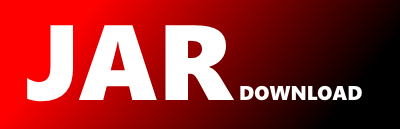
com.amazonaws.services.databasemigrationservice.AWSDatabaseMigrationServiceAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-dms Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.databasemigrationservice;
import javax.annotation.Generated;
import com.amazonaws.services.databasemigrationservice.model.*;
/**
* Interface for accessing AWS Database Migration Service asynchronously. Each asynchronous method will return a Java
* Future object representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to
* receive notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.databasemigrationservice.AbstractAWSDatabaseMigrationServiceAsync} instead.
*
*
* AWS Database Migration Service
*
* AWS Database Migration Service (AWS DMS) can migrate your data to and from the most widely used commercial and
* open-source databases such as Oracle, PostgreSQL, Microsoft SQL Server, Amazon Redshift, MariaDB, Amazon Aurora,
* MySQL, and SAP Adaptive Server Enterprise (ASE). The service supports homogeneous migrations such as Oracle to
* Oracle, as well as heterogeneous migrations between different database platforms, such as Oracle to MySQL or SQL
* Server to PostgreSQL.
*
*
* For more information about AWS DMS, see What
* Is AWS Database Migration Service? in the AWS Database Migration User Guide.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSDatabaseMigrationServiceAsync extends AWSDatabaseMigrationService {
/**
*
* Adds metadata tags to an AWS DMS resource, including replication instance, endpoint, security group, and
* migration task. These tags can also be used with cost allocation reporting to track cost associated with DMS
* resources, or used in a Condition statement in an IAM policy for DMS.
*
*
* @param addTagsToResourceRequest
* @return A Java Future containing the result of the AddTagsToResource operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.AddTagsToResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future addTagsToResourceAsync(AddTagsToResourceRequest addTagsToResourceRequest);
/**
*
* Adds metadata tags to an AWS DMS resource, including replication instance, endpoint, security group, and
* migration task. These tags can also be used with cost allocation reporting to track cost associated with DMS
* resources, or used in a Condition statement in an IAM policy for DMS.
*
*
* @param addTagsToResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddTagsToResource operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.AddTagsToResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future addTagsToResourceAsync(AddTagsToResourceRequest addTagsToResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Applies a pending maintenance action to a resource (for example, to a replication instance).
*
*
* @param applyPendingMaintenanceActionRequest
* @return A Java Future containing the result of the ApplyPendingMaintenanceAction operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.ApplyPendingMaintenanceAction
* @see AWS API Documentation
*/
java.util.concurrent.Future applyPendingMaintenanceActionAsync(
ApplyPendingMaintenanceActionRequest applyPendingMaintenanceActionRequest);
/**
*
* Applies a pending maintenance action to a resource (for example, to a replication instance).
*
*
* @param applyPendingMaintenanceActionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ApplyPendingMaintenanceAction operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.ApplyPendingMaintenanceAction
* @see AWS API Documentation
*/
java.util.concurrent.Future applyPendingMaintenanceActionAsync(
ApplyPendingMaintenanceActionRequest applyPendingMaintenanceActionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an endpoint using the provided settings.
*
*
* @param createEndpointRequest
* @return A Java Future containing the result of the CreateEndpoint operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.CreateEndpoint
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createEndpointAsync(CreateEndpointRequest createEndpointRequest);
/**
*
* Creates an endpoint using the provided settings.
*
*
* @param createEndpointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateEndpoint operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.CreateEndpoint
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createEndpointAsync(CreateEndpointRequest createEndpointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an AWS DMS event notification subscription.
*
*
* You can specify the type of source (SourceType
) you want to be notified of, provide a list of AWS
* DMS source IDs (SourceIds
) that triggers the events, and provide a list of event categories (
* EventCategories
) for events you want to be notified of. If you specify both the
* SourceType
and SourceIds
, such as SourceType = replication-instance
and
* SourceIdentifier = my-replinstance
, you will be notified of all the replication instance events for
* the specified source. If you specify a SourceType
but don't specify a SourceIdentifier
,
* you receive notice of the events for that source type for all your AWS DMS sources. If you don't specify either
* SourceType
nor SourceIdentifier
, you will be notified of events generated from all AWS
* DMS sources belonging to your customer account.
