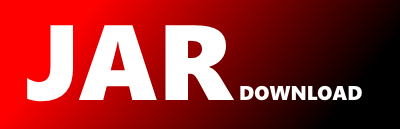
com.amazonaws.services.ec2.AmazonEC2Async Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ec2 Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ec2;
import javax.annotation.Generated;
import com.amazonaws.services.ec2.model.*;
/**
* Interface for accessing Amazon EC2 asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.ec2.AbstractAmazonEC2Async} instead.
*
*
* Amazon Elastic Compute Cloud
*
* Amazon Elastic Compute Cloud (Amazon EC2) provides secure and resizable computing capacity in the Amazon Web Services
* Cloud. Using Amazon EC2 eliminates the need to invest in hardware up front, so you can develop and deploy
* applications faster. Amazon Virtual Private Cloud (Amazon VPC) enables you to provision a logically isolated section
* of the Amazon Web Services Cloud where you can launch Amazon Web Services resources in a virtual network that you've
* defined. Amazon Elastic Block Store (Amazon EBS) provides block level storage volumes for use with EC2 instances. EBS
* volumes are highly available and reliable storage volumes that can be attached to any running instance and used like
* a hard drive.
*
*
* To learn more, see the following resources:
*
*
* -
*
* Amazon EC2: Amazon EC2 product page, Amazon EC2 documentation
*
*
* -
*
* Amazon EBS: Amazon EBS product page, Amazon EBS documentation
*
*
* -
*
* Amazon VPC: Amazon VPC product page, Amazon VPC documentation
*
*
* -
*
* VPN: VPN product page, VPN documentation
*
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonEC2Async extends AmazonEC2 {
/**
*
* Accepts an Elastic IP address transfer. For more information, see Accept a transferred Elastic IP address in the Amazon Virtual Private Cloud User Guide.
*
*
* @param acceptAddressTransferRequest
* @return A Java Future containing the result of the AcceptAddressTransfer operation returned by the service.
* @sample AmazonEC2Async.AcceptAddressTransfer
* @see AWS API
* Documentation
*/
java.util.concurrent.Future acceptAddressTransferAsync(AcceptAddressTransferRequest acceptAddressTransferRequest);
/**
*
* Accepts an Elastic IP address transfer. For more information, see Accept a transferred Elastic IP address in the Amazon Virtual Private Cloud User Guide.
*
*
* @param acceptAddressTransferRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AcceptAddressTransfer operation returned by the service.
* @sample AmazonEC2AsyncHandler.AcceptAddressTransfer
* @see AWS API
* Documentation
*/
java.util.concurrent.Future acceptAddressTransferAsync(AcceptAddressTransferRequest acceptAddressTransferRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Accepts the Convertible Reserved Instance exchange quote described in the
* GetReservedInstancesExchangeQuote call.
*
*
* @param acceptReservedInstancesExchangeQuoteRequest
* Contains the parameters for accepting the quote.
* @return A Java Future containing the result of the AcceptReservedInstancesExchangeQuote operation returned by the
* service.
* @sample AmazonEC2Async.AcceptReservedInstancesExchangeQuote
* @see AWS API Documentation
*/
java.util.concurrent.Future acceptReservedInstancesExchangeQuoteAsync(
AcceptReservedInstancesExchangeQuoteRequest acceptReservedInstancesExchangeQuoteRequest);
/**
*
* Accepts the Convertible Reserved Instance exchange quote described in the
* GetReservedInstancesExchangeQuote call.
*
*
* @param acceptReservedInstancesExchangeQuoteRequest
* Contains the parameters for accepting the quote.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AcceptReservedInstancesExchangeQuote operation returned by the
* service.
* @sample AmazonEC2AsyncHandler.AcceptReservedInstancesExchangeQuote
* @see AWS API Documentation
*/
java.util.concurrent.Future acceptReservedInstancesExchangeQuoteAsync(
AcceptReservedInstancesExchangeQuoteRequest acceptReservedInstancesExchangeQuoteRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Accepts a request to associate subnets with a transit gateway multicast domain.
*
*
* @param acceptTransitGatewayMulticastDomainAssociationsRequest
* @return A Java Future containing the result of the AcceptTransitGatewayMulticastDomainAssociations operation
* returned by the service.
* @sample AmazonEC2Async.AcceptTransitGatewayMulticastDomainAssociations
* @see AWS API Documentation
*/
java.util.concurrent.Future acceptTransitGatewayMulticastDomainAssociationsAsync(
AcceptTransitGatewayMulticastDomainAssociationsRequest acceptTransitGatewayMulticastDomainAssociationsRequest);
/**
*
* Accepts a request to associate subnets with a transit gateway multicast domain.
*
*
* @param acceptTransitGatewayMulticastDomainAssociationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AcceptTransitGatewayMulticastDomainAssociations operation
* returned by the service.
* @sample AmazonEC2AsyncHandler.AcceptTransitGatewayMulticastDomainAssociations
* @see AWS API Documentation
*/
java.util.concurrent.Future acceptTransitGatewayMulticastDomainAssociationsAsync(
AcceptTransitGatewayMulticastDomainAssociationsRequest acceptTransitGatewayMulticastDomainAssociationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Accepts a transit gateway peering attachment request. The peering attachment must be in the
* pendingAcceptance
state.
