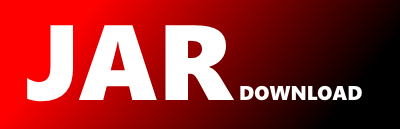
com.amazonaws.services.ec2.AmazonEC2Async Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ec2 Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ec2;
import javax.annotation.Generated;
import com.amazonaws.services.ec2.model.*;
/**
* Interface for accessing Amazon EC2 asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.ec2.AbstractAmazonEC2Async} instead.
*
*
* Amazon Elastic Compute Cloud
*
* Amazon Elastic Compute Cloud (Amazon EC2) provides secure and resizable computing capacity in the AWS cloud. Using
* Amazon EC2 eliminates the need to invest in hardware up front, so you can develop and deploy applications faster.
*
*
* To learn more, see the following resources:
*
*
* -
*
* Amazon EC2: AmazonEC2 product page, Amazon EC2 documentation
*
*
* -
*
* Amazon EBS: Amazon EBS product page, Amazon EBS documentation
*
*
* -
*
* Amazon VPC: Amazon VPC product page, Amazon VPC documentation
*
*
* -
*
* AWS VPN: AWS VPN product page, AWS VPN documentation
*
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonEC2Async extends AmazonEC2 {
/**
*
* Accepts the Convertible Reserved Instance exchange quote described in the
* GetReservedInstancesExchangeQuote call.
*
*
* @param acceptReservedInstancesExchangeQuoteRequest
* Contains the parameters for accepting the quote.
* @return A Java Future containing the result of the AcceptReservedInstancesExchangeQuote operation returned by the
* service.
* @sample AmazonEC2Async.AcceptReservedInstancesExchangeQuote
* @see AWS API Documentation
*/
java.util.concurrent.Future acceptReservedInstancesExchangeQuoteAsync(
AcceptReservedInstancesExchangeQuoteRequest acceptReservedInstancesExchangeQuoteRequest);
/**
*
* Accepts the Convertible Reserved Instance exchange quote described in the
* GetReservedInstancesExchangeQuote call.
*
*
* @param acceptReservedInstancesExchangeQuoteRequest
* Contains the parameters for accepting the quote.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AcceptReservedInstancesExchangeQuote operation returned by the
* service.
* @sample AmazonEC2AsyncHandler.AcceptReservedInstancesExchangeQuote
* @see AWS API Documentation
*/
java.util.concurrent.Future acceptReservedInstancesExchangeQuoteAsync(
AcceptReservedInstancesExchangeQuoteRequest acceptReservedInstancesExchangeQuoteRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Accepts a request to attach a VPC to a transit gateway.
*
*
* The VPC attachment must be in the pendingAcceptance
state. Use
* DescribeTransitGatewayVpcAttachments to view your pending VPC attachment requests. Use
* RejectTransitGatewayVpcAttachment to reject a VPC attachment request.
*
*
* @param acceptTransitGatewayVpcAttachmentRequest
* @return A Java Future containing the result of the AcceptTransitGatewayVpcAttachment operation returned by the
* service.
* @sample AmazonEC2Async.AcceptTransitGatewayVpcAttachment
* @see AWS API Documentation
*/
java.util.concurrent.Future acceptTransitGatewayVpcAttachmentAsync(
AcceptTransitGatewayVpcAttachmentRequest acceptTransitGatewayVpcAttachmentRequest);
/**
*
* Accepts a request to attach a VPC to a transit gateway.
*
*
* The VPC attachment must be in the pendingAcceptance
state. Use
* DescribeTransitGatewayVpcAttachments to view your pending VPC attachment requests. Use
* RejectTransitGatewayVpcAttachment to reject a VPC attachment request.
*
*
* @param acceptTransitGatewayVpcAttachmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AcceptTransitGatewayVpcAttachment operation returned by the
* service.
* @sample AmazonEC2AsyncHandler.AcceptTransitGatewayVpcAttachment
* @see AWS API Documentation
*/
java.util.concurrent.Future acceptTransitGatewayVpcAttachmentAsync(
AcceptTransitGatewayVpcAttachmentRequest acceptTransitGatewayVpcAttachmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Accepts one or more interface VPC endpoint connection requests to your VPC endpoint service.
*
*
* @param acceptVpcEndpointConnectionsRequest
* @return A Java Future containing the result of the AcceptVpcEndpointConnections operation returned by the
* service.
* @sample AmazonEC2Async.AcceptVpcEndpointConnections
* @see AWS API Documentation
*/
java.util.concurrent.Future acceptVpcEndpointConnectionsAsync(
AcceptVpcEndpointConnectionsRequest acceptVpcEndpointConnectionsRequest);
/**
*
* Accepts one or more interface VPC endpoint connection requests to your VPC endpoint service.
*
*
* @param acceptVpcEndpointConnectionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AcceptVpcEndpointConnections operation returned by the
* service.
* @sample AmazonEC2AsyncHandler.AcceptVpcEndpointConnections
* @see AWS API Documentation
*/
java.util.concurrent.Future acceptVpcEndpointConnectionsAsync(
AcceptVpcEndpointConnectionsRequest acceptVpcEndpointConnectionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Accept a VPC peering connection request. To accept a request, the VPC peering connection must be in the
* pending-acceptance
state, and you must be the owner of the peer VPC. Use
* DescribeVpcPeeringConnections to view your outstanding VPC peering connection requests.
*
*
* For an inter-Region VPC peering connection request, you must accept the VPC peering connection in the Region of
* the accepter VPC.
*
*
* @param acceptVpcPeeringConnectionRequest
* @return A Java Future containing the result of the AcceptVpcPeeringConnection operation returned by the service.
* @sample AmazonEC2Async.AcceptVpcPeeringConnection
* @see AWS
* API Documentation
*/
java.util.concurrent.Future acceptVpcPeeringConnectionAsync(
AcceptVpcPeeringConnectionRequest acceptVpcPeeringConnectionRequest);
/**
*
* Accept a VPC peering connection request. To accept a request, the VPC peering connection must be in the
* pending-acceptance
state, and you must be the owner of the peer VPC. Use
* DescribeVpcPeeringConnections to view your outstanding VPC peering connection requests.
*
*
* For an inter-Region VPC peering connection request, you must accept the VPC peering connection in the Region of
* the accepter VPC.
*
*
* @param acceptVpcPeeringConnectionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AcceptVpcPeeringConnection operation returned by the service.
* @sample AmazonEC2AsyncHandler.AcceptVpcPeeringConnection
* @see AWS
* API Documentation
*/
java.util.concurrent.Future acceptVpcPeeringConnectionAsync(
AcceptVpcPeeringConnectionRequest acceptVpcPeeringConnectionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the AcceptVpcPeeringConnection operation.
*
* @see #acceptVpcPeeringConnectionAsync(AcceptVpcPeeringConnectionRequest)
*/
java.util.concurrent.Future acceptVpcPeeringConnectionAsync();
/**
* Simplified method form for invoking the AcceptVpcPeeringConnection operation with an AsyncHandler.
*
* @see #acceptVpcPeeringConnectionAsync(AcceptVpcPeeringConnectionRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future acceptVpcPeeringConnectionAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Advertises an IPv4 address range that is provisioned for use with your AWS resources through bring your own IP
* addresses (BYOIP).
*
*
* You can perform this operation at most once every 10 seconds, even if you specify different address ranges each
* time.
*
*
* We recommend that you stop advertising the BYOIP CIDR from other locations when you advertise it from AWS. To
* minimize down time, you can configure your AWS resources to use an address from a BYOIP CIDR before it is
* advertised, and then simultaneously stop advertising it from the current location and start advertising it
* through AWS.
*
*
* It can take a few minutes before traffic to the specified addresses starts routing to AWS because of BGP
* propagation delays.
*
*
* To stop advertising the BYOIP CIDR, use WithdrawByoipCidr.
*
*
* @param advertiseByoipCidrRequest
* @return A Java Future containing the result of the AdvertiseByoipCidr operation returned by the service.
* @sample AmazonEC2Async.AdvertiseByoipCidr
* @see AWS API
* Documentation
*/
java.util.concurrent.Future advertiseByoipCidrAsync(AdvertiseByoipCidrRequest advertiseByoipCidrRequest);
/**
*
* Advertises an IPv4 address range that is provisioned for use with your AWS resources through bring your own IP
* addresses (BYOIP).
*
*
* You can perform this operation at most once every 10 seconds, even if you specify different address ranges each
* time.
*
*
* We recommend that you stop advertising the BYOIP CIDR from other locations when you advertise it from AWS. To
* minimize down time, you can configure your AWS resources to use an address from a BYOIP CIDR before it is
* advertised, and then simultaneously stop advertising it from the current location and start advertising it
* through AWS.
*
*
* It can take a few minutes before traffic to the specified addresses starts routing to AWS because of BGP
* propagation delays.
*
*
* To stop advertising the BYOIP CIDR, use WithdrawByoipCidr.
*
*
* @param advertiseByoipCidrRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AdvertiseByoipCidr operation returned by the service.
* @sample AmazonEC2AsyncHandler.AdvertiseByoipCidr
* @see AWS API
* Documentation
*/
java.util.concurrent.Future advertiseByoipCidrAsync(AdvertiseByoipCidrRequest advertiseByoipCidrRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Allocates an Elastic IP address to your AWS account. After you allocate the Elastic IP address you can associate
* it with an instance or network interface. After you release an Elastic IP address, it is released to the IP
* address pool and can be allocated to a different AWS account.
*
*
* You can allocate an Elastic IP address from an address pool owned by AWS or from an address pool created from a
* public IPv4 address range that you have brought to AWS for use with your AWS resources using bring your own IP
* addresses (BYOIP). For more information, see Bring Your Own IP Addresses (BYOIP)
* in the Amazon Elastic Compute Cloud User Guide.
*
*
* [EC2-VPC] If you release an Elastic IP address, you might be able to recover it. You cannot recover an Elastic IP
* address that you released after it is allocated to another AWS account. You cannot recover an Elastic IP address
* for EC2-Classic. To attempt to recover an Elastic IP address that you released, specify it in this operation.
*
*
* An Elastic IP address is for use either in the EC2-Classic platform or in a VPC. By default, you can allocate 5
* Elastic IP addresses for EC2-Classic per Region and 5 Elastic IP addresses for EC2-VPC per Region.
*
*
* For more information, see Elastic IP Addresses
* in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param allocateAddressRequest
* @return A Java Future containing the result of the AllocateAddress operation returned by the service.
* @sample AmazonEC2Async.AllocateAddress
* @see AWS API
* Documentation
*/
java.util.concurrent.Future allocateAddressAsync(AllocateAddressRequest allocateAddressRequest);
/**
*
* Allocates an Elastic IP address to your AWS account. After you allocate the Elastic IP address you can associate
* it with an instance or network interface. After you release an Elastic IP address, it is released to the IP
* address pool and can be allocated to a different AWS account.
*
*
* You can allocate an Elastic IP address from an address pool owned by AWS or from an address pool created from a
* public IPv4 address range that you have brought to AWS for use with your AWS resources using bring your own IP
* addresses (BYOIP). For more information, see Bring Your Own IP Addresses (BYOIP)
* in the Amazon Elastic Compute Cloud User Guide.
*
*
* [EC2-VPC] If you release an Elastic IP address, you might be able to recover it. You cannot recover an Elastic IP
* address that you released after it is allocated to another AWS account. You cannot recover an Elastic IP address
* for EC2-Classic. To attempt to recover an Elastic IP address that you released, specify it in this operation.
*
*
* An Elastic IP address is for use either in the EC2-Classic platform or in a VPC. By default, you can allocate 5
* Elastic IP addresses for EC2-Classic per Region and 5 Elastic IP addresses for EC2-VPC per Region.
*
*
* For more information, see Elastic IP Addresses
* in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param allocateAddressRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AllocateAddress operation returned by the service.
* @sample AmazonEC2AsyncHandler.AllocateAddress
* @see AWS API
* Documentation
*/
java.util.concurrent.Future allocateAddressAsync(AllocateAddressRequest allocateAddressRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the AllocateAddress operation.
*
* @see #allocateAddressAsync(AllocateAddressRequest)
*/
java.util.concurrent.Future allocateAddressAsync();
/**
* Simplified method form for invoking the AllocateAddress operation with an AsyncHandler.
*
* @see #allocateAddressAsync(AllocateAddressRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future allocateAddressAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Allocates a Dedicated Host to your account. At a minimum, specify the instance size type, Availability Zone, and
* quantity of hosts to allocate.
*
*
* @param allocateHostsRequest
* @return A Java Future containing the result of the AllocateHosts operation returned by the service.
* @sample AmazonEC2Async.AllocateHosts
* @see AWS API
* Documentation
*/
java.util.concurrent.Future allocateHostsAsync(AllocateHostsRequest allocateHostsRequest);
/**
*
* Allocates a Dedicated Host to your account. At a minimum, specify the instance size type, Availability Zone, and
* quantity of hosts to allocate.
*
*
* @param allocateHostsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AllocateHosts operation returned by the service.
* @sample AmazonEC2AsyncHandler.AllocateHosts
* @see AWS API
* Documentation
*/
java.util.concurrent.Future allocateHostsAsync(AllocateHostsRequest allocateHostsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Applies a security group to the association between the target network and the Client VPN endpoint. This action
* replaces the existing security groups with the specified security groups.
*
*
* @param applySecurityGroupsToClientVpnTargetNetworkRequest
* @return A Java Future containing the result of the ApplySecurityGroupsToClientVpnTargetNetwork operation returned
* by the service.
* @sample AmazonEC2Async.ApplySecurityGroupsToClientVpnTargetNetwork
* @see AWS API Documentation
*/
java.util.concurrent.Future applySecurityGroupsToClientVpnTargetNetworkAsync(
ApplySecurityGroupsToClientVpnTargetNetworkRequest applySecurityGroupsToClientVpnTargetNetworkRequest);
/**
*
* Applies a security group to the association between the target network and the Client VPN endpoint. This action
* replaces the existing security groups with the specified security groups.
*
*
* @param applySecurityGroupsToClientVpnTargetNetworkRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ApplySecurityGroupsToClientVpnTargetNetwork operation returned
* by the service.
* @sample AmazonEC2AsyncHandler.ApplySecurityGroupsToClientVpnTargetNetwork
* @see AWS API Documentation
*/
java.util.concurrent.Future applySecurityGroupsToClientVpnTargetNetworkAsync(
ApplySecurityGroupsToClientVpnTargetNetworkRequest applySecurityGroupsToClientVpnTargetNetworkRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Assigns one or more IPv6 addresses to the specified network interface. You can specify one or more specific IPv6
* addresses, or you can specify the number of IPv6 addresses to be automatically assigned from within the subnet's
* IPv6 CIDR block range. You can assign as many IPv6 addresses to a network interface as you can assign private
* IPv4 addresses, and the limit varies per instance type. For information, see IP Addresses Per
* Network Interface Per Instance Type in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param assignIpv6AddressesRequest
* @return A Java Future containing the result of the AssignIpv6Addresses operation returned by the service.
* @sample AmazonEC2Async.AssignIpv6Addresses
* @see AWS API
* Documentation
*/
java.util.concurrent.Future assignIpv6AddressesAsync(AssignIpv6AddressesRequest assignIpv6AddressesRequest);
/**
*
* Assigns one or more IPv6 addresses to the specified network interface. You can specify one or more specific IPv6
* addresses, or you can specify the number of IPv6 addresses to be automatically assigned from within the subnet's
* IPv6 CIDR block range. You can assign as many IPv6 addresses to a network interface as you can assign private
* IPv4 addresses, and the limit varies per instance type. For information, see IP Addresses Per
* Network Interface Per Instance Type in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param assignIpv6AddressesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssignIpv6Addresses operation returned by the service.
