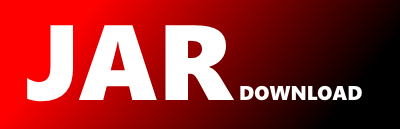
com.amazonaws.services.databasemigrationservice.model.transform.ModifyEndpointRequestMarshaller Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of aws-java-sdk-dms Show documentation
Show all versions of aws-java-sdk-dms Show documentation
The AWS Java SDK for AWS Database Migration Service module holds the client classes that are used for communicating with AWS Database Migration Service.
The newest version!
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.databasemigrationservice.model.transform;
import javax.annotation.Generated;
import com.amazonaws.SdkClientException;
import com.amazonaws.services.databasemigrationservice.model.*;
import com.amazonaws.protocol.*;
import com.amazonaws.annotation.SdkInternalApi;
/**
* ModifyEndpointRequestMarshaller
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
@SdkInternalApi
public class ModifyEndpointRequestMarshaller {
private static final MarshallingInfo ENDPOINTARN_BINDING = MarshallingInfo.builder(MarshallingType.STRING)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("EndpointArn").build();
private static final MarshallingInfo ENDPOINTIDENTIFIER_BINDING = MarshallingInfo.builder(MarshallingType.STRING)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("EndpointIdentifier").build();
private static final MarshallingInfo ENDPOINTTYPE_BINDING = MarshallingInfo.builder(MarshallingType.STRING)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("EndpointType").build();
private static final MarshallingInfo ENGINENAME_BINDING = MarshallingInfo.builder(MarshallingType.STRING)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("EngineName").build();
private static final MarshallingInfo USERNAME_BINDING = MarshallingInfo.builder(MarshallingType.STRING).marshallLocation(MarshallLocation.PAYLOAD)
.marshallLocationName("Username").build();
private static final MarshallingInfo PASSWORD_BINDING = MarshallingInfo.builder(MarshallingType.STRING).marshallLocation(MarshallLocation.PAYLOAD)
.marshallLocationName("Password").build();
private static final MarshallingInfo SERVERNAME_BINDING = MarshallingInfo.builder(MarshallingType.STRING)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("ServerName").build();
private static final MarshallingInfo PORT_BINDING = MarshallingInfo.builder(MarshallingType.INTEGER).marshallLocation(MarshallLocation.PAYLOAD)
.marshallLocationName("Port").build();
private static final MarshallingInfo DATABASENAME_BINDING = MarshallingInfo.builder(MarshallingType.STRING)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("DatabaseName").build();
private static final MarshallingInfo EXTRACONNECTIONATTRIBUTES_BINDING = MarshallingInfo.builder(MarshallingType.STRING)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("ExtraConnectionAttributes").build();
private static final MarshallingInfo CERTIFICATEARN_BINDING = MarshallingInfo.builder(MarshallingType.STRING)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("CertificateArn").build();
private static final MarshallingInfo SSLMODE_BINDING = MarshallingInfo.builder(MarshallingType.STRING).marshallLocation(MarshallLocation.PAYLOAD)
.marshallLocationName("SslMode").build();
private static final MarshallingInfo SERVICEACCESSROLEARN_BINDING = MarshallingInfo.builder(MarshallingType.STRING)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("ServiceAccessRoleArn").build();
private static final MarshallingInfo EXTERNALTABLEDEFINITION_BINDING = MarshallingInfo.builder(MarshallingType.STRING)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("ExternalTableDefinition").build();
private static final MarshallingInfo DYNAMODBSETTINGS_BINDING = MarshallingInfo.builder(MarshallingType.STRUCTURED)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("DynamoDbSettings").build();
