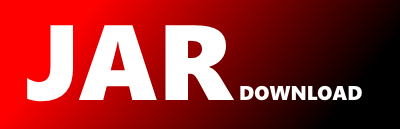
com.amazonaws.services.databasemigrationservice.model.ReplicationSubnetGroup Maven / Gradle / Ivy
Show all versions of aws-java-sdk-dms Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.databasemigrationservice.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Describes a subnet group in response to a request by the DescribeReplicationSubnetGroups
operation.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ReplicationSubnetGroup implements Serializable, Cloneable, StructuredPojo {
/**
*
* The identifier of the replication instance subnet group.
*
*/
private String replicationSubnetGroupIdentifier;
/**
*
* A description for the replication subnet group.
*
*/
private String replicationSubnetGroupDescription;
/**
*
* The ID of the VPC.
*
*/
private String vpcId;
/**
*
* The status of the subnet group.
*
*/
private String subnetGroupStatus;
/**
*
* The subnets that are in the subnet group.
*
*/
private java.util.List subnets;
/**
*
* The IP addressing protocol supported by the subnet group. This is used by a replication instance with values such
* as IPv4 only or Dual-stack that supports both IPv4 and IPv6 addressing. IPv6 only is not yet supported.
*
*/
private java.util.List supportedNetworkTypes;
/**
*
* The identifier of the replication instance subnet group.
*
*
* @param replicationSubnetGroupIdentifier
* The identifier of the replication instance subnet group.
*/
public void setReplicationSubnetGroupIdentifier(String replicationSubnetGroupIdentifier) {
this.replicationSubnetGroupIdentifier = replicationSubnetGroupIdentifier;
}
/**
*
* The identifier of the replication instance subnet group.
*
*
* @return The identifier of the replication instance subnet group.
*/
public String getReplicationSubnetGroupIdentifier() {
return this.replicationSubnetGroupIdentifier;
}
/**
*
* The identifier of the replication instance subnet group.
*
*
* @param replicationSubnetGroupIdentifier
* The identifier of the replication instance subnet group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationSubnetGroup withReplicationSubnetGroupIdentifier(String replicationSubnetGroupIdentifier) {
setReplicationSubnetGroupIdentifier(replicationSubnetGroupIdentifier);
return this;
}
/**
*
* A description for the replication subnet group.
*
*
* @param replicationSubnetGroupDescription
* A description for the replication subnet group.
*/
public void setReplicationSubnetGroupDescription(String replicationSubnetGroupDescription) {
this.replicationSubnetGroupDescription = replicationSubnetGroupDescription;
}
/**
*
* A description for the replication subnet group.
*
*
* @return A description for the replication subnet group.
*/
public String getReplicationSubnetGroupDescription() {
return this.replicationSubnetGroupDescription;
}
/**
*
* A description for the replication subnet group.
*
*
* @param replicationSubnetGroupDescription
* A description for the replication subnet group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationSubnetGroup withReplicationSubnetGroupDescription(String replicationSubnetGroupDescription) {
setReplicationSubnetGroupDescription(replicationSubnetGroupDescription);
return this;
}
/**
*
* The ID of the VPC.
*
*
* @param vpcId
* The ID of the VPC.
*/
public void setVpcId(String vpcId) {
this.vpcId = vpcId;
}
/**
*
* The ID of the VPC.
*
*
* @return The ID of the VPC.
*/
public String getVpcId() {
return this.vpcId;
}
/**
*
* The ID of the VPC.
*
*
* @param vpcId
* The ID of the VPC.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationSubnetGroup withVpcId(String vpcId) {
setVpcId(vpcId);
return this;
}
/**
*
* The status of the subnet group.
*
*
* @param subnetGroupStatus
* The status of the subnet group.
*/
public void setSubnetGroupStatus(String subnetGroupStatus) {
this.subnetGroupStatus = subnetGroupStatus;
}
/**
*
* The status of the subnet group.
