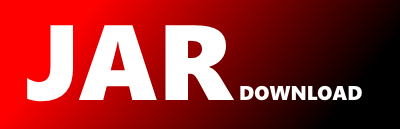
com.amazonaws.services.databasemigrationservice.model.Replication Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.databasemigrationservice.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Provides information that describes a serverless replication created by the CreateReplication
operation.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Replication implements Serializable, Cloneable, StructuredPojo {
/**
*
* The identifier for the ReplicationConfig
associated with the replication.
*
*/
private String replicationConfigIdentifier;
/**
*
* The Amazon Resource Name for the ReplicationConfig
associated with the replication.
*
*/
private String replicationConfigArn;
/**
*
* The Amazon Resource Name for an existing Endpoint
the serverless replication uses for its data
* source.
*
*/
private String sourceEndpointArn;
/**
*
* The Amazon Resource Name for an existing Endpoint
the serverless replication uses for its data
* target.
*
*/
private String targetEndpointArn;
/**
*
* The type of the serverless replication.
*
*/
private String replicationType;
/**
*
* The current status of the serverless replication.
*
*/
private String status;
/**
*
* Information about provisioning resources for an DMS serverless replication.
*
*/
private ProvisionData provisionData;
/**
*
* The reason the replication task was stopped. This response parameter can return one of the following values:
*
*
* -
*
* "Stop Reason NORMAL"
*
*
* -
*
* "Stop Reason RECOVERABLE_ERROR"
*
*
* -
*
* "Stop Reason FATAL_ERROR"
*
*
* -
*
* "Stop Reason FULL_LOAD_ONLY_FINISHED"
*
*
* -
*
* "Stop Reason STOPPED_AFTER_FULL_LOAD"
– Full load completed, with cached changes not applied
*
*
* -
*
* "Stop Reason STOPPED_AFTER_CACHED_EVENTS"
– Full load completed, with cached changes applied
*
*
* -
*
* "Stop Reason EXPRESS_LICENSE_LIMITS_REACHED"
*
*
* -
*
* "Stop Reason STOPPED_AFTER_DDL_APPLY"
– User-defined stop task after DDL applied
*
*
* -
*
* "Stop Reason STOPPED_DUE_TO_LOW_MEMORY"
*
*
* -
*
* "Stop Reason STOPPED_DUE_TO_LOW_DISK"
*
*
* -
*
* "Stop Reason STOPPED_AT_SERVER_TIME"
– User-defined server time for stopping task
*
*
* -
*
* "Stop Reason STOPPED_AT_COMMIT_TIME"
– User-defined commit time for stopping task
*
*
* -
*
* "Stop Reason RECONFIGURATION_RESTART"
*
*
* -
*
* "Stop Reason RECYCLE_TASK"
*
*
*
*/
private String stopReason;
/**
*
* Error and other information about why a serverless replication failed.
*
*/
private java.util.List failureMessages;
/**
*
* This object provides a collection of statistics about a serverless replication.
*
*/
private ReplicationStats replicationStats;
/**
*
* The replication type.
*
*/
private String startReplicationType;
/**
*
* Indicates the start time for a change data capture (CDC) operation. Use either CdcStartTime
or
* CdcStartPosition
to specify when you want a CDC operation to start. Specifying both values results
* in an error.
*
*/
private java.util.Date cdcStartTime;
/**
*
* Indicates the start time for a change data capture (CDC) operation. Use either CdcStartTime
or
* CdcStartPosition
to specify when you want a CDC operation to start. Specifying both values results
* in an error.
*
*/
private String cdcStartPosition;
/**
*
* Indicates when you want a change data capture (CDC) operation to stop. The value can be either server time or
* commit time.
*
*/
private String cdcStopPosition;
/**
*
* Indicates the last checkpoint that occurred during a change data capture (CDC) operation. You can provide this
* value to the CdcStartPosition
parameter to start a CDC operation that begins at that checkpoint.
*
*/
private String recoveryCheckpoint;
/**
*
* The time the serverless replication was created.
*
*/
private java.util.Date replicationCreateTime;
/**
*
* The time the serverless replication was updated.
*
*/
private java.util.Date replicationUpdateTime;
/**
*
* The timestamp when replication was last stopped.
*
*/
private java.util.Date replicationLastStopTime;
/**
*
* The timestamp when DMS will deprovision the replication.
*
*/
private java.util.Date replicationDeprovisionTime;
/**
*
* The identifier for the ReplicationConfig
associated with the replication.
*
*
* @param replicationConfigIdentifier
* The identifier for the ReplicationConfig
associated with the replication.
*/
public void setReplicationConfigIdentifier(String replicationConfigIdentifier) {
this.replicationConfigIdentifier = replicationConfigIdentifier;
}
/**
*
* The identifier for the ReplicationConfig
associated with the replication.
*
*
* @return The identifier for the ReplicationConfig
associated with the replication.
*/
public String getReplicationConfigIdentifier() {
return this.replicationConfigIdentifier;
}
/**
*
* The identifier for the ReplicationConfig
associated with the replication.