*
*
* @param acceptTransitGatewayPeeringAttachmentRequest
* @return A Java Future containing the result of the AcceptTransitGatewayPeeringAttachment operation returned by
* the service.
* @sample AmazonEC2Async.AcceptTransitGatewayPeeringAttachment
* @see AWS API Documentation
*/
java.util.concurrent.Future acceptTransitGatewayPeeringAttachmentAsync(
AcceptTransitGatewayPeeringAttachmentRequest acceptTransitGatewayPeeringAttachmentRequest);
/**
*
* Accepts a transit gateway peering attachment request. The peering attachment must be in the
* pendingAcceptance
state.
*
*
* @param acceptTransitGatewayPeeringAttachmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AcceptTransitGatewayPeeringAttachment operation returned by
* the service.
* @sample AmazonEC2AsyncHandler.AcceptTransitGatewayPeeringAttachment
* @see AWS API Documentation
*/
java.util.concurrent.Future acceptTransitGatewayPeeringAttachmentAsync(
AcceptTransitGatewayPeeringAttachmentRequest acceptTransitGatewayPeeringAttachmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Accepts a request to attach a VPC to a transit gateway.
*
*
* The VPC attachment must be in the pendingAcceptance
state. Use
* DescribeTransitGatewayVpcAttachments to view your pending VPC attachment requests. Use
* RejectTransitGatewayVpcAttachment to reject a VPC attachment request.
*
*
* @param acceptTransitGatewayVpcAttachmentRequest
* @return A Java Future containing the result of the AcceptTransitGatewayVpcAttachment operation returned by the
* service.
* @sample AmazonEC2Async.AcceptTransitGatewayVpcAttachment
* @see AWS API Documentation
*/
java.util.concurrent.Future acceptTransitGatewayVpcAttachmentAsync(
AcceptTransitGatewayVpcAttachmentRequest acceptTransitGatewayVpcAttachmentRequest);
/**
*
* Accepts a request to attach a VPC to a transit gateway.
*
*
* The VPC attachment must be in the pendingAcceptance
state. Use
* DescribeTransitGatewayVpcAttachments to view your pending VPC attachment requests. Use
* RejectTransitGatewayVpcAttachment to reject a VPC attachment request.
*
*
* @param acceptTransitGatewayVpcAttachmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AcceptTransitGatewayVpcAttachment operation returned by the
* service.
* @sample AmazonEC2AsyncHandler.AcceptTransitGatewayVpcAttachment
* @see AWS API Documentation
*/
java.util.concurrent.Future acceptTransitGatewayVpcAttachmentAsync(
AcceptTransitGatewayVpcAttachmentRequest acceptTransitGatewayVpcAttachmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Accepts connection requests to your VPC endpoint service.
*
*
* @param acceptVpcEndpointConnectionsRequest
* @return A Java Future containing the result of the AcceptVpcEndpointConnections operation returned by the
* service.
* @sample AmazonEC2Async.AcceptVpcEndpointConnections
* @see AWS API Documentation
*/
java.util.concurrent.Future acceptVpcEndpointConnectionsAsync(
AcceptVpcEndpointConnectionsRequest acceptVpcEndpointConnectionsRequest);
/**
*
* Accepts connection requests to your VPC endpoint service.
*
*
* @param acceptVpcEndpointConnectionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AcceptVpcEndpointConnections operation returned by the
* service.
* @sample AmazonEC2AsyncHandler.AcceptVpcEndpointConnections
* @see AWS API Documentation
*/
java.util.concurrent.Future acceptVpcEndpointConnectionsAsync(
AcceptVpcEndpointConnectionsRequest acceptVpcEndpointConnectionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Accept a VPC peering connection request. To accept a request, the VPC peering connection must be in the
* pending-acceptance
state, and you must be the owner of the peer VPC. Use
* DescribeVpcPeeringConnections to view your outstanding VPC peering connection requests.
*
*
* For an inter-Region VPC peering connection request, you must accept the VPC peering connection in the Region of
* the accepter VPC.
*
*
* @param acceptVpcPeeringConnectionRequest
* @return A Java Future containing the result of the AcceptVpcPeeringConnection operation returned by the service.
* @sample AmazonEC2Async.AcceptVpcPeeringConnection
* @see AWS
* API Documentation
*/
java.util.concurrent.Future acceptVpcPeeringConnectionAsync(
AcceptVpcPeeringConnectionRequest acceptVpcPeeringConnectionRequest);
/**
*
* Accept a VPC peering connection request. To accept a request, the VPC peering connection must be in the
* pending-acceptance
state, and you must be the owner of the peer VPC. Use
* DescribeVpcPeeringConnections to view your outstanding VPC peering connection requests.
*
*
* For an inter-Region VPC peering connection request, you must accept the VPC peering connection in the Region of
* the accepter VPC.
*
*
* @param acceptVpcPeeringConnectionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AcceptVpcPeeringConnection operation returned by the service.
* @sample AmazonEC2AsyncHandler.AcceptVpcPeeringConnection
* @see AWS
* API Documentation
*/
java.util.concurrent.Future acceptVpcPeeringConnectionAsync(
AcceptVpcPeeringConnectionRequest acceptVpcPeeringConnectionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the AcceptVpcPeeringConnection operation.
*
* @see #acceptVpcPeeringConnectionAsync(AcceptVpcPeeringConnectionRequest)
*/
java.util.concurrent.Future acceptVpcPeeringConnectionAsync();
/**
* Simplified method form for invoking the AcceptVpcPeeringConnection operation with an AsyncHandler.
