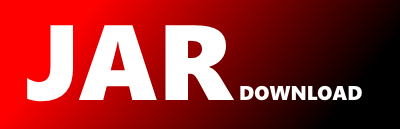
com.amazonaws.services.databasemigrationservice.model.TableStatistics Maven / Gradle / Ivy
Show all versions of aws-java-sdk-dms Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.databasemigrationservice.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Provides a collection of table statistics in response to a request by the DescribeTableStatistics
* operation.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class TableStatistics implements Serializable, Cloneable, StructuredPojo {
/**
*
* The schema name.
*
*/
private String schemaName;
/**
*
* The name of the table.
*
*/
private String tableName;
/**
*
* The number of insert actions performed on a table.
*
*/
private Long inserts;
/**
*
* The number of delete actions performed on a table.
*
*/
private Long deletes;
/**
*
* The number of update actions performed on a table.
*
*/
private Long updates;
/**
*
* The data definition language (DDL) used to build and modify the structure of your tables.
*
*/
private Long ddls;
/**
*
* The number of insert actions applied on a target table.
*
*/
private Long appliedInserts;
/**
*
* The number of delete actions applied on a target table.
*
*/
private Long appliedDeletes;
/**
*
* The number of update actions applied on a target table.
*
*/
private Long appliedUpdates;
/**
*
* The number of data definition language (DDL) statements used to build and modify the structure of your tables
* applied on the target.
*
*/
private Long appliedDdls;
/**
*
* The number of rows added during the full load operation.
*
*/
private Long fullLoadRows;
/**
*
* The number of rows that failed conditional checks during the full load operation (valid only for migrations where
* DynamoDB is the target).
*
*/
private Long fullLoadCondtnlChkFailedRows;
/**
*
* The number of rows that failed to load during the full load operation (valid only for migrations where DynamoDB
* is the target).
*
*/
private Long fullLoadErrorRows;
/**
*
* The time when the full load operation started.
*
*/
private java.util.Date fullLoadStartTime;
/**
*
* The time when the full load operation completed.
*
*/
private java.util.Date fullLoadEndTime;
/**
*
* A value that indicates if the table was reloaded (true
) or loaded as part of a new full load
* operation (false
).
*
*/
private Boolean fullLoadReloaded;
/**
*
* The last time a table was updated.
*
*/
private java.util.Date lastUpdateTime;
/**
*
* The state of the tables described.
*
*
* Valid states: Table does not exist | Before load | Full load | Table completed | Table cancelled | Table error |
* Table is being reloaded
*
*/
private String tableState;
/**
*
* The number of records that have yet to be validated.
*
*/
private Long validationPendingRecords;
/**
*
* The number of records that failed validation.
*
*/
private Long validationFailedRecords;
/**
*
* The number of records that couldn't be validated.
*
*/
private Long validationSuspendedRecords;
/**
*
* The validation state of the table.
*
*
* This parameter can have the following values:
*
*
* -
*
* Not enabled – Validation isn't enabled for the table in the migration task.
*
*
* -
*
* Pending records – Some records in the table are waiting for validation.
*
*
* -
*
* Mismatched records – Some records in the table don't match between the source and target.
*
*
* -
*
* Suspended records – Some records in the table couldn't be validated.
*
*
* -
*
* No primary key –The table couldn't be validated because it has no primary key.
*
*
* -
*
* Table error – The table wasn't validated because it's in an error state and some data wasn't migrated.
*
*
* -
*
* Validated – All rows in the table are validated. If the table is updated, the status can change from Validated.
*
*
* -
*
* Error – The table couldn't be validated because of an unexpected error.
*
*
* -
*
* Pending validation – The table is waiting validation.
*
*
* -
*
* Preparing table – Preparing the table enabled in the migration task for validation.
*
*
* -
*
* Pending revalidation – All rows in the table are pending validation after the table was updated.
*
*
*
*/
private String validationState;
/**
*
* Additional details about the state of validation.
*
*/
private String validationStateDetails;
/**
*
* The schema name.
*
*
* @param schemaName
* The schema name.
*/
public void setSchemaName(String schemaName) {
this.schemaName = schemaName;
}
/**
*
* The schema name.
*
*
* @return The schema name.
*/
public String getSchemaName() {
return this.schemaName;
}
/**
*
* The schema name.
*
*
* @param schemaName
* The schema name.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TableStatistics withSchemaName(String schemaName) {
setSchemaName(schemaName);
return this;
}
/**
*
* The name of the table.
*
*
* @param tableName
* The name of the table.
*/
public void setTableName(String tableName) {
this.tableName = tableName;
}
/**
*
* The name of the table.
*
*
* @return The name of the table.
*/
public String getTableName() {
return this.tableName;
}
/**
*
* The name of the table.