*
*
* For more information about AWS DMS events, see Working with Events and
* Notifications in the AWS Database Migration Service User Guide.
*
*
* @param createEventSubscriptionRequest
* @return A Java Future containing the result of the CreateEventSubscription operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.CreateEventSubscription
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createEventSubscriptionAsync(CreateEventSubscriptionRequest createEventSubscriptionRequest);
/**
*
* Creates an AWS DMS event notification subscription.
*
*
* You can specify the type of source (SourceType
) you want to be notified of, provide a list of AWS
* DMS source IDs (SourceIds
) that triggers the events, and provide a list of event categories (
* EventCategories
) for events you want to be notified of. If you specify both the
* SourceType
and SourceIds
, such as SourceType = replication-instance
and
* SourceIdentifier = my-replinstance
, you will be notified of all the replication instance events for
* the specified source. If you specify a SourceType
but don't specify a SourceIdentifier
,
* you receive notice of the events for that source type for all your AWS DMS sources. If you don't specify either
* SourceType
nor SourceIdentifier
, you will be notified of events generated from all AWS
* DMS sources belonging to your customer account.
*
*
* For more information about AWS DMS events, see Working with Events and
* Notifications in the AWS Database Migration Service User Guide.
*
*
* @param createEventSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateEventSubscription operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.CreateEventSubscription
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createEventSubscriptionAsync(CreateEventSubscriptionRequest createEventSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates the replication instance using the specified parameters.
*
*
* @param createReplicationInstanceRequest
* @return A Java Future containing the result of the CreateReplicationInstance operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.CreateReplicationInstance
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createReplicationInstanceAsync(
CreateReplicationInstanceRequest createReplicationInstanceRequest);
/**
*
* Creates the replication instance using the specified parameters.
*
*
* @param createReplicationInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateReplicationInstance operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.CreateReplicationInstance
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createReplicationInstanceAsync(
CreateReplicationInstanceRequest createReplicationInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a replication subnet group given a list of the subnet IDs in a VPC.
*
*
* @param createReplicationSubnetGroupRequest
* @return A Java Future containing the result of the CreateReplicationSubnetGroup operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.CreateReplicationSubnetGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future createReplicationSubnetGroupAsync(
CreateReplicationSubnetGroupRequest createReplicationSubnetGroupRequest);
/**
*
* Creates a replication subnet group given a list of the subnet IDs in a VPC.
*
*
* @param createReplicationSubnetGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateReplicationSubnetGroup operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.CreateReplicationSubnetGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future createReplicationSubnetGroupAsync(
CreateReplicationSubnetGroupRequest createReplicationSubnetGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a replication task using the specified parameters.
*
*
* @param createReplicationTaskRequest
* @return A Java Future containing the result of the CreateReplicationTask operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.CreateReplicationTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createReplicationTaskAsync(CreateReplicationTaskRequest createReplicationTaskRequest);
/**
*
* Creates a replication task using the specified parameters.
*
*
* @param createReplicationTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateReplicationTask operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.CreateReplicationTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createReplicationTaskAsync(CreateReplicationTaskRequest createReplicationTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified certificate.
*
*
* @param deleteCertificateRequest
* @return A Java Future containing the result of the DeleteCertificate operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DeleteCertificate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteCertificateAsync(DeleteCertificateRequest deleteCertificateRequest);
/**
*
* Deletes the specified certificate.
*
*
* @param deleteCertificateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteCertificate operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DeleteCertificate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteCertificateAsync(DeleteCertificateRequest deleteCertificateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified endpoint.
*
*
*
* All tasks associated with the endpoint must be deleted before you can delete the endpoint.
*
*
*
*
* @param deleteEndpointRequest
* @return A Java Future containing the result of the DeleteEndpoint operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DeleteEndpoint
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteEndpointAsync(DeleteEndpointRequest deleteEndpointRequest);
/**
*
* Deletes the specified endpoint.