*
* @see #acceptVpcPeeringConnectionAsync(AcceptVpcPeeringConnectionRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future acceptVpcPeeringConnectionAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Advertises an IPv4 or IPv6 address range that is provisioned for use with your Amazon Web Services resources
* through bring your own IP addresses (BYOIP).
*
*
* You can perform this operation at most once every 10 seconds, even if you specify different address ranges each
* time.
*
*
* We recommend that you stop advertising the BYOIP CIDR from other locations when you advertise it from Amazon Web
* Services. To minimize down time, you can configure your Amazon Web Services resources to use an address from a
* BYOIP CIDR before it is advertised, and then simultaneously stop advertising it from the current location and
* start advertising it through Amazon Web Services.
*
*
* It can take a few minutes before traffic to the specified addresses starts routing to Amazon Web Services because
* of BGP propagation delays.
*
*
* To stop advertising the BYOIP CIDR, use WithdrawByoipCidr.
*
*
* @param advertiseByoipCidrRequest
* @return A Java Future containing the result of the AdvertiseByoipCidr operation returned by the service.
* @sample AmazonEC2Async.AdvertiseByoipCidr
* @see AWS API
* Documentation
*/
java.util.concurrent.Future advertiseByoipCidrAsync(AdvertiseByoipCidrRequest advertiseByoipCidrRequest);
/**
*
* Advertises an IPv4 or IPv6 address range that is provisioned for use with your Amazon Web Services resources
* through bring your own IP addresses (BYOIP).
*
*
* You can perform this operation at most once every 10 seconds, even if you specify different address ranges each
* time.
*
*
* We recommend that you stop advertising the BYOIP CIDR from other locations when you advertise it from Amazon Web
* Services. To minimize down time, you can configure your Amazon Web Services resources to use an address from a
* BYOIP CIDR before it is advertised, and then simultaneously stop advertising it from the current location and
* start advertising it through Amazon Web Services.
*
*
* It can take a few minutes before traffic to the specified addresses starts routing to Amazon Web Services because
* of BGP propagation delays.
*
*
* To stop advertising the BYOIP CIDR, use WithdrawByoipCidr.
*
*
* @param advertiseByoipCidrRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AdvertiseByoipCidr operation returned by the service.
* @sample AmazonEC2AsyncHandler.AdvertiseByoipCidr
* @see AWS API
* Documentation
*/
java.util.concurrent.Future advertiseByoipCidrAsync(AdvertiseByoipCidrRequest advertiseByoipCidrRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Allocates an Elastic IP address to your Amazon Web Services account. After you allocate the Elastic IP address
* you can associate it with an instance or network interface. After you release an Elastic IP address, it is
* released to the IP address pool and can be allocated to a different Amazon Web Services account.
*
*
* You can allocate an Elastic IP address from an address pool owned by Amazon Web Services or from an address pool
* created from a public IPv4 address range that you have brought to Amazon Web Services for use with your Amazon
* Web Services resources using bring your own IP addresses (BYOIP). For more information, see Bring Your Own IP Addresses (BYOIP)
* in the Amazon Elastic Compute Cloud User Guide.
*
*
* [EC2-VPC] If you release an Elastic IP address, you might be able to recover it. You cannot recover an Elastic IP
* address that you released after it is allocated to another Amazon Web Services account. You cannot recover an
* Elastic IP address for EC2-Classic. To attempt to recover an Elastic IP address that you released, specify it in
* this operation.
*
*
* An Elastic IP address is for use either in the EC2-Classic platform or in a VPC. By default, you can allocate 5
* Elastic IP addresses for EC2-Classic per Region and 5 Elastic IP addresses for EC2-VPC per Region.
*
*
* For more information, see Elastic IP Addresses
* in the Amazon Elastic Compute Cloud User Guide.
*
*
* You can allocate a carrier IP address which is a public IP address from a telecommunication carrier, to a network
* interface which resides in a subnet in a Wavelength Zone (for example an EC2 instance).
*
*
*
* We are retiring EC2-Classic. We recommend that you migrate from EC2-Classic to a VPC. For more information, see
* Migrate from EC2-Classic to a
* VPC in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* @param allocateAddressRequest
* @return A Java Future containing the result of the AllocateAddress operation returned by the service.
* @sample AmazonEC2Async.AllocateAddress
* @see AWS API
* Documentation
*/
java.util.concurrent.Future allocateAddressAsync(AllocateAddressRequest allocateAddressRequest);
/**
*
* Allocates an Elastic IP address to your Amazon Web Services account. After you allocate the Elastic IP address
* you can associate it with an instance or network interface. After you release an Elastic IP address, it is
* released to the IP address pool and can be allocated to a different Amazon Web Services account.
*
*
* You can allocate an Elastic IP address from an address pool owned by Amazon Web Services or from an address pool
* created from a public IPv4 address range that you have brought to Amazon Web Services for use with your Amazon
* Web Services resources using bring your own IP addresses (BYOIP). For more information, see Bring Your Own IP Addresses (BYOIP)
* in the Amazon Elastic Compute Cloud User Guide.
*
*
* [EC2-VPC] If you release an Elastic IP address, you might be able to recover it. You cannot recover an Elastic IP
* address that you released after it is allocated to another Amazon Web Services account. You cannot recover an
* Elastic IP address for EC2-Classic. To attempt to recover an Elastic IP address that you released, specify it in
* this operation.
