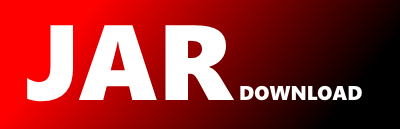
com.amazonaws.services.databasemigrationservice.model.ReplicationTaskAssessmentRun Maven / Gradle / Ivy
Show all versions of aws-java-sdk-dms Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.databasemigrationservice.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Provides information that describes a premigration assessment run that you have started using the
* StartReplicationTaskAssessmentRun
operation.
*
*
* Some of the information appears based on other operations that can return the
* ReplicationTaskAssessmentRun
object.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ReplicationTaskAssessmentRun implements Serializable, Cloneable, StructuredPojo {
/**
*
* Amazon Resource Name (ARN) of this assessment run.
*
*/
private String replicationTaskAssessmentRunArn;
/**
*
* ARN of the migration task associated with this premigration assessment run.
*
*/
private String replicationTaskArn;
/**
*
* Assessment run status.
*
*
* This status can have one of the following values:
*
*
* -
*
* "cancelling"
– The assessment run was canceled by the
* CancelReplicationTaskAssessmentRun
operation.
*
*
* -
*
* "deleting"
– The assessment run was deleted by the DeleteReplicationTaskAssessmentRun
* operation.
*
*
* -
*
* "failed"
– At least one individual assessment completed with a failed
status.
*
*
* -
*
* "error-provisioning"
– An internal error occurred while resources were provisioned (during
* provisioning
status).
*
*
* -
*
* "error-executing"
– An internal error occurred while individual assessments ran (during
* running
status).
*
*
* -
*
* "invalid state"
– The assessment run is in an unknown state.
*
*
* -
*
* "passed"
– All individual assessments have completed, and none has a failed
status.
*
*
* -
*
* "provisioning"
– Resources required to run individual assessments are being provisioned.
*
*
* -
*
* "running"
– Individual assessments are being run.
*
*
* -
*
* "starting"
– The assessment run is starting, but resources are not yet being provisioned for
* individual assessments.
*
*
*
*/
private String status;
/**
*
* Date on which the assessment run was created using the StartReplicationTaskAssessmentRun
operation.
*
*/
private java.util.Date replicationTaskAssessmentRunCreationDate;
/**
*
* Indication of the completion progress for the individual assessments specified to run.
*
*/
private ReplicationTaskAssessmentRunProgress assessmentProgress;
/**
*
* Last message generated by an individual assessment failure.
*
*/
private String lastFailureMessage;
/**
*
* ARN of the service role used to start the assessment run using the StartReplicationTaskAssessmentRun
* operation. The role must allow the iam:PassRole
action.
*
*/
private String serviceAccessRoleArn;
/**
*
* Amazon S3 bucket where DMS stores the results of this assessment run.
*
*/
private String resultLocationBucket;
/**
*
* Folder in an Amazon S3 bucket where DMS stores the results of this assessment run.
*
*/
private String resultLocationFolder;
/**
*
* Encryption mode used to encrypt the assessment run results.
*
*/
private String resultEncryptionMode;
/**
*
* ARN of the KMS encryption key used to encrypt the assessment run results.
*
*/
private String resultKmsKeyArn;
/**
*
* Unique name of the assessment run.
*
*/
private String assessmentRunName;
/**
*
* Amazon Resource Name (ARN) of this assessment run.
*
*
* @param replicationTaskAssessmentRunArn
* Amazon Resource Name (ARN) of this assessment run.
*/
public void setReplicationTaskAssessmentRunArn(String replicationTaskAssessmentRunArn) {
this.replicationTaskAssessmentRunArn = replicationTaskAssessmentRunArn;
}
/**
*
* Amazon Resource Name (ARN) of this assessment run.
*
*
* @return Amazon Resource Name (ARN) of this assessment run.
*/
public String getReplicationTaskAssessmentRunArn() {
return this.replicationTaskAssessmentRunArn;
}
/**
*
* Amazon Resource Name (ARN) of this assessment run.
*
*
* @param replicationTaskAssessmentRunArn
* Amazon Resource Name (ARN) of this assessment run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationTaskAssessmentRun withReplicationTaskAssessmentRunArn(String replicationTaskAssessmentRunArn) {
setReplicationTaskAssessmentRunArn(replicationTaskAssessmentRunArn);
return this;
}
/**
*
* ARN of the migration task associated with this premigration assessment run.
*
*
* @param replicationTaskArn
* ARN of the migration task associated with this premigration assessment run.