*
*
* @return The status of the subnet group.
*/
public String getSubnetGroupStatus() {
return this.subnetGroupStatus;
}
/**
*
* The status of the subnet group.
*
*
* @param subnetGroupStatus
* The status of the subnet group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationSubnetGroup withSubnetGroupStatus(String subnetGroupStatus) {
setSubnetGroupStatus(subnetGroupStatus);
return this;
}
/**
*
* The subnets that are in the subnet group.
*
*
* @return The subnets that are in the subnet group.
*/
public java.util.List getSubnets() {
return subnets;
}
/**
*
* The subnets that are in the subnet group.
*
*
* @param subnets
* The subnets that are in the subnet group.
*/
public void setSubnets(java.util.Collection subnets) {
if (subnets == null) {
this.subnets = null;
return;
}
this.subnets = new java.util.ArrayList(subnets);
}
/**
*
* The subnets that are in the subnet group.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSubnets(java.util.Collection)} or {@link #withSubnets(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param subnets
* The subnets that are in the subnet group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationSubnetGroup withSubnets(Subnet... subnets) {
if (this.subnets == null) {
setSubnets(new java.util.ArrayList(subnets.length));
}
for (Subnet ele : subnets) {
this.subnets.add(ele);
}
return this;
}
/**
*
* The subnets that are in the subnet group.
*
*
* @param subnets
* The subnets that are in the subnet group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationSubnetGroup withSubnets(java.util.Collection subnets) {
setSubnets(subnets);
return this;
}
/**
*
* The IP addressing protocol supported by the subnet group. This is used by a replication instance with values such
* as IPv4 only or Dual-stack that supports both IPv4 and IPv6 addressing. IPv6 only is not yet supported.
*
*
* @return The IP addressing protocol supported by the subnet group. This is used by a replication instance with
* values such as IPv4 only or Dual-stack that supports both IPv4 and IPv6 addressing. IPv6 only is not yet
* supported.
*/
public java.util.List getSupportedNetworkTypes() {
return supportedNetworkTypes;
}
/**
*
* The IP addressing protocol supported by the subnet group. This is used by a replication instance with values such
* as IPv4 only or Dual-stack that supports both IPv4 and IPv6 addressing. IPv6 only is not yet supported.
*
*
* @param supportedNetworkTypes
* The IP addressing protocol supported by the subnet group. This is used by a replication instance with
* values such as IPv4 only or Dual-stack that supports both IPv4 and IPv6 addressing. IPv6 only is not yet
* supported.
*/
public void setSupportedNetworkTypes(java.util.Collection supportedNetworkTypes) {
if (supportedNetworkTypes == null) {
this.supportedNetworkTypes = null;
return;
}
this.supportedNetworkTypes = new java.util.ArrayList(supportedNetworkTypes);
}
/**
*
* The IP addressing protocol supported by the subnet group. This is used by a replication instance with values such
* as IPv4 only or Dual-stack that supports both IPv4 and IPv6 addressing. IPv6 only is not yet supported.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSupportedNetworkTypes(java.util.Collection)} or
* {@link #withSupportedNetworkTypes(java.util.Collection)} if you want to override the existing values.
*
*
* @param supportedNetworkTypes
* The IP addressing protocol supported by the subnet group. This is used by a replication instance with
* values such as IPv4 only or Dual-stack that supports both IPv4 and IPv6 addressing. IPv6 only is not yet
* supported.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationSubnetGroup withSupportedNetworkTypes(String... supportedNetworkTypes) {
if (this.supportedNetworkTypes == null) {
setSupportedNetworkTypes(new java.util.ArrayList(supportedNetworkTypes.length));
}
for (String ele : supportedNetworkTypes) {
this.supportedNetworkTypes.add(ele);
}
return this;
}
/**
*
* The IP addressing protocol supported by the subnet group. This is used by a replication instance with values such
* as IPv4 only or Dual-stack that supports both IPv4 and IPv6 addressing. IPv6 only is not yet supported.