*
*
* An Elastic IP address is for use either in the EC2-Classic platform or in a VPC. By default, you can allocate 5
* Elastic IP addresses for EC2-Classic per Region and 5 Elastic IP addresses for EC2-VPC per Region.
*
*
* For more information, see Elastic IP Addresses
* in the Amazon Elastic Compute Cloud User Guide.
*
*
* You can allocate a carrier IP address which is a public IP address from a telecommunication carrier, to a network
* interface which resides in a subnet in a Wavelength Zone (for example an EC2 instance).
*
*
*
* We are retiring EC2-Classic. We recommend that you migrate from EC2-Classic to a VPC. For more information, see
* Migrate from EC2-Classic to a
* VPC in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* @param allocateAddressRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AllocateAddress operation returned by the service.
* @sample AmazonEC2AsyncHandler.AllocateAddress
* @see AWS API
* Documentation
*/
java.util.concurrent.Future allocateAddressAsync(AllocateAddressRequest allocateAddressRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the AllocateAddress operation.
*
* @see #allocateAddressAsync(AllocateAddressRequest)
*/
java.util.concurrent.Future allocateAddressAsync();
/**
* Simplified method form for invoking the AllocateAddress operation with an AsyncHandler.
*
* @see #allocateAddressAsync(AllocateAddressRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future allocateAddressAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Allocates a Dedicated Host to your account. At a minimum, specify the supported instance type or instance family,
* the Availability Zone in which to allocate the host, and the number of hosts to allocate.
*
*
* @param allocateHostsRequest
* @return A Java Future containing the result of the AllocateHosts operation returned by the service.
* @sample AmazonEC2Async.AllocateHosts
* @see AWS API
* Documentation
*/
java.util.concurrent.Future allocateHostsAsync(AllocateHostsRequest allocateHostsRequest);
/**
*
* Allocates a Dedicated Host to your account. At a minimum, specify the supported instance type or instance family,
* the Availability Zone in which to allocate the host, and the number of hosts to allocate.
*
*
* @param allocateHostsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AllocateHosts operation returned by the service.
* @sample AmazonEC2AsyncHandler.AllocateHosts
* @see AWS API
* Documentation
*/
java.util.concurrent.Future allocateHostsAsync(AllocateHostsRequest allocateHostsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Allocate a CIDR from an IPAM pool. In IPAM, an allocation is a CIDR assignment from an IPAM pool to another IPAM
* pool or to a resource. For more information, see Allocate CIDRs in the Amazon
* VPC IPAM User Guide.
*
*
*
* This action creates an allocation with strong consistency. The returned CIDR will not overlap with any other
* allocations from the same pool.
*
*
*
* @param allocateIpamPoolCidrRequest
* @return A Java Future containing the result of the AllocateIpamPoolCidr operation returned by the service.
* @sample AmazonEC2Async.AllocateIpamPoolCidr
* @see AWS API
* Documentation
*/
java.util.concurrent.Future allocateIpamPoolCidrAsync(AllocateIpamPoolCidrRequest allocateIpamPoolCidrRequest);
/**
*
* Allocate a CIDR from an IPAM pool. In IPAM, an allocation is a CIDR assignment from an IPAM pool to another IPAM
* pool or to a resource. For more information, see Allocate CIDRs in the Amazon
* VPC IPAM User Guide.
*
*
*
* This action creates an allocation with strong consistency. The returned CIDR will not overlap with any other
* allocations from the same pool.
*
*
*
* @param allocateIpamPoolCidrRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AllocateIpamPoolCidr operation returned by the service.
* @sample AmazonEC2AsyncHandler.AllocateIpamPoolCidr
* @see AWS API
* Documentation
*/
java.util.concurrent.Future allocateIpamPoolCidrAsync(AllocateIpamPoolCidrRequest allocateIpamPoolCidrRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Applies a security group to the association between the target network and the Client VPN endpoint. This action
* replaces the existing security groups with the specified security groups.
*
*
* @param applySecurityGroupsToClientVpnTargetNetworkRequest
* @return A Java Future containing the result of the ApplySecurityGroupsToClientVpnTargetNetwork operation returned
* by the service.
* @sample AmazonEC2Async.ApplySecurityGroupsToClientVpnTargetNetwork
* @see AWS API Documentation
*/
java.util.concurrent.Future applySecurityGroupsToClientVpnTargetNetworkAsync(
ApplySecurityGroupsToClientVpnTargetNetworkRequest applySecurityGroupsToClientVpnTargetNetworkRequest);
/**
*
* Applies a security group to the association between the target network and the Client VPN endpoint. This action
* replaces the existing security groups with the specified security groups.
*
*
* @param applySecurityGroupsToClientVpnTargetNetworkRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ApplySecurityGroupsToClientVpnTargetNetwork operation returned
* by the service.
* @sample AmazonEC2AsyncHandler.ApplySecurityGroupsToClientVpnTargetNetwork
* @see AWS API Documentation
*/
java.util.concurrent.Future applySecurityGroupsToClientVpnTargetNetworkAsync(
ApplySecurityGroupsToClientVpnTargetNetworkRequest applySecurityGroupsToClientVpnTargetNetworkRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Assigns one or more IPv6 addresses to the specified network interface. You can specify one or more specific IPv6
* addresses, or you can specify the number of IPv6 addresses to be automatically assigned from within the subnet's
* IPv6 CIDR block range. You can assign as many IPv6 addresses to a network interface as you can assign private
* IPv4 addresses, and the limit varies per instance type. For information, see IP Addresses Per
* Network Interface Per Instance Type in the Amazon Elastic Compute Cloud User Guide.
