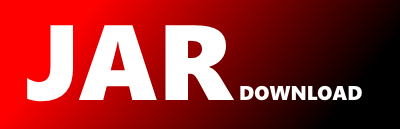
com.amazonaws.services.databasemigrationservice.model.ReplicationStats Maven / Gradle / Ivy
Show all versions of aws-java-sdk-dms Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.databasemigrationservice.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* This object provides a collection of statistics about a serverless replication.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ReplicationStats implements Serializable, Cloneable, StructuredPojo {
/**
*
* The percent complete for the full load serverless replication.
*
*/
private Integer fullLoadProgressPercent;
/**
*
* The elapsed time of the replication, in milliseconds.
*
*/
private Long elapsedTimeMillis;
/**
*
* The number of tables loaded for this replication.
*
*/
private Integer tablesLoaded;
/**
*
* The number of tables currently loading for this replication.
*
*/
private Integer tablesLoading;
/**
*
* The number of tables queued for this replication.
*
*/
private Integer tablesQueued;
/**
*
* The number of errors that have occured for this replication.
*
*/
private Integer tablesErrored;
/**
*
* The date the replication was started either with a fresh start or a target reload.
*
*/
private java.util.Date freshStartDate;
/**
*
* The date the replication is scheduled to start.
*
*/
private java.util.Date startDate;
/**
*
* The date the replication was stopped.
*
*/
private java.util.Date stopDate;
/**
*
* The date the replication full load was started.
*
*/
private java.util.Date fullLoadStartDate;
/**
*
* The date the replication full load was finished.
*
*/
private java.util.Date fullLoadFinishDate;
/**
*
* The percent complete for the full load serverless replication.
*
*
* @param fullLoadProgressPercent
* The percent complete for the full load serverless replication.
*/
public void setFullLoadProgressPercent(Integer fullLoadProgressPercent) {
this.fullLoadProgressPercent = fullLoadProgressPercent;
}
/**
*
* The percent complete for the full load serverless replication.
*
*
* @return The percent complete for the full load serverless replication.
*/
public Integer getFullLoadProgressPercent() {
return this.fullLoadProgressPercent;
}
/**
*
* The percent complete for the full load serverless replication.
*
*
* @param fullLoadProgressPercent
* The percent complete for the full load serverless replication.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationStats withFullLoadProgressPercent(Integer fullLoadProgressPercent) {
setFullLoadProgressPercent(fullLoadProgressPercent);
return this;
}
/**
*
* The elapsed time of the replication, in milliseconds.
*
*
* @param elapsedTimeMillis
* The elapsed time of the replication, in milliseconds.
*/
public void setElapsedTimeMillis(Long elapsedTimeMillis) {
this.elapsedTimeMillis = elapsedTimeMillis;
}
/**
*
* The elapsed time of the replication, in milliseconds.
*
*
* @return The elapsed time of the replication, in milliseconds.
*/
public Long getElapsedTimeMillis() {
return this.elapsedTimeMillis;
}
/**
*
* The elapsed time of the replication, in milliseconds.
*
*
* @param elapsedTimeMillis
* The elapsed time of the replication, in milliseconds.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationStats withElapsedTimeMillis(Long elapsedTimeMillis) {
setElapsedTimeMillis(elapsedTimeMillis);
return this;
}
/**
*
* The number of tables loaded for this replication.
*
*
* @param tablesLoaded
* The number of tables loaded for this replication.
*/
public void setTablesLoaded(Integer tablesLoaded) {
this.tablesLoaded = tablesLoaded;
}
/**
*
* The number of tables loaded for this replication.
*
*
* @return The number of tables loaded for this replication.
*/
public Integer getTablesLoaded() {
return this.tablesLoaded;
}
/**
*
* The number of tables loaded for this replication.
*
*
* @param tablesLoaded
* The number of tables loaded for this replication.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationStats withTablesLoaded(Integer tablesLoaded) {
setTablesLoaded(tablesLoaded);
return this;
}
/**
*
* The number of tables currently loading for this replication.
*
*
* @param tablesLoading
* The number of tables currently loading for this replication.
*/
public void setTablesLoading(Integer tablesLoading) {
this.tablesLoading = tablesLoading;
}
/**
*
* The number of tables currently loading for this replication.
*
*
* @return The number of tables currently loading for this replication.
*/
public Integer getTablesLoading() {
return this.tablesLoading;
}
/**
*
* The number of tables currently loading for this replication.
*
*
* @param tablesLoading
* The number of tables currently loading for this replication.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationStats withTablesLoading(Integer tablesLoading) {
setTablesLoading(tablesLoading);
return this;
}
/**
*
* The number of tables queued for this replication.
*
*
* @param tablesQueued
* The number of tables queued for this replication.
*/
public void setTablesQueued(Integer tablesQueued) {
this.tablesQueued = tablesQueued;
}
/**
*
* The number of tables queued for this replication.
