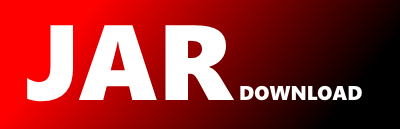
com.amazonaws.services.databasemigrationservice.model.NeptuneSettings Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.databasemigrationservice.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Provides information that defines an Amazon Neptune endpoint.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class NeptuneSettings implements Serializable, Cloneable, StructuredPojo {
/**
*
* The Amazon Resource Name (ARN) of the service role that you created for the Neptune target endpoint. The role
* must allow the iam:PassRole
action. For more information, see Creating an IAM Service Role for Accessing Amazon Neptune as a Target in the Database Migration Service
* User Guide.
*
*/
private String serviceAccessRoleArn;
/**
*
* The name of the Amazon S3 bucket where DMS can temporarily store migrated graph data in .csv files before
* bulk-loading it to the Neptune target database. DMS maps the SQL source data to graph data before storing it in
* these .csv files.
*
*/
private String s3BucketName;
/**
*
* A folder path where you want DMS to store migrated graph data in the S3 bucket specified by
* S3BucketName
*
*/
private String s3BucketFolder;
/**
*
* The number of milliseconds for DMS to wait to retry a bulk-load of migrated graph data to the Neptune target
* database before raising an error. The default is 250.
*
*/
private Integer errorRetryDuration;
/**
*
* The maximum size in kilobytes of migrated graph data stored in a .csv file before DMS bulk-loads the data to the
* Neptune target database. The default is 1,048,576 KB. If the bulk load is successful, DMS clears the bucket,
* ready to store the next batch of migrated graph data.
*
*/
private Integer maxFileSize;
/**
*
* The number of times for DMS to retry a bulk load of migrated graph data to the Neptune target database before
* raising an error. The default is 5.
*
*/
private Integer maxRetryCount;
/**
*
* If you want Identity and Access Management (IAM) authorization enabled for this endpoint, set this parameter to
* true
. Then attach the appropriate IAM policy document to your service role specified by
* ServiceAccessRoleArn
. The default is false
.
*
*/
private Boolean iamAuthEnabled;
/**
*
* The Amazon Resource Name (ARN) of the service role that you created for the Neptune target endpoint. The role
* must allow the iam:PassRole
action. For more information, see Creating an IAM Service Role for Accessing Amazon Neptune as a Target in the Database Migration Service
* User Guide.
*
*
* @param serviceAccessRoleArn
* The Amazon Resource Name (ARN) of the service role that you created for the Neptune target endpoint. The
* role must allow the iam:PassRole
action. For more information, see Creating an IAM Service Role for Accessing Amazon Neptune as a Target in the Database Migration
* Service User Guide.
*/
public void setServiceAccessRoleArn(String serviceAccessRoleArn) {
this.serviceAccessRoleArn = serviceAccessRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the service role that you created for the Neptune target endpoint. The role
* must allow the iam:PassRole
action. For more information, see Creating an IAM Service Role for Accessing Amazon Neptune as a Target in the Database Migration Service
* User Guide.
*
*
* @return The Amazon Resource Name (ARN) of the service role that you created for the Neptune target endpoint. The
* role must allow the iam:PassRole
action. For more information, see Creating an IAM Service Role for Accessing Amazon Neptune as a Target in the Database Migration
* Service User Guide.
*/
public String getServiceAccessRoleArn() {
return this.serviceAccessRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the service role that you created for the Neptune target endpoint. The role
* must allow the iam:PassRole
action. For more information, see Creating an IAM Service Role for Accessing Amazon Neptune as a Target in the Database Migration Service
* User Guide.
*
*
* @param serviceAccessRoleArn
* The Amazon Resource Name (ARN) of the service role that you created for the Neptune target endpoint. The
* role must allow the iam:PassRole
action. For more information, see Creating an IAM Service Role for Accessing Amazon Neptune as a Target in the Database Migration
* Service User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NeptuneSettings withServiceAccessRoleArn(String serviceAccessRoleArn) {
setServiceAccessRoleArn(serviceAccessRoleArn);
return this;
}
/**
*
* The name of the Amazon S3 bucket where DMS can temporarily store migrated graph data in .csv files before
* bulk-loading it to the Neptune target database. DMS maps the SQL source data to graph data before storing it in
* these .csv files.
