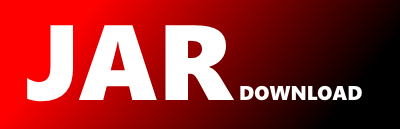
com.amazonaws.services.ec2.model.CreateCapacityReservationFleetRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ec2 Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ec2.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
import com.amazonaws.Request;
import com.amazonaws.services.ec2.model.transform.CreateCapacityReservationFleetRequestMarshaller;
/**
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateCapacityReservationFleetRequest extends AmazonWebServiceRequest implements Serializable, Cloneable,
DryRunSupportedRequest {
/**
*
* The strategy used by the Capacity Reservation Fleet to determine which of the specified instance types to use.
* Currently, only the prioritized
allocation strategy is supported. For more information, see Allocation
* strategy in the Amazon EC2 User Guide.
*
*
* Valid values: prioritized
*
*/
private String allocationStrategy;
/**
*
* Unique, case-sensitive identifier that you provide to ensure the idempotency of the request. For more
* information, see Ensure
* Idempotency.
*
*/
private String clientToken;
/**
*
* Information about the instance types for which to reserve the capacity.
*
*/
private com.amazonaws.internal.SdkInternalList instanceTypeSpecifications;
/**
*
* Indicates the tenancy of the Capacity Reservation Fleet. All Capacity Reservations in the Fleet inherit this
* tenancy. The Capacity Reservation Fleet can have one of the following tenancy settings:
*
*
* -
*
* default
- The Capacity Reservation Fleet is created on hardware that is shared with other Amazon Web
* Services accounts.
*
*
* -
*
* dedicated
- The Capacity Reservations are created on single-tenant hardware that is dedicated to a
* single Amazon Web Services account.
*
*
*
*/
private String tenancy;
/**
*
* The total number of capacity units to be reserved by the Capacity Reservation Fleet. This value, together with
* the instance type weights that you assign to each instance type used by the Fleet determine the number of
* instances for which the Fleet reserves capacity. Both values are based on units that make sense for your
* workload. For more information, see Total target
* capacity in the Amazon EC2 User Guide.
*
*/
private Integer totalTargetCapacity;
/**
*
* The date and time at which the Capacity Reservation Fleet expires. When the Capacity Reservation Fleet expires,
* its state changes to expired
and all of the Capacity Reservations in the Fleet expire.
*
*
* The Capacity Reservation Fleet expires within an hour after the specified time. For example, if you specify
* 5/31/2019
, 13:30:55
, the Capacity Reservation Fleet is guaranteed to expire between
* 13:30:55
and 14:30:55
on 5/31/2019
.
*
*/
private java.util.Date endDate;
/**
*
* Indicates the type of instance launches that the Capacity Reservation Fleet accepts. All Capacity Reservations in
* the Fleet inherit this instance matching criteria.
*
*
* Currently, Capacity Reservation Fleets support open
instance matching criteria only. This means that
* instances that have matching attributes (instance type, platform, and Availability Zone) run in the Capacity
* Reservations automatically. Instances do not need to explicitly target a Capacity Reservation Fleet to use its
* reserved capacity.
*
*/
private String instanceMatchCriteria;
/**
*
* The tags to assign to the Capacity Reservation Fleet. The tags are automatically assigned to the Capacity
* Reservations in the Fleet.
*
*/
private com.amazonaws.internal.SdkInternalList tagSpecifications;
/**
*
* The strategy used by the Capacity Reservation Fleet to determine which of the specified instance types to use.
* Currently, only the prioritized
allocation strategy is supported. For more information, see Allocation
* strategy in the Amazon EC2 User Guide.
*
*
* Valid values: prioritized
*
*
* @param allocationStrategy
* The strategy used by the Capacity Reservation Fleet to determine which of the specified instance types to
* use. Currently, only the prioritized
allocation strategy is supported. For more information,
* see
* Allocation strategy in the Amazon EC2 User Guide.
*
* Valid values: prioritized
*/
public void setAllocationStrategy(String allocationStrategy) {
this.allocationStrategy = allocationStrategy;
}
/**
*
* The strategy used by the Capacity Reservation Fleet to determine which of the specified instance types to use.
* Currently, only the prioritized
allocation strategy is supported. For more information, see Allocation
* strategy in the Amazon EC2 User Guide.