* @sample AmazonEC2AsyncHandler.AssignIpv6Addresses
* @see AWS API
* Documentation
*/
java.util.concurrent.Future assignIpv6AddressesAsync(AssignIpv6AddressesRequest assignIpv6AddressesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Assigns one or more secondary private IP addresses to the specified network interface.
*
*
* You can specify one or more specific secondary IP addresses, or you can specify the number of secondary IP
* addresses to be automatically assigned within the subnet's CIDR block range. The number of secondary IP addresses
* that you can assign to an instance varies by instance type. For information about instance types, see Instance Types in the
* Amazon Elastic Compute Cloud User Guide. For more information about Elastic IP addresses, see Elastic IP Addresses
* in the Amazon Elastic Compute Cloud User Guide.
*
*
* When you move a secondary private IP address to another network interface, any Elastic IP address that is
* associated with the IP address is also moved.
*
*
* Remapping an IP address is an asynchronous operation. When you move an IP address from one network interface to
* another, check network/interfaces/macs/mac/local-ipv4s
in the instance metadata to confirm that the
* remapping is complete.
*
*
* @param assignPrivateIpAddressesRequest
* Contains the parameters for AssignPrivateIpAddresses.
* @return A Java Future containing the result of the AssignPrivateIpAddresses operation returned by the service.
* @sample AmazonEC2Async.AssignPrivateIpAddresses
* @see AWS
* API Documentation
*/
java.util.concurrent.Future assignPrivateIpAddressesAsync(AssignPrivateIpAddressesRequest assignPrivateIpAddressesRequest);
/**
*
* Assigns one or more secondary private IP addresses to the specified network interface.
*
*
* You can specify one or more specific secondary IP addresses, or you can specify the number of secondary IP
* addresses to be automatically assigned within the subnet's CIDR block range. The number of secondary IP addresses
* that you can assign to an instance varies by instance type. For information about instance types, see Instance Types in the
* Amazon Elastic Compute Cloud User Guide. For more information about Elastic IP addresses, see Elastic IP Addresses
* in the Amazon Elastic Compute Cloud User Guide.
*
*
* When you move a secondary private IP address to another network interface, any Elastic IP address that is
* associated with the IP address is also moved.
*
*
* Remapping an IP address is an asynchronous operation. When you move an IP address from one network interface to
* another, check network/interfaces/macs/mac/local-ipv4s
in the instance metadata to confirm that the
* remapping is complete.
*
*
* @param assignPrivateIpAddressesRequest
* Contains the parameters for AssignPrivateIpAddresses.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssignPrivateIpAddresses operation returned by the service.
* @sample AmazonEC2AsyncHandler.AssignPrivateIpAddresses
* @see AWS
* API Documentation
*/
java.util.concurrent.Future assignPrivateIpAddressesAsync(AssignPrivateIpAddressesRequest assignPrivateIpAddressesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates an Elastic IP address with an instance or a network interface. Before you can use an Elastic IP
* address, you must allocate it to your account.
*
*
* An Elastic IP address is for use in either the EC2-Classic platform or in a VPC. For more information, see Elastic IP Addresses
* in the Amazon Elastic Compute Cloud User Guide.
*
*
* [EC2-Classic, VPC in an EC2-VPC-only account] If the Elastic IP address is already associated with a different
* instance, it is disassociated from that instance and associated with the specified instance. If you associate an
* Elastic IP address with an instance that has an existing Elastic IP address, the existing address is
* disassociated from the instance, but remains allocated to your account.
*
*
* [VPC in an EC2-Classic account] If you don't specify a private IP address, the Elastic IP address is associated
* with the primary IP address. If the Elastic IP address is already associated with a different instance or a
* network interface, you get an error unless you allow reassociation. You cannot associate an Elastic IP address
* with an instance or network interface that has an existing Elastic IP address.
*
*
*
* This is an idempotent operation. If you perform the operation more than once, Amazon EC2 doesn't return an error,
* and you may be charged for each time the Elastic IP address is remapped to the same instance. For more
* information, see the Elastic IP Addresses section of Amazon
* EC2 Pricing.
*
*
*
* @param associateAddressRequest
* @return A Java Future containing the result of the AssociateAddress operation returned by the service.
* @sample AmazonEC2Async.AssociateAddress
* @see AWS API
* Documentation
*/
java.util.concurrent.Future associateAddressAsync(AssociateAddressRequest associateAddressRequest);
/**
*
* Associates an Elastic IP address with an instance or a network interface. Before you can use an Elastic IP
* address, you must allocate it to your account.
*
*
* An Elastic IP address is for use in either the EC2-Classic platform or in a VPC. For more information, see Elastic IP Addresses
* in the Amazon Elastic Compute Cloud User Guide.
*
*
* [EC2-Classic, VPC in an EC2-VPC-only account] If the Elastic IP address is already associated with a different
* instance, it is disassociated from that instance and associated with the specified instance. If you associate an
* Elastic IP address with an instance that has an existing Elastic IP address, the existing address is
* disassociated from the instance, but remains allocated to your account.
*
*
* [VPC in an EC2-Classic account] If you don't specify a private IP address, the Elastic IP address is associated
* with the primary IP address. If the Elastic IP address is already associated with a different instance or a
* network interface, you get an error unless you allow reassociation. You cannot associate an Elastic IP address
* with an instance or network interface that has an existing Elastic IP address.
*
*
*
* This is an idempotent operation. If you perform the operation more than once, Amazon EC2 doesn't return an error,
* and you may be charged for each time the Elastic IP address is remapped to the same instance. For more
* information, see the Elastic IP Addresses section of Amazon
* EC2 Pricing.
*
*
*
* @param associateAddressRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateAddress operation returned by the service.
* @sample AmazonEC2AsyncHandler.AssociateAddress
* @see AWS API
* Documentation
*/
java.util.concurrent.Future associateAddressAsync(AssociateAddressRequest associateAddressRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates a target network with a Client VPN endpoint. A target network is a subnet in a VPC. You can associate
* multiple subnets from the same VPC with a Client VPN endpoint. You can associate only one subnet in each
* Availability Zone. We recommend that you associate at least two subnets to provide Availability Zone redundancy.
*
*
* @param associateClientVpnTargetNetworkRequest
* @return A Java Future containing the result of the AssociateClientVpnTargetNetwork operation returned by the
* service.
* @sample AmazonEC2Async.AssociateClientVpnTargetNetwork
* @see AWS API Documentation
*/
java.util.concurrent.Future associateClientVpnTargetNetworkAsync(
AssociateClientVpnTargetNetworkRequest associateClientVpnTargetNetworkRequest);
/**
*
* Associates a target network with a Client VPN endpoint. A target network is a subnet in a VPC. You can associate
* multiple subnets from the same VPC with a Client VPN endpoint. You can associate only one subnet in each
* Availability Zone. We recommend that you associate at least two subnets to provide Availability Zone redundancy.
*
*
* @param associateClientVpnTargetNetworkRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateClientVpnTargetNetwork operation returned by the
* service.
* @sample AmazonEC2AsyncHandler.AssociateClientVpnTargetNetwork
* @see AWS API Documentation
*/
java.util.concurrent.Future associateClientVpnTargetNetworkAsync(
AssociateClientVpnTargetNetworkRequest associateClientVpnTargetNetworkRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates a set of DHCP options (that you've previously created) with the specified VPC, or associates no DHCP
* options with the VPC.
*
*
* After you associate the options with the VPC, any existing instances and all new instances that you launch in
* that VPC use the options. You don't need to restart or relaunch the instances. They automatically pick up the
* changes within a few hours, depending on how frequently the instance renews its DHCP lease. You can explicitly
* renew the lease using the operating system on the instance.
*
*
* For more information, see DHCP
* Options Sets in the Amazon Virtual Private Cloud User Guide.
*
*
* @param associateDhcpOptionsRequest
* @return A Java Future containing the result of the AssociateDhcpOptions operation returned by the service.
* @sample AmazonEC2Async.AssociateDhcpOptions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future associateDhcpOptionsAsync(AssociateDhcpOptionsRequest associateDhcpOptionsRequest);
/**
*
* Associates a set of DHCP options (that you've previously created) with the specified VPC, or associates no DHCP
* options with the VPC.
*
*
* After you associate the options with the VPC, any existing instances and all new instances that you launch in
* that VPC use the options. You don't need to restart or relaunch the instances. They automatically pick up the
* changes within a few hours, depending on how frequently the instance renews its DHCP lease. You can explicitly
* renew the lease using the operating system on the instance.
*
*
* For more information, see DHCP
* Options Sets in the Amazon Virtual Private Cloud User Guide.
*
*
* @param associateDhcpOptionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateDhcpOptions operation returned by the service.
* @sample AmazonEC2AsyncHandler.AssociateDhcpOptions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future associateDhcpOptionsAsync(AssociateDhcpOptionsRequest associateDhcpOptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates an IAM instance profile with a running or stopped instance. You cannot associate more than one IAM
* instance profile with an instance.
*
*
* @param associateIamInstanceProfileRequest
* @return A Java Future containing the result of the AssociateIamInstanceProfile operation returned by the service.
* @sample AmazonEC2Async.AssociateIamInstanceProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future associateIamInstanceProfileAsync(
AssociateIamInstanceProfileRequest associateIamInstanceProfileRequest);
/**
*
* Associates an IAM instance profile with a running or stopped instance. You cannot associate more than one IAM
* instance profile with an instance.
*
*
* @param associateIamInstanceProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateIamInstanceProfile operation returned by the service.
* @sample AmazonEC2AsyncHandler.AssociateIamInstanceProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future associateIamInstanceProfileAsync(
AssociateIamInstanceProfileRequest associateIamInstanceProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates a subnet with a route table. The subnet and route table must be in the same VPC. This association
* causes traffic originating from the subnet to be routed according to the routes in the route table. The action
* returns an association ID, which you need in order to disassociate the route table from the subnet later. A route
* table can be associated with multiple subnets.
*
*
* For more information, see Route
* Tables in the Amazon Virtual Private Cloud User Guide.
*
*
* @param associateRouteTableRequest
* @return A Java Future containing the result of the AssociateRouteTable operation returned by the service.
* @sample AmazonEC2Async.AssociateRouteTable
* @see AWS API
* Documentation
*/
java.util.concurrent.Future associateRouteTableAsync(AssociateRouteTableRequest associateRouteTableRequest);
/**
*
* Associates a subnet with a route table. The subnet and route table must be in the same VPC. This association
* causes traffic originating from the subnet to be routed according to the routes in the route table. The action
* returns an association ID, which you need in order to disassociate the route table from the subnet later. A route
* table can be associated with multiple subnets.
*
*
* For more information, see Route
* Tables in the Amazon Virtual Private Cloud User Guide.
*
*
* @param associateRouteTableRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateRouteTable operation returned by the service.
* @sample AmazonEC2AsyncHandler.AssociateRouteTable
* @see AWS API
* Documentation
*/
java.util.concurrent.Future associateRouteTableAsync(AssociateRouteTableRequest associateRouteTableRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates a CIDR block with your subnet. You can only associate a single IPv6 CIDR block with your subnet. An
* IPv6 CIDR block must have a prefix length of /64.
*
*
* @param associateSubnetCidrBlockRequest
* @return A Java Future containing the result of the AssociateSubnetCidrBlock operation returned by the service.
* @sample AmazonEC2Async.AssociateSubnetCidrBlock
* @see AWS
* API Documentation
*/
java.util.concurrent.Future associateSubnetCidrBlockAsync(AssociateSubnetCidrBlockRequest associateSubnetCidrBlockRequest);
/**
*
* Associates a CIDR block with your subnet. You can only associate a single IPv6 CIDR block with your subnet. An
* IPv6 CIDR block must have a prefix length of /64.
*
*
* @param associateSubnetCidrBlockRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateSubnetCidrBlock operation returned by the service.
* @sample AmazonEC2AsyncHandler.AssociateSubnetCidrBlock
* @see AWS
* API Documentation
*/
java.util.concurrent.Future associateSubnetCidrBlockAsync(AssociateSubnetCidrBlockRequest associateSubnetCidrBlockRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates the specified attachment with the specified transit gateway route table. You can associate only one
* route table with an attachment.
*
*
* @param associateTransitGatewayRouteTableRequest
* @return A Java Future containing the result of the AssociateTransitGatewayRouteTable operation returned by the
* service.
* @sample AmazonEC2Async.AssociateTransitGatewayRouteTable
* @see AWS API Documentation
*/
java.util.concurrent.Future associateTransitGatewayRouteTableAsync(
AssociateTransitGatewayRouteTableRequest associateTransitGatewayRouteTableRequest);
/**
*
* Associates the specified attachment with the specified transit gateway route table. You can associate only one
* route table with an attachment.
*
*
* @param associateTransitGatewayRouteTableRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateTransitGatewayRouteTable operation returned by the
* service.
* @sample AmazonEC2AsyncHandler.AssociateTransitGatewayRouteTable
* @see AWS API Documentation
*/
java.util.concurrent.Future associateTransitGatewayRouteTableAsync(
AssociateTransitGatewayRouteTableRequest associateTransitGatewayRouteTableRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates a CIDR block with your VPC. You can associate a secondary IPv4 CIDR block, or you can associate an
* Amazon-provided IPv6 CIDR block. The IPv6 CIDR block size is fixed at /56.
*
*
* For more information about associating CIDR blocks with your VPC and applicable restrictions, see VPC and Subnet Sizing in
* the Amazon Virtual Private Cloud User Guide.
*
*
* @param associateVpcCidrBlockRequest
* @return A Java Future containing the result of the AssociateVpcCidrBlock operation returned by the service.
* @sample AmazonEC2Async.AssociateVpcCidrBlock
* @see AWS API
* Documentation
*/
java.util.concurrent.Future associateVpcCidrBlockAsync(AssociateVpcCidrBlockRequest associateVpcCidrBlockRequest);
/**
*
* Associates a CIDR block with your VPC. You can associate a secondary IPv4 CIDR block, or you can associate an
* Amazon-provided IPv6 CIDR block. The IPv6 CIDR block size is fixed at /56.
*
*
* For more information about associating CIDR blocks with your VPC and applicable restrictions, see VPC and Subnet Sizing in
* the Amazon Virtual Private Cloud User Guide.
*
*
* @param associateVpcCidrBlockRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateVpcCidrBlock operation returned by the service.
* @sample AmazonEC2AsyncHandler.AssociateVpcCidrBlock
* @see AWS API
* Documentation
*/
java.util.concurrent.Future associateVpcCidrBlockAsync(AssociateVpcCidrBlockRequest associateVpcCidrBlockRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Links an EC2-Classic instance to a ClassicLink-enabled VPC through one or more of the VPC's security groups. You
* cannot link an EC2-Classic instance to more than one VPC at a time. You can only link an instance that's in the
* running
state. An instance is automatically unlinked from a VPC when it's stopped - you can link it
* to the VPC again when you restart it.
*
*
* After you've linked an instance, you cannot change the VPC security groups that are associated with it. To change
* the security groups, you must first unlink the instance, and then link it again.
