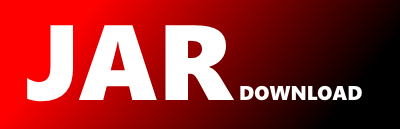
com.amazonaws.services.databasemigrationservice.model.Recommendation Maven / Gradle / Ivy
Show all versions of aws-java-sdk-dms Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.databasemigrationservice.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Provides information that describes a recommendation of a target engine.
*
*
* A recommendation is a set of possible Amazon Web Services target engines that you can choose to migrate your
* source on-premises database. In this set, Fleet Advisor suggests a single target engine as the right sized migration
* destination. To determine this rightsized migration destination, Fleet Advisor uses the inventory metadata and
* metrics from data collector. You can use recommendations before the start of migration to save costs and reduce
* risks.
*
*
* With recommendations, you can explore different target options and compare metrics, so you can make an informed
* decision when you choose the migration target.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Recommendation implements Serializable, Cloneable, StructuredPojo {
/**
*
* The identifier of the source database for which Fleet Advisor provided this recommendation.
*
*/
private String databaseId;
/**
*
* The name of the target engine. Valid values include "rds-aurora-mysql"
,
* "rds-aurora-postgresql"
, "rds-mysql"
, "rds-oracle"
,
* "rds-sql-server"
, and "rds-postgresql"
.
*
*/
private String engineName;
/**
*
* The date when Fleet Advisor created the target engine recommendation.
*
*/
private String createdDate;
/**
*
* The status of the target engine recommendation. Valid values include "alternate"
,
* "in-progress"
, "not-viable"
, and "recommended"
.
*
*/
private String status;
/**
*
* Indicates that this target is the rightsized migration destination.
*
*/
private Boolean preferred;
/**
*
* The settings in JSON format for the preferred target engine parameters. These parameters include capacity,
* resource utilization, and the usage type (production, development, or testing).
*
*/
private RecommendationSettings settings;
/**
*
* The recommendation of a target engine for the specified source database.
*
*/
private RecommendationData data;
/**
*
* The identifier of the source database for which Fleet Advisor provided this recommendation.
*
*
* @param databaseId
* The identifier of the source database for which Fleet Advisor provided this recommendation.
*/
public void setDatabaseId(String databaseId) {
this.databaseId = databaseId;
}
/**
*
* The identifier of the source database for which Fleet Advisor provided this recommendation.
*
*
* @return The identifier of the source database for which Fleet Advisor provided this recommendation.
*/
public String getDatabaseId() {
return this.databaseId;
}
/**
*
* The identifier of the source database for which Fleet Advisor provided this recommendation.
*
*
* @param databaseId
* The identifier of the source database for which Fleet Advisor provided this recommendation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Recommendation withDatabaseId(String databaseId) {
setDatabaseId(databaseId);
return this;
}
/**
*
* The name of the target engine. Valid values include "rds-aurora-mysql"
,
* "rds-aurora-postgresql"
, "rds-mysql"
, "rds-oracle"
,
* "rds-sql-server"
, and "rds-postgresql"
.
*
*
* @param engineName
* The name of the target engine. Valid values include "rds-aurora-mysql"
,
* "rds-aurora-postgresql"
, "rds-mysql"
, "rds-oracle"
,
* "rds-sql-server"
, and "rds-postgresql"
.
*/
public void setEngineName(String engineName) {
this.engineName = engineName;
}
/**
*
* The name of the target engine. Valid values include "rds-aurora-mysql"
,
* "rds-aurora-postgresql"
, "rds-mysql"
, "rds-oracle"
,
* "rds-sql-server"
, and "rds-postgresql"
.
*
*
* @return The name of the target engine. Valid values include "rds-aurora-mysql"
,
* "rds-aurora-postgresql"
, "rds-mysql"
, "rds-oracle"
,
* "rds-sql-server"
, and "rds-postgresql"
.
*/
public String getEngineName() {
return this.engineName;
}
/**
*
* The name of the target engine. Valid values include "rds-aurora-mysql"
,
* "rds-aurora-postgresql"
, "rds-mysql"
, "rds-oracle"
,
* "rds-sql-server"
, and "rds-postgresql"
.
*
*
* @param engineName
* The name of the target engine. Valid values include "rds-aurora-mysql"
,
* "rds-aurora-postgresql"
, "rds-mysql"
, "rds-oracle"
,
* "rds-sql-server"
, and "rds-postgresql"
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Recommendation withEngineName(String engineName) {
setEngineName(engineName);
return this;
}
/**
*
* The date when Fleet Advisor created the target engine recommendation.
*
*
* @param createdDate
* The date when Fleet Advisor created the target engine recommendation.
*/
public void setCreatedDate(String createdDate) {
this.createdDate = createdDate;
}
/**
*
* The date when Fleet Advisor created the target engine recommendation.
*
*
* @return The date when Fleet Advisor created the target engine recommendation.
*/
public String getCreatedDate() {
return this.createdDate;
}
/**
*
* The date when Fleet Advisor created the target engine recommendation.
*
*
* @param createdDate
* The date when Fleet Advisor created the target engine recommendation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Recommendation withCreatedDate(String createdDate) {
setCreatedDate(createdDate);
return this;
}
/**
*
* The status of the target engine recommendation. Valid values include "alternate"
,
* "in-progress"
, "not-viable"
, and "recommended"
.
*
*
* @param status
* The status of the target engine recommendation. Valid values include "alternate"
,
* "in-progress"
, "not-viable"
, and "recommended"
.