*
*
* You must specify either the IPv6 addresses or the IPv6 address count in the request.
*
*
* You can optionally use Prefix Delegation on the network interface. You must specify either the IPV6 Prefix
* Delegation prefixes, or the IPv6 Prefix Delegation count. For information, see Assigning prefixes to Amazon EC2
* network interfaces in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param assignIpv6AddressesRequest
* @return A Java Future containing the result of the AssignIpv6Addresses operation returned by the service.
* @sample AmazonEC2Async.AssignIpv6Addresses
* @see AWS API
* Documentation
*/
java.util.concurrent.Future assignIpv6AddressesAsync(AssignIpv6AddressesRequest assignIpv6AddressesRequest);
/**
*
* Assigns one or more IPv6 addresses to the specified network interface. You can specify one or more specific IPv6
* addresses, or you can specify the number of IPv6 addresses to be automatically assigned from within the subnet's
* IPv6 CIDR block range. You can assign as many IPv6 addresses to a network interface as you can assign private
* IPv4 addresses, and the limit varies per instance type. For information, see IP Addresses Per
* Network Interface Per Instance Type in the Amazon Elastic Compute Cloud User Guide.
*
*
* You must specify either the IPv6 addresses or the IPv6 address count in the request.
*
*
* You can optionally use Prefix Delegation on the network interface. You must specify either the IPV6 Prefix
* Delegation prefixes, or the IPv6 Prefix Delegation count. For information, see Assigning prefixes to Amazon EC2
* network interfaces in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param assignIpv6AddressesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssignIpv6Addresses operation returned by the service.
* @sample AmazonEC2AsyncHandler.AssignIpv6Addresses
* @see AWS API
* Documentation
*/
java.util.concurrent.Future assignIpv6AddressesAsync(AssignIpv6AddressesRequest assignIpv6AddressesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Assigns one or more secondary private IP addresses to the specified network interface.
*
*
* You can specify one or more specific secondary IP addresses, or you can specify the number of secondary IP
* addresses to be automatically assigned within the subnet's CIDR block range. The number of secondary IP addresses
* that you can assign to an instance varies by instance type. For information about instance types, see Instance Types in the
* Amazon Elastic Compute Cloud User Guide. For more information about Elastic IP addresses, see Elastic IP Addresses
* in the Amazon Elastic Compute Cloud User Guide.
*
*
* When you move a secondary private IP address to another network interface, any Elastic IP address that is
* associated with the IP address is also moved.
*
*
* Remapping an IP address is an asynchronous operation. When you move an IP address from one network interface to
* another, check network/interfaces/macs/mac/local-ipv4s
in the instance metadata to confirm that the
* remapping is complete.
*
*
* You must specify either the IP addresses or the IP address count in the request.
*
*
* You can optionally use Prefix Delegation on the network interface. You must specify either the IPv4 Prefix
* Delegation prefixes, or the IPv4 Prefix Delegation count. For information, see Assigning prefixes to Amazon EC2
* network interfaces in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param assignPrivateIpAddressesRequest
* Contains the parameters for AssignPrivateIpAddresses.
* @return A Java Future containing the result of the AssignPrivateIpAddresses operation returned by the service.
* @sample AmazonEC2Async.AssignPrivateIpAddresses
* @see AWS
* API Documentation
*/
java.util.concurrent.Future assignPrivateIpAddressesAsync(AssignPrivateIpAddressesRequest assignPrivateIpAddressesRequest);
/**
*
* Assigns one or more secondary private IP addresses to the specified network interface.
*
*
* You can specify one or more specific secondary IP addresses, or you can specify the number of secondary IP
* addresses to be automatically assigned within the subnet's CIDR block range. The number of secondary IP addresses
* that you can assign to an instance varies by instance type. For information about instance types, see Instance Types in the
* Amazon Elastic Compute Cloud User Guide. For more information about Elastic IP addresses, see Elastic IP Addresses
* in the Amazon Elastic Compute Cloud User Guide.
*
*
* When you move a secondary private IP address to another network interface, any Elastic IP address that is
* associated with the IP address is also moved.
*
*
* Remapping an IP address is an asynchronous operation. When you move an IP address from one network interface to
* another, check network/interfaces/macs/mac/local-ipv4s
in the instance metadata to confirm that the
* remapping is complete.
*
*
* You must specify either the IP addresses or the IP address count in the request.
*
*
* You can optionally use Prefix Delegation on the network interface. You must specify either the IPv4 Prefix
* Delegation prefixes, or the IPv4 Prefix Delegation count. For information, see Assigning prefixes to Amazon EC2
* network interfaces in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param assignPrivateIpAddressesRequest
* Contains the parameters for AssignPrivateIpAddresses.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssignPrivateIpAddresses operation returned by the service.
* @sample AmazonEC2AsyncHandler.AssignPrivateIpAddresses
* @see AWS
* API Documentation
*/
java.util.concurrent.Future assignPrivateIpAddressesAsync(AssignPrivateIpAddressesRequest assignPrivateIpAddressesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Assigns one or more private IPv4 addresses to a private NAT gateway. For more information, see Work with
* NAT gateways in the Amazon Virtual Private Cloud User Guide.
