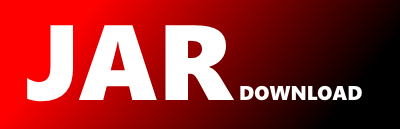
com.amazonaws.services.databasemigrationservice.model.CreateReplicationInstanceRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-dms Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.databasemigrationservice.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateReplicationInstanceRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The replication instance identifier. This parameter is stored as a lowercase string.
*
*
* Constraints:
*
*
* -
*
* Must contain 1-63 alphanumeric characters or hyphens.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Can't end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: myrepinstance
*
*/
private String replicationInstanceIdentifier;
/**
*
* The amount of storage (in gigabytes) to be initially allocated for the replication instance.
*
*/
private Integer allocatedStorage;
/**
*
* The compute and memory capacity of the replication instance as defined for the specified replication instance
* class. For example to specify the instance class dms.c4.large, set this parameter to "dms.c4.large"
.
*
*
* For more information on the settings and capacities for the available replication instance classes, see Selecting the right DMS replication instance for your migration.
*
*/
private String replicationInstanceClass;
/**
*
* Specifies the VPC security group to be used with the replication instance. The VPC security group must work with
* the VPC containing the replication instance.
*
*/
private java.util.List vpcSecurityGroupIds;
/**
*
* The Availability Zone where the replication instance will be created. The default value is a random,
* system-chosen Availability Zone in the endpoint's Amazon Web Services Region, for example:
* us-east-1d
*
*/
private String availabilityZone;
/**
*
* A subnet group to associate with the replication instance.
*
*/
private String replicationSubnetGroupIdentifier;
/**
*
* The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
*
* Format: ddd:hh24:mi-ddd:hh24:mi
*
*
* Default: A 30-minute window selected at random from an 8-hour block of time per Amazon Web Services Region,
* occurring on a random day of the week.
*
*
* Valid Days: Mon, Tue, Wed, Thu, Fri, Sat, Sun
*
*
* Constraints: Minimum 30-minute window.
*
*/
private String preferredMaintenanceWindow;
/**
*
* Specifies whether the replication instance is a Multi-AZ deployment. You can't set the
* AvailabilityZone
parameter if the Multi-AZ parameter is set to true
.
*
*/
private Boolean multiAZ;
/**
*
* The engine version number of the replication instance.
*
*
* If an engine version number is not specified when a replication instance is created, the default is the latest
* engine version available.
*
*/
private String engineVersion;
/**
*
* A value that indicates whether minor engine upgrades are applied automatically to the replication instance during
* the maintenance window. This parameter defaults to true
.
*
*
* Default: true
*
*/
private Boolean autoMinorVersionUpgrade;
/**
*
* One or more tags to be assigned to the replication instance.
*
*/
private java.util.List tags;
/**
*
* An KMS key identifier that is used to encrypt the data on the replication instance.
*
*
* If you don't specify a value for the KmsKeyId
parameter, then DMS uses your default encryption key.
*
*
* KMS creates the default encryption key for your Amazon Web Services account. Your Amazon Web Services account has
* a different default encryption key for each Amazon Web Services Region.
*
*/
private String kmsKeyId;
/**
*
* Specifies the accessibility options for the replication instance. A value of true
represents an
* instance with a public IP address. A value of false
represents an instance with a private IP
* address. The default value is true
.
*
*/
private Boolean publiclyAccessible;
/**
*
* A list of custom DNS name servers supported for the replication instance to access your on-premise source or
* target database. This list overrides the default name servers supported by the replication instance. You can
* specify a comma-separated list of internet addresses for up to four on-premise DNS name servers. For example:
* "1.1.1.1,2.2.2.2,3.3.3.3,4.4.4.4"
*
*/
private String dnsNameServers;
/**
*
* A friendly name for the resource identifier at the end of the EndpointArn
response parameter that is
* returned in the created Endpoint
object. The value for this parameter can have up to 31 characters.
* It can contain only ASCII letters, digits, and hyphen ('-'). Also, it can't end with a hyphen or contain two
* consecutive hyphens, and can only begin with a letter, such as Example-App-ARN1
. For example, this
* value might result in the EndpointArn
value
* arn:aws:dms:eu-west-1:012345678901:rep:Example-App-ARN1
. If you don't specify a
* ResourceIdentifier
value, DMS generates a default identifier value for the end of
* EndpointArn
.
*
*/
private String resourceIdentifier;
/**
*
* The replication instance identifier. This parameter is stored as a lowercase string.
*
*
* Constraints:
*
*
* -
*
* Must contain 1-63 alphanumeric characters or hyphens.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Can't end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: myrepinstance
*
*
* @param replicationInstanceIdentifier
* The replication instance identifier. This parameter is stored as a lowercase string.
*
* Constraints:
*
*
* -
*
* Must contain 1-63 alphanumeric characters or hyphens.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Can't end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: myrepinstance
*/
public void setReplicationInstanceIdentifier(String replicationInstanceIdentifier) {
this.replicationInstanceIdentifier = replicationInstanceIdentifier;
}
/**
*
* The replication instance identifier. This parameter is stored as a lowercase string.