*
*
* @param s3BucketName
* The name of the Amazon S3 bucket where DMS can temporarily store migrated graph data in .csv files before
* bulk-loading it to the Neptune target database. DMS maps the SQL source data to graph data before storing
* it in these .csv files.
*/
public void setS3BucketName(String s3BucketName) {
this.s3BucketName = s3BucketName;
}
/**
*
* The name of the Amazon S3 bucket where DMS can temporarily store migrated graph data in .csv files before
* bulk-loading it to the Neptune target database. DMS maps the SQL source data to graph data before storing it in
* these .csv files.
*
*
* @return The name of the Amazon S3 bucket where DMS can temporarily store migrated graph data in .csv files before
* bulk-loading it to the Neptune target database. DMS maps the SQL source data to graph data before storing
* it in these .csv files.
*/
public String getS3BucketName() {
return this.s3BucketName;
}
/**
*
* The name of the Amazon S3 bucket where DMS can temporarily store migrated graph data in .csv files before
* bulk-loading it to the Neptune target database. DMS maps the SQL source data to graph data before storing it in
* these .csv files.
*
*
* @param s3BucketName
* The name of the Amazon S3 bucket where DMS can temporarily store migrated graph data in .csv files before
* bulk-loading it to the Neptune target database. DMS maps the SQL source data to graph data before storing
* it in these .csv files.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NeptuneSettings withS3BucketName(String s3BucketName) {
setS3BucketName(s3BucketName);
return this;
}
/**
*
* A folder path where you want DMS to store migrated graph data in the S3 bucket specified by
* S3BucketName
*
*
* @param s3BucketFolder
* A folder path where you want DMS to store migrated graph data in the S3 bucket specified by
* S3BucketName
*/
public void setS3BucketFolder(String s3BucketFolder) {
this.s3BucketFolder = s3BucketFolder;
}
/**
*
* A folder path where you want DMS to store migrated graph data in the S3 bucket specified by
* S3BucketName
*
*
* @return A folder path where you want DMS to store migrated graph data in the S3 bucket specified by
* S3BucketName
*/
public String getS3BucketFolder() {
return this.s3BucketFolder;
}
/**
*
* A folder path where you want DMS to store migrated graph data in the S3 bucket specified by
* S3BucketName
*
*
* @param s3BucketFolder
* A folder path where you want DMS to store migrated graph data in the S3 bucket specified by
* S3BucketName
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NeptuneSettings withS3BucketFolder(String s3BucketFolder) {
setS3BucketFolder(s3BucketFolder);
return this;
}
/**
*
* The number of milliseconds for DMS to wait to retry a bulk-load of migrated graph data to the Neptune target
* database before raising an error. The default is 250.
*
*
* @param errorRetryDuration
* The number of milliseconds for DMS to wait to retry a bulk-load of migrated graph data to the Neptune
* target database before raising an error. The default is 250.
*/
public void setErrorRetryDuration(Integer errorRetryDuration) {
this.errorRetryDuration = errorRetryDuration;
}
/**
*
* The number of milliseconds for DMS to wait to retry a bulk-load of migrated graph data to the Neptune target
* database before raising an error. The default is 250.
*
*
* @return The number of milliseconds for DMS to wait to retry a bulk-load of migrated graph data to the Neptune
* target database before raising an error. The default is 250.
*/
public Integer getErrorRetryDuration() {
return this.errorRetryDuration;
}
/**
*
* The number of milliseconds for DMS to wait to retry a bulk-load of migrated graph data to the Neptune target
* database before raising an error. The default is 250.
*
*
* @param errorRetryDuration
* The number of milliseconds for DMS to wait to retry a bulk-load of migrated graph data to the Neptune
* target database before raising an error. The default is 250.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NeptuneSettings withErrorRetryDuration(Integer errorRetryDuration) {
setErrorRetryDuration(errorRetryDuration);
return this;
}
/**
*
* The maximum size in kilobytes of migrated graph data stored in a .csv file before DMS bulk-loads the data to the
* Neptune target database. The default is 1,048,576 KB. If the bulk load is successful, DMS clears the bucket,
* ready to store the next batch of migrated graph data.
*
*
* @param maxFileSize
* The maximum size in kilobytes of migrated graph data stored in a .csv file before DMS bulk-loads the data
* to the Neptune target database. The default is 1,048,576 KB. If the bulk load is successful, DMS clears
* the bucket, ready to store the next batch of migrated graph data.