*
*
* Linking your instance to a VPC is sometimes referred to as attaching your instance.
*
*
* @param attachClassicLinkVpcRequest
* @return A Java Future containing the result of the AttachClassicLinkVpc operation returned by the service.
* @sample AmazonEC2Async.AttachClassicLinkVpc
* @see AWS API
* Documentation
*/
java.util.concurrent.Future attachClassicLinkVpcAsync(AttachClassicLinkVpcRequest attachClassicLinkVpcRequest);
/**
*
* Links an EC2-Classic instance to a ClassicLink-enabled VPC through one or more of the VPC's security groups. You
* cannot link an EC2-Classic instance to more than one VPC at a time. You can only link an instance that's in the
* running
state. An instance is automatically unlinked from a VPC when it's stopped - you can link it
* to the VPC again when you restart it.
*
*
* After you've linked an instance, you cannot change the VPC security groups that are associated with it. To change
* the security groups, you must first unlink the instance, and then link it again.
*
*
* Linking your instance to a VPC is sometimes referred to as attaching your instance.
*
*
* @param attachClassicLinkVpcRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AttachClassicLinkVpc operation returned by the service.
* @sample AmazonEC2AsyncHandler.AttachClassicLinkVpc
* @see AWS API
* Documentation
*/
java.util.concurrent.Future attachClassicLinkVpcAsync(AttachClassicLinkVpcRequest attachClassicLinkVpcRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Attaches an internet gateway to a VPC, enabling connectivity between the internet and the VPC. For more
* information about your VPC and internet gateway, see the Amazon Virtual Private Cloud User Guide.
*
*
* @param attachInternetGatewayRequest
* @return A Java Future containing the result of the AttachInternetGateway operation returned by the service.
* @sample AmazonEC2Async.AttachInternetGateway
* @see AWS API
* Documentation
*/
java.util.concurrent.Future attachInternetGatewayAsync(AttachInternetGatewayRequest attachInternetGatewayRequest);
/**
*
* Attaches an internet gateway to a VPC, enabling connectivity between the internet and the VPC. For more
* information about your VPC and internet gateway, see the Amazon Virtual Private Cloud User Guide.
*
*
* @param attachInternetGatewayRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AttachInternetGateway operation returned by the service.
* @sample AmazonEC2AsyncHandler.AttachInternetGateway
* @see AWS API
* Documentation
*/
java.util.concurrent.Future attachInternetGatewayAsync(AttachInternetGatewayRequest attachInternetGatewayRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Attaches a network interface to an instance.
*
*
* @param attachNetworkInterfaceRequest
* Contains the parameters for AttachNetworkInterface.
* @return A Java Future containing the result of the AttachNetworkInterface operation returned by the service.
* @sample AmazonEC2Async.AttachNetworkInterface
* @see AWS API
* Documentation
*/
java.util.concurrent.Future attachNetworkInterfaceAsync(AttachNetworkInterfaceRequest attachNetworkInterfaceRequest);
/**
*
* Attaches a network interface to an instance.
*
*
* @param attachNetworkInterfaceRequest
* Contains the parameters for AttachNetworkInterface.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AttachNetworkInterface operation returned by the service.
* @sample AmazonEC2AsyncHandler.AttachNetworkInterface
* @see AWS API
* Documentation
*/
java.util.concurrent.Future attachNetworkInterfaceAsync(AttachNetworkInterfaceRequest attachNetworkInterfaceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Attaches an EBS volume to a running or stopped instance and exposes it to the instance with the specified device
* name.
*
*
* Encrypted EBS volumes must be attached to instances that support Amazon EBS encryption. For more information, see
* Amazon EBS Encryption in the
* Amazon Elastic Compute Cloud User Guide.
*
*
* After you attach an EBS volume, you must make it available. For more information, see Making an EBS Volume Available
* For Use.
*
*
* If a volume has an AWS Marketplace product code:
*
*
* -
*
* The volume can be attached only to a stopped instance.
*
*
* -
*
* AWS Marketplace product codes are copied from the volume to the instance.
*
*
* -
*
* You must be subscribed to the product.
*
*
* -
*
* The instance type and operating system of the instance must support the product. For example, you can't detach a
* volume from a Windows instance and attach it to a Linux instance.
*
*
*
*
* For more information, see Attaching Amazon EBS
* Volumes in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param attachVolumeRequest
* Contains the parameters for AttachVolume.
* @return A Java Future containing the result of the AttachVolume operation returned by the service.
* @sample AmazonEC2Async.AttachVolume
* @see AWS API
* Documentation
*/
java.util.concurrent.Future attachVolumeAsync(AttachVolumeRequest attachVolumeRequest);
/**
*
* Attaches an EBS volume to a running or stopped instance and exposes it to the instance with the specified device
* name.
*
*
* Encrypted EBS volumes must be attached to instances that support Amazon EBS encryption. For more information, see
* Amazon EBS Encryption in the
* Amazon Elastic Compute Cloud User Guide.
*
*
* After you attach an EBS volume, you must make it available. For more information, see Making an EBS Volume Available
* For Use.
*
*
* If a volume has an AWS Marketplace product code:
*
*
* -
*
* The volume can be attached only to a stopped instance.
*
*
* -
*
* AWS Marketplace product codes are copied from the volume to the instance.
*
*
* -
*
* You must be subscribed to the product.
*
*
* -
*
* The instance type and operating system of the instance must support the product. For example, you can't detach a
* volume from a Windows instance and attach it to a Linux instance.
*
*
*
*
* For more information, see Attaching Amazon EBS
* Volumes in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param attachVolumeRequest
* Contains the parameters for AttachVolume.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AttachVolume operation returned by the service.
* @sample AmazonEC2AsyncHandler.AttachVolume
* @see AWS API
* Documentation
*/
java.util.concurrent.Future attachVolumeAsync(AttachVolumeRequest attachVolumeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Attaches a virtual private gateway to a VPC. You can attach one virtual private gateway to one VPC at a time.
*
*
* For more information, see AWS Site-to-Site
* VPN in the AWS Site-to-Site VPN User Guide.
*
*
* @param attachVpnGatewayRequest
* Contains the parameters for AttachVpnGateway.
* @return A Java Future containing the result of the AttachVpnGateway operation returned by the service.
* @sample AmazonEC2Async.AttachVpnGateway
* @see AWS API
* Documentation
*/
java.util.concurrent.Future attachVpnGatewayAsync(AttachVpnGatewayRequest attachVpnGatewayRequest);
/**
*
* Attaches a virtual private gateway to a VPC. You can attach one virtual private gateway to one VPC at a time.
*
*
* For more information, see AWS Site-to-Site
* VPN in the AWS Site-to-Site VPN User Guide.
*
*
* @param attachVpnGatewayRequest
* Contains the parameters for AttachVpnGateway.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AttachVpnGateway operation returned by the service.
* @sample AmazonEC2AsyncHandler.AttachVpnGateway
* @see AWS API
* Documentation
*/
java.util.concurrent.Future attachVpnGatewayAsync(AttachVpnGatewayRequest attachVpnGatewayRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds an ingress authorization rule to a Client VPN endpoint. Ingress authorization rules act as firewall rules
* that grant access to networks. You must configure ingress authorization rules to enable clients to access
* resources in AWS or on-premises networks.
*
*
* @param authorizeClientVpnIngressRequest
* @return A Java Future containing the result of the AuthorizeClientVpnIngress operation returned by the service.
* @sample AmazonEC2Async.AuthorizeClientVpnIngress
* @see AWS
* API Documentation
*/
java.util.concurrent.Future authorizeClientVpnIngressAsync(
AuthorizeClientVpnIngressRequest authorizeClientVpnIngressRequest);
/**
*
* Adds an ingress authorization rule to a Client VPN endpoint. Ingress authorization rules act as firewall rules
* that grant access to networks. You must configure ingress authorization rules to enable clients to access
* resources in AWS or on-premises networks.
*
*
* @param authorizeClientVpnIngressRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AuthorizeClientVpnIngress operation returned by the service.
* @sample AmazonEC2AsyncHandler.AuthorizeClientVpnIngress
* @see AWS
* API Documentation
*/
java.util.concurrent.Future authorizeClientVpnIngressAsync(
AuthorizeClientVpnIngressRequest authorizeClientVpnIngressRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* [VPC only] Adds the specified egress rules to a security group for use with a VPC.
*
*
* An outbound rule permits instances to send traffic to the specified IPv4 or IPv6 CIDR address ranges, or to the
* instances associated with the specified destination security groups.
*
*
* You specify a protocol for each rule (for example, TCP). For the TCP and UDP protocols, you must also specify the
* destination port or port range. For the ICMP protocol, you must also specify the ICMP type and code. You can use
* -1 for the type or code to mean all types or all codes.
*
*
* Rule changes are propagated to affected instances as quickly as possible. However, a small delay might occur.
*
*
* For more information about VPC security group limits, see Amazon VPC Limits.
*
*
* @param authorizeSecurityGroupEgressRequest
* @return A Java Future containing the result of the AuthorizeSecurityGroupEgress operation returned by the
* service.
* @sample AmazonEC2Async.AuthorizeSecurityGroupEgress
* @see AWS API Documentation
*/
java.util.concurrent.Future authorizeSecurityGroupEgressAsync(
AuthorizeSecurityGroupEgressRequest authorizeSecurityGroupEgressRequest);
/**
*
* [VPC only] Adds the specified egress rules to a security group for use with a VPC.
*
*
* An outbound rule permits instances to send traffic to the specified IPv4 or IPv6 CIDR address ranges, or to the
* instances associated with the specified destination security groups.
*
*
* You specify a protocol for each rule (for example, TCP). For the TCP and UDP protocols, you must also specify the
* destination port or port range. For the ICMP protocol, you must also specify the ICMP type and code. You can use
* -1 for the type or code to mean all types or all codes.
*
*
* Rule changes are propagated to affected instances as quickly as possible. However, a small delay might occur.
*
*
* For more information about VPC security group limits, see Amazon VPC Limits.
*
*
* @param authorizeSecurityGroupEgressRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AuthorizeSecurityGroupEgress operation returned by the
* service.
* @sample AmazonEC2AsyncHandler.AuthorizeSecurityGroupEgress
* @see AWS API Documentation
*/
java.util.concurrent.Future authorizeSecurityGroupEgressAsync(
AuthorizeSecurityGroupEgressRequest authorizeSecurityGroupEgressRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds the specified ingress rules to a security group.
*
*
* An inbound rule permits instances to receive traffic from the specified IPv4 or IPv6 CIDR address ranges, or from
* the instances associated with the specified destination security groups.
*
*
* You specify a protocol for each rule (for example, TCP). For TCP and UDP, you must also specify the destination
* port or port range. For ICMP/ICMPv6, you must also specify the ICMP/ICMPv6 type and code. You can use -1 to mean
* all types or all codes.
*
*
* Rule changes are propagated to instances within the security group as quickly as possible. However, a small delay
* might occur.
*
*
* For more information about VPC security group limits, see Amazon VPC Limits.
*
*
* @param authorizeSecurityGroupIngressRequest
* @return A Java Future containing the result of the AuthorizeSecurityGroupIngress operation returned by the
* service.
* @sample AmazonEC2Async.AuthorizeSecurityGroupIngress
* @see AWS API Documentation
*/
java.util.concurrent.Future authorizeSecurityGroupIngressAsync(
AuthorizeSecurityGroupIngressRequest authorizeSecurityGroupIngressRequest);
/**
*
* Adds the specified ingress rules to a security group.
*
*
* An inbound rule permits instances to receive traffic from the specified IPv4 or IPv6 CIDR address ranges, or from
* the instances associated with the specified destination security groups.
*
*
* You specify a protocol for each rule (for example, TCP). For TCP and UDP, you must also specify the destination
* port or port range. For ICMP/ICMPv6, you must also specify the ICMP/ICMPv6 type and code. You can use -1 to mean
* all types or all codes.
*
*
* Rule changes are propagated to instances within the security group as quickly as possible. However, a small delay
* might occur.
*
*
* For more information about VPC security group limits, see Amazon VPC Limits.
*
*
* @param authorizeSecurityGroupIngressRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AuthorizeSecurityGroupIngress operation returned by the
* service.
* @sample AmazonEC2AsyncHandler.AuthorizeSecurityGroupIngress
* @see AWS API Documentation
*/
java.util.concurrent.Future authorizeSecurityGroupIngressAsync(
AuthorizeSecurityGroupIngressRequest authorizeSecurityGroupIngressRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Bundles an Amazon instance store-backed Windows instance.
*
*
* During bundling, only the root device volume (C:\) is bundled. Data on other instance store volumes is not
* preserved.
*
*
*
* This action is not applicable for Linux/Unix instances or Windows instances that are backed by Amazon EBS.
*
*
*
* @param bundleInstanceRequest
* Contains the parameters for BundleInstance.
* @return A Java Future containing the result of the BundleInstance operation returned by the service.
* @sample AmazonEC2Async.BundleInstance
* @see AWS API
* Documentation
*/
java.util.concurrent.Future bundleInstanceAsync(BundleInstanceRequest bundleInstanceRequest);
/**
*
* Bundles an Amazon instance store-backed Windows instance.
*
*
* During bundling, only the root device volume (C:\) is bundled. Data on other instance store volumes is not
* preserved.
*
*
*
* This action is not applicable for Linux/Unix instances or Windows instances that are backed by Amazon EBS.
*
*
*
* @param bundleInstanceRequest
* Contains the parameters for BundleInstance.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BundleInstance operation returned by the service.
* @sample AmazonEC2AsyncHandler.BundleInstance
* @see AWS API
* Documentation
*/
java.util.concurrent.Future bundleInstanceAsync(BundleInstanceRequest bundleInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels a bundling operation for an instance store-backed Windows instance.
*
*
* @param cancelBundleTaskRequest
* Contains the parameters for CancelBundleTask.
* @return A Java Future containing the result of the CancelBundleTask operation returned by the service.
* @sample AmazonEC2Async.CancelBundleTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelBundleTaskAsync(CancelBundleTaskRequest cancelBundleTaskRequest);
/**
*
* Cancels a bundling operation for an instance store-backed Windows instance.
*
*
* @param cancelBundleTaskRequest
* Contains the parameters for CancelBundleTask.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelBundleTask operation returned by the service.
* @sample AmazonEC2AsyncHandler.CancelBundleTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelBundleTaskAsync(CancelBundleTaskRequest cancelBundleTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels the specified Capacity Reservation, releases the reserved capacity, and changes the Capacity
* Reservation's state to cancelled
.
*
*
* Instances running in the reserved capacity continue running until you stop them. Stopped instances that target
* the Capacity Reservation can no longer launch. Modify these instances to either target a different Capacity
* Reservation, launch On-Demand Instance capacity, or run in any open Capacity Reservation that has matching
* attributes and sufficient capacity.
*
*
* @param cancelCapacityReservationRequest
* @return A Java Future containing the result of the CancelCapacityReservation operation returned by the service.
* @sample AmazonEC2Async.CancelCapacityReservation
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelCapacityReservationAsync(
CancelCapacityReservationRequest cancelCapacityReservationRequest);
/**
*
* Cancels the specified Capacity Reservation, releases the reserved capacity, and changes the Capacity
* Reservation's state to cancelled
.
*
*
* Instances running in the reserved capacity continue running until you stop them. Stopped instances that target
* the Capacity Reservation can no longer launch. Modify these instances to either target a different Capacity
* Reservation, launch On-Demand Instance capacity, or run in any open Capacity Reservation that has matching
* attributes and sufficient capacity.