*
*
* Constraints:
*
*
* -
*
* Must contain 1-63 alphanumeric characters or hyphens.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Can't end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: myrepinstance
*
*
* @return The replication instance identifier. This parameter is stored as a lowercase string.
*
* Constraints:
*
*
* -
*
* Must contain 1-63 alphanumeric characters or hyphens.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Can't end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: myrepinstance
*/
public String getReplicationInstanceIdentifier() {
return this.replicationInstanceIdentifier;
}
/**
*
* The replication instance identifier. This parameter is stored as a lowercase string.
*
*
* Constraints:
*
*
* -
*
* Must contain 1-63 alphanumeric characters or hyphens.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Can't end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: myrepinstance
*
*
* @param replicationInstanceIdentifier
* The replication instance identifier. This parameter is stored as a lowercase string.
*
* Constraints:
*
*
* -
*
* Must contain 1-63 alphanumeric characters or hyphens.
*
*
* -
*
* First character must be a letter.
*
*
* -
*
* Can't end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: myrepinstance
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateReplicationInstanceRequest withReplicationInstanceIdentifier(String replicationInstanceIdentifier) {
setReplicationInstanceIdentifier(replicationInstanceIdentifier);
return this;
}
/**
*
* The amount of storage (in gigabytes) to be initially allocated for the replication instance.
*
*
* @param allocatedStorage
* The amount of storage (in gigabytes) to be initially allocated for the replication instance.
*/
public void setAllocatedStorage(Integer allocatedStorage) {
this.allocatedStorage = allocatedStorage;
}
/**
*
* The amount of storage (in gigabytes) to be initially allocated for the replication instance.
*
*
* @return The amount of storage (in gigabytes) to be initially allocated for the replication instance.
*/
public Integer getAllocatedStorage() {
return this.allocatedStorage;
}
/**
*
* The amount of storage (in gigabytes) to be initially allocated for the replication instance.
*
*
* @param allocatedStorage
* The amount of storage (in gigabytes) to be initially allocated for the replication instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateReplicationInstanceRequest withAllocatedStorage(Integer allocatedStorage) {
setAllocatedStorage(allocatedStorage);
return this;
}
/**
*
* The compute and memory capacity of the replication instance as defined for the specified replication instance
* class. For example to specify the instance class dms.c4.large, set this parameter to "dms.c4.large"
.
*
*
* For more information on the settings and capacities for the available replication instance classes, see Selecting the right DMS replication instance for your migration.
*
*
* @param replicationInstanceClass
* The compute and memory capacity of the replication instance as defined for the specified replication
* instance class. For example to specify the instance class dms.c4.large, set this parameter to
* "dms.c4.large"
.
*
* For more information on the settings and capacities for the available replication instance classes, see Selecting the right DMS replication instance for your migration.
*/
public void setReplicationInstanceClass(String replicationInstanceClass) {
this.replicationInstanceClass = replicationInstanceClass;
}
/**
*
* The compute and memory capacity of the replication instance as defined for the specified replication instance
* class. For example to specify the instance class dms.c4.large, set this parameter to "dms.c4.large"
.
*
*
* For more information on the settings and capacities for the available replication instance classes, see Selecting the right DMS replication instance for your migration.
*
*
* @return The compute and memory capacity of the replication instance as defined for the specified replication
* instance class. For example to specify the instance class dms.c4.large, set this parameter to
* "dms.c4.large"
.
*
* For more information on the settings and capacities for the available replication instance classes, see
* Selecting the right DMS replication instance for your migration.
*/
public String getReplicationInstanceClass() {
return this.replicationInstanceClass;
}
/**
*
* The compute and memory capacity of the replication instance as defined for the specified replication instance
* class. For example to specify the instance class dms.c4.large, set this parameter to "dms.c4.large"
.
*
*
* For more information on the settings and capacities for the available replication instance classes, see Selecting the right DMS replication instance for your migration.
*
*
* @param replicationInstanceClass
* The compute and memory capacity of the replication instance as defined for the specified replication
* instance class. For example to specify the instance class dms.c4.large, set this parameter to
* "dms.c4.large"
.
*
* For more information on the settings and capacities for the available replication instance classes, see Selecting the right DMS replication instance for your migration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateReplicationInstanceRequest withReplicationInstanceClass(String replicationInstanceClass) {
setReplicationInstanceClass(replicationInstanceClass);
return this;
}
/**
*
* Specifies the VPC security group to be used with the replication instance. The VPC security group must work with
* the VPC containing the replication instance.
*
*
* @return Specifies the VPC security group to be used with the replication instance. The VPC security group must
* work with the VPC containing the replication instance.
*/
public java.util.List getVpcSecurityGroupIds() {
return vpcSecurityGroupIds;
}
/**
*
* Specifies the VPC security group to be used with the replication instance. The VPC security group must work with
* the VPC containing the replication instance.
*
*
* @param vpcSecurityGroupIds
* Specifies the VPC security group to be used with the replication instance. The VPC security group must
* work with the VPC containing the replication instance.
*/
public void setVpcSecurityGroupIds(java.util.Collection vpcSecurityGroupIds) {
if (vpcSecurityGroupIds == null) {
this.vpcSecurityGroupIds = null;
return;
}
this.vpcSecurityGroupIds = new java.util.ArrayList(vpcSecurityGroupIds);
}
/**
*
* Specifies the VPC security group to be used with the replication instance. The VPC security group must work with
* the VPC containing the replication instance.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setVpcSecurityGroupIds(java.util.Collection)} or {@link #withVpcSecurityGroupIds(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param vpcSecurityGroupIds
* Specifies the VPC security group to be used with the replication instance. The VPC security group must
* work with the VPC containing the replication instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateReplicationInstanceRequest withVpcSecurityGroupIds(String... vpcSecurityGroupIds) {
if (this.vpcSecurityGroupIds == null) {
setVpcSecurityGroupIds(new java.util.ArrayList(vpcSecurityGroupIds.length));
}
for (String ele : vpcSecurityGroupIds) {
this.vpcSecurityGroupIds.add(ele);
}
return this;
}
/**
*
* Specifies the VPC security group to be used with the replication instance. The VPC security group must work with
* the VPC containing the replication instance.
*
*
* @param vpcSecurityGroupIds
* Specifies the VPC security group to be used with the replication instance. The VPC security group must
* work with the VPC containing the replication instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateReplicationInstanceRequest withVpcSecurityGroupIds(java.util.Collection vpcSecurityGroupIds) {
setVpcSecurityGroupIds(vpcSecurityGroupIds);
return this;
}
/**
*
* The Availability Zone where the replication instance will be created. The default value is a random,
* system-chosen Availability Zone in the endpoint's Amazon Web Services Region, for example:
* us-east-1d
*
*
* @param availabilityZone
* The Availability Zone where the replication instance will be created. The default value is a random,
* system-chosen Availability Zone in the endpoint's Amazon Web Services Region, for example:
* us-east-1d
*/
public void setAvailabilityZone(String availabilityZone) {
this.availabilityZone = availabilityZone;
}
/**
*
* The Availability Zone where the replication instance will be created. The default value is a random,
* system-chosen Availability Zone in the endpoint's Amazon Web Services Region, for example:
* us-east-1d
*
*
* @return The Availability Zone where the replication instance will be created. The default value is a random,
* system-chosen Availability Zone in the endpoint's Amazon Web Services Region, for example:
* us-east-1d
*/
public String getAvailabilityZone() {
return this.availabilityZone;
}
/**
*
* The Availability Zone where the replication instance will be created. The default value is a random,
* system-chosen Availability Zone in the endpoint's Amazon Web Services Region, for example:
* us-east-1d
*
*
* @param availabilityZone
* The Availability Zone where the replication instance will be created. The default value is a random,
* system-chosen Availability Zone in the endpoint's Amazon Web Services Region, for example:
* us-east-1d
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateReplicationInstanceRequest withAvailabilityZone(String availabilityZone) {
setAvailabilityZone(availabilityZone);
return this;
}
/**
*
* A subnet group to associate with the replication instance.
*
*
* @param replicationSubnetGroupIdentifier
* A subnet group to associate with the replication instance.
*/
public void setReplicationSubnetGroupIdentifier(String replicationSubnetGroupIdentifier) {
this.replicationSubnetGroupIdentifier = replicationSubnetGroupIdentifier;
}
/**
*
* A subnet group to associate with the replication instance.
*
*
* @return A subnet group to associate with the replication instance.
*/
public String getReplicationSubnetGroupIdentifier() {
return this.replicationSubnetGroupIdentifier;
}
/**
*
* A subnet group to associate with the replication instance.
*
*
* @param replicationSubnetGroupIdentifier
* A subnet group to associate with the replication instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateReplicationInstanceRequest withReplicationSubnetGroupIdentifier(String replicationSubnetGroupIdentifier) {
setReplicationSubnetGroupIdentifier(replicationSubnetGroupIdentifier);
return this;
}
/**
*
* The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
*
* Format: ddd:hh24:mi-ddd:hh24:mi
*
*
* Default: A 30-minute window selected at random from an 8-hour block of time per Amazon Web Services Region,
* occurring on a random day of the week.
*
*
* Valid Days: Mon, Tue, Wed, Thu, Fri, Sat, Sun
*
*
* Constraints: Minimum 30-minute window.
*
*
* @param preferredMaintenanceWindow
* The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
* Format: ddd:hh24:mi-ddd:hh24:mi
*
*
* Default: A 30-minute window selected at random from an 8-hour block of time per Amazon Web Services
* Region, occurring on a random day of the week.
*
*
* Valid Days: Mon, Tue, Wed, Thu, Fri, Sat, Sun
*
*
* Constraints: Minimum 30-minute window.
*/
public void setPreferredMaintenanceWindow(String preferredMaintenanceWindow) {
this.preferredMaintenanceWindow = preferredMaintenanceWindow;
}
/**
*
* The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
*
* Format: ddd:hh24:mi-ddd:hh24:mi
*
*
* Default: A 30-minute window selected at random from an 8-hour block of time per Amazon Web Services Region,
* occurring on a random day of the week.