*
*
* @param tableName
* The name of the table.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TableStatistics withTableName(String tableName) {
setTableName(tableName);
return this;
}
/**
*
* The number of insert actions performed on a table.
*
*
* @param inserts
* The number of insert actions performed on a table.
*/
public void setInserts(Long inserts) {
this.inserts = inserts;
}
/**
*
* The number of insert actions performed on a table.
*
*
* @return The number of insert actions performed on a table.
*/
public Long getInserts() {
return this.inserts;
}
/**
*
* The number of insert actions performed on a table.
*
*
* @param inserts
* The number of insert actions performed on a table.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TableStatistics withInserts(Long inserts) {
setInserts(inserts);
return this;
}
/**
*
* The number of delete actions performed on a table.
*
*
* @param deletes
* The number of delete actions performed on a table.
*/
public void setDeletes(Long deletes) {
this.deletes = deletes;
}
/**
*
* The number of delete actions performed on a table.
*
*
* @return The number of delete actions performed on a table.
*/
public Long getDeletes() {
return this.deletes;
}
/**
*
* The number of delete actions performed on a table.
*
*
* @param deletes
* The number of delete actions performed on a table.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TableStatistics withDeletes(Long deletes) {
setDeletes(deletes);
return this;
}
/**
*
* The number of update actions performed on a table.
*
*
* @param updates
* The number of update actions performed on a table.
*/
public void setUpdates(Long updates) {
this.updates = updates;
}
/**
*
* The number of update actions performed on a table.
*
*
* @return The number of update actions performed on a table.
*/
public Long getUpdates() {
return this.updates;
}
/**
*
* The number of update actions performed on a table.
*
*
* @param updates
* The number of update actions performed on a table.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TableStatistics withUpdates(Long updates) {
setUpdates(updates);
return this;
}
/**
*
* The data definition language (DDL) used to build and modify the structure of your tables.
*
*
* @param ddls
* The data definition language (DDL) used to build and modify the structure of your tables.
*/
public void setDdls(Long ddls) {
this.ddls = ddls;
}
/**
*
* The data definition language (DDL) used to build and modify the structure of your tables.
*
*
* @return The data definition language (DDL) used to build and modify the structure of your tables.
*/
public Long getDdls() {
return this.ddls;
}
/**
*
* The data definition language (DDL) used to build and modify the structure of your tables.
*
*
* @param ddls
* The data definition language (DDL) used to build and modify the structure of your tables.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TableStatistics withDdls(Long ddls) {
setDdls(ddls);
return this;
}
/**
*
* The number of insert actions applied on a target table.
*
*
* @param appliedInserts
* The number of insert actions applied on a target table.
*/
public void setAppliedInserts(Long appliedInserts) {
this.appliedInserts = appliedInserts;
}
/**
*
* The number of insert actions applied on a target table.
*
*
* @return The number of insert actions applied on a target table.
*/
public Long getAppliedInserts() {
return this.appliedInserts;
}
/**
*
* The number of insert actions applied on a target table.
*
*
* @param appliedInserts
* The number of insert actions applied on a target table.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TableStatistics withAppliedInserts(Long appliedInserts) {
setAppliedInserts(appliedInserts);
return this;
}
/**
*
* The number of delete actions applied on a target table.
*
*
* @param appliedDeletes
* The number of delete actions applied on a target table.
*/
public void setAppliedDeletes(Long appliedDeletes) {
this.appliedDeletes = appliedDeletes;
}
/**
*
* The number of delete actions applied on a target table.
*
*
* @return The number of delete actions applied on a target table.
*/
public Long getAppliedDeletes() {
return this.appliedDeletes;
}
/**
*
* The number of delete actions applied on a target table.
*
*
* @param appliedDeletes
* The number of delete actions applied on a target table.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TableStatistics withAppliedDeletes(Long appliedDeletes) {
setAppliedDeletes(appliedDeletes);
return this;
}
/**
*
* The number of update actions applied on a target table.
*
*
* @param appliedUpdates
* The number of update actions applied on a target table.
*/
public void setAppliedUpdates(Long appliedUpdates) {
this.appliedUpdates = appliedUpdates;
}
/**
*
* The number of update actions applied on a target table.
*
*
* @return The number of update actions applied on a target table.
*/
public Long getAppliedUpdates() {
return this.appliedUpdates;
}
/**
*
* The number of update actions applied on a target table.
*
*
* @param appliedUpdates
* The number of update actions applied on a target table.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TableStatistics withAppliedUpdates(Long appliedUpdates) {
setAppliedUpdates(appliedUpdates);
return this;
}
/**
*
* The number of data definition language (DDL) statements used to build and modify the structure of your tables
* applied on the target.
*
*
* @param appliedDdls
* The number of data definition language (DDL) statements used to build and modify the structure of your
* tables applied on the target.