*
*
* @param replicationConfigIdentifier
* The identifier for the ReplicationConfig
associated with the replication.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Replication withReplicationConfigIdentifier(String replicationConfigIdentifier) {
setReplicationConfigIdentifier(replicationConfigIdentifier);
return this;
}
/**
*
* The Amazon Resource Name for the ReplicationConfig
associated with the replication.
*
*
* @param replicationConfigArn
* The Amazon Resource Name for the ReplicationConfig
associated with the replication.
*/
public void setReplicationConfigArn(String replicationConfigArn) {
this.replicationConfigArn = replicationConfigArn;
}
/**
*
* The Amazon Resource Name for the ReplicationConfig
associated with the replication.
*
*
* @return The Amazon Resource Name for the ReplicationConfig
associated with the replication.
*/
public String getReplicationConfigArn() {
return this.replicationConfigArn;
}
/**
*
* The Amazon Resource Name for the ReplicationConfig
associated with the replication.
*
*
* @param replicationConfigArn
* The Amazon Resource Name for the ReplicationConfig
associated with the replication.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Replication withReplicationConfigArn(String replicationConfigArn) {
setReplicationConfigArn(replicationConfigArn);
return this;
}
/**
*
* The Amazon Resource Name for an existing Endpoint
the serverless replication uses for its data
* source.
*
*
* @param sourceEndpointArn
* The Amazon Resource Name for an existing Endpoint
the serverless replication uses for its
* data source.
*/
public void setSourceEndpointArn(String sourceEndpointArn) {
this.sourceEndpointArn = sourceEndpointArn;
}
/**
*
* The Amazon Resource Name for an existing Endpoint
the serverless replication uses for its data
* source.
*
*
* @return The Amazon Resource Name for an existing Endpoint
the serverless replication uses for its
* data source.
*/
public String getSourceEndpointArn() {
return this.sourceEndpointArn;
}
/**
*
* The Amazon Resource Name for an existing Endpoint
the serverless replication uses for its data
* source.
*
*
* @param sourceEndpointArn
* The Amazon Resource Name for an existing Endpoint
the serverless replication uses for its
* data source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Replication withSourceEndpointArn(String sourceEndpointArn) {
setSourceEndpointArn(sourceEndpointArn);
return this;
}
/**
*
* The Amazon Resource Name for an existing Endpoint
the serverless replication uses for its data
* target.
*
*
* @param targetEndpointArn
* The Amazon Resource Name for an existing Endpoint
the serverless replication uses for its
* data target.
*/
public void setTargetEndpointArn(String targetEndpointArn) {
this.targetEndpointArn = targetEndpointArn;
}
/**
*
* The Amazon Resource Name for an existing Endpoint
the serverless replication uses for its data
* target.
*
*
* @return The Amazon Resource Name for an existing Endpoint
the serverless replication uses for its
* data target.
*/
public String getTargetEndpointArn() {
return this.targetEndpointArn;
}
/**
*
* The Amazon Resource Name for an existing Endpoint
the serverless replication uses for its data
* target.
*
*
* @param targetEndpointArn
* The Amazon Resource Name for an existing Endpoint
the serverless replication uses for its
* data target.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Replication withTargetEndpointArn(String targetEndpointArn) {
setTargetEndpointArn(targetEndpointArn);
return this;
}
/**
*
* The type of the serverless replication.
*
*
* @param replicationType
* The type of the serverless replication.
* @see MigrationTypeValue
*/
public void setReplicationType(String replicationType) {
this.replicationType = replicationType;
}
/**
*
* The type of the serverless replication.
*
*
* @return The type of the serverless replication.
* @see MigrationTypeValue
*/
public String getReplicationType() {
return this.replicationType;
}
/**
*
* The type of the serverless replication.
*
*
* @param replicationType
* The type of the serverless replication.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MigrationTypeValue
*/
public Replication withReplicationType(String replicationType) {
setReplicationType(replicationType);
return this;
}
/**
*
* The type of the serverless replication.
*
*
* @param replicationType
* The type of the serverless replication.
* @return Returns a reference to this object so that method calls can be chained together.
* @see MigrationTypeValue
*/
public Replication withReplicationType(MigrationTypeValue replicationType) {
this.replicationType = replicationType.toString();
return this;
}
/**
*
* The current status of the serverless replication.
*
*
* @param status
* The current status of the serverless replication.
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The current status of the serverless replication.
*
*
* @return The current status of the serverless replication.
*/
public String getStatus() {
return this.status;
}
/**
*
* The current status of the serverless replication.
*
*
* @param status
* The current status of the serverless replication.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Replication withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* Information about provisioning resources for an DMS serverless replication.
*
*
* @param provisionData
* Information about provisioning resources for an DMS serverless replication.
*/
public void setProvisionData(ProvisionData provisionData) {
this.provisionData = provisionData;
}
/**
*
* Information about provisioning resources for an DMS serverless replication.
*
*
* @return Information about provisioning resources for an DMS serverless replication.