*
*
* Valid values: prioritized
*
*
* @return The strategy used by the Capacity Reservation Fleet to determine which of the specified instance types to
* use. Currently, only the prioritized
allocation strategy is supported. For more information,
* see
* Allocation strategy in the Amazon EC2 User Guide.
*
* Valid values: prioritized
*/
public String getAllocationStrategy() {
return this.allocationStrategy;
}
/**
*
* The strategy used by the Capacity Reservation Fleet to determine which of the specified instance types to use.
* Currently, only the prioritized
allocation strategy is supported. For more information, see Allocation
* strategy in the Amazon EC2 User Guide.
*
*
* Valid values: prioritized
*
*
* @param allocationStrategy
* The strategy used by the Capacity Reservation Fleet to determine which of the specified instance types to
* use. Currently, only the prioritized
allocation strategy is supported. For more information,
* see
* Allocation strategy in the Amazon EC2 User Guide.
*
* Valid values: prioritized
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCapacityReservationFleetRequest withAllocationStrategy(String allocationStrategy) {
setAllocationStrategy(allocationStrategy);
return this;
}
/**
*
* Unique, case-sensitive identifier that you provide to ensure the idempotency of the request. For more
* information, see Ensure
* Idempotency.
*
*
* @param clientToken
* Unique, case-sensitive identifier that you provide to ensure the idempotency of the request. For more
* information, see Ensure
* Idempotency.
*/
public void setClientToken(String clientToken) {
this.clientToken = clientToken;
}
/**
*
* Unique, case-sensitive identifier that you provide to ensure the idempotency of the request. For more
* information, see Ensure
* Idempotency.
*
*
* @return Unique, case-sensitive identifier that you provide to ensure the idempotency of the request. For more
* information, see Ensure
* Idempotency.
*/
public String getClientToken() {
return this.clientToken;
}
/**
*
* Unique, case-sensitive identifier that you provide to ensure the idempotency of the request. For more
* information, see Ensure
* Idempotency.
*
*
* @param clientToken
* Unique, case-sensitive identifier that you provide to ensure the idempotency of the request. For more
* information, see Ensure
* Idempotency.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCapacityReservationFleetRequest withClientToken(String clientToken) {
setClientToken(clientToken);
return this;
}
/**
*
* Information about the instance types for which to reserve the capacity.
*
*
* @return Information about the instance types for which to reserve the capacity.
*/
public java.util.List getInstanceTypeSpecifications() {
if (instanceTypeSpecifications == null) {
instanceTypeSpecifications = new com.amazonaws.internal.SdkInternalList();
}
return instanceTypeSpecifications;
}
/**
*
* Information about the instance types for which to reserve the capacity.
*
*
* @param instanceTypeSpecifications
* Information about the instance types for which to reserve the capacity.
*/
public void setInstanceTypeSpecifications(java.util.Collection instanceTypeSpecifications) {
if (instanceTypeSpecifications == null) {
this.instanceTypeSpecifications = null;
return;
}
this.instanceTypeSpecifications = new com.amazonaws.internal.SdkInternalList(instanceTypeSpecifications);
}
/**
*
* Information about the instance types for which to reserve the capacity.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setInstanceTypeSpecifications(java.util.Collection)} or
* {@link #withInstanceTypeSpecifications(java.util.Collection)} if you want to override the existing values.
*
*
* @param instanceTypeSpecifications
* Information about the instance types for which to reserve the capacity.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCapacityReservationFleetRequest withInstanceTypeSpecifications(ReservationFleetInstanceSpecification... instanceTypeSpecifications) {
if (this.instanceTypeSpecifications == null) {
setInstanceTypeSpecifications(new com.amazonaws.internal.SdkInternalList(instanceTypeSpecifications.length));
}
for (ReservationFleetInstanceSpecification ele : instanceTypeSpecifications) {
this.instanceTypeSpecifications.add(ele);
}
return this;
}
/**
*
* Information about the instance types for which to reserve the capacity.
*
*
* @param instanceTypeSpecifications
* Information about the instance types for which to reserve the capacity.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCapacityReservationFleetRequest withInstanceTypeSpecifications(
java.util.Collection instanceTypeSpecifications) {
setInstanceTypeSpecifications(instanceTypeSpecifications);
return this;
}
/**
*
* Indicates the tenancy of the Capacity Reservation Fleet. All Capacity Reservations in the Fleet inherit this
* tenancy. The Capacity Reservation Fleet can have one of the following tenancy settings:
*
*
* -
*
* default
- The Capacity Reservation Fleet is created on hardware that is shared with other Amazon Web
* Services accounts.
*
*
* -
*
* dedicated
- The Capacity Reservations are created on single-tenant hardware that is dedicated to a
* single Amazon Web Services account.
*
*
*
*
* @param tenancy
* Indicates the tenancy of the Capacity Reservation Fleet. All Capacity Reservations in the Fleet inherit
* this tenancy. The Capacity Reservation Fleet can have one of the following tenancy settings:
*
* -
*
* default
- The Capacity Reservation Fleet is created on hardware that is shared with other
* Amazon Web Services accounts.
*
*
* -
*
* dedicated
- The Capacity Reservations are created on single-tenant hardware that is dedicated
* to a single Amazon Web Services account.
*
*
* @see FleetCapacityReservationTenancy
*/
public void setTenancy(String tenancy) {
this.tenancy = tenancy;
}
/**
*
* Indicates the tenancy of the Capacity Reservation Fleet. All Capacity Reservations in the Fleet inherit this
* tenancy. The Capacity Reservation Fleet can have one of the following tenancy settings:
*
*
* -
*
* default
- The Capacity Reservation Fleet is created on hardware that is shared with other Amazon Web
* Services accounts.
*
*
* -
*
* dedicated
- The Capacity Reservations are created on single-tenant hardware that is dedicated to a
* single Amazon Web Services account.
*
*
*
*
* @return Indicates the tenancy of the Capacity Reservation Fleet. All Capacity Reservations in the Fleet inherit
* this tenancy. The Capacity Reservation Fleet can have one of the following tenancy settings:
*
* -
*
* default
- The Capacity Reservation Fleet is created on hardware that is shared with other
* Amazon Web Services accounts.
*
*
* -
*
* dedicated
- The Capacity Reservations are created on single-tenant hardware that is
* dedicated to a single Amazon Web Services account.
*
*
* @see FleetCapacityReservationTenancy
*/
public String getTenancy() {
return this.tenancy;
}
/**
*
* Indicates the tenancy of the Capacity Reservation Fleet. All Capacity Reservations in the Fleet inherit this
* tenancy. The Capacity Reservation Fleet can have one of the following tenancy settings:
*
*
* -
*
* default
- The Capacity Reservation Fleet is created on hardware that is shared with other Amazon Web
* Services accounts.
*
*
* -
*
* dedicated
- The Capacity Reservations are created on single-tenant hardware that is dedicated to a
* single Amazon Web Services account.
*
*
*
*
* @param tenancy
* Indicates the tenancy of the Capacity Reservation Fleet. All Capacity Reservations in the Fleet inherit
* this tenancy. The Capacity Reservation Fleet can have one of the following tenancy settings:
*
* -
*
* default
- The Capacity Reservation Fleet is created on hardware that is shared with other
* Amazon Web Services accounts.
*
*
* -
*
* dedicated
- The Capacity Reservations are created on single-tenant hardware that is dedicated
* to a single Amazon Web Services account.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see FleetCapacityReservationTenancy
*/
public CreateCapacityReservationFleetRequest withTenancy(String tenancy) {
setTenancy(tenancy);
return this;
}
/**
*
* Indicates the tenancy of the Capacity Reservation Fleet. All Capacity Reservations in the Fleet inherit this
* tenancy. The Capacity Reservation Fleet can have one of the following tenancy settings:
*
*
* -
*
* default
- The Capacity Reservation Fleet is created on hardware that is shared with other Amazon Web
* Services accounts.
*
*
* -
*
* dedicated
- The Capacity Reservations are created on single-tenant hardware that is dedicated to a
* single Amazon Web Services account.
*
*
*
*
* @param tenancy
* Indicates the tenancy of the Capacity Reservation Fleet. All Capacity Reservations in the Fleet inherit
* this tenancy. The Capacity Reservation Fleet can have one of the following tenancy settings:
*
* -
*
* default
- The Capacity Reservation Fleet is created on hardware that is shared with other
* Amazon Web Services accounts.