*/
public void setAppliedDdls(Long appliedDdls) {
this.appliedDdls = appliedDdls;
}
/**
*
* The number of data definition language (DDL) statements used to build and modify the structure of your tables
* applied on the target.
*
*
* @return The number of data definition language (DDL) statements used to build and modify the structure of your
* tables applied on the target.
*/
public Long getAppliedDdls() {
return this.appliedDdls;
}
/**
*
* The number of data definition language (DDL) statements used to build and modify the structure of your tables
* applied on the target.
*
*
* @param appliedDdls
* The number of data definition language (DDL) statements used to build and modify the structure of your
* tables applied on the target.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TableStatistics withAppliedDdls(Long appliedDdls) {
setAppliedDdls(appliedDdls);
return this;
}
/**
*
* The number of rows added during the full load operation.
*
*
* @param fullLoadRows
* The number of rows added during the full load operation.
*/
public void setFullLoadRows(Long fullLoadRows) {
this.fullLoadRows = fullLoadRows;
}
/**
*
* The number of rows added during the full load operation.
*
*
* @return The number of rows added during the full load operation.
*/
public Long getFullLoadRows() {
return this.fullLoadRows;
}
/**
*
* The number of rows added during the full load operation.
*
*
* @param fullLoadRows
* The number of rows added during the full load operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TableStatistics withFullLoadRows(Long fullLoadRows) {
setFullLoadRows(fullLoadRows);
return this;
}
/**
*
* The number of rows that failed conditional checks during the full load operation (valid only for migrations where
* DynamoDB is the target).
*
*
* @param fullLoadCondtnlChkFailedRows
* The number of rows that failed conditional checks during the full load operation (valid only for
* migrations where DynamoDB is the target).
*/
public void setFullLoadCondtnlChkFailedRows(Long fullLoadCondtnlChkFailedRows) {
this.fullLoadCondtnlChkFailedRows = fullLoadCondtnlChkFailedRows;
}
/**
*
* The number of rows that failed conditional checks during the full load operation (valid only for migrations where
* DynamoDB is the target).
*
*
* @return The number of rows that failed conditional checks during the full load operation (valid only for
* migrations where DynamoDB is the target).
*/
public Long getFullLoadCondtnlChkFailedRows() {
return this.fullLoadCondtnlChkFailedRows;
}
/**
*
* The number of rows that failed conditional checks during the full load operation (valid only for migrations where
* DynamoDB is the target).
*
*
* @param fullLoadCondtnlChkFailedRows
* The number of rows that failed conditional checks during the full load operation (valid only for
* migrations where DynamoDB is the target).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TableStatistics withFullLoadCondtnlChkFailedRows(Long fullLoadCondtnlChkFailedRows) {
setFullLoadCondtnlChkFailedRows(fullLoadCondtnlChkFailedRows);
return this;
}
/**
*
* The number of rows that failed to load during the full load operation (valid only for migrations where DynamoDB
* is the target).
*
*
* @param fullLoadErrorRows
* The number of rows that failed to load during the full load operation (valid only for migrations where
* DynamoDB is the target).
*/
public void setFullLoadErrorRows(Long fullLoadErrorRows) {
this.fullLoadErrorRows = fullLoadErrorRows;
}
/**
*
* The number of rows that failed to load during the full load operation (valid only for migrations where DynamoDB
* is the target).
*
*
* @return The number of rows that failed to load during the full load operation (valid only for migrations where
* DynamoDB is the target).
*/
public Long getFullLoadErrorRows() {
return this.fullLoadErrorRows;
}
/**
*
* The number of rows that failed to load during the full load operation (valid only for migrations where DynamoDB
* is the target).
*
*
* @param fullLoadErrorRows
* The number of rows that failed to load during the full load operation (valid only for migrations where
* DynamoDB is the target).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TableStatistics withFullLoadErrorRows(Long fullLoadErrorRows) {
setFullLoadErrorRows(fullLoadErrorRows);
return this;
}
/**
*
* The time when the full load operation started.
*
*
* @param fullLoadStartTime
* The time when the full load operation started.
*/
public void setFullLoadStartTime(java.util.Date fullLoadStartTime) {
this.fullLoadStartTime = fullLoadStartTime;
}
/**
*
* The time when the full load operation started.
*
*
* @return The time when the full load operation started.
*/
public java.util.Date getFullLoadStartTime() {
return this.fullLoadStartTime;
}
/**
*
* The time when the full load operation started.
*
*
* @param fullLoadStartTime
* The time when the full load operation started.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TableStatistics withFullLoadStartTime(java.util.Date fullLoadStartTime) {
setFullLoadStartTime(fullLoadStartTime);
return this;
}
/**
*
* The time when the full load operation completed.
*
*
* @param fullLoadEndTime
* The time when the full load operation completed.
