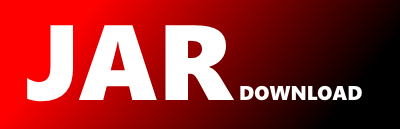
com.amazonaws.services.databasemigrationservice.AWSDatabaseMigrationServiceAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-dms Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.databasemigrationservice;
import javax.annotation.Generated;
import com.amazonaws.services.databasemigrationservice.model.*;
/**
* Interface for accessing AWS Database Migration Service asynchronously. Each asynchronous method will return a Java
* Future object representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to
* receive notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.databasemigrationservice.AbstractAWSDatabaseMigrationServiceAsync} instead.
*
*
* Database Migration Service
*
* Database Migration Service (DMS) can migrate your data to and from the most widely used commercial and open-source
* databases such as Oracle, PostgreSQL, Microsoft SQL Server, Amazon Redshift, MariaDB, Amazon Aurora, MySQL, and SAP
* Adaptive Server Enterprise (ASE). The service supports homogeneous migrations such as Oracle to Oracle, as well as
* heterogeneous migrations between different database platforms, such as Oracle to MySQL or SQL Server to PostgreSQL.
*
*
* For more information about DMS, see What Is
* Database Migration Service? in the Database Migration Service User Guide.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSDatabaseMigrationServiceAsync extends AWSDatabaseMigrationService {
/**
*
* Adds metadata tags to an DMS resource, including replication instance, endpoint, subnet group, and migration
* task. These tags can also be used with cost allocation reporting to track cost associated with DMS resources, or
* used in a Condition statement in an IAM policy for DMS. For more information, see Tag
data type
* description.
*
*
* @param addTagsToResourceRequest
* Associates a set of tags with an DMS resource.
* @return A Java Future containing the result of the AddTagsToResource operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.AddTagsToResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future addTagsToResourceAsync(AddTagsToResourceRequest addTagsToResourceRequest);
/**
*
* Adds metadata tags to an DMS resource, including replication instance, endpoint, subnet group, and migration
* task. These tags can also be used with cost allocation reporting to track cost associated with DMS resources, or
* used in a Condition statement in an IAM policy for DMS. For more information, see Tag
data type
* description.
*
*
* @param addTagsToResourceRequest
* Associates a set of tags with an DMS resource.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AddTagsToResource operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.AddTagsToResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future addTagsToResourceAsync(AddTagsToResourceRequest addTagsToResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Applies a pending maintenance action to a resource (for example, to a replication instance).
*
*
* @param applyPendingMaintenanceActionRequest
* @return A Java Future containing the result of the ApplyPendingMaintenanceAction operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.ApplyPendingMaintenanceAction
* @see AWS API Documentation
*/
java.util.concurrent.Future applyPendingMaintenanceActionAsync(
ApplyPendingMaintenanceActionRequest applyPendingMaintenanceActionRequest);
/**
*
* Applies a pending maintenance action to a resource (for example, to a replication instance).
*
*
* @param applyPendingMaintenanceActionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ApplyPendingMaintenanceAction operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.ApplyPendingMaintenanceAction
* @see AWS API Documentation
*/
java.util.concurrent.Future applyPendingMaintenanceActionAsync(
ApplyPendingMaintenanceActionRequest applyPendingMaintenanceActionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts the analysis of up to 20 source databases to recommend target engines for each source database. This is a
* batch version of StartRecommendations
* .
*
*
* The result of analysis of each source database is reported individually in the response. Because the batch
* request can result in a combination of successful and unsuccessful actions, you should check for batch errors
* even when the call returns an HTTP status code of 200
.
*
*
* @param batchStartRecommendationsRequest
* @return A Java Future containing the result of the BatchStartRecommendations operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.BatchStartRecommendations
* @see AWS
* API Documentation
*/
java.util.concurrent.Future batchStartRecommendationsAsync(
BatchStartRecommendationsRequest batchStartRecommendationsRequest);
/**
*
* Starts the analysis of up to 20 source databases to recommend target engines for each source database. This is a
* batch version of StartRecommendations
* .
*
*
* The result of analysis of each source database is reported individually in the response. Because the batch
* request can result in a combination of successful and unsuccessful actions, you should check for batch errors
* even when the call returns an HTTP status code of 200
.
*
*
* @param batchStartRecommendationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchStartRecommendations operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.BatchStartRecommendations
* @see AWS
* API Documentation
*/
java.util.concurrent.Future batchStartRecommendationsAsync(
BatchStartRecommendationsRequest batchStartRecommendationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels a single premigration assessment run.
*
*
* This operation prevents any individual assessments from running if they haven't started running. It also attempts
* to cancel any individual assessments that are currently running.
*
*
* @param cancelReplicationTaskAssessmentRunRequest
* @return A Java Future containing the result of the CancelReplicationTaskAssessmentRun operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.CancelReplicationTaskAssessmentRun
* @see AWS API Documentation
*/
java.util.concurrent.Future cancelReplicationTaskAssessmentRunAsync(
CancelReplicationTaskAssessmentRunRequest cancelReplicationTaskAssessmentRunRequest);
/**
*
* Cancels a single premigration assessment run.
*
*
* This operation prevents any individual assessments from running if they haven't started running. It also attempts
* to cancel any individual assessments that are currently running.
*
*
* @param cancelReplicationTaskAssessmentRunRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelReplicationTaskAssessmentRun operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.CancelReplicationTaskAssessmentRun
* @see AWS API Documentation
*/
java.util.concurrent.Future cancelReplicationTaskAssessmentRunAsync(
CancelReplicationTaskAssessmentRunRequest cancelReplicationTaskAssessmentRunRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a data provider using the provided settings. A data provider stores a data store type and location
* information about your database.
*
*
* @param createDataProviderRequest
* @return A Java Future containing the result of the CreateDataProvider operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.CreateDataProvider
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDataProviderAsync(CreateDataProviderRequest createDataProviderRequest);
/**
*
* Creates a data provider using the provided settings. A data provider stores a data store type and location
* information about your database.
*
*
* @param createDataProviderRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDataProvider operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.CreateDataProvider
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDataProviderAsync(CreateDataProviderRequest createDataProviderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an endpoint using the provided settings.
*
*
*
* For a MySQL source or target endpoint, don't explicitly specify the database using the DatabaseName
* request parameter on the CreateEndpoint
API call. Specifying DatabaseName
when you
* create a MySQL endpoint replicates all the task tables to this single database. For MySQL endpoints, you specify
* the database only when you specify the schema in the table-mapping rules of the DMS task.
*
*
*
* @param createEndpointRequest
* @return A Java Future containing the result of the CreateEndpoint operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.CreateEndpoint
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createEndpointAsync(CreateEndpointRequest createEndpointRequest);
/**
*
* Creates an endpoint using the provided settings.
*
*
*
* For a MySQL source or target endpoint, don't explicitly specify the database using the DatabaseName
* request parameter on the CreateEndpoint
API call. Specifying DatabaseName
when you
* create a MySQL endpoint replicates all the task tables to this single database. For MySQL endpoints, you specify
* the database only when you specify the schema in the table-mapping rules of the DMS task.
*
*
*
* @param createEndpointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateEndpoint operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.CreateEndpoint
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createEndpointAsync(CreateEndpointRequest createEndpointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an DMS event notification subscription.
*
*
* You can specify the type of source (SourceType
) you want to be notified of, provide a list of DMS
* source IDs (SourceIds
) that triggers the events, and provide a list of event categories (
* EventCategories
) for events you want to be notified of. If you specify both the
* SourceType
and SourceIds
, such as SourceType = replication-instance
and
* SourceIdentifier = my-replinstance
, you will be notified of all the replication instance events for
* the specified source. If you specify a SourceType
but don't specify a SourceIdentifier
,
* you receive notice of the events for that source type for all your DMS sources. If you don't specify either
* SourceType
nor SourceIdentifier
, you will be notified of events generated from all DMS
* sources belonging to your customer account.
*
*
* For more information about DMS events, see Working with Events and
* Notifications in the Database Migration Service User Guide.
*
*
* @param createEventSubscriptionRequest
* @return A Java Future containing the result of the CreateEventSubscription operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.CreateEventSubscription
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createEventSubscriptionAsync(CreateEventSubscriptionRequest createEventSubscriptionRequest);
/**
*
* Creates an DMS event notification subscription.
*
*
* You can specify the type of source (SourceType
) you want to be notified of, provide a list of DMS
* source IDs (SourceIds
) that triggers the events, and provide a list of event categories (
* EventCategories
) for events you want to be notified of. If you specify both the
* SourceType
and SourceIds
, such as SourceType = replication-instance
and
* SourceIdentifier = my-replinstance
, you will be notified of all the replication instance events for
* the specified source. If you specify a SourceType
but don't specify a SourceIdentifier
,
* you receive notice of the events for that source type for all your DMS sources. If you don't specify either
* SourceType
nor SourceIdentifier
, you will be notified of events generated from all DMS
* sources belonging to your customer account.
*
*
* For more information about DMS events, see Working with Events and
* Notifications in the Database Migration Service User Guide.
*
*
* @param createEventSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateEventSubscription operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.CreateEventSubscription
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createEventSubscriptionAsync(CreateEventSubscriptionRequest createEventSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a Fleet Advisor collector using the specified parameters.
*
*
* @param createFleetAdvisorCollectorRequest
* @return A Java Future containing the result of the CreateFleetAdvisorCollector operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.CreateFleetAdvisorCollector
* @see AWS API Documentation
*/
java.util.concurrent.Future createFleetAdvisorCollectorAsync(
CreateFleetAdvisorCollectorRequest createFleetAdvisorCollectorRequest);
/**
*
* Creates a Fleet Advisor collector using the specified parameters.
*
*
* @param createFleetAdvisorCollectorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateFleetAdvisorCollector operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.CreateFleetAdvisorCollector
* @see AWS API Documentation
*/
java.util.concurrent.Future createFleetAdvisorCollectorAsync(
CreateFleetAdvisorCollectorRequest createFleetAdvisorCollectorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates the instance profile using the specified parameters.
*
*
* @param createInstanceProfileRequest
* @return A Java Future containing the result of the CreateInstanceProfile operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.CreateInstanceProfile
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createInstanceProfileAsync(CreateInstanceProfileRequest createInstanceProfileRequest);
/**
*
* Creates the instance profile using the specified parameters.
*
*
* @param createInstanceProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateInstanceProfile operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.CreateInstanceProfile
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createInstanceProfileAsync(CreateInstanceProfileRequest createInstanceProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates the migration project using the specified parameters.
*
*
* You can run this action only after you create an instance profile and data providers using CreateInstanceProfile and CreateDataProvider.
*
*
* @param createMigrationProjectRequest
* @return A Java Future containing the result of the CreateMigrationProject operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.CreateMigrationProject
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createMigrationProjectAsync(CreateMigrationProjectRequest createMigrationProjectRequest);
/**
*
* Creates the migration project using the specified parameters.
*
*
* You can run this action only after you create an instance profile and data providers using CreateInstanceProfile and CreateDataProvider.
*
*
* @param createMigrationProjectRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateMigrationProject operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.CreateMigrationProject
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createMigrationProjectAsync(CreateMigrationProjectRequest createMigrationProjectRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a configuration that you can later provide to configure and start an DMS Serverless replication. You can
* also provide options to validate the configuration inputs before you start the replication.
*
*
* @param createReplicationConfigRequest
* @return A Java Future containing the result of the CreateReplicationConfig operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.CreateReplicationConfig
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createReplicationConfigAsync(CreateReplicationConfigRequest createReplicationConfigRequest);
/**
*
* Creates a configuration that you can later provide to configure and start an DMS Serverless replication. You can
* also provide options to validate the configuration inputs before you start the replication.
*
*
* @param createReplicationConfigRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateReplicationConfig operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.CreateReplicationConfig
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createReplicationConfigAsync(CreateReplicationConfigRequest createReplicationConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates the replication instance using the specified parameters.
*
*
* DMS requires that your account have certain roles with appropriate permissions before you can create a
* replication instance. For information on the required roles, see Creating the IAM
* Roles to Use With the CLI and DMS API. For information on the required permissions, see IAM
* Permissions Needed to Use DMS.
*
*
*
* If you don't specify a version when creating a replication instance, DMS will create the instance using the
* default engine version. For information about the default engine version, see Release Notes.
*
*
*
* @param createReplicationInstanceRequest
* @return A Java Future containing the result of the CreateReplicationInstance operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.CreateReplicationInstance
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createReplicationInstanceAsync(
CreateReplicationInstanceRequest createReplicationInstanceRequest);
/**
*
* Creates the replication instance using the specified parameters.
*
*
* DMS requires that your account have certain roles with appropriate permissions before you can create a
* replication instance. For information on the required roles, see Creating the IAM
* Roles to Use With the CLI and DMS API. For information on the required permissions, see IAM
* Permissions Needed to Use DMS.
*
*
*
* If you don't specify a version when creating a replication instance, DMS will create the instance using the
* default engine version. For information about the default engine version, see Release Notes.
*
*
*
* @param createReplicationInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateReplicationInstance operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.CreateReplicationInstance
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createReplicationInstanceAsync(
CreateReplicationInstanceRequest createReplicationInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a replication subnet group given a list of the subnet IDs in a VPC.
*
*
* The VPC needs to have at least one subnet in at least two availability zones in the Amazon Web Services Region,
* otherwise the service will throw a ReplicationSubnetGroupDoesNotCoverEnoughAZs
exception.
*
*
* If a replication subnet group exists in your Amazon Web Services account, the CreateReplicationSubnetGroup action
* returns the following error message: The Replication Subnet Group already exists. In this case, delete the
* existing replication subnet group. To do so, use the DeleteReplicationSubnetGroup action. Optionally, choose Subnet groups in the DMS console, then choose your
* subnet group. Next, choose Delete from Actions.
*
*
* @param createReplicationSubnetGroupRequest
* @return A Java Future containing the result of the CreateReplicationSubnetGroup operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.CreateReplicationSubnetGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future createReplicationSubnetGroupAsync(
CreateReplicationSubnetGroupRequest createReplicationSubnetGroupRequest);
/**
*
* Creates a replication subnet group given a list of the subnet IDs in a VPC.
*
*
* The VPC needs to have at least one subnet in at least two availability zones in the Amazon Web Services Region,
* otherwise the service will throw a ReplicationSubnetGroupDoesNotCoverEnoughAZs
exception.
*
*
* If a replication subnet group exists in your Amazon Web Services account, the CreateReplicationSubnetGroup action
* returns the following error message: The Replication Subnet Group already exists. In this case, delete the
* existing replication subnet group. To do so, use the DeleteReplicationSubnetGroup action. Optionally, choose Subnet groups in the DMS console, then choose your
* subnet group. Next, choose Delete from Actions.
*
*
* @param createReplicationSubnetGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateReplicationSubnetGroup operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.CreateReplicationSubnetGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future createReplicationSubnetGroupAsync(
CreateReplicationSubnetGroupRequest createReplicationSubnetGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a replication task using the specified parameters.
*
*
* @param createReplicationTaskRequest
* @return A Java Future containing the result of the CreateReplicationTask operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.CreateReplicationTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createReplicationTaskAsync(CreateReplicationTaskRequest createReplicationTaskRequest);
/**
*
* Creates a replication task using the specified parameters.
*
*
* @param createReplicationTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateReplicationTask operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.CreateReplicationTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createReplicationTaskAsync(CreateReplicationTaskRequest createReplicationTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified certificate.
*
*
* @param deleteCertificateRequest
* @return A Java Future containing the result of the DeleteCertificate operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DeleteCertificate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteCertificateAsync(DeleteCertificateRequest deleteCertificateRequest);
/**
*
* Deletes the specified certificate.
*
*
* @param deleteCertificateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteCertificate operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DeleteCertificate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteCertificateAsync(DeleteCertificateRequest deleteCertificateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the connection between a replication instance and an endpoint.
*
*
* @param deleteConnectionRequest
* @return A Java Future containing the result of the DeleteConnection operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DeleteConnection
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteConnectionAsync(DeleteConnectionRequest deleteConnectionRequest);
/**
*
* Deletes the connection between a replication instance and an endpoint.
*
*
* @param deleteConnectionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteConnection operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DeleteConnection
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteConnectionAsync(DeleteConnectionRequest deleteConnectionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified data provider.
*
*
*
* All migration projects associated with the data provider must be deleted or modified before you can delete the
* data provider.
*
*
*
* @param deleteDataProviderRequest
* @return A Java Future containing the result of the DeleteDataProvider operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DeleteDataProvider
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDataProviderAsync(DeleteDataProviderRequest deleteDataProviderRequest);
/**
*
* Deletes the specified data provider.
*
*
*
* All migration projects associated with the data provider must be deleted or modified before you can delete the
* data provider.
*
*
*
* @param deleteDataProviderRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteDataProvider operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DeleteDataProvider
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteDataProviderAsync(DeleteDataProviderRequest deleteDataProviderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified endpoint.
*
*
*
* All tasks associated with the endpoint must be deleted before you can delete the endpoint.
*
*
*
*
* @param deleteEndpointRequest
* @return A Java Future containing the result of the DeleteEndpoint operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DeleteEndpoint
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteEndpointAsync(DeleteEndpointRequest deleteEndpointRequest);
/**
*
* Deletes the specified endpoint.
*
*
*
* All tasks associated with the endpoint must be deleted before you can delete the endpoint.
*
*
*
*
* @param deleteEndpointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteEndpoint operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DeleteEndpoint
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteEndpointAsync(DeleteEndpointRequest deleteEndpointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an DMS event subscription.
*
*
* @param deleteEventSubscriptionRequest
* @return A Java Future containing the result of the DeleteEventSubscription operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DeleteEventSubscription
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteEventSubscriptionAsync(DeleteEventSubscriptionRequest deleteEventSubscriptionRequest);
/**
*
* Deletes an DMS event subscription.
*
*
* @param deleteEventSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteEventSubscription operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DeleteEventSubscription
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteEventSubscriptionAsync(DeleteEventSubscriptionRequest deleteEventSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified Fleet Advisor collector.
*
*
* @param deleteFleetAdvisorCollectorRequest
* @return A Java Future containing the result of the DeleteFleetAdvisorCollector operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DeleteFleetAdvisorCollector
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteFleetAdvisorCollectorAsync(
DeleteFleetAdvisorCollectorRequest deleteFleetAdvisorCollectorRequest);
/**
*
* Deletes the specified Fleet Advisor collector.
*
*
* @param deleteFleetAdvisorCollectorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteFleetAdvisorCollector operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DeleteFleetAdvisorCollector
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteFleetAdvisorCollectorAsync(
DeleteFleetAdvisorCollectorRequest deleteFleetAdvisorCollectorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified Fleet Advisor collector databases.
*
*
* @param deleteFleetAdvisorDatabasesRequest
* @return A Java Future containing the result of the DeleteFleetAdvisorDatabases operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DeleteFleetAdvisorDatabases
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteFleetAdvisorDatabasesAsync(
DeleteFleetAdvisorDatabasesRequest deleteFleetAdvisorDatabasesRequest);
/**
*
* Deletes the specified Fleet Advisor collector databases.
*
*
* @param deleteFleetAdvisorDatabasesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteFleetAdvisorDatabases operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DeleteFleetAdvisorDatabases
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteFleetAdvisorDatabasesAsync(
DeleteFleetAdvisorDatabasesRequest deleteFleetAdvisorDatabasesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified instance profile.
*
*
*
* All migration projects associated with the instance profile must be deleted or modified before you can delete the
* instance profile.
*
*
*
* @param deleteInstanceProfileRequest
* @return A Java Future containing the result of the DeleteInstanceProfile operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DeleteInstanceProfile
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteInstanceProfileAsync(DeleteInstanceProfileRequest deleteInstanceProfileRequest);
/**
*
* Deletes the specified instance profile.
*
*
*
* All migration projects associated with the instance profile must be deleted or modified before you can delete the
* instance profile.
*
*
*
* @param deleteInstanceProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteInstanceProfile operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DeleteInstanceProfile
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteInstanceProfileAsync(DeleteInstanceProfileRequest deleteInstanceProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified migration project.
*
*
*
* The migration project must be closed before you can delete it.
*
*
*
* @param deleteMigrationProjectRequest
* @return A Java Future containing the result of the DeleteMigrationProject operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DeleteMigrationProject
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteMigrationProjectAsync(DeleteMigrationProjectRequest deleteMigrationProjectRequest);
/**
*
* Deletes the specified migration project.
*
*
*
* The migration project must be closed before you can delete it.
*
*
*
* @param deleteMigrationProjectRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteMigrationProject operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DeleteMigrationProject
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteMigrationProjectAsync(DeleteMigrationProjectRequest deleteMigrationProjectRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an DMS Serverless replication configuration. This effectively deprovisions any and all replications that
* use this configuration. You can't delete the configuration for an DMS Serverless replication that is ongoing. You
* can delete the configuration when the replication is in a non-RUNNING and non-STARTING state.
*
*
* @param deleteReplicationConfigRequest
* @return A Java Future containing the result of the DeleteReplicationConfig operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DeleteReplicationConfig
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteReplicationConfigAsync(DeleteReplicationConfigRequest deleteReplicationConfigRequest);
/**
*
* Deletes an DMS Serverless replication configuration. This effectively deprovisions any and all replications that
* use this configuration. You can't delete the configuration for an DMS Serverless replication that is ongoing. You
* can delete the configuration when the replication is in a non-RUNNING and non-STARTING state.
*
*
* @param deleteReplicationConfigRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteReplicationConfig operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DeleteReplicationConfig
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteReplicationConfigAsync(DeleteReplicationConfigRequest deleteReplicationConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified replication instance.
*
*
*
* You must delete any migration tasks that are associated with the replication instance before you can delete it.
*
*
*
*
* @param deleteReplicationInstanceRequest
* @return A Java Future containing the result of the DeleteReplicationInstance operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DeleteReplicationInstance
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteReplicationInstanceAsync(
DeleteReplicationInstanceRequest deleteReplicationInstanceRequest);
/**
*
* Deletes the specified replication instance.
*
*
*
* You must delete any migration tasks that are associated with the replication instance before you can delete it.
*
*
*
*
* @param deleteReplicationInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteReplicationInstance operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DeleteReplicationInstance
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteReplicationInstanceAsync(
DeleteReplicationInstanceRequest deleteReplicationInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a subnet group.
*
*
* @param deleteReplicationSubnetGroupRequest
* @return A Java Future containing the result of the DeleteReplicationSubnetGroup operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.DeleteReplicationSubnetGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteReplicationSubnetGroupAsync(
DeleteReplicationSubnetGroupRequest deleteReplicationSubnetGroupRequest);
/**
*
* Deletes a subnet group.
*
*
* @param deleteReplicationSubnetGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteReplicationSubnetGroup operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DeleteReplicationSubnetGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteReplicationSubnetGroupAsync(
DeleteReplicationSubnetGroupRequest deleteReplicationSubnetGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified replication task.
*
*
* @param deleteReplicationTaskRequest
* @return A Java Future containing the result of the DeleteReplicationTask operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DeleteReplicationTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteReplicationTaskAsync(DeleteReplicationTaskRequest deleteReplicationTaskRequest);
/**
*
* Deletes the specified replication task.
*
*
* @param deleteReplicationTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteReplicationTask operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DeleteReplicationTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteReplicationTaskAsync(DeleteReplicationTaskRequest deleteReplicationTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the record of a single premigration assessment run.
*
*
* This operation removes all metadata that DMS maintains about this assessment run. However, the operation leaves
* untouched all information about this assessment run that is stored in your Amazon S3 bucket.
*
*
* @param deleteReplicationTaskAssessmentRunRequest
* @return A Java Future containing the result of the DeleteReplicationTaskAssessmentRun operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.DeleteReplicationTaskAssessmentRun
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteReplicationTaskAssessmentRunAsync(
DeleteReplicationTaskAssessmentRunRequest deleteReplicationTaskAssessmentRunRequest);
/**
*
* Deletes the record of a single premigration assessment run.
*
*
* This operation removes all metadata that DMS maintains about this assessment run. However, the operation leaves
* untouched all information about this assessment run that is stored in your Amazon S3 bucket.
*
*
* @param deleteReplicationTaskAssessmentRunRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteReplicationTaskAssessmentRun operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DeleteReplicationTaskAssessmentRun
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteReplicationTaskAssessmentRunAsync(
DeleteReplicationTaskAssessmentRunRequest deleteReplicationTaskAssessmentRunRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all of the DMS attributes for a customer account. These attributes include DMS quotas for the account and a
* unique account identifier in a particular DMS region. DMS quotas include a list of resource quotas supported by
* the account, such as the number of replication instances allowed. The description for each resource quota,
* includes the quota name, current usage toward that quota, and the quota's maximum value. DMS uses the unique
* account identifier to name each artifact used by DMS in the given region.
*
*
* This command does not take any parameters.
*
*
* @param describeAccountAttributesRequest
* @return A Java Future containing the result of the DescribeAccountAttributes operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeAccountAttributes
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeAccountAttributesAsync(
DescribeAccountAttributesRequest describeAccountAttributesRequest);
/**
*
* Lists all of the DMS attributes for a customer account. These attributes include DMS quotas for the account and a
* unique account identifier in a particular DMS region. DMS quotas include a list of resource quotas supported by
* the account, such as the number of replication instances allowed. The description for each resource quota,
* includes the quota name, current usage toward that quota, and the quota's maximum value. DMS uses the unique
* account identifier to name each artifact used by DMS in the given region.