*
*
* Valid Days: Mon, Tue, Wed, Thu, Fri, Sat, Sun
*
*
* Constraints: Minimum 30-minute window.
*
*
* @return The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
* Format: ddd:hh24:mi-ddd:hh24:mi
*
*
* Default: A 30-minute window selected at random from an 8-hour block of time per Amazon Web Services
* Region, occurring on a random day of the week.
*
*
* Valid Days: Mon, Tue, Wed, Thu, Fri, Sat, Sun
*
*
* Constraints: Minimum 30-minute window.
*/
public String getPreferredMaintenanceWindow() {
return this.preferredMaintenanceWindow;
}
/**
*
* The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
*
* Format: ddd:hh24:mi-ddd:hh24:mi
*
*
* Default: A 30-minute window selected at random from an 8-hour block of time per Amazon Web Services Region,
* occurring on a random day of the week.
*
*
* Valid Days: Mon, Tue, Wed, Thu, Fri, Sat, Sun
*
*
* Constraints: Minimum 30-minute window.
*
*
* @param preferredMaintenanceWindow
* The weekly time range during which system maintenance can occur, in Universal Coordinated Time (UTC).
*
* Format: ddd:hh24:mi-ddd:hh24:mi
*
*
* Default: A 30-minute window selected at random from an 8-hour block of time per Amazon Web Services
* Region, occurring on a random day of the week.
*
*
* Valid Days: Mon, Tue, Wed, Thu, Fri, Sat, Sun
*
*
* Constraints: Minimum 30-minute window.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateReplicationInstanceRequest withPreferredMaintenanceWindow(String preferredMaintenanceWindow) {
setPreferredMaintenanceWindow(preferredMaintenanceWindow);
return this;
}
/**
*
* Specifies whether the replication instance is a Multi-AZ deployment. You can't set the
* AvailabilityZone
parameter if the Multi-AZ parameter is set to true
.
*
*
* @param multiAZ
* Specifies whether the replication instance is a Multi-AZ deployment. You can't set the
* AvailabilityZone
parameter if the Multi-AZ parameter is set to true
.
*/
public void setMultiAZ(Boolean multiAZ) {
this.multiAZ = multiAZ;
}
/**
*
* Specifies whether the replication instance is a Multi-AZ deployment. You can't set the
* AvailabilityZone
parameter if the Multi-AZ parameter is set to true
.
*
*
* @return Specifies whether the replication instance is a Multi-AZ deployment. You can't set the
* AvailabilityZone
parameter if the Multi-AZ parameter is set to true
.
*/
public Boolean getMultiAZ() {
return this.multiAZ;
}
/**
*
* Specifies whether the replication instance is a Multi-AZ deployment. You can't set the
* AvailabilityZone
parameter if the Multi-AZ parameter is set to true
.
*
*
* @param multiAZ
* Specifies whether the replication instance is a Multi-AZ deployment. You can't set the
* AvailabilityZone
parameter if the Multi-AZ parameter is set to true
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateReplicationInstanceRequest withMultiAZ(Boolean multiAZ) {
setMultiAZ(multiAZ);
return this;
}
/**
*
* Specifies whether the replication instance is a Multi-AZ deployment. You can't set the
* AvailabilityZone
parameter if the Multi-AZ parameter is set to true
.
*
*
* @return Specifies whether the replication instance is a Multi-AZ deployment. You can't set the
* AvailabilityZone
parameter if the Multi-AZ parameter is set to true
.
*/
public Boolean isMultiAZ() {
return this.multiAZ;
}
/**
*
* The engine version number of the replication instance.
*
*
* If an engine version number is not specified when a replication instance is created, the default is the latest
* engine version available.
*
*
* @param engineVersion
* The engine version number of the replication instance.
*
* If an engine version number is not specified when a replication instance is created, the default is the
* latest engine version available.
*/
public void setEngineVersion(String engineVersion) {
this.engineVersion = engineVersion;
}
/**
*
* The engine version number of the replication instance.
*
*
* If an engine version number is not specified when a replication instance is created, the default is the latest
* engine version available.
*
*
* @return The engine version number of the replication instance.
*
* If an engine version number is not specified when a replication instance is created, the default is the
* latest engine version available.
*/
public String getEngineVersion() {
return this.engineVersion;
}
/**
*
* The engine version number of the replication instance.
*
*
* If an engine version number is not specified when a replication instance is created, the default is the latest
* engine version available.
*
*
* @param engineVersion
* The engine version number of the replication instance.
*
* If an engine version number is not specified when a replication instance is created, the default is the
* latest engine version available.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateReplicationInstanceRequest withEngineVersion(String engineVersion) {
setEngineVersion(engineVersion);
return this;
}
/**
*
* A value that indicates whether minor engine upgrades are applied automatically to the replication instance during
* the maintenance window. This parameter defaults to true
.
*
*
* Default: true
*
*
* @param autoMinorVersionUpgrade
* A value that indicates whether minor engine upgrades are applied automatically to the replication instance
* during the maintenance window. This parameter defaults to true
.
