*
* Default: 1
*
*/
private Integer createInterval;
/**
*
* [Default policies only] Specifies how long the policy should retain snapshots or AMIs before deleting
* them. The retention period can range from 2 to 14 days, but it must be greater than the creation frequency to
* ensure that the policy retains at least 1 snapshot or AMI at any given time. If you do not specify a value, the
* default is 7.
*
*
* Default: 7
*
*/
private Integer retainInterval;
/**
*
* [Default policies only] Indicates whether the policy should copy tags from the source resource to the
* snapshot or AMI. If you do not specify a value, the default is false
.
*
*
* Default: false
*
*/
private Boolean copyTags;
/**
*
* [Default policies only] Defines the snapshot or AMI retention behavior for the policy if the source volume
* or instance is deleted, or if the policy enters the error, disabled, or deleted state.
*
*
* By default (ExtendDeletion=false):
*
*
* -
*
* If a source resource is deleted, Amazon Data Lifecycle Manager will continue to delete previously created
* snapshots or AMIs, up to but not including the last one, based on the specified retention period. If you want
* Amazon Data Lifecycle Manager to delete all snapshots or AMIs, including the last one, specify true
.
*
*
* -
*
* If a policy enters the error, disabled, or deleted state, Amazon Data Lifecycle Manager stops deleting snapshots
* and AMIs. If you want Amazon Data Lifecycle Manager to continue deleting snapshots or AMIs, including the last
* one, if the policy enters one of these states, specify true
.
*
*
*
*
* If you enable extended deletion (ExtendDeletion=true), you override both default behaviors simultaneously.
*
*
* If you do not specify a value, the default is false
.
*
*
* Default: false
*
*/
private Boolean extendDeletion;
/**
*
* [Default policies only] Specifies destination Regions for snapshot or AMI copies. You can specify up to 3
* destination Regions. If you do not want to create cross-Region copies, omit this parameter.
*
*/
private java.util.List crossRegionCopyTargets;
/**
*
* [Default policies only] Specifies exclusion parameters for volumes or instances for which you do not want
* to create snapshots or AMIs. The policy will not create snapshots or AMIs for target resources that match any of
* the specified exclusion parameters.
*
*/
private Exclusions exclusions;
/**
*
* The Amazon Resource Name (ARN) of the IAM role used to run the operations specified by the lifecycle policy.
*
*
* @param executionRoleArn
* The Amazon Resource Name (ARN) of the IAM role used to run the operations specified by the lifecycle
* policy.
*/
public void setExecutionRoleArn(String executionRoleArn) {
this.executionRoleArn = executionRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role used to run the operations specified by the lifecycle policy.
*
*
* @return The Amazon Resource Name (ARN) of the IAM role used to run the operations specified by the lifecycle
* policy.
*/
public String getExecutionRoleArn() {
return this.executionRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role used to run the operations specified by the lifecycle policy.
*
*
* @param executionRoleArn
* The Amazon Resource Name (ARN) of the IAM role used to run the operations specified by the lifecycle
* policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateLifecyclePolicyRequest withExecutionRoleArn(String executionRoleArn) {
setExecutionRoleArn(executionRoleArn);
return this;
}
/**
*
* A description of the lifecycle policy. The characters ^[0-9A-Za-z _-]+$ are supported.
*
*
* @param description
* A description of the lifecycle policy. The characters ^[0-9A-Za-z _-]+$ are supported.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A description of the lifecycle policy. The characters ^[0-9A-Za-z _-]+$ are supported.
*
*
* @return A description of the lifecycle policy. The characters ^[0-9A-Za-z _-]+$ are supported.
*/
public String getDescription() {
return this.description;
}
/**
*
* A description of the lifecycle policy. The characters ^[0-9A-Za-z _-]+$ are supported.
*
*
* @param description
* A description of the lifecycle policy. The characters ^[0-9A-Za-z _-]+$ are supported.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateLifecyclePolicyRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The activation state of the lifecycle policy after creation.
*
*
* @param state
* The activation state of the lifecycle policy after creation.
* @see SettablePolicyStateValues
*/
public void setState(String state) {
this.state = state;
}
/**
*
* The activation state of the lifecycle policy after creation.
*
*
* @return The activation state of the lifecycle policy after creation.
* @see SettablePolicyStateValues
*/
public String getState() {
return this.state;
}
/**
*
* The activation state of the lifecycle policy after creation.