*/
public void setFullLoadEndTime(java.util.Date fullLoadEndTime) {
this.fullLoadEndTime = fullLoadEndTime;
}
/**
*
* The time when the full load operation completed.
*
*
* @return The time when the full load operation completed.
*/
public java.util.Date getFullLoadEndTime() {
return this.fullLoadEndTime;
}
/**
*
* The time when the full load operation completed.
*
*
* @param fullLoadEndTime
* The time when the full load operation completed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TableStatistics withFullLoadEndTime(java.util.Date fullLoadEndTime) {
setFullLoadEndTime(fullLoadEndTime);
return this;
}
/**
*
* A value that indicates if the table was reloaded (true
) or loaded as part of a new full load
* operation (false
).
*
*
* @param fullLoadReloaded
* A value that indicates if the table was reloaded (true
) or loaded as part of a new full load
* operation (false
).
*/
public void setFullLoadReloaded(Boolean fullLoadReloaded) {
this.fullLoadReloaded = fullLoadReloaded;
}
/**
*
* A value that indicates if the table was reloaded (true
) or loaded as part of a new full load
* operation (false
).
*
*
* @return A value that indicates if the table was reloaded (true
) or loaded as part of a new full load
* operation (false
).
*/
public Boolean getFullLoadReloaded() {
return this.fullLoadReloaded;
}
/**
*
* A value that indicates if the table was reloaded (true
) or loaded as part of a new full load
* operation (false
).
*
*
* @param fullLoadReloaded
* A value that indicates if the table was reloaded (true
) or loaded as part of a new full load
* operation (false
).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TableStatistics withFullLoadReloaded(Boolean fullLoadReloaded) {
setFullLoadReloaded(fullLoadReloaded);
return this;
}
/**
*
* A value that indicates if the table was reloaded (true
) or loaded as part of a new full load
* operation (false
).
*
*
* @return A value that indicates if the table was reloaded (true
) or loaded as part of a new full load
* operation (false
).
*/
public Boolean isFullLoadReloaded() {
return this.fullLoadReloaded;
}
/**
*
* The last time a table was updated.
*
*
* @param lastUpdateTime
* The last time a table was updated.
*/
public void setLastUpdateTime(java.util.Date lastUpdateTime) {
this.lastUpdateTime = lastUpdateTime;
}
/**
*
* The last time a table was updated.
*
*
* @return The last time a table was updated.
*/
public java.util.Date getLastUpdateTime() {
return this.lastUpdateTime;
}
/**
*
* The last time a table was updated.
*
*
* @param lastUpdateTime
* The last time a table was updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TableStatistics withLastUpdateTime(java.util.Date lastUpdateTime) {
setLastUpdateTime(lastUpdateTime);
return this;
}
/**
*
* The state of the tables described.
*
*
* Valid states: Table does not exist | Before load | Full load | Table completed | Table cancelled | Table error |
* Table is being reloaded
*
*
* @param tableState
* The state of the tables described.
*
* Valid states: Table does not exist | Before load | Full load | Table completed | Table cancelled | Table
* error | Table is being reloaded
*/
public void setTableState(String tableState) {
this.tableState = tableState;
}
/**
*
* The state of the tables described.
*
*
* Valid states: Table does not exist | Before load | Full load | Table completed | Table cancelled | Table error |
* Table is being reloaded
*
*
* @return The state of the tables described.
*
* Valid states: Table does not exist | Before load | Full load | Table completed | Table cancelled | Table
* error | Table is being reloaded
*/
public String getTableState() {
return this.tableState;
}
/**
*
* The state of the tables described.
*
*
* Valid states: Table does not exist | Before load | Full load | Table completed | Table cancelled | Table error |
* Table is being reloaded
*
*
* @param tableState
* The state of the tables described.
*
* Valid states: Table does not exist | Before load | Full load | Table completed | Table cancelled | Table
* error | Table is being reloaded
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TableStatistics withTableState(String tableState) {
setTableState(tableState);
return this;
}
/**
*
* The number of records that have yet to be validated.
*
*
* @param validationPendingRecords
* The number of records that have yet to be validated.
*/
public void setValidationPendingRecords(Long validationPendingRecords) {
this.validationPendingRecords = validationPendingRecords;
}
/**
*
* The number of records that have yet to be validated.
*
*
* @return The number of records that have yet to be validated.
*/
public Long getValidationPendingRecords() {
return this.validationPendingRecords;
}
/**
*
* The number of records that have yet to be validated.
*
*
* @param validationPendingRecords
* The number of records that have yet to be validated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TableStatistics withValidationPendingRecords(Long validationPendingRecords) {
setValidationPendingRecords(validationPendingRecords);
return this;
}
/**
*
* The number of records that failed validation.
*
*
* @param validationFailedRecords
* The number of records that failed validation.
