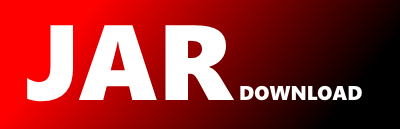
com.amazonaws.services.databasemigrationservice.model.ModifyDataProviderRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-dms Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.databasemigrationservice.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ModifyDataProviderRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The identifier of the data provider. Identifiers must begin with a letter and must contain only ASCII letters,
* digits, and hyphens. They can't end with a hyphen, or contain two consecutive hyphens.
*
*/
private String dataProviderIdentifier;
/**
*
* The name of the data provider.
*
*/
private String dataProviderName;
/**
*
* A user-friendly description of the data provider.
*
*/
private String description;
/**
*
* The type of database engine for the data provider. Valid values include "aurora"
,
* "aurora-postgresql"
, "mysql"
, "oracle"
, "postgres"
,
* "sqlserver"
, redshift
, mariadb
, mongodb
, and
* docdb
. A value of "aurora"
represents Amazon Aurora MySQL-Compatible Edition.
*
*/
private String engine;
/**
*
* If this attribute is Y, the current call to ModifyDataProvider
replaces all existing data provider
* settings with the exact settings that you specify in this call. If this attribute is N, the current call to
* ModifyDataProvider
does two things:
*
*
* -
*
* It replaces any data provider settings that already exist with new values, for settings with the same names.
*
*
* -
*
* It creates new data provider settings that you specify in the call, for settings with different names.
*
*
*
*/
private Boolean exactSettings;
/**
*
* The settings in JSON format for a data provider.
*
*/
private DataProviderSettings settings;
/**
*
* The identifier of the data provider. Identifiers must begin with a letter and must contain only ASCII letters,
* digits, and hyphens. They can't end with a hyphen, or contain two consecutive hyphens.
*
*
* @param dataProviderIdentifier
* The identifier of the data provider. Identifiers must begin with a letter and must contain only ASCII
* letters, digits, and hyphens. They can't end with a hyphen, or contain two consecutive hyphens.
*/
public void setDataProviderIdentifier(String dataProviderIdentifier) {
this.dataProviderIdentifier = dataProviderIdentifier;
}
/**
*
* The identifier of the data provider. Identifiers must begin with a letter and must contain only ASCII letters,
* digits, and hyphens. They can't end with a hyphen, or contain two consecutive hyphens.
*
*
* @return The identifier of the data provider. Identifiers must begin with a letter and must contain only ASCII
* letters, digits, and hyphens. They can't end with a hyphen, or contain two consecutive hyphens.
*/
public String getDataProviderIdentifier() {
return this.dataProviderIdentifier;
}
/**
*
* The identifier of the data provider. Identifiers must begin with a letter and must contain only ASCII letters,
* digits, and hyphens. They can't end with a hyphen, or contain two consecutive hyphens.
*
*
* @param dataProviderIdentifier
* The identifier of the data provider. Identifiers must begin with a letter and must contain only ASCII
* letters, digits, and hyphens. They can't end with a hyphen, or contain two consecutive hyphens.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDataProviderRequest withDataProviderIdentifier(String dataProviderIdentifier) {
setDataProviderIdentifier(dataProviderIdentifier);
return this;
}
/**
*
* The name of the data provider.
*
*
* @param dataProviderName
* The name of the data provider.
*/
public void setDataProviderName(String dataProviderName) {
this.dataProviderName = dataProviderName;
}
/**
*
* The name of the data provider.
*
*
* @return The name of the data provider.
*/
public String getDataProviderName() {
return this.dataProviderName;
}
/**
*
* The name of the data provider.
*
*
* @param dataProviderName
* The name of the data provider.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDataProviderRequest withDataProviderName(String dataProviderName) {
setDataProviderName(dataProviderName);
return this;
}
/**
*
* A user-friendly description of the data provider.
*
*
* @param description
* A user-friendly description of the data provider.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A user-friendly description of the data provider.
*
*
* @return A user-friendly description of the data provider.
*/
public String getDescription() {
return this.description;
}
/**
*
* A user-friendly description of the data provider.