*
*
* @param assignPrivateNatGatewayAddressRequest
* @return A Java Future containing the result of the AssignPrivateNatGatewayAddress operation returned by the
* service.
* @sample AmazonEC2Async.AssignPrivateNatGatewayAddress
* @see AWS API Documentation
*/
java.util.concurrent.Future assignPrivateNatGatewayAddressAsync(
AssignPrivateNatGatewayAddressRequest assignPrivateNatGatewayAddressRequest);
/**
*
* Assigns one or more private IPv4 addresses to a private NAT gateway. For more information, see Work with
* NAT gateways in the Amazon Virtual Private Cloud User Guide.
*
*
* @param assignPrivateNatGatewayAddressRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssignPrivateNatGatewayAddress operation returned by the
* service.
* @sample AmazonEC2AsyncHandler.AssignPrivateNatGatewayAddress
* @see AWS API Documentation
*/
java.util.concurrent.Future assignPrivateNatGatewayAddressAsync(
AssignPrivateNatGatewayAddressRequest assignPrivateNatGatewayAddressRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates an Elastic IP address, or carrier IP address (for instances that are in subnets in Wavelength Zones)
* with an instance or a network interface. Before you can use an Elastic IP address, you must allocate it to your
* account.
*
*
* An Elastic IP address is for use in either the EC2-Classic platform or in a VPC. For more information, see Elastic IP Addresses
* in the Amazon Elastic Compute Cloud User Guide.
*
*
* [EC2-Classic, VPC in an EC2-VPC-only account] If the Elastic IP address is already associated with a different
* instance, it is disassociated from that instance and associated with the specified instance. If you associate an
* Elastic IP address with an instance that has an existing Elastic IP address, the existing address is
* disassociated from the instance, but remains allocated to your account.
*
*
* [VPC in an EC2-Classic account] If you don't specify a private IP address, the Elastic IP address is associated
* with the primary IP address. If the Elastic IP address is already associated with a different instance or a
* network interface, you get an error unless you allow reassociation. You cannot associate an Elastic IP address
* with an instance or network interface that has an existing Elastic IP address.
*
*
* [Subnets in Wavelength Zones] You can associate an IP address from the telecommunication carrier to the instance
* or network interface.
*
*
* You cannot associate an Elastic IP address with an interface in a different network border group.
*
*
*
* This is an idempotent operation. If you perform the operation more than once, Amazon EC2 doesn't return an error,
* and you may be charged for each time the Elastic IP address is remapped to the same instance. For more
* information, see the Elastic IP Addresses section of Amazon
* EC2 Pricing.
*
*
*
* We are retiring EC2-Classic. We recommend that you migrate from EC2-Classic to a VPC. For more information, see
* Migrate from EC2-Classic to a
* VPC in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* @param associateAddressRequest
* @return A Java Future containing the result of the AssociateAddress operation returned by the service.
* @sample AmazonEC2Async.AssociateAddress
* @see AWS API
* Documentation
*/
java.util.concurrent.Future associateAddressAsync(AssociateAddressRequest associateAddressRequest);
/**
*
* Associates an Elastic IP address, or carrier IP address (for instances that are in subnets in Wavelength Zones)
* with an instance or a network interface. Before you can use an Elastic IP address, you must allocate it to your
* account.
*
*
* An Elastic IP address is for use in either the EC2-Classic platform or in a VPC. For more information, see Elastic IP Addresses
* in the Amazon Elastic Compute Cloud User Guide.
*
*
* [EC2-Classic, VPC in an EC2-VPC-only account] If the Elastic IP address is already associated with a different
* instance, it is disassociated from that instance and associated with the specified instance. If you associate an
* Elastic IP address with an instance that has an existing Elastic IP address, the existing address is
* disassociated from the instance, but remains allocated to your account.
*
*
* [VPC in an EC2-Classic account] If you don't specify a private IP address, the Elastic IP address is associated
* with the primary IP address. If the Elastic IP address is already associated with a different instance or a
* network interface, you get an error unless you allow reassociation. You cannot associate an Elastic IP address
* with an instance or network interface that has an existing Elastic IP address.
*
*
* [Subnets in Wavelength Zones] You can associate an IP address from the telecommunication carrier to the instance
* or network interface.
*
*
* You cannot associate an Elastic IP address with an interface in a different network border group.
*
*
*
* This is an idempotent operation. If you perform the operation more than once, Amazon EC2 doesn't return an error,
* and you may be charged for each time the Elastic IP address is remapped to the same instance. For more
* information, see the Elastic IP Addresses section of Amazon
* EC2 Pricing.
*
*
*
* We are retiring EC2-Classic. We recommend that you migrate from EC2-Classic to a VPC. For more information, see
* Migrate from EC2-Classic to a
* VPC in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* @param associateAddressRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateAddress operation returned by the service.
* @sample AmazonEC2AsyncHandler.AssociateAddress
* @see AWS API
* Documentation
*/
java.util.concurrent.Future associateAddressAsync(AssociateAddressRequest associateAddressRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates a target network with a Client VPN endpoint. A target network is a subnet in a VPC. You can associate
* multiple subnets from the same VPC with a Client VPN endpoint. You can associate only one subnet in each
* Availability Zone. We recommend that you associate at least two subnets to provide Availability Zone redundancy.