*
* Default: true
*/
public void setAutoMinorVersionUpgrade(Boolean autoMinorVersionUpgrade) {
this.autoMinorVersionUpgrade = autoMinorVersionUpgrade;
}
/**
*
* A value that indicates whether minor engine upgrades are applied automatically to the replication instance during
* the maintenance window. This parameter defaults to true
.
*
*
* Default: true
*
*
* @return A value that indicates whether minor engine upgrades are applied automatically to the replication
* instance during the maintenance window. This parameter defaults to true
.
*
* Default: true
*/
public Boolean getAutoMinorVersionUpgrade() {
return this.autoMinorVersionUpgrade;
}
/**
*
* A value that indicates whether minor engine upgrades are applied automatically to the replication instance during
* the maintenance window. This parameter defaults to true
.
*
*
* Default: true
*
*
* @param autoMinorVersionUpgrade
* A value that indicates whether minor engine upgrades are applied automatically to the replication instance
* during the maintenance window. This parameter defaults to true
.
*
* Default: true
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateReplicationInstanceRequest withAutoMinorVersionUpgrade(Boolean autoMinorVersionUpgrade) {
setAutoMinorVersionUpgrade(autoMinorVersionUpgrade);
return this;
}
/**
*
* A value that indicates whether minor engine upgrades are applied automatically to the replication instance during
* the maintenance window. This parameter defaults to true
.
*
*
* Default: true
*
*
* @return A value that indicates whether minor engine upgrades are applied automatically to the replication
* instance during the maintenance window. This parameter defaults to true
.
*
* Default: true
*/
public Boolean isAutoMinorVersionUpgrade() {
return this.autoMinorVersionUpgrade;
}
/**
*
* One or more tags to be assigned to the replication instance.
*
*
* @return One or more tags to be assigned to the replication instance.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* One or more tags to be assigned to the replication instance.
*
*
* @param tags
* One or more tags to be assigned to the replication instance.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* One or more tags to be assigned to the replication instance.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* One or more tags to be assigned to the replication instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateReplicationInstanceRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* One or more tags to be assigned to the replication instance.
*
*
* @param tags
* One or more tags to be assigned to the replication instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateReplicationInstanceRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* An KMS key identifier that is used to encrypt the data on the replication instance.
*
*
* If you don't specify a value for the KmsKeyId
parameter, then DMS uses your default encryption key.
*
*
* KMS creates the default encryption key for your Amazon Web Services account. Your Amazon Web Services account has
* a different default encryption key for each Amazon Web Services Region.
*
*
* @param kmsKeyId
* An KMS key identifier that is used to encrypt the data on the replication instance.
*
* If you don't specify a value for the KmsKeyId
parameter, then DMS uses your default
* encryption key.
*
*
* KMS creates the default encryption key for your Amazon Web Services account. Your Amazon Web Services
* account has a different default encryption key for each Amazon Web Services Region.
*/
public void setKmsKeyId(String kmsKeyId) {
this.kmsKeyId = kmsKeyId;
}
/**
*
* An KMS key identifier that is used to encrypt the data on the replication instance.
*
*
* If you don't specify a value for the KmsKeyId
parameter, then DMS uses your default encryption key.
*
*
* KMS creates the default encryption key for your Amazon Web Services account. Your Amazon Web Services account has
* a different default encryption key for each Amazon Web Services Region.
*
*
* @return An KMS key identifier that is used to encrypt the data on the replication instance.
*
* If you don't specify a value for the KmsKeyId
parameter, then DMS uses your default
* encryption key.
*
*
* KMS creates the default encryption key for your Amazon Web Services account. Your Amazon Web Services
* account has a different default encryption key for each Amazon Web Services Region.
*/
public String getKmsKeyId() {
return this.kmsKeyId;
}
/**
*
* An KMS key identifier that is used to encrypt the data on the replication instance.
*
*
* If you don't specify a value for the KmsKeyId
parameter, then DMS uses your default encryption key.
*
*
* KMS creates the default encryption key for your Amazon Web Services account. Your Amazon Web Services account has
* a different default encryption key for each Amazon Web Services Region.
*
*
* @param kmsKeyId
* An KMS key identifier that is used to encrypt the data on the replication instance.
*
* If you don't specify a value for the KmsKeyId
parameter, then DMS uses your default
* encryption key.
*
*
* KMS creates the default encryption key for your Amazon Web Services account. Your Amazon Web Services
* account has a different default encryption key for each Amazon Web Services Region.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateReplicationInstanceRequest withKmsKeyId(String kmsKeyId) {
setKmsKeyId(kmsKeyId);
return this;
}
/**
*
* Specifies the accessibility options for the replication instance. A value of true
represents an
* instance with a public IP address. A value of false
represents an instance with a private IP
* address. The default value is true
.
*
*
* @param publiclyAccessible
* Specifies the accessibility options for the replication instance. A value of true
represents
* an instance with a public IP address. A value of false
represents an instance with a private
* IP address. The default value is true
.
*/
public void setPubliclyAccessible(Boolean publiclyAccessible) {
this.publiclyAccessible = publiclyAccessible;
}
/**
*
* Specifies the accessibility options for the replication instance. A value of true
represents an
* instance with a public IP address. A value of false
represents an instance with a private IP
* address. The default value is true
.