*
*
*
* All tasks associated with the endpoint must be deleted before you can delete the endpoint.
*
*
*
*
* @param deleteEndpointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteEndpoint operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DeleteEndpoint
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteEndpointAsync(DeleteEndpointRequest deleteEndpointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an AWS DMS event subscription.
*
*
* @param deleteEventSubscriptionRequest
* @return A Java Future containing the result of the DeleteEventSubscription operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DeleteEventSubscription
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteEventSubscriptionAsync(DeleteEventSubscriptionRequest deleteEventSubscriptionRequest);
/**
*
* Deletes an AWS DMS event subscription.
*
*
* @param deleteEventSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteEventSubscription operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DeleteEventSubscription
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteEventSubscriptionAsync(DeleteEventSubscriptionRequest deleteEventSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified replication instance.
*
*
*
* You must delete any migration tasks that are associated with the replication instance before you can delete it.
*
*
*
*
* @param deleteReplicationInstanceRequest
* @return A Java Future containing the result of the DeleteReplicationInstance operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DeleteReplicationInstance
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteReplicationInstanceAsync(
DeleteReplicationInstanceRequest deleteReplicationInstanceRequest);
/**
*
* Deletes the specified replication instance.
*
*
*
* You must delete any migration tasks that are associated with the replication instance before you can delete it.
*
*
*
*
* @param deleteReplicationInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteReplicationInstance operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DeleteReplicationInstance
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteReplicationInstanceAsync(
DeleteReplicationInstanceRequest deleteReplicationInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a subnet group.
*
*
* @param deleteReplicationSubnetGroupRequest
* @return A Java Future containing the result of the DeleteReplicationSubnetGroup operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.DeleteReplicationSubnetGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteReplicationSubnetGroupAsync(
DeleteReplicationSubnetGroupRequest deleteReplicationSubnetGroupRequest);
/**
*
* Deletes a subnet group.
*
*
* @param deleteReplicationSubnetGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteReplicationSubnetGroup operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DeleteReplicationSubnetGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteReplicationSubnetGroupAsync(
DeleteReplicationSubnetGroupRequest deleteReplicationSubnetGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified replication task.
*
*
* @param deleteReplicationTaskRequest
* @return A Java Future containing the result of the DeleteReplicationTask operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DeleteReplicationTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteReplicationTaskAsync(DeleteReplicationTaskRequest deleteReplicationTaskRequest);
/**
*
* Deletes the specified replication task.
*
*
* @param deleteReplicationTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteReplicationTask operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DeleteReplicationTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteReplicationTaskAsync(DeleteReplicationTaskRequest deleteReplicationTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all of the AWS DMS attributes for a customer account. The attributes include AWS DMS quotas for the
* account, such as the number of replication instances allowed. The description for a quota includes the quota
* name, current usage toward that quota, and the quota's maximum value.
*
*
* This command does not take any parameters.
*
*
* @param describeAccountAttributesRequest
* @return A Java Future containing the result of the DescribeAccountAttributes operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeAccountAttributes
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeAccountAttributesAsync(
DescribeAccountAttributesRequest describeAccountAttributesRequest);
/**
*
* Lists all of the AWS DMS attributes for a customer account. The attributes include AWS DMS quotas for the
* account, such as the number of replication instances allowed. The description for a quota includes the quota
* name, current usage toward that quota, and the quota's maximum value.
*
*
* This command does not take any parameters.
*
*
* @param describeAccountAttributesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAccountAttributes operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeAccountAttributes
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeAccountAttributesAsync(
DescribeAccountAttributesRequest describeAccountAttributesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides a description of the certificate.
*
*
* @param describeCertificatesRequest
* @return A Java Future containing the result of the DescribeCertificates operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeCertificates
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeCertificatesAsync(DescribeCertificatesRequest describeCertificatesRequest);
/**
*
* Provides a description of the certificate.
*
*
* @param describeCertificatesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeCertificates operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeCertificates
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeCertificatesAsync(DescribeCertificatesRequest describeCertificatesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the status of the connections that have been made between the replication instance and an endpoint.
* Connections are created when you test an endpoint.