*/
public ProvisionData getProvisionData() {
return this.provisionData;
}
/**
*
* Information about provisioning resources for an DMS serverless replication.
*
*
* @param provisionData
* Information about provisioning resources for an DMS serverless replication.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Replication withProvisionData(ProvisionData provisionData) {
setProvisionData(provisionData);
return this;
}
/**
*
* The reason the replication task was stopped. This response parameter can return one of the following values:
*
*
* -
*
* "Stop Reason NORMAL"
*
*
* -
*
* "Stop Reason RECOVERABLE_ERROR"
*
*
* -
*
* "Stop Reason FATAL_ERROR"
*
*
* -
*
* "Stop Reason FULL_LOAD_ONLY_FINISHED"
*
*
* -
*
* "Stop Reason STOPPED_AFTER_FULL_LOAD"
– Full load completed, with cached changes not applied
*
*
* -
*
* "Stop Reason STOPPED_AFTER_CACHED_EVENTS"
– Full load completed, with cached changes applied
*
*
* -
*
* "Stop Reason EXPRESS_LICENSE_LIMITS_REACHED"
*
*
* -
*
* "Stop Reason STOPPED_AFTER_DDL_APPLY"
– User-defined stop task after DDL applied
*
*
* -
*
* "Stop Reason STOPPED_DUE_TO_LOW_MEMORY"
*
*
* -
*
* "Stop Reason STOPPED_DUE_TO_LOW_DISK"
*
*
* -
*
* "Stop Reason STOPPED_AT_SERVER_TIME"
– User-defined server time for stopping task
*
*
* -
*
* "Stop Reason STOPPED_AT_COMMIT_TIME"
– User-defined commit time for stopping task
*
*
* -
*
* "Stop Reason RECONFIGURATION_RESTART"
*
*
* -
*
* "Stop Reason RECYCLE_TASK"
*
*
*
*
* @param stopReason
* The reason the replication task was stopped. This response parameter can return one of the following
* values:
*
* -
*
* "Stop Reason NORMAL"
*
*
* -
*
* "Stop Reason RECOVERABLE_ERROR"
*
*
* -
*
* "Stop Reason FATAL_ERROR"
*
*
* -
*
* "Stop Reason FULL_LOAD_ONLY_FINISHED"
*
*
* -
*
* "Stop Reason STOPPED_AFTER_FULL_LOAD"
– Full load completed, with cached changes not applied
*
*
* -
*
* "Stop Reason STOPPED_AFTER_CACHED_EVENTS"
– Full load completed, with cached changes applied
*
*
* -
*
* "Stop Reason EXPRESS_LICENSE_LIMITS_REACHED"
*
*
* -
*
* "Stop Reason STOPPED_AFTER_DDL_APPLY"
– User-defined stop task after DDL applied
*
*
* -
*
* "Stop Reason STOPPED_DUE_TO_LOW_MEMORY"
*
*
* -
*
* "Stop Reason STOPPED_DUE_TO_LOW_DISK"
*
*
* -
*
* "Stop Reason STOPPED_AT_SERVER_TIME"
– User-defined server time for stopping task
*
*
* -
*
* "Stop Reason STOPPED_AT_COMMIT_TIME"
– User-defined commit time for stopping task
*
*
* -
*
* "Stop Reason RECONFIGURATION_RESTART"
*
*
* -
*
* "Stop Reason RECYCLE_TASK"
*
*
*/
public void setStopReason(String stopReason) {
this.stopReason = stopReason;
}
/**
*
* The reason the replication task was stopped. This response parameter can return one of the following values:
*
*
* -
*
* "Stop Reason NORMAL"
*
*
* -
*
* "Stop Reason RECOVERABLE_ERROR"
*
*
* -
*
* "Stop Reason FATAL_ERROR"
*
*
* -
*
* "Stop Reason FULL_LOAD_ONLY_FINISHED"
*
*
* -
*
* "Stop Reason STOPPED_AFTER_FULL_LOAD"
– Full load completed, with cached changes not applied
*
*
* -
*
* "Stop Reason STOPPED_AFTER_CACHED_EVENTS"
– Full load completed, with cached changes applied
*
*
* -
*
* "Stop Reason EXPRESS_LICENSE_LIMITS_REACHED"
*
*
* -
*
* "Stop Reason STOPPED_AFTER_DDL_APPLY"
– User-defined stop task after DDL applied
*
*
* -
*
* "Stop Reason STOPPED_DUE_TO_LOW_MEMORY"
*
*
* -
*
* "Stop Reason STOPPED_DUE_TO_LOW_DISK"
*
*
* -
*
* "Stop Reason STOPPED_AT_SERVER_TIME"
– User-defined server time for stopping task
*
*
* -
*
* "Stop Reason STOPPED_AT_COMMIT_TIME"
– User-defined commit time for stopping task
*
*
* -
*
* "Stop Reason RECONFIGURATION_RESTART"
*
*
* -
*
* "Stop Reason RECYCLE_TASK"
*
*
*
*