*
*
* @return Specifies the accessibility options for the replication instance. A value of true
represents
* an instance with a public IP address. A value of false
represents an instance with a private
* IP address. The default value is true
.
*/
public Boolean getPubliclyAccessible() {
return this.publiclyAccessible;
}
/**
*
* Specifies the accessibility options for the replication instance. A value of true
represents an
* instance with a public IP address. A value of false
represents an instance with a private IP
* address. The default value is true
.
*
*
* @param publiclyAccessible
* Specifies the accessibility options for the replication instance. A value of true
represents
* an instance with a public IP address. A value of false
represents an instance with a private
* IP address. The default value is true
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateReplicationInstanceRequest withPubliclyAccessible(Boolean publiclyAccessible) {
setPubliclyAccessible(publiclyAccessible);
return this;
}
/**
*
* Specifies the accessibility options for the replication instance. A value of true
represents an
* instance with a public IP address. A value of false
represents an instance with a private IP
* address. The default value is true
.
*
*
* @return Specifies the accessibility options for the replication instance. A value of true
represents
* an instance with a public IP address. A value of false
represents an instance with a private
* IP address. The default value is true
.
*/
public Boolean isPubliclyAccessible() {
return this.publiclyAccessible;
}
/**
*
* A list of custom DNS name servers supported for the replication instance to access your on-premise source or
* target database. This list overrides the default name servers supported by the replication instance. You can
* specify a comma-separated list of internet addresses for up to four on-premise DNS name servers. For example:
* "1.1.1.1,2.2.2.2,3.3.3.3,4.4.4.4"
*
*
* @param dnsNameServers
* A list of custom DNS name servers supported for the replication instance to access your on-premise source
* or target database. This list overrides the default name servers supported by the replication instance.
* You can specify a comma-separated list of internet addresses for up to four on-premise DNS name servers.
* For example: "1.1.1.1,2.2.2.2,3.3.3.3,4.4.4.4"
*/
public void setDnsNameServers(String dnsNameServers) {
this.dnsNameServers = dnsNameServers;
}
/**
*
* A list of custom DNS name servers supported for the replication instance to access your on-premise source or
* target database. This list overrides the default name servers supported by the replication instance. You can
* specify a comma-separated list of internet addresses for up to four on-premise DNS name servers. For example:
* "1.1.1.1,2.2.2.2,3.3.3.3,4.4.4.4"
*
*
* @return A list of custom DNS name servers supported for the replication instance to access your on-premise source
* or target database. This list overrides the default name servers supported by the replication instance.
* You can specify a comma-separated list of internet addresses for up to four on-premise DNS name servers.
* For example: "1.1.1.1,2.2.2.2,3.3.3.3,4.4.4.4"
*/
public String getDnsNameServers() {
return this.dnsNameServers;
}
/**
*
* A list of custom DNS name servers supported for the replication instance to access your on-premise source or
* target database. This list overrides the default name servers supported by the replication instance. You can
* specify a comma-separated list of internet addresses for up to four on-premise DNS name servers. For example:
* "1.1.1.1,2.2.2.2,3.3.3.3,4.4.4.4"
*
*
* @param dnsNameServers
* A list of custom DNS name servers supported for the replication instance to access your on-premise source
* or target database. This list overrides the default name servers supported by the replication instance.
* You can specify a comma-separated list of internet addresses for up to four on-premise DNS name servers.
* For example: "1.1.1.1,2.2.2.2,3.3.3.3,4.4.4.4"
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateReplicationInstanceRequest withDnsNameServers(String dnsNameServers) {
setDnsNameServers(dnsNameServers);
return this;
}
/**
*
* A friendly name for the resource identifier at the end of the EndpointArn
response parameter that is
* returned in the created Endpoint
object. The value for this parameter can have up to 31 characters.
* It can contain only ASCII letters, digits, and hyphen ('-'). Also, it can't end with a hyphen or contain two
* consecutive hyphens, and can only begin with a letter, such as Example-App-ARN1
. For example, this
* value might result in the EndpointArn
value
* arn:aws:dms:eu-west-1:012345678901:rep:Example-App-ARN1
. If you don't specify a
* ResourceIdentifier
value, DMS generates a default identifier value for the end of
* EndpointArn
.
*
*
* @param resourceIdentifier
* A friendly name for the resource identifier at the end of the EndpointArn
response parameter
* that is returned in the created Endpoint
object. The value for this parameter can have up to
* 31 characters. It can contain only ASCII letters, digits, and hyphen ('-'). Also, it can't end with a
* hyphen or contain two consecutive hyphens, and can only begin with a letter, such as
* Example-App-ARN1
. For example, this value might result in the EndpointArn
value
* arn:aws:dms:eu-west-1:012345678901:rep:Example-App-ARN1
. If you don't specify a
* ResourceIdentifier
value, DMS generates a default identifier value for the end of
* EndpointArn
.