*
*
* @param cancelCapacityReservationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelCapacityReservation operation returned by the service.
* @sample AmazonEC2AsyncHandler.CancelCapacityReservation
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelCapacityReservationAsync(
CancelCapacityReservationRequest cancelCapacityReservationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels an active conversion task. The task can be the import of an instance or volume. The action removes all
* artifacts of the conversion, including a partially uploaded volume or instance. If the conversion is complete or
* is in the process of transferring the final disk image, the command fails and returns an exception.
*
*
* For more information, see Importing a
* Virtual Machine Using the Amazon EC2 CLI.
*
*
* @param cancelConversionTaskRequest
* @return A Java Future containing the result of the CancelConversionTask operation returned by the service.
* @sample AmazonEC2Async.CancelConversionTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelConversionTaskAsync(CancelConversionTaskRequest cancelConversionTaskRequest);
/**
*
* Cancels an active conversion task. The task can be the import of an instance or volume. The action removes all
* artifacts of the conversion, including a partially uploaded volume or instance. If the conversion is complete or
* is in the process of transferring the final disk image, the command fails and returns an exception.
*
*
* For more information, see Importing a
* Virtual Machine Using the Amazon EC2 CLI.
*
*
* @param cancelConversionTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelConversionTask operation returned by the service.
* @sample AmazonEC2AsyncHandler.CancelConversionTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelConversionTaskAsync(CancelConversionTaskRequest cancelConversionTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels an active export task. The request removes all artifacts of the export, including any partially-created
* Amazon S3 objects. If the export task is complete or is in the process of transferring the final disk image, the
* command fails and returns an error.
*
*
* @param cancelExportTaskRequest
* @return A Java Future containing the result of the CancelExportTask operation returned by the service.
* @sample AmazonEC2Async.CancelExportTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelExportTaskAsync(CancelExportTaskRequest cancelExportTaskRequest);
/**
*
* Cancels an active export task. The request removes all artifacts of the export, including any partially-created
* Amazon S3 objects. If the export task is complete or is in the process of transferring the final disk image, the
* command fails and returns an error.
*
*
* @param cancelExportTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelExportTask operation returned by the service.
* @sample AmazonEC2AsyncHandler.CancelExportTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelExportTaskAsync(CancelExportTaskRequest cancelExportTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels an in-process import virtual machine or import snapshot task.
*
*
* @param cancelImportTaskRequest
* @return A Java Future containing the result of the CancelImportTask operation returned by the service.
* @sample AmazonEC2Async.CancelImportTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelImportTaskAsync(CancelImportTaskRequest cancelImportTaskRequest);
/**
*
* Cancels an in-process import virtual machine or import snapshot task.
*
*
* @param cancelImportTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelImportTask operation returned by the service.
* @sample AmazonEC2AsyncHandler.CancelImportTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelImportTaskAsync(CancelImportTaskRequest cancelImportTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the CancelImportTask operation.
*
* @see #cancelImportTaskAsync(CancelImportTaskRequest)
*/
java.util.concurrent.Future cancelImportTaskAsync();
/**
* Simplified method form for invoking the CancelImportTask operation with an AsyncHandler.
*
* @see #cancelImportTaskAsync(CancelImportTaskRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future cancelImportTaskAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels the specified Reserved Instance listing in the Reserved Instance Marketplace.
*
*
* For more information, see Reserved Instance
* Marketplace in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param cancelReservedInstancesListingRequest
* Contains the parameters for CancelReservedInstancesListing.
* @return A Java Future containing the result of the CancelReservedInstancesListing operation returned by the
* service.
* @sample AmazonEC2Async.CancelReservedInstancesListing
* @see AWS API Documentation
*/
java.util.concurrent.Future cancelReservedInstancesListingAsync(
CancelReservedInstancesListingRequest cancelReservedInstancesListingRequest);
/**
*
* Cancels the specified Reserved Instance listing in the Reserved Instance Marketplace.
*
*
* For more information, see Reserved Instance
* Marketplace in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param cancelReservedInstancesListingRequest
* Contains the parameters for CancelReservedInstancesListing.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelReservedInstancesListing operation returned by the
* service.
* @sample AmazonEC2AsyncHandler.CancelReservedInstancesListing
* @see AWS API Documentation
*/
java.util.concurrent.Future cancelReservedInstancesListingAsync(
CancelReservedInstancesListingRequest cancelReservedInstancesListingRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels the specified Spot Fleet requests.
*
*
* After you cancel a Spot Fleet request, the Spot Fleet launches no new Spot Instances. You must specify whether
* the Spot Fleet should also terminate its Spot Instances. If you terminate the instances, the Spot Fleet request
* enters the cancelled_terminating
state. Otherwise, the Spot Fleet request enters the
* cancelled_running
state and the instances continue to run until they are interrupted or you
* terminate them manually.
*
*
* @param cancelSpotFleetRequestsRequest
* Contains the parameters for CancelSpotFleetRequests.
* @return A Java Future containing the result of the CancelSpotFleetRequests operation returned by the service.
* @sample AmazonEC2Async.CancelSpotFleetRequests
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelSpotFleetRequestsAsync(CancelSpotFleetRequestsRequest cancelSpotFleetRequestsRequest);
/**
*
* Cancels the specified Spot Fleet requests.
*
*
* After you cancel a Spot Fleet request, the Spot Fleet launches no new Spot Instances. You must specify whether
* the Spot Fleet should also terminate its Spot Instances. If you terminate the instances, the Spot Fleet request
* enters the cancelled_terminating
state. Otherwise, the Spot Fleet request enters the
* cancelled_running
state and the instances continue to run until they are interrupted or you
* terminate them manually.
*
*
* @param cancelSpotFleetRequestsRequest
* Contains the parameters for CancelSpotFleetRequests.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelSpotFleetRequests operation returned by the service.
* @sample AmazonEC2AsyncHandler.CancelSpotFleetRequests
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelSpotFleetRequestsAsync(CancelSpotFleetRequestsRequest cancelSpotFleetRequestsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels one or more Spot Instance requests.
*
*
*
* Canceling a Spot Instance request does not terminate running Spot Instances associated with the request.
*
*
*
* @param cancelSpotInstanceRequestsRequest
* Contains the parameters for CancelSpotInstanceRequests.
* @return A Java Future containing the result of the CancelSpotInstanceRequests operation returned by the service.
* @sample AmazonEC2Async.CancelSpotInstanceRequests
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelSpotInstanceRequestsAsync(
CancelSpotInstanceRequestsRequest cancelSpotInstanceRequestsRequest);
/**
*
* Cancels one or more Spot Instance requests.
*
*
*
* Canceling a Spot Instance request does not terminate running Spot Instances associated with the request.
*
*
*
* @param cancelSpotInstanceRequestsRequest
* Contains the parameters for CancelSpotInstanceRequests.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelSpotInstanceRequests operation returned by the service.
* @sample AmazonEC2AsyncHandler.CancelSpotInstanceRequests
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelSpotInstanceRequestsAsync(
CancelSpotInstanceRequestsRequest cancelSpotInstanceRequestsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Determines whether a product code is associated with an instance. This action can only be used by the owner of
* the product code. It is useful when a product code owner must verify whether another user's instance is eligible
* for support.
*
*
* @param confirmProductInstanceRequest
* @return A Java Future containing the result of the ConfirmProductInstance operation returned by the service.
* @sample AmazonEC2Async.ConfirmProductInstance
* @see AWS API
* Documentation
*/
java.util.concurrent.Future confirmProductInstanceAsync(ConfirmProductInstanceRequest confirmProductInstanceRequest);
/**
*
* Determines whether a product code is associated with an instance. This action can only be used by the owner of
* the product code. It is useful when a product code owner must verify whether another user's instance is eligible
* for support.
*
*
* @param confirmProductInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ConfirmProductInstance operation returned by the service.
* @sample AmazonEC2AsyncHandler.ConfirmProductInstance
* @see AWS API
* Documentation
*/
java.util.concurrent.Future confirmProductInstanceAsync(ConfirmProductInstanceRequest confirmProductInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Copies the specified Amazon FPGA Image (AFI) to the current Region.
*
*
* @param copyFpgaImageRequest
* @return A Java Future containing the result of the CopyFpgaImage operation returned by the service.
* @sample AmazonEC2Async.CopyFpgaImage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future copyFpgaImageAsync(CopyFpgaImageRequest copyFpgaImageRequest);
/**
*
* Copies the specified Amazon FPGA Image (AFI) to the current Region.
*
*
* @param copyFpgaImageRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CopyFpgaImage operation returned by the service.
* @sample AmazonEC2AsyncHandler.CopyFpgaImage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future copyFpgaImageAsync(CopyFpgaImageRequest copyFpgaImageRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Initiates the copy of an AMI from the specified source Region to the current Region. You specify the destination
* Region by using its endpoint when making the request.
*
*
* Copies of encrypted backing snapshots for the AMI are encrypted. Copies of unencrypted backing snapshots remain
* unencrypted, unless you set Encrypted
during the copy operation. You cannot create an unencrypted
* copy of an encrypted backing snapshot.
*
*
* For more information about the prerequisites and limits when copying an AMI, see Copying an AMI in the Amazon
* Elastic Compute Cloud User Guide.
*
*
* @param copyImageRequest
* Contains the parameters for CopyImage.
* @return A Java Future containing the result of the CopyImage operation returned by the service.
* @sample AmazonEC2Async.CopyImage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future copyImageAsync(CopyImageRequest copyImageRequest);
/**
*
* Initiates the copy of an AMI from the specified source Region to the current Region. You specify the destination
* Region by using its endpoint when making the request.
*
*
* Copies of encrypted backing snapshots for the AMI are encrypted. Copies of unencrypted backing snapshots remain
* unencrypted, unless you set Encrypted
during the copy operation. You cannot create an unencrypted
* copy of an encrypted backing snapshot.
*
*
* For more information about the prerequisites and limits when copying an AMI, see Copying an AMI in the Amazon
* Elastic Compute Cloud User Guide.
*
*
* @param copyImageRequest
* Contains the parameters for CopyImage.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CopyImage operation returned by the service.
* @sample AmazonEC2AsyncHandler.CopyImage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future copyImageAsync(CopyImageRequest copyImageRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Copies a point-in-time snapshot of an EBS volume and stores it in Amazon S3. You can copy the snapshot within the
* same Region or from one Region to another. You can use the snapshot to create EBS volumes or Amazon Machine
* Images (AMIs).
*
*
* Copies of encrypted EBS snapshots remain encrypted. Copies of unencrypted snapshots remain unencrypted, unless
* you enable encryption for the snapshot copy operation. By default, encrypted snapshot copies use the default AWS
* Key Management Service (AWS KMS) customer master key (CMK); however, you can specify a different CMK.
*
*
* To copy an encrypted snapshot that has been shared from another account, you must have permissions for the CMK
* used to encrypt the snapshot.
*
*
* Snapshots created by copying another snapshot have an arbitrary volume ID that should not be used for any
* purpose.
*
*
* For more information, see Copying an Amazon EBS
* Snapshot in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param copySnapshotRequest
* Contains the parameters for CopySnapshot.
* @return A Java Future containing the result of the CopySnapshot operation returned by the service.
* @sample AmazonEC2Async.CopySnapshot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future copySnapshotAsync(CopySnapshotRequest copySnapshotRequest);
/**
*
* Copies a point-in-time snapshot of an EBS volume and stores it in Amazon S3. You can copy the snapshot within the
* same Region or from one Region to another. You can use the snapshot to create EBS volumes or Amazon Machine
* Images (AMIs).
*
*
* Copies of encrypted EBS snapshots remain encrypted. Copies of unencrypted snapshots remain unencrypted, unless
* you enable encryption for the snapshot copy operation. By default, encrypted snapshot copies use the default AWS
* Key Management Service (AWS KMS) customer master key (CMK); however, you can specify a different CMK.
*
*
* To copy an encrypted snapshot that has been shared from another account, you must have permissions for the CMK
* used to encrypt the snapshot.
*
*
* Snapshots created by copying another snapshot have an arbitrary volume ID that should not be used for any
* purpose.
*
*
* For more information, see Copying an Amazon EBS
* Snapshot in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param copySnapshotRequest
* Contains the parameters for CopySnapshot.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CopySnapshot operation returned by the service.
* @sample AmazonEC2AsyncHandler.CopySnapshot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future copySnapshotAsync(CopySnapshotRequest copySnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new Capacity Reservation with the specified attributes.
*
*
* Capacity Reservations enable you to reserve capacity for your Amazon EC2 instances in a specific Availability
* Zone for any duration. This gives you the flexibility to selectively add capacity reservations and still get the
* Regional RI discounts for that usage. By creating Capacity Reservations, you ensure that you always have access
* to Amazon EC2 capacity when you need it, for as long as you need it. For more information, see Capacity
* Reservations in the Amazon Elastic Compute Cloud User Guide.
*
*
* Your request to create a Capacity Reservation could fail if Amazon EC2 does not have sufficient capacity to
* fulfill the request. If your request fails due to Amazon EC2 capacity constraints, either try again at a later
* time, try in a different Availability Zone, or request a smaller capacity reservation. If your application is
* flexible across instance types and sizes, try to create a Capacity Reservation with different instance
* attributes.
*
*
* Your request could also fail if the requested quantity exceeds your On-Demand Instance limit for the selected
* instance type. If your request fails due to limit constraints, increase your On-Demand Instance limit for the
* required instance type and try again. For more information about increasing your instance limits, see Amazon EC2 Service Limits
* in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param createCapacityReservationRequest
* @return A Java Future containing the result of the CreateCapacityReservation operation returned by the service.
* @sample AmazonEC2Async.CreateCapacityReservation
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createCapacityReservationAsync(
CreateCapacityReservationRequest createCapacityReservationRequest);
/**
*
* Creates a new Capacity Reservation with the specified attributes.
*
*
* Capacity Reservations enable you to reserve capacity for your Amazon EC2 instances in a specific Availability
* Zone for any duration. This gives you the flexibility to selectively add capacity reservations and still get the
* Regional RI discounts for that usage. By creating Capacity Reservations, you ensure that you always have access
* to Amazon EC2 capacity when you need it, for as long as you need it. For more information, see Capacity
* Reservations in the Amazon Elastic Compute Cloud User Guide.
*
*
* Your request to create a Capacity Reservation could fail if Amazon EC2 does not have sufficient capacity to
* fulfill the request. If your request fails due to Amazon EC2 capacity constraints, either try again at a later
* time, try in a different Availability Zone, or request a smaller capacity reservation. If your application is
* flexible across instance types and sizes, try to create a Capacity Reservation with different instance
* attributes.
*
*
* Your request could also fail if the requested quantity exceeds your On-Demand Instance limit for the selected
* instance type. If your request fails due to limit constraints, increase your On-Demand Instance limit for the
* required instance type and try again. For more information about increasing your instance limits, see Amazon EC2 Service Limits
* in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param createCapacityReservationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateCapacityReservation operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateCapacityReservation
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createCapacityReservationAsync(
CreateCapacityReservationRequest createCapacityReservationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a Client VPN endpoint. A Client VPN endpoint is the resource you create and configure to enable and
* manage client VPN sessions. It is the destination endpoint at which all client VPN sessions are terminated.
