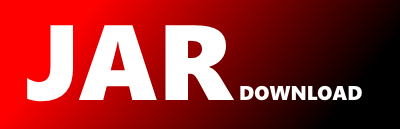
com.amazonaws.services.databasemigrationservice.model.OracleSettings Maven / Gradle / Ivy
Show all versions of aws-java-sdk-dms Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.databasemigrationservice.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Provides information that defines an Oracle endpoint.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class OracleSettings implements Serializable, Cloneable, StructuredPojo {
/**
*
* Set this attribute to set up table-level supplemental logging for the Oracle database. This attribute enables
* PRIMARY KEY supplemental logging on all tables selected for a migration task.
*
*
* If you use this option, you still need to enable database-level supplemental logging.
*
*/
private Boolean addSupplementalLogging;
/**
*
* Specifies the ID of the destination for the archived redo logs. This value should be the same as a number in the
* dest_id column of the v$archived_log view. If you work with an additional redo log destination, use the
* AdditionalArchivedLogDestId
option to specify the additional destination ID. Doing this improves
* performance by ensuring that the correct logs are accessed from the outset.
*
*/
private Integer archivedLogDestId;
/**
*
* Set this attribute with ArchivedLogDestId
in a primary/ standby setup. This attribute is useful in
* the case of a switchover. In this case, DMS needs to know which destination to get archive redo logs from to read
* changes. This need arises because the previous primary instance is now a standby instance after switchover.
*
*
* Although DMS supports the use of the Oracle RESETLOGS
option to open the database, never use
* RESETLOGS
unless necessary. For additional information about RESETLOGS
, see RMAN Data Repair Concepts in the Oracle Database Backup and Recovery User's Guide.
*
*/
private Integer additionalArchivedLogDestId;
/**
*
* Specifies the IDs of one more destinations for one or more archived redo logs. These IDs are the values of the
* dest_id
column in the v$archived_log
view. Use this setting with the
* archivedLogDestId
extra connection attribute in a primary-to-single setup or a
* primary-to-multiple-standby setup.
*
*
* This setting is useful in a switchover when you use an Oracle Data Guard database as a source. In this case, DMS
* needs information about what destination to get archive redo logs from to read changes. DMS needs this because
* after the switchover the previous primary is a standby instance. For example, in a primary-to-single standby
* setup you might apply the following settings.
*
*
* archivedLogDestId=1; ExtraArchivedLogDestIds=[2]
*
*
* In a primary-to-multiple-standby setup, you might apply the following settings.
*
*
* archivedLogDestId=1; ExtraArchivedLogDestIds=[2,3,4]
*
*
* Although DMS supports the use of the Oracle RESETLOGS
option to open the database, never use
* RESETLOGS
unless it's necessary. For more information about RESETLOGS
, see RMAN Data Repair Concepts in the Oracle Database Backup and Recovery User's Guide.
*
*/
private java.util.List extraArchivedLogDestIds;
/**
*
* Set this attribute to true
to enable replication of Oracle tables containing columns that are nested
* tables or defined types.
*
*/
private Boolean allowSelectNestedTables;
/**
*
* Set this attribute to change the number of threads that DMS configures to perform a change data capture (CDC)
* load using Oracle Automatic Storage Management (ASM). You can specify an integer value between 2 (the default)
* and 8 (the maximum). Use this attribute together with the readAheadBlocks
attribute.
*
*/
private Integer parallelAsmReadThreads;
/**
*
* Set this attribute to change the number of read-ahead blocks that DMS configures to perform a change data capture
* (CDC) load using Oracle Automatic Storage Management (ASM). You can specify an integer value between 1000 (the
* default) and 200,000 (the maximum).
*
*/
private Integer readAheadBlocks;
/**
*
* Set this attribute to false
in order to use the Binary Reader to capture change data for an Amazon
* RDS for Oracle as the source. This tells the DMS instance to not access redo logs through any specified path
* prefix replacement using direct file access.
*
*/
private Boolean accessAlternateDirectly;
/**
*
* Set this attribute to true
in order to use the Binary Reader to capture change data for an Amazon
* RDS for Oracle as the source. This tells the DMS instance to use any specified prefix replacement to access all
* online redo logs.
*
*/
private Boolean useAlternateFolderForOnline;
/**
*
* Set this string attribute to the required value in order to use the Binary Reader to capture change data for an
* Amazon RDS for Oracle as the source. This value specifies the default Oracle root used to access the redo logs.
*
*/
private String oraclePathPrefix;
/**
*
* Set this string attribute to the required value in order to use the Binary Reader to capture change data for an
* Amazon RDS for Oracle as the source. This value specifies the path prefix used to replace the default Oracle root
* to access the redo logs.
*
*/
private String usePathPrefix;
/**
*
* Set this attribute to true in order to use the Binary Reader to capture change data for an Amazon RDS for Oracle
* as the source. This setting tells DMS instance to replace the default Oracle root with the specified
* usePathPrefix
setting to access the redo logs.
*
*/
private Boolean replacePathPrefix;
/**
*
* Set this attribute to enable homogenous tablespace replication and create existing tables or indexes under the
* same tablespace on the target.
*
*/
private Boolean enableHomogenousTablespace;
/**
*
* When set to true
, this attribute helps to increase the commit rate on the Oracle target database by
* writing directly to tables and not writing a trail to database logs.
*
*/
private Boolean directPathNoLog;
/**
*
* When this field is set to Y
, DMS only accesses the archived redo logs. If the archived redo logs are
* stored on Automatic Storage Management (ASM) only, the DMS user account needs to be granted ASM privileges.
*
*/
private Boolean archivedLogsOnly;
/**
*
* For an Oracle source endpoint, your Oracle Automatic Storage Management (ASM) password. You can set this value
* from the asm_user_password
value. You set this value as part of the comma-separated value
* that you set to the Password
request parameter when you create the endpoint to access transaction
* logs using Binary Reader. For more information, see Configuration for change data capture (CDC) on an Oracle source database.
*
*/
private String asmPassword;
/**
*
* For an Oracle source endpoint, your ASM server address. You can set this value from the asm_server
* value. You set asm_server
as part of the extra connection attribute string to access an Oracle
* server with Binary Reader that uses ASM. For more information, see Configuration for change data capture (CDC) on an Oracle source database.
*
*/
private String asmServer;
/**
*
* For an Oracle source endpoint, your ASM user name. You can set this value from the asm_user
value.
* You set asm_user
as part of the extra connection attribute string to access an Oracle server with
* Binary Reader that uses ASM. For more information, see Configuration for change data capture (CDC) on an Oracle source database.
*
*/
private String asmUser;
/**
*
* Specifies whether the length of a character column is in bytes or in characters. To indicate that the character
* column length is in characters, set this attribute to CHAR
. Otherwise, the character column length
* is in bytes.
*
*
* Example: charLengthSemantics=CHAR;
*
*/
private String charLengthSemantics;
/**
*
* Database name for the endpoint.
*
*/
private String databaseName;
/**
*
* When set to true
, this attribute specifies a parallel load when useDirectPathFullLoad
* is set to Y
. This attribute also only applies when you use the DMS parallel load feature. Note that
* the target table cannot have any constraints or indexes.
*
*/
private Boolean directPathParallelLoad;
/**
*
* When set to true
, this attribute causes a task to fail if the actual size of an LOB column is
* greater than the specified LobMaxSize
.
*
*
* If a task is set to limited LOB mode and this option is set to true
, the task fails instead of
* truncating the LOB data.
*
*/
private Boolean failTasksOnLobTruncation;
/**
*
* Specifies the number scale. You can select a scale up to 38, or you can select FLOAT. By default, the NUMBER data
* type is converted to precision 38, scale 10.
*
*
* Example: numberDataTypeScale=12
*
*/
private Integer numberDatatypeScale;
/**
*
* Endpoint connection password.
*
*/
private String password;
/**
*
* Endpoint TCP port.
*
*/
private Integer port;
/**
*
* When set to true
, this attribute supports tablespace replication.
*
*/
private Boolean readTableSpaceName;
/**
*
* Specifies the number of seconds that the system waits before resending a query.
*
*
* Example: retryInterval=6;
*
*/
private Integer retryInterval;
/**
*
* For an Oracle source endpoint, the transparent data encryption (TDE) password required by AWM DMS to access
* Oracle redo logs encrypted by TDE using Binary Reader. It is also the TDE_Password
part of
* the comma-separated value you set to the Password
request parameter when you create the endpoint.
* The SecurityDbEncryptian
setting is related to this SecurityDbEncryptionName
setting.
* For more information, see
* Supported encryption methods for using Oracle as a source for DMS in the Database Migration Service User
* Guide.
*
*/
private String securityDbEncryption;
/**
*
* For an Oracle source endpoint, the name of a key used for the transparent data encryption (TDE) of the columns
* and tablespaces in an Oracle source database that is encrypted using TDE. The key value is the value of the
* SecurityDbEncryption
setting. For more information on setting the key name value of
* SecurityDbEncryptionName
, see the information and example for setting the
* securityDbEncryptionName
extra connection attribute in
* Supported encryption methods for using Oracle as a source for DMS in the Database Migration Service User
* Guide.
*
*/
private String securityDbEncryptionName;
/**
*
* Fully qualified domain name of the endpoint.
*
*
* For an Amazon RDS Oracle instance, this is the output of DescribeDBInstances, in the
* Endpoint.Address
* field.
*
*/
private String serverName;
/**
*
* Use this attribute to convert SDO_GEOMETRY
to GEOJSON
format. By default, DMS calls the
* SDO2GEOJSON
custom function if present and accessible. Or you can create your own custom function
* that mimics the operation of SDOGEOJSON
and set SpatialDataOptionToGeoJsonFunctionName
* to call it instead.
*
*/
private String spatialDataOptionToGeoJsonFunctionName;
/**
*
* Use this attribute to specify a time in minutes for the delay in standby sync. If the source is an Oracle Active
* Data Guard standby database, use this attribute to specify the time lag between primary and standby databases.
*
*
* In DMS, you can create an Oracle CDC task that uses an Active Data Guard standby instance as a source for
* replicating ongoing changes. Doing this eliminates the need to connect to an active database that might be in
* production.
*
*/
private Integer standbyDelayTime;
/**
*
* Endpoint connection user name.
*
*/
private String username;
/**
*
* Set this attribute to Y to capture change data using the Binary Reader utility. Set
* UseLogminerReader
to N to set this attribute to Y. To use Binary Reader with Amazon RDS for Oracle
* as the source, you set additional attributes. For more information about using this setting with Oracle Automatic
* Storage Management (ASM), see Using
* Oracle LogMiner or DMS Binary Reader for CDC.
*
*/
private Boolean useBFile;
/**
*
* Set this attribute to Y to have DMS use a direct path full load. Specify this value to use the direct path
* protocol in the Oracle Call Interface (OCI). By using this OCI protocol, you can bulk-load Oracle target tables
* during a full load.
*
*/
private Boolean useDirectPathFullLoad;
/**
*
* Set this attribute to Y to capture change data using the Oracle LogMiner utility (the default). Set this
* attribute to N if you want to access the redo logs as a binary file. When you set UseLogminerReader
* to N, also set UseBfile
to Y. For more information on this setting and using Oracle ASM, see Using
* Oracle LogMiner or DMS Binary Reader for CDC in the DMS User Guide.
*
*/
private Boolean useLogminerReader;
/**
*
* The full Amazon Resource Name (ARN) of the IAM role that specifies DMS as the trusted entity and grants the
* required permissions to access the value in SecretsManagerSecret
. The role must allow the
* iam:PassRole
action. SecretsManagerSecret
has the value of the Amazon Web Services
* Secrets Manager secret that allows access to the Oracle endpoint.
*
*
*
* You can specify one of two sets of values for these permissions. You can specify the values for this setting and
* SecretsManagerSecretId
. Or you can specify clear-text values for UserName
,
* Password
, ServerName
, and Port
. You can't specify both. For more
* information on creating this SecretsManagerSecret
and the SecretsManagerAccessRoleArn
* and SecretsManagerSecretId
required to access it, see Using
* secrets to access Database Migration Service resources in the Database Migration Service User Guide.
*
*
*/
private String secretsManagerAccessRoleArn;
/**
*
* The full ARN, partial ARN, or friendly name of the SecretsManagerSecret
that contains the Oracle
* endpoint connection details.
*
*/
private String secretsManagerSecretId;
/**
*
* Required only if your Oracle endpoint uses Automatic Storage Management (ASM). The full ARN of the IAM role that
* specifies DMS as the trusted entity and grants the required permissions to access the
* SecretsManagerOracleAsmSecret
. This SecretsManagerOracleAsmSecret
has the secret value
* that allows access to the Oracle ASM of the endpoint.
*
*
*
* You can specify one of two sets of values for these permissions. You can specify the values for this setting and
* SecretsManagerOracleAsmSecretId
. Or you can specify clear-text values for AsmUser
,
* AsmPassword
, and AsmServerName
. You can't specify both. For more information on
* creating this SecretsManagerOracleAsmSecret
and the
* SecretsManagerOracleAsmAccessRoleArn
and SecretsManagerOracleAsmSecretId
required to
* access it, see Using
* secrets to access Database Migration Service resources in the Database Migration Service User Guide.
*
*
*/
private String secretsManagerOracleAsmAccessRoleArn;
/**
*
* Required only if your Oracle endpoint uses Automatic Storage Management (ASM). The full ARN, partial ARN, or
* friendly name of the SecretsManagerOracleAsmSecret
that contains the Oracle ASM connection details
* for the Oracle endpoint.
*
*/
private String secretsManagerOracleAsmSecretId;
/**
*
* Use the TrimSpaceInChar
source endpoint setting to trim data on CHAR and NCHAR data types during
* migration. The default value is true
.
*
*/
private Boolean trimSpaceInChar;
/**
*
* When true, converts timestamps with the timezone
datatype to their UTC value.
*
*/
private Boolean convertTimestampWithZoneToUTC;
/**
*
* The timeframe in minutes to check for open transactions for a CDC-only task.
*
*
* You can specify an integer value between 0 (the default) and 240 (the maximum).
*
*
*
* This parameter is only valid in DMS version 3.5.0 and later. DMS supports a window of up to 9.5 hours including
* the value for OpenTransactionWindow
.
*
*
*/
private Integer openTransactionWindow;
/**
*
* Set this attribute to set up table-level supplemental logging for the Oracle database. This attribute enables
* PRIMARY KEY supplemental logging on all tables selected for a migration task.
*
*
* If you use this option, you still need to enable database-level supplemental logging.
*
*
* @param addSupplementalLogging
* Set this attribute to set up table-level supplemental logging for the Oracle database. This attribute
* enables PRIMARY KEY supplemental logging on all tables selected for a migration task.
*
* If you use this option, you still need to enable database-level supplemental logging.
*/
public void setAddSupplementalLogging(Boolean addSupplementalLogging) {
this.addSupplementalLogging = addSupplementalLogging;
}
/**
*
* Set this attribute to set up table-level supplemental logging for the Oracle database. This attribute enables
* PRIMARY KEY supplemental logging on all tables selected for a migration task.
*
*
* If you use this option, you still need to enable database-level supplemental logging.
*
*
* @return Set this attribute to set up table-level supplemental logging for the Oracle database. This attribute
* enables PRIMARY KEY supplemental logging on all tables selected for a migration task.
*
* If you use this option, you still need to enable database-level supplemental logging.
*/
public Boolean getAddSupplementalLogging() {
return this.addSupplementalLogging;
}
/**
*
* Set this attribute to set up table-level supplemental logging for the Oracle database. This attribute enables
* PRIMARY KEY supplemental logging on all tables selected for a migration task.
*
*
* If you use this option, you still need to enable database-level supplemental logging.
*
*
* @param addSupplementalLogging
* Set this attribute to set up table-level supplemental logging for the Oracle database. This attribute
* enables PRIMARY KEY supplemental logging on all tables selected for a migration task.
*
* If you use this option, you still need to enable database-level supplemental logging.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withAddSupplementalLogging(Boolean addSupplementalLogging) {
setAddSupplementalLogging(addSupplementalLogging);
return this;
}
/**
*
* Set this attribute to set up table-level supplemental logging for the Oracle database. This attribute enables
* PRIMARY KEY supplemental logging on all tables selected for a migration task.
*
*
* If you use this option, you still need to enable database-level supplemental logging.
*
*
* @return Set this attribute to set up table-level supplemental logging for the Oracle database. This attribute
* enables PRIMARY KEY supplemental logging on all tables selected for a migration task.
*
* If you use this option, you still need to enable database-level supplemental logging.
*/
public Boolean isAddSupplementalLogging() {
return this.addSupplementalLogging;
}
/**
*
* Specifies the ID of the destination for the archived redo logs. This value should be the same as a number in the
* dest_id column of the v$archived_log view. If you work with an additional redo log destination, use the
* AdditionalArchivedLogDestId
option to specify the additional destination ID. Doing this improves
* performance by ensuring that the correct logs are accessed from the outset.