*
*
* @param state
* The activation state of the lifecycle policy after creation.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SettablePolicyStateValues
*/
public CreateLifecyclePolicyRequest withState(String state) {
setState(state);
return this;
}
/**
*
* The activation state of the lifecycle policy after creation.
*
*
* @param state
* The activation state of the lifecycle policy after creation.
* @return Returns a reference to this object so that method calls can be chained together.
* @see SettablePolicyStateValues
*/
public CreateLifecyclePolicyRequest withState(SettablePolicyStateValues state) {
this.state = state.toString();
return this;
}
/**
*
* The configuration details of the lifecycle policy.
*
*
*
* If you create a default policy, you can specify the request parameters either in the request body, or in the
* PolicyDetails request structure, but not both.
*
*
*
* @param policyDetails
* The configuration details of the lifecycle policy.
*
* If you create a default policy, you can specify the request parameters either in the request body, or in
* the PolicyDetails request structure, but not both.
*
*/
public void setPolicyDetails(PolicyDetails policyDetails) {
this.policyDetails = policyDetails;
}
/**
*
* The configuration details of the lifecycle policy.
*
*
*
* If you create a default policy, you can specify the request parameters either in the request body, or in the
* PolicyDetails request structure, but not both.
*
*
*
* @return The configuration details of the lifecycle policy.
*
* If you create a default policy, you can specify the request parameters either in the request body, or in
* the PolicyDetails request structure, but not both.
*
*/
public PolicyDetails getPolicyDetails() {
return this.policyDetails;
}
/**
*
* The configuration details of the lifecycle policy.
*
*
*
* If you create a default policy, you can specify the request parameters either in the request body, or in the
* PolicyDetails request structure, but not both.
*
*
*
* @param policyDetails
* The configuration details of the lifecycle policy.
*
* If you create a default policy, you can specify the request parameters either in the request body, or in
* the PolicyDetails request structure, but not both.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateLifecyclePolicyRequest withPolicyDetails(PolicyDetails policyDetails) {
setPolicyDetails(policyDetails);
return this;
}
/**
*
* The tags to apply to the lifecycle policy during creation.
*
*
* @return The tags to apply to the lifecycle policy during creation.
*/
public java.util.Map getTags() {
return tags;
}
/**
*
* The tags to apply to the lifecycle policy during creation.
*
*
* @param tags
* The tags to apply to the lifecycle policy during creation.
*/
public void setTags(java.util.Map tags) {
this.tags = tags;
}
/**
*
* The tags to apply to the lifecycle policy during creation.
*
*
* @param tags
* The tags to apply to the lifecycle policy during creation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateLifecyclePolicyRequest withTags(java.util.Map tags) {
setTags(tags);
return this;
}
/**
* Add a single Tags entry
*
* @see CreateLifecyclePolicyRequest#withTags
* @returns a reference to this object so that method calls can be chained together.
*/
public CreateLifecyclePolicyRequest addTagsEntry(String key, String value) {
if (null == this.tags) {
this.tags = new java.util.HashMap();
}
if (this.tags.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.tags.put(key, value);
return this;
}
/**
* Removes all the entries added into Tags.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateLifecyclePolicyRequest clearTagsEntries() {
this.tags = null;
return this;
}
/**
*
* [Default policies only] Specify the type of default policy to create.
*
*
* -
*
* To create a default policy for EBS snapshots, that creates snapshots of all volumes in the Region that do not
* have recent backups, specify VOLUME
.
*
*
* -
*
* To create a default policy for EBS-backed AMIs, that creates EBS-backed AMIs from all instances in the Region
* that do not have recent backups, specify INSTANCE
.
*
*
*
*
* @param defaultPolicy
* [Default policies only] Specify the type of default policy to create.
*
* -
*
* To create a default policy for EBS snapshots, that creates snapshots of all volumes in the Region that do
* not have recent backups, specify VOLUME
.
*
*
* -
*
* To create a default policy for EBS-backed AMIs, that creates EBS-backed AMIs from all instances in the
* Region that do not have recent backups, specify INSTANCE
.
*
*
* @see DefaultPolicyTypeValues
*/
public void setDefaultPolicy(String defaultPolicy) {
this.defaultPolicy = defaultPolicy;
}
/**
*
* [Default policies only] Specify the type of default policy to create.
*
*
* -
*
* To create a default policy for EBS snapshots, that creates snapshots of all volumes in the Region that do not
* have recent backups, specify VOLUME
.
*
*
* -
*
* To create a default policy for EBS-backed AMIs, that creates EBS-backed AMIs from all instances in the Region
* that do not have recent backups, specify INSTANCE
.
*
*
*
*
* @return [Default policies only] Specify the type of default policy to create.
*
* -
*
* To create a default policy for EBS snapshots, that creates snapshots of all volumes in the Region that do
* not have recent backups, specify VOLUME
.
*
*
* -
*
* To create a default policy for EBS-backed AMIs, that creates EBS-backed AMIs from all instances in the
* Region that do not have recent backups, specify INSTANCE
.
*
*
* @see DefaultPolicyTypeValues
*/
public String getDefaultPolicy() {
return this.defaultPolicy;
}
/**
*
* [Default policies only] Specify the type of default policy to create.
*
*
* -
*
* To create a default policy for EBS snapshots, that creates snapshots of all volumes in the Region that do not
* have recent backups, specify VOLUME
.
*
*
* -
*
* To create a default policy for EBS-backed AMIs, that creates EBS-backed AMIs from all instances in the Region
* that do not have recent backups, specify INSTANCE
.
*
*
*
*
* @param defaultPolicy
* [Default policies only] Specify the type of default policy to create.
*
* -
*
* To create a default policy for EBS snapshots, that creates snapshots of all volumes in the Region that do
* not have recent backups, specify VOLUME
.
*
*
* -
*
* To create a default policy for EBS-backed AMIs, that creates EBS-backed AMIs from all instances in the
* Region that do not have recent backups, specify INSTANCE
.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see DefaultPolicyTypeValues
*/
public CreateLifecyclePolicyRequest withDefaultPolicy(String defaultPolicy) {
setDefaultPolicy(defaultPolicy);
return this;
}
/**
*
* [Default policies only] Specify the type of default policy to create.
*
*
* -
*
* To create a default policy for EBS snapshots, that creates snapshots of all volumes in the Region that do not
* have recent backups, specify VOLUME
.
*
*
* -
*
* To create a default policy for EBS-backed AMIs, that creates EBS-backed AMIs from all instances in the Region
* that do not have recent backups, specify INSTANCE
.
*
*
*
*
* @param defaultPolicy
* [Default policies only] Specify the type of default policy to create.
*
* -
*
* To create a default policy for EBS snapshots, that creates snapshots of all volumes in the Region that do
* not have recent backups, specify VOLUME
.
*
*
* -
*
* To create a default policy for EBS-backed AMIs, that creates EBS-backed AMIs from all instances in the
* Region that do not have recent backups, specify INSTANCE
.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see DefaultPolicyTypeValues
*/
public CreateLifecyclePolicyRequest withDefaultPolicy(DefaultPolicyTypeValues defaultPolicy) {
this.defaultPolicy = defaultPolicy.toString();
return this;
}
/**
*
* [Default policies only] Specifies how often the policy should run and create snapshots or AMIs. The
* creation frequency can range from 1 to 7 days. If you do not specify a value, the default is 1.
*
*
* Default: 1
*
*
* @param createInterval
* [Default policies only] Specifies how often the policy should run and create snapshots or AMIs. The
* creation frequency can range from 1 to 7 days. If you do not specify a value, the default is 1.
*
* Default: 1
*/
public void setCreateInterval(Integer createInterval) {
this.createInterval = createInterval;
}
/**
*
* [Default policies only] Specifies how often the policy should run and create snapshots or AMIs. The
* creation frequency can range from 1 to 7 days. If you do not specify a value, the default is 1.
*
*
* Default: 1
*
*
* @return [Default policies only] Specifies how often the policy should run and create snapshots or AMIs.
* The creation frequency can range from 1 to 7 days. If you do not specify a value, the default is 1.
*
* Default: 1
*/
public Integer getCreateInterval() {
return this.createInterval;
}
/**
*
* [Default policies only] Specifies how often the policy should run and create snapshots or AMIs. The
* creation frequency can range from 1 to 7 days. If you do not specify a value, the default is 1.
*
*
* Default: 1
*
*
* @param createInterval
* [Default policies only] Specifies how often the policy should run and create snapshots or AMIs. The
* creation frequency can range from 1 to 7 days. If you do not specify a value, the default is 1.
*
* Default: 1
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateLifecyclePolicyRequest withCreateInterval(Integer createInterval) {
setCreateInterval(createInterval);
return this;
}
/**
*
* [Default policies only] Specifies how long the policy should retain snapshots or AMIs before deleting
* them. The retention period can range from 2 to 14 days, but it must be greater than the creation frequency to
* ensure that the policy retains at least 1 snapshot or AMI at any given time. If you do not specify a value, the
* default is 7.
*
*
* Default: 7
*
*
* @param retainInterval
* [Default policies only] Specifies how long the policy should retain snapshots or AMIs before
* deleting them. The retention period can range from 2 to 14 days, but it must be greater than the creation
* frequency to ensure that the policy retains at least 1 snapshot or AMI at any given time. If you do not
* specify a value, the default is 7.
*
* Default: 7
*/
public void setRetainInterval(Integer retainInterval) {
this.retainInterval = retainInterval;
}
/**
*
* [Default policies only] Specifies how long the policy should retain snapshots or AMIs before deleting
* them. The retention period can range from 2 to 14 days, but it must be greater than the creation frequency to
* ensure that the policy retains at least 1 snapshot or AMI at any given time. If you do not specify a value, the
* default is 7.
*
*
* Default: 7
*
*
* @return [Default policies only] Specifies how long the policy should retain snapshots or AMIs before
* deleting them. The retention period can range from 2 to 14 days, but it must be greater than the creation
* frequency to ensure that the policy retains at least 1 snapshot or AMI at any given time. If you do not
* specify a value, the default is 7.
*
* Default: 7
*/
public Integer getRetainInterval() {
return this.retainInterval;
}
/**
*
* [Default policies only] Specifies how long the policy should retain snapshots or AMIs before deleting
* them. The retention period can range from 2 to 14 days, but it must be greater than the creation frequency to
* ensure that the policy retains at least 1 snapshot or AMI at any given time. If you do not specify a value, the
* default is 7.
*
*
* Default: 7
*
*
* @param retainInterval
* [Default policies only] Specifies how long the policy should retain snapshots or AMIs before
* deleting them. The retention period can range from 2 to 14 days, but it must be greater than the creation
* frequency to ensure that the policy retains at least 1 snapshot or AMI at any given time. If you do not
* specify a value, the default is 7.
*
* Default: 7
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateLifecyclePolicyRequest withRetainInterval(Integer retainInterval) {
setRetainInterval(retainInterval);
return this;
}
/**
*
* [Default policies only] Indicates whether the policy should copy tags from the source resource to the
* snapshot or AMI. If you do not specify a value, the default is false
.
*
*
* Default: false
*
*
* @param copyTags
* [Default policies only] Indicates whether the policy should copy tags from the source resource to
* the snapshot or AMI. If you do not specify a value, the default is false
.
*
* Default: false
*/
public void setCopyTags(Boolean copyTags) {
this.copyTags = copyTags;
}
/**
*
* [Default policies only] Indicates whether the policy should copy tags from the source resource to the
* snapshot or AMI. If you do not specify a value, the default is false
.
*
*
* Default: false
*
*
* @return [Default policies only] Indicates whether the policy should copy tags from the source resource to
* the snapshot or AMI. If you do not specify a value, the default is false
.
*
* Default: false
*/
public Boolean getCopyTags() {
return this.copyTags;
}
/**
*
* [Default policies only] Indicates whether the policy should copy tags from the source resource to the
* snapshot or AMI. If you do not specify a value, the default is false
.
*
*
* Default: false
*
*
* @param copyTags
* [Default policies only] Indicates whether the policy should copy tags from the source resource to
* the snapshot or AMI. If you do not specify a value, the default is false
.
*
* Default: false
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateLifecyclePolicyRequest withCopyTags(Boolean copyTags) {
setCopyTags(copyTags);
return this;
}
/**
*
* [Default policies only] Indicates whether the policy should copy tags from the source resource to the
* snapshot or AMI. If you do not specify a value, the default is false
.
*
*
* Default: false
*
*
* @return [Default policies only] Indicates whether the policy should copy tags from the source resource to
* the snapshot or AMI. If you do not specify a value, the default is false
.
*
* Default: false
*/
public Boolean isCopyTags() {
return this.copyTags;
}
/**
*
* [Default policies only] Defines the snapshot or AMI retention behavior for the policy if the source volume
* or instance is deleted, or if the policy enters the error, disabled, or deleted state.
*
*
* By default (ExtendDeletion=false):
*
*
* -
*
* If a source resource is deleted, Amazon Data Lifecycle Manager will continue to delete previously created
* snapshots or AMIs, up to but not including the last one, based on the specified retention period. If you want
* Amazon Data Lifecycle Manager to delete all snapshots or AMIs, including the last one, specify true
.
*
*
* -
*
* If a policy enters the error, disabled, or deleted state, Amazon Data Lifecycle Manager stops deleting snapshots
* and AMIs. If you want Amazon Data Lifecycle Manager to continue deleting snapshots or AMIs, including the last
* one, if the policy enters one of these states, specify true
.
*
*
*
*
* If you enable extended deletion (ExtendDeletion=true), you override both default behaviors simultaneously.
*
*
* If you do not specify a value, the default is false
.
*
*
* Default: false
*
*
* @param extendDeletion
* [Default policies only] Defines the snapshot or AMI retention behavior for the policy if the source
* volume or instance is deleted, or if the policy enters the error, disabled, or deleted state.
*
* By default (ExtendDeletion=false):
*
*
* -
*
* If a source resource is deleted, Amazon Data Lifecycle Manager will continue to delete previously created
* snapshots or AMIs, up to but not including the last one, based on the specified retention period. If you
* want Amazon Data Lifecycle Manager to delete all snapshots or AMIs, including the last one, specify
* true
.
*
*
* -
*
* If a policy enters the error, disabled, or deleted state, Amazon Data Lifecycle Manager stops deleting
* snapshots and AMIs. If you want Amazon Data Lifecycle Manager to continue deleting snapshots or AMIs,
* including the last one, if the policy enters one of these states, specify true
.
*
*
*
*
* If you enable extended deletion (ExtendDeletion=true), you override both default behaviors
* simultaneously.
*
*
* If you do not specify a value, the default is false
.
*
*
* Default: false
*/
public void setExtendDeletion(Boolean extendDeletion) {
this.extendDeletion = extendDeletion;
}
/**
*
* [Default policies only] Defines the snapshot or AMI retention behavior for the policy if the source volume
* or instance is deleted, or if the policy enters the error, disabled, or deleted state.
*
*
* By default (ExtendDeletion=false):
*
*
* -
*
* If a source resource is deleted, Amazon Data Lifecycle Manager will continue to delete previously created
* snapshots or AMIs, up to but not including the last one, based on the specified retention period. If you want
* Amazon Data Lifecycle Manager to delete all snapshots or AMIs, including the last one, specify true
.
*
*
* -
*
* If a policy enters the error, disabled, or deleted state, Amazon Data Lifecycle Manager stops deleting snapshots
* and AMIs. If you want Amazon Data Lifecycle Manager to continue deleting snapshots or AMIs, including the last
* one, if the policy enters one of these states, specify true
.
*
*
*
*
* If you enable extended deletion (ExtendDeletion=true), you override both default behaviors simultaneously.
*
*
* If you do not specify a value, the default is false
.
*
*
* Default: false
*
*
* @return [Default policies only] Defines the snapshot or AMI retention behavior for the policy if the
* source volume or instance is deleted, or if the policy enters the error, disabled, or deleted state.
*
* By default (ExtendDeletion=false):
*
*
* -
*
* If a source resource is deleted, Amazon Data Lifecycle Manager will continue to delete previously created
* snapshots or AMIs, up to but not including the last one, based on the specified retention period. If you
* want Amazon Data Lifecycle Manager to delete all snapshots or AMIs, including the last one, specify
* true
.
*
*
* -
*
* If a policy enters the error, disabled, or deleted state, Amazon Data Lifecycle Manager stops deleting
* snapshots and AMIs. If you want Amazon Data Lifecycle Manager to continue deleting snapshots or AMIs,
* including the last one, if the policy enters one of these states, specify true
.
*
*
*
*
* If you enable extended deletion (ExtendDeletion=true), you override both default behaviors
* simultaneously.
*
*
* If you do not specify a value, the default is false
.
*
*
* Default: false
*/
public Boolean getExtendDeletion() {
return this.extendDeletion;
}
/**
*
* [Default policies only] Defines the snapshot or AMI retention behavior for the policy if the source volume
* or instance is deleted, or if the policy enters the error, disabled, or deleted state.
*
*
* By default (ExtendDeletion=false):
*
*
* -
*
* If a source resource is deleted, Amazon Data Lifecycle Manager will continue to delete previously created
* snapshots or AMIs, up to but not including the last one, based on the specified retention period. If you want
* Amazon Data Lifecycle Manager to delete all snapshots or AMIs, including the last one, specify true
.
*
*
* -
*
* If a policy enters the error, disabled, or deleted state, Amazon Data Lifecycle Manager stops deleting snapshots
* and AMIs. If you want Amazon Data Lifecycle Manager to continue deleting snapshots or AMIs, including the last
* one, if the policy enters one of these states, specify true
.
*
*
*
*
* If you enable extended deletion (ExtendDeletion=true), you override both default behaviors simultaneously.
*
*
* If you do not specify a value, the default is false
.
*
*
* Default: false
*
*
* @param extendDeletion
* [Default policies only] Defines the snapshot or AMI retention behavior for the policy if the source
* volume or instance is deleted, or if the policy enters the error, disabled, or deleted state.
*
* By default (ExtendDeletion=false):
*
*
* -
*
* If a source resource is deleted, Amazon Data Lifecycle Manager will continue to delete previously created
* snapshots or AMIs, up to but not including the last one, based on the specified retention period. If you
* want Amazon Data Lifecycle Manager to delete all snapshots or AMIs, including the last one, specify
* true
.
*
*
* -
*
* If a policy enters the error, disabled, or deleted state, Amazon Data Lifecycle Manager stops deleting
* snapshots and AMIs. If you want Amazon Data Lifecycle Manager to continue deleting snapshots or AMIs,
* including the last one, if the policy enters one of these states, specify true
.
*
*
*
*
* If you enable extended deletion (ExtendDeletion=true), you override both default behaviors
* simultaneously.
*
*
* If you do not specify a value, the default is false
.
*
*
* Default: false
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateLifecyclePolicyRequest withExtendDeletion(Boolean extendDeletion) {
setExtendDeletion(extendDeletion);
return this;
}
/**
*
* [Default policies only] Defines the snapshot or AMI retention behavior for the policy if the source volume
* or instance is deleted, or if the policy enters the error, disabled, or deleted state.
*
*
* By default (ExtendDeletion=false):
*
*
* -
*
* If a source resource is deleted, Amazon Data Lifecycle Manager will continue to delete previously created
* snapshots or AMIs, up to but not including the last one, based on the specified retention period. If you want
* Amazon Data Lifecycle Manager to delete all snapshots or AMIs, including the last one, specify true
.
*
*
* -
*
* If a policy enters the error, disabled, or deleted state, Amazon Data Lifecycle Manager stops deleting snapshots
* and AMIs. If you want Amazon Data Lifecycle Manager to continue deleting snapshots or AMIs, including the last
* one, if the policy enters one of these states, specify true
.
*
*
*
*
* If you enable extended deletion (ExtendDeletion=true), you override both default behaviors simultaneously.
*
*
* If you do not specify a value, the default is false
.
*
*
* Default: false
*
*
* @return [Default policies only] Defines the snapshot or AMI retention behavior for the policy if the
* source volume or instance is deleted, or if the policy enters the error, disabled, or deleted state.
*
* By default (ExtendDeletion=false):
*
*
* -
*
* If a source resource is deleted, Amazon Data Lifecycle Manager will continue to delete previously created
* snapshots or AMIs, up to but not including the last one, based on the specified retention period. If you
* want Amazon Data Lifecycle Manager to delete all snapshots or AMIs, including the last one, specify
* true
.
*
*
* -
*
* If a policy enters the error, disabled, or deleted state, Amazon Data Lifecycle Manager stops deleting
* snapshots and AMIs. If you want Amazon Data Lifecycle Manager to continue deleting snapshots or AMIs,
* including the last one, if the policy enters one of these states, specify true
.
*
*
*
*
* If you enable extended deletion (ExtendDeletion=true), you override both default behaviors
* simultaneously.
*
*
* If you do not specify a value, the default is false
.
*
*
* Default: false
*/
public Boolean isExtendDeletion() {
return this.extendDeletion;
}
/**
*
* [Default policies only] Specifies destination Regions for snapshot or AMI copies. You can specify up to 3
* destination Regions. If you do not want to create cross-Region copies, omit this parameter.