*/
public void setMaxFileSize(Integer maxFileSize) {
this.maxFileSize = maxFileSize;
}
/**
*
* The maximum size in kilobytes of migrated graph data stored in a .csv file before DMS bulk-loads the data to the
* Neptune target database. The default is 1,048,576 KB. If the bulk load is successful, DMS clears the bucket,
* ready to store the next batch of migrated graph data.
*
*
* @return The maximum size in kilobytes of migrated graph data stored in a .csv file before DMS bulk-loads the data
* to the Neptune target database. The default is 1,048,576 KB. If the bulk load is successful, DMS clears
* the bucket, ready to store the next batch of migrated graph data.
*/
public Integer getMaxFileSize() {
return this.maxFileSize;
}
/**
*
* The maximum size in kilobytes of migrated graph data stored in a .csv file before DMS bulk-loads the data to the
* Neptune target database. The default is 1,048,576 KB. If the bulk load is successful, DMS clears the bucket,
* ready to store the next batch of migrated graph data.
*
*
* @param maxFileSize
* The maximum size in kilobytes of migrated graph data stored in a .csv file before DMS bulk-loads the data
* to the Neptune target database. The default is 1,048,576 KB. If the bulk load is successful, DMS clears
* the bucket, ready to store the next batch of migrated graph data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NeptuneSettings withMaxFileSize(Integer maxFileSize) {
setMaxFileSize(maxFileSize);
return this;
}
/**
*
* The number of times for DMS to retry a bulk load of migrated graph data to the Neptune target database before
* raising an error. The default is 5.
*
*
* @param maxRetryCount
* The number of times for DMS to retry a bulk load of migrated graph data to the Neptune target database
* before raising an error. The default is 5.
*/
public void setMaxRetryCount(Integer maxRetryCount) {
this.maxRetryCount = maxRetryCount;
}
/**
*
* The number of times for DMS to retry a bulk load of migrated graph data to the Neptune target database before
* raising an error. The default is 5.
*
*
* @return The number of times for DMS to retry a bulk load of migrated graph data to the Neptune target database
* before raising an error. The default is 5.
*/
public Integer getMaxRetryCount() {
return this.maxRetryCount;
}
/**
*
* The number of times for DMS to retry a bulk load of migrated graph data to the Neptune target database before
* raising an error. The default is 5.
*
*
* @param maxRetryCount
* The number of times for DMS to retry a bulk load of migrated graph data to the Neptune target database
* before raising an error. The default is 5.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NeptuneSettings withMaxRetryCount(Integer maxRetryCount) {
setMaxRetryCount(maxRetryCount);
return this;
}
/**
*
* If you want Identity and Access Management (IAM) authorization enabled for this endpoint, set this parameter to
* true
. Then attach the appropriate IAM policy document to your service role specified by
* ServiceAccessRoleArn
. The default is false
.
*
*
* @param iamAuthEnabled
* If you want Identity and Access Management (IAM) authorization enabled for this endpoint, set this
* parameter to true
. Then attach the appropriate IAM policy document to your service role
* specified by ServiceAccessRoleArn
. The default is false
.
*/
public void setIamAuthEnabled(Boolean iamAuthEnabled) {
this.iamAuthEnabled = iamAuthEnabled;
}
/**
*
* If you want Identity and Access Management (IAM) authorization enabled for this endpoint, set this parameter to
* true
. Then attach the appropriate IAM policy document to your service role specified by
* ServiceAccessRoleArn
. The default is false
.
*
*
* @return If you want Identity and Access Management (IAM) authorization enabled for this endpoint, set this
* parameter to true
. Then attach the appropriate IAM policy document to your service role
* specified by ServiceAccessRoleArn
. The default is false
.
*/
public Boolean getIamAuthEnabled() {
return this.iamAuthEnabled;
}
/**
*
* If you want Identity and Access Management (IAM) authorization enabled for this endpoint, set this parameter to
* true
. Then attach the appropriate IAM policy document to your service role specified by
* ServiceAccessRoleArn
. The default is false
.