*/
public void setValidationFailedRecords(Long validationFailedRecords) {
this.validationFailedRecords = validationFailedRecords;
}
/**
*
* The number of records that failed validation.
*
*
* @return The number of records that failed validation.
*/
public Long getValidationFailedRecords() {
return this.validationFailedRecords;
}
/**
*
* The number of records that failed validation.
*
*
* @param validationFailedRecords
* The number of records that failed validation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TableStatistics withValidationFailedRecords(Long validationFailedRecords) {
setValidationFailedRecords(validationFailedRecords);
return this;
}
/**
*
* The number of records that couldn't be validated.
*
*
* @param validationSuspendedRecords
* The number of records that couldn't be validated.
*/
public void setValidationSuspendedRecords(Long validationSuspendedRecords) {
this.validationSuspendedRecords = validationSuspendedRecords;
}
/**
*
* The number of records that couldn't be validated.
*
*
* @return The number of records that couldn't be validated.
*/
public Long getValidationSuspendedRecords() {
return this.validationSuspendedRecords;
}
/**
*
* The number of records that couldn't be validated.
*
*
* @param validationSuspendedRecords
* The number of records that couldn't be validated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TableStatistics withValidationSuspendedRecords(Long validationSuspendedRecords) {
setValidationSuspendedRecords(validationSuspendedRecords);
return this;
}
/**
*
* The validation state of the table.
*
*
* This parameter can have the following values:
*
*
* -
*
* Not enabled – Validation isn't enabled for the table in the migration task.
*
*
* -
*
* Pending records – Some records in the table are waiting for validation.
*
*
* -
*
* Mismatched records – Some records in the table don't match between the source and target.
*
*
* -
*
* Suspended records – Some records in the table couldn't be validated.
*
*
* -
*
* No primary key –The table couldn't be validated because it has no primary key.
*
*
* -
*
* Table error – The table wasn't validated because it's in an error state and some data wasn't migrated.
*
*
* -
*
* Validated – All rows in the table are validated. If the table is updated, the status can change from Validated.
*
*
* -
*
* Error – The table couldn't be validated because of an unexpected error.
*
*
* -
*
* Pending validation – The table is waiting validation.
*
*
* -
*
* Preparing table – Preparing the table enabled in the migration task for validation.
*
*
* -
*
* Pending revalidation – All rows in the table are pending validation after the table was updated.
*
*
*
*
* @param validationState
* The validation state of the table.
*
* This parameter can have the following values:
*
*
* -
*
* Not enabled – Validation isn't enabled for the table in the migration task.
*
*
* -
*
* Pending records – Some records in the table are waiting for validation.
*
*
* -
*
* Mismatched records – Some records in the table don't match between the source and target.
*
*
* -
*
* Suspended records – Some records in the table couldn't be validated.
*
*
* -
*
* No primary key –The table couldn't be validated because it has no primary key.
*
*
* -
*
* Table error – The table wasn't validated because it's in an error state and some data wasn't migrated.
*
*
* -
*
* Validated – All rows in the table are validated. If the table is updated, the status can change from
* Validated.
*
*
* -
*
* Error – The table couldn't be validated because of an unexpected error.
*
*
* -
*
* Pending validation – The table is waiting validation.
*
*
* -
*
* Preparing table – Preparing the table enabled in the migration task for validation.
*
*
* -
*
* Pending revalidation – All rows in the table are pending validation after the table was updated.
*
*
*/
public void setValidationState(String validationState) {
this.validationState = validationState;
}
/**
*
* The validation state of the table.
*
*
* This parameter can have the following values:
*
*
* -
*
* Not enabled – Validation isn't enabled for the table in the migration task.
*
*
* -
*
* Pending records – Some records in the table are waiting for validation.
*
*
* -
*
* Mismatched records – Some records in the table don't match between the source and target.
*
*
* -
*
* Suspended records – Some records in the table couldn't be validated.
*
*
* -
*
* No primary key –The table couldn't be validated because it has no primary key.
*
*
* -
*
* Table error – The table wasn't validated because it's in an error state and some data wasn't migrated.
*
*
* -
*
* Validated – All rows in the table are validated. If the table is updated, the status can change from Validated.
*
*
* -
*
* Error – The table couldn't be validated because of an unexpected error.
*
*
* -
*
* Pending validation – The table is waiting validation.
*
*
* -
*
* Preparing table – Preparing the table enabled in the migration task for validation.
*
*
* -
*
* Pending revalidation – All rows in the table are pending validation after the table was updated.
*
*
*
*
* @return The validation state of the table.
*
* This parameter can have the following values:
*
*
* -
*
* Not enabled – Validation isn't enabled for the table in the migration task.
*
*
* -
*
* Pending records – Some records in the table are waiting for validation.
*
*
* -
*
* Mismatched records – Some records in the table don't match between the source and target.