*
*
* @return [Default policies only] Specifies destination Regions for snapshot or AMI copies. You can specify
* up to 3 destination Regions. If you do not want to create cross-Region copies, omit this parameter.
*/
public java.util.List getCrossRegionCopyTargets() {
return crossRegionCopyTargets;
}
/**
*
* [Default policies only] Specifies destination Regions for snapshot or AMI copies. You can specify up to 3
* destination Regions. If you do not want to create cross-Region copies, omit this parameter.
*
*
* @param crossRegionCopyTargets
* [Default policies only] Specifies destination Regions for snapshot or AMI copies. You can specify
* up to 3 destination Regions. If you do not want to create cross-Region copies, omit this parameter.
*/
public void setCrossRegionCopyTargets(java.util.Collection crossRegionCopyTargets) {
if (crossRegionCopyTargets == null) {
this.crossRegionCopyTargets = null;
return;
}
this.crossRegionCopyTargets = new java.util.ArrayList(crossRegionCopyTargets);
}
/**
*
* [Default policies only] Specifies destination Regions for snapshot or AMI copies. You can specify up to 3
* destination Regions. If you do not want to create cross-Region copies, omit this parameter.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCrossRegionCopyTargets(java.util.Collection)} or
* {@link #withCrossRegionCopyTargets(java.util.Collection)} if you want to override the existing values.
*
*
* @param crossRegionCopyTargets
* [Default policies only] Specifies destination Regions for snapshot or AMI copies. You can specify
* up to 3 destination Regions. If you do not want to create cross-Region copies, omit this parameter.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateLifecyclePolicyRequest withCrossRegionCopyTargets(CrossRegionCopyTarget... crossRegionCopyTargets) {
if (this.crossRegionCopyTargets == null) {
setCrossRegionCopyTargets(new java.util.ArrayList(crossRegionCopyTargets.length));
}
for (CrossRegionCopyTarget ele : crossRegionCopyTargets) {
this.crossRegionCopyTargets.add(ele);
}
return this;
}
/**
*
* [Default policies only] Specifies destination Regions for snapshot or AMI copies. You can specify up to 3
* destination Regions. If you do not want to create cross-Region copies, omit this parameter.
*
*
* @param crossRegionCopyTargets
* [Default policies only] Specifies destination Regions for snapshot or AMI copies. You can specify
* up to 3 destination Regions. If you do not want to create cross-Region copies, omit this parameter.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateLifecyclePolicyRequest withCrossRegionCopyTargets(java.util.Collection crossRegionCopyTargets) {
setCrossRegionCopyTargets(crossRegionCopyTargets);
return this;
}
/**
*
* [Default policies only] Specifies exclusion parameters for volumes or instances for which you do not want
* to create snapshots or AMIs. The policy will not create snapshots or AMIs for target resources that match any of
* the specified exclusion parameters.
*
*
* @param exclusions
* [Default policies only] Specifies exclusion parameters for volumes or instances for which you do
* not want to create snapshots or AMIs. The policy will not create snapshots or AMIs for target resources
* that match any of the specified exclusion parameters.
*/
public void setExclusions(Exclusions exclusions) {
this.exclusions = exclusions;
}
/**
*
* [Default policies only] Specifies exclusion parameters for volumes or instances for which you do not want
* to create snapshots or AMIs. The policy will not create snapshots or AMIs for target resources that match any of
* the specified exclusion parameters.
*
*
* @return [Default policies only] Specifies exclusion parameters for volumes or instances for which you do
* not want to create snapshots or AMIs. The policy will not create snapshots or AMIs for target resources
* that match any of the specified exclusion parameters.
*/
public Exclusions getExclusions() {
return this.exclusions;
}
/**
*
* [Default policies only] Specifies exclusion parameters for volumes or instances for which you do not want
* to create snapshots or AMIs. The policy will not create snapshots or AMIs for target resources that match any of
* the specified exclusion parameters.
