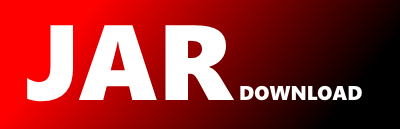
com.amazonaws.services.databasemigrationservice.model.StartReplicationTaskAssessmentRunRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-dms Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.databasemigrationservice.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class StartReplicationTaskAssessmentRunRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* Amazon Resource Name (ARN) of the migration task associated with the premigration assessment run that you want to
* start.
*
*/
private String replicationTaskArn;
/**
*
* ARN of the service role needed to start the assessment run. The role must allow the iam:PassRole
* action.
*
*/
private String serviceAccessRoleArn;
/**
*
* Amazon S3 bucket where you want DMS to store the results of this assessment run.
*
*/
private String resultLocationBucket;
/**
*
* Folder within an Amazon S3 bucket where you want DMS to store the results of this assessment run.
*
*/
private String resultLocationFolder;
/**
*
* Encryption mode that you can specify to encrypt the results of this assessment run. If you don't specify this
* request parameter, DMS stores the assessment run results without encryption. You can specify one of the options
* following:
*
*
* -
*
* "SSE_S3"
– The server-side encryption provided as a default by Amazon S3.
*
*
* -
*
* "SSE_KMS"
– Key Management Service (KMS) encryption. This encryption can use either a custom KMS
* encryption key that you specify or the default KMS encryption key that DMS provides.
*
*
*
*/
private String resultEncryptionMode;
/**
*
* ARN of a custom KMS encryption key that you specify when you set ResultEncryptionMode
to
* "SSE_KMS
".
*
*/
private String resultKmsKeyArn;
/**
*
* Unique name to identify the assessment run.
*
*/
private String assessmentRunName;
/**
*
* Space-separated list of names for specific individual assessments that you want to include. These names come from
* the default list of individual assessments that DMS supports for the associated migration task. This task is
* specified by ReplicationTaskArn
.
*
*
*
* You can't set a value for IncludeOnly
if you also set a value for Exclude
in the API
* operation.
*
*
* To identify the names of the default individual assessments that DMS supports for the associated migration task,
* run the DescribeApplicableIndividualAssessments
operation using its own
* ReplicationTaskArn
request parameter.
*
*
*/
private java.util.List includeOnly;
/**
*
* Space-separated list of names for specific individual assessments that you want to exclude. These names come from
* the default list of individual assessments that DMS supports for the associated migration task. This task is
* specified by ReplicationTaskArn
.
*
*
*
* You can't set a value for Exclude
if you also set a value for IncludeOnly
in the API
* operation.
*
*
* To identify the names of the default individual assessments that DMS supports for the associated migration task,
* run the DescribeApplicableIndividualAssessments
operation using its own
* ReplicationTaskArn
request parameter.
*
*
*/
private java.util.List exclude;
/**
*
* Amazon Resource Name (ARN) of the migration task associated with the premigration assessment run that you want to
* start.
*
*
* @param replicationTaskArn
* Amazon Resource Name (ARN) of the migration task associated with the premigration assessment run that you
* want to start.
*/
public void setReplicationTaskArn(String replicationTaskArn) {
this.replicationTaskArn = replicationTaskArn;
}
/**
*
* Amazon Resource Name (ARN) of the migration task associated with the premigration assessment run that you want to
* start.
*
*
* @return Amazon Resource Name (ARN) of the migration task associated with the premigration assessment run that you
* want to start.
*/
public String getReplicationTaskArn() {
return this.replicationTaskArn;
}
/**
*
* Amazon Resource Name (ARN) of the migration task associated with the premigration assessment run that you want to
* start.
*
*
* @param replicationTaskArn
* Amazon Resource Name (ARN) of the migration task associated with the premigration assessment run that you
* want to start.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartReplicationTaskAssessmentRunRequest withReplicationTaskArn(String replicationTaskArn) {
setReplicationTaskArn(replicationTaskArn);
return this;
}
/**
*
* ARN of the service role needed to start the assessment run. The role must allow the iam:PassRole
* action.
*
*
* @param serviceAccessRoleArn
* ARN of the service role needed to start the assessment run. The role must allow the
* iam:PassRole
action.
*/
public void setServiceAccessRoleArn(String serviceAccessRoleArn) {
this.serviceAccessRoleArn = serviceAccessRoleArn;
}
/**
*
* ARN of the service role needed to start the assessment run. The role must allow the iam:PassRole
* action.