*
*
* @param archivedLogDestId
* Specifies the ID of the destination for the archived redo logs. This value should be the same as a number
* in the dest_id column of the v$archived_log view. If you work with an additional redo log destination, use
* the AdditionalArchivedLogDestId
option to specify the additional destination ID. Doing this
* improves performance by ensuring that the correct logs are accessed from the outset.
*/
public void setArchivedLogDestId(Integer archivedLogDestId) {
this.archivedLogDestId = archivedLogDestId;
}
/**
*
* Specifies the ID of the destination for the archived redo logs. This value should be the same as a number in the
* dest_id column of the v$archived_log view. If you work with an additional redo log destination, use the
* AdditionalArchivedLogDestId
option to specify the additional destination ID. Doing this improves
* performance by ensuring that the correct logs are accessed from the outset.
*
*
* @return Specifies the ID of the destination for the archived redo logs. This value should be the same as a number
* in the dest_id column of the v$archived_log view. If you work with an additional redo log destination,
* use the AdditionalArchivedLogDestId
option to specify the additional destination ID. Doing
* this improves performance by ensuring that the correct logs are accessed from the outset.
*/
public Integer getArchivedLogDestId() {
return this.archivedLogDestId;
}
/**
*
* Specifies the ID of the destination for the archived redo logs. This value should be the same as a number in the
* dest_id column of the v$archived_log view. If you work with an additional redo log destination, use the
* AdditionalArchivedLogDestId
option to specify the additional destination ID. Doing this improves
* performance by ensuring that the correct logs are accessed from the outset.
*
*
* @param archivedLogDestId
* Specifies the ID of the destination for the archived redo logs. This value should be the same as a number
* in the dest_id column of the v$archived_log view. If you work with an additional redo log destination, use
* the AdditionalArchivedLogDestId
option to specify the additional destination ID. Doing this
* improves performance by ensuring that the correct logs are accessed from the outset.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withArchivedLogDestId(Integer archivedLogDestId) {
setArchivedLogDestId(archivedLogDestId);
return this;
}
/**
*
* Set this attribute with ArchivedLogDestId
in a primary/ standby setup. This attribute is useful in
* the case of a switchover. In this case, DMS needs to know which destination to get archive redo logs from to read
* changes. This need arises because the previous primary instance is now a standby instance after switchover.
*
*
* Although DMS supports the use of the Oracle RESETLOGS
option to open the database, never use
* RESETLOGS
unless necessary. For additional information about RESETLOGS
, see RMAN Data Repair Concepts in the Oracle Database Backup and Recovery User's Guide.
*
*
* @param additionalArchivedLogDestId
* Set this attribute with ArchivedLogDestId
in a primary/ standby setup. This attribute is
* useful in the case of a switchover. In this case, DMS needs to know which destination to get archive redo
* logs from to read changes. This need arises because the previous primary instance is now a standby
* instance after switchover.
*
* Although DMS supports the use of the Oracle RESETLOGS
option to open the database, never use
* RESETLOGS
unless necessary. For additional information about RESETLOGS
, see RMAN Data Repair Concepts in the Oracle Database Backup and Recovery User's Guide.
*/
public void setAdditionalArchivedLogDestId(Integer additionalArchivedLogDestId) {
this.additionalArchivedLogDestId = additionalArchivedLogDestId;
}
/**
*
* Set this attribute with ArchivedLogDestId
in a primary/ standby setup. This attribute is useful in
* the case of a switchover. In this case, DMS needs to know which destination to get archive redo logs from to read
* changes. This need arises because the previous primary instance is now a standby instance after switchover.
*
*
* Although DMS supports the use of the Oracle RESETLOGS
option to open the database, never use
* RESETLOGS
unless necessary. For additional information about RESETLOGS
, see RMAN Data Repair Concepts in the Oracle Database Backup and Recovery User's Guide.
*
*
* @return Set this attribute with ArchivedLogDestId
in a primary/ standby setup. This attribute is
* useful in the case of a switchover. In this case, DMS needs to know which destination to get archive redo
* logs from to read changes. This need arises because the previous primary instance is now a standby
* instance after switchover.
*
* Although DMS supports the use of the Oracle RESETLOGS
option to open the database, never use
* RESETLOGS
unless necessary. For additional information about RESETLOGS
, see RMAN Data Repair Concepts in the Oracle Database Backup and Recovery User's Guide.
*/
public Integer getAdditionalArchivedLogDestId() {
return this.additionalArchivedLogDestId;
}
/**
*
* Set this attribute with ArchivedLogDestId
in a primary/ standby setup. This attribute is useful in
* the case of a switchover. In this case, DMS needs to know which destination to get archive redo logs from to read
* changes. This need arises because the previous primary instance is now a standby instance after switchover.
*
*
* Although DMS supports the use of the Oracle RESETLOGS
option to open the database, never use
* RESETLOGS
unless necessary. For additional information about RESETLOGS
, see RMAN Data Repair Concepts in the Oracle Database Backup and Recovery User's Guide.
*
*
* @param additionalArchivedLogDestId
* Set this attribute with ArchivedLogDestId
in a primary/ standby setup. This attribute is
* useful in the case of a switchover. In this case, DMS needs to know which destination to get archive redo
* logs from to read changes. This need arises because the previous primary instance is now a standby
* instance after switchover.
*
* Although DMS supports the use of the Oracle RESETLOGS
option to open the database, never use
* RESETLOGS
unless necessary. For additional information about RESETLOGS
, see RMAN Data Repair Concepts in the Oracle Database Backup and Recovery User's Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withAdditionalArchivedLogDestId(Integer additionalArchivedLogDestId) {
setAdditionalArchivedLogDestId(additionalArchivedLogDestId);
return this;
}
/**
*
* Specifies the IDs of one more destinations for one or more archived redo logs. These IDs are the values of the
* dest_id
column in the v$archived_log
view. Use this setting with the
* archivedLogDestId
extra connection attribute in a primary-to-single setup or a
* primary-to-multiple-standby setup.
*
*
* This setting is useful in a switchover when you use an Oracle Data Guard database as a source. In this case, DMS
* needs information about what destination to get archive redo logs from to read changes. DMS needs this because
* after the switchover the previous primary is a standby instance. For example, in a primary-to-single standby
* setup you might apply the following settings.
*
*
* archivedLogDestId=1; ExtraArchivedLogDestIds=[2]
*
*
* In a primary-to-multiple-standby setup, you might apply the following settings.
*
*
* archivedLogDestId=1; ExtraArchivedLogDestIds=[2,3,4]
*
*
* Although DMS supports the use of the Oracle RESETLOGS
option to open the database, never use
* RESETLOGS
unless it's necessary. For more information about RESETLOGS
, see RMAN Data Repair Concepts in the Oracle Database Backup and Recovery User's Guide.
*
*
* @return Specifies the IDs of one more destinations for one or more archived redo logs. These IDs are the values
* of the dest_id
column in the v$archived_log
view. Use this setting with the
* archivedLogDestId
extra connection attribute in a primary-to-single setup or a
* primary-to-multiple-standby setup.
*
* This setting is useful in a switchover when you use an Oracle Data Guard database as a source. In this
* case, DMS needs information about what destination to get archive redo logs from to read changes. DMS
* needs this because after the switchover the previous primary is a standby instance. For example, in a
* primary-to-single standby setup you might apply the following settings.
*
*
* archivedLogDestId=1; ExtraArchivedLogDestIds=[2]
*
*
* In a primary-to-multiple-standby setup, you might apply the following settings.
*
*
* archivedLogDestId=1; ExtraArchivedLogDestIds=[2,3,4]
*
*
* Although DMS supports the use of the Oracle RESETLOGS
option to open the database, never use
* RESETLOGS
unless it's necessary. For more information about RESETLOGS
, see RMAN Data Repair Concepts in the Oracle Database Backup and Recovery User's Guide.
*/
public java.util.List getExtraArchivedLogDestIds() {
return extraArchivedLogDestIds;
}
/**
*
* Specifies the IDs of one more destinations for one or more archived redo logs. These IDs are the values of the
* dest_id
column in the v$archived_log
view. Use this setting with the
* archivedLogDestId
extra connection attribute in a primary-to-single setup or a
* primary-to-multiple-standby setup.
*
*
* This setting is useful in a switchover when you use an Oracle Data Guard database as a source. In this case, DMS
* needs information about what destination to get archive redo logs from to read changes. DMS needs this because
* after the switchover the previous primary is a standby instance. For example, in a primary-to-single standby
* setup you might apply the following settings.
*
*
* archivedLogDestId=1; ExtraArchivedLogDestIds=[2]
*
*
* In a primary-to-multiple-standby setup, you might apply the following settings.
*
*
* archivedLogDestId=1; ExtraArchivedLogDestIds=[2,3,4]
*
*
* Although DMS supports the use of the Oracle RESETLOGS
option to open the database, never use
* RESETLOGS
unless it's necessary. For more information about RESETLOGS
, see RMAN Data Repair Concepts in the Oracle Database Backup and Recovery User's Guide.
*
*
* @param extraArchivedLogDestIds
* Specifies the IDs of one more destinations for one or more archived redo logs. These IDs are the values of
* the dest_id
column in the v$archived_log
view. Use this setting with the
* archivedLogDestId
extra connection attribute in a primary-to-single setup or a
* primary-to-multiple-standby setup.
*
* This setting is useful in a switchover when you use an Oracle Data Guard database as a source. In this
* case, DMS needs information about what destination to get archive redo logs from to read changes. DMS
* needs this because after the switchover the previous primary is a standby instance. For example, in a
* primary-to-single standby setup you might apply the following settings.
*
*
* archivedLogDestId=1; ExtraArchivedLogDestIds=[2]
*
*
* In a primary-to-multiple-standby setup, you might apply the following settings.
*
*
* archivedLogDestId=1; ExtraArchivedLogDestIds=[2,3,4]
*
*
* Although DMS supports the use of the Oracle RESETLOGS
option to open the database, never use
* RESETLOGS
unless it's necessary. For more information about RESETLOGS
, see RMAN Data Repair Concepts in the Oracle Database Backup and Recovery User's Guide.
*/
public void setExtraArchivedLogDestIds(java.util.Collection extraArchivedLogDestIds) {
if (extraArchivedLogDestIds == null) {
this.extraArchivedLogDestIds = null;
return;
}
this.extraArchivedLogDestIds = new java.util.ArrayList(extraArchivedLogDestIds);
}
/**
*
* Specifies the IDs of one more destinations for one or more archived redo logs. These IDs are the values of the
* dest_id
column in the v$archived_log
view. Use this setting with the
* archivedLogDestId
extra connection attribute in a primary-to-single setup or a
* primary-to-multiple-standby setup.
*
*
* This setting is useful in a switchover when you use an Oracle Data Guard database as a source. In this case, DMS
* needs information about what destination to get archive redo logs from to read changes. DMS needs this because
* after the switchover the previous primary is a standby instance. For example, in a primary-to-single standby
* setup you might apply the following settings.
*
*
* archivedLogDestId=1; ExtraArchivedLogDestIds=[2]
*
*
* In a primary-to-multiple-standby setup, you might apply the following settings.
*
*
* archivedLogDestId=1; ExtraArchivedLogDestIds=[2,3,4]
*
*
* Although DMS supports the use of the Oracle RESETLOGS
option to open the database, never use
* RESETLOGS
unless it's necessary. For more information about RESETLOGS
, see RMAN Data Repair Concepts in the Oracle Database Backup and Recovery User's Guide.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setExtraArchivedLogDestIds(java.util.Collection)} or
* {@link #withExtraArchivedLogDestIds(java.util.Collection)} if you want to override the existing values.
*
*
* @param extraArchivedLogDestIds
* Specifies the IDs of one more destinations for one or more archived redo logs. These IDs are the values of
* the dest_id
column in the v$archived_log
view. Use this setting with the
* archivedLogDestId
extra connection attribute in a primary-to-single setup or a
* primary-to-multiple-standby setup.
*
* This setting is useful in a switchover when you use an Oracle Data Guard database as a source. In this
* case, DMS needs information about what destination to get archive redo logs from to read changes. DMS
* needs this because after the switchover the previous primary is a standby instance. For example, in a
* primary-to-single standby setup you might apply the following settings.
*
*
* archivedLogDestId=1; ExtraArchivedLogDestIds=[2]
*
*
* In a primary-to-multiple-standby setup, you might apply the following settings.
*
*
* archivedLogDestId=1; ExtraArchivedLogDestIds=[2,3,4]
*
*
* Although DMS supports the use of the Oracle RESETLOGS
option to open the database, never use
* RESETLOGS
unless it's necessary. For more information about RESETLOGS
, see RMAN Data Repair Concepts in the Oracle Database Backup and Recovery User's Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withExtraArchivedLogDestIds(Integer... extraArchivedLogDestIds) {
if (this.extraArchivedLogDestIds == null) {
setExtraArchivedLogDestIds(new java.util.ArrayList(extraArchivedLogDestIds.length));
}
for (Integer ele : extraArchivedLogDestIds) {
this.extraArchivedLogDestIds.add(ele);
}
return this;
}
/**
*
* Specifies the IDs of one more destinations for one or more archived redo logs. These IDs are the values of the
* dest_id
column in the v$archived_log
view. Use this setting with the
* archivedLogDestId
extra connection attribute in a primary-to-single setup or a
* primary-to-multiple-standby setup.
*
*
* This setting is useful in a switchover when you use an Oracle Data Guard database as a source. In this case, DMS
* needs information about what destination to get archive redo logs from to read changes. DMS needs this because
* after the switchover the previous primary is a standby instance. For example, in a primary-to-single standby
* setup you might apply the following settings.
*
*
* archivedLogDestId=1; ExtraArchivedLogDestIds=[2]
*
*
* In a primary-to-multiple-standby setup, you might apply the following settings.
*
*
* archivedLogDestId=1; ExtraArchivedLogDestIds=[2,3,4]
*
*
* Although DMS supports the use of the Oracle RESETLOGS
option to open the database, never use
* RESETLOGS
unless it's necessary. For more information about RESETLOGS
, see RMAN Data Repair Concepts in the Oracle Database Backup and Recovery User's Guide.
*
*
* @param extraArchivedLogDestIds
* Specifies the IDs of one more destinations for one or more archived redo logs. These IDs are the values of
* the dest_id
column in the v$archived_log
view. Use this setting with the
* archivedLogDestId
extra connection attribute in a primary-to-single setup or a
* primary-to-multiple-standby setup.
*
* This setting is useful in a switchover when you use an Oracle Data Guard database as a source. In this
* case, DMS needs information about what destination to get archive redo logs from to read changes. DMS
* needs this because after the switchover the previous primary is a standby instance. For example, in a
* primary-to-single standby setup you might apply the following settings.
*
*
* archivedLogDestId=1; ExtraArchivedLogDestIds=[2]
*
*
* In a primary-to-multiple-standby setup, you might apply the following settings.
*
*
* archivedLogDestId=1; ExtraArchivedLogDestIds=[2,3,4]
*
*
* Although DMS supports the use of the Oracle RESETLOGS
option to open the database, never use
* RESETLOGS
unless it's necessary. For more information about RESETLOGS
, see RMAN Data Repair Concepts in the Oracle Database Backup and Recovery User's Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withExtraArchivedLogDestIds(java.util.Collection extraArchivedLogDestIds) {
setExtraArchivedLogDestIds(extraArchivedLogDestIds);
return this;
}
/**
*
* Set this attribute to true
to enable replication of Oracle tables containing columns that are nested
* tables or defined types.
*
*
* @param allowSelectNestedTables
* Set this attribute to true
to enable replication of Oracle tables containing columns that are
* nested tables or defined types.
*/
public void setAllowSelectNestedTables(Boolean allowSelectNestedTables) {
this.allowSelectNestedTables = allowSelectNestedTables;
}
/**
*
* Set this attribute to true
to enable replication of Oracle tables containing columns that are nested
* tables or defined types.
*
*
* @return Set this attribute to true
to enable replication of Oracle tables containing columns that
* are nested tables or defined types.
*/
public Boolean getAllowSelectNestedTables() {
return this.allowSelectNestedTables;
}
/**
*
* Set this attribute to true
to enable replication of Oracle tables containing columns that are nested
* tables or defined types.
*
*
* @param allowSelectNestedTables
* Set this attribute to true
to enable replication of Oracle tables containing columns that are
* nested tables or defined types.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withAllowSelectNestedTables(Boolean allowSelectNestedTables) {
setAllowSelectNestedTables(allowSelectNestedTables);
return this;
}
/**
*
* Set this attribute to true
to enable replication of Oracle tables containing columns that are nested
* tables or defined types.
*
*
* @return Set this attribute to true
to enable replication of Oracle tables containing columns that
* are nested tables or defined types.
*/
public Boolean isAllowSelectNestedTables() {
return this.allowSelectNestedTables;
}
/**
*
* Set this attribute to change the number of threads that DMS configures to perform a change data capture (CDC)
* load using Oracle Automatic Storage Management (ASM). You can specify an integer value between 2 (the default)
* and 8 (the maximum). Use this attribute together with the readAheadBlocks
attribute.