*
*
* If you specified a VPC when you created the Client VPN endpoint or if you have previous subnet associations, the
* specified subnet must be in the same VPC. To specify a subnet that's in a different VPC, you must first modify
* the Client VPN endpoint (ModifyClientVpnEndpoint) and change the VPC that's associated with it.
*
*
* @param associateClientVpnTargetNetworkRequest
* @return A Java Future containing the result of the AssociateClientVpnTargetNetwork operation returned by the
* service.
* @sample AmazonEC2Async.AssociateClientVpnTargetNetwork
* @see AWS API Documentation
*/
java.util.concurrent.Future associateClientVpnTargetNetworkAsync(
AssociateClientVpnTargetNetworkRequest associateClientVpnTargetNetworkRequest);
/**
*
* Associates a target network with a Client VPN endpoint. A target network is a subnet in a VPC. You can associate
* multiple subnets from the same VPC with a Client VPN endpoint. You can associate only one subnet in each
* Availability Zone. We recommend that you associate at least two subnets to provide Availability Zone redundancy.
*
*
* If you specified a VPC when you created the Client VPN endpoint or if you have previous subnet associations, the
* specified subnet must be in the same VPC. To specify a subnet that's in a different VPC, you must first modify
* the Client VPN endpoint (ModifyClientVpnEndpoint) and change the VPC that's associated with it.
*
*
* @param associateClientVpnTargetNetworkRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateClientVpnTargetNetwork operation returned by the
* service.
* @sample AmazonEC2AsyncHandler.AssociateClientVpnTargetNetwork
* @see AWS API Documentation
*/
java.util.concurrent.Future associateClientVpnTargetNetworkAsync(
AssociateClientVpnTargetNetworkRequest associateClientVpnTargetNetworkRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates a set of DHCP options (that you've previously created) with the specified VPC, or associates no DHCP
* options with the VPC.
*
*
* After you associate the options with the VPC, any existing instances and all new instances that you launch in
* that VPC use the options. You don't need to restart or relaunch the instances. They automatically pick up the
* changes within a few hours, depending on how frequently the instance renews its DHCP lease. You can explicitly
* renew the lease using the operating system on the instance.
*
*
* For more information, see DHCP
* options sets in the Amazon Virtual Private Cloud User Guide.
*
*
* @param associateDhcpOptionsRequest
* @return A Java Future containing the result of the AssociateDhcpOptions operation returned by the service.
* @sample AmazonEC2Async.AssociateDhcpOptions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future associateDhcpOptionsAsync(AssociateDhcpOptionsRequest associateDhcpOptionsRequest);
/**
*
* Associates a set of DHCP options (that you've previously created) with the specified VPC, or associates no DHCP
* options with the VPC.
*
*
* After you associate the options with the VPC, any existing instances and all new instances that you launch in
* that VPC use the options. You don't need to restart or relaunch the instances. They automatically pick up the
* changes within a few hours, depending on how frequently the instance renews its DHCP lease. You can explicitly
* renew the lease using the operating system on the instance.
*
*
* For more information, see DHCP
* options sets in the Amazon Virtual Private Cloud User Guide.
*
*
* @param associateDhcpOptionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateDhcpOptions operation returned by the service.
* @sample AmazonEC2AsyncHandler.AssociateDhcpOptions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future associateDhcpOptionsAsync(AssociateDhcpOptionsRequest associateDhcpOptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates an Identity and Access Management (IAM) role with an Certificate Manager (ACM) certificate. This
* enables the certificate to be used by the ACM for Nitro Enclaves application inside an enclave. For more
* information, see Certificate
* Manager for Nitro Enclaves in the Amazon Web Services Nitro Enclaves User Guide.
*
*
* When the IAM role is associated with the ACM certificate, the certificate, certificate chain, and encrypted
* private key are placed in an Amazon S3 location that only the associated IAM role can access. The private key of
* the certificate is encrypted with an Amazon Web Services managed key that has an attached attestation-based key
* policy.
*
*
* To enable the IAM role to access the Amazon S3 object, you must grant it permission to call
* s3:GetObject
on the Amazon S3 bucket returned by the command. To enable the IAM role to access the
* KMS key, you must grant it permission to call kms:Decrypt
on the KMS key returned by the command.
* For more information, see Grant the role
* permission to access the certificate and encryption key in the Amazon Web Services Nitro Enclaves User
* Guide.
*
*
* @param associateEnclaveCertificateIamRoleRequest
* @return A Java Future containing the result of the AssociateEnclaveCertificateIamRole operation returned by the
* service.
* @sample AmazonEC2Async.AssociateEnclaveCertificateIamRole
* @see AWS API Documentation
*/
java.util.concurrent.Future associateEnclaveCertificateIamRoleAsync(
AssociateEnclaveCertificateIamRoleRequest associateEnclaveCertificateIamRoleRequest);
/**
*
* Associates an Identity and Access Management (IAM) role with an Certificate Manager (ACM) certificate. This
* enables the certificate to be used by the ACM for Nitro Enclaves application inside an enclave. For more
* information, see Certificate
* Manager for Nitro Enclaves in the Amazon Web Services Nitro Enclaves User Guide.
*
*
* When the IAM role is associated with the ACM certificate, the certificate, certificate chain, and encrypted
* private key are placed in an Amazon S3 location that only the associated IAM role can access. The private key of
* the certificate is encrypted with an Amazon Web Services managed key that has an attached attestation-based key
* policy.