*
*
* @return ARN of the service role needed to start the assessment run. The role must allow the
* iam:PassRole
action.
*/
public String getServiceAccessRoleArn() {
return this.serviceAccessRoleArn;
}
/**
*
* ARN of the service role needed to start the assessment run. The role must allow the iam:PassRole
* action.
*
*
* @param serviceAccessRoleArn
* ARN of the service role needed to start the assessment run. The role must allow the
* iam:PassRole
action.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartReplicationTaskAssessmentRunRequest withServiceAccessRoleArn(String serviceAccessRoleArn) {
setServiceAccessRoleArn(serviceAccessRoleArn);
return this;
}
/**
*
* Amazon S3 bucket where you want DMS to store the results of this assessment run.
*
*
* @param resultLocationBucket
* Amazon S3 bucket where you want DMS to store the results of this assessment run.
*/
public void setResultLocationBucket(String resultLocationBucket) {
this.resultLocationBucket = resultLocationBucket;
}
/**
*
* Amazon S3 bucket where you want DMS to store the results of this assessment run.
*
*
* @return Amazon S3 bucket where you want DMS to store the results of this assessment run.
*/
public String getResultLocationBucket() {
return this.resultLocationBucket;
}
/**
*
* Amazon S3 bucket where you want DMS to store the results of this assessment run.
*
*
* @param resultLocationBucket
* Amazon S3 bucket where you want DMS to store the results of this assessment run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartReplicationTaskAssessmentRunRequest withResultLocationBucket(String resultLocationBucket) {
setResultLocationBucket(resultLocationBucket);
return this;
}
/**
*
* Folder within an Amazon S3 bucket where you want DMS to store the results of this assessment run.
*
*
* @param resultLocationFolder
* Folder within an Amazon S3 bucket where you want DMS to store the results of this assessment run.
*/
public void setResultLocationFolder(String resultLocationFolder) {
this.resultLocationFolder = resultLocationFolder;
}
/**
*
* Folder within an Amazon S3 bucket where you want DMS to store the results of this assessment run.
*
*
* @return Folder within an Amazon S3 bucket where you want DMS to store the results of this assessment run.
*/
public String getResultLocationFolder() {
return this.resultLocationFolder;
}
/**
*
* Folder within an Amazon S3 bucket where you want DMS to store the results of this assessment run.
*
*
* @param resultLocationFolder
* Folder within an Amazon S3 bucket where you want DMS to store the results of this assessment run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartReplicationTaskAssessmentRunRequest withResultLocationFolder(String resultLocationFolder) {
setResultLocationFolder(resultLocationFolder);
return this;
}
/**
*
* Encryption mode that you can specify to encrypt the results of this assessment run. If you don't specify this
* request parameter, DMS stores the assessment run results without encryption. You can specify one of the options
* following:
*
*
* -
*
* "SSE_S3"
– The server-side encryption provided as a default by Amazon S3.
*
*
* -
*
* "SSE_KMS"
– Key Management Service (KMS) encryption. This encryption can use either a custom KMS
* encryption key that you specify or the default KMS encryption key that DMS provides.
*
*
*
*
* @param resultEncryptionMode
* Encryption mode that you can specify to encrypt the results of this assessment run. If you don't specify
* this request parameter, DMS stores the assessment run results without encryption. You can specify one of
* the options following:
*
* -
*
* "SSE_S3"
– The server-side encryption provided as a default by Amazon S3.
*
*
* -
*
* "SSE_KMS"
– Key Management Service (KMS) encryption. This encryption can use either a custom
* KMS encryption key that you specify or the default KMS encryption key that DMS provides.
*
*
*/
public void setResultEncryptionMode(String resultEncryptionMode) {
this.resultEncryptionMode = resultEncryptionMode;
}
/**
*
* Encryption mode that you can specify to encrypt the results of this assessment run. If you don't specify this
* request parameter, DMS stores the assessment run results without encryption. You can specify one of the options
* following:
*
*
* -
*
* "SSE_S3"
– The server-side encryption provided as a default by Amazon S3.
*
*
* -
*
* "SSE_KMS"
– Key Management Service (KMS) encryption. This encryption can use either a custom KMS
* encryption key that you specify or the default KMS encryption key that DMS provides.
*
*
*
*
* @return Encryption mode that you can specify to encrypt the results of this assessment run. If you don't specify
* this request parameter, DMS stores the assessment run results without encryption. You can specify one of
* the options following:
*
* -
*
* "SSE_S3"
– The server-side encryption provided as a default by Amazon S3.