*
*
* @param description
* A user-friendly description of the data provider.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDataProviderRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The type of database engine for the data provider. Valid values include "aurora"
,
* "aurora-postgresql"
, "mysql"
, "oracle"
, "postgres"
,
* "sqlserver"
, redshift
, mariadb
, mongodb
, and
* docdb
. A value of "aurora"
represents Amazon Aurora MySQL-Compatible Edition.
*
*
* @param engine
* The type of database engine for the data provider. Valid values include "aurora"
,
* "aurora-postgresql"
, "mysql"
, "oracle"
, "postgres"
,
* "sqlserver"
, redshift
, mariadb
, mongodb
, and
* docdb
. A value of "aurora"
represents Amazon Aurora MySQL-Compatible Edition.
*/
public void setEngine(String engine) {
this.engine = engine;
}
/**
*
* The type of database engine for the data provider. Valid values include "aurora"
,
* "aurora-postgresql"
, "mysql"
, "oracle"
, "postgres"
,
* "sqlserver"
, redshift
, mariadb
, mongodb
, and
* docdb
. A value of "aurora"
represents Amazon Aurora MySQL-Compatible Edition.
*
*
* @return The type of database engine for the data provider. Valid values include "aurora"
,
* "aurora-postgresql"
, "mysql"
, "oracle"
, "postgres"
,
* "sqlserver"
, redshift
, mariadb
, mongodb
, and
* docdb
. A value of "aurora"
represents Amazon Aurora MySQL-Compatible Edition.
*/
public String getEngine() {
return this.engine;
}
/**
*
* The type of database engine for the data provider. Valid values include "aurora"
,
* "aurora-postgresql"
, "mysql"
, "oracle"
, "postgres"
,
* "sqlserver"
, redshift
, mariadb
, mongodb
, and
* docdb
. A value of "aurora"
represents Amazon Aurora MySQL-Compatible Edition.
*
*
* @param engine
* The type of database engine for the data provider. Valid values include "aurora"
,
* "aurora-postgresql"
, "mysql"
, "oracle"
, "postgres"
,
* "sqlserver"
, redshift
, mariadb
, mongodb
, and
* docdb
. A value of "aurora"
represents Amazon Aurora MySQL-Compatible Edition.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDataProviderRequest withEngine(String engine) {
setEngine(engine);
return this;
}
/**
*
* If this attribute is Y, the current call to ModifyDataProvider
replaces all existing data provider
* settings with the exact settings that you specify in this call. If this attribute is N, the current call to
* ModifyDataProvider
does two things:
*
*
* -
*
* It replaces any data provider settings that already exist with new values, for settings with the same names.
*
*
* -
*
* It creates new data provider settings that you specify in the call, for settings with different names.
*
*
*
*
* @param exactSettings
* If this attribute is Y, the current call to ModifyDataProvider
replaces all existing data
* provider settings with the exact settings that you specify in this call. If this attribute is N, the
* current call to ModifyDataProvider
does two things:
*
* -
*
* It replaces any data provider settings that already exist with new values, for settings with the same
* names.
*
*
* -
*
* It creates new data provider settings that you specify in the call, for settings with different names.
*
*
*/
public void setExactSettings(Boolean exactSettings) {
this.exactSettings = exactSettings;
}
/**
*
* If this attribute is Y, the current call to ModifyDataProvider
replaces all existing data provider
* settings with the exact settings that you specify in this call. If this attribute is N, the current call to
* ModifyDataProvider
does two things:
*
*
* -
*
* It replaces any data provider settings that already exist with new values, for settings with the same names.
*
*
* -
*
* It creates new data provider settings that you specify in the call, for settings with different names.
*
*
*
*
* @return If this attribute is Y, the current call to ModifyDataProvider
replaces all existing data
* provider settings with the exact settings that you specify in this call. If this attribute is N, the
* current call to ModifyDataProvider
does two things:
*
* -
*
* It replaces any data provider settings that already exist with new values, for settings with the same
* names.
*
*
* -
*
* It creates new data provider settings that you specify in the call, for settings with different names.
*
*
*/
public Boolean getExactSettings() {
return this.exactSettings;
}
/**
*
* If this attribute is Y, the current call to ModifyDataProvider
replaces all existing data provider
* settings with the exact settings that you specify in this call. If this attribute is N, the current call to
* ModifyDataProvider
does two things:
*
*
* -
*
* It replaces any data provider settings that already exist with new values, for settings with the same names.