*/
public void setReplicationTaskArn(String replicationTaskArn) {
this.replicationTaskArn = replicationTaskArn;
}
/**
*
* ARN of the migration task associated with this premigration assessment run.
*
*
* @return ARN of the migration task associated with this premigration assessment run.
*/
public String getReplicationTaskArn() {
return this.replicationTaskArn;
}
/**
*
* ARN of the migration task associated with this premigration assessment run.
*
*
* @param replicationTaskArn
* ARN of the migration task associated with this premigration assessment run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationTaskAssessmentRun withReplicationTaskArn(String replicationTaskArn) {
setReplicationTaskArn(replicationTaskArn);
return this;
}
/**
*
* Assessment run status.
*
*
* This status can have one of the following values:
*
*
* -
*
* "cancelling"
– The assessment run was canceled by the
* CancelReplicationTaskAssessmentRun
operation.
*
*
* -
*
* "deleting"
– The assessment run was deleted by the DeleteReplicationTaskAssessmentRun
* operation.
*
*
* -
*
* "failed"
– At least one individual assessment completed with a failed
status.
*
*
* -
*
* "error-provisioning"
– An internal error occurred while resources were provisioned (during
* provisioning
status).
*
*
* -
*
* "error-executing"
– An internal error occurred while individual assessments ran (during
* running
status).
*
*
* -
*
* "invalid state"
– The assessment run is in an unknown state.
*
*
* -
*
* "passed"
– All individual assessments have completed, and none has a failed
status.
*
*
* -
*
* "provisioning"
– Resources required to run individual assessments are being provisioned.
*
*
* -
*
* "running"
– Individual assessments are being run.
*
*
* -
*
* "starting"
– The assessment run is starting, but resources are not yet being provisioned for
* individual assessments.
*
*
*
*
* @param status
* Assessment run status.
*
* This status can have one of the following values:
*
*
* -
*
* "cancelling"
– The assessment run was canceled by the
* CancelReplicationTaskAssessmentRun
operation.
*
*
* -
*
* "deleting"
– The assessment run was deleted by the
* DeleteReplicationTaskAssessmentRun
operation.
*
*
* -
*
* "failed"
– At least one individual assessment completed with a failed
status.
*
*
* -
*
* "error-provisioning"
– An internal error occurred while resources were provisioned (during
* provisioning
status).
*
*
* -
*
* "error-executing"
– An internal error occurred while individual assessments ran (during
* running
status).
*
*
* -
*
* "invalid state"
– The assessment run is in an unknown state.
*
*
* -
*
* "passed"
– All individual assessments have completed, and none has a failed
* status.
*
*
* -
*
* "provisioning"
– Resources required to run individual assessments are being provisioned.
*
*
* -
*
* "running"
– Individual assessments are being run.
*
*
* -
*
* "starting"
– The assessment run is starting, but resources are not yet being provisioned for
* individual assessments.
*
*
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* Assessment run status.
*
*
* This status can have one of the following values:
*
*
* -
*
* "cancelling"
– The assessment run was canceled by the
* CancelReplicationTaskAssessmentRun
operation.
*
*
* -
*
* "deleting"
– The assessment run was deleted by the DeleteReplicationTaskAssessmentRun
* operation.
*
*
* -
*
* "failed"
– At least one individual assessment completed with a failed
status.
*
*
* -
*
* "error-provisioning"
– An internal error occurred while resources were provisioned (during
* provisioning
status).
*
*
* -
*
* "error-executing"
– An internal error occurred while individual assessments ran (during
* running
status).
*
*
* -
*
* "invalid state"
– The assessment run is in an unknown state.
*
*
* -
*
* "passed"
– All individual assessments have completed, and none has a failed
status.
*
*
* -
*
* "provisioning"
– Resources required to run individual assessments are being provisioned.
*
*
* -
*
* "running"
– Individual assessments are being run.
*
*
* -
*
* "starting"
– The assessment run is starting, but resources are not yet being provisioned for
* individual assessments.
*
*
*
*
* @return Assessment run status.
*
* This status can have one of the following values:
*
*
* -
*
* "cancelling"
– The assessment run was canceled by the
* CancelReplicationTaskAssessmentRun
operation.
*
*
* -
*
* "deleting"
– The assessment run was deleted by the
* DeleteReplicationTaskAssessmentRun
operation.
*
*
* -
*
* "failed"
– At least one individual assessment completed with a failed
status.