private static final MarshallingInfo S3SETTINGS_BINDING = MarshallingInfo.builder(MarshallingType.STRUCTURED)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("S3Settings").build();
private static final MarshallingInfo DMSTRANSFERSETTINGS_BINDING = MarshallingInfo.builder(MarshallingType.STRUCTURED)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("DmsTransferSettings").build();
private static final MarshallingInfo MONGODBSETTINGS_BINDING = MarshallingInfo.builder(MarshallingType.STRUCTURED)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("MongoDbSettings").build();
private static final MarshallingInfo KINESISSETTINGS_BINDING = MarshallingInfo.builder(MarshallingType.STRUCTURED)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("KinesisSettings").build();
private static final MarshallingInfo KAFKASETTINGS_BINDING = MarshallingInfo.builder(MarshallingType.STRUCTURED)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("KafkaSettings").build();
private static final MarshallingInfo ELASTICSEARCHSETTINGS_BINDING = MarshallingInfo.builder(MarshallingType.STRUCTURED)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("ElasticsearchSettings").build();
private static final MarshallingInfo NEPTUNESETTINGS_BINDING = MarshallingInfo.builder(MarshallingType.STRUCTURED)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("NeptuneSettings").build();
private static final MarshallingInfo REDSHIFTSETTINGS_BINDING = MarshallingInfo.builder(MarshallingType.STRUCTURED)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("RedshiftSettings").build();
private static final MarshallingInfo POSTGRESQLSETTINGS_BINDING = MarshallingInfo.builder(MarshallingType.STRUCTURED)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("PostgreSQLSettings").build();
private static final MarshallingInfo MYSQLSETTINGS_BINDING = MarshallingInfo.builder(MarshallingType.STRUCTURED)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("MySQLSettings").build();
private static final MarshallingInfo ORACLESETTINGS_BINDING = MarshallingInfo.builder(MarshallingType.STRUCTURED)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("OracleSettings").build();
private static final MarshallingInfo SYBASESETTINGS_BINDING = MarshallingInfo.builder(MarshallingType.STRUCTURED)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("SybaseSettings").build();
private static final MarshallingInfo MICROSOFTSQLSERVERSETTINGS_BINDING = MarshallingInfo.builder(MarshallingType.STRUCTURED)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("MicrosoftSQLServerSettings").build();
private static final MarshallingInfo IBMDB2SETTINGS_BINDING = MarshallingInfo.builder(MarshallingType.STRUCTURED)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("IBMDb2Settings").build();
private static final MarshallingInfo DOCDBSETTINGS_BINDING = MarshallingInfo.builder(MarshallingType.STRUCTURED)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("DocDbSettings").build();
private static final MarshallingInfo REDISSETTINGS_BINDING = MarshallingInfo.builder(MarshallingType.STRUCTURED)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("RedisSettings").build();
private static final MarshallingInfo EXACTSETTINGS_BINDING = MarshallingInfo.builder(MarshallingType.BOOLEAN)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("ExactSettings").build();
private static final MarshallingInfo GCPMYSQLSETTINGS_BINDING = MarshallingInfo.builder(MarshallingType.STRUCTURED)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("GcpMySQLSettings").build();
private static final MarshallingInfo TIMESTREAMSETTINGS_BINDING = MarshallingInfo.builder(MarshallingType.STRUCTURED)
.marshallLocation(MarshallLocation.PAYLOAD).marshallLocationName("TimestreamSettings").build();
private static final ModifyEndpointRequestMarshaller instance = new ModifyEndpointRequestMarshaller();
public static ModifyEndpointRequestMarshaller getInstance() {
return instance;
}
/**
* Marshall the given parameter object.