*
*
* -
*
* dedicated
- The Capacity Reservations are created on single-tenant hardware that is dedicated
* to a single Amazon Web Services account.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see FleetCapacityReservationTenancy
*/
public CreateCapacityReservationFleetRequest withTenancy(FleetCapacityReservationTenancy tenancy) {
this.tenancy = tenancy.toString();
return this;
}
/**
*
* The total number of capacity units to be reserved by the Capacity Reservation Fleet. This value, together with
* the instance type weights that you assign to each instance type used by the Fleet determine the number of
* instances for which the Fleet reserves capacity. Both values are based on units that make sense for your
* workload. For more information, see Total target
* capacity in the Amazon EC2 User Guide.
*
*
* @param totalTargetCapacity
* The total number of capacity units to be reserved by the Capacity Reservation Fleet. This value, together
* with the instance type weights that you assign to each instance type used by the Fleet determine the
* number of instances for which the Fleet reserves capacity. Both values are based on units that make sense
* for your workload. For more information, see Total
* target capacity in the Amazon EC2 User Guide.
*/
public void setTotalTargetCapacity(Integer totalTargetCapacity) {
this.totalTargetCapacity = totalTargetCapacity;
}
/**
*
* The total number of capacity units to be reserved by the Capacity Reservation Fleet. This value, together with
* the instance type weights that you assign to each instance type used by the Fleet determine the number of
* instances for which the Fleet reserves capacity. Both values are based on units that make sense for your
* workload. For more information, see Total target
* capacity in the Amazon EC2 User Guide.
*
*
* @return The total number of capacity units to be reserved by the Capacity Reservation Fleet. This value, together
* with the instance type weights that you assign to each instance type used by the Fleet determine the
* number of instances for which the Fleet reserves capacity. Both values are based on units that make sense
* for your workload. For more information, see Total
* target capacity in the Amazon EC2 User Guide.
*/
public Integer getTotalTargetCapacity() {
return this.totalTargetCapacity;
}
/**
*
* The total number of capacity units to be reserved by the Capacity Reservation Fleet. This value, together with
* the instance type weights that you assign to each instance type used by the Fleet determine the number of
* instances for which the Fleet reserves capacity. Both values are based on units that make sense for your
* workload. For more information, see Total target
* capacity in the Amazon EC2 User Guide.
*
*
* @param totalTargetCapacity
* The total number of capacity units to be reserved by the Capacity Reservation Fleet. This value, together
* with the instance type weights that you assign to each instance type used by the Fleet determine the
* number of instances for which the Fleet reserves capacity. Both values are based on units that make sense
* for your workload. For more information, see Total
* target capacity in the Amazon EC2 User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCapacityReservationFleetRequest withTotalTargetCapacity(Integer totalTargetCapacity) {
setTotalTargetCapacity(totalTargetCapacity);
return this;
}
/**
*
* The date and time at which the Capacity Reservation Fleet expires. When the Capacity Reservation Fleet expires,
* its state changes to expired
and all of the Capacity Reservations in the Fleet expire.
*
*
* The Capacity Reservation Fleet expires within an hour after the specified time. For example, if you specify
* 5/31/2019
, 13:30:55
, the Capacity Reservation Fleet is guaranteed to expire between
* 13:30:55
and 14:30:55
on 5/31/2019
.
*
*
* @param endDate
* The date and time at which the Capacity Reservation Fleet expires. When the Capacity Reservation Fleet
* expires, its state changes to expired
and all of the Capacity Reservations in the Fleet
* expire.
*
* The Capacity Reservation Fleet expires within an hour after the specified time. For example, if you
* specify 5/31/2019
, 13:30:55
, the Capacity Reservation Fleet is guaranteed to
* expire between 13:30:55
and 14:30:55
on 5/31/2019
.
*/
public void setEndDate(java.util.Date endDate) {
this.endDate = endDate;
}
/**
*
* The date and time at which the Capacity Reservation Fleet expires. When the Capacity Reservation Fleet expires,
* its state changes to expired
and all of the Capacity Reservations in the Fleet expire.
*
*
* The Capacity Reservation Fleet expires within an hour after the specified time. For example, if you specify
* 5/31/2019
, 13:30:55
, the Capacity Reservation Fleet is guaranteed to expire between
* 13:30:55
and 14:30:55
on 5/31/2019
.