*
*
* -
*
* "SSE_KMS"
– Key Management Service (KMS) encryption. This encryption can use either a custom
* KMS encryption key that you specify or the default KMS encryption key that DMS provides.
*
*
*/
public String getResultEncryptionMode() {
return this.resultEncryptionMode;
}
/**
*
* Encryption mode that you can specify to encrypt the results of this assessment run. If you don't specify this
* request parameter, DMS stores the assessment run results without encryption. You can specify one of the options
* following:
*
*
* -
*
* "SSE_S3"
– The server-side encryption provided as a default by Amazon S3.
*
*
* -
*
* "SSE_KMS"
– Key Management Service (KMS) encryption. This encryption can use either a custom KMS
* encryption key that you specify or the default KMS encryption key that DMS provides.
*
*
*
*
* @param resultEncryptionMode
* Encryption mode that you can specify to encrypt the results of this assessment run. If you don't specify
* this request parameter, DMS stores the assessment run results without encryption. You can specify one of
* the options following:
*
* -
*
* "SSE_S3"
– The server-side encryption provided as a default by Amazon S3.
*
*
* -
*
* "SSE_KMS"
– Key Management Service (KMS) encryption. This encryption can use either a custom
* KMS encryption key that you specify or the default KMS encryption key that DMS provides.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartReplicationTaskAssessmentRunRequest withResultEncryptionMode(String resultEncryptionMode) {
setResultEncryptionMode(resultEncryptionMode);
return this;
}
/**
*
* ARN of a custom KMS encryption key that you specify when you set ResultEncryptionMode
to
* "SSE_KMS
".
*
*
* @param resultKmsKeyArn
* ARN of a custom KMS encryption key that you specify when you set ResultEncryptionMode
to
* "SSE_KMS
".
*/
public void setResultKmsKeyArn(String resultKmsKeyArn) {
this.resultKmsKeyArn = resultKmsKeyArn;
}
/**
*
* ARN of a custom KMS encryption key that you specify when you set ResultEncryptionMode
to
* "SSE_KMS
".
*
*
* @return ARN of a custom KMS encryption key that you specify when you set ResultEncryptionMode
to
* "SSE_KMS
".
*/
public String getResultKmsKeyArn() {
return this.resultKmsKeyArn;
}
/**
*
* ARN of a custom KMS encryption key that you specify when you set ResultEncryptionMode
to
* "SSE_KMS
".
*
*
* @param resultKmsKeyArn
* ARN of a custom KMS encryption key that you specify when you set ResultEncryptionMode
to
* "SSE_KMS
".
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartReplicationTaskAssessmentRunRequest withResultKmsKeyArn(String resultKmsKeyArn) {
setResultKmsKeyArn(resultKmsKeyArn);
return this;
}
/**
*
* Unique name to identify the assessment run.
*
*
* @param assessmentRunName
* Unique name to identify the assessment run.
*/
public void setAssessmentRunName(String assessmentRunName) {
this.assessmentRunName = assessmentRunName;
}
/**
*
* Unique name to identify the assessment run.
*
*
* @return Unique name to identify the assessment run.
*/
public String getAssessmentRunName() {
return this.assessmentRunName;
}
/**
*
* Unique name to identify the assessment run.
*
*
* @param assessmentRunName
* Unique name to identify the assessment run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartReplicationTaskAssessmentRunRequest withAssessmentRunName(String assessmentRunName) {
setAssessmentRunName(assessmentRunName);
return this;
}
/**
*
* Space-separated list of names for specific individual assessments that you want to include. These names come from
* the default list of individual assessments that DMS supports for the associated migration task. This task is
* specified by ReplicationTaskArn
.
*
*
*
* You can't set a value for IncludeOnly
if you also set a value for Exclude
in the API
* operation.
*
*
* To identify the names of the default individual assessments that DMS supports for the associated migration task,
* run the DescribeApplicableIndividualAssessments
operation using its own
* ReplicationTaskArn
request parameter.
*
*
*
* @return Space-separated list of names for specific individual assessments that you want to include. These names
* come from the default list of individual assessments that DMS supports for the associated migration task.
* This task is specified by ReplicationTaskArn
.
*
* You can't set a value for IncludeOnly
if you also set a value for Exclude
in
* the API operation.