*
*
* @param parallelAsmReadThreads
* Set this attribute to change the number of threads that DMS configures to perform a change data capture
* (CDC) load using Oracle Automatic Storage Management (ASM). You can specify an integer value between 2
* (the default) and 8 (the maximum). Use this attribute together with the readAheadBlocks
* attribute.
*/
public void setParallelAsmReadThreads(Integer parallelAsmReadThreads) {
this.parallelAsmReadThreads = parallelAsmReadThreads;
}
/**
*
* Set this attribute to change the number of threads that DMS configures to perform a change data capture (CDC)
* load using Oracle Automatic Storage Management (ASM). You can specify an integer value between 2 (the default)
* and 8 (the maximum). Use this attribute together with the readAheadBlocks
attribute.
*
*
* @return Set this attribute to change the number of threads that DMS configures to perform a change data capture
* (CDC) load using Oracle Automatic Storage Management (ASM). You can specify an integer value between 2
* (the default) and 8 (the maximum). Use this attribute together with the readAheadBlocks
* attribute.
*/
public Integer getParallelAsmReadThreads() {
return this.parallelAsmReadThreads;
}
/**
*
* Set this attribute to change the number of threads that DMS configures to perform a change data capture (CDC)
* load using Oracle Automatic Storage Management (ASM). You can specify an integer value between 2 (the default)
* and 8 (the maximum). Use this attribute together with the readAheadBlocks
attribute.
*
*
* @param parallelAsmReadThreads
* Set this attribute to change the number of threads that DMS configures to perform a change data capture
* (CDC) load using Oracle Automatic Storage Management (ASM). You can specify an integer value between 2
* (the default) and 8 (the maximum). Use this attribute together with the readAheadBlocks
* attribute.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withParallelAsmReadThreads(Integer parallelAsmReadThreads) {
setParallelAsmReadThreads(parallelAsmReadThreads);
return this;
}
/**
*
* Set this attribute to change the number of read-ahead blocks that DMS configures to perform a change data capture
* (CDC) load using Oracle Automatic Storage Management (ASM). You can specify an integer value between 1000 (the
* default) and 200,000 (the maximum).
*
*
* @param readAheadBlocks
* Set this attribute to change the number of read-ahead blocks that DMS configures to perform a change data
* capture (CDC) load using Oracle Automatic Storage Management (ASM). You can specify an integer value
* between 1000 (the default) and 200,000 (the maximum).
*/
public void setReadAheadBlocks(Integer readAheadBlocks) {
this.readAheadBlocks = readAheadBlocks;
}
/**
*
* Set this attribute to change the number of read-ahead blocks that DMS configures to perform a change data capture
* (CDC) load using Oracle Automatic Storage Management (ASM). You can specify an integer value between 1000 (the
* default) and 200,000 (the maximum).
*
*
* @return Set this attribute to change the number of read-ahead blocks that DMS configures to perform a change data
* capture (CDC) load using Oracle Automatic Storage Management (ASM). You can specify an integer value
* between 1000 (the default) and 200,000 (the maximum).
*/
public Integer getReadAheadBlocks() {
return this.readAheadBlocks;
}
/**
*
* Set this attribute to change the number of read-ahead blocks that DMS configures to perform a change data capture
* (CDC) load using Oracle Automatic Storage Management (ASM). You can specify an integer value between 1000 (the
* default) and 200,000 (the maximum).
*
*
* @param readAheadBlocks
* Set this attribute to change the number of read-ahead blocks that DMS configures to perform a change data
* capture (CDC) load using Oracle Automatic Storage Management (ASM). You can specify an integer value
* between 1000 (the default) and 200,000 (the maximum).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withReadAheadBlocks(Integer readAheadBlocks) {
setReadAheadBlocks(readAheadBlocks);
return this;
}
/**
*
* Set this attribute to false
in order to use the Binary Reader to capture change data for an Amazon
* RDS for Oracle as the source. This tells the DMS instance to not access redo logs through any specified path
* prefix replacement using direct file access.
*
*
* @param accessAlternateDirectly
* Set this attribute to false
in order to use the Binary Reader to capture change data for an
* Amazon RDS for Oracle as the source. This tells the DMS instance to not access redo logs through any
* specified path prefix replacement using direct file access.
*/
public void setAccessAlternateDirectly(Boolean accessAlternateDirectly) {
this.accessAlternateDirectly = accessAlternateDirectly;
}
/**
*
* Set this attribute to false
in order to use the Binary Reader to capture change data for an Amazon
* RDS for Oracle as the source. This tells the DMS instance to not access redo logs through any specified path
* prefix replacement using direct file access.
*
*
* @return Set this attribute to false
in order to use the Binary Reader to capture change data for an
* Amazon RDS for Oracle as the source. This tells the DMS instance to not access redo logs through any
* specified path prefix replacement using direct file access.
*/
public Boolean getAccessAlternateDirectly() {
return this.accessAlternateDirectly;
}
/**
*
* Set this attribute to false
in order to use the Binary Reader to capture change data for an Amazon
* RDS for Oracle as the source. This tells the DMS instance to not access redo logs through any specified path
* prefix replacement using direct file access.
*
*
* @param accessAlternateDirectly
* Set this attribute to false
in order to use the Binary Reader to capture change data for an
* Amazon RDS for Oracle as the source. This tells the DMS instance to not access redo logs through any
* specified path prefix replacement using direct file access.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withAccessAlternateDirectly(Boolean accessAlternateDirectly) {
setAccessAlternateDirectly(accessAlternateDirectly);
return this;
}
/**
*
* Set this attribute to false
in order to use the Binary Reader to capture change data for an Amazon
* RDS for Oracle as the source. This tells the DMS instance to not access redo logs through any specified path
* prefix replacement using direct file access.
*
*
* @return Set this attribute to false
in order to use the Binary Reader to capture change data for an
* Amazon RDS for Oracle as the source. This tells the DMS instance to not access redo logs through any
* specified path prefix replacement using direct file access.
*/
public Boolean isAccessAlternateDirectly() {
return this.accessAlternateDirectly;
}
/**
*
* Set this attribute to true
in order to use the Binary Reader to capture change data for an Amazon
* RDS for Oracle as the source. This tells the DMS instance to use any specified prefix replacement to access all
* online redo logs.
*
*
* @param useAlternateFolderForOnline
* Set this attribute to true
in order to use the Binary Reader to capture change data for an
* Amazon RDS for Oracle as the source. This tells the DMS instance to use any specified prefix replacement
* to access all online redo logs.
*/
public void setUseAlternateFolderForOnline(Boolean useAlternateFolderForOnline) {
this.useAlternateFolderForOnline = useAlternateFolderForOnline;
}
/**
*
* Set this attribute to true
in order to use the Binary Reader to capture change data for an Amazon
* RDS for Oracle as the source. This tells the DMS instance to use any specified prefix replacement to access all
* online redo logs.
*
*
* @return Set this attribute to true
in order to use the Binary Reader to capture change data for an
* Amazon RDS for Oracle as the source. This tells the DMS instance to use any specified prefix replacement
* to access all online redo logs.
*/
public Boolean getUseAlternateFolderForOnline() {
return this.useAlternateFolderForOnline;
}
/**
*
* Set this attribute to true
in order to use the Binary Reader to capture change data for an Amazon
* RDS for Oracle as the source. This tells the DMS instance to use any specified prefix replacement to access all
* online redo logs.
*
*
* @param useAlternateFolderForOnline
* Set this attribute to true
in order to use the Binary Reader to capture change data for an
* Amazon RDS for Oracle as the source. This tells the DMS instance to use any specified prefix replacement
* to access all online redo logs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withUseAlternateFolderForOnline(Boolean useAlternateFolderForOnline) {
setUseAlternateFolderForOnline(useAlternateFolderForOnline);
return this;
}
/**
*
* Set this attribute to true
in order to use the Binary Reader to capture change data for an Amazon
* RDS for Oracle as the source. This tells the DMS instance to use any specified prefix replacement to access all
* online redo logs.
*
*
* @return Set this attribute to true
in order to use the Binary Reader to capture change data for an
* Amazon RDS for Oracle as the source. This tells the DMS instance to use any specified prefix replacement
* to access all online redo logs.
*/
public Boolean isUseAlternateFolderForOnline() {
return this.useAlternateFolderForOnline;
}
/**
*
* Set this string attribute to the required value in order to use the Binary Reader to capture change data for an
* Amazon RDS for Oracle as the source. This value specifies the default Oracle root used to access the redo logs.
*
*
* @param oraclePathPrefix
* Set this string attribute to the required value in order to use the Binary Reader to capture change data
* for an Amazon RDS for Oracle as the source. This value specifies the default Oracle root used to access
* the redo logs.
*/
public void setOraclePathPrefix(String oraclePathPrefix) {
this.oraclePathPrefix = oraclePathPrefix;
}
/**
*
* Set this string attribute to the required value in order to use the Binary Reader to capture change data for an
* Amazon RDS for Oracle as the source. This value specifies the default Oracle root used to access the redo logs.
*
*
* @return Set this string attribute to the required value in order to use the Binary Reader to capture change data
* for an Amazon RDS for Oracle as the source. This value specifies the default Oracle root used to access
* the redo logs.
*/
public String getOraclePathPrefix() {
return this.oraclePathPrefix;
}
/**
*
* Set this string attribute to the required value in order to use the Binary Reader to capture change data for an
* Amazon RDS for Oracle as the source. This value specifies the default Oracle root used to access the redo logs.
*
*
* @param oraclePathPrefix
* Set this string attribute to the required value in order to use the Binary Reader to capture change data
* for an Amazon RDS for Oracle as the source. This value specifies the default Oracle root used to access
* the redo logs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withOraclePathPrefix(String oraclePathPrefix) {
setOraclePathPrefix(oraclePathPrefix);
return this;
}
/**
*
* Set this string attribute to the required value in order to use the Binary Reader to capture change data for an
* Amazon RDS for Oracle as the source. This value specifies the path prefix used to replace the default Oracle root
* to access the redo logs.
*
*
* @param usePathPrefix
* Set this string attribute to the required value in order to use the Binary Reader to capture change data
* for an Amazon RDS for Oracle as the source. This value specifies the path prefix used to replace the
* default Oracle root to access the redo logs.
*/
public void setUsePathPrefix(String usePathPrefix) {
this.usePathPrefix = usePathPrefix;
}
/**
*
* Set this string attribute to the required value in order to use the Binary Reader to capture change data for an
* Amazon RDS for Oracle as the source. This value specifies the path prefix used to replace the default Oracle root
* to access the redo logs.
*
*
* @return Set this string attribute to the required value in order to use the Binary Reader to capture change data
* for an Amazon RDS for Oracle as the source. This value specifies the path prefix used to replace the
* default Oracle root to access the redo logs.
*/
public String getUsePathPrefix() {
return this.usePathPrefix;
}
/**
*
* Set this string attribute to the required value in order to use the Binary Reader to capture change data for an
* Amazon RDS for Oracle as the source. This value specifies the path prefix used to replace the default Oracle root
* to access the redo logs.
*
*
* @param usePathPrefix
* Set this string attribute to the required value in order to use the Binary Reader to capture change data
* for an Amazon RDS for Oracle as the source. This value specifies the path prefix used to replace the
* default Oracle root to access the redo logs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withUsePathPrefix(String usePathPrefix) {
setUsePathPrefix(usePathPrefix);
return this;
}
/**
*
* Set this attribute to true in order to use the Binary Reader to capture change data for an Amazon RDS for Oracle
* as the source. This setting tells DMS instance to replace the default Oracle root with the specified
* usePathPrefix
setting to access the redo logs.
*
*
* @param replacePathPrefix
* Set this attribute to true in order to use the Binary Reader to capture change data for an Amazon RDS for
* Oracle as the source. This setting tells DMS instance to replace the default Oracle root with the
* specified usePathPrefix
setting to access the redo logs.
*/
public void setReplacePathPrefix(Boolean replacePathPrefix) {
this.replacePathPrefix = replacePathPrefix;
}
/**
*
* Set this attribute to true in order to use the Binary Reader to capture change data for an Amazon RDS for Oracle
* as the source. This setting tells DMS instance to replace the default Oracle root with the specified
* usePathPrefix
setting to access the redo logs.
*
*
* @return Set this attribute to true in order to use the Binary Reader to capture change data for an Amazon RDS for
* Oracle as the source. This setting tells DMS instance to replace the default Oracle root with the
* specified usePathPrefix
setting to access the redo logs.
*/
public Boolean getReplacePathPrefix() {
return this.replacePathPrefix;
}
/**
*
* Set this attribute to true in order to use the Binary Reader to capture change data for an Amazon RDS for Oracle
* as the source. This setting tells DMS instance to replace the default Oracle root with the specified
* usePathPrefix
setting to access the redo logs.
*
*
* @param replacePathPrefix
* Set this attribute to true in order to use the Binary Reader to capture change data for an Amazon RDS for
* Oracle as the source. This setting tells DMS instance to replace the default Oracle root with the
* specified usePathPrefix
setting to access the redo logs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withReplacePathPrefix(Boolean replacePathPrefix) {
setReplacePathPrefix(replacePathPrefix);
return this;
}
/**
*
* Set this attribute to true in order to use the Binary Reader to capture change data for an Amazon RDS for Oracle
* as the source. This setting tells DMS instance to replace the default Oracle root with the specified
* usePathPrefix
setting to access the redo logs.
*
*
* @return Set this attribute to true in order to use the Binary Reader to capture change data for an Amazon RDS for
* Oracle as the source. This setting tells DMS instance to replace the default Oracle root with the
* specified usePathPrefix
setting to access the redo logs.
*/
public Boolean isReplacePathPrefix() {
return this.replacePathPrefix;
}
/**
*
* Set this attribute to enable homogenous tablespace replication and create existing tables or indexes under the
* same tablespace on the target.
*
*
* @param enableHomogenousTablespace
* Set this attribute to enable homogenous tablespace replication and create existing tables or indexes under
* the same tablespace on the target.
*/
public void setEnableHomogenousTablespace(Boolean enableHomogenousTablespace) {
this.enableHomogenousTablespace = enableHomogenousTablespace;
}
/**
*
* Set this attribute to enable homogenous tablespace replication and create existing tables or indexes under the
* same tablespace on the target.
*
*
* @return Set this attribute to enable homogenous tablespace replication and create existing tables or indexes
* under the same tablespace on the target.
*/
public Boolean getEnableHomogenousTablespace() {
return this.enableHomogenousTablespace;
}
/**
*
* Set this attribute to enable homogenous tablespace replication and create existing tables or indexes under the
* same tablespace on the target.
*
*
* @param enableHomogenousTablespace
* Set this attribute to enable homogenous tablespace replication and create existing tables or indexes under
* the same tablespace on the target.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withEnableHomogenousTablespace(Boolean enableHomogenousTablespace) {
setEnableHomogenousTablespace(enableHomogenousTablespace);
return this;
}
/**
*
* Set this attribute to enable homogenous tablespace replication and create existing tables or indexes under the
* same tablespace on the target.
*
*
* @return Set this attribute to enable homogenous tablespace replication and create existing tables or indexes
* under the same tablespace on the target.
*/
public Boolean isEnableHomogenousTablespace() {
return this.enableHomogenousTablespace;
}
/**
*
* When set to true
, this attribute helps to increase the commit rate on the Oracle target database by
* writing directly to tables and not writing a trail to database logs.
*
*
* @param directPathNoLog
* When set to true
, this attribute helps to increase the commit rate on the Oracle target
* database by writing directly to tables and not writing a trail to database logs.
*/
public void setDirectPathNoLog(Boolean directPathNoLog) {
this.directPathNoLog = directPathNoLog;
}
/**
*
* When set to true
, this attribute helps to increase the commit rate on the Oracle target database by
* writing directly to tables and not writing a trail to database logs.
*
*
* @return When set to true
, this attribute helps to increase the commit rate on the Oracle target
* database by writing directly to tables and not writing a trail to database logs.
*/
public Boolean getDirectPathNoLog() {
return this.directPathNoLog;
}
/**
*
* When set to true
, this attribute helps to increase the commit rate on the Oracle target database by
* writing directly to tables and not writing a trail to database logs.
*
*
* @param directPathNoLog
* When set to true
, this attribute helps to increase the commit rate on the Oracle target
* database by writing directly to tables and not writing a trail to database logs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withDirectPathNoLog(Boolean directPathNoLog) {
setDirectPathNoLog(directPathNoLog);
return this;
}
/**
*
* When set to true
, this attribute helps to increase the commit rate on the Oracle target database by
* writing directly to tables and not writing a trail to database logs.
*
*
* @return When set to true
, this attribute helps to increase the commit rate on the Oracle target
* database by writing directly to tables and not writing a trail to database logs.
*/
public Boolean isDirectPathNoLog() {
return this.directPathNoLog;
}
/**
*
* When this field is set to Y
, DMS only accesses the archived redo logs. If the archived redo logs are
* stored on Automatic Storage Management (ASM) only, the DMS user account needs to be granted ASM privileges.
*
*
* @param archivedLogsOnly
* When this field is set to Y
, DMS only accesses the archived redo logs. If the archived redo
* logs are stored on Automatic Storage Management (ASM) only, the DMS user account needs to be granted ASM
* privileges.