*
*
* -
*
* "error-provisioning"
– An internal error occurred while resources were provisioned (during
* provisioning
status).
*
*
* -
*
* "error-executing"
– An internal error occurred while individual assessments ran (during
* running
status).
*
*
* -
*
* "invalid state"
– The assessment run is in an unknown state.
*
*
* -
*
* "passed"
– All individual assessments have completed, and none has a failed
* status.
*
*
* -
*
* "provisioning"
– Resources required to run individual assessments are being provisioned.
*
*
* -
*
* "running"
– Individual assessments are being run.
*
*
* -
*
* "starting"
– The assessment run is starting, but resources are not yet being provisioned for
* individual assessments.
*
*
*/
public String getStatus() {
return this.status;
}
/**
*
* Assessment run status.
*
*
* This status can have one of the following values:
*
*
* -
*
* "cancelling"
– The assessment run was canceled by the
* CancelReplicationTaskAssessmentRun
operation.
*
*
* -
*
* "deleting"
– The assessment run was deleted by the DeleteReplicationTaskAssessmentRun
* operation.
*
*
* -
*
* "failed"
– At least one individual assessment completed with a failed
status.
*
*
* -
*
* "error-provisioning"
– An internal error occurred while resources were provisioned (during
* provisioning
status).
*
*
* -
*
* "error-executing"
– An internal error occurred while individual assessments ran (during
* running
status).
*
*
* -
*
* "invalid state"
– The assessment run is in an unknown state.
*
*
* -
*
* "passed"
– All individual assessments have completed, and none has a failed
status.
*
*
* -
*
* "provisioning"
– Resources required to run individual assessments are being provisioned.
*
*
* -
*
* "running"
– Individual assessments are being run.
*
*
* -
*
* "starting"
– The assessment run is starting, but resources are not yet being provisioned for
* individual assessments.
*
*
*
*
* @param status
* Assessment run status.
*
* This status can have one of the following values:
*
*
* -
*
* "cancelling"
– The assessment run was canceled by the
* CancelReplicationTaskAssessmentRun
operation.
*
*
* -
*
* "deleting"
– The assessment run was deleted by the
* DeleteReplicationTaskAssessmentRun
operation.
*
*
* -
*
* "failed"
– At least one individual assessment completed with a failed
status.
*
*
* -
*
* "error-provisioning"
– An internal error occurred while resources were provisioned (during
* provisioning
status).
*
*
* -
*
* "error-executing"
– An internal error occurred while individual assessments ran (during
* running
status).
*
*
* -
*
* "invalid state"
– The assessment run is in an unknown state.
*
*
* -
*
* "passed"
– All individual assessments have completed, and none has a failed
* status.
*
*
* -
*
* "provisioning"
– Resources required to run individual assessments are being provisioned.
*
*
* -
*
* "running"
– Individual assessments are being run.
*
*
* -
*
* "starting"
– The assessment run is starting, but resources are not yet being provisioned for
* individual assessments.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationTaskAssessmentRun withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* Date on which the assessment run was created using the StartReplicationTaskAssessmentRun
operation.
*
*
* @param replicationTaskAssessmentRunCreationDate
* Date on which the assessment run was created using the StartReplicationTaskAssessmentRun
* operation.
*/
public void setReplicationTaskAssessmentRunCreationDate(java.util.Date replicationTaskAssessmentRunCreationDate) {
this.replicationTaskAssessmentRunCreationDate = replicationTaskAssessmentRunCreationDate;
}
/**
*
* Date on which the assessment run was created using the StartReplicationTaskAssessmentRun
operation.
*
*
* @return Date on which the assessment run was created using the StartReplicationTaskAssessmentRun
* operation.
*/
public java.util.Date getReplicationTaskAssessmentRunCreationDate() {
return this.replicationTaskAssessmentRunCreationDate;
}
/**
*
* Date on which the assessment run was created using the StartReplicationTaskAssessmentRun
operation.
*
*
* @param replicationTaskAssessmentRunCreationDate
* Date on which the assessment run was created using the StartReplicationTaskAssessmentRun
* operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationTaskAssessmentRun withReplicationTaskAssessmentRunCreationDate(java.util.Date replicationTaskAssessmentRunCreationDate) {
setReplicationTaskAssessmentRunCreationDate(replicationTaskAssessmentRunCreationDate);
return this;
}
/**
*
* Indication of the completion progress for the individual assessments specified to run.
*
*
* @param assessmentProgress
* Indication of the completion progress for the individual assessments specified to run.