*
*
* @return The date and time at which the Capacity Reservation Fleet expires. When the Capacity Reservation Fleet
* expires, its state changes to expired
and all of the Capacity Reservations in the Fleet
* expire.
*
* The Capacity Reservation Fleet expires within an hour after the specified time. For example, if you
* specify 5/31/2019
, 13:30:55
, the Capacity Reservation Fleet is guaranteed to
* expire between 13:30:55
and 14:30:55
on 5/31/2019
.
*/
public java.util.Date getEndDate() {
return this.endDate;
}
/**
*
* The date and time at which the Capacity Reservation Fleet expires. When the Capacity Reservation Fleet expires,
* its state changes to expired
and all of the Capacity Reservations in the Fleet expire.
*
*
* The Capacity Reservation Fleet expires within an hour after the specified time. For example, if you specify
* 5/31/2019
, 13:30:55
, the Capacity Reservation Fleet is guaranteed to expire between
* 13:30:55
and 14:30:55
on 5/31/2019
.
*
*
* @param endDate
* The date and time at which the Capacity Reservation Fleet expires. When the Capacity Reservation Fleet
* expires, its state changes to expired
and all of the Capacity Reservations in the Fleet
* expire.
*
* The Capacity Reservation Fleet expires within an hour after the specified time. For example, if you
* specify 5/31/2019
, 13:30:55
, the Capacity Reservation Fleet is guaranteed to
* expire between 13:30:55
and 14:30:55
on 5/31/2019
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCapacityReservationFleetRequest withEndDate(java.util.Date endDate) {
setEndDate(endDate);
return this;
}
/**
*
* Indicates the type of instance launches that the Capacity Reservation Fleet accepts. All Capacity Reservations in
* the Fleet inherit this instance matching criteria.
*
*
* Currently, Capacity Reservation Fleets support open
instance matching criteria only. This means that
* instances that have matching attributes (instance type, platform, and Availability Zone) run in the Capacity
* Reservations automatically. Instances do not need to explicitly target a Capacity Reservation Fleet to use its
* reserved capacity.
*
*
* @param instanceMatchCriteria
* Indicates the type of instance launches that the Capacity Reservation Fleet accepts. All Capacity
* Reservations in the Fleet inherit this instance matching criteria.
*
* Currently, Capacity Reservation Fleets support open
instance matching criteria only. This
* means that instances that have matching attributes (instance type, platform, and Availability Zone) run in
* the Capacity Reservations automatically. Instances do not need to explicitly target a Capacity Reservation
* Fleet to use its reserved capacity.
* @see FleetInstanceMatchCriteria
*/
public void setInstanceMatchCriteria(String instanceMatchCriteria) {
this.instanceMatchCriteria = instanceMatchCriteria;
}
/**
*
* Indicates the type of instance launches that the Capacity Reservation Fleet accepts. All Capacity Reservations in
* the Fleet inherit this instance matching criteria.
*
*
* Currently, Capacity Reservation Fleets support open
instance matching criteria only. This means that
* instances that have matching attributes (instance type, platform, and Availability Zone) run in the Capacity
* Reservations automatically. Instances do not need to explicitly target a Capacity Reservation Fleet to use its
* reserved capacity.
*
*
* @return Indicates the type of instance launches that the Capacity Reservation Fleet accepts. All Capacity
* Reservations in the Fleet inherit this instance matching criteria.
*
* Currently, Capacity Reservation Fleets support open
instance matching criteria only. This
* means that instances that have matching attributes (instance type, platform, and Availability Zone) run
* in the Capacity Reservations automatically. Instances do not need to explicitly target a Capacity
* Reservation Fleet to use its reserved capacity.
* @see FleetInstanceMatchCriteria
*/
public String getInstanceMatchCriteria() {
return this.instanceMatchCriteria;
}
/**
*
* Indicates the type of instance launches that the Capacity Reservation Fleet accepts. All Capacity Reservations in
* the Fleet inherit this instance matching criteria.
*
*
* Currently, Capacity Reservation Fleets support open
instance matching criteria only. This means that
* instances that have matching attributes (instance type, platform, and Availability Zone) run in the Capacity
* Reservations automatically. Instances do not need to explicitly target a Capacity Reservation Fleet to use its
* reserved capacity.