*/
public void setArchivedLogsOnly(Boolean archivedLogsOnly) {
this.archivedLogsOnly = archivedLogsOnly;
}
/**
*
* When this field is set to Y
, DMS only accesses the archived redo logs. If the archived redo logs are
* stored on Automatic Storage Management (ASM) only, the DMS user account needs to be granted ASM privileges.
*
*
* @return When this field is set to Y
, DMS only accesses the archived redo logs. If the archived redo
* logs are stored on Automatic Storage Management (ASM) only, the DMS user account needs to be granted ASM
* privileges.
*/
public Boolean getArchivedLogsOnly() {
return this.archivedLogsOnly;
}
/**
*
* When this field is set to Y
, DMS only accesses the archived redo logs. If the archived redo logs are
* stored on Automatic Storage Management (ASM) only, the DMS user account needs to be granted ASM privileges.
*
*
* @param archivedLogsOnly
* When this field is set to Y
, DMS only accesses the archived redo logs. If the archived redo
* logs are stored on Automatic Storage Management (ASM) only, the DMS user account needs to be granted ASM
* privileges.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withArchivedLogsOnly(Boolean archivedLogsOnly) {
setArchivedLogsOnly(archivedLogsOnly);
return this;
}
/**
*
* When this field is set to Y
, DMS only accesses the archived redo logs. If the archived redo logs are
* stored on Automatic Storage Management (ASM) only, the DMS user account needs to be granted ASM privileges.
*
*
* @return When this field is set to Y
, DMS only accesses the archived redo logs. If the archived redo
* logs are stored on Automatic Storage Management (ASM) only, the DMS user account needs to be granted ASM
* privileges.
*/
public Boolean isArchivedLogsOnly() {
return this.archivedLogsOnly;
}
/**
*
* For an Oracle source endpoint, your Oracle Automatic Storage Management (ASM) password. You can set this value
* from the asm_user_password
value. You set this value as part of the comma-separated value
* that you set to the Password
request parameter when you create the endpoint to access transaction
* logs using Binary Reader. For more information, see Configuration for change data capture (CDC) on an Oracle source database.
*
*
* @param asmPassword
* For an Oracle source endpoint, your Oracle Automatic Storage Management (ASM) password. You can set this
* value from the asm_user_password
value. You set this value as part of the
* comma-separated value that you set to the Password
request parameter when you create the
* endpoint to access transaction logs using Binary Reader. For more information, see Configuration for change data capture (CDC) on an Oracle source database.
*/
public void setAsmPassword(String asmPassword) {
this.asmPassword = asmPassword;
}
/**
*
* For an Oracle source endpoint, your Oracle Automatic Storage Management (ASM) password. You can set this value
* from the asm_user_password
value. You set this value as part of the comma-separated value
* that you set to the Password
request parameter when you create the endpoint to access transaction
* logs using Binary Reader. For more information, see Configuration for change data capture (CDC) on an Oracle source database.
*
*
* @return For an Oracle source endpoint, your Oracle Automatic Storage Management (ASM) password. You can set this
* value from the asm_user_password
value. You set this value as part of the
* comma-separated value that you set to the Password
request parameter when you create the
* endpoint to access transaction logs using Binary Reader. For more information, see Configuration for change data capture (CDC) on an Oracle source database.
*/
public String getAsmPassword() {
return this.asmPassword;
}
/**
*
* For an Oracle source endpoint, your Oracle Automatic Storage Management (ASM) password. You can set this value
* from the asm_user_password
value. You set this value as part of the comma-separated value
* that you set to the Password
request parameter when you create the endpoint to access transaction
* logs using Binary Reader. For more information, see Configuration for change data capture (CDC) on an Oracle source database.
*
*
* @param asmPassword
* For an Oracle source endpoint, your Oracle Automatic Storage Management (ASM) password. You can set this
* value from the asm_user_password
value. You set this value as part of the
* comma-separated value that you set to the Password
request parameter when you create the
* endpoint to access transaction logs using Binary Reader. For more information, see Configuration for change data capture (CDC) on an Oracle source database.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withAsmPassword(String asmPassword) {
setAsmPassword(asmPassword);
return this;
}
/**
*
* For an Oracle source endpoint, your ASM server address. You can set this value from the asm_server
* value. You set asm_server
as part of the extra connection attribute string to access an Oracle
* server with Binary Reader that uses ASM. For more information, see Configuration for change data capture (CDC) on an Oracle source database.
*
*
* @param asmServer
* For an Oracle source endpoint, your ASM server address. You can set this value from the
* asm_server
value. You set asm_server
as part of the extra connection attribute
* string to access an Oracle server with Binary Reader that uses ASM. For more information, see Configuration for change data capture (CDC) on an Oracle source database.
*/
public void setAsmServer(String asmServer) {
this.asmServer = asmServer;
}
/**
*
* For an Oracle source endpoint, your ASM server address. You can set this value from the asm_server
* value. You set asm_server
as part of the extra connection attribute string to access an Oracle
* server with Binary Reader that uses ASM. For more information, see Configuration for change data capture (CDC) on an Oracle source database.
*
*
* @return For an Oracle source endpoint, your ASM server address. You can set this value from the
* asm_server
value. You set asm_server
as part of the extra connection attribute
* string to access an Oracle server with Binary Reader that uses ASM. For more information, see Configuration for change data capture (CDC) on an Oracle source database.
*/
public String getAsmServer() {
return this.asmServer;
}
/**
*
* For an Oracle source endpoint, your ASM server address. You can set this value from the asm_server
* value. You set asm_server
as part of the extra connection attribute string to access an Oracle
* server with Binary Reader that uses ASM. For more information, see Configuration for change data capture (CDC) on an Oracle source database.
*
*
* @param asmServer
* For an Oracle source endpoint, your ASM server address. You can set this value from the
* asm_server
value. You set asm_server
as part of the extra connection attribute
* string to access an Oracle server with Binary Reader that uses ASM. For more information, see Configuration for change data capture (CDC) on an Oracle source database.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withAsmServer(String asmServer) {
setAsmServer(asmServer);
return this;
}
/**
*
* For an Oracle source endpoint, your ASM user name. You can set this value from the asm_user
value.
* You set asm_user
as part of the extra connection attribute string to access an Oracle server with
* Binary Reader that uses ASM. For more information, see Configuration for change data capture (CDC) on an Oracle source database.
*
*
* @param asmUser
* For an Oracle source endpoint, your ASM user name. You can set this value from the asm_user
* value. You set asm_user
as part of the extra connection attribute string to access an Oracle
* server with Binary Reader that uses ASM. For more information, see Configuration for change data capture (CDC) on an Oracle source database.
*/
public void setAsmUser(String asmUser) {
this.asmUser = asmUser;
}
/**
*
* For an Oracle source endpoint, your ASM user name. You can set this value from the asm_user
value.
* You set asm_user
as part of the extra connection attribute string to access an Oracle server with
* Binary Reader that uses ASM. For more information, see Configuration for change data capture (CDC) on an Oracle source database.
*
*
* @return For an Oracle source endpoint, your ASM user name. You can set this value from the asm_user
* value. You set asm_user
as part of the extra connection attribute string to access an Oracle
* server with Binary Reader that uses ASM. For more information, see Configuration for change data capture (CDC) on an Oracle source database.
*/
public String getAsmUser() {
return this.asmUser;
}
/**
*
* For an Oracle source endpoint, your ASM user name. You can set this value from the asm_user
value.
* You set asm_user
as part of the extra connection attribute string to access an Oracle server with
* Binary Reader that uses ASM. For more information, see Configuration for change data capture (CDC) on an Oracle source database.
*
*
* @param asmUser
* For an Oracle source endpoint, your ASM user name. You can set this value from the asm_user
* value. You set asm_user
as part of the extra connection attribute string to access an Oracle
* server with Binary Reader that uses ASM. For more information, see Configuration for change data capture (CDC) on an Oracle source database.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withAsmUser(String asmUser) {
setAsmUser(asmUser);
return this;
}
/**
*
* Specifies whether the length of a character column is in bytes or in characters. To indicate that the character
* column length is in characters, set this attribute to CHAR
. Otherwise, the character column length
* is in bytes.
*
*
* Example: charLengthSemantics=CHAR;
*
*
* @param charLengthSemantics
* Specifies whether the length of a character column is in bytes or in characters. To indicate that the
* character column length is in characters, set this attribute to CHAR
. Otherwise, the
* character column length is in bytes.
*
* Example: charLengthSemantics=CHAR;
* @see CharLengthSemantics
*/
public void setCharLengthSemantics(String charLengthSemantics) {
this.charLengthSemantics = charLengthSemantics;
}
/**
*
* Specifies whether the length of a character column is in bytes or in characters. To indicate that the character
* column length is in characters, set this attribute to CHAR
. Otherwise, the character column length
* is in bytes.
*
*
* Example: charLengthSemantics=CHAR;
*
*
* @return Specifies whether the length of a character column is in bytes or in characters. To indicate that the
* character column length is in characters, set this attribute to CHAR
. Otherwise, the
* character column length is in bytes.
*
* Example: charLengthSemantics=CHAR;
* @see CharLengthSemantics
*/
public String getCharLengthSemantics() {
return this.charLengthSemantics;
}
/**
*
* Specifies whether the length of a character column is in bytes or in characters. To indicate that the character
* column length is in characters, set this attribute to CHAR
. Otherwise, the character column length
* is in bytes.
*
*
* Example: charLengthSemantics=CHAR;
*
*
* @param charLengthSemantics
* Specifies whether the length of a character column is in bytes or in characters. To indicate that the
* character column length is in characters, set this attribute to CHAR
. Otherwise, the
* character column length is in bytes.
*
* Example: charLengthSemantics=CHAR;
* @return Returns a reference to this object so that method calls can be chained together.
* @see CharLengthSemantics
*/
public OracleSettings withCharLengthSemantics(String charLengthSemantics) {
setCharLengthSemantics(charLengthSemantics);
return this;
}
/**
*
* Specifies whether the length of a character column is in bytes or in characters. To indicate that the character
* column length is in characters, set this attribute to CHAR
. Otherwise, the character column length
* is in bytes.
*
*
* Example: charLengthSemantics=CHAR;
*
*
* @param charLengthSemantics
* Specifies whether the length of a character column is in bytes or in characters. To indicate that the
* character column length is in characters, set this attribute to CHAR
. Otherwise, the
* character column length is in bytes.
*
* Example: charLengthSemantics=CHAR;
* @return Returns a reference to this object so that method calls can be chained together.
* @see CharLengthSemantics
*/
public OracleSettings withCharLengthSemantics(CharLengthSemantics charLengthSemantics) {
this.charLengthSemantics = charLengthSemantics.toString();
return this;
}
/**
*
* Database name for the endpoint.
*
*
* @param databaseName
* Database name for the endpoint.
*/
public void setDatabaseName(String databaseName) {
this.databaseName = databaseName;
}
/**
*
* Database name for the endpoint.
*
*
* @return Database name for the endpoint.
*/
public String getDatabaseName() {
return this.databaseName;
}
/**
*
* Database name for the endpoint.
*
*
* @param databaseName
* Database name for the endpoint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withDatabaseName(String databaseName) {
setDatabaseName(databaseName);
return this;
}
/**
*
* When set to true
, this attribute specifies a parallel load when useDirectPathFullLoad
* is set to Y
. This attribute also only applies when you use the DMS parallel load feature. Note that
* the target table cannot have any constraints or indexes.
*
*
* @param directPathParallelLoad
* When set to true
, this attribute specifies a parallel load when
* useDirectPathFullLoad
is set to Y
. This attribute also only applies when you use
* the DMS parallel load feature. Note that the target table cannot have any constraints or indexes.
*/
public void setDirectPathParallelLoad(Boolean directPathParallelLoad) {
this.directPathParallelLoad = directPathParallelLoad;
}
/**
*
* When set to true
, this attribute specifies a parallel load when useDirectPathFullLoad
* is set to Y
. This attribute also only applies when you use the DMS parallel load feature. Note that
* the target table cannot have any constraints or indexes.
*
*
* @return When set to true
, this attribute specifies a parallel load when
* useDirectPathFullLoad
is set to Y
. This attribute also only applies when you
* use the DMS parallel load feature. Note that the target table cannot have any constraints or indexes.
*/
public Boolean getDirectPathParallelLoad() {
return this.directPathParallelLoad;
}
/**
*
* When set to true
, this attribute specifies a parallel load when useDirectPathFullLoad
* is set to Y
. This attribute also only applies when you use the DMS parallel load feature. Note that
* the target table cannot have any constraints or indexes.
*
*
* @param directPathParallelLoad
* When set to true
, this attribute specifies a parallel load when
* useDirectPathFullLoad
is set to Y
. This attribute also only applies when you use
* the DMS parallel load feature. Note that the target table cannot have any constraints or indexes.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withDirectPathParallelLoad(Boolean directPathParallelLoad) {
setDirectPathParallelLoad(directPathParallelLoad);
return this;
}
/**
*
* When set to true
, this attribute specifies a parallel load when useDirectPathFullLoad
* is set to Y
. This attribute also only applies when you use the DMS parallel load feature. Note that
* the target table cannot have any constraints or indexes.
*
*
* @return When set to true
, this attribute specifies a parallel load when
* useDirectPathFullLoad
is set to Y
. This attribute also only applies when you
* use the DMS parallel load feature. Note that the target table cannot have any constraints or indexes.
*/
public Boolean isDirectPathParallelLoad() {
return this.directPathParallelLoad;
}
/**
*
* When set to true
, this attribute causes a task to fail if the actual size of an LOB column is
* greater than the specified LobMaxSize
.
*
*
* If a task is set to limited LOB mode and this option is set to true
, the task fails instead of
* truncating the LOB data.
*
*
* @param failTasksOnLobTruncation
* When set to true
, this attribute causes a task to fail if the actual size of an LOB column is
* greater than the specified LobMaxSize
.
*
* If a task is set to limited LOB mode and this option is set to true
, the task fails instead
* of truncating the LOB data.
*/
public void setFailTasksOnLobTruncation(Boolean failTasksOnLobTruncation) {
this.failTasksOnLobTruncation = failTasksOnLobTruncation;
}
/**
*
* When set to true
, this attribute causes a task to fail if the actual size of an LOB column is
* greater than the specified LobMaxSize
.
*
*
* If a task is set to limited LOB mode and this option is set to true
, the task fails instead of
* truncating the LOB data.
*
*
* @return When set to true
, this attribute causes a task to fail if the actual size of an LOB column
* is greater than the specified LobMaxSize
.
*
* If a task is set to limited LOB mode and this option is set to true
, the task fails instead
* of truncating the LOB data.
*/
public Boolean getFailTasksOnLobTruncation() {
return this.failTasksOnLobTruncation;
}
/**
*
* When set to true
, this attribute causes a task to fail if the actual size of an LOB column is
* greater than the specified LobMaxSize
.
*
*
* If a task is set to limited LOB mode and this option is set to true
, the task fails instead of
* truncating the LOB data.
*
*
* @param failTasksOnLobTruncation
* When set to true
, this attribute causes a task to fail if the actual size of an LOB column is
* greater than the specified LobMaxSize
.
*
* If a task is set to limited LOB mode and this option is set to true
, the task fails instead
* of truncating the LOB data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withFailTasksOnLobTruncation(Boolean failTasksOnLobTruncation) {
setFailTasksOnLobTruncation(failTasksOnLobTruncation);
return this;
}
/**
*
* When set to true
, this attribute causes a task to fail if the actual size of an LOB column is
* greater than the specified LobMaxSize
.
*
*
* If a task is set to limited LOB mode and this option is set to true
, the task fails instead of
* truncating the LOB data.
*
*
* @return When set to true
, this attribute causes a task to fail if the actual size of an LOB column
* is greater than the specified LobMaxSize
.
*
* If a task is set to limited LOB mode and this option is set to true
, the task fails instead
* of truncating the LOB data.
*/
public Boolean isFailTasksOnLobTruncation() {
return this.failTasksOnLobTruncation;
}
/**
*
* Specifies the number scale. You can select a scale up to 38, or you can select FLOAT. By default, the NUMBER data
* type is converted to precision 38, scale 10.
*
*
* Example: numberDataTypeScale=12
*
*
* @param numberDatatypeScale
* Specifies the number scale. You can select a scale up to 38, or you can select FLOAT. By default, the
* NUMBER data type is converted to precision 38, scale 10.
*
* Example: numberDataTypeScale=12
*/
public void setNumberDatatypeScale(Integer numberDatatypeScale) {
this.numberDatatypeScale = numberDatatypeScale;
}
/**
*
* Specifies the number scale. You can select a scale up to 38, or you can select FLOAT. By default, the NUMBER data
* type is converted to precision 38, scale 10.
*
*
* Example: numberDataTypeScale=12
*
*
* @return Specifies the number scale. You can select a scale up to 38, or you can select FLOAT. By default, the
* NUMBER data type is converted to precision 38, scale 10.