*
*
* @param describeConnectionsRequest
* @return A Java Future containing the result of the DescribeConnections operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeConnections
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeConnectionsAsync(DescribeConnectionsRequest describeConnectionsRequest);
/**
*
* Describes the status of the connections that have been made between the replication instance and an endpoint.
* Connections are created when you test an endpoint.
*
*
* @param describeConnectionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeConnections operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeConnections
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeConnectionsAsync(DescribeConnectionsRequest describeConnectionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the type of endpoints available.
*
*
* @param describeEndpointTypesRequest
* @return A Java Future containing the result of the DescribeEndpointTypes operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeEndpointTypes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeEndpointTypesAsync(DescribeEndpointTypesRequest describeEndpointTypesRequest);
/**
*
* Returns information about the type of endpoints available.
*
*
* @param describeEndpointTypesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEndpointTypes operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeEndpointTypes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeEndpointTypesAsync(DescribeEndpointTypesRequest describeEndpointTypesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the endpoints for your account in the current region.
*
*
* @param describeEndpointsRequest
* @return A Java Future containing the result of the DescribeEndpoints operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeEndpoints
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeEndpointsAsync(DescribeEndpointsRequest describeEndpointsRequest);
/**
*
* Returns information about the endpoints for your account in the current region.
*
*
* @param describeEndpointsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEndpoints operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeEndpoints
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeEndpointsAsync(DescribeEndpointsRequest describeEndpointsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists categories for all event source types, or, if specified, for a specified source type. You can see a list of
* the event categories and source types in Working with Events and
* Notifications in the AWS Database Migration Service User Guide.
*
*
* @param describeEventCategoriesRequest
* @return A Java Future containing the result of the DescribeEventCategories operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeEventCategories
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeEventCategoriesAsync(DescribeEventCategoriesRequest describeEventCategoriesRequest);
/**
*
* Lists categories for all event source types, or, if specified, for a specified source type. You can see a list of
* the event categories and source types in Working with Events and
* Notifications in the AWS Database Migration Service User Guide.
*
*
* @param describeEventCategoriesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEventCategories operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeEventCategories
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeEventCategoriesAsync(DescribeEventCategoriesRequest describeEventCategoriesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all the event subscriptions for a customer account. The description of a subscription includes
* SubscriptionName
, SNSTopicARN
, CustomerID
, SourceType
,
* SourceID
, CreationTime
, and Status
.
*
*
* If you specify SubscriptionName
, this action lists the description for that subscription.
*
*
* @param describeEventSubscriptionsRequest
* @return A Java Future containing the result of the DescribeEventSubscriptions operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeEventSubscriptions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeEventSubscriptionsAsync(
DescribeEventSubscriptionsRequest describeEventSubscriptionsRequest);
/**
*
* Lists all the event subscriptions for a customer account. The description of a subscription includes
* SubscriptionName
, SNSTopicARN
, CustomerID
, SourceType
,
* SourceID
, CreationTime
, and Status
.
*
*
* If you specify SubscriptionName
, this action lists the description for that subscription.
*
*
* @param describeEventSubscriptionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEventSubscriptions operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeEventSubscriptions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeEventSubscriptionsAsync(
DescribeEventSubscriptionsRequest describeEventSubscriptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists events for a given source identifier and source type. You can also specify a start and end time. For more
* information on AWS DMS events, see Working with Events and
* Notifications in the AWS Database Migration User Guide.
*
*
* @param describeEventsRequest
* @return A Java Future containing the result of the DescribeEvents operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeEvents
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeEventsAsync(DescribeEventsRequest describeEventsRequest);
/**
*
* Lists events for a given source identifier and source type. You can also specify a start and end time. For more
* information on AWS DMS events, see Working with Events and
* Notifications in the AWS Database Migration User Guide.
*
*
* @param describeEventsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEvents operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeEvents
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeEventsAsync(DescribeEventsRequest describeEventsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the replication instance types that can be created in the specified region.
*
*
* @param describeOrderableReplicationInstancesRequest
* @return A Java Future containing the result of the DescribeOrderableReplicationInstances operation returned by
* the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeOrderableReplicationInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future describeOrderableReplicationInstancesAsync(
DescribeOrderableReplicationInstancesRequest describeOrderableReplicationInstancesRequest);
/**
*
* Returns information about the replication instance types that can be created in the specified region.