*
*
* @param exclusions
* [Default policies only] Specifies exclusion parameters for volumes or instances for which you do
* not want to create snapshots or AMIs. The policy will not create snapshots or AMIs for target resources
* that match any of the specified exclusion parameters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateLifecyclePolicyRequest withExclusions(Exclusions exclusions) {
setExclusions(exclusions);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getExecutionRoleArn() != null)
sb.append("ExecutionRoleArn: ").append(getExecutionRoleArn()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getState() != null)
sb.append("State: ").append(getState()).append(",");
if (getPolicyDetails() != null)
sb.append("PolicyDetails: ").append(getPolicyDetails()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getDefaultPolicy() != null)
sb.append("DefaultPolicy: ").append(getDefaultPolicy()).append(",");
if (getCreateInterval() != null)
sb.append("CreateInterval: ").append(getCreateInterval()).append(",");
if (getRetainInterval() != null)
sb.append("RetainInterval: ").append(getRetainInterval()).append(",");
if (getCopyTags() != null)
sb.append("CopyTags: ").append(getCopyTags()).append(",");
if (getExtendDeletion() != null)
sb.append("ExtendDeletion: ").append(getExtendDeletion()).append(",");
if (getCrossRegionCopyTargets() != null)
sb.append("CrossRegionCopyTargets: ").append(getCrossRegionCopyTargets()).append(",");
if (getExclusions() != null)
sb.append("Exclusions: ").append(getExclusions());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateLifecyclePolicyRequest == false)
return false;
CreateLifecyclePolicyRequest other = (CreateLifecyclePolicyRequest) obj;
if (other.getExecutionRoleArn() == null ^ this.getExecutionRoleArn() == null)
return false;
if (other.getExecutionRoleArn() != null && other.getExecutionRoleArn().equals(this.getExecutionRoleArn()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getState() == null ^ this.getState() == null)
return false;
if (other.getState() != null && other.getState().equals(this.getState()) == false)
return false;
if (other.getPolicyDetails() == null ^ this.getPolicyDetails() == null)
return false;
if (other.getPolicyDetails() != null && other.getPolicyDetails().equals(this.getPolicyDetails()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getDefaultPolicy() == null ^ this.getDefaultPolicy() == null)
return false;
if (other.getDefaultPolicy() != null && other.getDefaultPolicy().equals(this.getDefaultPolicy()) == false)
return false;
if (other.getCreateInterval() == null ^ this.getCreateInterval() == null)
return false;
if (other.getCreateInterval() != null && other.getCreateInterval().equals(this.getCreateInterval()) == false)
return false;
if (other.getRetainInterval() == null ^ this.getRetainInterval() == null)
return false;
if (other.getRetainInterval() != null && other.getRetainInterval().equals(this.getRetainInterval()) == false)
return false;
if (other.getCopyTags() == null ^ this.getCopyTags() == null)
return false;
if (other.getCopyTags() != null && other.getCopyTags().equals(this.getCopyTags()) == false)
return false;
if (other.getExtendDeletion() == null ^ this.getExtendDeletion() == null)
return false;
if (other.getExtendDeletion() != null && other.getExtendDeletion().equals(this.getExtendDeletion()) == false)
return false;
if (other.getCrossRegionCopyTargets() == null ^ this.getCrossRegionCopyTargets() == null)
return false;
if (other.getCrossRegionCopyTargets() != null && other.getCrossRegionCopyTargets().equals(this.getCrossRegionCopyTargets()) == false)
return false;
if (other.getExclusions() == null ^ this.getExclusions() == null)
return false;
if (other.getExclusions() != null && other.getExclusions().equals(this.getExclusions()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getExecutionRoleArn() == null) ? 0 : getExecutionRoleArn().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getState() == null) ? 0 : getState().hashCode());
hashCode = prime * hashCode + ((getPolicyDetails() == null) ? 0 : getPolicyDetails().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getDefaultPolicy() == null) ? 0 : getDefaultPolicy().hashCode());
hashCode = prime * hashCode + ((getCreateInterval() == null) ? 0 : getCreateInterval().hashCode());
hashCode = prime * hashCode + ((getRetainInterval() == null) ? 0 : getRetainInterval().hashCode());
hashCode = prime * hashCode + ((getCopyTags() == null) ? 0 : getCopyTags().hashCode());
hashCode = prime * hashCode + ((getExtendDeletion() == null) ? 0 : getExtendDeletion().hashCode());
hashCode = prime * hashCode + ((getCrossRegionCopyTargets() == null) ? 0 : getCrossRegionCopyTargets().hashCode());
hashCode = prime * hashCode + ((getExclusions() == null) ? 0 : getExclusions().hashCode());
return hashCode;
}
@Override
public CreateLifecyclePolicyRequest clone() {
return (CreateLifecyclePolicyRequest) super.clone();
}
}