*
*
* To identify the names of the default individual assessments that DMS supports for the associated
* migration task, run the DescribeApplicableIndividualAssessments
operation using its own
* ReplicationTaskArn
request parameter.
*
*/
public java.util.List getIncludeOnly() {
return includeOnly;
}
/**
*
* Space-separated list of names for specific individual assessments that you want to include. These names come from
* the default list of individual assessments that DMS supports for the associated migration task. This task is
* specified by ReplicationTaskArn
.
*
*
*
* You can't set a value for IncludeOnly
if you also set a value for Exclude
in the API
* operation.
*
*
* To identify the names of the default individual assessments that DMS supports for the associated migration task,
* run the DescribeApplicableIndividualAssessments
operation using its own
* ReplicationTaskArn
request parameter.
*
*
*
* @param includeOnly
* Space-separated list of names for specific individual assessments that you want to include. These names
* come from the default list of individual assessments that DMS supports for the associated migration task.
* This task is specified by ReplicationTaskArn
.
*
* You can't set a value for IncludeOnly
if you also set a value for Exclude
in the
* API operation.
*
*
* To identify the names of the default individual assessments that DMS supports for the associated migration
* task, run the DescribeApplicableIndividualAssessments
operation using its own
* ReplicationTaskArn
request parameter.
*
*/
public void setIncludeOnly(java.util.Collection includeOnly) {
if (includeOnly == null) {
this.includeOnly = null;
return;
}
this.includeOnly = new java.util.ArrayList(includeOnly);
}
/**
*
* Space-separated list of names for specific individual assessments that you want to include. These names come from
* the default list of individual assessments that DMS supports for the associated migration task. This task is
* specified by ReplicationTaskArn
.
*
*
*
* You can't set a value for IncludeOnly
if you also set a value for Exclude
in the API
* operation.
*
*
* To identify the names of the default individual assessments that DMS supports for the associated migration task,
* run the DescribeApplicableIndividualAssessments
operation using its own
* ReplicationTaskArn
request parameter.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setIncludeOnly(java.util.Collection)} or {@link #withIncludeOnly(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param includeOnly
* Space-separated list of names for specific individual assessments that you want to include. These names
* come from the default list of individual assessments that DMS supports for the associated migration task.
* This task is specified by ReplicationTaskArn
.
*
* You can't set a value for IncludeOnly
if you also set a value for Exclude
in the
* API operation.
*
*
* To identify the names of the default individual assessments that DMS supports for the associated migration
* task, run the DescribeApplicableIndividualAssessments
operation using its own
* ReplicationTaskArn
request parameter.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartReplicationTaskAssessmentRunRequest withIncludeOnly(String... includeOnly) {
if (this.includeOnly == null) {
setIncludeOnly(new java.util.ArrayList(includeOnly.length));
}
for (String ele : includeOnly) {
this.includeOnly.add(ele);
}
return this;
}
/**
*
* Space-separated list of names for specific individual assessments that you want to include. These names come from
* the default list of individual assessments that DMS supports for the associated migration task. This task is
* specified by ReplicationTaskArn
.
*
*
*
* You can't set a value for IncludeOnly
if you also set a value for Exclude
in the API
* operation.
*
*
* To identify the names of the default individual assessments that DMS supports for the associated migration task,
* run the DescribeApplicableIndividualAssessments
operation using its own
* ReplicationTaskArn
request parameter.
*
*
*
* @param includeOnly
* Space-separated list of names for specific individual assessments that you want to include. These names
* come from the default list of individual assessments that DMS supports for the associated migration task.
* This task is specified by ReplicationTaskArn
.
*
* You can't set a value for IncludeOnly
if you also set a value for Exclude
in the
* API operation.
*
*
* To identify the names of the default individual assessments that DMS supports for the associated migration
* task, run the DescribeApplicableIndividualAssessments
operation using its own
* ReplicationTaskArn
request parameter.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartReplicationTaskAssessmentRunRequest withIncludeOnly(java.util.Collection includeOnly) {
setIncludeOnly(includeOnly);
return this;
}
/**
*
* Space-separated list of names for specific individual assessments that you want to exclude. These names come from
* the default list of individual assessments that DMS supports for the associated migration task. This task is
* specified by ReplicationTaskArn
.
*
*
*
* You can't set a value for Exclude
if you also set a value for IncludeOnly
in the API
* operation.
*
*
* To identify the names of the default individual assessments that DMS supports for the associated migration task,
* run the DescribeApplicableIndividualAssessments
operation using its own
* ReplicationTaskArn
request parameter.