*
*
* -
*
* Suspended records – Some records in the table couldn't be validated.
*
*
* -
*
* No primary key –The table couldn't be validated because it has no primary key.
*
*
* -
*
* Table error – The table wasn't validated because it's in an error state and some data wasn't migrated.
*
*
* -
*
* Validated – All rows in the table are validated. If the table is updated, the status can change from
* Validated.
*
*
* -
*
* Error – The table couldn't be validated because of an unexpected error.
*
*
* -
*
* Pending validation – The table is waiting validation.
*
*
* -
*
* Preparing table – Preparing the table enabled in the migration task for validation.
*
*
* -
*
* Pending revalidation – All rows in the table are pending validation after the table was updated.
*
*
*/
public String getValidationState() {
return this.validationState;
}
/**
*
* The validation state of the table.
*
*
* This parameter can have the following values:
*
*
* -
*
* Not enabled – Validation isn't enabled for the table in the migration task.
*
*
* -
*
* Pending records – Some records in the table are waiting for validation.
*
*
* -
*
* Mismatched records – Some records in the table don't match between the source and target.
*
*
* -
*
* Suspended records – Some records in the table couldn't be validated.
*
*
* -
*
* No primary key –The table couldn't be validated because it has no primary key.
*
*
* -
*
* Table error – The table wasn't validated because it's in an error state and some data wasn't migrated.
*
*
* -
*
* Validated – All rows in the table are validated. If the table is updated, the status can change from Validated.
*
*
* -
*
* Error – The table couldn't be validated because of an unexpected error.
*
*
* -
*
* Pending validation – The table is waiting validation.
*
*
* -
*
* Preparing table – Preparing the table enabled in the migration task for validation.
*
*
* -
*
* Pending revalidation – All rows in the table are pending validation after the table was updated.
*
*
*
*
* @param validationState
* The validation state of the table.
*
* This parameter can have the following values:
*
*
* -
*
* Not enabled – Validation isn't enabled for the table in the migration task.
*
*
* -
*
* Pending records – Some records in the table are waiting for validation.
*
*
* -
*
* Mismatched records – Some records in the table don't match between the source and target.
*
*
* -
*
* Suspended records – Some records in the table couldn't be validated.
*
*
* -
*
* No primary key –The table couldn't be validated because it has no primary key.
*
*
* -
*
* Table error – The table wasn't validated because it's in an error state and some data wasn't migrated.
*
*
* -
*
* Validated – All rows in the table are validated. If the table is updated, the status can change from
* Validated.
*
*
* -
*
* Error – The table couldn't be validated because of an unexpected error.
*
*
* -
*
* Pending validation – The table is waiting validation.
*
*
* -
*
* Preparing table – Preparing the table enabled in the migration task for validation.
*
*
* -
*
* Pending revalidation – All rows in the table are pending validation after the table was updated.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TableStatistics withValidationState(String validationState) {
setValidationState(validationState);
return this;
}
/**
*
* Additional details about the state of validation.
*
*
* @param validationStateDetails
* Additional details about the state of validation.
*/
public void setValidationStateDetails(String validationStateDetails) {
this.validationStateDetails = validationStateDetails;
}
/**
*
* Additional details about the state of validation.
*
*
* @return Additional details about the state of validation.
*/
public String getValidationStateDetails() {
return this.validationStateDetails;
}
/**
*
* Additional details about the state of validation.