* @return The reason the replication task was stopped. This response parameter can return one of the following
* values:
*
* -
*
* "Stop Reason NORMAL"
*
*
* -
*
* "Stop Reason RECOVERABLE_ERROR"
*
*
* -
*
* "Stop Reason FATAL_ERROR"
*
*
* -
*
* "Stop Reason FULL_LOAD_ONLY_FINISHED"
*
*
* -
*
* "Stop Reason STOPPED_AFTER_FULL_LOAD"
– Full load completed, with cached changes not applied
*
*
* -
*
* "Stop Reason STOPPED_AFTER_CACHED_EVENTS"
– Full load completed, with cached changes applied
*
*
* -
*
* "Stop Reason EXPRESS_LICENSE_LIMITS_REACHED"
*
*
* -
*
* "Stop Reason STOPPED_AFTER_DDL_APPLY"
– User-defined stop task after DDL applied
*
*
* -
*
* "Stop Reason STOPPED_DUE_TO_LOW_MEMORY"
*
*
* -
*
* "Stop Reason STOPPED_DUE_TO_LOW_DISK"
*
*
* -
*
* "Stop Reason STOPPED_AT_SERVER_TIME"
– User-defined server time for stopping task
*
*
* -
*
* "Stop Reason STOPPED_AT_COMMIT_TIME"
– User-defined commit time for stopping task
*
*
* -
*
* "Stop Reason RECONFIGURATION_RESTART"
*
*
* -
*
* "Stop Reason RECYCLE_TASK"
*
*
*/
public String getStopReason() {
return this.stopReason;
}
/**
*
* The reason the replication task was stopped. This response parameter can return one of the following values:
*
*
* -
*
* "Stop Reason NORMAL"
*
*
* -
*
* "Stop Reason RECOVERABLE_ERROR"
*
*
* -
*
* "Stop Reason FATAL_ERROR"
*
*
* -
*
* "Stop Reason FULL_LOAD_ONLY_FINISHED"
*
*
* -
*
* "Stop Reason STOPPED_AFTER_FULL_LOAD"
– Full load completed, with cached changes not applied
*
*
* -
*
* "Stop Reason STOPPED_AFTER_CACHED_EVENTS"
– Full load completed, with cached changes applied
*
*
* -
*
* "Stop Reason EXPRESS_LICENSE_LIMITS_REACHED"
*
*
* -
*
* "Stop Reason STOPPED_AFTER_DDL_APPLY"
– User-defined stop task after DDL applied
*
*
* -
*
* "Stop Reason STOPPED_DUE_TO_LOW_MEMORY"
*
*
* -
*
* "Stop Reason STOPPED_DUE_TO_LOW_DISK"
*
*
* -
*
* "Stop Reason STOPPED_AT_SERVER_TIME"
– User-defined server time for stopping task
*
*
* -
*
* "Stop Reason STOPPED_AT_COMMIT_TIME"
– User-defined commit time for stopping task
*
*
* -
*
* "Stop Reason RECONFIGURATION_RESTART"
*
*
* -
*
* "Stop Reason RECYCLE_TASK"
*
*
*
*
* @param stopReason
* The reason the replication task was stopped. This response parameter can return one of the following
* values:
*
* -
*
* "Stop Reason NORMAL"
*
*
* -
*
* "Stop Reason RECOVERABLE_ERROR"
*
*
* -
*
* "Stop Reason FATAL_ERROR"
*
*
* -
*
* "Stop Reason FULL_LOAD_ONLY_FINISHED"
*
*
* -
*
* "Stop Reason STOPPED_AFTER_FULL_LOAD"
– Full load completed, with cached changes not applied
*
*
* -
*
* "Stop Reason STOPPED_AFTER_CACHED_EVENTS"
– Full load completed, with cached changes applied
*
*
* -
*
* "Stop Reason EXPRESS_LICENSE_LIMITS_REACHED"
*
*
* -
*
* "Stop Reason STOPPED_AFTER_DDL_APPLY"
– User-defined stop task after DDL applied
*
*
* -
*
* "Stop Reason STOPPED_DUE_TO_LOW_MEMORY"
*
*
* -
*
* "Stop Reason STOPPED_DUE_TO_LOW_DISK"
*
*
* -
*
* "Stop Reason STOPPED_AT_SERVER_TIME"
– User-defined server time for stopping task
*
*
* -
*
* "Stop Reason STOPPED_AT_COMMIT_TIME"
– User-defined commit time for stopping task
*
*
* -
*
* "Stop Reason RECONFIGURATION_RESTART"
*
*
* -
*
* "Stop Reason RECYCLE_TASK"
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Replication withStopReason(String stopReason) {
setStopReason(stopReason);
return this;
}
/**
*
* Error and other information about why a serverless replication failed.
*
*
* @return Error and other information about why a serverless replication failed.
*/
public java.util.List getFailureMessages() {
return failureMessages;
}
/**
*
* Error and other information about why a serverless replication failed.