*
*
*
* @return Space-separated list of names for specific individual assessments that you want to exclude. These names
* come from the default list of individual assessments that DMS supports for the associated migration task.
* This task is specified by ReplicationTaskArn
.
*
* You can't set a value for Exclude
if you also set a value for IncludeOnly
in
* the API operation.
*
*
* To identify the names of the default individual assessments that DMS supports for the associated
* migration task, run the DescribeApplicableIndividualAssessments
operation using its own
* ReplicationTaskArn
request parameter.
*
*/
public java.util.List getExclude() {
return exclude;
}
/**
*
* Space-separated list of names for specific individual assessments that you want to exclude. These names come from
* the default list of individual assessments that DMS supports for the associated migration task. This task is
* specified by ReplicationTaskArn
.
*
*
*
* You can't set a value for Exclude
if you also set a value for IncludeOnly
in the API
* operation.
*
*
* To identify the names of the default individual assessments that DMS supports for the associated migration task,
* run the DescribeApplicableIndividualAssessments
operation using its own
* ReplicationTaskArn
request parameter.
*
*
*
* @param exclude
* Space-separated list of names for specific individual assessments that you want to exclude. These names
* come from the default list of individual assessments that DMS supports for the associated migration task.
* This task is specified by ReplicationTaskArn
.
*
* You can't set a value for Exclude
if you also set a value for IncludeOnly
in the
* API operation.
*
*
* To identify the names of the default individual assessments that DMS supports for the associated migration
* task, run the DescribeApplicableIndividualAssessments
operation using its own
* ReplicationTaskArn
request parameter.
*
*/
public void setExclude(java.util.Collection exclude) {
if (exclude == null) {
this.exclude = null;
return;
}
this.exclude = new java.util.ArrayList(exclude);
}
/**
*
* Space-separated list of names for specific individual assessments that you want to exclude. These names come from
* the default list of individual assessments that DMS supports for the associated migration task. This task is
* specified by ReplicationTaskArn
.
*
*
*
* You can't set a value for Exclude
if you also set a value for IncludeOnly
in the API
* operation.
*
*
* To identify the names of the default individual assessments that DMS supports for the associated migration task,
* run the DescribeApplicableIndividualAssessments
operation using its own
* ReplicationTaskArn
request parameter.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setExclude(java.util.Collection)} or {@link #withExclude(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param exclude
* Space-separated list of names for specific individual assessments that you want to exclude. These names
* come from the default list of individual assessments that DMS supports for the associated migration task.
* This task is specified by ReplicationTaskArn
.
*
* You can't set a value for Exclude
if you also set a value for IncludeOnly
in the
* API operation.
*
*
* To identify the names of the default individual assessments that DMS supports for the associated migration
* task, run the DescribeApplicableIndividualAssessments
operation using its own
* ReplicationTaskArn
request parameter.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartReplicationTaskAssessmentRunRequest withExclude(String... exclude) {
if (this.exclude == null) {
setExclude(new java.util.ArrayList(exclude.length));
}
for (String ele : exclude) {
this.exclude.add(ele);
}
return this;
}
/**
*
* Space-separated list of names for specific individual assessments that you want to exclude. These names come from
* the default list of individual assessments that DMS supports for the associated migration task. This task is
* specified by ReplicationTaskArn
.
*
*
*
* You can't set a value for Exclude
if you also set a value for IncludeOnly
in the API
* operation.
*
*
* To identify the names of the default individual assessments that DMS supports for the associated migration task,
* run the DescribeApplicableIndividualAssessments
operation using its own
* ReplicationTaskArn
request parameter.
*
*
*
* @param exclude
* Space-separated list of names for specific individual assessments that you want to exclude. These names
* come from the default list of individual assessments that DMS supports for the associated migration task.
* This task is specified by ReplicationTaskArn
.
*
* You can't set a value for Exclude
if you also set a value for IncludeOnly
in the
* API operation.