*
*
* -
*
* It creates new data provider settings that you specify in the call, for settings with different names.
*
*
*
*
* @param exactSettings
* If this attribute is Y, the current call to ModifyDataProvider
replaces all existing data
* provider settings with the exact settings that you specify in this call. If this attribute is N, the
* current call to ModifyDataProvider
does two things:
*
* -
*
* It replaces any data provider settings that already exist with new values, for settings with the same
* names.
*
*
* -
*
* It creates new data provider settings that you specify in the call, for settings with different names.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDataProviderRequest withExactSettings(Boolean exactSettings) {
setExactSettings(exactSettings);
return this;
}
/**
*
* If this attribute is Y, the current call to ModifyDataProvider
replaces all existing data provider
* settings with the exact settings that you specify in this call. If this attribute is N, the current call to
* ModifyDataProvider
does two things:
*
*
* -
*
* It replaces any data provider settings that already exist with new values, for settings with the same names.
*
*
* -
*
* It creates new data provider settings that you specify in the call, for settings with different names.
*
*
*
*
* @return If this attribute is Y, the current call to ModifyDataProvider
replaces all existing data
* provider settings with the exact settings that you specify in this call. If this attribute is N, the
* current call to ModifyDataProvider
does two things:
*
* -
*
* It replaces any data provider settings that already exist with new values, for settings with the same
* names.
*
*
* -
*
* It creates new data provider settings that you specify in the call, for settings with different names.
*
*
*/
public Boolean isExactSettings() {
return this.exactSettings;
}
/**
*
* The settings in JSON format for a data provider.
*
*
* @param settings
* The settings in JSON format for a data provider.
*/
public void setSettings(DataProviderSettings settings) {
this.settings = settings;
}
/**
*
* The settings in JSON format for a data provider.
*
*
* @return The settings in JSON format for a data provider.
*/
public DataProviderSettings getSettings() {
return this.settings;
}
/**
*
* The settings in JSON format for a data provider.
*
*
* @param settings
* The settings in JSON format for a data provider.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyDataProviderRequest withSettings(DataProviderSettings settings) {
setSettings(settings);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDataProviderIdentifier() != null)
sb.append("DataProviderIdentifier: ").append(getDataProviderIdentifier()).append(",");
if (getDataProviderName() != null)
sb.append("DataProviderName: ").append(getDataProviderName()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getEngine() != null)
sb.append("Engine: ").append(getEngine()).append(",");
if (getExactSettings() != null)
sb.append("ExactSettings: ").append(getExactSettings()).append(",");
if (getSettings() != null)
sb.append("Settings: ").append(getSettings());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ModifyDataProviderRequest == false)
return false;
ModifyDataProviderRequest other = (ModifyDataProviderRequest) obj;
if (other.getDataProviderIdentifier() == null ^ this.getDataProviderIdentifier() == null)
return false;
if (other.getDataProviderIdentifier() != null && other.getDataProviderIdentifier().equals(this.getDataProviderIdentifier()) == false)
return false;
if (other.getDataProviderName() == null ^ this.getDataProviderName() == null)
return false;
if (other.getDataProviderName() != null && other.getDataProviderName().equals(this.getDataProviderName()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getEngine() == null ^ this.getEngine() == null)
return false;
if (other.getEngine() != null && other.getEngine().equals(this.getEngine()) == false)
return false;
if (other.getExactSettings() == null ^ this.getExactSettings() == null)
return false;
if (other.getExactSettings() != null && other.getExactSettings().equals(this.getExactSettings()) == false)
return false;
if (other.getSettings() == null ^ this.getSettings() == null)
return false;
if (other.getSettings() != null && other.getSettings().equals(this.getSettings()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDataProviderIdentifier() == null) ? 0 : getDataProviderIdentifier().hashCode());
hashCode = prime * hashCode + ((getDataProviderName() == null) ? 0 : getDataProviderName().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getEngine() == null) ? 0 : getEngine().hashCode());
hashCode = prime * hashCode + ((getExactSettings() == null) ? 0 : getExactSettings().hashCode());
hashCode = prime * hashCode + ((getSettings() == null) ? 0 : getSettings().hashCode());
return hashCode;
}
@Override
public ModifyDataProviderRequest clone() {
return (ModifyDataProviderRequest) super.clone();
}
}