*
*
* @param validationStateDetails
* Additional details about the state of validation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TableStatistics withValidationStateDetails(String validationStateDetails) {
setValidationStateDetails(validationStateDetails);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getSchemaName() != null)
sb.append("SchemaName: ").append(getSchemaName()).append(",");
if (getTableName() != null)
sb.append("TableName: ").append(getTableName()).append(",");
if (getInserts() != null)
sb.append("Inserts: ").append(getInserts()).append(",");
if (getDeletes() != null)
sb.append("Deletes: ").append(getDeletes()).append(",");
if (getUpdates() != null)
sb.append("Updates: ").append(getUpdates()).append(",");
if (getDdls() != null)
sb.append("Ddls: ").append(getDdls()).append(",");
if (getAppliedInserts() != null)
sb.append("AppliedInserts: ").append(getAppliedInserts()).append(",");
if (getAppliedDeletes() != null)
sb.append("AppliedDeletes: ").append(getAppliedDeletes()).append(",");
if (getAppliedUpdates() != null)
sb.append("AppliedUpdates: ").append(getAppliedUpdates()).append(",");
if (getAppliedDdls() != null)
sb.append("AppliedDdls: ").append(getAppliedDdls()).append(",");
if (getFullLoadRows() != null)
sb.append("FullLoadRows: ").append(getFullLoadRows()).append(",");
if (getFullLoadCondtnlChkFailedRows() != null)
sb.append("FullLoadCondtnlChkFailedRows: ").append(getFullLoadCondtnlChkFailedRows()).append(",");
if (getFullLoadErrorRows() != null)
sb.append("FullLoadErrorRows: ").append(getFullLoadErrorRows()).append(",");
if (getFullLoadStartTime() != null)
sb.append("FullLoadStartTime: ").append(getFullLoadStartTime()).append(",");
if (getFullLoadEndTime() != null)
sb.append("FullLoadEndTime: ").append(getFullLoadEndTime()).append(",");
if (getFullLoadReloaded() != null)
sb.append("FullLoadReloaded: ").append(getFullLoadReloaded()).append(",");
if (getLastUpdateTime() != null)
sb.append("LastUpdateTime: ").append(getLastUpdateTime()).append(",");
if (getTableState() != null)
sb.append("TableState: ").append(getTableState()).append(",");
if (getValidationPendingRecords() != null)
sb.append("ValidationPendingRecords: ").append(getValidationPendingRecords()).append(",");
if (getValidationFailedRecords() != null)
sb.append("ValidationFailedRecords: ").append(getValidationFailedRecords()).append(",");
if (getValidationSuspendedRecords() != null)
sb.append("ValidationSuspendedRecords: ").append(getValidationSuspendedRecords()).append(",");
if (getValidationState() != null)
sb.append("ValidationState: ").append(getValidationState()).append(",");
if (getValidationStateDetails() != null)
sb.append("ValidationStateDetails: ").append(getValidationStateDetails());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof TableStatistics == false)
return false;
TableStatistics other = (TableStatistics) obj;
if (other.getSchemaName() == null ^ this.getSchemaName() == null)
return false;
if (other.getSchemaName() != null && other.getSchemaName().equals(this.getSchemaName()) == false)
return false;
if (other.getTableName() == null ^ this.getTableName() == null)
return false;
if (other.getTableName() != null && other.getTableName().equals(this.getTableName()) == false)
return false;
if (other.getInserts() == null ^ this.getInserts() == null)
return false;
if (other.getInserts() != null && other.getInserts().equals(this.getInserts()) == false)
return false;
if (other.getDeletes() == null ^ this.getDeletes() == null)
return false;
if (other.getDeletes() != null && other.getDeletes().equals(this.getDeletes()) == false)
return false;
if (other.getUpdates() == null ^ this.getUpdates() == null)
return false;
if (other.getUpdates() != null && other.getUpdates().equals(this.getUpdates()) == false)
return false;
if (other.getDdls() == null ^ this.getDdls() == null)
return false;
if (other.getDdls() != null && other.getDdls().equals(this.getDdls()) == false)
return false;
if (other.getAppliedInserts() == null ^ this.getAppliedInserts() == null)
return false;
if (other.getAppliedInserts() != null && other.getAppliedInserts().equals(this.getAppliedInserts()) == false)
return false;
if (other.getAppliedDeletes() == null ^ this.getAppliedDeletes() == null)
return false;
if (other.getAppliedDeletes() != null && other.getAppliedDeletes().equals(this.getAppliedDeletes()) == false)
return false;
if (other.getAppliedUpdates() == null ^ this.getAppliedUpdates() == null)
return false;
if (other.getAppliedUpdates() != null && other.getAppliedUpdates().equals(this.getAppliedUpdates()) == false)
return false;
if (other.getAppliedDdls() == null ^ this.getAppliedDdls() == null)
return false;
if (other.getAppliedDdls() != null && other.getAppliedDdls().equals(this.getAppliedDdls()) == false)
return false;
if (other.getFullLoadRows() == null ^ this.getFullLoadRows() == null)
return false;
if (other.getFullLoadRows() != null && other.getFullLoadRows().equals(this.getFullLoadRows()) == false)
return false;
if (other.getFullLoadCondtnlChkFailedRows() == null ^ this.getFullLoadCondtnlChkFailedRows() == null)
return false;
if (other.getFullLoadCondtnlChkFailedRows() != null && other.getFullLoadCondtnlChkFailedRows().equals(this.getFullLoadCondtnlChkFailedRows()) == false)