*/
public void setAssessmentProgress(ReplicationTaskAssessmentRunProgress assessmentProgress) {
this.assessmentProgress = assessmentProgress;
}
/**
*
* Indication of the completion progress for the individual assessments specified to run.
*
*
* @return Indication of the completion progress for the individual assessments specified to run.
*/
public ReplicationTaskAssessmentRunProgress getAssessmentProgress() {
return this.assessmentProgress;
}
/**
*
* Indication of the completion progress for the individual assessments specified to run.
*
*
* @param assessmentProgress
* Indication of the completion progress for the individual assessments specified to run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationTaskAssessmentRun withAssessmentProgress(ReplicationTaskAssessmentRunProgress assessmentProgress) {
setAssessmentProgress(assessmentProgress);
return this;
}
/**
*
* Last message generated by an individual assessment failure.
*
*
* @param lastFailureMessage
* Last message generated by an individual assessment failure.
*/
public void setLastFailureMessage(String lastFailureMessage) {
this.lastFailureMessage = lastFailureMessage;
}
/**
*
* Last message generated by an individual assessment failure.
*
*
* @return Last message generated by an individual assessment failure.
*/
public String getLastFailureMessage() {
return this.lastFailureMessage;
}
/**
*
* Last message generated by an individual assessment failure.
*
*
* @param lastFailureMessage
* Last message generated by an individual assessment failure.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationTaskAssessmentRun withLastFailureMessage(String lastFailureMessage) {
setLastFailureMessage(lastFailureMessage);
return this;
}
/**
*
* ARN of the service role used to start the assessment run using the StartReplicationTaskAssessmentRun
* operation. The role must allow the iam:PassRole
action.
*
*
* @param serviceAccessRoleArn
* ARN of the service role used to start the assessment run using the
* StartReplicationTaskAssessmentRun
operation. The role must allow the
* iam:PassRole
action.
*/
public void setServiceAccessRoleArn(String serviceAccessRoleArn) {
this.serviceAccessRoleArn = serviceAccessRoleArn;
}
/**
*
* ARN of the service role used to start the assessment run using the StartReplicationTaskAssessmentRun
* operation. The role must allow the iam:PassRole
action.
*
*
* @return ARN of the service role used to start the assessment run using the
* StartReplicationTaskAssessmentRun
operation. The role must allow the
* iam:PassRole
action.
*/
public String getServiceAccessRoleArn() {
return this.serviceAccessRoleArn;
}
/**
*
* ARN of the service role used to start the assessment run using the StartReplicationTaskAssessmentRun
* operation. The role must allow the iam:PassRole
action.
*
*
* @param serviceAccessRoleArn
* ARN of the service role used to start the assessment run using the
* StartReplicationTaskAssessmentRun
operation. The role must allow the
* iam:PassRole
action.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationTaskAssessmentRun withServiceAccessRoleArn(String serviceAccessRoleArn) {
setServiceAccessRoleArn(serviceAccessRoleArn);
return this;
}
/**
*
* Amazon S3 bucket where DMS stores the results of this assessment run.
*
*
* @param resultLocationBucket
* Amazon S3 bucket where DMS stores the results of this assessment run.
*/
public void setResultLocationBucket(String resultLocationBucket) {
this.resultLocationBucket = resultLocationBucket;
}
/**
*
* Amazon S3 bucket where DMS stores the results of this assessment run.
*
*
* @return Amazon S3 bucket where DMS stores the results of this assessment run.
*/
public String getResultLocationBucket() {
return this.resultLocationBucket;
}
/**
*
* Amazon S3 bucket where DMS stores the results of this assessment run.
*
*
* @param resultLocationBucket
* Amazon S3 bucket where DMS stores the results of this assessment run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationTaskAssessmentRun withResultLocationBucket(String resultLocationBucket) {
setResultLocationBucket(resultLocationBucket);
return this;
}
/**
*
* Folder in an Amazon S3 bucket where DMS stores the results of this assessment run.
*
*
* @param resultLocationFolder
* Folder in an Amazon S3 bucket where DMS stores the results of this assessment run.
*/
public void setResultLocationFolder(String resultLocationFolder) {
this.resultLocationFolder = resultLocationFolder;
}
/**
*
* Folder in an Amazon S3 bucket where DMS stores the results of this assessment run.
*
*
* @return Folder in an Amazon S3 bucket where DMS stores the results of this assessment run.
*/
public String getResultLocationFolder() {
return this.resultLocationFolder;
}
/**
*
* Folder in an Amazon S3 bucket where DMS stores the results of this assessment run.