*
*
* @param instanceMatchCriteria
* Indicates the type of instance launches that the Capacity Reservation Fleet accepts. All Capacity
* Reservations in the Fleet inherit this instance matching criteria.
*
* Currently, Capacity Reservation Fleets support open
instance matching criteria only. This
* means that instances that have matching attributes (instance type, platform, and Availability Zone) run in
* the Capacity Reservations automatically. Instances do not need to explicitly target a Capacity Reservation
* Fleet to use its reserved capacity.
* @return Returns a reference to this object so that method calls can be chained together.
* @see FleetInstanceMatchCriteria
*/
public CreateCapacityReservationFleetRequest withInstanceMatchCriteria(String instanceMatchCriteria) {
setInstanceMatchCriteria(instanceMatchCriteria);
return this;
}
/**
*
* Indicates the type of instance launches that the Capacity Reservation Fleet accepts. All Capacity Reservations in
* the Fleet inherit this instance matching criteria.
*
*
* Currently, Capacity Reservation Fleets support open
instance matching criteria only. This means that
* instances that have matching attributes (instance type, platform, and Availability Zone) run in the Capacity
* Reservations automatically. Instances do not need to explicitly target a Capacity Reservation Fleet to use its
* reserved capacity.
*
*
* @param instanceMatchCriteria
* Indicates the type of instance launches that the Capacity Reservation Fleet accepts. All Capacity
* Reservations in the Fleet inherit this instance matching criteria.
*
* Currently, Capacity Reservation Fleets support open
instance matching criteria only. This
* means that instances that have matching attributes (instance type, platform, and Availability Zone) run in
* the Capacity Reservations automatically. Instances do not need to explicitly target a Capacity Reservation
* Fleet to use its reserved capacity.
* @return Returns a reference to this object so that method calls can be chained together.
* @see FleetInstanceMatchCriteria
*/
public CreateCapacityReservationFleetRequest withInstanceMatchCriteria(FleetInstanceMatchCriteria instanceMatchCriteria) {
this.instanceMatchCriteria = instanceMatchCriteria.toString();
return this;
}
/**
*
* The tags to assign to the Capacity Reservation Fleet. The tags are automatically assigned to the Capacity
* Reservations in the Fleet.
*
*
* @return The tags to assign to the Capacity Reservation Fleet. The tags are automatically assigned to the Capacity
* Reservations in the Fleet.
*/
public java.util.List getTagSpecifications() {
if (tagSpecifications == null) {
tagSpecifications = new com.amazonaws.internal.SdkInternalList();
}
return tagSpecifications;
}
/**
*
* The tags to assign to the Capacity Reservation Fleet. The tags are automatically assigned to the Capacity
* Reservations in the Fleet.
*
*
* @param tagSpecifications
* The tags to assign to the Capacity Reservation Fleet. The tags are automatically assigned to the Capacity
* Reservations in the Fleet.
*/
public void setTagSpecifications(java.util.Collection tagSpecifications) {
if (tagSpecifications == null) {
this.tagSpecifications = null;
return;
}
this.tagSpecifications = new com.amazonaws.internal.SdkInternalList(tagSpecifications);
}
/**
*
* The tags to assign to the Capacity Reservation Fleet. The tags are automatically assigned to the Capacity
* Reservations in the Fleet.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTagSpecifications(java.util.Collection)} or {@link #withTagSpecifications(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param tagSpecifications
* The tags to assign to the Capacity Reservation Fleet. The tags are automatically assigned to the Capacity
* Reservations in the Fleet.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCapacityReservationFleetRequest withTagSpecifications(TagSpecification... tagSpecifications) {
if (this.tagSpecifications == null) {
setTagSpecifications(new com.amazonaws.internal.SdkInternalList(tagSpecifications.length));
}
for (TagSpecification ele : tagSpecifications) {
this.tagSpecifications.add(ele);
}
return this;
}
/**
*
* The tags to assign to the Capacity Reservation Fleet. The tags are automatically assigned to the Capacity
* Reservations in the Fleet.
*
*
* @param tagSpecifications
* The tags to assign to the Capacity Reservation Fleet. The tags are automatically assigned to the Capacity
* Reservations in the Fleet.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateCapacityReservationFleetRequest withTagSpecifications(java.util.Collection tagSpecifications) {
setTagSpecifications(tagSpecifications);
return this;
}
/**
* This method is intended for internal use only. Returns the marshaled request configured with additional
* parameters to enable operation dry-run.