*
*
* @return The number of tables queued for this replication.
*/
public Integer getTablesQueued() {
return this.tablesQueued;
}
/**
*
* The number of tables queued for this replication.
*
*
* @param tablesQueued
* The number of tables queued for this replication.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationStats withTablesQueued(Integer tablesQueued) {
setTablesQueued(tablesQueued);
return this;
}
/**
*
* The number of errors that have occured for this replication.
*
*
* @param tablesErrored
* The number of errors that have occured for this replication.
*/
public void setTablesErrored(Integer tablesErrored) {
this.tablesErrored = tablesErrored;
}
/**
*
* The number of errors that have occured for this replication.
*
*
* @return The number of errors that have occured for this replication.
*/
public Integer getTablesErrored() {
return this.tablesErrored;
}
/**
*
* The number of errors that have occured for this replication.
*
*
* @param tablesErrored
* The number of errors that have occured for this replication.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationStats withTablesErrored(Integer tablesErrored) {
setTablesErrored(tablesErrored);
return this;
}
/**
*
* The date the replication was started either with a fresh start or a target reload.
*
*
* @param freshStartDate
* The date the replication was started either with a fresh start or a target reload.
*/
public void setFreshStartDate(java.util.Date freshStartDate) {
this.freshStartDate = freshStartDate;
}
/**
*
* The date the replication was started either with a fresh start or a target reload.
*
*
* @return The date the replication was started either with a fresh start or a target reload.
*/
public java.util.Date getFreshStartDate() {
return this.freshStartDate;
}
/**
*
* The date the replication was started either with a fresh start or a target reload.
*
*
* @param freshStartDate
* The date the replication was started either with a fresh start or a target reload.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationStats withFreshStartDate(java.util.Date freshStartDate) {
setFreshStartDate(freshStartDate);
return this;
}
/**
*
* The date the replication is scheduled to start.
*
*
* @param startDate
* The date the replication is scheduled to start.
*/
public void setStartDate(java.util.Date startDate) {
this.startDate = startDate;
}
/**
*
* The date the replication is scheduled to start.
*
*
* @return The date the replication is scheduled to start.
*/
public java.util.Date getStartDate() {
return this.startDate;
}
/**
*
* The date the replication is scheduled to start.
*
*
* @param startDate
* The date the replication is scheduled to start.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationStats withStartDate(java.util.Date startDate) {
setStartDate(startDate);
return this;
}
/**
*
* The date the replication was stopped.
*
*
* @param stopDate
* The date the replication was stopped.
*/
public void setStopDate(java.util.Date stopDate) {
this.stopDate = stopDate;
}
/**
*
* The date the replication was stopped.
*
*
* @return The date the replication was stopped.
*/
public java.util.Date getStopDate() {
return this.stopDate;
}
/**
*
* The date the replication was stopped.
*
*
* @param stopDate
* The date the replication was stopped.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationStats withStopDate(java.util.Date stopDate) {
setStopDate(stopDate);
return this;
}
/**
*
* The date the replication full load was started.
*
*
* @param fullLoadStartDate
* The date the replication full load was started.
*/
public void setFullLoadStartDate(java.util.Date fullLoadStartDate) {
this.fullLoadStartDate = fullLoadStartDate;
}
/**
*
* The date the replication full load was started.
*
*
* @return The date the replication full load was started.
*/
public java.util.Date getFullLoadStartDate() {
return this.fullLoadStartDate;
}
/**
*
* The date the replication full load was started.
*
*
* @param fullLoadStartDate
* The date the replication full load was started.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationStats withFullLoadStartDate(java.util.Date fullLoadStartDate) {
setFullLoadStartDate(fullLoadStartDate);
return this;
}
/**
*
* The date the replication full load was finished.
*
*
* @param fullLoadFinishDate
* The date the replication full load was finished.
*/
public void setFullLoadFinishDate(java.util.Date fullLoadFinishDate) {
this.fullLoadFinishDate = fullLoadFinishDate;
}
/**
*
* The date the replication full load was finished.
*
*
* @return The date the replication full load was finished.
*/
public java.util.Date getFullLoadFinishDate() {
return this.fullLoadFinishDate;
}
/**
*
* The date the replication full load was finished.