*
*
* @param supportedNetworkTypes
* The IP addressing protocol supported by the subnet group. This is used by a replication instance with
* values such as IPv4 only or Dual-stack that supports both IPv4 and IPv6 addressing. IPv6 only is not yet
* supported.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationSubnetGroup withSupportedNetworkTypes(java.util.Collection supportedNetworkTypes) {
setSupportedNetworkTypes(supportedNetworkTypes);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getReplicationSubnetGroupIdentifier() != null)
sb.append("ReplicationSubnetGroupIdentifier: ").append(getReplicationSubnetGroupIdentifier()).append(",");
if (getReplicationSubnetGroupDescription() != null)
sb.append("ReplicationSubnetGroupDescription: ").append(getReplicationSubnetGroupDescription()).append(",");
if (getVpcId() != null)
sb.append("VpcId: ").append(getVpcId()).append(",");
if (getSubnetGroupStatus() != null)
sb.append("SubnetGroupStatus: ").append(getSubnetGroupStatus()).append(",");
if (getSubnets() != null)
sb.append("Subnets: ").append(getSubnets()).append(",");
if (getSupportedNetworkTypes() != null)
sb.append("SupportedNetworkTypes: ").append(getSupportedNetworkTypes());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ReplicationSubnetGroup == false)
return false;
ReplicationSubnetGroup other = (ReplicationSubnetGroup) obj;
if (other.getReplicationSubnetGroupIdentifier() == null ^ this.getReplicationSubnetGroupIdentifier() == null)
return false;
if (other.getReplicationSubnetGroupIdentifier() != null
&& other.getReplicationSubnetGroupIdentifier().equals(this.getReplicationSubnetGroupIdentifier()) == false)
return false;
if (other.getReplicationSubnetGroupDescription() == null ^ this.getReplicationSubnetGroupDescription() == null)
return false;
if (other.getReplicationSubnetGroupDescription() != null
&& other.getReplicationSubnetGroupDescription().equals(this.getReplicationSubnetGroupDescription()) == false)
return false;
if (other.getVpcId() == null ^ this.getVpcId() == null)
return false;
if (other.getVpcId() != null && other.getVpcId().equals(this.getVpcId()) == false)
return false;
if (other.getSubnetGroupStatus() == null ^ this.getSubnetGroupStatus() == null)
return false;
if (other.getSubnetGroupStatus() != null && other.getSubnetGroupStatus().equals(this.getSubnetGroupStatus()) == false)
return false;
if (other.getSubnets() == null ^ this.getSubnets() == null)
return false;
if (other.getSubnets() != null && other.getSubnets().equals(this.getSubnets()) == false)
return false;
if (other.getSupportedNetworkTypes() == null ^ this.getSupportedNetworkTypes() == null)
return false;
if (other.getSupportedNetworkTypes() != null && other.getSupportedNetworkTypes().equals(this.getSupportedNetworkTypes()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getReplicationSubnetGroupIdentifier() == null) ? 0 : getReplicationSubnetGroupIdentifier().hashCode());
hashCode = prime * hashCode + ((getReplicationSubnetGroupDescription() == null) ? 0 : getReplicationSubnetGroupDescription().hashCode());
hashCode = prime * hashCode + ((getVpcId() == null) ? 0 : getVpcId().hashCode());
hashCode = prime * hashCode + ((getSubnetGroupStatus() == null) ? 0 : getSubnetGroupStatus().hashCode());
hashCode = prime * hashCode + ((getSubnets() == null) ? 0 : getSubnets().hashCode());
hashCode = prime * hashCode + ((getSupportedNetworkTypes() == null) ? 0 : getSupportedNetworkTypes().hashCode());
return hashCode;
}
@Override
public ReplicationSubnetGroup clone() {
try {
return (ReplicationSubnetGroup) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.databasemigrationservice.model.transform.ReplicationSubnetGroupMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}