*/
@Override
public Request getDryRunRequest() {
Request request = new CreateCapacityReservationFleetRequestMarshaller().marshall(this);
request.addParameter("DryRun", Boolean.toString(true));
return request;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAllocationStrategy() != null)
sb.append("AllocationStrategy: ").append(getAllocationStrategy()).append(",");
if (getClientToken() != null)
sb.append("ClientToken: ").append(getClientToken()).append(",");
if (getInstanceTypeSpecifications() != null)
sb.append("InstanceTypeSpecifications: ").append(getInstanceTypeSpecifications()).append(",");
if (getTenancy() != null)
sb.append("Tenancy: ").append(getTenancy()).append(",");
if (getTotalTargetCapacity() != null)
sb.append("TotalTargetCapacity: ").append(getTotalTargetCapacity()).append(",");
if (getEndDate() != null)
sb.append("EndDate: ").append(getEndDate()).append(",");
if (getInstanceMatchCriteria() != null)
sb.append("InstanceMatchCriteria: ").append(getInstanceMatchCriteria()).append(",");
if (getTagSpecifications() != null)
sb.append("TagSpecifications: ").append(getTagSpecifications());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateCapacityReservationFleetRequest == false)
return false;
CreateCapacityReservationFleetRequest other = (CreateCapacityReservationFleetRequest) obj;
if (other.getAllocationStrategy() == null ^ this.getAllocationStrategy() == null)
return false;
if (other.getAllocationStrategy() != null && other.getAllocationStrategy().equals(this.getAllocationStrategy()) == false)
return false;
if (other.getClientToken() == null ^ this.getClientToken() == null)
return false;
if (other.getClientToken() != null && other.getClientToken().equals(this.getClientToken()) == false)
return false;
if (other.getInstanceTypeSpecifications() == null ^ this.getInstanceTypeSpecifications() == null)
return false;
if (other.getInstanceTypeSpecifications() != null && other.getInstanceTypeSpecifications().equals(this.getInstanceTypeSpecifications()) == false)
return false;
if (other.getTenancy() == null ^ this.getTenancy() == null)
return false;
if (other.getTenancy() != null && other.getTenancy().equals(this.getTenancy()) == false)
return false;
if (other.getTotalTargetCapacity() == null ^ this.getTotalTargetCapacity() == null)
return false;
if (other.getTotalTargetCapacity() != null && other.getTotalTargetCapacity().equals(this.getTotalTargetCapacity()) == false)
return false;
if (other.getEndDate() == null ^ this.getEndDate() == null)
return false;
if (other.getEndDate() != null && other.getEndDate().equals(this.getEndDate()) == false)
return false;
if (other.getInstanceMatchCriteria() == null ^ this.getInstanceMatchCriteria() == null)
return false;
if (other.getInstanceMatchCriteria() != null && other.getInstanceMatchCriteria().equals(this.getInstanceMatchCriteria()) == false)
return false;
if (other.getTagSpecifications() == null ^ this.getTagSpecifications() == null)
return false;
if (other.getTagSpecifications() != null && other.getTagSpecifications().equals(this.getTagSpecifications()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAllocationStrategy() == null) ? 0 : getAllocationStrategy().hashCode());
hashCode = prime * hashCode + ((getClientToken() == null) ? 0 : getClientToken().hashCode());
hashCode = prime * hashCode + ((getInstanceTypeSpecifications() == null) ? 0 : getInstanceTypeSpecifications().hashCode());
hashCode = prime * hashCode + ((getTenancy() == null) ? 0 : getTenancy().hashCode());
hashCode = prime * hashCode + ((getTotalTargetCapacity() == null) ? 0 : getTotalTargetCapacity().hashCode());
hashCode = prime * hashCode + ((getEndDate() == null) ? 0 : getEndDate().hashCode());
hashCode = prime * hashCode + ((getInstanceMatchCriteria() == null) ? 0 : getInstanceMatchCriteria().hashCode());
hashCode = prime * hashCode + ((getTagSpecifications() == null) ? 0 : getTagSpecifications().hashCode());
return hashCode;
}
@Override
public CreateCapacityReservationFleetRequest clone() {
return (CreateCapacityReservationFleetRequest) super.clone();
}
}