*
*
* @param describeOrderableReplicationInstancesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeOrderableReplicationInstances operation returned by
* the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeOrderableReplicationInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future describeOrderableReplicationInstancesAsync(
DescribeOrderableReplicationInstancesRequest describeOrderableReplicationInstancesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* For internal use only
*
*
* @param describePendingMaintenanceActionsRequest
* @return A Java Future containing the result of the DescribePendingMaintenanceActions operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.DescribePendingMaintenanceActions
* @see AWS API Documentation
*/
java.util.concurrent.Future describePendingMaintenanceActionsAsync(
DescribePendingMaintenanceActionsRequest describePendingMaintenanceActionsRequest);
/**
*
* For internal use only
*
*
* @param describePendingMaintenanceActionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribePendingMaintenanceActions operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribePendingMaintenanceActions
* @see AWS API Documentation
*/
java.util.concurrent.Future describePendingMaintenanceActionsAsync(
DescribePendingMaintenanceActionsRequest describePendingMaintenanceActionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the status of the RefreshSchemas operation.
*
*
* @param describeRefreshSchemasStatusRequest
* @return A Java Future containing the result of the DescribeRefreshSchemasStatus operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeRefreshSchemasStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRefreshSchemasStatusAsync(
DescribeRefreshSchemasStatusRequest describeRefreshSchemasStatusRequest);
/**
*
* Returns the status of the RefreshSchemas operation.
*
*
* @param describeRefreshSchemasStatusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeRefreshSchemasStatus operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeRefreshSchemasStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRefreshSchemasStatusAsync(
DescribeRefreshSchemasStatusRequest describeRefreshSchemasStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the task logs for the specified task.
*
*
* @param describeReplicationInstanceTaskLogsRequest
* @return A Java Future containing the result of the DescribeReplicationInstanceTaskLogs operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeReplicationInstanceTaskLogs
* @see AWS API Documentation
*/
java.util.concurrent.Future describeReplicationInstanceTaskLogsAsync(
DescribeReplicationInstanceTaskLogsRequest describeReplicationInstanceTaskLogsRequest);
/**
*
* Returns information about the task logs for the specified task.
*
*
* @param describeReplicationInstanceTaskLogsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeReplicationInstanceTaskLogs operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeReplicationInstanceTaskLogs
* @see AWS API Documentation
*/
java.util.concurrent.Future describeReplicationInstanceTaskLogsAsync(
DescribeReplicationInstanceTaskLogsRequest describeReplicationInstanceTaskLogsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about replication instances for your account in the current region.
*
*
* @param describeReplicationInstancesRequest
* @return A Java Future containing the result of the DescribeReplicationInstances operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeReplicationInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future describeReplicationInstancesAsync(
DescribeReplicationInstancesRequest describeReplicationInstancesRequest);
/**
*
* Returns information about replication instances for your account in the current region.
*
*
* @param describeReplicationInstancesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeReplicationInstances operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeReplicationInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future describeReplicationInstancesAsync(
DescribeReplicationInstancesRequest describeReplicationInstancesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the replication subnet groups.
*
*
* @param describeReplicationSubnetGroupsRequest
* @return A Java Future containing the result of the DescribeReplicationSubnetGroups operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeReplicationSubnetGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future describeReplicationSubnetGroupsAsync(
DescribeReplicationSubnetGroupsRequest describeReplicationSubnetGroupsRequest);
/**
*
* Returns information about the replication subnet groups.
*
*
* @param describeReplicationSubnetGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeReplicationSubnetGroups operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeReplicationSubnetGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future describeReplicationSubnetGroupsAsync(
DescribeReplicationSubnetGroupsRequest describeReplicationSubnetGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the task assessment results from Amazon S3. This action always returns the latest results.