*/
public void setResourceIdentifier(String resourceIdentifier) {
this.resourceIdentifier = resourceIdentifier;
}
/**
*
* A friendly name for the resource identifier at the end of the EndpointArn
response parameter that is
* returned in the created Endpoint
object. The value for this parameter can have up to 31 characters.
* It can contain only ASCII letters, digits, and hyphen ('-'). Also, it can't end with a hyphen or contain two
* consecutive hyphens, and can only begin with a letter, such as Example-App-ARN1
. For example, this
* value might result in the EndpointArn
value
* arn:aws:dms:eu-west-1:012345678901:rep:Example-App-ARN1
. If you don't specify a
* ResourceIdentifier
value, DMS generates a default identifier value for the end of
* EndpointArn
.
*
*
* @return A friendly name for the resource identifier at the end of the EndpointArn
response parameter
* that is returned in the created Endpoint
object. The value for this parameter can have up to
* 31 characters. It can contain only ASCII letters, digits, and hyphen ('-'). Also, it can't end with a
* hyphen or contain two consecutive hyphens, and can only begin with a letter, such as
* Example-App-ARN1
. For example, this value might result in the EndpointArn
value
* arn:aws:dms:eu-west-1:012345678901:rep:Example-App-ARN1
. If you don't specify a
* ResourceIdentifier
value, DMS generates a default identifier value for the end of
* EndpointArn
.
*/
public String getResourceIdentifier() {
return this.resourceIdentifier;
}
/**
*
* A friendly name for the resource identifier at the end of the EndpointArn
response parameter that is
* returned in the created Endpoint
object. The value for this parameter can have up to 31 characters.
* It can contain only ASCII letters, digits, and hyphen ('-'). Also, it can't end with a hyphen or contain two
* consecutive hyphens, and can only begin with a letter, such as Example-App-ARN1
. For example, this
* value might result in the EndpointArn
value
* arn:aws:dms:eu-west-1:012345678901:rep:Example-App-ARN1
. If you don't specify a
* ResourceIdentifier
value, DMS generates a default identifier value for the end of
* EndpointArn
.
*
*
* @param resourceIdentifier
* A friendly name for the resource identifier at the end of the EndpointArn
response parameter
* that is returned in the created Endpoint
object. The value for this parameter can have up to
* 31 characters. It can contain only ASCII letters, digits, and hyphen ('-'). Also, it can't end with a
* hyphen or contain two consecutive hyphens, and can only begin with a letter, such as
* Example-App-ARN1
. For example, this value might result in the EndpointArn
value
* arn:aws:dms:eu-west-1:012345678901:rep:Example-App-ARN1
. If you don't specify a
* ResourceIdentifier
value, DMS generates a default identifier value for the end of
* EndpointArn
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateReplicationInstanceRequest withResourceIdentifier(String resourceIdentifier) {
setResourceIdentifier(resourceIdentifier);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getReplicationInstanceIdentifier() != null)
sb.append("ReplicationInstanceIdentifier: ").append(getReplicationInstanceIdentifier()).append(",");
if (getAllocatedStorage() != null)
sb.append("AllocatedStorage: ").append(getAllocatedStorage()).append(",");
if (getReplicationInstanceClass() != null)
sb.append("ReplicationInstanceClass: ").append(getReplicationInstanceClass()).append(",");
if (getVpcSecurityGroupIds() != null)
sb.append("VpcSecurityGroupIds: ").append(getVpcSecurityGroupIds()).append(",");
if (getAvailabilityZone() != null)
sb.append("AvailabilityZone: ").append(getAvailabilityZone()).append(",");
if (getReplicationSubnetGroupIdentifier() != null)
sb.append("ReplicationSubnetGroupIdentifier: ").append(getReplicationSubnetGroupIdentifier()).append(",");
if (getPreferredMaintenanceWindow() != null)
sb.append("PreferredMaintenanceWindow: ").append(getPreferredMaintenanceWindow()).append(",");
if (getMultiAZ() != null)
sb.append("MultiAZ: ").append(getMultiAZ()).append(",");
if (getEngineVersion() != null)
sb.append("EngineVersion: ").append(getEngineVersion()).append(",");
if (getAutoMinorVersionUpgrade() != null)
sb.append("AutoMinorVersionUpgrade: ").append(getAutoMinorVersionUpgrade()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getKmsKeyId() != null)
sb.append("KmsKeyId: ").append(getKmsKeyId()).append(",");
if (getPubliclyAccessible() != null)
sb.append("PubliclyAccessible: ").append(getPubliclyAccessible()).append(",");
if (getDnsNameServers() != null)
sb.append("DnsNameServers: ").append(getDnsNameServers()).append(",");
if (getResourceIdentifier() != null)
sb.append("ResourceIdentifier: ").append(getResourceIdentifier());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateReplicationInstanceRequest == false)
return false;
CreateReplicationInstanceRequest other = (CreateReplicationInstanceRequest) obj;
