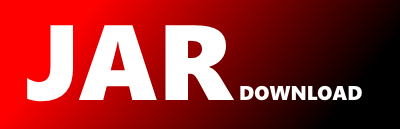
com.amazonaws.services.ebs.AmazonEBS Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ebs;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.ebs.model.*;
/**
* Interface for accessing Amazon EBS.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.ebs.AbstractAmazonEBS} instead.
*
*
*
* You can use the Amazon Elastic Block Store (Amazon EBS) direct APIs to create Amazon EBS snapshots, write data
* directly to your snapshots, read data on your snapshots, and identify the differences or changes between two
* snapshots. If you’re an independent software vendor (ISV) who offers backup services for Amazon EBS, the EBS direct
* APIs make it more efficient and cost-effective to track incremental changes on your Amazon EBS volumes through
* snapshots. This can be done without having to create new volumes from snapshots, and then use Amazon Elastic Compute
* Cloud (Amazon EC2) instances to compare the differences.
*
*
* You can create incremental snapshots directly from data on-premises into volumes and the cloud to use for quick
* disaster recovery. With the ability to write and read snapshots, you can write your on-premises data to an snapshot
* during a disaster. Then after recovery, you can restore it back to Amazon Web Services or on-premises from the
* snapshot. You no longer need to build and maintain complex mechanisms to copy data to and from Amazon EBS.
*
*
* This API reference provides detailed information about the actions, data types, parameters, and errors of the EBS
* direct APIs. For more information about the elements that make up the EBS direct APIs, and examples of how to use
* them effectively, see Accessing the Contents of an
* Amazon EBS Snapshot in the Amazon Elastic Compute Cloud User Guide. For more information about the
* supported Amazon Web Services Regions, endpoints, and service quotas for the EBS direct APIs, see Amazon Elastic Block Store Endpoints and
* Quotas in the Amazon Web Services General Reference.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonEBS {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "ebs";
/**
*
* Seals and completes the snapshot after all of the required blocks of data have been written to it. Completing the
* snapshot changes the status to completed
. You cannot write new blocks to a snapshot after it has
* been completed.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* @param completeSnapshotRequest
* @return Result of the CompleteSnapshot operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @sample AmazonEBS.CompleteSnapshot
* @see AWS API
* Documentation
*/
CompleteSnapshotResult completeSnapshot(CompleteSnapshotRequest completeSnapshotRequest);
/**
*
* Returns the data in a block in an Amazon Elastic Block Store snapshot.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* @param getSnapshotBlockRequest
* @return Result of the GetSnapshotBlock operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @sample AmazonEBS.GetSnapshotBlock
* @see AWS API
* Documentation
*/
GetSnapshotBlockResult getSnapshotBlock(GetSnapshotBlockRequest getSnapshotBlockRequest);
/**
*
* Returns information about the blocks that are different between two Amazon Elastic Block Store snapshots of the
* same volume/snapshot lineage.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* @param listChangedBlocksRequest
* @return Result of the ListChangedBlocks operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @sample AmazonEBS.ListChangedBlocks
* @see AWS API
* Documentation
*/
ListChangedBlocksResult listChangedBlocks(ListChangedBlocksRequest listChangedBlocksRequest);
/**
*
* Returns information about the blocks in an Amazon Elastic Block Store snapshot.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* @param listSnapshotBlocksRequest
* @return Result of the ListSnapshotBlocks operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @sample AmazonEBS.ListSnapshotBlocks
* @see AWS API
* Documentation
*/
ListSnapshotBlocksResult listSnapshotBlocks(ListSnapshotBlocksRequest listSnapshotBlocksRequest);
/**
*
* Writes a block of data to a snapshot. If the specified block contains data, the existing data is overwritten. The
* target snapshot must be in the pending
state.
*
*
* Data written to a snapshot must be aligned with 512-KiB sectors.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* @param putSnapshotBlockRequest
* @return Result of the PutSnapshotBlock operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @sample AmazonEBS.PutSnapshotBlock
* @see AWS API
* Documentation
*/
PutSnapshotBlockResult putSnapshotBlock(PutSnapshotBlockRequest putSnapshotBlockRequest);
/**
*
* Creates a new Amazon EBS snapshot. The new snapshot enters the pending
state after the request
* completes.
*
*
* After creating the snapshot, use PutSnapshotBlock to
* write blocks of data to the snapshot.
*
*
*
* You should always retry requests that receive server (5xx
) error responses, and
* ThrottlingException
and RequestThrottledException
client error responses. For more
* information see Error
* retries in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* @param startSnapshotRequest
* @return Result of the StartSnapshot operation returned by the service.
* @throws AccessDeniedException
* You do not have sufficient access to perform this action.
* @throws ValidationException
* The input fails to satisfy the constraints of the EBS direct APIs.
* @throws RequestThrottledException
* The number of API requests has exceeded the maximum allowed API request throttling limit for the
* snapshot. For more information see Error retries.
* @throws ResourceNotFoundException
* The specified resource does not exist.
* @throws ServiceQuotaExceededException
* Your current service quotas do not allow you to perform this action.
* @throws InternalServerException
* An internal error has occurred. For more information see Error retries.
* @throws ConcurrentLimitExceededException
* You have reached the limit for concurrent API requests. For more information, see Optimizing performance of the EBS direct APIs in the Amazon Elastic Compute Cloud User Guide.
* @throws ConflictException
* The request uses the same client token as a previous, but non-identical request.
* @sample AmazonEBS.StartSnapshot
* @see AWS API
* Documentation
*/
StartSnapshotResult startSnapshot(StartSnapshotRequest startSnapshotRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}