*
*
* @param describeReplicationTaskAssessmentResultsRequest
* @return A Java Future containing the result of the DescribeReplicationTaskAssessmentResults operation returned by
* the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeReplicationTaskAssessmentResults
* @see AWS API Documentation
*/
java.util.concurrent.Future describeReplicationTaskAssessmentResultsAsync(
DescribeReplicationTaskAssessmentResultsRequest describeReplicationTaskAssessmentResultsRequest);
/**
*
* Returns the task assessment results from Amazon S3. This action always returns the latest results.
*
*
* @param describeReplicationTaskAssessmentResultsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeReplicationTaskAssessmentResults operation returned by
* the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeReplicationTaskAssessmentResults
* @see AWS API Documentation
*/
java.util.concurrent.Future describeReplicationTaskAssessmentResultsAsync(
DescribeReplicationTaskAssessmentResultsRequest describeReplicationTaskAssessmentResultsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about replication tasks for your account in the current region.
*
*
* @param describeReplicationTasksRequest
* @return A Java Future containing the result of the DescribeReplicationTasks operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeReplicationTasks
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeReplicationTasksAsync(DescribeReplicationTasksRequest describeReplicationTasksRequest);
/**
*
* Returns information about replication tasks for your account in the current region.
*
*
* @param describeReplicationTasksRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeReplicationTasks operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeReplicationTasks
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeReplicationTasksAsync(DescribeReplicationTasksRequest describeReplicationTasksRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the schema for the specified endpoint.
*
*
*
* @param describeSchemasRequest
* @return A Java Future containing the result of the DescribeSchemas operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeSchemas
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeSchemasAsync(DescribeSchemasRequest describeSchemasRequest);
/**
*
* Returns information about the schema for the specified endpoint.
*
*
*
* @param describeSchemasRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeSchemas operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeSchemas
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeSchemasAsync(DescribeSchemasRequest describeSchemasRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns table statistics on the database migration task, including table name, rows inserted, rows updated, and
* rows deleted.
*
*
* Note that the "last updated" column the DMS console only indicates the time that AWS DMS last updated the table
* statistics record for a table. It does not indicate the time of the last update to the table.
*
*
* @param describeTableStatisticsRequest
* @return A Java Future containing the result of the DescribeTableStatistics operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeTableStatistics
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeTableStatisticsAsync(DescribeTableStatisticsRequest describeTableStatisticsRequest);
/**
*
* Returns table statistics on the database migration task, including table name, rows inserted, rows updated, and
* rows deleted.
*
*
* Note that the "last updated" column the DMS console only indicates the time that AWS DMS last updated the table
* statistics record for a table. It does not indicate the time of the last update to the table.
*
*
* @param describeTableStatisticsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeTableStatistics operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeTableStatistics
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeTableStatisticsAsync(DescribeTableStatisticsRequest describeTableStatisticsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Uploads the specified certificate.
*
*
* @param importCertificateRequest
* @return A Java Future containing the result of the ImportCertificate operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.ImportCertificate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future importCertificateAsync(ImportCertificateRequest importCertificateRequest);
/**
*
* Uploads the specified certificate.
*
*
* @param importCertificateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ImportCertificate operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.ImportCertificate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future importCertificateAsync(ImportCertificateRequest importCertificateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all tags for an AWS DMS resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.ListTagsForResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists all tags for an AWS DMS resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.ListTagsForResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the specified endpoint.
*
*
* @param modifyEndpointRequest
* @return A Java Future containing the result of the ModifyEndpoint operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.ModifyEndpoint
* @see AWS API
* Documentation
*/
java.util.concurrent.Future modifyEndpointAsync(ModifyEndpointRequest modifyEndpointRequest);
/**
*
* Modifies the specified endpoint.
*
*
* @param modifyEndpointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyEndpoint operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.ModifyEndpoint
* @see AWS API
* Documentation
*/
java.util.concurrent.Future modifyEndpointAsync(ModifyEndpointRequest modifyEndpointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies an existing AWS DMS event notification subscription.
*
*
* @param modifyEventSubscriptionRequest
* @return A Java Future containing the result of the ModifyEventSubscription operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.ModifyEventSubscription
* @see AWS
* API Documentation
*/
java.util.concurrent.Future modifyEventSubscriptionAsync(ModifyEventSubscriptionRequest modifyEventSubscriptionRequest);
/**
*
* Modifies an existing AWS DMS event notification subscription.
*
*
* @param modifyEventSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyEventSubscription operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.ModifyEventSubscription
* @see AWS
* API Documentation
*/
java.util.concurrent.Future modifyEventSubscriptionAsync(ModifyEventSubscriptionRequest modifyEventSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the replication instance to apply new settings. You can change one or more parameters by specifying
* these parameters and the new values in the request.
*
*
* Some settings are applied during the maintenance window.
*
*
*
* @param modifyReplicationInstanceRequest
* @return A Java Future containing the result of the ModifyReplicationInstance operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.ModifyReplicationInstance
* @see AWS
* API Documentation
*/
java.util.concurrent.Future modifyReplicationInstanceAsync(
ModifyReplicationInstanceRequest modifyReplicationInstanceRequest);
/**
*
* Modifies the replication instance to apply new settings. You can change one or more parameters by specifying
* these parameters and the new values in the request.
*
*
* Some settings are applied during the maintenance window.
*
*
*
* @param modifyReplicationInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyReplicationInstance operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.ModifyReplicationInstance
* @see AWS
* API Documentation
*/
java.util.concurrent.Future modifyReplicationInstanceAsync(
ModifyReplicationInstanceRequest modifyReplicationInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the settings for the specified replication subnet group.
*
*
* @param modifyReplicationSubnetGroupRequest
* @return A Java Future containing the result of the ModifyReplicationSubnetGroup operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.ModifyReplicationSubnetGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future modifyReplicationSubnetGroupAsync(
ModifyReplicationSubnetGroupRequest modifyReplicationSubnetGroupRequest);
/**
*
* Modifies the settings for the specified replication subnet group.
*
*
* @param modifyReplicationSubnetGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyReplicationSubnetGroup operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.ModifyReplicationSubnetGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future modifyReplicationSubnetGroupAsync(
ModifyReplicationSubnetGroupRequest modifyReplicationSubnetGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the specified replication task.
*
*
* You can't modify the task endpoints. The task must be stopped before you can modify it.
*
*
* For more information about AWS DMS tasks, see Working with Migration Tasks in the
* AWS Database Migration Service User Guide.
*
*
* @param modifyReplicationTaskRequest
* @return A Java Future containing the result of the ModifyReplicationTask operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.ModifyReplicationTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future modifyReplicationTaskAsync(ModifyReplicationTaskRequest modifyReplicationTaskRequest);
/**
*
* Modifies the specified replication task.
*
*
* You can't modify the task endpoints. The task must be stopped before you can modify it.
*
*
* For more information about AWS DMS tasks, see Working with Migration Tasks in the
* AWS Database Migration Service User Guide.
*
*
* @param modifyReplicationTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyReplicationTask operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.ModifyReplicationTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future modifyReplicationTaskAsync(ModifyReplicationTaskRequest modifyReplicationTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Reboots a replication instance. Rebooting results in a momentary outage, until the replication instance becomes
* available again.
*
*
* @param rebootReplicationInstanceRequest
* @return A Java Future containing the result of the RebootReplicationInstance operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.RebootReplicationInstance
* @see AWS
* API Documentation
*/
java.util.concurrent.Future rebootReplicationInstanceAsync(
RebootReplicationInstanceRequest rebootReplicationInstanceRequest);
/**
*
* Reboots a replication instance. Rebooting results in a momentary outage, until the replication instance becomes
* available again.
*
*
* @param rebootReplicationInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RebootReplicationInstance operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.RebootReplicationInstance
* @see AWS
* API Documentation
*/
java.util.concurrent.Future rebootReplicationInstanceAsync(
RebootReplicationInstanceRequest rebootReplicationInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Populates the schema for the specified endpoint. This is an asynchronous operation and can take several minutes.
* You can check the status of this operation by calling the DescribeRefreshSchemasStatus operation.
*
*
* @param refreshSchemasRequest
* @return A Java Future containing the result of the RefreshSchemas operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.RefreshSchemas
* @see AWS API
* Documentation
*/
java.util.concurrent.Future refreshSchemasAsync(RefreshSchemasRequest refreshSchemasRequest);
/**
*
* Populates the schema for the specified endpoint. This is an asynchronous operation and can take several minutes.