if (other.getReplicationInstanceIdentifier() == null ^ this.getReplicationInstanceIdentifier() == null)
return false;
if (other.getReplicationInstanceIdentifier() != null
&& other.getReplicationInstanceIdentifier().equals(this.getReplicationInstanceIdentifier()) == false)
return false;
if (other.getAllocatedStorage() == null ^ this.getAllocatedStorage() == null)
return false;
if (other.getAllocatedStorage() != null && other.getAllocatedStorage().equals(this.getAllocatedStorage()) == false)
return false;
if (other.getReplicationInstanceClass() == null ^ this.getReplicationInstanceClass() == null)
return false;
if (other.getReplicationInstanceClass() != null && other.getReplicationInstanceClass().equals(this.getReplicationInstanceClass()) == false)
return false;
if (other.getVpcSecurityGroupIds() == null ^ this.getVpcSecurityGroupIds() == null)
return false;
if (other.getVpcSecurityGroupIds() != null && other.getVpcSecurityGroupIds().equals(this.getVpcSecurityGroupIds()) == false)
return false;
if (other.getAvailabilityZone() == null ^ this.getAvailabilityZone() == null)
return false;
if (other.getAvailabilityZone() != null && other.getAvailabilityZone().equals(this.getAvailabilityZone()) == false)
return false;
if (other.getReplicationSubnetGroupIdentifier() == null ^ this.getReplicationSubnetGroupIdentifier() == null)
return false;
if (other.getReplicationSubnetGroupIdentifier() != null
&& other.getReplicationSubnetGroupIdentifier().equals(this.getReplicationSubnetGroupIdentifier()) == false)
return false;
if (other.getPreferredMaintenanceWindow() == null ^ this.getPreferredMaintenanceWindow() == null)
return false;
if (other.getPreferredMaintenanceWindow() != null && other.getPreferredMaintenanceWindow().equals(this.getPreferredMaintenanceWindow()) == false)
return false;
if (other.getMultiAZ() == null ^ this.getMultiAZ() == null)
return false;
if (other.getMultiAZ() != null && other.getMultiAZ().equals(this.getMultiAZ()) == false)
return false;
if (other.getEngineVersion() == null ^ this.getEngineVersion() == null)
return false;
if (other.getEngineVersion() != null && other.getEngineVersion().equals(this.getEngineVersion()) == false)
return false;
if (other.getAutoMinorVersionUpgrade() == null ^ this.getAutoMinorVersionUpgrade() == null)
return false;
if (other.getAutoMinorVersionUpgrade() != null && other.getAutoMinorVersionUpgrade().equals(this.getAutoMinorVersionUpgrade()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getKmsKeyId() == null ^ this.getKmsKeyId() == null)
return false;
if (other.getKmsKeyId() != null && other.getKmsKeyId().equals(this.getKmsKeyId()) == false)
return false;
if (other.getPubliclyAccessible() == null ^ this.getPubliclyAccessible() == null)
return false;
if (other.getPubliclyAccessible() != null && other.getPubliclyAccessible().equals(this.getPubliclyAccessible()) == false)
return false;
if (other.getDnsNameServers() == null ^ this.getDnsNameServers() == null)
return false;
if (other.getDnsNameServers() != null && other.getDnsNameServers().equals(this.getDnsNameServers()) == false)
return false;
if (other.getResourceIdentifier() == null ^ this.getResourceIdentifier() == null)
return false;
if (other.getResourceIdentifier() != null && other.getResourceIdentifier().equals(this.getResourceIdentifier()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getReplicationInstanceIdentifier() == null) ? 0 : getReplicationInstanceIdentifier().hashCode());
hashCode = prime * hashCode + ((getAllocatedStorage() == null) ? 0 : getAllocatedStorage().hashCode());
hashCode = prime * hashCode + ((getReplicationInstanceClass() == null) ? 0 : getReplicationInstanceClass().hashCode());
hashCode = prime * hashCode + ((getVpcSecurityGroupIds() == null) ? 0 : getVpcSecurityGroupIds().hashCode());
hashCode = prime * hashCode + ((getAvailabilityZone() == null) ? 0 : getAvailabilityZone().hashCode());
hashCode = prime * hashCode + ((getReplicationSubnetGroupIdentifier() == null) ? 0 : getReplicationSubnetGroupIdentifier().hashCode());
hashCode = prime * hashCode + ((getPreferredMaintenanceWindow() == null) ? 0 : getPreferredMaintenanceWindow().hashCode());
hashCode = prime * hashCode + ((getMultiAZ() == null) ? 0 : getMultiAZ().hashCode());
hashCode = prime * hashCode + ((getEngineVersion() == null) ? 0 : getEngineVersion().hashCode());
hashCode = prime * hashCode + ((getAutoMinorVersionUpgrade() == null) ? 0 : getAutoMinorVersionUpgrade().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getKmsKeyId() == null) ? 0 : getKmsKeyId().hashCode());
hashCode = prime * hashCode + ((getPubliclyAccessible() == null) ? 0 : getPubliclyAccessible().hashCode());
hashCode = prime * hashCode + ((getDnsNameServers() == null) ? 0 : getDnsNameServers().hashCode());
hashCode = prime * hashCode + ((getResourceIdentifier() == null) ? 0 : getResourceIdentifier().hashCode());
return hashCode;
}
@Override
public CreateReplicationInstanceRequest clone() {
return (CreateReplicationInstanceRequest) super.clone();
}
}