*
*
* @param fullLoadFinishDate
* The date the replication full load was finished.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationStats withFullLoadFinishDate(java.util.Date fullLoadFinishDate) {
setFullLoadFinishDate(fullLoadFinishDate);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getFullLoadProgressPercent() != null)
sb.append("FullLoadProgressPercent: ").append(getFullLoadProgressPercent()).append(",");
if (getElapsedTimeMillis() != null)
sb.append("ElapsedTimeMillis: ").append(getElapsedTimeMillis()).append(",");
if (getTablesLoaded() != null)
sb.append("TablesLoaded: ").append(getTablesLoaded()).append(",");
if (getTablesLoading() != null)
sb.append("TablesLoading: ").append(getTablesLoading()).append(",");
if (getTablesQueued() != null)
sb.append("TablesQueued: ").append(getTablesQueued()).append(",");
if (getTablesErrored() != null)
sb.append("TablesErrored: ").append(getTablesErrored()).append(",");
if (getFreshStartDate() != null)
sb.append("FreshStartDate: ").append(getFreshStartDate()).append(",");
if (getStartDate() != null)
sb.append("StartDate: ").append(getStartDate()).append(",");
if (getStopDate() != null)
sb.append("StopDate: ").append(getStopDate()).append(",");
if (getFullLoadStartDate() != null)
sb.append("FullLoadStartDate: ").append(getFullLoadStartDate()).append(",");
if (getFullLoadFinishDate() != null)
sb.append("FullLoadFinishDate: ").append(getFullLoadFinishDate());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ReplicationStats == false)
return false;
ReplicationStats other = (ReplicationStats) obj;
if (other.getFullLoadProgressPercent() == null ^ this.getFullLoadProgressPercent() == null)
return false;
if (other.getFullLoadProgressPercent() != null && other.getFullLoadProgressPercent().equals(this.getFullLoadProgressPercent()) == false)
return false;
if (other.getElapsedTimeMillis() == null ^ this.getElapsedTimeMillis() == null)
return false;
if (other.getElapsedTimeMillis() != null && other.getElapsedTimeMillis().equals(this.getElapsedTimeMillis()) == false)
return false;
if (other.getTablesLoaded() == null ^ this.getTablesLoaded() == null)
return false;
if (other.getTablesLoaded() != null && other.getTablesLoaded().equals(this.getTablesLoaded()) == false)
return false;
if (other.getTablesLoading() == null ^ this.getTablesLoading() == null)
return false;
if (other.getTablesLoading() != null && other.getTablesLoading().equals(this.getTablesLoading()) == false)
return false;
if (other.getTablesQueued() == null ^ this.getTablesQueued() == null)
return false;
if (other.getTablesQueued() != null && other.getTablesQueued().equals(this.getTablesQueued()) == false)
return false;
if (other.getTablesErrored() == null ^ this.getTablesErrored() == null)
return false;
if (other.getTablesErrored() != null && other.getTablesErrored().equals(this.getTablesErrored()) == false)
return false;
if (other.getFreshStartDate() == null ^ this.getFreshStartDate() == null)
return false;
if (other.getFreshStartDate() != null && other.getFreshStartDate().equals(this.getFreshStartDate()) == false)
return false;
if (other.getStartDate() == null ^ this.getStartDate() == null)
return false;
if (other.getStartDate() != null && other.getStartDate().equals(this.getStartDate()) == false)
return false;
if (other.getStopDate() == null ^ this.getStopDate() == null)
return false;
if (other.getStopDate() != null && other.getStopDate().equals(this.getStopDate()) == false)
return false;
if (other.getFullLoadStartDate() == null ^ this.getFullLoadStartDate() == null)
return false;
if (other.getFullLoadStartDate() != null && other.getFullLoadStartDate().equals(this.getFullLoadStartDate()) == false)
return false;
if (other.getFullLoadFinishDate() == null ^ this.getFullLoadFinishDate() == null)
return false;
if (other.getFullLoadFinishDate() != null && other.getFullLoadFinishDate().equals(this.getFullLoadFinishDate()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getFullLoadProgressPercent() == null) ? 0 : getFullLoadProgressPercent().hashCode());
hashCode = prime * hashCode + ((getElapsedTimeMillis() == null) ? 0 : getElapsedTimeMillis().hashCode());
hashCode = prime * hashCode + ((getTablesLoaded() == null) ? 0 : getTablesLoaded().hashCode());
hashCode = prime * hashCode + ((getTablesLoading() == null) ? 0 : getTablesLoading().hashCode());
hashCode = prime * hashCode + ((getTablesQueued() == null) ? 0 : getTablesQueued().hashCode());
hashCode = prime * hashCode + ((getTablesErrored() == null) ? 0 : getTablesErrored().hashCode());
hashCode = prime * hashCode + ((getFreshStartDate() == null) ? 0 : getFreshStartDate().hashCode());
hashCode = prime * hashCode + ((getStartDate() == null) ? 0 : getStartDate().hashCode());
hashCode = prime * hashCode + ((getStopDate() == null) ? 0 : getStopDate().hashCode());
hashCode = prime * hashCode + ((getFullLoadStartDate() == null) ? 0 : getFullLoadStartDate().hashCode());
hashCode = prime * hashCode + ((getFullLoadFinishDate() == null) ? 0 : getFullLoadFinishDate().hashCode());
return hashCode;
}
@Override
public ReplicationStats clone() {
try {
return (ReplicationStats) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.databasemigrationservice.model.transform.ReplicationStatsMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}