* You can check the status of this operation by calling the DescribeRefreshSchemasStatus operation.
*
*
* @param refreshSchemasRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RefreshSchemas operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.RefreshSchemas
* @see AWS API
* Documentation
*/
java.util.concurrent.Future refreshSchemasAsync(RefreshSchemasRequest refreshSchemasRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Reloads the target database table with the source data.
*
*
* @param reloadTablesRequest
* @return A Java Future containing the result of the ReloadTables operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.ReloadTables
* @see AWS API
* Documentation
*/
java.util.concurrent.Future reloadTablesAsync(ReloadTablesRequest reloadTablesRequest);
/**
*
* Reloads the target database table with the source data.
*
*
* @param reloadTablesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ReloadTables operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.ReloadTables
* @see AWS API
* Documentation
*/
java.util.concurrent.Future reloadTablesAsync(ReloadTablesRequest reloadTablesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes metadata tags from a DMS resource.
*
*
* @param removeTagsFromResourceRequest
* @return A Java Future containing the result of the RemoveTagsFromResource operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.RemoveTagsFromResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future removeTagsFromResourceAsync(RemoveTagsFromResourceRequest removeTagsFromResourceRequest);
/**
*
* Removes metadata tags from a DMS resource.
*
*
* @param removeTagsFromResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RemoveTagsFromResource operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.RemoveTagsFromResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future removeTagsFromResourceAsync(RemoveTagsFromResourceRequest removeTagsFromResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts the replication task.
*
*
* For more information about AWS DMS tasks, see Working with Migration Tasks in the
* AWS Database Migration Service User Guide.
*
*
* @param startReplicationTaskRequest
* @return A Java Future containing the result of the StartReplicationTask operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.StartReplicationTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startReplicationTaskAsync(StartReplicationTaskRequest startReplicationTaskRequest);
/**
*
* Starts the replication task.
*
*
* For more information about AWS DMS tasks, see Working with Migration Tasks in the
* AWS Database Migration Service User Guide.
*
*
* @param startReplicationTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartReplicationTask operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.StartReplicationTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future startReplicationTaskAsync(StartReplicationTaskRequest startReplicationTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts the replication task assessment for unsupported data types in the source database.
*
*
* @param startReplicationTaskAssessmentRequest
* @return A Java Future containing the result of the StartReplicationTaskAssessment operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.StartReplicationTaskAssessment
* @see AWS API Documentation
*/
java.util.concurrent.Future startReplicationTaskAssessmentAsync(
StartReplicationTaskAssessmentRequest startReplicationTaskAssessmentRequest);
/**
*
* Starts the replication task assessment for unsupported data types in the source database.
*
*
* @param startReplicationTaskAssessmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartReplicationTaskAssessment operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.StartReplicationTaskAssessment
* @see AWS API Documentation
*/
java.util.concurrent.Future startReplicationTaskAssessmentAsync(
StartReplicationTaskAssessmentRequest startReplicationTaskAssessmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Stops the replication task.
*
*
*
* @param stopReplicationTaskRequest
* @return A Java Future containing the result of the StopReplicationTask operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.StopReplicationTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future stopReplicationTaskAsync(StopReplicationTaskRequest stopReplicationTaskRequest);
/**
*
* Stops the replication task.
*
*
*
* @param stopReplicationTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StopReplicationTask operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.StopReplicationTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future stopReplicationTaskAsync(StopReplicationTaskRequest stopReplicationTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Tests the connection between the replication instance and the endpoint.
*
*
* @param testConnectionRequest
* @return A Java Future containing the result of the TestConnection operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.TestConnection
* @see AWS API
* Documentation
*/
java.util.concurrent.Future testConnectionAsync(TestConnectionRequest testConnectionRequest);
/**
*
* Tests the connection between the replication instance and the endpoint.
*
*
* @param testConnectionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TestConnection operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.TestConnection
* @see AWS API
* Documentation
*/
java.util.concurrent.Future testConnectionAsync(TestConnectionRequest testConnectionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}