return false;
if (other.getFullLoadErrorRows() == null ^ this.getFullLoadErrorRows() == null)
return false;
if (other.getFullLoadErrorRows() != null && other.getFullLoadErrorRows().equals(this.getFullLoadErrorRows()) == false)
return false;
if (other.getFullLoadStartTime() == null ^ this.getFullLoadStartTime() == null)
return false;
if (other.getFullLoadStartTime() != null && other.getFullLoadStartTime().equals(this.getFullLoadStartTime()) == false)
return false;
if (other.getFullLoadEndTime() == null ^ this.getFullLoadEndTime() == null)
return false;
if (other.getFullLoadEndTime() != null && other.getFullLoadEndTime().equals(this.getFullLoadEndTime()) == false)
return false;
if (other.getFullLoadReloaded() == null ^ this.getFullLoadReloaded() == null)
return false;
if (other.getFullLoadReloaded() != null && other.getFullLoadReloaded().equals(this.getFullLoadReloaded()) == false)
return false;
if (other.getLastUpdateTime() == null ^ this.getLastUpdateTime() == null)
return false;
if (other.getLastUpdateTime() != null && other.getLastUpdateTime().equals(this.getLastUpdateTime()) == false)
return false;
if (other.getTableState() == null ^ this.getTableState() == null)
return false;
if (other.getTableState() != null && other.getTableState().equals(this.getTableState()) == false)
return false;
if (other.getValidationPendingRecords() == null ^ this.getValidationPendingRecords() == null)
return false;
if (other.getValidationPendingRecords() != null && other.getValidationPendingRecords().equals(this.getValidationPendingRecords()) == false)
return false;
if (other.getValidationFailedRecords() == null ^ this.getValidationFailedRecords() == null)
return false;
if (other.getValidationFailedRecords() != null && other.getValidationFailedRecords().equals(this.getValidationFailedRecords()) == false)
return false;
if (other.getValidationSuspendedRecords() == null ^ this.getValidationSuspendedRecords() == null)
return false;
if (other.getValidationSuspendedRecords() != null && other.getValidationSuspendedRecords().equals(this.getValidationSuspendedRecords()) == false)
return false;
if (other.getValidationState() == null ^ this.getValidationState() == null)
return false;
if (other.getValidationState() != null && other.getValidationState().equals(this.getValidationState()) == false)
return false;
if (other.getValidationStateDetails() == null ^ this.getValidationStateDetails() == null)
return false;
if (other.getValidationStateDetails() != null && other.getValidationStateDetails().equals(this.getValidationStateDetails()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getSchemaName() == null) ? 0 : getSchemaName().hashCode());
hashCode = prime * hashCode + ((getTableName() == null) ? 0 : getTableName().hashCode());
hashCode = prime * hashCode + ((getInserts() == null) ? 0 : getInserts().hashCode());
hashCode = prime * hashCode + ((getDeletes() == null) ? 0 : getDeletes().hashCode());
hashCode = prime * hashCode + ((getUpdates() == null) ? 0 : getUpdates().hashCode());
hashCode = prime * hashCode + ((getDdls() == null) ? 0 : getDdls().hashCode());
hashCode = prime * hashCode + ((getAppliedInserts() == null) ? 0 : getAppliedInserts().hashCode());
hashCode = prime * hashCode + ((getAppliedDeletes() == null) ? 0 : getAppliedDeletes().hashCode());
hashCode = prime * hashCode + ((getAppliedUpdates() == null) ? 0 : getAppliedUpdates().hashCode());
hashCode = prime * hashCode + ((getAppliedDdls() == null) ? 0 : getAppliedDdls().hashCode());
hashCode = prime * hashCode + ((getFullLoadRows() == null) ? 0 : getFullLoadRows().hashCode());
hashCode = prime * hashCode + ((getFullLoadCondtnlChkFailedRows() == null) ? 0 : getFullLoadCondtnlChkFailedRows().hashCode());
hashCode = prime * hashCode + ((getFullLoadErrorRows() == null) ? 0 : getFullLoadErrorRows().hashCode());
hashCode = prime * hashCode + ((getFullLoadStartTime() == null) ? 0 : getFullLoadStartTime().hashCode());
hashCode = prime * hashCode + ((getFullLoadEndTime() == null) ? 0 : getFullLoadEndTime().hashCode());
hashCode = prime * hashCode + ((getFullLoadReloaded() == null) ? 0 : getFullLoadReloaded().hashCode());
hashCode = prime * hashCode + ((getLastUpdateTime() == null) ? 0 : getLastUpdateTime().hashCode());
hashCode = prime * hashCode + ((getTableState() == null) ? 0 : getTableState().hashCode());
hashCode = prime * hashCode + ((getValidationPendingRecords() == null) ? 0 : getValidationPendingRecords().hashCode());
hashCode = prime * hashCode + ((getValidationFailedRecords() == null) ? 0 : getValidationFailedRecords().hashCode());
hashCode = prime * hashCode + ((getValidationSuspendedRecords() == null) ? 0 : getValidationSuspendedRecords().hashCode());
hashCode = prime * hashCode + ((getValidationState() == null) ? 0 : getValidationState().hashCode());
hashCode = prime * hashCode + ((getValidationStateDetails() == null) ? 0 : getValidationStateDetails().hashCode());
return hashCode;
}
@Override
public TableStatistics clone() {
try {
return (TableStatistics) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.databasemigrationservice.model.transform.TableStatisticsMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}