*/
public void marshall(ModifyEndpointRequest modifyEndpointRequest, ProtocolMarshaller protocolMarshaller) {
if (modifyEndpointRequest == null) {
throw new SdkClientException("Invalid argument passed to marshall(...)");
}
try {
protocolMarshaller.marshall(modifyEndpointRequest.getEndpointArn(), ENDPOINTARN_BINDING);
protocolMarshaller.marshall(modifyEndpointRequest.getEndpointIdentifier(), ENDPOINTIDENTIFIER_BINDING);
protocolMarshaller.marshall(modifyEndpointRequest.getEndpointType(), ENDPOINTTYPE_BINDING);
protocolMarshaller.marshall(modifyEndpointRequest.getEngineName(), ENGINENAME_BINDING);
protocolMarshaller.marshall(modifyEndpointRequest.getUsername(), USERNAME_BINDING);
protocolMarshaller.marshall(modifyEndpointRequest.getPassword(), PASSWORD_BINDING);
protocolMarshaller.marshall(modifyEndpointRequest.getServerName(), SERVERNAME_BINDING);
protocolMarshaller.marshall(modifyEndpointRequest.getPort(), PORT_BINDING);
protocolMarshaller.marshall(modifyEndpointRequest.getDatabaseName(), DATABASENAME_BINDING);
protocolMarshaller.marshall(modifyEndpointRequest.getExtraConnectionAttributes(), EXTRACONNECTIONATTRIBUTES_BINDING);
protocolMarshaller.marshall(modifyEndpointRequest.getCertificateArn(), CERTIFICATEARN_BINDING);
protocolMarshaller.marshall(modifyEndpointRequest.getSslMode(), SSLMODE_BINDING);
protocolMarshaller.marshall(modifyEndpointRequest.getServiceAccessRoleArn(), SERVICEACCESSROLEARN_BINDING);
protocolMarshaller.marshall(modifyEndpointRequest.getExternalTableDefinition(), EXTERNALTABLEDEFINITION_BINDING);
protocolMarshaller.marshall(modifyEndpointRequest.getDynamoDbSettings(), DYNAMODBSETTINGS_BINDING);
protocolMarshaller.marshall(modifyEndpointRequest.getS3Settings(), S3SETTINGS_BINDING);
protocolMarshaller.marshall(modifyEndpointRequest.getDmsTransferSettings(), DMSTRANSFERSETTINGS_BINDING);
protocolMarshaller.marshall(modifyEndpointRequest.getMongoDbSettings(), MONGODBSETTINGS_BINDING);
protocolMarshaller.marshall(modifyEndpointRequest.getKinesisSettings(), KINESISSETTINGS_BINDING);
protocolMarshaller.marshall(modifyEndpointRequest.getKafkaSettings(), KAFKASETTINGS_BINDING);
protocolMarshaller.marshall(modifyEndpointRequest.getElasticsearchSettings(), ELASTICSEARCHSETTINGS_BINDING);
protocolMarshaller.marshall(modifyEndpointRequest.getNeptuneSettings(), NEPTUNESETTINGS_BINDING);
protocolMarshaller.marshall(modifyEndpointRequest.getRedshiftSettings(), REDSHIFTSETTINGS_BINDING);
protocolMarshaller.marshall(modifyEndpointRequest.getPostgreSQLSettings(), POSTGRESQLSETTINGS_BINDING);
protocolMarshaller.marshall(modifyEndpointRequest.getMySQLSettings(), MYSQLSETTINGS_BINDING);
protocolMarshaller.marshall(modifyEndpointRequest.getOracleSettings(), ORACLESETTINGS_BINDING);
protocolMarshaller.marshall(modifyEndpointRequest.getSybaseSettings(), SYBASESETTINGS_BINDING);
protocolMarshaller.marshall(modifyEndpointRequest.getMicrosoftSQLServerSettings(), MICROSOFTSQLSERVERSETTINGS_BINDING);
protocolMarshaller.marshall(modifyEndpointRequest.getIBMDb2Settings(), IBMDB2SETTINGS_BINDING);
protocolMarshaller.marshall(modifyEndpointRequest.getDocDbSettings(), DOCDBSETTINGS_BINDING);
protocolMarshaller.marshall(modifyEndpointRequest.getRedisSettings(), REDISSETTINGS_BINDING);
protocolMarshaller.marshall(modifyEndpointRequest.getExactSettings(), EXACTSETTINGS_BINDING);
protocolMarshaller.marshall(modifyEndpointRequest.getGcpMySQLSettings(), GCPMYSQLSETTINGS_BINDING);
protocolMarshaller.marshall(modifyEndpointRequest.getTimestreamSettings(), TIMESTREAMSETTINGS_BINDING);
} catch (Exception e) {
throw new SdkClientException("Unable to marshall request to JSON: " + e.getMessage(), e);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy