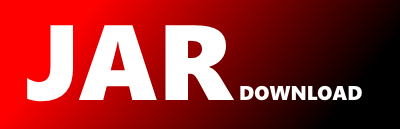
com.amazonaws.services.docdbelastic.model.CopyClusterSnapshotRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-docdbelastic Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.docdbelastic.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CopyClusterSnapshotRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* Set to true
to copy all tags from the source cluster snapshot to the target elastic cluster
* snapshot. The default is false
.
*
*/
private Boolean copyTags;
/**
*
* The Amazon Web Services KMS key ID for an encrypted elastic cluster snapshot. The Amazon Web Services KMS key ID
* is the Amazon Resource Name (ARN), Amazon Web Services KMS key identifier, or the Amazon Web Services KMS key
* alias for the Amazon Web Services KMS encryption key.
*
*
* If you copy an encrypted elastic cluster snapshot from your Amazon Web Services account, you can specify a value
* for KmsKeyId
to encrypt the copy with a new Amazon Web ServicesS KMS encryption key. If you don't
* specify a value for KmsKeyId
, then the copy of the elastic cluster snapshot is encrypted with the
* same AWS
KMS key as the source elastic cluster snapshot.
*
*
* To copy an encrypted elastic cluster snapshot to another Amazon Web Services region, set KmsKeyId
to
* the Amazon Web Services KMS key ID that you want to use to encrypt the copy of the elastic cluster snapshot in
* the destination region. Amazon Web Services KMS encryption keys are specific to the Amazon Web Services region
* that they are created in, and you can't use encryption keys from one Amazon Web Services region in another Amazon
* Web Services region.
*
*
* If you copy an unencrypted elastic cluster snapshot and specify a value for the KmsKeyId
parameter,
* an error is returned.
*
*/
private String kmsKeyId;
/**
*
* The Amazon Resource Name (ARN) identifier of the elastic cluster snapshot.
*
*/
private String snapshotArn;
/**
*
* The tags to be assigned to the elastic cluster snapshot.
*
*/
private java.util.Map tags;
/**
*
* The identifier of the new elastic cluster snapshot to create from the source cluster snapshot. This parameter is
* not case sensitive.
*
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 letters, numbers, or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: elastic-cluster-snapshot-5
*
*/
private String targetSnapshotName;
/**
*
* Set to true
to copy all tags from the source cluster snapshot to the target elastic cluster
* snapshot. The default is false
.
*
*
* @param copyTags
* Set to true
to copy all tags from the source cluster snapshot to the target elastic cluster
* snapshot. The default is false
.
*/
public void setCopyTags(Boolean copyTags) {
this.copyTags = copyTags;
}
/**
*
* Set to true
to copy all tags from the source cluster snapshot to the target elastic cluster
* snapshot. The default is false
.
*
*
* @return Set to true
to copy all tags from the source cluster snapshot to the target elastic cluster
* snapshot. The default is false
.
*/
public Boolean getCopyTags() {
return this.copyTags;
}
/**
*
* Set to true
to copy all tags from the source cluster snapshot to the target elastic cluster
* snapshot. The default is false
.
*
*
* @param copyTags
* Set to true
to copy all tags from the source cluster snapshot to the target elastic cluster
* snapshot. The default is false
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyClusterSnapshotRequest withCopyTags(Boolean copyTags) {
setCopyTags(copyTags);
return this;
}
/**
*
* Set to true
to copy all tags from the source cluster snapshot to the target elastic cluster
* snapshot. The default is false
.
*
*
* @return Set to true
to copy all tags from the source cluster snapshot to the target elastic cluster
* snapshot. The default is false
.
*/
public Boolean isCopyTags() {
return this.copyTags;
}
/**
*
* The Amazon Web Services KMS key ID for an encrypted elastic cluster snapshot. The Amazon Web Services KMS key ID
* is the Amazon Resource Name (ARN), Amazon Web Services KMS key identifier, or the Amazon Web Services KMS key
* alias for the Amazon Web Services KMS encryption key.
*
*
* If you copy an encrypted elastic cluster snapshot from your Amazon Web Services account, you can specify a value
* for KmsKeyId
to encrypt the copy with a new Amazon Web ServicesS KMS encryption key. If you don't
* specify a value for KmsKeyId
, then the copy of the elastic cluster snapshot is encrypted with the
* same AWS
KMS key as the source elastic cluster snapshot.
*
*
* To copy an encrypted elastic cluster snapshot to another Amazon Web Services region, set KmsKeyId
to
* the Amazon Web Services KMS key ID that you want to use to encrypt the copy of the elastic cluster snapshot in
* the destination region. Amazon Web Services KMS encryption keys are specific to the Amazon Web Services region
* that they are created in, and you can't use encryption keys from one Amazon Web Services region in another Amazon
* Web Services region.
*
*
* If you copy an unencrypted elastic cluster snapshot and specify a value for the KmsKeyId
parameter,
* an error is returned.
*
*
* @param kmsKeyId
* The Amazon Web Services KMS key ID for an encrypted elastic cluster snapshot. The Amazon Web Services KMS
* key ID is the Amazon Resource Name (ARN), Amazon Web Services KMS key identifier, or the Amazon Web
* Services KMS key alias for the Amazon Web Services KMS encryption key.
*
* If you copy an encrypted elastic cluster snapshot from your Amazon Web Services account, you can specify a
* value for KmsKeyId
to encrypt the copy with a new Amazon Web ServicesS KMS encryption key. If
* you don't specify a value for KmsKeyId
, then the copy of the elastic cluster snapshot is
* encrypted with the same AWS
KMS key as the source elastic cluster snapshot.
*
*
* To copy an encrypted elastic cluster snapshot to another Amazon Web Services region, set
* KmsKeyId
to the Amazon Web Services KMS key ID that you want to use to encrypt the copy of
* the elastic cluster snapshot in the destination region. Amazon Web Services KMS encryption keys are
* specific to the Amazon Web Services region that they are created in, and you can't use encryption keys
* from one Amazon Web Services region in another Amazon Web Services region.
*
*
* If you copy an unencrypted elastic cluster snapshot and specify a value for the KmsKeyId
* parameter, an error is returned.
*/
public void setKmsKeyId(String kmsKeyId) {
this.kmsKeyId = kmsKeyId;
}
/**
*
* The Amazon Web Services KMS key ID for an encrypted elastic cluster snapshot. The Amazon Web Services KMS key ID
* is the Amazon Resource Name (ARN), Amazon Web Services KMS key identifier, or the Amazon Web Services KMS key
* alias for the Amazon Web Services KMS encryption key.
*
*
* If you copy an encrypted elastic cluster snapshot from your Amazon Web Services account, you can specify a value
* for KmsKeyId
to encrypt the copy with a new Amazon Web ServicesS KMS encryption key. If you don't
* specify a value for KmsKeyId
, then the copy of the elastic cluster snapshot is encrypted with the
* same AWS
KMS key as the source elastic cluster snapshot.
*
*
* To copy an encrypted elastic cluster snapshot to another Amazon Web Services region, set KmsKeyId
to
* the Amazon Web Services KMS key ID that you want to use to encrypt the copy of the elastic cluster snapshot in
* the destination region. Amazon Web Services KMS encryption keys are specific to the Amazon Web Services region
* that they are created in, and you can't use encryption keys from one Amazon Web Services region in another Amazon
* Web Services region.
*
*
* If you copy an unencrypted elastic cluster snapshot and specify a value for the KmsKeyId
parameter,
* an error is returned.
*
*
* @return The Amazon Web Services KMS key ID for an encrypted elastic cluster snapshot. The Amazon Web Services KMS
* key ID is the Amazon Resource Name (ARN), Amazon Web Services KMS key identifier, or the Amazon Web
* Services KMS key alias for the Amazon Web Services KMS encryption key.
*
* If you copy an encrypted elastic cluster snapshot from your Amazon Web Services account, you can specify
* a value for KmsKeyId
to encrypt the copy with a new Amazon Web ServicesS KMS encryption key.
* If you don't specify a value for KmsKeyId
, then the copy of the elastic cluster snapshot is
* encrypted with the same AWS
KMS key as the source elastic cluster snapshot.
*
*
* To copy an encrypted elastic cluster snapshot to another Amazon Web Services region, set
* KmsKeyId
to the Amazon Web Services KMS key ID that you want to use to encrypt the copy of
* the elastic cluster snapshot in the destination region. Amazon Web Services KMS encryption keys are
* specific to the Amazon Web Services region that they are created in, and you can't use encryption keys
* from one Amazon Web Services region in another Amazon Web Services region.
*
*
* If you copy an unencrypted elastic cluster snapshot and specify a value for the KmsKeyId
* parameter, an error is returned.
*/
public String getKmsKeyId() {
return this.kmsKeyId;
}
/**
*
* The Amazon Web Services KMS key ID for an encrypted elastic cluster snapshot. The Amazon Web Services KMS key ID
* is the Amazon Resource Name (ARN), Amazon Web Services KMS key identifier, or the Amazon Web Services KMS key
* alias for the Amazon Web Services KMS encryption key.
*
*
* If you copy an encrypted elastic cluster snapshot from your Amazon Web Services account, you can specify a value
* for KmsKeyId
to encrypt the copy with a new Amazon Web ServicesS KMS encryption key. If you don't
* specify a value for KmsKeyId
, then the copy of the elastic cluster snapshot is encrypted with the
* same AWS
KMS key as the source elastic cluster snapshot.
*
*
* To copy an encrypted elastic cluster snapshot to another Amazon Web Services region, set KmsKeyId
to
* the Amazon Web Services KMS key ID that you want to use to encrypt the copy of the elastic cluster snapshot in
* the destination region. Amazon Web Services KMS encryption keys are specific to the Amazon Web Services region
* that they are created in, and you can't use encryption keys from one Amazon Web Services region in another Amazon
* Web Services region.
*
*
* If you copy an unencrypted elastic cluster snapshot and specify a value for the KmsKeyId
parameter,
* an error is returned.
*
*
* @param kmsKeyId
* The Amazon Web Services KMS key ID for an encrypted elastic cluster snapshot. The Amazon Web Services KMS
* key ID is the Amazon Resource Name (ARN), Amazon Web Services KMS key identifier, or the Amazon Web
* Services KMS key alias for the Amazon Web Services KMS encryption key.
*
* If you copy an encrypted elastic cluster snapshot from your Amazon Web Services account, you can specify a
* value for KmsKeyId
to encrypt the copy with a new Amazon Web ServicesS KMS encryption key. If
* you don't specify a value for KmsKeyId
, then the copy of the elastic cluster snapshot is
* encrypted with the same AWS
KMS key as the source elastic cluster snapshot.
*
*
* To copy an encrypted elastic cluster snapshot to another Amazon Web Services region, set
* KmsKeyId
to the Amazon Web Services KMS key ID that you want to use to encrypt the copy of
* the elastic cluster snapshot in the destination region. Amazon Web Services KMS encryption keys are
* specific to the Amazon Web Services region that they are created in, and you can't use encryption keys
* from one Amazon Web Services region in another Amazon Web Services region.
*
*
* If you copy an unencrypted elastic cluster snapshot and specify a value for the KmsKeyId
* parameter, an error is returned.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyClusterSnapshotRequest withKmsKeyId(String kmsKeyId) {
setKmsKeyId(kmsKeyId);
return this;
}
/**
*
* The Amazon Resource Name (ARN) identifier of the elastic cluster snapshot.
*
*
* @param snapshotArn
* The Amazon Resource Name (ARN) identifier of the elastic cluster snapshot.
*/
public void setSnapshotArn(String snapshotArn) {
this.snapshotArn = snapshotArn;
}
/**
*
* The Amazon Resource Name (ARN) identifier of the elastic cluster snapshot.
*
*
* @return The Amazon Resource Name (ARN) identifier of the elastic cluster snapshot.
*/
public String getSnapshotArn() {
return this.snapshotArn;
}
/**
*
* The Amazon Resource Name (ARN) identifier of the elastic cluster snapshot.
*
*
* @param snapshotArn
* The Amazon Resource Name (ARN) identifier of the elastic cluster snapshot.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyClusterSnapshotRequest withSnapshotArn(String snapshotArn) {
setSnapshotArn(snapshotArn);
return this;
}
/**
*
* The tags to be assigned to the elastic cluster snapshot.
*
*
* @return The tags to be assigned to the elastic cluster snapshot.
*/
public java.util.Map getTags() {
return tags;
}
/**
*
* The tags to be assigned to the elastic cluster snapshot.
*
*
* @param tags
* The tags to be assigned to the elastic cluster snapshot.
*/
public void setTags(java.util.Map tags) {
this.tags = tags;
}
/**
*
* The tags to be assigned to the elastic cluster snapshot.
*
*
* @param tags
* The tags to be assigned to the elastic cluster snapshot.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyClusterSnapshotRequest withTags(java.util.Map tags) {
setTags(tags);
return this;
}
/**
* Add a single Tags entry
*
* @see CopyClusterSnapshotRequest#withTags
* @returns a reference to this object so that method calls can be chained together.
*/
public CopyClusterSnapshotRequest addTagsEntry(String key, String value) {
if (null == this.tags) {
this.tags = new java.util.HashMap();
}
if (this.tags.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.tags.put(key, value);
return this;
}
/**
* Removes all the entries added into Tags.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyClusterSnapshotRequest clearTagsEntries() {
this.tags = null;
return this;
}
/**
*
* The identifier of the new elastic cluster snapshot to create from the source cluster snapshot. This parameter is
* not case sensitive.
*
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 letters, numbers, or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: elastic-cluster-snapshot-5
*
*
* @param targetSnapshotName
* The identifier of the new elastic cluster snapshot to create from the source cluster snapshot. This
* parameter is not case sensitive.
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 letters, numbers, or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: elastic-cluster-snapshot-5
*/
public void setTargetSnapshotName(String targetSnapshotName) {
this.targetSnapshotName = targetSnapshotName;
}
/**
*
* The identifier of the new elastic cluster snapshot to create from the source cluster snapshot. This parameter is
* not case sensitive.
*
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 letters, numbers, or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: elastic-cluster-snapshot-5
*
*
* @return The identifier of the new elastic cluster snapshot to create from the source cluster snapshot. This
* parameter is not case sensitive.
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 letters, numbers, or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: elastic-cluster-snapshot-5
*/
public String getTargetSnapshotName() {
return this.targetSnapshotName;
}
/**
*
* The identifier of the new elastic cluster snapshot to create from the source cluster snapshot. This parameter is
* not case sensitive.
*
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 letters, numbers, or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: elastic-cluster-snapshot-5
*
*
* @param targetSnapshotName
* The identifier of the new elastic cluster snapshot to create from the source cluster snapshot. This
* parameter is not case sensitive.
*
* Constraints:
*
*
* -
*
* Must contain from 1 to 63 letters, numbers, or hyphens.
*
*
* -
*
* The first character must be a letter.
*
*
* -
*
* Cannot end with a hyphen or contain two consecutive hyphens.
*
*
*
*
* Example: elastic-cluster-snapshot-5
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CopyClusterSnapshotRequest withTargetSnapshotName(String targetSnapshotName) {
setTargetSnapshotName(targetSnapshotName);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCopyTags() != null)
sb.append("CopyTags: ").append(getCopyTags()).append(",");
if (getKmsKeyId() != null)
sb.append("KmsKeyId: ").append(getKmsKeyId()).append(",");
if (getSnapshotArn() != null)
sb.append("SnapshotArn: ").append(getSnapshotArn()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getTargetSnapshotName() != null)
sb.append("TargetSnapshotName: ").append(getTargetSnapshotName());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CopyClusterSnapshotRequest == false)
return false;
CopyClusterSnapshotRequest other = (CopyClusterSnapshotRequest) obj;
if (other.getCopyTags() == null ^ this.getCopyTags() == null)
return false;
if (other.getCopyTags() != null && other.getCopyTags().equals(this.getCopyTags()) == false)
return false;
if (other.getKmsKeyId() == null ^ this.getKmsKeyId() == null)
return false;
if (other.getKmsKeyId() != null && other.getKmsKeyId().equals(this.getKmsKeyId()) == false)
return false;
if (other.getSnapshotArn() == null ^ this.getSnapshotArn() == null)
return false;
if (other.getSnapshotArn() != null && other.getSnapshotArn().equals(this.getSnapshotArn()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getTargetSnapshotName() == null ^ this.getTargetSnapshotName() == null)
return false;
if (other.getTargetSnapshotName() != null && other.getTargetSnapshotName().equals(this.getTargetSnapshotName()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCopyTags() == null) ? 0 : getCopyTags().hashCode());
hashCode = prime * hashCode + ((getKmsKeyId() == null) ? 0 : getKmsKeyId().hashCode());
hashCode = prime * hashCode + ((getSnapshotArn() == null) ? 0 : getSnapshotArn().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getTargetSnapshotName() == null) ? 0 : getTargetSnapshotName().hashCode());
return hashCode;
}
@Override
public CopyClusterSnapshotRequest clone() {
return (CopyClusterSnapshotRequest) super.clone();
}
}