*
*
* This command does not take any parameters.
*
*
* @param describeAccountAttributesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAccountAttributes operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeAccountAttributes
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeAccountAttributesAsync(
DescribeAccountAttributesRequest describeAccountAttributesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides a list of individual assessments that you can specify for a new premigration assessment run, given one
* or more parameters.
*
*
* If you specify an existing migration task, this operation provides the default individual assessments you can
* specify for that task. Otherwise, the specified parameters model elements of a possible migration task on which
* to base a premigration assessment run.
*
*
* To use these migration task modeling parameters, you must specify an existing replication instance, a source
* database engine, a target database engine, and a migration type. This combination of parameters potentially
* limits the default individual assessments available for an assessment run created for a corresponding migration
* task.
*
*
* If you specify no parameters, this operation provides a list of all possible individual assessments that you can
* specify for an assessment run. If you specify any one of the task modeling parameters, you must specify all of
* them or the operation cannot provide a list of individual assessments. The only parameter that you can specify
* alone is for an existing migration task. The specified task definition then determines the default list of
* individual assessments that you can specify in an assessment run for the task.
*
*
* @param describeApplicableIndividualAssessmentsRequest
* @return A Java Future containing the result of the DescribeApplicableIndividualAssessments operation returned by
* the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeApplicableIndividualAssessments
* @see AWS API Documentation
*/
java.util.concurrent.Future describeApplicableIndividualAssessmentsAsync(
DescribeApplicableIndividualAssessmentsRequest describeApplicableIndividualAssessmentsRequest);
/**
*
* Provides a list of individual assessments that you can specify for a new premigration assessment run, given one
* or more parameters.
*
*
* If you specify an existing migration task, this operation provides the default individual assessments you can
* specify for that task. Otherwise, the specified parameters model elements of a possible migration task on which
* to base a premigration assessment run.
*
*
* To use these migration task modeling parameters, you must specify an existing replication instance, a source
* database engine, a target database engine, and a migration type. This combination of parameters potentially
* limits the default individual assessments available for an assessment run created for a corresponding migration
* task.
*
*
* If you specify no parameters, this operation provides a list of all possible individual assessments that you can
* specify for an assessment run. If you specify any one of the task modeling parameters, you must specify all of
* them or the operation cannot provide a list of individual assessments. The only parameter that you can specify
* alone is for an existing migration task. The specified task definition then determines the default list of
* individual assessments that you can specify in an assessment run for the task.
*
*
* @param describeApplicableIndividualAssessmentsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeApplicableIndividualAssessments operation returned by
* the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeApplicableIndividualAssessments
* @see AWS API Documentation
*/
java.util.concurrent.Future describeApplicableIndividualAssessmentsAsync(
DescribeApplicableIndividualAssessmentsRequest describeApplicableIndividualAssessmentsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides a description of the certificate.
*
*
* @param describeCertificatesRequest
* @return A Java Future containing the result of the DescribeCertificates operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeCertificates
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeCertificatesAsync(DescribeCertificatesRequest describeCertificatesRequest);
/**
*
* Provides a description of the certificate.
*
*
* @param describeCertificatesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeCertificates operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeCertificates
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeCertificatesAsync(DescribeCertificatesRequest describeCertificatesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the status of the connections that have been made between the replication instance and an endpoint.
* Connections are created when you test an endpoint.
*
*
* @param describeConnectionsRequest
* @return A Java Future containing the result of the DescribeConnections operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeConnections
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeConnectionsAsync(DescribeConnectionsRequest describeConnectionsRequest);
/**
*
* Describes the status of the connections that have been made between the replication instance and an endpoint.
* Connections are created when you test an endpoint.
*
*
* @param describeConnectionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeConnections operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeConnections
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeConnectionsAsync(DescribeConnectionsRequest describeConnectionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns configuration parameters for a schema conversion project.
*
*
* @param describeConversionConfigurationRequest
* @return A Java Future containing the result of the DescribeConversionConfiguration operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeConversionConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future describeConversionConfigurationAsync(
DescribeConversionConfigurationRequest describeConversionConfigurationRequest);
/**
*
* Returns configuration parameters for a schema conversion project.
*
*
* @param describeConversionConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeConversionConfiguration operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeConversionConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future describeConversionConfigurationAsync(
DescribeConversionConfigurationRequest describeConversionConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a paginated list of data providers for your account in the current region.
*
*
* @param describeDataProvidersRequest
* @return A Java Future containing the result of the DescribeDataProviders operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeDataProviders
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDataProvidersAsync(DescribeDataProvidersRequest describeDataProvidersRequest);
/**
*
* Returns a paginated list of data providers for your account in the current region.
*
*
* @param describeDataProvidersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeDataProviders operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeDataProviders
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeDataProvidersAsync(DescribeDataProvidersRequest describeDataProvidersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the possible endpoint settings available when you create an endpoint for a specific
* database engine.
*
*
* @param describeEndpointSettingsRequest
* @return A Java Future containing the result of the DescribeEndpointSettings operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeEndpointSettings
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeEndpointSettingsAsync(DescribeEndpointSettingsRequest describeEndpointSettingsRequest);
/**
*
* Returns information about the possible endpoint settings available when you create an endpoint for a specific
* database engine.
*
*
* @param describeEndpointSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEndpointSettings operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeEndpointSettings
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeEndpointSettingsAsync(DescribeEndpointSettingsRequest describeEndpointSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the type of endpoints available.
*
*
* @param describeEndpointTypesRequest
* @return A Java Future containing the result of the DescribeEndpointTypes operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeEndpointTypes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeEndpointTypesAsync(DescribeEndpointTypesRequest describeEndpointTypesRequest);
/**
*
* Returns information about the type of endpoints available.
*
*
* @param describeEndpointTypesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEndpointTypes operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeEndpointTypes
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeEndpointTypesAsync(DescribeEndpointTypesRequest describeEndpointTypesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the endpoints for your account in the current region.
*
*
* @param describeEndpointsRequest
* @return A Java Future containing the result of the DescribeEndpoints operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeEndpoints
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeEndpointsAsync(DescribeEndpointsRequest describeEndpointsRequest);
/**
*
* Returns information about the endpoints for your account in the current region.
*
*
* @param describeEndpointsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEndpoints operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeEndpoints
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeEndpointsAsync(DescribeEndpointsRequest describeEndpointsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the replication instance versions used in the project.
*
*
* @param describeEngineVersionsRequest
* @return A Java Future containing the result of the DescribeEngineVersions operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeEngineVersions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeEngineVersionsAsync(DescribeEngineVersionsRequest describeEngineVersionsRequest);
/**
*
* Returns information about the replication instance versions used in the project.
*
*
* @param describeEngineVersionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEngineVersions operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeEngineVersions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeEngineVersionsAsync(DescribeEngineVersionsRequest describeEngineVersionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists categories for all event source types, or, if specified, for a specified source type. You can see a list of
* the event categories and source types in Working with Events and
* Notifications in the Database Migration Service User Guide.
*
*
* @param describeEventCategoriesRequest
* @return A Java Future containing the result of the DescribeEventCategories operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeEventCategories
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeEventCategoriesAsync(DescribeEventCategoriesRequest describeEventCategoriesRequest);
/**
*
* Lists categories for all event source types, or, if specified, for a specified source type. You can see a list of
* the event categories and source types in Working with Events and
* Notifications in the Database Migration Service User Guide.
*
*
* @param describeEventCategoriesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEventCategories operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeEventCategories
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeEventCategoriesAsync(DescribeEventCategoriesRequest describeEventCategoriesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all the event subscriptions for a customer account. The description of a subscription includes
* SubscriptionName
, SNSTopicARN
, CustomerID
, SourceType
,
* SourceID
, CreationTime
, and Status
.
*
*
* If you specify SubscriptionName
, this action lists the description for that subscription.
*
*
* @param describeEventSubscriptionsRequest
* @return A Java Future containing the result of the DescribeEventSubscriptions operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeEventSubscriptions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeEventSubscriptionsAsync(
DescribeEventSubscriptionsRequest describeEventSubscriptionsRequest);
/**
*
* Lists all the event subscriptions for a customer account. The description of a subscription includes
* SubscriptionName
, SNSTopicARN
, CustomerID
, SourceType
,
* SourceID
, CreationTime
, and Status
.
*
*
* If you specify SubscriptionName
, this action lists the description for that subscription.
*
*
* @param describeEventSubscriptionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEventSubscriptions operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeEventSubscriptions
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeEventSubscriptionsAsync(
DescribeEventSubscriptionsRequest describeEventSubscriptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists events for a given source identifier and source type. You can also specify a start and end time. For more
* information on DMS events, see Working with Events and
* Notifications in the Database Migration Service User Guide.
*
*
* @param describeEventsRequest
* @return A Java Future containing the result of the DescribeEvents operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeEvents
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeEventsAsync(DescribeEventsRequest describeEventsRequest);
/**
*
* Lists events for a given source identifier and source type. You can also specify a start and end time. For more
* information on DMS events, see Working with Events and
* Notifications in the Database Migration Service User Guide.
*
*
* @param describeEventsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEvents operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeEvents
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeEventsAsync(DescribeEventsRequest describeEventsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a paginated list of extension pack associations for the specified migration project. An extension pack is
* an add-on module that emulates functions present in a source database that are required when converting objects
* to the target database.
*
*
* @param describeExtensionPackAssociationsRequest
* @return A Java Future containing the result of the DescribeExtensionPackAssociations operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeExtensionPackAssociations
* @see AWS API Documentation
*/
java.util.concurrent.Future describeExtensionPackAssociationsAsync(
DescribeExtensionPackAssociationsRequest describeExtensionPackAssociationsRequest);
/**
*
* Returns a paginated list of extension pack associations for the specified migration project. An extension pack is
* an add-on module that emulates functions present in a source database that are required when converting objects
* to the target database.
*
*
* @param describeExtensionPackAssociationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeExtensionPackAssociations operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeExtensionPackAssociations
* @see AWS API Documentation
*/
java.util.concurrent.Future describeExtensionPackAssociationsAsync(
DescribeExtensionPackAssociationsRequest describeExtensionPackAssociationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of the Fleet Advisor collectors in your account.
*
*
* @param describeFleetAdvisorCollectorsRequest
* @return A Java Future containing the result of the DescribeFleetAdvisorCollectors operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeFleetAdvisorCollectors
* @see AWS API Documentation
*/
java.util.concurrent.Future describeFleetAdvisorCollectorsAsync(
DescribeFleetAdvisorCollectorsRequest describeFleetAdvisorCollectorsRequest);
/**
*
* Returns a list of the Fleet Advisor collectors in your account.
*
*
* @param describeFleetAdvisorCollectorsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeFleetAdvisorCollectors operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeFleetAdvisorCollectors
* @see AWS API Documentation
*/
java.util.concurrent.Future describeFleetAdvisorCollectorsAsync(
DescribeFleetAdvisorCollectorsRequest describeFleetAdvisorCollectorsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of Fleet Advisor databases in your account.
*
*
* @param describeFleetAdvisorDatabasesRequest
* @return A Java Future containing the result of the DescribeFleetAdvisorDatabases operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeFleetAdvisorDatabases
* @see AWS API Documentation
*/
java.util.concurrent.Future describeFleetAdvisorDatabasesAsync(
DescribeFleetAdvisorDatabasesRequest describeFleetAdvisorDatabasesRequest);
/**
*
* Returns a list of Fleet Advisor databases in your account.
*
*
* @param describeFleetAdvisorDatabasesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeFleetAdvisorDatabases operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeFleetAdvisorDatabases
* @see AWS API Documentation
*/
java.util.concurrent.Future describeFleetAdvisorDatabasesAsync(
DescribeFleetAdvisorDatabasesRequest describeFleetAdvisorDatabasesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides descriptions of large-scale assessment (LSA) analyses produced by your Fleet Advisor collectors.
*
*
* @param describeFleetAdvisorLsaAnalysisRequest
* @return A Java Future containing the result of the DescribeFleetAdvisorLsaAnalysis operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeFleetAdvisorLsaAnalysis
* @see AWS API Documentation
*/
java.util.concurrent.Future describeFleetAdvisorLsaAnalysisAsync(
DescribeFleetAdvisorLsaAnalysisRequest describeFleetAdvisorLsaAnalysisRequest);
/**
*
* Provides descriptions of large-scale assessment (LSA) analyses produced by your Fleet Advisor collectors.
*
*
* @param describeFleetAdvisorLsaAnalysisRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeFleetAdvisorLsaAnalysis operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeFleetAdvisorLsaAnalysis
* @see AWS API Documentation
*/
java.util.concurrent.Future describeFleetAdvisorLsaAnalysisAsync(
DescribeFleetAdvisorLsaAnalysisRequest describeFleetAdvisorLsaAnalysisRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides descriptions of the schemas discovered by your Fleet Advisor collectors.
*
*
* @param describeFleetAdvisorSchemaObjectSummaryRequest
* @return A Java Future containing the result of the DescribeFleetAdvisorSchemaObjectSummary operation returned by
* the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeFleetAdvisorSchemaObjectSummary
* @see AWS API Documentation
*/
java.util.concurrent.Future describeFleetAdvisorSchemaObjectSummaryAsync(
DescribeFleetAdvisorSchemaObjectSummaryRequest describeFleetAdvisorSchemaObjectSummaryRequest);
/**
*
* Provides descriptions of the schemas discovered by your Fleet Advisor collectors.
*
*
* @param describeFleetAdvisorSchemaObjectSummaryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeFleetAdvisorSchemaObjectSummary operation returned by
* the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeFleetAdvisorSchemaObjectSummary
* @see AWS API Documentation
*/
java.util.concurrent.Future describeFleetAdvisorSchemaObjectSummaryAsync(
DescribeFleetAdvisorSchemaObjectSummaryRequest describeFleetAdvisorSchemaObjectSummaryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of schemas detected by Fleet Advisor Collectors in your account.
*
*
* @param describeFleetAdvisorSchemasRequest
* @return A Java Future containing the result of the DescribeFleetAdvisorSchemas operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeFleetAdvisorSchemas
* @see AWS API Documentation
*/
java.util.concurrent.Future describeFleetAdvisorSchemasAsync(
DescribeFleetAdvisorSchemasRequest describeFleetAdvisorSchemasRequest);
/**
*
* Returns a list of schemas detected by Fleet Advisor Collectors in your account.
*
*
* @param describeFleetAdvisorSchemasRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeFleetAdvisorSchemas operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeFleetAdvisorSchemas
* @see AWS API Documentation
*/
java.util.concurrent.Future describeFleetAdvisorSchemasAsync(
DescribeFleetAdvisorSchemasRequest describeFleetAdvisorSchemasRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a paginated list of instance profiles for your account in the current region.
*
*
* @param describeInstanceProfilesRequest
* @return A Java Future containing the result of the DescribeInstanceProfiles operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeInstanceProfiles
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeInstanceProfilesAsync(DescribeInstanceProfilesRequest describeInstanceProfilesRequest);
/**
*
* Returns a paginated list of instance profiles for your account in the current region.
*
*
* @param describeInstanceProfilesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeInstanceProfiles operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeInstanceProfiles
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeInstanceProfilesAsync(DescribeInstanceProfilesRequest describeInstanceProfilesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a paginated list of metadata model assessments for your account in the current region.
*
*
* @param describeMetadataModelAssessmentsRequest
* @return A Java Future containing the result of the DescribeMetadataModelAssessments operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeMetadataModelAssessments
* @see AWS API Documentation
*/
java.util.concurrent.Future describeMetadataModelAssessmentsAsync(
DescribeMetadataModelAssessmentsRequest describeMetadataModelAssessmentsRequest);
/**
*
* Returns a paginated list of metadata model assessments for your account in the current region.
*
*
* @param describeMetadataModelAssessmentsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeMetadataModelAssessments operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeMetadataModelAssessments
* @see AWS API Documentation
*/
java.util.concurrent.Future describeMetadataModelAssessmentsAsync(
DescribeMetadataModelAssessmentsRequest describeMetadataModelAssessmentsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a paginated list of metadata model conversions for a migration project.
*
*
* @param describeMetadataModelConversionsRequest
* @return A Java Future containing the result of the DescribeMetadataModelConversions operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeMetadataModelConversions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeMetadataModelConversionsAsync(
DescribeMetadataModelConversionsRequest describeMetadataModelConversionsRequest);
/**
*
* Returns a paginated list of metadata model conversions for a migration project.
*
*
* @param describeMetadataModelConversionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeMetadataModelConversions operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeMetadataModelConversions
* @see AWS API Documentation
*/
java.util.concurrent.Future describeMetadataModelConversionsAsync(
DescribeMetadataModelConversionsRequest describeMetadataModelConversionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a paginated list of metadata model exports.
*
*
* @param describeMetadataModelExportsAsScriptRequest
* @return A Java Future containing the result of the DescribeMetadataModelExportsAsScript operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeMetadataModelExportsAsScript
* @see AWS API Documentation
*/
java.util.concurrent.Future describeMetadataModelExportsAsScriptAsync(
DescribeMetadataModelExportsAsScriptRequest describeMetadataModelExportsAsScriptRequest);
/**
*
* Returns a paginated list of metadata model exports.
*
*
* @param describeMetadataModelExportsAsScriptRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeMetadataModelExportsAsScript operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeMetadataModelExportsAsScript
* @see AWS API Documentation
*/
java.util.concurrent.Future describeMetadataModelExportsAsScriptAsync(
DescribeMetadataModelExportsAsScriptRequest describeMetadataModelExportsAsScriptRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a paginated list of metadata model exports.
*
*
* @param describeMetadataModelExportsToTargetRequest
* @return A Java Future containing the result of the DescribeMetadataModelExportsToTarget operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeMetadataModelExportsToTarget
* @see AWS API Documentation
*/
java.util.concurrent.Future describeMetadataModelExportsToTargetAsync(
DescribeMetadataModelExportsToTargetRequest describeMetadataModelExportsToTargetRequest);
/**
*
* Returns a paginated list of metadata model exports.
*
*
* @param describeMetadataModelExportsToTargetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeMetadataModelExportsToTarget operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeMetadataModelExportsToTarget
* @see AWS API Documentation
*/
java.util.concurrent.Future describeMetadataModelExportsToTargetAsync(
DescribeMetadataModelExportsToTargetRequest describeMetadataModelExportsToTargetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a paginated list of metadata model imports.
*
*
* @param describeMetadataModelImportsRequest
* @return A Java Future containing the result of the DescribeMetadataModelImports operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeMetadataModelImports
* @see AWS API Documentation
*/
java.util.concurrent.Future describeMetadataModelImportsAsync(
DescribeMetadataModelImportsRequest describeMetadataModelImportsRequest);
/**
*
* Returns a paginated list of metadata model imports.
*
*
* @param describeMetadataModelImportsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeMetadataModelImports operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeMetadataModelImports
* @see AWS API Documentation
*/
java.util.concurrent.Future describeMetadataModelImportsAsync(
DescribeMetadataModelImportsRequest describeMetadataModelImportsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a paginated list of migration projects for your account in the current region.
*
*
* @param describeMigrationProjectsRequest
* @return A Java Future containing the result of the DescribeMigrationProjects operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeMigrationProjects
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeMigrationProjectsAsync(
DescribeMigrationProjectsRequest describeMigrationProjectsRequest);
/**
*
* Returns a paginated list of migration projects for your account in the current region.
*
*
* @param describeMigrationProjectsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeMigrationProjects operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeMigrationProjects
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeMigrationProjectsAsync(
DescribeMigrationProjectsRequest describeMigrationProjectsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the replication instance types that can be created in the specified region.
*
*
* @param describeOrderableReplicationInstancesRequest
* @return A Java Future containing the result of the DescribeOrderableReplicationInstances operation returned by
* the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeOrderableReplicationInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future describeOrderableReplicationInstancesAsync(
DescribeOrderableReplicationInstancesRequest describeOrderableReplicationInstancesRequest);
/**
*
* Returns information about the replication instance types that can be created in the specified region.
*
*
* @param describeOrderableReplicationInstancesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeOrderableReplicationInstances operation returned by
* the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeOrderableReplicationInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future describeOrderableReplicationInstancesAsync(
DescribeOrderableReplicationInstancesRequest describeOrderableReplicationInstancesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* For internal use only
*
*
* @param describePendingMaintenanceActionsRequest
* @return A Java Future containing the result of the DescribePendingMaintenanceActions operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.DescribePendingMaintenanceActions
* @see AWS API Documentation
*/
java.util.concurrent.Future describePendingMaintenanceActionsAsync(
DescribePendingMaintenanceActionsRequest describePendingMaintenanceActionsRequest);
/**
*
* For internal use only
*
*
* @param describePendingMaintenanceActionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribePendingMaintenanceActions operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribePendingMaintenanceActions
* @see AWS API Documentation
*/
java.util.concurrent.Future describePendingMaintenanceActionsAsync(
DescribePendingMaintenanceActionsRequest describePendingMaintenanceActionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a paginated list of limitations for recommendations of target Amazon Web Services engines.
*
*
* @param describeRecommendationLimitationsRequest
* @return A Java Future containing the result of the DescribeRecommendationLimitations operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeRecommendationLimitations
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRecommendationLimitationsAsync(
DescribeRecommendationLimitationsRequest describeRecommendationLimitationsRequest);
/**
*
* Returns a paginated list of limitations for recommendations of target Amazon Web Services engines.
*
*
* @param describeRecommendationLimitationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeRecommendationLimitations operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeRecommendationLimitations
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRecommendationLimitationsAsync(
DescribeRecommendationLimitationsRequest describeRecommendationLimitationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a paginated list of target engine recommendations for your source databases.
*
*
* @param describeRecommendationsRequest
* @return A Java Future containing the result of the DescribeRecommendations operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeRecommendations
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeRecommendationsAsync(DescribeRecommendationsRequest describeRecommendationsRequest);
/**
*
* Returns a paginated list of target engine recommendations for your source databases.
*
*
* @param describeRecommendationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeRecommendations operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeRecommendations
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeRecommendationsAsync(DescribeRecommendationsRequest describeRecommendationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the status of the RefreshSchemas operation.
*
*
* @param describeRefreshSchemasStatusRequest
* @return A Java Future containing the result of the DescribeRefreshSchemasStatus operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeRefreshSchemasStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRefreshSchemasStatusAsync(
DescribeRefreshSchemasStatusRequest describeRefreshSchemasStatusRequest);
/**
*
* Returns the status of the RefreshSchemas operation.
*
*
* @param describeRefreshSchemasStatusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeRefreshSchemasStatus operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeRefreshSchemasStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future describeRefreshSchemasStatusAsync(
DescribeRefreshSchemasStatusRequest describeRefreshSchemasStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns one or more existing DMS Serverless replication configurations as a list of structures.
*
*
* @param describeReplicationConfigsRequest
* @return A Java Future containing the result of the DescribeReplicationConfigs operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeReplicationConfigs
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeReplicationConfigsAsync(
DescribeReplicationConfigsRequest describeReplicationConfigsRequest);
/**
*
* Returns one or more existing DMS Serverless replication configurations as a list of structures.
*
*
* @param describeReplicationConfigsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeReplicationConfigs operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeReplicationConfigs
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeReplicationConfigsAsync(
DescribeReplicationConfigsRequest describeReplicationConfigsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the task logs for the specified task.
*
*
* @param describeReplicationInstanceTaskLogsRequest
* @return A Java Future containing the result of the DescribeReplicationInstanceTaskLogs operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeReplicationInstanceTaskLogs
* @see AWS API Documentation
*/
java.util.concurrent.Future describeReplicationInstanceTaskLogsAsync(
DescribeReplicationInstanceTaskLogsRequest describeReplicationInstanceTaskLogsRequest);
/**
*
* Returns information about the task logs for the specified task.
*
*
* @param describeReplicationInstanceTaskLogsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeReplicationInstanceTaskLogs operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeReplicationInstanceTaskLogs
* @see AWS API Documentation
*/
java.util.concurrent.Future describeReplicationInstanceTaskLogsAsync(
DescribeReplicationInstanceTaskLogsRequest describeReplicationInstanceTaskLogsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about replication instances for your account in the current region.
*
*
* @param describeReplicationInstancesRequest
* @return A Java Future containing the result of the DescribeReplicationInstances operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeReplicationInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future describeReplicationInstancesAsync(
DescribeReplicationInstancesRequest describeReplicationInstancesRequest);
/**
*
* Returns information about replication instances for your account in the current region.
*
*
* @param describeReplicationInstancesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeReplicationInstances operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeReplicationInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future describeReplicationInstancesAsync(
DescribeReplicationInstancesRequest describeReplicationInstancesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the replication subnet groups.
*
*
* @param describeReplicationSubnetGroupsRequest
* @return A Java Future containing the result of the DescribeReplicationSubnetGroups operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeReplicationSubnetGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future describeReplicationSubnetGroupsAsync(
DescribeReplicationSubnetGroupsRequest describeReplicationSubnetGroupsRequest);
/**
*
* Returns information about the replication subnet groups.
*
*
* @param describeReplicationSubnetGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeReplicationSubnetGroups operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeReplicationSubnetGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future describeReplicationSubnetGroupsAsync(
DescribeReplicationSubnetGroupsRequest describeReplicationSubnetGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns table and schema statistics for one or more provisioned replications that use a given DMS Serverless
* replication configuration.
*
*
* @param describeReplicationTableStatisticsRequest
* @return A Java Future containing the result of the DescribeReplicationTableStatistics operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeReplicationTableStatistics
* @see AWS API Documentation
*/
java.util.concurrent.Future describeReplicationTableStatisticsAsync(
DescribeReplicationTableStatisticsRequest describeReplicationTableStatisticsRequest);
/**
*
* Returns table and schema statistics for one or more provisioned replications that use a given DMS Serverless
* replication configuration.
*
*
* @param describeReplicationTableStatisticsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeReplicationTableStatistics operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeReplicationTableStatistics
* @see AWS API Documentation
*/
java.util.concurrent.Future describeReplicationTableStatisticsAsync(
DescribeReplicationTableStatisticsRequest describeReplicationTableStatisticsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the task assessment results from the Amazon S3 bucket that DMS creates in your Amazon Web Services
* account. This action always returns the latest results.
*
*
* For more information about DMS task assessments, see Creating a task
* assessment report in the Database Migration Service User Guide.
*
*
* @param describeReplicationTaskAssessmentResultsRequest
* @return A Java Future containing the result of the DescribeReplicationTaskAssessmentResults operation returned by
* the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeReplicationTaskAssessmentResults
* @see AWS API Documentation
*/
java.util.concurrent.Future describeReplicationTaskAssessmentResultsAsync(
DescribeReplicationTaskAssessmentResultsRequest describeReplicationTaskAssessmentResultsRequest);
/**
*
* Returns the task assessment results from the Amazon S3 bucket that DMS creates in your Amazon Web Services
* account. This action always returns the latest results.
*
*
* For more information about DMS task assessments, see Creating a task
* assessment report in the Database Migration Service User Guide.
*
*
* @param describeReplicationTaskAssessmentResultsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeReplicationTaskAssessmentResults operation returned by
* the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeReplicationTaskAssessmentResults
* @see AWS API Documentation
*/
java.util.concurrent.Future describeReplicationTaskAssessmentResultsAsync(
DescribeReplicationTaskAssessmentResultsRequest describeReplicationTaskAssessmentResultsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a paginated list of premigration assessment runs based on filter settings.
*
*
* These filter settings can specify a combination of premigration assessment runs, migration tasks, replication
* instances, and assessment run status values.
*
*
*
* This operation doesn't return information about individual assessments. For this information, see the
* DescribeReplicationTaskIndividualAssessments
operation.
*
*
*
* @param describeReplicationTaskAssessmentRunsRequest
* @return A Java Future containing the result of the DescribeReplicationTaskAssessmentRuns operation returned by
* the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeReplicationTaskAssessmentRuns
* @see AWS API Documentation
*/
java.util.concurrent.Future describeReplicationTaskAssessmentRunsAsync(
DescribeReplicationTaskAssessmentRunsRequest describeReplicationTaskAssessmentRunsRequest);
/**
*
* Returns a paginated list of premigration assessment runs based on filter settings.
*
*
* These filter settings can specify a combination of premigration assessment runs, migration tasks, replication
* instances, and assessment run status values.
*
*
*
* This operation doesn't return information about individual assessments. For this information, see the
* DescribeReplicationTaskIndividualAssessments
operation.
*
*
*
* @param describeReplicationTaskAssessmentRunsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeReplicationTaskAssessmentRuns operation returned by
* the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeReplicationTaskAssessmentRuns
* @see AWS API Documentation
*/
java.util.concurrent.Future describeReplicationTaskAssessmentRunsAsync(
DescribeReplicationTaskAssessmentRunsRequest describeReplicationTaskAssessmentRunsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a paginated list of individual assessments based on filter settings.
*
*
* These filter settings can specify a combination of premigration assessment runs, migration tasks, and assessment
* status values.
*
*
* @param describeReplicationTaskIndividualAssessmentsRequest
* @return A Java Future containing the result of the DescribeReplicationTaskIndividualAssessments operation
* returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeReplicationTaskIndividualAssessments
* @see AWS API Documentation
*/
java.util.concurrent.Future describeReplicationTaskIndividualAssessmentsAsync(
DescribeReplicationTaskIndividualAssessmentsRequest describeReplicationTaskIndividualAssessmentsRequest);
/**
*
* Returns a paginated list of individual assessments based on filter settings.
*
*
* These filter settings can specify a combination of premigration assessment runs, migration tasks, and assessment
* status values.
*
*
* @param describeReplicationTaskIndividualAssessmentsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeReplicationTaskIndividualAssessments operation
* returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeReplicationTaskIndividualAssessments
* @see AWS API Documentation
*/
java.util.concurrent.Future describeReplicationTaskIndividualAssessmentsAsync(
DescribeReplicationTaskIndividualAssessmentsRequest describeReplicationTaskIndividualAssessmentsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about replication tasks for your account in the current region.
*
*
* @param describeReplicationTasksRequest
* @return A Java Future containing the result of the DescribeReplicationTasks operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeReplicationTasks
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeReplicationTasksAsync(DescribeReplicationTasksRequest describeReplicationTasksRequest);
/**
*
* Returns information about replication tasks for your account in the current region.
*
*
* @param describeReplicationTasksRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeReplicationTasks operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeReplicationTasks
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeReplicationTasksAsync(DescribeReplicationTasksRequest describeReplicationTasksRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides details on replication progress by returning status information for one or more provisioned DMS
* Serverless replications.
*
*
* @param describeReplicationsRequest
* @return A Java Future containing the result of the DescribeReplications operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeReplications
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeReplicationsAsync(DescribeReplicationsRequest describeReplicationsRequest);
/**
*
* Provides details on replication progress by returning status information for one or more provisioned DMS
* Serverless replications.
*
*
* @param describeReplicationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeReplications operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeReplications
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeReplicationsAsync(DescribeReplicationsRequest describeReplicationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about the schema for the specified endpoint.
*
*
*
* @param describeSchemasRequest
* @return A Java Future containing the result of the DescribeSchemas operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeSchemas
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeSchemasAsync(DescribeSchemasRequest describeSchemasRequest);
/**
*
* Returns information about the schema for the specified endpoint.
*
*
*
* @param describeSchemasRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeSchemas operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeSchemas
* @see AWS API
* Documentation
*/
java.util.concurrent.Future describeSchemasAsync(DescribeSchemasRequest describeSchemasRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns table statistics on the database migration task, including table name, rows inserted, rows updated, and
* rows deleted.
*
*
* Note that the "last updated" column the DMS console only indicates the time that DMS last updated the table
* statistics record for a table. It does not indicate the time of the last update to the table.
*
*
* @param describeTableStatisticsRequest
* @return A Java Future containing the result of the DescribeTableStatistics operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.DescribeTableStatistics
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeTableStatisticsAsync(DescribeTableStatisticsRequest describeTableStatisticsRequest);
/**
*
* Returns table statistics on the database migration task, including table name, rows inserted, rows updated, and
* rows deleted.
*
*
* Note that the "last updated" column the DMS console only indicates the time that DMS last updated the table
* statistics record for a table. It does not indicate the time of the last update to the table.
*
*
* @param describeTableStatisticsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeTableStatistics operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.DescribeTableStatistics
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeTableStatisticsAsync(DescribeTableStatisticsRequest describeTableStatisticsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Saves a copy of a database migration assessment report to your Amazon S3 bucket. DMS can save your assessment
* report as a comma-separated value (CSV) or a PDF file.
*
*
* @param exportMetadataModelAssessmentRequest
* @return A Java Future containing the result of the ExportMetadataModelAssessment operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.ExportMetadataModelAssessment
* @see AWS API Documentation
*/
java.util.concurrent.Future exportMetadataModelAssessmentAsync(
ExportMetadataModelAssessmentRequest exportMetadataModelAssessmentRequest);
/**
*
* Saves a copy of a database migration assessment report to your Amazon S3 bucket. DMS can save your assessment
* report as a comma-separated value (CSV) or a PDF file.
*
*
* @param exportMetadataModelAssessmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ExportMetadataModelAssessment operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.ExportMetadataModelAssessment
* @see AWS API Documentation
*/
java.util.concurrent.Future exportMetadataModelAssessmentAsync(
ExportMetadataModelAssessmentRequest exportMetadataModelAssessmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Uploads the specified certificate.
*
*
* @param importCertificateRequest
* @return A Java Future containing the result of the ImportCertificate operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.ImportCertificate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future importCertificateAsync(ImportCertificateRequest importCertificateRequest);
/**
*
* Uploads the specified certificate.
*
*
* @param importCertificateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ImportCertificate operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.ImportCertificate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future importCertificateAsync(ImportCertificateRequest importCertificateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all metadata tags attached to an DMS resource, including replication instance, endpoint, subnet group, and
* migration task. For more information, see Tag
data type
* description.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.ListTagsForResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists all metadata tags attached to an DMS resource, including replication instance, endpoint, subnet group, and
* migration task. For more information, see Tag
data type
* description.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.ListTagsForResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the specified schema conversion configuration using the provided parameters.
*
*
* @param modifyConversionConfigurationRequest
* @return A Java Future containing the result of the ModifyConversionConfiguration operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.ModifyConversionConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future modifyConversionConfigurationAsync(
ModifyConversionConfigurationRequest modifyConversionConfigurationRequest);
/**
*
* Modifies the specified schema conversion configuration using the provided parameters.
*
*
* @param modifyConversionConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyConversionConfiguration operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.ModifyConversionConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future modifyConversionConfigurationAsync(
ModifyConversionConfigurationRequest modifyConversionConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the specified data provider using the provided settings.
*
*
*
* You must remove the data provider from all migration projects before you can modify it.
*
*
*
* @param modifyDataProviderRequest
* @return A Java Future containing the result of the ModifyDataProvider operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.ModifyDataProvider
* @see AWS API
* Documentation
*/
java.util.concurrent.Future modifyDataProviderAsync(ModifyDataProviderRequest modifyDataProviderRequest);
/**
*
* Modifies the specified data provider using the provided settings.
*
*
*
* You must remove the data provider from all migration projects before you can modify it.
*
*
*
* @param modifyDataProviderRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyDataProvider operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.ModifyDataProvider
* @see AWS API
* Documentation
*/
java.util.concurrent.Future modifyDataProviderAsync(ModifyDataProviderRequest modifyDataProviderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the specified endpoint.
*
*
*
* For a MySQL source or target endpoint, don't explicitly specify the database using the DatabaseName
* request parameter on the ModifyEndpoint
API call. Specifying DatabaseName
when you
* modify a MySQL endpoint replicates all the task tables to this single database. For MySQL endpoints, you specify
* the database only when you specify the schema in the table-mapping rules of the DMS task.
*
*
*
* @param modifyEndpointRequest
* @return A Java Future containing the result of the ModifyEndpoint operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.ModifyEndpoint
* @see AWS API
* Documentation
*/
java.util.concurrent.Future modifyEndpointAsync(ModifyEndpointRequest modifyEndpointRequest);
/**
*
* Modifies the specified endpoint.
*
*
*
* For a MySQL source or target endpoint, don't explicitly specify the database using the DatabaseName
* request parameter on the ModifyEndpoint
API call. Specifying DatabaseName
when you
* modify a MySQL endpoint replicates all the task tables to this single database. For MySQL endpoints, you specify
* the database only when you specify the schema in the table-mapping rules of the DMS task.
*
*
*
* @param modifyEndpointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyEndpoint operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.ModifyEndpoint
* @see AWS API
* Documentation
*/
java.util.concurrent.Future modifyEndpointAsync(ModifyEndpointRequest modifyEndpointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies an existing DMS event notification subscription.
*
*
* @param modifyEventSubscriptionRequest
* @return A Java Future containing the result of the ModifyEventSubscription operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.ModifyEventSubscription
* @see AWS
* API Documentation
*/
java.util.concurrent.Future modifyEventSubscriptionAsync(ModifyEventSubscriptionRequest modifyEventSubscriptionRequest);
/**
*
* Modifies an existing DMS event notification subscription.
*
*
* @param modifyEventSubscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyEventSubscription operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.ModifyEventSubscription
* @see AWS
* API Documentation
*/
java.util.concurrent.Future modifyEventSubscriptionAsync(ModifyEventSubscriptionRequest modifyEventSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the specified instance profile using the provided parameters.
*
*
*
* All migration projects associated with the instance profile must be deleted or modified before you can modify the
* instance profile.
*
*
*
* @param modifyInstanceProfileRequest
* @return A Java Future containing the result of the ModifyInstanceProfile operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.ModifyInstanceProfile
* @see AWS API
* Documentation
*/
java.util.concurrent.Future modifyInstanceProfileAsync(ModifyInstanceProfileRequest modifyInstanceProfileRequest);
/**
*
* Modifies the specified instance profile using the provided parameters.
*
*
*
* All migration projects associated with the instance profile must be deleted or modified before you can modify the
* instance profile.
*
*
*
* @param modifyInstanceProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyInstanceProfile operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.ModifyInstanceProfile
* @see AWS API
* Documentation
*/
java.util.concurrent.Future modifyInstanceProfileAsync(ModifyInstanceProfileRequest modifyInstanceProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the specified migration project using the provided parameters.
*
*
*
* The migration project must be closed before you can modify it.
*
*
*
* @param modifyMigrationProjectRequest
* @return A Java Future containing the result of the ModifyMigrationProject operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.ModifyMigrationProject
* @see AWS API
* Documentation
*/
java.util.concurrent.Future modifyMigrationProjectAsync(ModifyMigrationProjectRequest modifyMigrationProjectRequest);
/**
*
* Modifies the specified migration project using the provided parameters.
*
*
*
* The migration project must be closed before you can modify it.
*
*
*
* @param modifyMigrationProjectRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyMigrationProject operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.ModifyMigrationProject
* @see AWS API
* Documentation
*/
java.util.concurrent.Future modifyMigrationProjectAsync(ModifyMigrationProjectRequest modifyMigrationProjectRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies an existing DMS Serverless replication configuration that you can use to start a replication. This
* command includes input validation and logic to check the state of any replication that uses this configuration.
* You can only modify a replication configuration before any replication that uses it has started. As soon as you
* have initially started a replication with a given configuiration, you can't modify that configuration, even if
* you stop it.
*
*
* Other run statuses that allow you to run this command include FAILED and CREATED. A provisioning state that
* allows you to run this command is FAILED_PROVISION.
*
*
* @param modifyReplicationConfigRequest
* @return A Java Future containing the result of the ModifyReplicationConfig operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.ModifyReplicationConfig
* @see AWS
* API Documentation
*/
java.util.concurrent.Future modifyReplicationConfigAsync(ModifyReplicationConfigRequest modifyReplicationConfigRequest);
/**
*
* Modifies an existing DMS Serverless replication configuration that you can use to start a replication. This
* command includes input validation and logic to check the state of any replication that uses this configuration.
* You can only modify a replication configuration before any replication that uses it has started. As soon as you
* have initially started a replication with a given configuiration, you can't modify that configuration, even if
* you stop it.
*
*
* Other run statuses that allow you to run this command include FAILED and CREATED. A provisioning state that
* allows you to run this command is FAILED_PROVISION.
*
*
* @param modifyReplicationConfigRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyReplicationConfig operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.ModifyReplicationConfig
* @see AWS
* API Documentation
*/
java.util.concurrent.Future modifyReplicationConfigAsync(ModifyReplicationConfigRequest modifyReplicationConfigRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the replication instance to apply new settings. You can change one or more parameters by specifying
* these parameters and the new values in the request.
*
*
* Some settings are applied during the maintenance window.
*
*
*
* @param modifyReplicationInstanceRequest
* @return A Java Future containing the result of the ModifyReplicationInstance operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsync.ModifyReplicationInstance
* @see AWS
* API Documentation
*/
java.util.concurrent.Future modifyReplicationInstanceAsync(
ModifyReplicationInstanceRequest modifyReplicationInstanceRequest);
/**
*
* Modifies the replication instance to apply new settings. You can change one or more parameters by specifying
* these parameters and the new values in the request.
*
*
* Some settings are applied during the maintenance window.
*
*
*
* @param modifyReplicationInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyReplicationInstance operation returned by the service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.ModifyReplicationInstance
* @see AWS
* API Documentation
*/
java.util.concurrent.Future modifyReplicationInstanceAsync(
ModifyReplicationInstanceRequest modifyReplicationInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the settings for the specified replication subnet group.
*
*
* @param modifyReplicationSubnetGroupRequest
* @return A Java Future containing the result of the ModifyReplicationSubnetGroup operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsync.ModifyReplicationSubnetGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future modifyReplicationSubnetGroupAsync(
ModifyReplicationSubnetGroupRequest modifyReplicationSubnetGroupRequest);
/**
*
* Modifies the settings for the specified replication subnet group.
*
*
* @param modifyReplicationSubnetGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ModifyReplicationSubnetGroup operation returned by the
* service.
* @sample AWSDatabaseMigrationServiceAsyncHandler.ModifyReplicationSubnetGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future modifyReplicationSubnetGroupAsync(
ModifyReplicationSubnetGroupRequest modifyReplicationSubnetGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the specified replication task.
*
*
* You can't modify the task endpoints. The task must be stopped before you can modify it.
*
*
* For more information about DMS tasks, see