*
*
* To identify the names of the default individual assessments that DMS supports for the associated migration
* task, run the DescribeApplicableIndividualAssessments
operation using its own
* ReplicationTaskArn
request parameter.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StartReplicationTaskAssessmentRunRequest withExclude(java.util.Collection exclude) {
setExclude(exclude);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getReplicationTaskArn() != null)
sb.append("ReplicationTaskArn: ").append(getReplicationTaskArn()).append(",");
if (getServiceAccessRoleArn() != null)
sb.append("ServiceAccessRoleArn: ").append(getServiceAccessRoleArn()).append(",");
if (getResultLocationBucket() != null)
sb.append("ResultLocationBucket: ").append(getResultLocationBucket()).append(",");
if (getResultLocationFolder() != null)
sb.append("ResultLocationFolder: ").append(getResultLocationFolder()).append(",");
if (getResultEncryptionMode() != null)
sb.append("ResultEncryptionMode: ").append(getResultEncryptionMode()).append(",");
if (getResultKmsKeyArn() != null)
sb.append("ResultKmsKeyArn: ").append(getResultKmsKeyArn()).append(",");
if (getAssessmentRunName() != null)
sb.append("AssessmentRunName: ").append(getAssessmentRunName()).append(",");
if (getIncludeOnly() != null)
sb.append("IncludeOnly: ").append(getIncludeOnly()).append(",");
if (getExclude() != null)
sb.append("Exclude: ").append(getExclude());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof StartReplicationTaskAssessmentRunRequest == false)
return false;
StartReplicationTaskAssessmentRunRequest other = (StartReplicationTaskAssessmentRunRequest) obj;
if (other.getReplicationTaskArn() == null ^ this.getReplicationTaskArn() == null)
return false;
if (other.getReplicationTaskArn() != null && other.getReplicationTaskArn().equals(this.getReplicationTaskArn()) == false)
return false;
if (other.getServiceAccessRoleArn() == null ^ this.getServiceAccessRoleArn() == null)
return false;
if (other.getServiceAccessRoleArn() != null && other.getServiceAccessRoleArn().equals(this.getServiceAccessRoleArn()) == false)
return false;
if (other.getResultLocationBucket() == null ^ this.getResultLocationBucket() == null)
return false;
if (other.getResultLocationBucket() != null && other.getResultLocationBucket().equals(this.getResultLocationBucket()) == false)
return false;
if (other.getResultLocationFolder() == null ^ this.getResultLocationFolder() == null)
return false;
if (other.getResultLocationFolder() != null && other.getResultLocationFolder().equals(this.getResultLocationFolder()) == false)
return false;
if (other.getResultEncryptionMode() == null ^ this.getResultEncryptionMode() == null)
return false;
if (other.getResultEncryptionMode() != null && other.getResultEncryptionMode().equals(this.getResultEncryptionMode()) == false)
return false;
if (other.getResultKmsKeyArn() == null ^ this.getResultKmsKeyArn() == null)
return false;
if (other.getResultKmsKeyArn() != null && other.getResultKmsKeyArn().equals(this.getResultKmsKeyArn()) == false)
return false;
if (other.getAssessmentRunName() == null ^ this.getAssessmentRunName() == null)
return false;
if (other.getAssessmentRunName() != null && other.getAssessmentRunName().equals(this.getAssessmentRunName()) == false)
return false;
if (other.getIncludeOnly() == null ^ this.getIncludeOnly() == null)
return false;
if (other.getIncludeOnly() != null && other.getIncludeOnly().equals(this.getIncludeOnly()) == false)
return false;
if (other.getExclude() == null ^ this.getExclude() == null)
return false;
if (other.getExclude() != null && other.getExclude().equals(this.getExclude()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getReplicationTaskArn() == null) ? 0 : getReplicationTaskArn().hashCode());
hashCode = prime * hashCode + ((getServiceAccessRoleArn() == null) ? 0 : getServiceAccessRoleArn().hashCode());
hashCode = prime * hashCode + ((getResultLocationBucket() == null) ? 0 : getResultLocationBucket().hashCode());
hashCode = prime * hashCode + ((getResultLocationFolder() == null) ? 0 : getResultLocationFolder().hashCode());
hashCode = prime * hashCode + ((getResultEncryptionMode() == null) ? 0 : getResultEncryptionMode().hashCode());
hashCode = prime * hashCode + ((getResultKmsKeyArn() == null) ? 0 : getResultKmsKeyArn().hashCode());
hashCode = prime * hashCode + ((getAssessmentRunName() == null) ? 0 : getAssessmentRunName().hashCode());
hashCode = prime * hashCode + ((getIncludeOnly() == null) ? 0 : getIncludeOnly().hashCode());
hashCode = prime * hashCode + ((getExclude() == null) ? 0 : getExclude().hashCode());
return hashCode;
}
@Override
public StartReplicationTaskAssessmentRunRequest clone() {
return (StartReplicationTaskAssessmentRunRequest) super.clone();
}
}