*
*
* @param resultLocationFolder
* Folder in an Amazon S3 bucket where DMS stores the results of this assessment run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationTaskAssessmentRun withResultLocationFolder(String resultLocationFolder) {
setResultLocationFolder(resultLocationFolder);
return this;
}
/**
*
* Encryption mode used to encrypt the assessment run results.
*
*
* @param resultEncryptionMode
* Encryption mode used to encrypt the assessment run results.
*/
public void setResultEncryptionMode(String resultEncryptionMode) {
this.resultEncryptionMode = resultEncryptionMode;
}
/**
*
* Encryption mode used to encrypt the assessment run results.
*
*
* @return Encryption mode used to encrypt the assessment run results.
*/
public String getResultEncryptionMode() {
return this.resultEncryptionMode;
}
/**
*
* Encryption mode used to encrypt the assessment run results.
*
*
* @param resultEncryptionMode
* Encryption mode used to encrypt the assessment run results.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationTaskAssessmentRun withResultEncryptionMode(String resultEncryptionMode) {
setResultEncryptionMode(resultEncryptionMode);
return this;
}
/**
*
* ARN of the KMS encryption key used to encrypt the assessment run results.
*
*
* @param resultKmsKeyArn
* ARN of the KMS encryption key used to encrypt the assessment run results.
*/
public void setResultKmsKeyArn(String resultKmsKeyArn) {
this.resultKmsKeyArn = resultKmsKeyArn;
}
/**
*
* ARN of the KMS encryption key used to encrypt the assessment run results.
*
*
* @return ARN of the KMS encryption key used to encrypt the assessment run results.
*/
public String getResultKmsKeyArn() {
return this.resultKmsKeyArn;
}
/**
*
* ARN of the KMS encryption key used to encrypt the assessment run results.
*
*
* @param resultKmsKeyArn
* ARN of the KMS encryption key used to encrypt the assessment run results.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationTaskAssessmentRun withResultKmsKeyArn(String resultKmsKeyArn) {
setResultKmsKeyArn(resultKmsKeyArn);
return this;
}
/**
*
* Unique name of the assessment run.
*
*
* @param assessmentRunName
* Unique name of the assessment run.
*/
public void setAssessmentRunName(String assessmentRunName) {
this.assessmentRunName = assessmentRunName;
}
/**
*
* Unique name of the assessment run.
*
*
* @return Unique name of the assessment run.
*/
public String getAssessmentRunName() {
return this.assessmentRunName;
}
/**
*
* Unique name of the assessment run.
*
*
* @param assessmentRunName
* Unique name of the assessment run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ReplicationTaskAssessmentRun withAssessmentRunName(String assessmentRunName) {
setAssessmentRunName(assessmentRunName);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getReplicationTaskAssessmentRunArn() != null)
sb.append("ReplicationTaskAssessmentRunArn: ").append(getReplicationTaskAssessmentRunArn()).append(",");
if (getReplicationTaskArn() != null)
sb.append("ReplicationTaskArn: ").append(getReplicationTaskArn()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getReplicationTaskAssessmentRunCreationDate() != null)
sb.append("ReplicationTaskAssessmentRunCreationDate: ").append(getReplicationTaskAssessmentRunCreationDate()).append(",");
if (getAssessmentProgress() != null)
sb.append("AssessmentProgress: ").append(getAssessmentProgress()).append(",");
if (getLastFailureMessage() != null)
sb.append("LastFailureMessage: ").append(getLastFailureMessage()).append(",");
if (getServiceAccessRoleArn() != null)
sb.append("ServiceAccessRoleArn: ").append(getServiceAccessRoleArn()).append(",");
if (getResultLocationBucket() != null)
sb.append("ResultLocationBucket: ").append(getResultLocationBucket()).append(",");
if (getResultLocationFolder() != null)
sb.append("ResultLocationFolder: ").append(getResultLocationFolder()).append(",");
if (getResultEncryptionMode() != null)
sb.append("ResultEncryptionMode: ").append(getResultEncryptionMode()).append(",");
if (getResultKmsKeyArn() != null)
sb.append("ResultKmsKeyArn: ").append(getResultKmsKeyArn()).append(",");
if (getAssessmentRunName() != null)
sb.append("AssessmentRunName: ").append(getAssessmentRunName());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ReplicationTaskAssessmentRun == false)
return false;
ReplicationTaskAssessmentRun other = (ReplicationTaskAssessmentRun) obj;