*
* Example: numberDataTypeScale=12
*/
public Integer getNumberDatatypeScale() {
return this.numberDatatypeScale;
}
/**
*
* Specifies the number scale. You can select a scale up to 38, or you can select FLOAT. By default, the NUMBER data
* type is converted to precision 38, scale 10.
*
*
* Example: numberDataTypeScale=12
*
*
* @param numberDatatypeScale
* Specifies the number scale. You can select a scale up to 38, or you can select FLOAT. By default, the
* NUMBER data type is converted to precision 38, scale 10.
*
* Example: numberDataTypeScale=12
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withNumberDatatypeScale(Integer numberDatatypeScale) {
setNumberDatatypeScale(numberDatatypeScale);
return this;
}
/**
*
* Endpoint connection password.
*
*
* @param password
* Endpoint connection password.
*/
public void setPassword(String password) {
this.password = password;
}
/**
*
* Endpoint connection password.
*
*
* @return Endpoint connection password.
*/
public String getPassword() {
return this.password;
}
/**
*
* Endpoint connection password.
*
*
* @param password
* Endpoint connection password.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withPassword(String password) {
setPassword(password);
return this;
}
/**
*
* Endpoint TCP port.
*
*
* @param port
* Endpoint TCP port.
*/
public void setPort(Integer port) {
this.port = port;
}
/**
*
* Endpoint TCP port.
*
*
* @return Endpoint TCP port.
*/
public Integer getPort() {
return this.port;
}
/**
*
* Endpoint TCP port.
*
*
* @param port
* Endpoint TCP port.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withPort(Integer port) {
setPort(port);
return this;
}
/**
*
* When set to true
, this attribute supports tablespace replication.
*
*
* @param readTableSpaceName
* When set to true
, this attribute supports tablespace replication.
*/
public void setReadTableSpaceName(Boolean readTableSpaceName) {
this.readTableSpaceName = readTableSpaceName;
}
/**
*
* When set to true
, this attribute supports tablespace replication.
*
*
* @return When set to true
, this attribute supports tablespace replication.
*/
public Boolean getReadTableSpaceName() {
return this.readTableSpaceName;
}
/**
*
* When set to true
, this attribute supports tablespace replication.
*
*
* @param readTableSpaceName
* When set to true
, this attribute supports tablespace replication.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withReadTableSpaceName(Boolean readTableSpaceName) {
setReadTableSpaceName(readTableSpaceName);
return this;
}
/**
*
* When set to true
, this attribute supports tablespace replication.
*
*
* @return When set to true
, this attribute supports tablespace replication.
*/
public Boolean isReadTableSpaceName() {
return this.readTableSpaceName;
}
/**
*
* Specifies the number of seconds that the system waits before resending a query.
*
*
* Example: retryInterval=6;
*
*
* @param retryInterval
* Specifies the number of seconds that the system waits before resending a query.
*
* Example: retryInterval=6;
*/
public void setRetryInterval(Integer retryInterval) {
this.retryInterval = retryInterval;
}
/**
*
* Specifies the number of seconds that the system waits before resending a query.
*
*
* Example: retryInterval=6;
*
*
* @return Specifies the number of seconds that the system waits before resending a query.
*
* Example: retryInterval=6;
*/
public Integer getRetryInterval() {
return this.retryInterval;
}
/**
*
* Specifies the number of seconds that the system waits before resending a query.
*
*
* Example: retryInterval=6;
*
*
* @param retryInterval
* Specifies the number of seconds that the system waits before resending a query.
*
* Example: retryInterval=6;
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withRetryInterval(Integer retryInterval) {
setRetryInterval(retryInterval);
return this;
}
/**
*
* For an Oracle source endpoint, the transparent data encryption (TDE) password required by AWM DMS to access
* Oracle redo logs encrypted by TDE using Binary Reader. It is also the TDE_Password
part of
* the comma-separated value you set to the Password
request parameter when you create the endpoint.
* The SecurityDbEncryptian
setting is related to this SecurityDbEncryptionName
setting.
* For more information, see
* Supported encryption methods for using Oracle as a source for DMS in the Database Migration Service User
* Guide.
*
*
* @param securityDbEncryption
* For an Oracle source endpoint, the transparent data encryption (TDE) password required by AWM DMS to
* access Oracle redo logs encrypted by TDE using Binary Reader. It is also the
* TDE_Password
part of the comma-separated value you set to the Password
* request parameter when you create the endpoint. The SecurityDbEncryptian
setting is related
* to this SecurityDbEncryptionName
setting. For more information, see Supported encryption methods for using Oracle as a source for DMS in the Database Migration
* Service User Guide.
*/
public void setSecurityDbEncryption(String securityDbEncryption) {
this.securityDbEncryption = securityDbEncryption;
}
/**
*
* For an Oracle source endpoint, the transparent data encryption (TDE) password required by AWM DMS to access
* Oracle redo logs encrypted by TDE using Binary Reader. It is also the TDE_Password
part of
* the comma-separated value you set to the Password
request parameter when you create the endpoint.
* The SecurityDbEncryptian
setting is related to this SecurityDbEncryptionName
setting.
* For more information, see
* Supported encryption methods for using Oracle as a source for DMS in the Database Migration Service User
* Guide.
*
*
* @return For an Oracle source endpoint, the transparent data encryption (TDE) password required by AWM DMS to
* access Oracle redo logs encrypted by TDE using Binary Reader. It is also the
* TDE_Password
part of the comma-separated value you set to the Password
* request parameter when you create the endpoint. The SecurityDbEncryptian
setting is related
* to this SecurityDbEncryptionName
setting. For more information, see Supported encryption methods for using Oracle as a source for DMS in the Database Migration
* Service User Guide.
*/
public String getSecurityDbEncryption() {
return this.securityDbEncryption;
}
/**
*
* For an Oracle source endpoint, the transparent data encryption (TDE) password required by AWM DMS to access
* Oracle redo logs encrypted by TDE using Binary Reader. It is also the TDE_Password
part of
* the comma-separated value you set to the Password
request parameter when you create the endpoint.
* The SecurityDbEncryptian
setting is related to this SecurityDbEncryptionName
setting.
* For more information, see
* Supported encryption methods for using Oracle as a source for DMS in the Database Migration Service User
* Guide.
*
*
* @param securityDbEncryption
* For an Oracle source endpoint, the transparent data encryption (TDE) password required by AWM DMS to
* access Oracle redo logs encrypted by TDE using Binary Reader. It is also the
* TDE_Password
part of the comma-separated value you set to the Password
* request parameter when you create the endpoint. The SecurityDbEncryptian
setting is related
* to this SecurityDbEncryptionName
setting. For more information, see Supported encryption methods for using Oracle as a source for DMS in the Database Migration
* Service User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withSecurityDbEncryption(String securityDbEncryption) {
setSecurityDbEncryption(securityDbEncryption);
return this;
}
/**
*
* For an Oracle source endpoint, the name of a key used for the transparent data encryption (TDE) of the columns
* and tablespaces in an Oracle source database that is encrypted using TDE. The key value is the value of the
* SecurityDbEncryption
setting. For more information on setting the key name value of
* SecurityDbEncryptionName
, see the information and example for setting the
* securityDbEncryptionName
extra connection attribute in
* Supported encryption methods for using Oracle as a source for DMS in the Database Migration Service User
* Guide.
*
*
* @param securityDbEncryptionName
* For an Oracle source endpoint, the name of a key used for the transparent data encryption (TDE) of the
* columns and tablespaces in an Oracle source database that is encrypted using TDE. The key value is the
* value of the SecurityDbEncryption
setting. For more information on setting the key name value
* of SecurityDbEncryptionName
, see the information and example for setting the
* securityDbEncryptionName
extra connection attribute in Supported encryption methods for using Oracle as a source for DMS in the Database Migration
* Service User Guide.
*/
public void setSecurityDbEncryptionName(String securityDbEncryptionName) {
this.securityDbEncryptionName = securityDbEncryptionName;
}
/**
*
* For an Oracle source endpoint, the name of a key used for the transparent data encryption (TDE) of the columns
* and tablespaces in an Oracle source database that is encrypted using TDE. The key value is the value of the
* SecurityDbEncryption
setting. For more information on setting the key name value of
* SecurityDbEncryptionName
, see the information and example for setting the
* securityDbEncryptionName
extra connection attribute in
* Supported encryption methods for using Oracle as a source for DMS in the Database Migration Service User
* Guide.
*
*
* @return For an Oracle source endpoint, the name of a key used for the transparent data encryption (TDE) of the
* columns and tablespaces in an Oracle source database that is encrypted using TDE. The key value is the
* value of the SecurityDbEncryption
setting. For more information on setting the key name
* value of SecurityDbEncryptionName
, see the information and example for setting the
* securityDbEncryptionName
extra connection attribute in Supported encryption methods for using Oracle as a source for DMS in the Database Migration
* Service User Guide.
*/
public String getSecurityDbEncryptionName() {
return this.securityDbEncryptionName;
}
/**
*
* For an Oracle source endpoint, the name of a key used for the transparent data encryption (TDE) of the columns
* and tablespaces in an Oracle source database that is encrypted using TDE. The key value is the value of the
* SecurityDbEncryption
setting. For more information on setting the key name value of
* SecurityDbEncryptionName
, see the information and example for setting the
* securityDbEncryptionName
extra connection attribute in
* Supported encryption methods for using Oracle as a source for DMS in the Database Migration Service User
* Guide.
*
*
* @param securityDbEncryptionName
* For an Oracle source endpoint, the name of a key used for the transparent data encryption (TDE) of the
* columns and tablespaces in an Oracle source database that is encrypted using TDE. The key value is the
* value of the SecurityDbEncryption
setting. For more information on setting the key name value
* of SecurityDbEncryptionName
, see the information and example for setting the
* securityDbEncryptionName
extra connection attribute in Supported encryption methods for using Oracle as a source for DMS in the Database Migration
* Service User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withSecurityDbEncryptionName(String securityDbEncryptionName) {
setSecurityDbEncryptionName(securityDbEncryptionName);
return this;
}
/**
*
* Fully qualified domain name of the endpoint.
*
*
* For an Amazon RDS Oracle instance, this is the output of DescribeDBInstances, in the
* Endpoint.Address
* field.
*
*
* @param serverName
* Fully qualified domain name of the endpoint.
*
* For an Amazon RDS Oracle instance, this is the output of DescribeDBInstances, in the
* Endpoint.Address
* field.
*/
public void setServerName(String serverName) {
this.serverName = serverName;
}
/**
*
* Fully qualified domain name of the endpoint.
*
*
* For an Amazon RDS Oracle instance, this is the output of DescribeDBInstances, in the
* Endpoint.Address
* field.
*
*
* @return Fully qualified domain name of the endpoint.
*
* For an Amazon RDS Oracle instance, this is the output of DescribeDBInstances, in the
* Endpoint.Address
* field.
*/
public String getServerName() {
return this.serverName;
}
/**
*
* Fully qualified domain name of the endpoint.
*
*
* For an Amazon RDS Oracle instance, this is the output of DescribeDBInstances, in the
* Endpoint.Address
* field.
*
*
* @param serverName
* Fully qualified domain name of the endpoint.
*
* For an Amazon RDS Oracle instance, this is the output of DescribeDBInstances, in the
* Endpoint.Address
* field.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withServerName(String serverName) {
setServerName(serverName);
return this;
}
/**
*
* Use this attribute to convert SDO_GEOMETRY
to GEOJSON
format. By default, DMS calls the
* SDO2GEOJSON
custom function if present and accessible. Or you can create your own custom function
* that mimics the operation of SDOGEOJSON
and set SpatialDataOptionToGeoJsonFunctionName
* to call it instead.
*
*
* @param spatialDataOptionToGeoJsonFunctionName
* Use this attribute to convert SDO_GEOMETRY
to GEOJSON
format. By default, DMS
* calls the SDO2GEOJSON
custom function if present and accessible. Or you can create your own
* custom function that mimics the operation of SDOGEOJSON
and set
* SpatialDataOptionToGeoJsonFunctionName
to call it instead.
*/
public void setSpatialDataOptionToGeoJsonFunctionName(String spatialDataOptionToGeoJsonFunctionName) {
this.spatialDataOptionToGeoJsonFunctionName = spatialDataOptionToGeoJsonFunctionName;
}
/**
*
* Use this attribute to convert SDO_GEOMETRY
to GEOJSON
format. By default, DMS calls the
* SDO2GEOJSON
custom function if present and accessible. Or you can create your own custom function
* that mimics the operation of SDOGEOJSON
and set SpatialDataOptionToGeoJsonFunctionName
* to call it instead.
*
*
* @return Use this attribute to convert SDO_GEOMETRY
to GEOJSON
format. By default, DMS
* calls the SDO2GEOJSON
custom function if present and accessible. Or you can create your own
* custom function that mimics the operation of SDOGEOJSON
and set
* SpatialDataOptionToGeoJsonFunctionName
to call it instead.
*/
public String getSpatialDataOptionToGeoJsonFunctionName() {
return this.spatialDataOptionToGeoJsonFunctionName;
}
/**
*
* Use this attribute to convert SDO_GEOMETRY
to GEOJSON
format. By default, DMS calls the
* SDO2GEOJSON
custom function if present and accessible. Or you can create your own custom function
* that mimics the operation of SDOGEOJSON
and set SpatialDataOptionToGeoJsonFunctionName
* to call it instead.
*
*
* @param spatialDataOptionToGeoJsonFunctionName
* Use this attribute to convert SDO_GEOMETRY
to GEOJSON
format. By default, DMS
* calls the SDO2GEOJSON
custom function if present and accessible. Or you can create your own
* custom function that mimics the operation of SDOGEOJSON
and set
* SpatialDataOptionToGeoJsonFunctionName
to call it instead.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withSpatialDataOptionToGeoJsonFunctionName(String spatialDataOptionToGeoJsonFunctionName) {
setSpatialDataOptionToGeoJsonFunctionName(spatialDataOptionToGeoJsonFunctionName);
return this;
}
/**
*
* Use this attribute to specify a time in minutes for the delay in standby sync. If the source is an Oracle Active
* Data Guard standby database, use this attribute to specify the time lag between primary and standby databases.
*
*
* In DMS, you can create an Oracle CDC task that uses an Active Data Guard standby instance as a source for
* replicating ongoing changes. Doing this eliminates the need to connect to an active database that might be in
* production.
*
*
* @param standbyDelayTime
* Use this attribute to specify a time in minutes for the delay in standby sync. If the source is an Oracle
* Active Data Guard standby database, use this attribute to specify the time lag between primary and standby
* databases.
*
* In DMS, you can create an Oracle CDC task that uses an Active Data Guard standby instance as a source for
* replicating ongoing changes. Doing this eliminates the need to connect to an active database that might be
* in production.
*/
public void setStandbyDelayTime(Integer standbyDelayTime) {
this.standbyDelayTime = standbyDelayTime;
}
/**
*
* Use this attribute to specify a time in minutes for the delay in standby sync. If the source is an Oracle Active
* Data Guard standby database, use this attribute to specify the time lag between primary and standby databases.
*
*
* In DMS, you can create an Oracle CDC task that uses an Active Data Guard standby instance as a source for
* replicating ongoing changes. Doing this eliminates the need to connect to an active database that might be in
* production.
*
*
* @return Use this attribute to specify a time in minutes for the delay in standby sync. If the source is an Oracle
* Active Data Guard standby database, use this attribute to specify the time lag between primary and
* standby databases.
*
* In DMS, you can create an Oracle CDC task that uses an Active Data Guard standby instance as a source for
* replicating ongoing changes. Doing this eliminates the need to connect to an active database that might
* be in production.
*/
public Integer getStandbyDelayTime() {
return this.standbyDelayTime;
}
/**
*
* Use this attribute to specify a time in minutes for the delay in standby sync. If the source is an Oracle Active
* Data Guard standby database, use this attribute to specify the time lag between primary and standby databases.
*
*
* In DMS, you can create an Oracle CDC task that uses an Active Data Guard standby instance as a source for
* replicating ongoing changes. Doing this eliminates the need to connect to an active database that might be in
* production.
*
*
* @param standbyDelayTime
* Use this attribute to specify a time in minutes for the delay in standby sync. If the source is an Oracle
* Active Data Guard standby database, use this attribute to specify the time lag between primary and standby
* databases.
*
* In DMS, you can create an Oracle CDC task that uses an Active Data Guard standby instance as a source for
* replicating ongoing changes. Doing this eliminates the need to connect to an active database that might be
* in production.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withStandbyDelayTime(Integer standbyDelayTime) {
setStandbyDelayTime(standbyDelayTime);
return this;
}
/**
*
* Endpoint connection user name.
*
*
* @param username
* Endpoint connection user name.
*/
public void setUsername(String username) {
this.username = username;
}
/**
*
* Endpoint connection user name.
*
*
* @return Endpoint connection user name.
*/
public String getUsername() {
return this.username;
}
/**
*
* Endpoint connection user name.
*
*
* @param username
* Endpoint connection user name.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withUsername(String username) {
setUsername(username);
return this;
}
/**
*
* Set this attribute to Y to capture change data using the Binary Reader utility. Set
* UseLogminerReader
to N to set this attribute to Y. To use Binary Reader with Amazon RDS for Oracle
* as the source, you set additional attributes. For more information about using this setting with Oracle Automatic
* Storage Management (ASM), see Using
* Oracle LogMiner or DMS Binary Reader for CDC.
*
*
* @param useBFile
* Set this attribute to Y to capture change data using the Binary Reader utility. Set
* UseLogminerReader
to N to set this attribute to Y. To use Binary Reader with Amazon RDS for
* Oracle as the source, you set additional attributes. For more information about using this setting with
* Oracle Automatic Storage Management (ASM), see
* Using Oracle LogMiner or DMS Binary Reader for CDC.
*/
public void setUseBFile(Boolean useBFile) {
this.useBFile = useBFile;
}
/**
*
* Set this attribute to Y to capture change data using the Binary Reader utility. Set
* UseLogminerReader
to N to set this attribute to Y. To use Binary Reader with Amazon RDS for Oracle
* as the source, you set additional attributes. For more information about using this setting with Oracle Automatic
* Storage Management (ASM), see Using
* Oracle LogMiner or DMS Binary Reader for CDC.
*
*
* @return Set this attribute to Y to capture change data using the Binary Reader utility. Set
* UseLogminerReader
to N to set this attribute to Y. To use Binary Reader with Amazon RDS for
* Oracle as the source, you set additional attributes. For more information about using this setting with
* Oracle Automatic Storage Management (ASM), see
* Using Oracle LogMiner or DMS Binary Reader for CDC.
*/
public Boolean getUseBFile() {
return this.useBFile;
}
/**
*
* Set this attribute to Y to capture change data using the Binary Reader utility. Set
* UseLogminerReader
to N to set this attribute to Y. To use Binary Reader with Amazon RDS for Oracle
* as the source, you set additional attributes. For more information about using this setting with Oracle Automatic
* Storage Management (ASM), see Using
* Oracle LogMiner or DMS Binary Reader for CDC.
*
*
* @param useBFile
* Set this attribute to Y to capture change data using the Binary Reader utility. Set
* UseLogminerReader
to N to set this attribute to Y. To use Binary Reader with Amazon RDS for
* Oracle as the source, you set additional attributes. For more information about using this setting with
* Oracle Automatic Storage Management (ASM), see
* Using Oracle LogMiner or DMS Binary Reader for CDC.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withUseBFile(Boolean useBFile) {
setUseBFile(useBFile);
return this;
}
/**
*
* Set this attribute to Y to capture change data using the Binary Reader utility. Set
* UseLogminerReader
to N to set this attribute to Y. To use Binary Reader with Amazon RDS for Oracle
* as the source, you set additional attributes. For more information about using this setting with Oracle Automatic
* Storage Management (ASM), see Using
* Oracle LogMiner or DMS Binary Reader for CDC.
*
*
* @return Set this attribute to Y to capture change data using the Binary Reader utility. Set
* UseLogminerReader
to N to set this attribute to Y. To use Binary Reader with Amazon RDS for
* Oracle as the source, you set additional attributes. For more information about using this setting with
* Oracle Automatic Storage Management (ASM), see
* Using Oracle LogMiner or DMS Binary Reader for CDC.
*/
public Boolean isUseBFile() {
return this.useBFile;
}
/**
*
* Set this attribute to Y to have DMS use a direct path full load. Specify this value to use the direct path
* protocol in the Oracle Call Interface (OCI). By using this OCI protocol, you can bulk-load Oracle target tables
* during a full load.
*
*
* @param useDirectPathFullLoad
* Set this attribute to Y to have DMS use a direct path full load. Specify this value to use the direct path
* protocol in the Oracle Call Interface (OCI). By using this OCI protocol, you can bulk-load Oracle target
* tables during a full load.
*/
public void setUseDirectPathFullLoad(Boolean useDirectPathFullLoad) {
this.useDirectPathFullLoad = useDirectPathFullLoad;
}
/**
*
* Set this attribute to Y to have DMS use a direct path full load. Specify this value to use the direct path
* protocol in the Oracle Call Interface (OCI). By using this OCI protocol, you can bulk-load Oracle target tables
* during a full load.
*
*
* @return Set this attribute to Y to have DMS use a direct path full load. Specify this value to use the direct
* path protocol in the Oracle Call Interface (OCI). By using this OCI protocol, you can bulk-load Oracle
* target tables during a full load.
*/
public Boolean getUseDirectPathFullLoad() {
return this.useDirectPathFullLoad;
}
/**
*
* Set this attribute to Y to have DMS use a direct path full load. Specify this value to use the direct path
* protocol in the Oracle Call Interface (OCI). By using this OCI protocol, you can bulk-load Oracle target tables
* during a full load.
*
*
* @param useDirectPathFullLoad
* Set this attribute to Y to have DMS use a direct path full load. Specify this value to use the direct path
* protocol in the Oracle Call Interface (OCI). By using this OCI protocol, you can bulk-load Oracle target
* tables during a full load.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withUseDirectPathFullLoad(Boolean useDirectPathFullLoad) {
setUseDirectPathFullLoad(useDirectPathFullLoad);
return this;
}
/**
*
* Set this attribute to Y to have DMS use a direct path full load. Specify this value to use the direct path
* protocol in the Oracle Call Interface (OCI). By using this OCI protocol, you can bulk-load Oracle target tables
* during a full load.
*
*
* @return Set this attribute to Y to have DMS use a direct path full load. Specify this value to use the direct
* path protocol in the Oracle Call Interface (OCI). By using this OCI protocol, you can bulk-load Oracle
* target tables during a full load.
*/
public Boolean isUseDirectPathFullLoad() {
return this.useDirectPathFullLoad;
}
/**
*
* Set this attribute to Y to capture change data using the Oracle LogMiner utility (the default). Set this
* attribute to N if you want to access the redo logs as a binary file. When you set UseLogminerReader
* to N, also set UseBfile
to Y. For more information on this setting and using Oracle ASM, see Using
* Oracle LogMiner or DMS Binary Reader for CDC in the DMS User Guide.
*
*
* @param useLogminerReader
* Set this attribute to Y to capture change data using the Oracle LogMiner utility (the default). Set this
* attribute to N if you want to access the redo logs as a binary file. When you set
* UseLogminerReader
to N, also set UseBfile
to Y. For more information on this
* setting and using Oracle ASM, see
* Using Oracle LogMiner or DMS Binary Reader for CDC in the DMS User Guide.
*/
public void setUseLogminerReader(Boolean useLogminerReader) {
this.useLogminerReader = useLogminerReader;
}
/**
*
* Set this attribute to Y to capture change data using the Oracle LogMiner utility (the default). Set this
* attribute to N if you want to access the redo logs as a binary file. When you set UseLogminerReader
* to N, also set UseBfile
to Y. For more information on this setting and using Oracle ASM, see Using
* Oracle LogMiner or DMS Binary Reader for CDC in the DMS User Guide.
*
*
* @return Set this attribute to Y to capture change data using the Oracle LogMiner utility (the default). Set this
* attribute to N if you want to access the redo logs as a binary file. When you set
* UseLogminerReader
to N, also set UseBfile
to Y. For more information on this
* setting and using Oracle ASM, see
* Using Oracle LogMiner or DMS Binary Reader for CDC in the DMS User Guide.
*/
public Boolean getUseLogminerReader() {
return this.useLogminerReader;
}
/**
*
* Set this attribute to Y to capture change data using the Oracle LogMiner utility (the default). Set this
* attribute to N if you want to access the redo logs as a binary file. When you set UseLogminerReader
* to N, also set UseBfile
to Y. For more information on this setting and using Oracle ASM, see Using
* Oracle LogMiner or DMS Binary Reader for CDC in the DMS User Guide.
*
*
* @param useLogminerReader
* Set this attribute to Y to capture change data using the Oracle LogMiner utility (the default). Set this
* attribute to N if you want to access the redo logs as a binary file. When you set
* UseLogminerReader
to N, also set UseBfile
to Y. For more information on this
* setting and using Oracle ASM, see
* Using Oracle LogMiner or DMS Binary Reader for CDC in the DMS User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withUseLogminerReader(Boolean useLogminerReader) {
setUseLogminerReader(useLogminerReader);
return this;
}
/**
*
* Set this attribute to Y to capture change data using the Oracle LogMiner utility (the default). Set this
* attribute to N if you want to access the redo logs as a binary file. When you set UseLogminerReader
* to N, also set UseBfile
to Y. For more information on this setting and using Oracle ASM, see Using
* Oracle LogMiner or DMS Binary Reader for CDC in the DMS User Guide.
*
*
* @return Set this attribute to Y to capture change data using the Oracle LogMiner utility (the default). Set this
* attribute to N if you want to access the redo logs as a binary file. When you set
* UseLogminerReader
to N, also set UseBfile
to Y. For more information on this
* setting and using Oracle ASM, see
* Using Oracle LogMiner or DMS Binary Reader for CDC in the DMS User Guide.
*/
public Boolean isUseLogminerReader() {
return this.useLogminerReader;
}
/**
*
* The full Amazon Resource Name (ARN) of the IAM role that specifies DMS as the trusted entity and grants the
* required permissions to access the value in SecretsManagerSecret
. The role must allow the
* iam:PassRole
action. SecretsManagerSecret
has the value of the Amazon Web Services
* Secrets Manager secret that allows access to the Oracle endpoint.
*
*
*
* You can specify one of two sets of values for these permissions. You can specify the values for this setting and
* SecretsManagerSecretId
. Or you can specify clear-text values for UserName
,
* Password
, ServerName
, and Port
. You can't specify both. For more
* information on creating this SecretsManagerSecret
and the SecretsManagerAccessRoleArn
* and SecretsManagerSecretId
required to access it, see Using
* secrets to access Database Migration Service resources in the Database Migration Service User Guide.
*
*
*
* @param secretsManagerAccessRoleArn
* The full Amazon Resource Name (ARN) of the IAM role that specifies DMS as the trusted entity and grants
* the required permissions to access the value in SecretsManagerSecret
. The role must allow the
* iam:PassRole
action. SecretsManagerSecret
has the value of the Amazon Web
* Services Secrets Manager secret that allows access to the Oracle endpoint.
*
* You can specify one of two sets of values for these permissions. You can specify the values for this
* setting and SecretsManagerSecretId
. Or you can specify clear-text values for
* UserName
, Password
, ServerName
, and Port
. You can't
* specify both. For more information on creating this SecretsManagerSecret
and the
* SecretsManagerAccessRoleArn
and SecretsManagerSecretId
required to access it,
* see Using secrets to access Database Migration Service resources in the Database Migration Service
* User Guide.
*
*/
public void setSecretsManagerAccessRoleArn(String secretsManagerAccessRoleArn) {
this.secretsManagerAccessRoleArn = secretsManagerAccessRoleArn;
}
/**
*
* The full Amazon Resource Name (ARN) of the IAM role that specifies DMS as the trusted entity and grants the
* required permissions to access the value in SecretsManagerSecret
. The role must allow the
* iam:PassRole
action. SecretsManagerSecret
has the value of the Amazon Web Services
* Secrets Manager secret that allows access to the Oracle endpoint.
*
*
*
* You can specify one of two sets of values for these permissions. You can specify the values for this setting and
* SecretsManagerSecretId
. Or you can specify clear-text values for UserName
,
* Password
, ServerName
, and Port
. You can't specify both. For more
* information on creating this SecretsManagerSecret
and the SecretsManagerAccessRoleArn
* and SecretsManagerSecretId
required to access it, see Using
* secrets to access Database Migration Service resources in the Database Migration Service User Guide.
*
*
*
* @return The full Amazon Resource Name (ARN) of the IAM role that specifies DMS as the trusted entity and grants
* the required permissions to access the value in SecretsManagerSecret
. The role must allow
* the iam:PassRole
action. SecretsManagerSecret
has the value of the Amazon Web
* Services Secrets Manager secret that allows access to the Oracle endpoint.
*
* You can specify one of two sets of values for these permissions. You can specify the values for this
* setting and SecretsManagerSecretId
. Or you can specify clear-text values for
* UserName
, Password
, ServerName
, and Port
. You can't
* specify both. For more information on creating this SecretsManagerSecret
and the
* SecretsManagerAccessRoleArn
and SecretsManagerSecretId
required to access it,
* see Using secrets to access Database Migration Service resources in the Database Migration Service
* User Guide.
*
*/
public String getSecretsManagerAccessRoleArn() {
return this.secretsManagerAccessRoleArn;
}
/**
*
* The full Amazon Resource Name (ARN) of the IAM role that specifies DMS as the trusted entity and grants the
* required permissions to access the value in SecretsManagerSecret
. The role must allow the
* iam:PassRole
action. SecretsManagerSecret
has the value of the Amazon Web Services
* Secrets Manager secret that allows access to the Oracle endpoint.
*
*
*
* You can specify one of two sets of values for these permissions. You can specify the values for this setting and
* SecretsManagerSecretId
. Or you can specify clear-text values for UserName
,
* Password
, ServerName
, and Port
. You can't specify both. For more
* information on creating this SecretsManagerSecret
and the SecretsManagerAccessRoleArn
* and SecretsManagerSecretId
required to access it, see Using
* secrets to access Database Migration Service resources in the Database Migration Service User Guide.
*
*
*
* @param secretsManagerAccessRoleArn
* The full Amazon Resource Name (ARN) of the IAM role that specifies DMS as the trusted entity and grants
* the required permissions to access the value in SecretsManagerSecret
. The role must allow the
* iam:PassRole
action. SecretsManagerSecret
has the value of the Amazon Web
* Services Secrets Manager secret that allows access to the Oracle endpoint.
*
* You can specify one of two sets of values for these permissions. You can specify the values for this
* setting and SecretsManagerSecretId
. Or you can specify clear-text values for
* UserName
, Password
, ServerName
, and Port
. You can't
* specify both. For more information on creating this SecretsManagerSecret
and the
* SecretsManagerAccessRoleArn
and SecretsManagerSecretId
required to access it,
* see Using secrets to access Database Migration Service resources in the Database Migration Service
* User Guide.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withSecretsManagerAccessRoleArn(String secretsManagerAccessRoleArn) {
setSecretsManagerAccessRoleArn(secretsManagerAccessRoleArn);
return this;
}
/**
*
* The full ARN, partial ARN, or friendly name of the SecretsManagerSecret
that contains the Oracle
* endpoint connection details.
*
*
* @param secretsManagerSecretId
* The full ARN, partial ARN, or friendly name of the SecretsManagerSecret
that contains the
* Oracle endpoint connection details.
*/
public void setSecretsManagerSecretId(String secretsManagerSecretId) {
this.secretsManagerSecretId = secretsManagerSecretId;
}
/**
*
* The full ARN, partial ARN, or friendly name of the SecretsManagerSecret
that contains the Oracle
* endpoint connection details.
*
*
* @return The full ARN, partial ARN, or friendly name of the SecretsManagerSecret
that contains the
* Oracle endpoint connection details.
*/
public String getSecretsManagerSecretId() {
return this.secretsManagerSecretId;
}
/**
*
* The full ARN, partial ARN, or friendly name of the SecretsManagerSecret
that contains the Oracle
* endpoint connection details.
*
*
* @param secretsManagerSecretId
* The full ARN, partial ARN, or friendly name of the SecretsManagerSecret
that contains the
* Oracle endpoint connection details.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withSecretsManagerSecretId(String secretsManagerSecretId) {
setSecretsManagerSecretId(secretsManagerSecretId);
return this;
}
/**
*
* Required only if your Oracle endpoint uses Automatic Storage Management (ASM). The full ARN of the IAM role that
* specifies DMS as the trusted entity and grants the required permissions to access the
* SecretsManagerOracleAsmSecret
. This SecretsManagerOracleAsmSecret
has the secret value
* that allows access to the Oracle ASM of the endpoint.
*
*
*
* You can specify one of two sets of values for these permissions. You can specify the values for this setting and
* SecretsManagerOracleAsmSecretId
. Or you can specify clear-text values for AsmUser
,
* AsmPassword
, and AsmServerName
. You can't specify both. For more information on
* creating this SecretsManagerOracleAsmSecret
and the
* SecretsManagerOracleAsmAccessRoleArn
and SecretsManagerOracleAsmSecretId
required to
* access it, see Using
* secrets to access Database Migration Service resources in the Database Migration Service User Guide.
*
*
*
* @param secretsManagerOracleAsmAccessRoleArn
* Required only if your Oracle endpoint uses Automatic Storage Management (ASM). The full ARN of the IAM
* role that specifies DMS as the trusted entity and grants the required permissions to access the
* SecretsManagerOracleAsmSecret
. This SecretsManagerOracleAsmSecret
has the secret
* value that allows access to the Oracle ASM of the endpoint.
*
* You can specify one of two sets of values for these permissions. You can specify the values for this
* setting and SecretsManagerOracleAsmSecretId
. Or you can specify clear-text values for
* AsmUser
, AsmPassword
, and AsmServerName
. You can't specify both.
* For more information on creating this SecretsManagerOracleAsmSecret
and the
* SecretsManagerOracleAsmAccessRoleArn
and SecretsManagerOracleAsmSecretId
* required to access it, see Using secrets to access Database Migration Service resources in the Database Migration Service
* User Guide.
*
*/
public void setSecretsManagerOracleAsmAccessRoleArn(String secretsManagerOracleAsmAccessRoleArn) {
this.secretsManagerOracleAsmAccessRoleArn = secretsManagerOracleAsmAccessRoleArn;
}
/**
*
* Required only if your Oracle endpoint uses Automatic Storage Management (ASM). The full ARN of the IAM role that
* specifies DMS as the trusted entity and grants the required permissions to access the
* SecretsManagerOracleAsmSecret
. This SecretsManagerOracleAsmSecret
has the secret value
* that allows access to the Oracle ASM of the endpoint.
*
*
*
* You can specify one of two sets of values for these permissions. You can specify the values for this setting and
* SecretsManagerOracleAsmSecretId
. Or you can specify clear-text values for AsmUser
,
* AsmPassword
, and AsmServerName
. You can't specify both. For more information on
* creating this SecretsManagerOracleAsmSecret
and the
* SecretsManagerOracleAsmAccessRoleArn
and SecretsManagerOracleAsmSecretId
required to
* access it, see Using
* secrets to access Database Migration Service resources in the Database Migration Service User Guide.
*
*
*
* @return Required only if your Oracle endpoint uses Automatic Storage Management (ASM). The full ARN of the IAM
* role that specifies DMS as the trusted entity and grants the required permissions to access the
* SecretsManagerOracleAsmSecret
. This SecretsManagerOracleAsmSecret
has the
* secret value that allows access to the Oracle ASM of the endpoint.
*
* You can specify one of two sets of values for these permissions. You can specify the values for this
* setting and SecretsManagerOracleAsmSecretId
. Or you can specify clear-text values for
* AsmUser
, AsmPassword
, and AsmServerName
. You can't specify both.
* For more information on creating this SecretsManagerOracleAsmSecret
and the
* SecretsManagerOracleAsmAccessRoleArn
and SecretsManagerOracleAsmSecretId
* required to access it, see Using secrets to access Database Migration Service resources in the Database Migration Service
* User Guide.
*
*/
public String getSecretsManagerOracleAsmAccessRoleArn() {
return this.secretsManagerOracleAsmAccessRoleArn;
}
/**
*
* Required only if your Oracle endpoint uses Automatic Storage Management (ASM). The full ARN of the IAM role that
* specifies DMS as the trusted entity and grants the required permissions to access the
* SecretsManagerOracleAsmSecret
. This SecretsManagerOracleAsmSecret
has the secret value
* that allows access to the Oracle ASM of the endpoint.
*
*
*
* You can specify one of two sets of values for these permissions. You can specify the values for this setting and
* SecretsManagerOracleAsmSecretId
. Or you can specify clear-text values for AsmUser
,
* AsmPassword
, and AsmServerName
. You can't specify both. For more information on
* creating this SecretsManagerOracleAsmSecret
and the
* SecretsManagerOracleAsmAccessRoleArn
and SecretsManagerOracleAsmSecretId
required to
* access it, see Using
* secrets to access Database Migration Service resources in the Database Migration Service User Guide.
*
*
*
* @param secretsManagerOracleAsmAccessRoleArn
* Required only if your Oracle endpoint uses Automatic Storage Management (ASM). The full ARN of the IAM
* role that specifies DMS as the trusted entity and grants the required permissions to access the
* SecretsManagerOracleAsmSecret
. This SecretsManagerOracleAsmSecret
has the secret
* value that allows access to the Oracle ASM of the endpoint.
*
* You can specify one of two sets of values for these permissions. You can specify the values for this
* setting and SecretsManagerOracleAsmSecretId
. Or you can specify clear-text values for
* AsmUser
, AsmPassword
, and AsmServerName
. You can't specify both.
* For more information on creating this SecretsManagerOracleAsmSecret
and the
* SecretsManagerOracleAsmAccessRoleArn
and SecretsManagerOracleAsmSecretId
* required to access it, see Using secrets to access Database Migration Service resources in the Database Migration Service
* User Guide.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withSecretsManagerOracleAsmAccessRoleArn(String secretsManagerOracleAsmAccessRoleArn) {
setSecretsManagerOracleAsmAccessRoleArn(secretsManagerOracleAsmAccessRoleArn);
return this;
}
/**
*
* Required only if your Oracle endpoint uses Automatic Storage Management (ASM). The full ARN, partial ARN, or
* friendly name of the SecretsManagerOracleAsmSecret
that contains the Oracle ASM connection details
* for the Oracle endpoint.
*
*
* @param secretsManagerOracleAsmSecretId
* Required only if your Oracle endpoint uses Automatic Storage Management (ASM). The full ARN, partial ARN,
* or friendly name of the SecretsManagerOracleAsmSecret
that contains the Oracle ASM connection
* details for the Oracle endpoint.
*/
public void setSecretsManagerOracleAsmSecretId(String secretsManagerOracleAsmSecretId) {
this.secretsManagerOracleAsmSecretId = secretsManagerOracleAsmSecretId;
}
/**
*
* Required only if your Oracle endpoint uses Automatic Storage Management (ASM). The full ARN, partial ARN, or
* friendly name of the SecretsManagerOracleAsmSecret
that contains the Oracle ASM connection details
* for the Oracle endpoint.
*
*
* @return Required only if your Oracle endpoint uses Automatic Storage Management (ASM). The full ARN, partial ARN,
* or friendly name of the SecretsManagerOracleAsmSecret
that contains the Oracle ASM
* connection details for the Oracle endpoint.
*/
public String getSecretsManagerOracleAsmSecretId() {
return this.secretsManagerOracleAsmSecretId;
}
/**
*
* Required only if your Oracle endpoint uses Automatic Storage Management (ASM). The full ARN, partial ARN, or
* friendly name of the SecretsManagerOracleAsmSecret
that contains the Oracle ASM connection details
* for the Oracle endpoint.
*
*
* @param secretsManagerOracleAsmSecretId
* Required only if your Oracle endpoint uses Automatic Storage Management (ASM). The full ARN, partial ARN,
* or friendly name of the SecretsManagerOracleAsmSecret
that contains the Oracle ASM connection
* details for the Oracle endpoint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withSecretsManagerOracleAsmSecretId(String secretsManagerOracleAsmSecretId) {
setSecretsManagerOracleAsmSecretId(secretsManagerOracleAsmSecretId);
return this;
}
/**
*
* Use the TrimSpaceInChar
source endpoint setting to trim data on CHAR and NCHAR data types during
* migration. The default value is true
.
*
*
* @param trimSpaceInChar
* Use the TrimSpaceInChar
source endpoint setting to trim data on CHAR and NCHAR data types
* during migration. The default value is true
.
*/
public void setTrimSpaceInChar(Boolean trimSpaceInChar) {
this.trimSpaceInChar = trimSpaceInChar;
}
/**
*
* Use the TrimSpaceInChar
source endpoint setting to trim data on CHAR and NCHAR data types during
* migration. The default value is true
.
*
*
* @return Use the TrimSpaceInChar
source endpoint setting to trim data on CHAR and NCHAR data types
* during migration. The default value is true
.
*/
public Boolean getTrimSpaceInChar() {
return this.trimSpaceInChar;
}
/**
*
* Use the TrimSpaceInChar
source endpoint setting to trim data on CHAR and NCHAR data types during
* migration. The default value is true
.
*
*
* @param trimSpaceInChar
* Use the TrimSpaceInChar
source endpoint setting to trim data on CHAR and NCHAR data types
* during migration. The default value is true
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withTrimSpaceInChar(Boolean trimSpaceInChar) {
setTrimSpaceInChar(trimSpaceInChar);
return this;
}
/**
*
* Use the TrimSpaceInChar
source endpoint setting to trim data on CHAR and NCHAR data types during
* migration. The default value is true
.
*
*
* @return Use the TrimSpaceInChar
source endpoint setting to trim data on CHAR and NCHAR data types
* during migration. The default value is true
.
*/
public Boolean isTrimSpaceInChar() {
return this.trimSpaceInChar;
}
/**
*
* When true, converts timestamps with the timezone
datatype to their UTC value.
*
*
* @param convertTimestampWithZoneToUTC
* When true, converts timestamps with the timezone
datatype to their UTC value.
*/
public void setConvertTimestampWithZoneToUTC(Boolean convertTimestampWithZoneToUTC) {
this.convertTimestampWithZoneToUTC = convertTimestampWithZoneToUTC;
}
/**
*
* When true, converts timestamps with the timezone
datatype to their UTC value.
*
*
* @return When true, converts timestamps with the timezone
datatype to their UTC value.
*/
public Boolean getConvertTimestampWithZoneToUTC() {
return this.convertTimestampWithZoneToUTC;
}
/**
*
* When true, converts timestamps with the timezone
datatype to their UTC value.
*
*
* @param convertTimestampWithZoneToUTC
* When true, converts timestamps with the timezone
datatype to their UTC value.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withConvertTimestampWithZoneToUTC(Boolean convertTimestampWithZoneToUTC) {
setConvertTimestampWithZoneToUTC(convertTimestampWithZoneToUTC);
return this;
}
/**
*
* When true, converts timestamps with the timezone
datatype to their UTC value.
*
*
* @return When true, converts timestamps with the timezone
datatype to their UTC value.
*/
public Boolean isConvertTimestampWithZoneToUTC() {
return this.convertTimestampWithZoneToUTC;
}
/**
*
* The timeframe in minutes to check for open transactions for a CDC-only task.
*
*
* You can specify an integer value between 0 (the default) and 240 (the maximum).
*
*
*
* This parameter is only valid in DMS version 3.5.0 and later. DMS supports a window of up to 9.5 hours including
* the value for OpenTransactionWindow
.
*
*
*
* @param openTransactionWindow
* The timeframe in minutes to check for open transactions for a CDC-only task.
*
* You can specify an integer value between 0 (the default) and 240 (the maximum).
*
*
*
* This parameter is only valid in DMS version 3.5.0 and later. DMS supports a window of up to 9.5 hours
* including the value for OpenTransactionWindow
.
*
*/
public void setOpenTransactionWindow(Integer openTransactionWindow) {
this.openTransactionWindow = openTransactionWindow;
}
/**
*
* The timeframe in minutes to check for open transactions for a CDC-only task.
*
*
* You can specify an integer value between 0 (the default) and 240 (the maximum).
*
*
*
* This parameter is only valid in DMS version 3.5.0 and later. DMS supports a window of up to 9.5 hours including
* the value for OpenTransactionWindow
.
*
*
*
* @return The timeframe in minutes to check for open transactions for a CDC-only task.
*
* You can specify an integer value between 0 (the default) and 240 (the maximum).
*
*
*
* This parameter is only valid in DMS version 3.5.0 and later. DMS supports a window of up to 9.5 hours
* including the value for OpenTransactionWindow
.
*
*/
public Integer getOpenTransactionWindow() {
return this.openTransactionWindow;
}
/**
*
* The timeframe in minutes to check for open transactions for a CDC-only task.
*
*
* You can specify an integer value between 0 (the default) and 240 (the maximum).
*
*
*
* This parameter is only valid in DMS version 3.5.0 and later. DMS supports a window of up to 9.5 hours including
* the value for OpenTransactionWindow
.
*
*
*
* @param openTransactionWindow
* The timeframe in minutes to check for open transactions for a CDC-only task.
*
* You can specify an integer value between 0 (the default) and 240 (the maximum).
*
*
*
* This parameter is only valid in DMS version 3.5.0 and later. DMS supports a window of up to 9.5 hours
* including the value for OpenTransactionWindow
.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OracleSettings withOpenTransactionWindow(Integer openTransactionWindow) {
setOpenTransactionWindow(openTransactionWindow);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAddSupplementalLogging() != null)
sb.append("AddSupplementalLogging: ").append(getAddSupplementalLogging()).append(",");
if (getArchivedLogDestId() != null)
sb.append("ArchivedLogDestId: ").append(getArchivedLogDestId()).append(",");
if (getAdditionalArchivedLogDestId() != null)
sb.append("AdditionalArchivedLogDestId: ").append(getAdditionalArchivedLogDestId()).append(",");
if (getExtraArchivedLogDestIds() != null)
sb.append("ExtraArchivedLogDestIds: ").append(getExtraArchivedLogDestIds()).append(",");
if (getAllowSelectNestedTables() != null)
sb.append("AllowSelectNestedTables: ").append(getAllowSelectNestedTables()).append(",");
if (getParallelAsmReadThreads() != null)
sb.append("ParallelAsmReadThreads: ").append(getParallelAsmReadThreads()).append(",");
if (getReadAheadBlocks() != null)
sb.append("ReadAheadBlocks: ").append(getReadAheadBlocks()).append(",");
if (getAccessAlternateDirectly() != null)
sb.append("AccessAlternateDirectly: ").append(getAccessAlternateDirectly()).append(",");
if (getUseAlternateFolderForOnline() != null)
sb.append("UseAlternateFolderForOnline: ").append(getUseAlternateFolderForOnline()).append(",");
if (getOraclePathPrefix() != null)
sb.append("OraclePathPrefix: ").append(getOraclePathPrefix()).append(",");
if (getUsePathPrefix() != null)
sb.append("UsePathPrefix: ").append(getUsePathPrefix()).append(",");
if (getReplacePathPrefix() != null)
sb.append("ReplacePathPrefix: ").append(getReplacePathPrefix()).append(",");
if (getEnableHomogenousTablespace() != null)
sb.append("EnableHomogenousTablespace: ").append(getEnableHomogenousTablespace()).append(",");
if (getDirectPathNoLog() != null)
sb.append("DirectPathNoLog: ").append(getDirectPathNoLog()).append(",");
if (getArchivedLogsOnly() != null)
sb.append("ArchivedLogsOnly: ").append(getArchivedLogsOnly()).append(",");
if (getAsmPassword() != null)
sb.append("AsmPassword: ").append("***Sensitive Data Redacted***").append(",");
if (getAsmServer() != null)
sb.append("AsmServer: ").append(getAsmServer()).append(",");
if (getAsmUser() != null)
sb.append("AsmUser: ").append(getAsmUser()).append(",");
if (getCharLengthSemantics() != null)
sb.append("CharLengthSemantics: ").append(getCharLengthSemantics()).append(",");
if (getDatabaseName() != null)
sb.append("DatabaseName: ").append(getDatabaseName()).append(",");
if (getDirectPathParallelLoad() != null)
sb.append("DirectPathParallelLoad: ").append(getDirectPathParallelLoad()).append(",");
if (getFailTasksOnLobTruncation() != null)
sb.append("FailTasksOnLobTruncation: ").append(getFailTasksOnLobTruncation()).append(",");
if (getNumberDatatypeScale() != null)
sb.append("NumberDatatypeScale: ").append(getNumberDatatypeScale()).append(",");
if (getPassword() != null)
sb.append("Password: ").append("***Sensitive Data Redacted***").append(",");
if (getPort() != null)
sb.append("Port: ").append(getPort()).append(",");
if (getReadTableSpaceName() != null)
sb.append("ReadTableSpaceName: ").append(getReadTableSpaceName()).append(",");
if (getRetryInterval() != null)
sb.append("RetryInterval: ").append(getRetryInterval()).append(",");
if (getSecurityDbEncryption() != null)
sb.append("SecurityDbEncryption: ").append("***Sensitive Data Redacted***").append(",");
if (getSecurityDbEncryptionName() != null)
sb.append("SecurityDbEncryptionName: ").append(getSecurityDbEncryptionName()).append(",");
if (getServerName() != null)
sb.append("ServerName: ").append(getServerName()).append(",");
if (getSpatialDataOptionToGeoJsonFunctionName() != null)
sb.append("SpatialDataOptionToGeoJsonFunctionName: ").append(getSpatialDataOptionToGeoJsonFunctionName()).append(",");
if (getStandbyDelayTime() != null)
sb.append("StandbyDelayTime: ").append(getStandbyDelayTime()).append(",");
if (getUsername() != null)
sb.append("Username: ").append(getUsername()).append(",");
if (getUseBFile() != null)
sb.append("UseBFile: ").append(getUseBFile()).append(",");
if (getUseDirectPathFullLoad() != null)
sb.append("UseDirectPathFullLoad: ").append(getUseDirectPathFullLoad()).append(",");
if (getUseLogminerReader() != null)
sb.append("UseLogminerReader: ").append(getUseLogminerReader()).append(",");
if (getSecretsManagerAccessRoleArn() != null)
sb.append("SecretsManagerAccessRoleArn: ").append(getSecretsManagerAccessRoleArn()).append(",");
if (getSecretsManagerSecretId() != null)
sb.append("SecretsManagerSecretId: ").append(getSecretsManagerSecretId()).append(",");
if (getSecretsManagerOracleAsmAccessRoleArn() != null)
sb.append("SecretsManagerOracleAsmAccessRoleArn: ").append(getSecretsManagerOracleAsmAccessRoleArn()).append(",");
if (getSecretsManagerOracleAsmSecretId() != null)
sb.append("SecretsManagerOracleAsmSecretId: ").append(getSecretsManagerOracleAsmSecretId()).append(",");
if (getTrimSpaceInChar() != null)
sb.append("TrimSpaceInChar: ").append(getTrimSpaceInChar()).append(",");
if (getConvertTimestampWithZoneToUTC() != null)
sb.append("ConvertTimestampWithZoneToUTC: ").append(getConvertTimestampWithZoneToUTC()).append(",");
if (getOpenTransactionWindow() != null)
sb.append("OpenTransactionWindow: ").append(getOpenTransactionWindow());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof OracleSettings == false)
return false;
OracleSettings other = (OracleSettings) obj;
if (other.getAddSupplementalLogging() == null ^ this.getAddSupplementalLogging() == null)
return false;
if (other.getAddSupplementalLogging() != null && other.getAddSupplementalLogging().equals(this.getAddSupplementalLogging()) == false)
return false;
if (other.getArchivedLogDestId() == null ^ this.getArchivedLogDestId() == null)
return false;
if (other.getArchivedLogDestId() != null && other.getArchivedLogDestId().equals(this.getArchivedLogDestId()) == false)
return false;
if (other.getAdditionalArchivedLogDestId() == null ^ this.getAdditionalArchivedLogDestId() == null)
return false;
if (other.getAdditionalArchivedLogDestId() != null && other.getAdditionalArchivedLogDestId().equals(this.getAdditionalArchivedLogDestId()) == false)
return false;
if (other.getExtraArchivedLogDestIds() == null ^ this.getExtraArchivedLogDestIds() == null)
return false;
if (other.getExtraArchivedLogDestIds() != null && other.getExtraArchivedLogDestIds().equals(this.getExtraArchivedLogDestIds()) == false)
return false;
if (other.getAllowSelectNestedTables() == null ^ this.getAllowSelectNestedTables() == null)
return false;
if (other.getAllowSelectNestedTables() != null && other.getAllowSelectNestedTables().equals(this.getAllowSelectNestedTables()) == false)
return false;
if (other.getParallelAsmReadThreads() == null ^ this.getParallelAsmReadThreads() == null)
return false;
if (other.getParallelAsmReadThreads() != null && other.getParallelAsmReadThreads().equals(this.getParallelAsmReadThreads()) == false)
return false;
if (other.getReadAheadBlocks() == null ^ this.getReadAheadBlocks() == null)
return false;
if (other.getReadAheadBlocks() != null && other.getReadAheadBlocks().equals(this.getReadAheadBlocks()) == false)
return false;
if (other.getAccessAlternateDirectly() == null ^ this.getAccessAlternateDirectly() == null)
return false;
if (other.getAccessAlternateDirectly() != null && other.getAccessAlternateDirectly().equals(this.getAccessAlternateDirectly()) == false)
return false;
if (other.getUseAlternateFolderForOnline() == null ^ this.getUseAlternateFolderForOnline() == null)
return false;
if (other.getUseAlternateFolderForOnline() != null && other.getUseAlternateFolderForOnline().equals(this.getUseAlternateFolderForOnline()) == false)
return false;
if (other.getOraclePathPrefix() == null ^ this.getOraclePathPrefix() == null)
return false;
if (other.getOraclePathPrefix() != null && other.getOraclePathPrefix().equals(this.getOraclePathPrefix()) == false)
return false;
if (other.getUsePathPrefix() == null ^ this.getUsePathPrefix() == null)
return false;
if (other.getUsePathPrefix() != null && other.getUsePathPrefix().equals(this.getUsePathPrefix()) == false)
return false;
if (other.getReplacePathPrefix() == null ^ this.getReplacePathPrefix() == null)
return false;
if (other.getReplacePathPrefix() != null && other.getReplacePathPrefix().equals(this.getReplacePathPrefix()) == false)
return false;
if (other.getEnableHomogenousTablespace() == null ^ this.getEnableHomogenousTablespace() == null)
return false;
if (other.getEnableHomogenousTablespace() != null && other.getEnableHomogenousTablespace().equals(this.getEnableHomogenousTablespace()) == false)
return false;
if (other.getDirectPathNoLog() == null ^ this.getDirectPathNoLog() == null)
return false;
if (other.getDirectPathNoLog() != null && other.getDirectPathNoLog().equals(this.getDirectPathNoLog()) == false)
return false;
if (other.getArchivedLogsOnly() == null ^ this.getArchivedLogsOnly() == null)
return false;
if (other.getArchivedLogsOnly() != null && other.getArchivedLogsOnly().equals(this.getArchivedLogsOnly()) == false)
return false;
if (other.getAsmPassword() == null ^ this.getAsmPassword() == null)
return false;
if (other.getAsmPassword() != null && other.getAsmPassword().equals(this.getAsmPassword()) == false)
return false;
if (other.getAsmServer() == null ^ this.getAsmServer() == null)
return false;
if (other.getAsmServer() != null && other.getAsmServer().equals(this.getAsmServer()) == false)
return false;
if (other.getAsmUser() == null ^ this.getAsmUser() == null)
return false;
if (other.getAsmUser() != null && other.getAsmUser().equals(this.getAsmUser()) == false)
return false;
if (other.getCharLengthSemantics() == null ^ this.getCharLengthSemantics() == null)
return false;
if (other.getCharLengthSemantics() != null && other.getCharLengthSemantics().equals(this.getCharLengthSemantics()) == false)
return false;
if (other.getDatabaseName() == null ^ this.getDatabaseName() == null)
return false;
if (other.getDatabaseName() != null && other.getDatabaseName().equals(this.getDatabaseName()) == false)
return false;
if (other.getDirectPathParallelLoad() == null ^ this.getDirectPathParallelLoad() == null)
return false;
if (other.getDirectPathParallelLoad() != null && other.getDirectPathParallelLoad().equals(this.getDirectPathParallelLoad()) == false)
return false;
if (other.getFailTasksOnLobTruncation() == null ^ this.getFailTasksOnLobTruncation() == null)
return false;
if (other.getFailTasksOnLobTruncation() != null && other.getFailTasksOnLobTruncation().equals(this.getFailTasksOnLobTruncation()) == false)
return false;
if (other.getNumberDatatypeScale() == null ^ this.getNumberDatatypeScale() == null)
return false;
if (other.getNumberDatatypeScale() != null && other.getNumberDatatypeScale().equals(this.getNumberDatatypeScale()) == false)
return false;
if (other.getPassword() == null ^ this.getPassword() == null)
return false;
if (other.getPassword() != null && other.getPassword().equals(this.getPassword()) == false)
return false;
if (other.getPort() == null ^ this.getPort() == null)
return false;
if (other.getPort() != null && other.getPort().equals(this.getPort()) == false)
return false;
if (other.getReadTableSpaceName() == null ^ this.getReadTableSpaceName() == null)
return false;
if (other.getReadTableSpaceName() != null && other.getReadTableSpaceName().equals(this.getReadTableSpaceName()) == false)
return false;
if (other.getRetryInterval() == null ^ this.getRetryInterval() == null)
return false;
if (other.getRetryInterval() != null && other.getRetryInterval().equals(this.getRetryInterval()) == false)
return false;
if (other.getSecurityDbEncryption() == null ^ this.getSecurityDbEncryption() == null)
return false;
if (other.getSecurityDbEncryption() != null && other.getSecurityDbEncryption().equals(this.getSecurityDbEncryption()) == false)
return false;
if (other.getSecurityDbEncryptionName() == null ^ this.getSecurityDbEncryptionName() == null)
return false;
if (other.getSecurityDbEncryptionName() != null && other.getSecurityDbEncryptionName().equals(this.getSecurityDbEncryptionName()) == false)
return false;
if (other.getServerName() == null ^ this.getServerName() == null)
return false;
if (other.getServerName() != null && other.getServerName().equals(this.getServerName()) == false)
return false;
if (other.getSpatialDataOptionToGeoJsonFunctionName() == null ^ this.getSpatialDataOptionToGeoJsonFunctionName() == null)
return false;
if (other.getSpatialDataOptionToGeoJsonFunctionName() != null
&& other.getSpatialDataOptionToGeoJsonFunctionName().equals(this.getSpatialDataOptionToGeoJsonFunctionName()) == false)
return false;
if (other.getStandbyDelayTime() == null ^ this.getStandbyDelayTime() == null)
return false;
if (other.getStandbyDelayTime() != null && other.getStandbyDelayTime().equals(this.getStandbyDelayTime()) == false)
return false;
if (other.getUsername() == null ^ this.getUsername() == null)
return false;
if (other.getUsername() != null && other.getUsername().equals(this.getUsername()) == false)
return false;
if (other.getUseBFile() == null ^ this.getUseBFile() == null)
return false;
if (other.getUseBFile() != null && other.getUseBFile().equals(this.getUseBFile()) == false)
return false;
if (other.getUseDirectPathFullLoad() == null ^ this.getUseDirectPathFullLoad() == null)
return false;
if (other.getUseDirectPathFullLoad() != null && other.getUseDirectPathFullLoad().equals(this.getUseDirectPathFullLoad()) == false)
return false;
if (other.getUseLogminerReader() == null ^ this.getUseLogminerReader() == null)
return false;
if (other.getUseLogminerReader() != null && other.getUseLogminerReader().equals(this.getUseLogminerReader()) == false)
return false;
if (other.getSecretsManagerAccessRoleArn() == null ^ this.getSecretsManagerAccessRoleArn() == null)
return false;
if (other.getSecretsManagerAccessRoleArn() != null && other.getSecretsManagerAccessRoleArn().equals(this.getSecretsManagerAccessRoleArn()) == false)
return false;
if (other.getSecretsManagerSecretId() == null ^ this.getSecretsManagerSecretId() == null)
return false;
if (other.getSecretsManagerSecretId() != null && other.getSecretsManagerSecretId().equals(this.getSecretsManagerSecretId()) == false)
return false;
if (other.getSecretsManagerOracleAsmAccessRoleArn() == null ^ this.getSecretsManagerOracleAsmAccessRoleArn() == null)
return false;
if (other.getSecretsManagerOracleAsmAccessRoleArn() != null
&& other.getSecretsManagerOracleAsmAccessRoleArn().equals(this.getSecretsManagerOracleAsmAccessRoleArn()) == false)
return false;
if (other.getSecretsManagerOracleAsmSecretId() == null ^ this.getSecretsManagerOracleAsmSecretId() == null)
return false;
if (other.getSecretsManagerOracleAsmSecretId() != null
&& other.getSecretsManagerOracleAsmSecretId().equals(this.getSecretsManagerOracleAsmSecretId()) == false)
return false;
if (other.getTrimSpaceInChar() == null ^ this.getTrimSpaceInChar() == null)
return false;
if (other.getTrimSpaceInChar() != null && other.getTrimSpaceInChar().equals(this.getTrimSpaceInChar()) == false)
return false;
if (other.getConvertTimestampWithZoneToUTC() == null ^ this.getConvertTimestampWithZoneToUTC() == null)
return false;
if (other.getConvertTimestampWithZoneToUTC() != null
&& other.getConvertTimestampWithZoneToUTC().equals(this.getConvertTimestampWithZoneToUTC()) == false)
return false;
if (other.getOpenTransactionWindow() == null ^ this.getOpenTransactionWindow() == null)
return false;
if (other.getOpenTransactionWindow() != null && other.getOpenTransactionWindow().equals(this.getOpenTransactionWindow()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAddSupplementalLogging() == null) ? 0 : getAddSupplementalLogging().hashCode());
hashCode = prime * hashCode + ((getArchivedLogDestId() == null) ? 0 : getArchivedLogDestId().hashCode());
hashCode = prime * hashCode + ((getAdditionalArchivedLogDestId() == null) ? 0 : getAdditionalArchivedLogDestId().hashCode());
hashCode = prime * hashCode + ((getExtraArchivedLogDestIds() == null) ? 0 : getExtraArchivedLogDestIds().hashCode());
hashCode = prime * hashCode + ((getAllowSelectNestedTables() == null) ? 0 : getAllowSelectNestedTables().hashCode());
hashCode = prime * hashCode + ((getParallelAsmReadThreads() == null) ? 0 : getParallelAsmReadThreads().hashCode());
hashCode = prime * hashCode + ((getReadAheadBlocks() == null) ? 0 : getReadAheadBlocks().hashCode());
hashCode = prime * hashCode + ((getAccessAlternateDirectly() == null) ? 0 : getAccessAlternateDirectly().hashCode());
hashCode = prime * hashCode + ((getUseAlternateFolderForOnline() == null) ? 0 : getUseAlternateFolderForOnline().hashCode());
hashCode = prime * hashCode + ((getOraclePathPrefix() == null) ? 0 : getOraclePathPrefix().hashCode());
hashCode = prime * hashCode + ((getUsePathPrefix() == null) ? 0 : getUsePathPrefix().hashCode());
hashCode = prime * hashCode + ((getReplacePathPrefix() == null) ? 0 : getReplacePathPrefix().hashCode());
hashCode = prime * hashCode + ((getEnableHomogenousTablespace() == null) ? 0 : getEnableHomogenousTablespace().hashCode());
hashCode = prime * hashCode + ((getDirectPathNoLog() == null) ? 0 : getDirectPathNoLog().hashCode());
hashCode = prime * hashCode + ((getArchivedLogsOnly() == null) ? 0 : getArchivedLogsOnly().hashCode());
hashCode = prime * hashCode + ((getAsmPassword() == null) ? 0 : getAsmPassword().hashCode());
hashCode = prime * hashCode + ((getAsmServer() == null) ? 0 : getAsmServer().hashCode());
hashCode = prime * hashCode + ((getAsmUser() == null) ? 0 : getAsmUser().hashCode());
hashCode = prime * hashCode + ((getCharLengthSemantics() == null) ? 0 : getCharLengthSemantics().hashCode());
hashCode = prime * hashCode + ((getDatabaseName() == null) ? 0 : getDatabaseName().hashCode());
hashCode = prime * hashCode + ((getDirectPathParallelLoad() == null) ? 0 : getDirectPathParallelLoad().hashCode());
hashCode = prime * hashCode + ((getFailTasksOnLobTruncation() == null) ? 0 : getFailTasksOnLobTruncation().hashCode());
hashCode = prime * hashCode + ((getNumberDatatypeScale() == null) ? 0 : getNumberDatatypeScale().hashCode());
hashCode = prime * hashCode + ((getPassword() == null) ? 0 : getPassword().hashCode());
hashCode = prime * hashCode + ((getPort() == null) ? 0 : getPort().hashCode());
hashCode = prime * hashCode + ((getReadTableSpaceName() == null) ? 0 : getReadTableSpaceName().hashCode());
hashCode = prime * hashCode + ((getRetryInterval() == null) ? 0 : getRetryInterval().hashCode());
hashCode = prime * hashCode + ((getSecurityDbEncryption() == null) ? 0 : getSecurityDbEncryption().hashCode());
hashCode = prime * hashCode + ((getSecurityDbEncryptionName() == null) ? 0 : getSecurityDbEncryptionName().hashCode());
hashCode = prime * hashCode + ((getServerName() == null) ? 0 : getServerName().hashCode());
hashCode = prime * hashCode + ((getSpatialDataOptionToGeoJsonFunctionName() == null) ? 0 : getSpatialDataOptionToGeoJsonFunctionName().hashCode());
hashCode = prime * hashCode + ((getStandbyDelayTime() == null) ? 0 : getStandbyDelayTime().hashCode());
hashCode = prime * hashCode + ((getUsername() == null) ? 0 : getUsername().hashCode());
hashCode = prime * hashCode + ((getUseBFile() == null) ? 0 : getUseBFile().hashCode());
hashCode = prime * hashCode + ((getUseDirectPathFullLoad() == null) ? 0 : getUseDirectPathFullLoad().hashCode());
hashCode = prime * hashCode + ((getUseLogminerReader() == null) ? 0 : getUseLogminerReader().hashCode());
hashCode = prime * hashCode + ((getSecretsManagerAccessRoleArn() == null) ? 0 : getSecretsManagerAccessRoleArn().hashCode());
hashCode = prime * hashCode + ((getSecretsManagerSecretId() == null) ? 0 : getSecretsManagerSecretId().hashCode());
hashCode = prime * hashCode + ((getSecretsManagerOracleAsmAccessRoleArn() == null) ? 0 : getSecretsManagerOracleAsmAccessRoleArn().hashCode());
hashCode = prime * hashCode + ((getSecretsManagerOracleAsmSecretId() == null) ? 0 : getSecretsManagerOracleAsmSecretId().hashCode());
hashCode = prime * hashCode + ((getTrimSpaceInChar() == null) ? 0 : getTrimSpaceInChar().hashCode());
hashCode = prime * hashCode + ((getConvertTimestampWithZoneToUTC() == null) ? 0 : getConvertTimestampWithZoneToUTC().hashCode());
hashCode = prime * hashCode + ((getOpenTransactionWindow() == null) ? 0 : getOpenTransactionWindow().hashCode());
return hashCode;
}
@Override
public OracleSettings clone() {
try {
return (OracleSettings) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.databasemigrationservice.model.transform.OracleSettingsMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}