*
*
* @param createClientVpnEndpointRequest
* @return A Java Future containing the result of the CreateClientVpnEndpoint operation returned by the service.
* @sample AmazonEC2Async.CreateClientVpnEndpoint
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createClientVpnEndpointAsync(CreateClientVpnEndpointRequest createClientVpnEndpointRequest);
/**
*
* Creates a Client VPN endpoint. A Client VPN endpoint is the resource you create and configure to enable and
* manage client VPN sessions. It is the destination endpoint at which all client VPN sessions are terminated.
*
*
* @param createClientVpnEndpointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateClientVpnEndpoint operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateClientVpnEndpoint
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createClientVpnEndpointAsync(CreateClientVpnEndpointRequest createClientVpnEndpointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds a route to a network to a Client VPN endpoint. Each Client VPN endpoint has a route table that describes the
* available destination network routes. Each route in the route table specifies the path for traffic to specific
* resources or networks.
*
*
* @param createClientVpnRouteRequest
* @return A Java Future containing the result of the CreateClientVpnRoute operation returned by the service.
* @sample AmazonEC2Async.CreateClientVpnRoute
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createClientVpnRouteAsync(CreateClientVpnRouteRequest createClientVpnRouteRequest);
/**
*
* Adds a route to a network to a Client VPN endpoint. Each Client VPN endpoint has a route table that describes the
* available destination network routes. Each route in the route table specifies the path for traffic to specific
* resources or networks.
*
*
* @param createClientVpnRouteRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateClientVpnRoute operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateClientVpnRoute
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createClientVpnRouteAsync(CreateClientVpnRouteRequest createClientVpnRouteRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides information to AWS about your VPN customer gateway device. The customer gateway is the appliance at your
* end of the VPN connection. (The device on the AWS side of the VPN connection is the virtual private gateway.) You
* must provide the Internet-routable IP address of the customer gateway's external interface. The IP address must
* be static and can be behind a device performing network address translation (NAT).
*
*
* For devices that use Border Gateway Protocol (BGP), you can also provide the device's BGP Autonomous System
* Number (ASN). You can use an existing ASN assigned to your network. If you don't have an ASN already, you can use
* a private ASN (in the 64512 - 65534 range).
*
*
*
* Amazon EC2 supports all 2-byte ASN numbers in the range of 1 - 65534, with the exception of 7224, which is
* reserved in the us-east-1
Region, and 9059, which is reserved in the eu-west-1
Region.
*
*
*
* For more information, see AWS Site-to-Site
* VPN in the AWS Site-to-Site VPN User Guide.
*
*
*
* You cannot create more than one customer gateway with the same VPN type, IP address, and BGP ASN parameter
* values. If you run an identical request more than one time, the first request creates the customer gateway, and
* subsequent requests return information about the existing customer gateway. The subsequent requests do not create
* new customer gateway resources.
*
*
*
* @param createCustomerGatewayRequest
* Contains the parameters for CreateCustomerGateway.
* @return A Java Future containing the result of the CreateCustomerGateway operation returned by the service.
* @sample AmazonEC2Async.CreateCustomerGateway
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createCustomerGatewayAsync(CreateCustomerGatewayRequest createCustomerGatewayRequest);
/**
*
* Provides information to AWS about your VPN customer gateway device. The customer gateway is the appliance at your
* end of the VPN connection. (The device on the AWS side of the VPN connection is the virtual private gateway.) You
* must provide the Internet-routable IP address of the customer gateway's external interface. The IP address must
* be static and can be behind a device performing network address translation (NAT).
*
*
* For devices that use Border Gateway Protocol (BGP), you can also provide the device's BGP Autonomous System
* Number (ASN). You can use an existing ASN assigned to your network. If you don't have an ASN already, you can use
* a private ASN (in the 64512 - 65534 range).
*
*
*
* Amazon EC2 supports all 2-byte ASN numbers in the range of 1 - 65534, with the exception of 7224, which is
* reserved in the us-east-1
Region, and 9059, which is reserved in the eu-west-1
Region.
*
*
*
* For more information, see AWS Site-to-Site
* VPN in the AWS Site-to-Site VPN User Guide.
*
*
*
* You cannot create more than one customer gateway with the same VPN type, IP address, and BGP ASN parameter
* values. If you run an identical request more than one time, the first request creates the customer gateway, and
* subsequent requests return information about the existing customer gateway. The subsequent requests do not create
* new customer gateway resources.
*
*
*
* @param createCustomerGatewayRequest
* Contains the parameters for CreateCustomerGateway.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateCustomerGateway operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateCustomerGateway
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createCustomerGatewayAsync(CreateCustomerGatewayRequest createCustomerGatewayRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a default subnet with a size /20
IPv4 CIDR block in the specified Availability Zone in your
* default VPC. You can have only one default subnet per Availability Zone. For more information, see Creating a Default
* Subnet in the Amazon Virtual Private Cloud User Guide.
*
*
* @param createDefaultSubnetRequest
* @return A Java Future containing the result of the CreateDefaultSubnet operation returned by the service.
* @sample AmazonEC2Async.CreateDefaultSubnet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDefaultSubnetAsync(CreateDefaultSubnetRequest createDefaultSubnetRequest);
/**
*
* Creates a default subnet with a size /20
IPv4 CIDR block in the specified Availability Zone in your
* default VPC. You can have only one default subnet per Availability Zone. For more information, see Creating a Default
* Subnet in the Amazon Virtual Private Cloud User Guide.
*
*
* @param createDefaultSubnetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDefaultSubnet operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateDefaultSubnet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDefaultSubnetAsync(CreateDefaultSubnetRequest createDefaultSubnetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a default VPC with a size /16
IPv4 CIDR block and a default subnet in each Availability
* Zone. For more information about the components of a default VPC, see Default VPC and Default Subnets in
* the Amazon Virtual Private Cloud User Guide. You cannot specify the components of the default VPC
* yourself.
*
*
* If you deleted your previous default VPC, you can create a default VPC. You cannot have more than one default VPC
* per Region.
*
*
* If your account supports EC2-Classic, you cannot use this action to create a default VPC in a Region that
* supports EC2-Classic. If you want a default VPC in a Region that supports EC2-Classic, see
* "I really want a default VPC for my existing EC2 account. Is that possible?" in the Default VPCs FAQ.
*
*
* @param createDefaultVpcRequest
* @return A Java Future containing the result of the CreateDefaultVpc operation returned by the service.
* @sample AmazonEC2Async.CreateDefaultVpc
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDefaultVpcAsync(CreateDefaultVpcRequest createDefaultVpcRequest);
/**
*
* Creates a default VPC with a size /16
IPv4 CIDR block and a default subnet in each Availability
* Zone. For more information about the components of a default VPC, see Default VPC and Default Subnets in
* the Amazon Virtual Private Cloud User Guide. You cannot specify the components of the default VPC
* yourself.
*
*
* If you deleted your previous default VPC, you can create a default VPC. You cannot have more than one default VPC
* per Region.
*
*
* If your account supports EC2-Classic, you cannot use this action to create a default VPC in a Region that
* supports EC2-Classic. If you want a default VPC in a Region that supports EC2-Classic, see
* "I really want a default VPC for my existing EC2 account. Is that possible?" in the Default VPCs FAQ.
*
*
* @param createDefaultVpcRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDefaultVpc operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateDefaultVpc
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDefaultVpcAsync(CreateDefaultVpcRequest createDefaultVpcRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a set of DHCP options for your VPC. After creating the set, you must associate it with the VPC, causing
* all existing and new instances that you launch in the VPC to use this set of DHCP options. The following are the
* individual DHCP options you can specify. For more information about the options, see RFC 2132.
*
*
* -
*
* domain-name-servers
- The IP addresses of up to four domain name servers, or AmazonProvidedDNS. The
* default DHCP option set specifies AmazonProvidedDNS. If specifying more than one domain name server, specify the
* IP addresses in a single parameter, separated by commas. To have your instance receive a custom DNS hostname as
* specified in domain-name
, you must set domain-name-servers
to a custom DNS server.
*
*
* -
*
* domain-name
- If you're using AmazonProvidedDNS in us-east-1
, specify
* ec2.internal
. If you're using AmazonProvidedDNS in another Region, specify
* region.compute.internal
(for example, ap-northeast-1.compute.internal
). Otherwise,
* specify a domain name (for example, MyCompany.com
). This value is used to complete unqualified DNS
* hostnames. Important: Some Linux operating systems accept multiple domain names separated by spaces.
* However, Windows and other Linux operating systems treat the value as a single domain, which results in
* unexpected behavior. If your DHCP options set is associated with a VPC that has instances with multiple operating
* systems, specify only one domain name.
*
*
* -
*
* ntp-servers
- The IP addresses of up to four Network Time Protocol (NTP) servers.
*
*
* -
*
* netbios-name-servers
- The IP addresses of up to four NetBIOS name servers.
*
*
* -
*
* netbios-node-type
- The NetBIOS node type (1, 2, 4, or 8). We recommend that you specify 2
* (broadcast and multicast are not currently supported). For more information about these node types, see RFC 2132.
*
*
*
*
* Your VPC automatically starts out with a set of DHCP options that includes only a DNS server that we provide
* (AmazonProvidedDNS). If you create a set of options, and if your VPC has an internet gateway, make sure to set
* the domain-name-servers
option either to AmazonProvidedDNS
or to a domain name server
* of your choice. For more information, see DHCP Options Sets in the
* Amazon Virtual Private Cloud User Guide.
*
*
* @param createDhcpOptionsRequest
* @return A Java Future containing the result of the CreateDhcpOptions operation returned by the service.
* @sample AmazonEC2Async.CreateDhcpOptions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDhcpOptionsAsync(CreateDhcpOptionsRequest createDhcpOptionsRequest);
/**
*
* Creates a set of DHCP options for your VPC. After creating the set, you must associate it with the VPC, causing
* all existing and new instances that you launch in the VPC to use this set of DHCP options. The following are the
* individual DHCP options you can specify. For more information about the options, see RFC 2132.
*
*
* -
*
* domain-name-servers
- The IP addresses of up to four domain name servers, or AmazonProvidedDNS. The
* default DHCP option set specifies AmazonProvidedDNS. If specifying more than one domain name server, specify the
* IP addresses in a single parameter, separated by commas. To have your instance receive a custom DNS hostname as
* specified in domain-name
, you must set domain-name-servers
to a custom DNS server.
*
*
* -
*
* domain-name
- If you're using AmazonProvidedDNS in us-east-1
, specify
* ec2.internal
. If you're using AmazonProvidedDNS in another Region, specify
* region.compute.internal
(for example, ap-northeast-1.compute.internal
). Otherwise,
* specify a domain name (for example, MyCompany.com
). This value is used to complete unqualified DNS
* hostnames. Important: Some Linux operating systems accept multiple domain names separated by spaces.
* However, Windows and other Linux operating systems treat the value as a single domain, which results in
* unexpected behavior. If your DHCP options set is associated with a VPC that has instances with multiple operating
* systems, specify only one domain name.
*
*
* -
*
* ntp-servers
- The IP addresses of up to four Network Time Protocol (NTP) servers.
*
*
* -
*
* netbios-name-servers
- The IP addresses of up to four NetBIOS name servers.
*
*
* -
*
* netbios-node-type
- The NetBIOS node type (1, 2, 4, or 8). We recommend that you specify 2
* (broadcast and multicast are not currently supported). For more information about these node types, see RFC 2132.
*
*
*
*
* Your VPC automatically starts out with a set of DHCP options that includes only a DNS server that we provide
* (AmazonProvidedDNS). If you create a set of options, and if your VPC has an internet gateway, make sure to set
* the domain-name-servers
option either to AmazonProvidedDNS
or to a domain name server
* of your choice. For more information, see DHCP Options Sets in the
* Amazon Virtual Private Cloud User Guide.
*
*
* @param createDhcpOptionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDhcpOptions operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateDhcpOptions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDhcpOptionsAsync(CreateDhcpOptionsRequest createDhcpOptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* [IPv6 only] Creates an egress-only internet gateway for your VPC. An egress-only internet gateway is used to
* enable outbound communication over IPv6 from instances in your VPC to the internet, and prevents hosts outside of
* your VPC from initiating an IPv6 connection with your instance.
*
*
* @param createEgressOnlyInternetGatewayRequest
* @return A Java Future containing the result of the CreateEgressOnlyInternetGateway operation returned by the
* service.
* @sample AmazonEC2Async.CreateEgressOnlyInternetGateway
* @see AWS API Documentation
*/
java.util.concurrent.Future createEgressOnlyInternetGatewayAsync(
CreateEgressOnlyInternetGatewayRequest createEgressOnlyInternetGatewayRequest);
/**
*
* [IPv6 only] Creates an egress-only internet gateway for your VPC. An egress-only internet gateway is used to
* enable outbound communication over IPv6 from instances in your VPC to the internet, and prevents hosts outside of
* your VPC from initiating an IPv6 connection with your instance.
*
*
* @param createEgressOnlyInternetGatewayRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateEgressOnlyInternetGateway operation returned by the
* service.
* @sample AmazonEC2AsyncHandler.CreateEgressOnlyInternetGateway
* @see AWS API Documentation
*/
java.util.concurrent.Future createEgressOnlyInternetGatewayAsync(
CreateEgressOnlyInternetGatewayRequest createEgressOnlyInternetGatewayRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Launches an EC2 Fleet.
*
*
* You can create a single EC2 Fleet that includes multiple launch specifications that vary by instance type, AMI,
* Availability Zone, or subnet.
*
*
* For more information, see Launching
* an EC2 Fleet in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param createFleetRequest
* @return A Java Future containing the result of the CreateFleet operation returned by the service.
* @sample AmazonEC2Async.CreateFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFleetAsync(CreateFleetRequest createFleetRequest);
/**
*
* Launches an EC2 Fleet.
*
*
* You can create a single EC2 Fleet that includes multiple launch specifications that vary by instance type, AMI,
* Availability Zone, or subnet.
*
*
* For more information, see Launching
* an EC2 Fleet in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param createFleetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateFleet operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFleetAsync(CreateFleetRequest createFleetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates one or more flow logs to capture information about IP traffic for a specific network interface, subnet,
* or VPC.
*
*
* Flow log data for a monitored network interface is recorded as flow log records, which are log events consisting
* of fields that describe the traffic flow. For more information, see Flow Log Records in
* the Amazon Virtual Private Cloud User Guide.
*
*
* When publishing to CloudWatch Logs, flow log records are published to a log group, and each network interface has
* a unique log stream in the log group. When publishing to Amazon S3, flow log records for all of the monitored
* network interfaces are published to a single log file object that is stored in the specified bucket.
*
*
* For more information, see VPC Flow
* Logs in the Amazon Virtual Private Cloud User Guide.
*
*
* @param createFlowLogsRequest
* @return A Java Future containing the result of the CreateFlowLogs operation returned by the service.
* @sample AmazonEC2Async.CreateFlowLogs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFlowLogsAsync(CreateFlowLogsRequest createFlowLogsRequest);
/**
*
* Creates one or more flow logs to capture information about IP traffic for a specific network interface, subnet,
* or VPC.
*
*
* Flow log data for a monitored network interface is recorded as flow log records, which are log events consisting
* of fields that describe the traffic flow. For more information, see Flow Log Records in
* the Amazon Virtual Private Cloud User Guide.
*
*
* When publishing to CloudWatch Logs, flow log records are published to a log group, and each network interface has
* a unique log stream in the log group. When publishing to Amazon S3, flow log records for all of the monitored
* network interfaces are published to a single log file object that is stored in the specified bucket.
*
*
* For more information, see VPC Flow
* Logs in the Amazon Virtual Private Cloud User Guide.
*
*
* @param createFlowLogsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateFlowLogs operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateFlowLogs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFlowLogsAsync(CreateFlowLogsRequest createFlowLogsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Amazon FPGA Image (AFI) from the specified design checkpoint (DCP).
*
*
* The create operation is asynchronous. To verify that the AFI is ready for use, check the output logs.
*
*
* An AFI contains the FPGA bitstream that is ready to download to an FPGA. You can securely deploy an AFI on
* multiple FPGA-accelerated instances. For more information, see the AWS
* FPGA Hardware Development Kit.
*
*
* @param createFpgaImageRequest
* @return A Java Future containing the result of the CreateFpgaImage operation returned by the service.
* @sample AmazonEC2Async.CreateFpgaImage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFpgaImageAsync(CreateFpgaImageRequest createFpgaImageRequest);
/**
*
* Creates an Amazon FPGA Image (AFI) from the specified design checkpoint (DCP).
*
*
* The create operation is asynchronous. To verify that the AFI is ready for use, check the output logs.
*
*
* An AFI contains the FPGA bitstream that is ready to download to an FPGA. You can securely deploy an AFI on
* multiple FPGA-accelerated instances. For more information, see the AWS
* FPGA Hardware Development Kit.
*
*
* @param createFpgaImageRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateFpgaImage operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateFpgaImage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFpgaImageAsync(CreateFpgaImageRequest createFpgaImageRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Amazon EBS-backed AMI from an Amazon EBS-backed instance that is either running or stopped.
*
*
* If you customized your instance with instance store volumes or EBS volumes in addition to the root device volume,
* the new AMI contains block device mapping information for those volumes. When you launch an instance from this
* new AMI, the instance automatically launches with those additional volumes.
*
*
* For more information, see Creating Amazon EBS-Backed
* Linux AMIs in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param createImageRequest
* @return A Java Future containing the result of the CreateImage operation returned by the service.
* @sample AmazonEC2Async.CreateImage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createImageAsync(CreateImageRequest createImageRequest);
/**
*
* Creates an Amazon EBS-backed AMI from an Amazon EBS-backed instance that is either running or stopped.
*
*
* If you customized your instance with instance store volumes or EBS volumes in addition to the root device volume,
* the new AMI contains block device mapping information for those volumes. When you launch an instance from this
* new AMI, the instance automatically launches with those additional volumes.
*
*
* For more information, see Creating Amazon EBS-Backed
* Linux AMIs in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param createImageRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateImage operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateImage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createImageAsync(CreateImageRequest createImageRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Exports a running or stopped instance to an S3 bucket.
*
*
* For information about the supported operating systems, image formats, and known limitations for the types of
* instances you can export, see Exporting an Instance as a VM Using
* VM Import/Export in the VM Import/Export User Guide.
*
*
* @param createInstanceExportTaskRequest
* @return A Java Future containing the result of the CreateInstanceExportTask operation returned by the service.
* @sample AmazonEC2Async.CreateInstanceExportTask
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createInstanceExportTaskAsync(CreateInstanceExportTaskRequest createInstanceExportTaskRequest);
/**
*
* Exports a running or stopped instance to an S3 bucket.
*
*
* For information about the supported operating systems, image formats, and known limitations for the types of
* instances you can export, see Exporting an Instance as a VM Using
* VM Import/Export in the VM Import/Export User Guide.
*
*
* @param createInstanceExportTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateInstanceExportTask operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateInstanceExportTask
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createInstanceExportTaskAsync(CreateInstanceExportTaskRequest createInstanceExportTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an internet gateway for use with a VPC. After creating the internet gateway, you attach it to a VPC using
* AttachInternetGateway.
*
*
* For more information about your VPC and internet gateway, see the Amazon Virtual Private Cloud User Guide.
*
*
* @param createInternetGatewayRequest
* @return A Java Future containing the result of the CreateInternetGateway operation returned by the service.
* @sample AmazonEC2Async.CreateInternetGateway
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createInternetGatewayAsync(CreateInternetGatewayRequest createInternetGatewayRequest);
/**
*
* Creates an internet gateway for use with a VPC. After creating the internet gateway, you attach it to a VPC using
* AttachInternetGateway.
*
*
* For more information about your VPC and internet gateway, see the Amazon Virtual Private Cloud User Guide.
*
*
* @param createInternetGatewayRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateInternetGateway operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateInternetGateway
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createInternetGatewayAsync(CreateInternetGatewayRequest createInternetGatewayRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the CreateInternetGateway operation.
*
* @see #createInternetGatewayAsync(CreateInternetGatewayRequest)
*/
java.util.concurrent.Future createInternetGatewayAsync();
/**
* Simplified method form for invoking the CreateInternetGateway operation with an AsyncHandler.
*
* @see #createInternetGatewayAsync(CreateInternetGatewayRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future createInternetGatewayAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a 2048-bit RSA key pair with the specified name. Amazon EC2 stores the public key and displays the
* private key for you to save to a file. The private key is returned as an unencrypted PEM encoded PKCS#1 private
* key. If a key with the specified name already exists, Amazon EC2 returns an error.
*
*
* You can have up to five thousand key pairs per Region.
*
*
* The key pair returned to you is available only in the Region in which you create it. If you prefer, you can
* create your own key pair using a third-party tool and upload it to any Region using ImportKeyPair.
*
*
* For more information, see Key
* Pairs in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param createKeyPairRequest
* @return A Java Future containing the result of the CreateKeyPair operation returned by the service.
* @sample AmazonEC2Async.CreateKeyPair
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createKeyPairAsync(CreateKeyPairRequest createKeyPairRequest);
/**
*
* Creates a 2048-bit RSA key pair with the specified name. Amazon EC2 stores the public key and displays the
* private key for you to save to a file. The private key is returned as an unencrypted PEM encoded PKCS#1 private
* key. If a key with the specified name already exists, Amazon EC2 returns an error.
*
*
* You can have up to five thousand key pairs per Region.
*
*
* The key pair returned to you is available only in the Region in which you create it. If you prefer, you can
* create your own key pair using a third-party tool and upload it to any Region using ImportKeyPair.
*
*
* For more information, see Key
* Pairs in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param createKeyPairRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateKeyPair operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateKeyPair
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createKeyPairAsync(CreateKeyPairRequest createKeyPairRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a launch template. A launch template contains the parameters to launch an instance. When you launch an
* instance using RunInstances, you can specify a launch template instead of providing the launch parameters
* in the request.
*
*
* @param createLaunchTemplateRequest
* @return A Java Future containing the result of the CreateLaunchTemplate operation returned by the service.
* @sample AmazonEC2Async.CreateLaunchTemplate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createLaunchTemplateAsync(CreateLaunchTemplateRequest createLaunchTemplateRequest);
/**
*
* Creates a launch template. A launch template contains the parameters to launch an instance. When you launch an
* instance using RunInstances, you can specify a launch template instead of providing the launch parameters
* in the request.
*
*
* @param createLaunchTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateLaunchTemplate operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateLaunchTemplate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createLaunchTemplateAsync(CreateLaunchTemplateRequest createLaunchTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new version for a launch template. You can specify an existing version of launch template from which to
* base the new version.
*
*
* Launch template versions are numbered in the order in which they are created. You cannot specify, change, or
* replace the numbering of launch template versions.
*
*
* @param createLaunchTemplateVersionRequest
* @return A Java Future containing the result of the CreateLaunchTemplateVersion operation returned by the service.
* @sample AmazonEC2Async.CreateLaunchTemplateVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future createLaunchTemplateVersionAsync(
CreateLaunchTemplateVersionRequest createLaunchTemplateVersionRequest);
/**
*
* Creates a new version for a launch template. You can specify an existing version of launch template from which to
* base the new version.
*
*
* Launch template versions are numbered in the order in which they are created. You cannot specify, change, or
* replace the numbering of launch template versions.
*
*
* @param createLaunchTemplateVersionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateLaunchTemplateVersion operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateLaunchTemplateVersion
* @see AWS API Documentation
*/
java.util.concurrent.Future createLaunchTemplateVersionAsync(
CreateLaunchTemplateVersionRequest createLaunchTemplateVersionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a NAT gateway in the specified public subnet. This action creates a network interface in the specified
* subnet with a private IP address from the IP address range of the subnet. Internet-bound traffic from a private
* subnet can be routed to the NAT gateway, therefore enabling instances in the private subnet to connect to the
* internet. For more information, see NAT Gateways in the Amazon
* Virtual Private Cloud User Guide.
*
*
* @param createNatGatewayRequest
* @return A Java Future containing the result of the CreateNatGateway operation returned by the service.
* @sample AmazonEC2Async.CreateNatGateway
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createNatGatewayAsync(CreateNatGatewayRequest createNatGatewayRequest);
/**
*
* Creates a NAT gateway in the specified public subnet. This action creates a network interface in the specified
* subnet with a private IP address from the IP address range of the subnet. Internet-bound traffic from a private
* subnet can be routed to the NAT gateway, therefore enabling instances in the private subnet to connect to the
* internet. For more information, see NAT Gateways in the Amazon
* Virtual Private Cloud User Guide.
*
*
* @param createNatGatewayRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateNatGateway operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateNatGateway
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createNatGatewayAsync(CreateNatGatewayRequest createNatGatewayRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a network ACL in a VPC. Network ACLs provide an optional layer of security (in addition to security
* groups) for the instances in your VPC.
*
*
* For more information, see Network
* ACLs in the Amazon Virtual Private Cloud User Guide.
*
*
* @param createNetworkAclRequest
* @return A Java Future containing the result of the CreateNetworkAcl operation returned by the service.
* @sample AmazonEC2Async.CreateNetworkAcl
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createNetworkAclAsync(CreateNetworkAclRequest createNetworkAclRequest);
/**
*
* Creates a network ACL in a VPC. Network ACLs provide an optional layer of security (in addition to security
* groups) for the instances in your VPC.
*
*
* For more information, see Network
* ACLs in the Amazon Virtual Private Cloud User Guide.
*
*
* @param createNetworkAclRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateNetworkAcl operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateNetworkAcl
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createNetworkAclAsync(CreateNetworkAclRequest createNetworkAclRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an entry (a rule) in a network ACL with the specified rule number. Each network ACL has a set of numbered
* ingress rules and a separate set of numbered egress rules. When determining whether a packet should be allowed in
* or out of a subnet associated with the ACL, we process the entries in the ACL according to the rule numbers, in
* ascending order. Each network ACL has a set of ingress rules and a separate set of egress rules.
*
*
* We recommend that you leave room between the rule numbers (for example, 100, 110, 120, ...), and not number them
* one right after the other (for example, 101, 102, 103, ...). This makes it easier to add a rule between existing
* ones without having to renumber the rules.
*
*
* After you add an entry, you can't modify it; you must either replace it, or create an entry and delete the old
* one.
*
*
* For more information about network ACLs, see Network ACLs in the Amazon Virtual
* Private Cloud User Guide.
*
*
* @param createNetworkAclEntryRequest
* @return A Java Future containing the result of the CreateNetworkAclEntry operation returned by the service.
* @sample AmazonEC2Async.CreateNetworkAclEntry
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createNetworkAclEntryAsync(CreateNetworkAclEntryRequest createNetworkAclEntryRequest);
/**
*
* Creates an entry (a rule) in a network ACL with the specified rule number. Each network ACL has a set of numbered
* ingress rules and a separate set of numbered egress rules. When determining whether a packet should be allowed in
* or out of a subnet associated with the ACL, we process the entries in the ACL according to the rule numbers, in
* ascending order. Each network ACL has a set of ingress rules and a separate set of egress rules.
*
*
* We recommend that you leave room between the rule numbers (for example, 100, 110, 120, ...), and not number them
* one right after the other (for example, 101, 102, 103, ...). This makes it easier to add a rule between existing
* ones without having to renumber the rules.
*
*
* After you add an entry, you can't modify it; you must either replace it, or create an entry and delete the old
* one.
*
*
* For more information about network ACLs, see Network ACLs in the Amazon Virtual
* Private Cloud User Guide.
*
*
* @param createNetworkAclEntryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateNetworkAclEntry operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateNetworkAclEntry
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createNetworkAclEntryAsync(CreateNetworkAclEntryRequest createNetworkAclEntryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a network interface in the specified subnet.
*
*
* For more information about network interfaces, see Elastic Network Interfaces in the
* Amazon Virtual Private Cloud User Guide.
*
*
* @param createNetworkInterfaceRequest
* Contains the parameters for CreateNetworkInterface.
* @return A Java Future containing the result of the CreateNetworkInterface operation returned by the service.
* @sample AmazonEC2Async.CreateNetworkInterface
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createNetworkInterfaceAsync(CreateNetworkInterfaceRequest createNetworkInterfaceRequest);
/**
*
* Creates a network interface in the specified subnet.
*
*
* For more information about network interfaces, see Elastic Network Interfaces in the
* Amazon Virtual Private Cloud User Guide.
*
*
* @param createNetworkInterfaceRequest
* Contains the parameters for CreateNetworkInterface.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateNetworkInterface operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateNetworkInterface
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createNetworkInterfaceAsync(CreateNetworkInterfaceRequest createNetworkInterfaceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Grants an AWS-authorized account permission to attach the specified network interface to an instance in their
* account.
*
*
* You can grant permission to a single AWS account only, and only one account at a time.
*
*
* @param createNetworkInterfacePermissionRequest
* Contains the parameters for CreateNetworkInterfacePermission.
* @return A Java Future containing the result of the CreateNetworkInterfacePermission operation returned by the
* service.
* @sample AmazonEC2Async.CreateNetworkInterfacePermission
* @see AWS API Documentation
*/
java.util.concurrent.Future createNetworkInterfacePermissionAsync(
CreateNetworkInterfacePermissionRequest createNetworkInterfacePermissionRequest);
/**
*
* Grants an AWS-authorized account permission to attach the specified network interface to an instance in their
* account.
*
*
* You can grant permission to a single AWS account only, and only one account at a time.
*
*
* @param createNetworkInterfacePermissionRequest
* Contains the parameters for CreateNetworkInterfacePermission.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateNetworkInterfacePermission operation returned by the
* service.
* @sample AmazonEC2AsyncHandler.CreateNetworkInterfacePermission
* @see AWS API Documentation
*/
java.util.concurrent.Future createNetworkInterfacePermissionAsync(
CreateNetworkInterfacePermissionRequest createNetworkInterfacePermissionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a placement group in which to launch instances. The strategy of the placement group determines how the
* instances are organized within the group.
*
*
* A cluster
placement group is a logical grouping of instances within a single Availability Zone that
* benefit from low network latency, high network throughput. A spread
placement group places instances
* on distinct hardware. A partition
placement group places groups of instances in different
* partitions, where instances in one partition do not share the same hardware with instances in another partition.
*
*
* For more information, see Placement Groups in the
* Amazon Elastic Compute Cloud User Guide.
*
*
* @param createPlacementGroupRequest
* @return A Java Future containing the result of the CreatePlacementGroup operation returned by the service.
* @sample AmazonEC2Async.CreatePlacementGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createPlacementGroupAsync(CreatePlacementGroupRequest createPlacementGroupRequest);
/**
*
* Creates a placement group in which to launch instances. The strategy of the placement group determines how the
* instances are organized within the group.
*
*
* A cluster
placement group is a logical grouping of instances within a single Availability Zone that
* benefit from low network latency, high network throughput. A spread
placement group places instances
* on distinct hardware. A partition
placement group places groups of instances in different
* partitions, where instances in one partition do not share the same hardware with instances in another partition.
*
*
* For more information, see Placement Groups in the
* Amazon Elastic Compute Cloud User Guide.
*
*
* @param createPlacementGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreatePlacementGroup operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreatePlacementGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createPlacementGroupAsync(CreatePlacementGroupRequest createPlacementGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a listing for Amazon EC2 Standard Reserved Instances to be sold in the Reserved Instance Marketplace. You
* can submit one Standard Reserved Instance listing at a time. To get a list of your Standard Reserved Instances,
* you can use the DescribeReservedInstances operation.
*
*
*
* Only Standard Reserved Instances can be sold in the Reserved Instance Marketplace. Convertible Reserved Instances
* cannot be sold.
*
*
*
* The Reserved Instance Marketplace matches sellers who want to resell Standard Reserved Instance capacity that
* they no longer need with buyers who want to purchase additional capacity. Reserved Instances bought and sold
* through the Reserved Instance Marketplace work like any other Reserved Instances.
*
*
* To sell your Standard Reserved Instances, you must first register as a seller in the Reserved Instance
* Marketplace. After completing the registration process, you can create a Reserved Instance Marketplace listing of
* some or all of your Standard Reserved Instances, and specify the upfront price to receive for them. Your Standard
* Reserved Instance listings then become available for purchase. To view the details of your Standard Reserved
* Instance listing, you can use the DescribeReservedInstancesListings operation.
*
*
* For more information, see Reserved Instance
* Marketplace in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param createReservedInstancesListingRequest
* Contains the parameters for CreateReservedInstancesListing.
* @return A Java Future containing the result of the CreateReservedInstancesListing operation returned by the
* service.
* @sample AmazonEC2Async.CreateReservedInstancesListing
* @see AWS API Documentation
*/
java.util.concurrent.Future createReservedInstancesListingAsync(
CreateReservedInstancesListingRequest createReservedInstancesListingRequest);
/**
*
* Creates a listing for Amazon EC2 Standard Reserved Instances to be sold in the Reserved Instance Marketplace. You
* can submit one Standard Reserved Instance listing at a time. To get a list of your Standard Reserved Instances,
* you can use the DescribeReservedInstances operation.
*
*
*
* Only Standard Reserved Instances can be sold in the Reserved Instance Marketplace. Convertible Reserved Instances
* cannot be sold.
*
*
*
* The Reserved Instance Marketplace matches sellers who want to resell Standard Reserved Instance capacity that
* they no longer need with buyers who want to purchase additional capacity. Reserved Instances bought and sold
* through the Reserved Instance Marketplace work like any other Reserved Instances.
*
*
* To sell your Standard Reserved Instances, you must first register as a seller in the Reserved Instance
* Marketplace. After completing the registration process, you can create a Reserved Instance Marketplace listing of
* some or all of your Standard Reserved Instances, and specify the upfront price to receive for them. Your Standard
* Reserved Instance listings then become available for purchase. To view the details of your Standard Reserved
* Instance listing, you can use the DescribeReservedInstancesListings operation.
*
*
* For more information, see Reserved Instance
* Marketplace in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param createReservedInstancesListingRequest
* Contains the parameters for CreateReservedInstancesListing.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateReservedInstancesListing operation returned by the
* service.
* @sample AmazonEC2AsyncHandler.CreateReservedInstancesListing
* @see AWS API Documentation
*/
java.util.concurrent.Future createReservedInstancesListingAsync(
CreateReservedInstancesListingRequest createReservedInstancesListingRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a route in a route table within a VPC.
*
*
* You must specify one of the following targets: internet gateway or virtual private gateway, NAT instance, NAT
* gateway, VPC peering connection, network interface, or egress-only internet gateway.
*
*
* When determining how to route traffic, we use the route with the most specific match. For example, traffic is
* destined for the IPv4 address 192.0.2.3
, and the route table includes the following two IPv4 routes:
*
*
* -
*
* 192.0.2.0/24
(goes to some target A)
*
*
* -
*
* 192.0.2.0/28
(goes to some target B)
*
*
*
*
* Both routes apply to the traffic destined for 192.0.2.3
. However, the second route in the list
* covers a smaller number of IP addresses and is therefore more specific, so we use that route to determine where
* to target the traffic.
*
*
* For more information about route tables, see Route Tables in the Amazon
* Virtual Private Cloud User Guide.
*
*
* @param createRouteRequest
* @return A Java Future containing the result of the CreateRoute operation returned by the service.
* @sample AmazonEC2Async.CreateRoute
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createRouteAsync(CreateRouteRequest createRouteRequest);
/**
*
* Creates a route in a route table within a VPC.
*
*
* You must specify one of the following targets: internet gateway or virtual private gateway, NAT instance, NAT
* gateway, VPC peering connection, network interface, or egress-only internet gateway.
*
*
* When determining how to route traffic, we use the route with the most specific match. For example, traffic is
* destined for the IPv4 address 192.0.2.3
, and the route table includes the following two IPv4 routes:
*
*
* -
*
* 192.0.2.0/24
(goes to some target A)
*
*
* -
*
* 192.0.2.0/28
(goes to some target B)
*
*
*
*
* Both routes apply to the traffic destined for 192.0.2.3
. However, the second route in the list
* covers a smaller number of IP addresses and is therefore more specific, so we use that route to determine where
* to target the traffic.
*
*
* For more information about route tables, see Route Tables in the Amazon
* Virtual Private Cloud User Guide.
*
*
* @param createRouteRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateRoute operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateRoute
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createRouteAsync(CreateRouteRequest createRouteRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a route table for the specified VPC. After you create a route table, you can add routes and associate the
* table with a subnet.
*
*
* For more information, see Route
* Tables in the Amazon Virtual Private Cloud User Guide.
*
*
* @param createRouteTableRequest
* @return A Java Future containing the result of the CreateRouteTable operation returned by the service.
* @sample AmazonEC2Async.CreateRouteTable
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createRouteTableAsync(CreateRouteTableRequest createRouteTableRequest);
/**
*
* Creates a route table for the specified VPC. After you create a route table, you can add routes and associate the
* table with a subnet.
*
*
* For more information, see Route
* Tables in the Amazon Virtual Private Cloud User Guide.
*
*
* @param createRouteTableRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateRouteTable operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateRouteTable
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createRouteTableAsync(CreateRouteTableRequest createRouteTableRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a security group.
*
*
* A security group acts as a virtual firewall for your instance to control inbound and outbound traffic. For more
* information, see Amazon
* EC2 Security Groups in the Amazon Elastic Compute Cloud User Guide and Security Groups for Your
* VPC in the Amazon Virtual Private Cloud User Guide.
*
*
* When you create a security group, you specify a friendly name of your choice. You can have a security group for
* use in EC2-Classic with the same name as a security group for use in a VPC. However, you can't have two security
* groups for use in EC2-Classic with the same name or two security groups for use in a VPC with the same name.
*
*
* You have a default security group for use in EC2-Classic and a default security group for use in your VPC. If you
* don't specify a security group when you launch an instance, the instance is launched into the appropriate default
* security group. A default security group includes a default rule that grants instances unrestricted network
* access to each other.
*
*
* You can add or remove rules from your security groups using AuthorizeSecurityGroupIngress,
* AuthorizeSecurityGroupEgress, RevokeSecurityGroupIngress, and RevokeSecurityGroupEgress.
*
*
* For more information about VPC security group limits, see Amazon VPC Limits.
*
*
* @param createSecurityGroupRequest
* @return A Java Future containing the result of the CreateSecurityGroup operation returned by the service.
* @sample AmazonEC2Async.CreateSecurityGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createSecurityGroupAsync(CreateSecurityGroupRequest createSecurityGroupRequest);
/**
*
* Creates a security group.
*
*
* A security group acts as a virtual firewall for your instance to control inbound and outbound traffic. For more
* information, see Amazon
* EC2 Security Groups in the Amazon Elastic Compute Cloud User Guide and Security Groups for Your
* VPC in the Amazon Virtual Private Cloud User Guide.
*
*
* When you create a security group, you specify a friendly name of your choice. You can have a security group for
* use in EC2-Classic with the same name as a security group for use in a VPC. However, you can't have two security
* groups for use in EC2-Classic with the same name or two security groups for use in a VPC with the same name.
*
*
* You have a default security group for use in EC2-Classic and a default security group for use in your VPC. If you
* don't specify a security group when you launch an instance, the instance is launched into the appropriate default
* security group. A default security group includes a default rule that grants instances unrestricted network
* access to each other.
*
*
* You can add or remove rules from your security groups using AuthorizeSecurityGroupIngress,
* AuthorizeSecurityGroupEgress, RevokeSecurityGroupIngress, and RevokeSecurityGroupEgress.
*
*
* For more information about VPC security group limits, see Amazon VPC Limits.
*
*
* @param createSecurityGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSecurityGroup operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateSecurityGroup
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createSecurityGroupAsync(CreateSecurityGroupRequest createSecurityGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a snapshot of an EBS volume and stores it in Amazon S3. You can use snapshots for backups, to make copies
* of EBS volumes, and to save data before shutting down an instance.
*
*
* When a snapshot is created, any AWS Marketplace product codes that are associated with the source volume are
* propagated to the snapshot.
*
*
* You can take a snapshot of an attached volume that is in use. However, snapshots only capture data that has been
* written to your EBS volume at the time the snapshot command is issued; this may exclude any data that has been
* cached by any applications or the operating system. If you can pause any file systems on the volume long enough
* to take a snapshot, your snapshot should be complete. However, if you cannot pause all file writes to the volume,
* you should unmount the volume from within the instance, issue the snapshot command, and then remount the volume
* to ensure a consistent and complete snapshot. You may remount and use your volume while the snapshot status is
* pending
.
*
*
* To create a snapshot for EBS volumes that serve as root devices, you should stop the instance before taking the
* snapshot.
*
*
* Snapshots that are taken from encrypted volumes are automatically encrypted. Volumes that are created from
* encrypted snapshots are also automatically encrypted. Your encrypted volumes and any associated snapshots always
* remain protected.
*
*
* You can tag your snapshots during creation. For more information, see Tagging Your Amazon EC2 Resources
* in the Amazon Elastic Compute Cloud User Guide.
*
*
* For more information, see Amazon
* Elastic Block Store and Amazon EBS Encryption in the
* Amazon Elastic Compute Cloud User Guide.
*
*
* @param createSnapshotRequest
* Contains the parameters for CreateSnapshot.
* @return A Java Future containing the result of the CreateSnapshot operation returned by the service.
* @sample AmazonEC2Async.CreateSnapshot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createSnapshotAsync(CreateSnapshotRequest createSnapshotRequest);
/**
*
* Creates a snapshot of an EBS volume and stores it in Amazon S3. You can use snapshots for backups, to make copies
* of EBS volumes, and to save data before shutting down an instance.
*
*
* When a snapshot is created, any AWS Marketplace product codes that are associated with the source volume are
* propagated to the snapshot.
*
*
* You can take a snapshot of an attached volume that is in use. However, snapshots only capture data that has been
* written to your EBS volume at the time the snapshot command is issued; this may exclude any data that has been
* cached by any applications or the operating system. If you can pause any file systems on the volume long enough
* to take a snapshot, your snapshot should be complete. However, if you cannot pause all file writes to the volume,
* you should unmount the volume from within the instance, issue the snapshot command, and then remount the volume
* to ensure a consistent and complete snapshot. You may remount and use your volume while the snapshot status is
* pending
.
*
*
* To create a snapshot for EBS volumes that serve as root devices, you should stop the instance before taking the
* snapshot.
*
*
* Snapshots that are taken from encrypted volumes are automatically encrypted. Volumes that are created from
* encrypted snapshots are also automatically encrypted. Your encrypted volumes and any associated snapshots always
* remain protected.
*
*
* You can tag your snapshots during creation. For more information, see Tagging Your Amazon EC2 Resources
* in the Amazon Elastic Compute Cloud User Guide.
*
*
* For more information, see Amazon
* Elastic Block Store and Amazon EBS Encryption in the
* Amazon Elastic Compute Cloud User Guide.
*
*
* @param createSnapshotRequest
* Contains the parameters for CreateSnapshot.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSnapshot operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateSnapshot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createSnapshotAsync(CreateSnapshotRequest createSnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates crash-consistent snapshots of multiple EBS volumes and stores the data in S3. Volumes are chosen by
* specifying an instance. Any attached volumes will produce one snapshot each that is crash-consistent across the
* instance. Boot volumes can be excluded by changing the paramaters.
*
*
* @param createSnapshotsRequest
* @return A Java Future containing the result of the CreateSnapshots operation returned by the service.
* @sample AmazonEC2Async.CreateSnapshots
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createSnapshotsAsync(CreateSnapshotsRequest createSnapshotsRequest);
/**
*
* Creates crash-consistent snapshots of multiple EBS volumes and stores the data in S3. Volumes are chosen by
* specifying an instance. Any attached volumes will produce one snapshot each that is crash-consistent across the
* instance. Boot volumes can be excluded by changing the paramaters.
*
*
* @param createSnapshotsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSnapshots operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateSnapshots
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createSnapshotsAsync(CreateSnapshotsRequest createSnapshotsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a data feed for Spot Instances, enabling you to view Spot Instance usage logs. You can create one data
* feed per AWS account. For more information, see Spot Instance Data Feed in
* the Amazon EC2 User Guide for Linux Instances.
*
*
* @param createSpotDatafeedSubscriptionRequest
* Contains the parameters for CreateSpotDatafeedSubscription.
* @return A Java Future containing the result of the CreateSpotDatafeedSubscription operation returned by the
* service.
* @sample AmazonEC2Async.CreateSpotDatafeedSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future createSpotDatafeedSubscriptionAsync(
CreateSpotDatafeedSubscriptionRequest createSpotDatafeedSubscriptionRequest);
/**
*
* Creates a data feed for Spot Instances, enabling you to view Spot Instance usage logs. You can create one data
* feed per AWS account. For more information, see Spot Instance Data Feed in
* the Amazon EC2 User Guide for Linux Instances.
*
*
* @param createSpotDatafeedSubscriptionRequest
* Contains the parameters for CreateSpotDatafeedSubscription.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSpotDatafeedSubscription operation returned by the
* service.
* @sample AmazonEC2AsyncHandler.CreateSpotDatafeedSubscription
* @see AWS API Documentation
*/
java.util.concurrent.Future createSpotDatafeedSubscriptionAsync(
CreateSpotDatafeedSubscriptionRequest createSpotDatafeedSubscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a subnet in an existing VPC.
*
*
* When you create each subnet, you provide the VPC ID and IPv4 CIDR block for the subnet. After you create a
* subnet, you can't change its CIDR block. The size of the subnet's IPv4 CIDR block can be the same as a VPC's IPv4
* CIDR block, or a subset of a VPC's IPv4 CIDR block. If you create more than one subnet in a VPC, the subnets'
* CIDR blocks must not overlap. The smallest IPv4 subnet (and VPC) you can create uses a /28 netmask (16 IPv4
* addresses), and the largest uses a /16 netmask (65,536 IPv4 addresses).
*
*
* If you've associated an IPv6 CIDR block with your VPC, you can create a subnet with an IPv6 CIDR block that uses
* a /64 prefix length.
*
*
*
* AWS reserves both the first four and the last IPv4 address in each subnet's CIDR block. They're not available for
* use.
*
*
*
* If you add more than one subnet to a VPC, they're set up in a star topology with a logical router in the middle.
*
*
* If you launch an instance in a VPC using an Amazon EBS-backed AMI, the IP address doesn't change if you stop and
* restart the instance (unlike a similar instance launched outside a VPC, which gets a new IP address when
* restarted). It's therefore possible to have a subnet with no running instances (they're all stopped), but no
* remaining IP addresses available.
*
*
* For more information about subnets, see Your VPC and Subnets in the
* Amazon Virtual Private Cloud User Guide.
*
*
* @param createSubnetRequest
* @return A Java Future containing the result of the CreateSubnet operation returned by the service.
* @sample AmazonEC2Async.CreateSubnet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createSubnetAsync(CreateSubnetRequest createSubnetRequest);
/**
*
* Creates a subnet in an existing VPC.
*
*
* When you create each subnet, you provide the VPC ID and IPv4 CIDR block for the subnet. After you create a
* subnet, you can't change its CIDR block. The size of the subnet's IPv4 CIDR block can be the same as a VPC's IPv4
* CIDR block, or a subset of a VPC's IPv4 CIDR block. If you create more than one subnet in a VPC, the subnets'
* CIDR blocks must not overlap. The smallest IPv4 subnet (and VPC) you can create uses a /28 netmask (16 IPv4
* addresses), and the largest uses a /16 netmask (65,536 IPv4 addresses).
*
*
* If you've associated an IPv6 CIDR block with your VPC, you can create a subnet with an IPv6 CIDR block that uses
* a /64 prefix length.
*
*
*
* AWS reserves both the first four and the last IPv4 address in each subnet's CIDR block. They're not available for
* use.
*
*
*
* If you add more than one subnet to a VPC, they're set up in a star topology with a logical router in the middle.
*
*
* If you launch an instance in a VPC using an Amazon EBS-backed AMI, the IP address doesn't change if you stop and
* restart the instance (unlike a similar instance launched outside a VPC, which gets a new IP address when
* restarted). It's therefore possible to have a subnet with no running instances (they're all stopped), but no
* remaining IP addresses available.
*
*
* For more information about subnets, see Your VPC and Subnets in the
* Amazon Virtual Private Cloud User Guide.
*
*
* @param createSubnetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSubnet operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateSubnet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createSubnetAsync(CreateSubnetRequest createSubnetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds or overwrites the specified tags for the specified Amazon EC2 resource or resources. Each resource can have
* a maximum of 50 tags. Each tag consists of a key and optional value. Tag keys must be unique per resource.
*
*
* For more information about tags, see Tagging Your Resources in the
* Amazon Elastic Compute Cloud User Guide. For more information about creating IAM policies that control
* users' access to resources based on tags, see Supported
* Resource-Level Permissions for Amazon EC2 API Actions in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param createTagsRequest
* @return A Java Future containing the result of the CreateTags operation returned by the service.
* @sample AmazonEC2Async.CreateTags
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createTagsAsync(CreateTagsRequest createTagsRequest);
/**
*
* Adds or overwrites the specified tags for the specified Amazon EC2 resource or resources. Each resource can have
* a maximum of 50 tags. Each tag consists of a key and optional value. Tag keys must be unique per resource.
*
*
* For more information about tags, see Tagging Your Resources in the
* Amazon Elastic Compute Cloud User Guide. For more information about creating IAM policies that control
* users' access to resources based on tags, see Supported
* Resource-Level Permissions for Amazon EC2 API Actions in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param createTagsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTags operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateTags
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createTagsAsync(CreateTagsRequest createTagsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a Traffic Mirror filter.
*
*
* A Traffic Mirror filter is a set of rules that defines the traffic to mirror.
*
*
* By default, no traffic is mirrored. To mirror traffic, use CreateTrafficMirrorFilterRule to add Traffic
* Mirror rules to the filter. The rules you add define what traffic gets mirrored. You can also use
* ModifyTrafficMirrorFilterNetworkServices to mirror supported network services.
*
*
* @param createTrafficMirrorFilterRequest
* @return A Java Future containing the result of the CreateTrafficMirrorFilter operation returned by the service.
* @sample AmazonEC2Async.CreateTrafficMirrorFilter
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createTrafficMirrorFilterAsync(
CreateTrafficMirrorFilterRequest createTrafficMirrorFilterRequest);
/**
*
* Creates a Traffic Mirror filter.
*
*
* A Traffic Mirror filter is a set of rules that defines the traffic to mirror.
*
*
* By default, no traffic is mirrored. To mirror traffic, use CreateTrafficMirrorFilterRule to add Traffic
* Mirror rules to the filter. The rules you add define what traffic gets mirrored. You can also use
* ModifyTrafficMirrorFilterNetworkServices to mirror supported network services.
*
*
* @param createTrafficMirrorFilterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTrafficMirrorFilter operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateTrafficMirrorFilter
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createTrafficMirrorFilterAsync(
CreateTrafficMirrorFilterRequest createTrafficMirrorFilterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a Traffic Mirror rule.
*
*
* A Traffic Mirror rule defines the Traffic Mirror source traffic to mirror.
*
*
* You need the Traffic Mirror filter ID when you create the rule.
*
*
* @param createTrafficMirrorFilterRuleRequest
* @return A Java Future containing the result of the CreateTrafficMirrorFilterRule operation returned by the
* service.
* @sample AmazonEC2Async.CreateTrafficMirrorFilterRule
* @see AWS API Documentation
*/
java.util.concurrent.Future createTrafficMirrorFilterRuleAsync(
CreateTrafficMirrorFilterRuleRequest createTrafficMirrorFilterRuleRequest);
/**
*
* Creates a Traffic Mirror rule.
*
*
* A Traffic Mirror rule defines the Traffic Mirror source traffic to mirror.
*
*
* You need the Traffic Mirror filter ID when you create the rule.
*
*
* @param createTrafficMirrorFilterRuleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTrafficMirrorFilterRule operation returned by the
* service.
* @sample AmazonEC2AsyncHandler.CreateTrafficMirrorFilterRule
* @see AWS API Documentation
*/
java.util.concurrent.Future createTrafficMirrorFilterRuleAsync(
CreateTrafficMirrorFilterRuleRequest createTrafficMirrorFilterRuleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a Traffic Mirror session.
*
*
* A Traffic Mirror session actively copies packets from a Traffic Mirror source to a Traffic Mirror target. Create
* a filter, and then assign it to the session to define a subset of the traffic to mirror, for example all TCP
* traffic.
*
*
* The Traffic Mirror source and the Traffic Mirror target (monitoring appliances) can be in the same VPC, or in a
* different VPC connected via VPC peering or a transit gateway.
*
*
* By default, no traffic is mirrored. Use CreateTrafficMirrorFilter to create filter rules that specify the
* traffic to mirror.
*
*
* @param createTrafficMirrorSessionRequest
* @return A Java Future containing the result of the CreateTrafficMirrorSession operation returned by the service.
* @sample AmazonEC2Async.CreateTrafficMirrorSession
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createTrafficMirrorSessionAsync(
CreateTrafficMirrorSessionRequest createTrafficMirrorSessionRequest);
/**
*
* Creates a Traffic Mirror session.
*
*
* A Traffic Mirror session actively copies packets from a Traffic Mirror source to a Traffic Mirror target. Create
* a filter, and then assign it to the session to define a subset of the traffic to mirror, for example all TCP
* traffic.
*
*
* The Traffic Mirror source and the Traffic Mirror target (monitoring appliances) can be in the same VPC, or in a
* different VPC connected via VPC peering or a transit gateway.
*
*
* By default, no traffic is mirrored. Use CreateTrafficMirrorFilter to create filter rules that specify the
* traffic to mirror.
*
*
* @param createTrafficMirrorSessionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTrafficMirrorSession operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateTrafficMirrorSession
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createTrafficMirrorSessionAsync(
CreateTrafficMirrorSessionRequest createTrafficMirrorSessionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a target for your Traffic Mirror session.
*
*
* A Traffic Mirror target is the destination for mirrored traffic. The Traffic Mirror source and the Traffic Mirror
* target (monitoring appliances) can be in the same VPC, or in different VPCs connected via VPC peering or a
* transit gateway.
*
*
* A Traffic Mirror target can be a network interface, or a Network Load Balancer.
*
*
* To use the target in a Traffic Mirror session, use CreateTrafficMirrorSession.
*
*
* @param createTrafficMirrorTargetRequest
* @return A Java Future containing the result of the CreateTrafficMirrorTarget operation returned by the service.
* @sample AmazonEC2Async.CreateTrafficMirrorTarget
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createTrafficMirrorTargetAsync(
CreateTrafficMirrorTargetRequest createTrafficMirrorTargetRequest);
/**
*
* Creates a target for your Traffic Mirror session.
*
*
* A Traffic Mirror target is the destination for mirrored traffic. The Traffic Mirror source and the Traffic Mirror
* target (monitoring appliances) can be in the same VPC, or in different VPCs connected via VPC peering or a
* transit gateway.
*
*
* A Traffic Mirror target can be a network interface, or a Network Load Balancer.
*
*
* To use the target in a Traffic Mirror session, use CreateTrafficMirrorSession.
*
*
* @param createTrafficMirrorTargetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTrafficMirrorTarget operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateTrafficMirrorTarget
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createTrafficMirrorTargetAsync(
CreateTrafficMirrorTargetRequest createTrafficMirrorTargetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a transit gateway.
*
*
* You can use a transit gateway to interconnect your virtual private clouds (VPC) and on-premises networks. After
* the transit gateway enters the available
state, you can attach your VPCs and VPN connections to the
* transit gateway.
*
*
* To attach your VPCs, use CreateTransitGatewayVpcAttachment.
*
*
* To attach a VPN connection, use CreateCustomerGateway to create a customer gateway and specify the ID of
* the customer gateway and the ID of the transit gateway in a call to CreateVpnConnection.
*
*
* When you create a transit gateway, we create a default transit gateway route table and use it as the default
* association route table and the default propagation route table. You can use
* CreateTransitGatewayRouteTable to create additional transit gateway route tables. If you disable automatic
* route propagation, we do not create a default transit gateway route table. You can use
* EnableTransitGatewayRouteTablePropagation to propagate routes from a resource attachment to a transit
* gateway route table. If you disable automatic associations, you can use AssociateTransitGatewayRouteTable
* to associate a resource attachment with a transit gateway route table.
*
*
* @param createTransitGatewayRequest
* @return A Java Future containing the result of the CreateTransitGateway operation returned by the service.
* @sample AmazonEC2Async.CreateTransitGateway
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createTransitGatewayAsync(CreateTransitGatewayRequest createTransitGatewayRequest);
/**
*
* Creates a transit gateway.
*
*
* You can use a transit gateway to interconnect your virtual private clouds (VPC) and on-premises networks. After
* the transit gateway enters the available
state, you can attach your VPCs and VPN connections to the
* transit gateway.
*
*
* To attach your VPCs, use CreateTransitGatewayVpcAttachment.
*
*
* To attach a VPN connection, use CreateCustomerGateway to create a customer gateway and specify the ID of
* the customer gateway and the ID of the transit gateway in a call to CreateVpnConnection.
*
*
* When you create a transit gateway, we create a default transit gateway route table and use it as the default
* association route table and the default propagation route table. You can use
* CreateTransitGatewayRouteTable to create additional transit gateway route tables. If you disable automatic
* route propagation, we do not create a default transit gateway route table. You can use
* EnableTransitGatewayRouteTablePropagation to propagate routes from a resource attachment to a transit
* gateway route table. If you disable automatic associations, you can use AssociateTransitGatewayRouteTable
* to associate a resource attachment with a transit gateway route table.
*
*
* @param createTransitGatewayRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTransitGateway operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateTransitGateway
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createTransitGatewayAsync(CreateTransitGatewayRequest createTransitGatewayRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a static route for the specified transit gateway route table.
*
*
* @param createTransitGatewayRouteRequest
* @return A Java Future containing the result of the CreateTransitGatewayRoute operation returned by the service.
* @sample AmazonEC2Async.CreateTransitGatewayRoute
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createTransitGatewayRouteAsync(
CreateTransitGatewayRouteRequest createTransitGatewayRouteRequest);
/**
*
* Creates a static route for the specified transit gateway route table.
*
*
* @param createTransitGatewayRouteRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTransitGatewayRoute operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateTransitGatewayRoute
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createTransitGatewayRouteAsync(
CreateTransitGatewayRouteRequest createTransitGatewayRouteRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a route table for the specified transit gateway.
*
*
* @param createTransitGatewayRouteTableRequest
* @return A Java Future containing the result of the CreateTransitGatewayRouteTable operation returned by the
* service.
* @sample AmazonEC2Async.CreateTransitGatewayRouteTable
* @see AWS API Documentation
*/
java.util.concurrent.Future createTransitGatewayRouteTableAsync(
CreateTransitGatewayRouteTableRequest createTransitGatewayRouteTableRequest);
/**
*
* Creates a route table for the specified transit gateway.
*
*
* @param createTransitGatewayRouteTableRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTransitGatewayRouteTable operation returned by the
* service.
* @sample AmazonEC2AsyncHandler.CreateTransitGatewayRouteTable
* @see AWS API Documentation
*/
java.util.concurrent.Future createTransitGatewayRouteTableAsync(
CreateTransitGatewayRouteTableRequest createTransitGatewayRouteTableRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Attaches the specified VPC to the specified transit gateway.
*
*
* If you attach a VPC with a CIDR range that overlaps the CIDR range of a VPC that is already attached, the new VPC
* CIDR range is not propagated to the default propagation route table.
*
*
* To send VPC traffic to an attached transit gateway, add a route to the VPC route table using CreateRoute.
*
*
* @param createTransitGatewayVpcAttachmentRequest
* @return A Java Future containing the result of the CreateTransitGatewayVpcAttachment operation returned by the
* service.
* @sample AmazonEC2Async.CreateTransitGatewayVpcAttachment
* @see AWS API Documentation
*/
java.util.concurrent.Future createTransitGatewayVpcAttachmentAsync(
CreateTransitGatewayVpcAttachmentRequest createTransitGatewayVpcAttachmentRequest);
/**
*
* Attaches the specified VPC to the specified transit gateway.
*
*
* If you attach a VPC with a CIDR range that overlaps the CIDR range of a VPC that is already attached, the new VPC
* CIDR range is not propagated to the default propagation route table.
*
*
* To send VPC traffic to an attached transit gateway, add a route to the VPC route table using CreateRoute.
*
*
* @param createTransitGatewayVpcAttachmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateTransitGatewayVpcAttachment operation returned by the
* service.
* @sample AmazonEC2AsyncHandler.CreateTransitGatewayVpcAttachment
* @see AWS API Documentation
*/
java.util.concurrent.Future createTransitGatewayVpcAttachmentAsync(
CreateTransitGatewayVpcAttachmentRequest createTransitGatewayVpcAttachmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an EBS volume that can be attached to an instance in the same Availability Zone. The volume is created in
* the regional endpoint that you send the HTTP request to. For more information see