if (other.getReplicationTaskAssessmentRunArn() == null ^ this.getReplicationTaskAssessmentRunArn() == null)
return false;
if (other.getReplicationTaskAssessmentRunArn() != null
&& other.getReplicationTaskAssessmentRunArn().equals(this.getReplicationTaskAssessmentRunArn()) == false)
return false;
if (other.getReplicationTaskArn() == null ^ this.getReplicationTaskArn() == null)
return false;
if (other.getReplicationTaskArn() != null && other.getReplicationTaskArn().equals(this.getReplicationTaskArn()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getReplicationTaskAssessmentRunCreationDate() == null ^ this.getReplicationTaskAssessmentRunCreationDate() == null)
return false;
if (other.getReplicationTaskAssessmentRunCreationDate() != null
&& other.getReplicationTaskAssessmentRunCreationDate().equals(this.getReplicationTaskAssessmentRunCreationDate()) == false)
return false;
if (other.getAssessmentProgress() == null ^ this.getAssessmentProgress() == null)
return false;
if (other.getAssessmentProgress() != null && other.getAssessmentProgress().equals(this.getAssessmentProgress()) == false)
return false;
if (other.getLastFailureMessage() == null ^ this.getLastFailureMessage() == null)
return false;
if (other.getLastFailureMessage() != null && other.getLastFailureMessage().equals(this.getLastFailureMessage()) == false)
return false;
if (other.getServiceAccessRoleArn() == null ^ this.getServiceAccessRoleArn() == null)
return false;
if (other.getServiceAccessRoleArn() != null && other.getServiceAccessRoleArn().equals(this.getServiceAccessRoleArn()) == false)
return false;
if (other.getResultLocationBucket() == null ^ this.getResultLocationBucket() == null)
return false;
if (other.getResultLocationBucket() != null && other.getResultLocationBucket().equals(this.getResultLocationBucket()) == false)
return false;
if (other.getResultLocationFolder() == null ^ this.getResultLocationFolder() == null)
return false;
if (other.getResultLocationFolder() != null && other.getResultLocationFolder().equals(this.getResultLocationFolder()) == false)
return false;
if (other.getResultEncryptionMode() == null ^ this.getResultEncryptionMode() == null)
return false;
if (other.getResultEncryptionMode() != null && other.getResultEncryptionMode().equals(this.getResultEncryptionMode()) == false)
return false;
if (other.getResultKmsKeyArn() == null ^ this.getResultKmsKeyArn() == null)
return false;
if (other.getResultKmsKeyArn() != null && other.getResultKmsKeyArn().equals(this.getResultKmsKeyArn()) == false)
return false;
if (other.getAssessmentRunName() == null ^ this.getAssessmentRunName() == null)
return false;
if (other.getAssessmentRunName() != null && other.getAssessmentRunName().equals(this.getAssessmentRunName()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getReplicationTaskAssessmentRunArn() == null) ? 0 : getReplicationTaskAssessmentRunArn().hashCode());
hashCode = prime * hashCode + ((getReplicationTaskArn() == null) ? 0 : getReplicationTaskArn().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getReplicationTaskAssessmentRunCreationDate() == null) ? 0 : getReplicationTaskAssessmentRunCreationDate().hashCode());
hashCode = prime * hashCode + ((getAssessmentProgress() == null) ? 0 : getAssessmentProgress().hashCode());
hashCode = prime * hashCode + ((getLastFailureMessage() == null) ? 0 : getLastFailureMessage().hashCode());
hashCode = prime * hashCode + ((getServiceAccessRoleArn() == null) ? 0 : getServiceAccessRoleArn().hashCode());
hashCode = prime * hashCode + ((getResultLocationBucket() == null) ? 0 : getResultLocationBucket().hashCode());
hashCode = prime * hashCode + ((getResultLocationFolder() == null) ? 0 : getResultLocationFolder().hashCode());
hashCode = prime * hashCode + ((getResultEncryptionMode() == null) ? 0 : getResultEncryptionMode().hashCode());
hashCode = prime * hashCode + ((getResultKmsKeyArn() == null) ? 0 : getResultKmsKeyArn().hashCode());
hashCode = prime * hashCode + ((getAssessmentRunName() == null) ? 0 : getAssessmentRunName().hashCode());
return hashCode;
}
@Override
public ReplicationTaskAssessmentRun clone() {
try {
return (ReplicationTaskAssessmentRun) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.databasemigrationservice.model.transform.ReplicationTaskAssessmentRunMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}