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the target engine recommendation. Valid values include "alternate"
,
* "in-progress"
, "not-viable"
, and "recommended"
.
*
*
* @return The status of the target engine recommendation. Valid values include "alternate"
,
* "in-progress"
, "not-viable"
, and "recommended"
.
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of the target engine recommendation. Valid values include "alternate"
,
* "in-progress"
, "not-viable"
, and "recommended"
.
*
*
* @param status
* The status of the target engine recommendation. Valid values include "alternate"
,
* "in-progress"
, "not-viable"
, and "recommended"
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Recommendation withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* Indicates that this target is the rightsized migration destination.
*
*
* @param preferred
* Indicates that this target is the rightsized migration destination.
*/
public void setPreferred(Boolean preferred) {
this.preferred = preferred;
}
/**
*
* Indicates that this target is the rightsized migration destination.
*
*
* @return Indicates that this target is the rightsized migration destination.
*/
public Boolean getPreferred() {
return this.preferred;
}
/**
*
* Indicates that this target is the rightsized migration destination.
*
*
* @param preferred
* Indicates that this target is the rightsized migration destination.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Recommendation withPreferred(Boolean preferred) {
setPreferred(preferred);
return this;
}
/**
*
* Indicates that this target is the rightsized migration destination.
*
*
* @return Indicates that this target is the rightsized migration destination.
*/
public Boolean isPreferred() {
return this.preferred;
}
/**
*
* The settings in JSON format for the preferred target engine parameters. These parameters include capacity,
* resource utilization, and the usage type (production, development, or testing).
*
*
* @param settings
* The settings in JSON format for the preferred target engine parameters. These parameters include capacity,
* resource utilization, and the usage type (production, development, or testing).
*/
public void setSettings(RecommendationSettings settings) {
this.settings = settings;
}
/**
*
* The settings in JSON format for the preferred target engine parameters. These parameters include capacity,
* resource utilization, and the usage type (production, development, or testing).
*
*
* @return The settings in JSON format for the preferred target engine parameters. These parameters include
* capacity, resource utilization, and the usage type (production, development, or testing).
*/
public RecommendationSettings getSettings() {
return this.settings;
}
/**
*
* The settings in JSON format for the preferred target engine parameters. These parameters include capacity,
* resource utilization, and the usage type (production, development, or testing).
*
*
* @param settings
* The settings in JSON format for the preferred target engine parameters. These parameters include capacity,
* resource utilization, and the usage type (production, development, or testing).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Recommendation withSettings(RecommendationSettings settings) {
setSettings(settings);
return this;
}
/**
*
* The recommendation of a target engine for the specified source database.
*
*
* @param data
* The recommendation of a target engine for the specified source database.
*/
public void setData(RecommendationData data) {
this.data = data;
}
/**
*
* The recommendation of a target engine for the specified source database.
*
*
* @return The recommendation of a target engine for the specified source database.
*/
public RecommendationData getData() {
return this.data;
}
/**
*
* The recommendation of a target engine for the specified source database.
*
*
* @param data
* The recommendation of a target engine for the specified source database.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Recommendation withData(RecommendationData data) {
setData(data);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getDatabaseId() != null)
sb.append("DatabaseId: ").append(getDatabaseId()).append(",");
if (getEngineName() != null)
sb.append("EngineName: ").append(getEngineName()).append(",");
if (getCreatedDate() != null)
sb.append("CreatedDate: ").append(getCreatedDate()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getPreferred() != null)
sb.append("Preferred: ").append(getPreferred()).append(",");
if (getSettings() != null)
sb.append("Settings: ").append(getSettings()).append(",");
if (getData() != null)
sb.append("Data: ").append(getData());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Recommendation == false)
return false;
Recommendation other = (Recommendation) obj;
if (other.getDatabaseId() == null ^ this.getDatabaseId() == null)
return false;
if (other.getDatabaseId() != null && other.getDatabaseId().equals(this.getDatabaseId()) == false)
return false;
if (other.getEngineName() == null ^ this.getEngineName() == null)
return false;
if (other.getEngineName() != null && other.getEngineName().equals(this.getEngineName()) == false)
return false;
if (other.getCreatedDate() == null ^ this.getCreatedDate() == null)
return false;
if (other.getCreatedDate() != null && other.getCreatedDate().equals(this.getCreatedDate()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getPreferred() == null ^ this.getPreferred() == null)
return false;
if (other.getPreferred() != null && other.getPreferred().equals(this.getPreferred()) == false)
return false;
if (other.getSettings() == null ^ this.getSettings() == null)
return false;
if (other.getSettings() != null && other.getSettings().equals(this.getSettings()) == false)
return false;
if (other.getData() == null ^ this.getData() == null)
return false;
if (other.getData() != null && other.getData().equals(this.getData()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getDatabaseId() == null) ? 0 : getDatabaseId().hashCode());
hashCode = prime * hashCode + ((getEngineName() == null) ? 0 : getEngineName().hashCode());
hashCode = prime * hashCode + ((getCreatedDate() == null) ? 0 : getCreatedDate().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getPreferred() == null) ? 0 : getPreferred().hashCode());
hashCode = prime * hashCode + ((getSettings() == null) ? 0 : getSettings().hashCode());
hashCode = prime * hashCode + ((getData() == null) ? 0 : getData().hashCode());
return hashCode;
}
@Override
public Recommendation clone() {
try {
return (Recommendation) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.databasemigrationservice.model.transform.RecommendationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}