*
*
* @param failureMessages
* Error and other information about why a serverless replication failed.
*/
public void setFailureMessages(java.util.Collection failureMessages) {
if (failureMessages == null) {
this.failureMessages = null;
return;
}
this.failureMessages = new java.util.ArrayList(failureMessages);
}
/**
*
* Error and other information about why a serverless replication failed.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setFailureMessages(java.util.Collection)} or {@link #withFailureMessages(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param failureMessages
* Error and other information about why a serverless replication failed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Replication withFailureMessages(String... failureMessages) {
if (this.failureMessages == null) {
setFailureMessages(new java.util.ArrayList(failureMessages.length));
}
for (String ele : failureMessages) {
this.failureMessages.add(ele);
}
return this;
}
/**
*
* Error and other information about why a serverless replication failed.
*
*
* @param failureMessages
* Error and other information about why a serverless replication failed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Replication withFailureMessages(java.util.Collection failureMessages) {
setFailureMessages(failureMessages);
return this;
}
/**
*
* This object provides a collection of statistics about a serverless replication.
*
*
* @param replicationStats
* This object provides a collection of statistics about a serverless replication.
*/
public void setReplicationStats(ReplicationStats replicationStats) {
this.replicationStats = replicationStats;
}
/**
*
* This object provides a collection of statistics about a serverless replication.
*
*
* @return This object provides a collection of statistics about a serverless replication.
*/
public ReplicationStats getReplicationStats() {
return this.replicationStats;
}
/**
*
* This object provides a collection of statistics about a serverless replication.
*
*
* @param replicationStats
* This object provides a collection of statistics about a serverless replication.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Replication withReplicationStats(ReplicationStats replicationStats) {
setReplicationStats(replicationStats);
return this;
}
/**
*
* The replication type.
*
*
* @param startReplicationType
* The replication type.
*/
public void setStartReplicationType(String startReplicationType) {
this.startReplicationType = startReplicationType;
}
/**
*
* The replication type.
*
*
* @return The replication type.
*/
public String getStartReplicationType() {
return this.startReplicationType;
}
/**
*
* The replication type.
*
*
* @param startReplicationType
* The replication type.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Replication withStartReplicationType(String startReplicationType) {
setStartReplicationType(startReplicationType);
return this;
}
/**
*
* Indicates the start time for a change data capture (CDC) operation. Use either CdcStartTime
or
* CdcStartPosition
to specify when you want a CDC operation to start. Specifying both values results
* in an error.
*
*
* @param cdcStartTime
* Indicates the start time for a change data capture (CDC) operation. Use either CdcStartTime
* or CdcStartPosition
to specify when you want a CDC operation to start. Specifying both values
* results in an error.
*/
public void setCdcStartTime(java.util.Date cdcStartTime) {
this.cdcStartTime = cdcStartTime;
}
/**
*
* Indicates the start time for a change data capture (CDC) operation. Use either CdcStartTime
or
* CdcStartPosition
to specify when you want a CDC operation to start. Specifying both values results
* in an error.
*
*
* @return Indicates the start time for a change data capture (CDC) operation. Use either CdcStartTime
* or CdcStartPosition
to specify when you want a CDC operation to start. Specifying both
* values results in an error.
*/
public java.util.Date getCdcStartTime() {
return this.cdcStartTime;
}
/**
*
* Indicates the start time for a change data capture (CDC) operation. Use either CdcStartTime
or
* CdcStartPosition
to specify when you want a CDC operation to start. Specifying both values results
* in an error.
*
*
* @param cdcStartTime
* Indicates the start time for a change data capture (CDC) operation. Use either CdcStartTime
* or CdcStartPosition
to specify when you want a CDC operation to start. Specifying both values
* results in an error.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Replication withCdcStartTime(java.util.Date cdcStartTime) {
setCdcStartTime(cdcStartTime);
return this;
}
/**
*
* Indicates the start time for a change data capture (CDC) operation. Use either CdcStartTime
or
* CdcStartPosition
to specify when you want a CDC operation to start. Specifying both values results
* in an error.
*
*
* @param cdcStartPosition
* Indicates the start time for a change data capture (CDC) operation. Use either CdcStartTime
* or CdcStartPosition
to specify when you want a CDC operation to start. Specifying both values
* results in an error.
*/
public void setCdcStartPosition(String cdcStartPosition) {
this.cdcStartPosition = cdcStartPosition;
}
/**
*
* Indicates the start time for a change data capture (CDC) operation. Use either CdcStartTime
or
* CdcStartPosition
to specify when you want a CDC operation to start. Specifying both values results
* in an error.
*
*
* @return Indicates the start time for a change data capture (CDC) operation. Use either CdcStartTime
* or CdcStartPosition
to specify when you want a CDC operation to start. Specifying both
* values results in an error.
*/
public String getCdcStartPosition() {
return this.cdcStartPosition;
}
/**
*
* Indicates the start time for a change data capture (CDC) operation. Use either CdcStartTime
or
* CdcStartPosition
to specify when you want a CDC operation to start. Specifying both values results
* in an error.