*
*
* @param iamAuthEnabled
* If you want Identity and Access Management (IAM) authorization enabled for this endpoint, set this
* parameter to true
. Then attach the appropriate IAM policy document to your service role
* specified by ServiceAccessRoleArn
. The default is false
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public NeptuneSettings withIamAuthEnabled(Boolean iamAuthEnabled) {
setIamAuthEnabled(iamAuthEnabled);
return this;
}
/**
*
* If you want Identity and Access Management (IAM) authorization enabled for this endpoint, set this parameter to
* true
. Then attach the appropriate IAM policy document to your service role specified by
* ServiceAccessRoleArn
. The default is false
.
*
*
* @return If you want Identity and Access Management (IAM) authorization enabled for this endpoint, set this
* parameter to true
. Then attach the appropriate IAM policy document to your service role
* specified by ServiceAccessRoleArn
. The default is false
.
*/
public Boolean isIamAuthEnabled() {
return this.iamAuthEnabled;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getServiceAccessRoleArn() != null)
sb.append("ServiceAccessRoleArn: ").append(getServiceAccessRoleArn()).append(",");
if (getS3BucketName() != null)
sb.append("S3BucketName: ").append(getS3BucketName()).append(",");
if (getS3BucketFolder() != null)
sb.append("S3BucketFolder: ").append(getS3BucketFolder()).append(",");
if (getErrorRetryDuration() != null)
sb.append("ErrorRetryDuration: ").append(getErrorRetryDuration()).append(",");
if (getMaxFileSize() != null)
sb.append("MaxFileSize: ").append(getMaxFileSize()).append(",");
if (getMaxRetryCount() != null)
sb.append("MaxRetryCount: ").append(getMaxRetryCount()).append(",");
if (getIamAuthEnabled() != null)
sb.append("IamAuthEnabled: ").append(getIamAuthEnabled());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof NeptuneSettings == false)
return false;
NeptuneSettings other = (NeptuneSettings) obj;
if (other.getServiceAccessRoleArn() == null ^ this.getServiceAccessRoleArn() == null)
return false;
if (other.getServiceAccessRoleArn() != null && other.getServiceAccessRoleArn().equals(this.getServiceAccessRoleArn()) == false)
return false;
if (other.getS3BucketName() == null ^ this.getS3BucketName() == null)
return false;
if (other.getS3BucketName() != null && other.getS3BucketName().equals(this.getS3BucketName()) == false)
return false;
if (other.getS3BucketFolder() == null ^ this.getS3BucketFolder() == null)
return false;
if (other.getS3BucketFolder() != null && other.getS3BucketFolder().equals(this.getS3BucketFolder()) == false)
return false;
if (other.getErrorRetryDuration() == null ^ this.getErrorRetryDuration() == null)
return false;
if (other.getErrorRetryDuration() != null && other.getErrorRetryDuration().equals(this.getErrorRetryDuration()) == false)
return false;
if (other.getMaxFileSize() == null ^ this.getMaxFileSize() == null)
return false;
if (other.getMaxFileSize() != null && other.getMaxFileSize().equals(this.getMaxFileSize()) == false)
return false;
if (other.getMaxRetryCount() == null ^ this.getMaxRetryCount() == null)
return false;
if (other.getMaxRetryCount() != null && other.getMaxRetryCount().equals(this.getMaxRetryCount()) == false)
return false;
if (other.getIamAuthEnabled() == null ^ this.getIamAuthEnabled() == null)
return false;
if (other.getIamAuthEnabled() != null && other.getIamAuthEnabled().equals(this.getIamAuthEnabled()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getServiceAccessRoleArn() == null) ? 0 : getServiceAccessRoleArn().hashCode());
hashCode = prime * hashCode + ((getS3BucketName() == null) ? 0 : getS3BucketName().hashCode());
hashCode = prime * hashCode + ((getS3BucketFolder() == null) ? 0 : getS3BucketFolder().hashCode());
hashCode = prime * hashCode + ((getErrorRetryDuration() == null) ? 0 : getErrorRetryDuration().hashCode());
hashCode = prime * hashCode + ((getMaxFileSize() == null) ? 0 : getMaxFileSize().hashCode());
hashCode = prime * hashCode + ((getMaxRetryCount() == null) ? 0 : getMaxRetryCount().hashCode());
hashCode = prime * hashCode + ((getIamAuthEnabled() == null) ? 0 : getIamAuthEnabled().hashCode());
return hashCode;
}
@Override
public NeptuneSettings clone() {
try {
return (NeptuneSettings) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.databasemigrationservice.model.transform.NeptuneSettingsMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}