*
*
* @param cdcStartPosition
* Indicates the start time for a change data capture (CDC) operation. Use either CdcStartTime
* or CdcStartPosition
to specify when you want a CDC operation to start. Specifying both values
* results in an error.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Replication withCdcStartPosition(String cdcStartPosition) {
setCdcStartPosition(cdcStartPosition);
return this;
}
/**
*
* Indicates when you want a change data capture (CDC) operation to stop. The value can be either server time or
* commit time.
*
*
* @param cdcStopPosition
* Indicates when you want a change data capture (CDC) operation to stop. The value can be either server time
* or commit time.
*/
public void setCdcStopPosition(String cdcStopPosition) {
this.cdcStopPosition = cdcStopPosition;
}
/**
*
* Indicates when you want a change data capture (CDC) operation to stop. The value can be either server time or
* commit time.
*
*
* @return Indicates when you want a change data capture (CDC) operation to stop. The value can be either server
* time or commit time.
*/
public String getCdcStopPosition() {
return this.cdcStopPosition;
}
/**
*
* Indicates when you want a change data capture (CDC) operation to stop. The value can be either server time or
* commit time.
*
*
* @param cdcStopPosition
* Indicates when you want a change data capture (CDC) operation to stop. The value can be either server time
* or commit time.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Replication withCdcStopPosition(String cdcStopPosition) {
setCdcStopPosition(cdcStopPosition);
return this;
}
/**
*
* Indicates the last checkpoint that occurred during a change data capture (CDC) operation. You can provide this
* value to the CdcStartPosition
parameter to start a CDC operation that begins at that checkpoint.
*
*
* @param recoveryCheckpoint
* Indicates the last checkpoint that occurred during a change data capture (CDC) operation. You can provide
* this value to the CdcStartPosition
parameter to start a CDC operation that begins at that
* checkpoint.
*/
public void setRecoveryCheckpoint(String recoveryCheckpoint) {
this.recoveryCheckpoint = recoveryCheckpoint;
}
/**
*
* Indicates the last checkpoint that occurred during a change data capture (CDC) operation. You can provide this
* value to the CdcStartPosition
parameter to start a CDC operation that begins at that checkpoint.
*
*
* @return Indicates the last checkpoint that occurred during a change data capture (CDC) operation. You can provide
* this value to the CdcStartPosition
parameter to start a CDC operation that begins at that
* checkpoint.
*/
public String getRecoveryCheckpoint() {
return this.recoveryCheckpoint;
}
/**
*
* Indicates the last checkpoint that occurred during a change data capture (CDC) operation. You can provide this
* value to the CdcStartPosition
parameter to start a CDC operation that begins at that checkpoint.
*
*
* @param recoveryCheckpoint
* Indicates the last checkpoint that occurred during a change data capture (CDC) operation. You can provide
* this value to the CdcStartPosition
parameter to start a CDC operation that begins at that
* checkpoint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Replication withRecoveryCheckpoint(String recoveryCheckpoint) {
setRecoveryCheckpoint(recoveryCheckpoint);
return this;
}
/**
*
* The time the serverless replication was created.
*
*
* @param replicationCreateTime
* The time the serverless replication was created.
*/
public void setReplicationCreateTime(java.util.Date replicationCreateTime) {
this.replicationCreateTime = replicationCreateTime;
}
/**
*
* The time the serverless replication was created.
*
*
* @return The time the serverless replication was created.
*/
public java.util.Date getReplicationCreateTime() {
return this.replicationCreateTime;
}
/**
*
* The time the serverless replication was created.
*
*
* @param replicationCreateTime
* The time the serverless replication was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Replication withReplicationCreateTime(java.util.Date replicationCreateTime) {
setReplicationCreateTime(replicationCreateTime);
return this;
}
/**
*
* The time the serverless replication was updated.
*
*
* @param replicationUpdateTime
* The time the serverless replication was updated.
*/
public void setReplicationUpdateTime(java.util.Date replicationUpdateTime) {
this.replicationUpdateTime = replicationUpdateTime;
}
/**
*
* The time the serverless replication was updated.
*
*
* @return The time the serverless replication was updated.
*/
public java.util.Date getReplicationUpdateTime() {
return this.replicationUpdateTime;
}
/**
*
* The time the serverless replication was updated.
*
*
* @param replicationUpdateTime
* The time the serverless replication was updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Replication withReplicationUpdateTime(java.util.Date replicationUpdateTime) {
setReplicationUpdateTime(replicationUpdateTime);
return this;
}
/**
*
* The timestamp when replication was last stopped.
*
*
* @param replicationLastStopTime
* The timestamp when replication was last stopped.
*/
public void setReplicationLastStopTime(java.util.Date replicationLastStopTime) {
this.replicationLastStopTime = replicationLastStopTime;
}
/**
*
* The timestamp when replication was last stopped.
*
*
* @return The timestamp when replication was last stopped.
*/
public java.util.Date getReplicationLastStopTime() {
return this.replicationLastStopTime;
}
/**
*
* The timestamp when replication was last stopped.
*
*
* @param replicationLastStopTime
* The timestamp when replication was last stopped.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Replication withReplicationLastStopTime(java.util.Date replicationLastStopTime) {
setReplicationLastStopTime(replicationLastStopTime);
return this;
}
/**
*
* The timestamp when DMS will deprovision the replication.
*
*
* @param replicationDeprovisionTime
* The timestamp when DMS will deprovision the replication.
*/
public void setReplicationDeprovisionTime(java.util.Date replicationDeprovisionTime) {
this.replicationDeprovisionTime = replicationDeprovisionTime;
}
/**
*
* The timestamp when DMS will deprovision the replication.
*
*
* @return The timestamp when DMS will deprovision the replication.
*/
public java.util.Date getReplicationDeprovisionTime() {
return this.replicationDeprovisionTime;
}
/**
*
* The timestamp when DMS will deprovision the replication.
*
*
* @param replicationDeprovisionTime
* The timestamp when DMS will deprovision the replication.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Replication withReplicationDeprovisionTime(java.util.Date replicationDeprovisionTime) {
setReplicationDeprovisionTime(replicationDeprovisionTime);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getReplicationConfigIdentifier() != null)
sb.append("ReplicationConfigIdentifier: ").append(getReplicationConfigIdentifier()).append(",");
if (getReplicationConfigArn() != null)
sb.append("ReplicationConfigArn: ").append(getReplicationConfigArn()).append(",");
if (getSourceEndpointArn() != null)
sb.append("SourceEndpointArn: ").append(getSourceEndpointArn()).append(",");
if (getTargetEndpointArn() != null)
sb.append("TargetEndpointArn: ").append(getTargetEndpointArn()).append(",");
if (getReplicationType() != null)
sb.append("ReplicationType: ").append(getReplicationType()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getProvisionData() != null)
sb.append("ProvisionData: ").append(getProvisionData()).append(",");
if (getStopReason() != null)
sb.append("StopReason: ").append(getStopReason()).append(",");
if (getFailureMessages() != null)
sb.append("FailureMessages: ").append(getFailureMessages()).append(",");
if (getReplicationStats() != null)
sb.append("ReplicationStats: ").append(getReplicationStats()).append(",");
if (getStartReplicationType() != null)
sb.append("StartReplicationType: ").append(getStartReplicationType()).append(",");
if (getCdcStartTime() != null)
sb.append("CdcStartTime: ").append(getCdcStartTime()).append(",");
if (getCdcStartPosition() != null)
sb.append("CdcStartPosition: ").append(getCdcStartPosition()).append(",");
if (getCdcStopPosition() != null)
sb.append("CdcStopPosition: ").append(getCdcStopPosition()).append(",");
if (getRecoveryCheckpoint() != null)
sb.append("RecoveryCheckpoint: ").append(getRecoveryCheckpoint()).append(",");
if (getReplicationCreateTime() != null)
sb.append("ReplicationCreateTime: ").append(getReplicationCreateTime()).append(",");
if (getReplicationUpdateTime() != null)
sb.append("ReplicationUpdateTime: ").append(getReplicationUpdateTime()).append(",");
if (getReplicationLastStopTime() != null)
sb.append("ReplicationLastStopTime: ").append(getReplicationLastStopTime()).append(",");
if (getReplicationDeprovisionTime() != null)
sb.append("ReplicationDeprovisionTime: ").append(getReplicationDeprovisionTime());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Replication == false)
return false;
Replication other = (Replication) obj;
if (other.getReplicationConfigIdentifier() == null ^ this.getReplicationConfigIdentifier() == null)
return false;
if (other.getReplicationConfigIdentifier() != null && other.getReplicationConfigIdentifier().equals(this.getReplicationConfigIdentifier()) == false)
return false;
if (other.getReplicationConfigArn() == null ^ this.getReplicationConfigArn() == null)
return false;
if (other.getReplicationConfigArn() != null && other.getReplicationConfigArn().equals(this.getReplicationConfigArn()) == false)
return false;
if (other.getSourceEndpointArn() == null ^ this.getSourceEndpointArn() == null)
return false;
if (other.getSourceEndpointArn() != null && other.getSourceEndpointArn().equals(this.getSourceEndpointArn()) == false)
return false;
if (other.getTargetEndpointArn() == null ^ this.getTargetEndpointArn() == null)
return false;
if (other.getTargetEndpointArn() != null && other.getTargetEndpointArn().equals(this.getTargetEndpointArn()) == false)
return false;
if (other.getReplicationType() == null ^ this.getReplicationType() == null)
return false;
if (other.getReplicationType() != null && other.getReplicationType().equals(this.getReplicationType()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getProvisionData() == null ^ this.getProvisionData() == null)
return false;
if (other.getProvisionData() != null && other.getProvisionData().equals(this.getProvisionData()) == false)
return false;
if (other.getStopReason() == null ^ this.getStopReason() == null)
return false;
if (other.getStopReason() != null && other.getStopReason().equals(this.getStopReason()) == false)
return false;
if (other.getFailureMessages() == null ^ this.getFailureMessages() == null)
return false;
if (other.getFailureMessages() != null && other.getFailureMessages().equals(this.getFailureMessages()) == false)
return false;
if (other.getReplicationStats() == null ^ this.getReplicationStats() == null)
return false;
if (other.getReplicationStats() != null && other.getReplicationStats().equals(this.getReplicationStats()) == false)
return false;
if (other.getStartReplicationType() == null ^ this.getStartReplicationType() == null)
return false;
if (other.getStartReplicationType() != null && other.getStartReplicationType().equals(this.getStartReplicationType()) == false)
return false;
if (other.getCdcStartTime() == null ^ this.getCdcStartTime() == null)
return false;
if (other.getCdcStartTime() != null && other.getCdcStartTime().equals(this.getCdcStartTime()) == false)
return false;
if (other.getCdcStartPosition() == null ^ this.getCdcStartPosition() == null)
return false;
if (other.getCdcStartPosition() != null && other.getCdcStartPosition().equals(this.getCdcStartPosition()) == false)
return false;
if (other.getCdcStopPosition() == null ^ this.getCdcStopPosition() == null)
return false;
if (other.getCdcStopPosition() != null && other.getCdcStopPosition().equals(this.getCdcStopPosition()) == false)
return false;
if (other.getRecoveryCheckpoint() == null ^ this.getRecoveryCheckpoint() == null)
return false;
if (other.getRecoveryCheckpoint() != null && other.getRecoveryCheckpoint().equals(this.getRecoveryCheckpoint()) == false)
return false;
if (other.getReplicationCreateTime() == null ^ this.getReplicationCreateTime() == null)
return false;
if (other.getReplicationCreateTime() != null && other.getReplicationCreateTime().equals(this.getReplicationCreateTime()) == false)
return false;
if (other.getReplicationUpdateTime() == null ^ this.getReplicationUpdateTime() == null)
return false;
if (other.getReplicationUpdateTime() != null && other.getReplicationUpdateTime().equals(this.getReplicationUpdateTime()) == false)
return false;
if (other.getReplicationLastStopTime() == null ^ this.getReplicationLastStopTime() == null)
return false;
if (other.getReplicationLastStopTime() != null && other.getReplicationLastStopTime().equals(this.getReplicationLastStopTime()) == false)
return false;
if (other.getReplicationDeprovisionTime() == null ^ this.getReplicationDeprovisionTime() == null)
return false;
if (other.getReplicationDeprovisionTime() != null && other.getReplicationDeprovisionTime().equals(this.getReplicationDeprovisionTime()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getReplicationConfigIdentifier() == null) ? 0 : getReplicationConfigIdentifier().hashCode());
hashCode = prime * hashCode + ((getReplicationConfigArn() == null) ? 0 : getReplicationConfigArn().hashCode());
hashCode = prime * hashCode + ((getSourceEndpointArn() == null) ? 0 : getSourceEndpointArn().hashCode());
hashCode = prime * hashCode + ((getTargetEndpointArn() == null) ? 0 : getTargetEndpointArn().hashCode());
hashCode = prime * hashCode + ((getReplicationType() == null) ? 0 : getReplicationType().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getProvisionData() == null) ? 0 : getProvisionData().hashCode());
hashCode = prime * hashCode + ((getStopReason() == null) ? 0 : getStopReason().hashCode());
hashCode = prime * hashCode + ((getFailureMessages() == null) ? 0 : getFailureMessages().hashCode());
hashCode = prime * hashCode + ((getReplicationStats() == null) ? 0 : getReplicationStats().hashCode());
hashCode = prime * hashCode + ((getStartReplicationType() == null) ? 0 : getStartReplicationType().hashCode());
hashCode = prime * hashCode + ((getCdcStartTime() == null) ? 0 : getCdcStartTime().hashCode());
hashCode = prime * hashCode + ((getCdcStartPosition() == null) ? 0 : getCdcStartPosition().hashCode());
hashCode = prime * hashCode + ((getCdcStopPosition() == null) ? 0 : getCdcStopPosition().hashCode());
hashCode = prime * hashCode + ((getRecoveryCheckpoint() == null) ? 0 : getRecoveryCheckpoint().hashCode());
hashCode = prime * hashCode + ((getReplicationCreateTime() == null) ? 0 : getReplicationCreateTime().hashCode());
hashCode = prime * hashCode + ((getReplicationUpdateTime() == null) ? 0 : getReplicationUpdateTime().hashCode());
hashCode = prime * hashCode + ((getReplicationLastStopTime() == null) ? 0 : getReplicationLastStopTime().hashCode());
hashCode = prime * hashCode + ((getReplicationDeprovisionTime() == null) ? 0 : getReplicationDeprovisionTime().hashCode());
return hashCode;
}
@Override
public Replication clone() {
try {
return (Replication) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.databasemigrationservice.model.transform.ReplicationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}