*
*
* To enable the IAM role to access the Amazon S3 object, you must grant it permission to call
* s3:GetObject
on the Amazon S3 bucket returned by the command. To enable the IAM role to access the
* KMS key, you must grant it permission to call kms:Decrypt
on the KMS key returned by the command.
* For more information, see Grant the role
* permission to access the certificate and encryption key in the Amazon Web Services Nitro Enclaves User
* Guide.
*
*
* @param associateEnclaveCertificateIamRoleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateEnclaveCertificateIamRole operation returned by the
* service.
* @sample AmazonEC2AsyncHandler.AssociateEnclaveCertificateIamRole
* @see AWS API Documentation
*/
java.util.concurrent.Future associateEnclaveCertificateIamRoleAsync(
AssociateEnclaveCertificateIamRoleRequest associateEnclaveCertificateIamRoleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates an IAM instance profile with a running or stopped instance. You cannot associate more than one IAM
* instance profile with an instance.
*
*
* @param associateIamInstanceProfileRequest
* @return A Java Future containing the result of the AssociateIamInstanceProfile operation returned by the service.
* @sample AmazonEC2Async.AssociateIamInstanceProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future associateIamInstanceProfileAsync(
AssociateIamInstanceProfileRequest associateIamInstanceProfileRequest);
/**
*
* Associates an IAM instance profile with a running or stopped instance. You cannot associate more than one IAM
* instance profile with an instance.
*
*
* @param associateIamInstanceProfileRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateIamInstanceProfile operation returned by the service.
* @sample AmazonEC2AsyncHandler.AssociateIamInstanceProfile
* @see AWS API Documentation
*/
java.util.concurrent.Future associateIamInstanceProfileAsync(
AssociateIamInstanceProfileRequest associateIamInstanceProfileRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates one or more targets with an event window. Only one type of target (instance IDs, Dedicated Host IDs,
* or tags) can be specified with an event window.
*
*
* For more information, see Define
* event windows for scheduled events in the Amazon EC2 User Guide.
*
*
* @param associateInstanceEventWindowRequest
* @return A Java Future containing the result of the AssociateInstanceEventWindow operation returned by the
* service.
* @sample AmazonEC2Async.AssociateInstanceEventWindow
* @see AWS API Documentation
*/
java.util.concurrent.Future associateInstanceEventWindowAsync(
AssociateInstanceEventWindowRequest associateInstanceEventWindowRequest);
/**
*
* Associates one or more targets with an event window. Only one type of target (instance IDs, Dedicated Host IDs,
* or tags) can be specified with an event window.
*
*
* For more information, see Define
* event windows for scheduled events in the Amazon EC2 User Guide.
*
*
* @param associateInstanceEventWindowRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateInstanceEventWindow operation returned by the
* service.
* @sample AmazonEC2AsyncHandler.AssociateInstanceEventWindow
* @see AWS API Documentation
*/
java.util.concurrent.Future associateInstanceEventWindowAsync(
AssociateInstanceEventWindowRequest associateInstanceEventWindowRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates an IPAM resource discovery with an Amazon VPC IPAM. A resource discovery is an IPAM component that
* enables IPAM to manage and monitor resources that belong to the owning account.
*
*
* @param associateIpamResourceDiscoveryRequest
* @return A Java Future containing the result of the AssociateIpamResourceDiscovery operation returned by the
* service.
* @sample AmazonEC2Async.AssociateIpamResourceDiscovery
* @see AWS API Documentation
*/
java.util.concurrent.Future associateIpamResourceDiscoveryAsync(
AssociateIpamResourceDiscoveryRequest associateIpamResourceDiscoveryRequest);
/**
*
* Associates an IPAM resource discovery with an Amazon VPC IPAM. A resource discovery is an IPAM component that
* enables IPAM to manage and monitor resources that belong to the owning account.
*
*
* @param associateIpamResourceDiscoveryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateIpamResourceDiscovery operation returned by the
* service.
* @sample AmazonEC2AsyncHandler.AssociateIpamResourceDiscovery
* @see AWS API Documentation
*/
java.util.concurrent.Future associateIpamResourceDiscoveryAsync(
AssociateIpamResourceDiscoveryRequest associateIpamResourceDiscoveryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates Elastic IP addresses (EIPs) and private IPv4 addresses with a public NAT gateway. For more
* information, see Work with
* NAT gateways in the Amazon Virtual Private Cloud User Guide.
*
*
* By default, you can associate up to 2 Elastic IP addresses per public NAT gateway. You can increase the limit by
* requesting a quota adjustment. For more information, see Elastic IP address
* quotas in the Amazon Virtual Private Cloud User Guide.
*
*
* @param associateNatGatewayAddressRequest
* @return A Java Future containing the result of the AssociateNatGatewayAddress operation returned by the service.
* @sample AmazonEC2Async.AssociateNatGatewayAddress
* @see AWS
* API Documentation
*/
java.util.concurrent.Future associateNatGatewayAddressAsync(
AssociateNatGatewayAddressRequest associateNatGatewayAddressRequest);
/**
*
* Associates Elastic IP addresses (EIPs) and private IPv4 addresses with a public NAT gateway. For more
* information, see Work with
* NAT gateways in the Amazon Virtual Private Cloud User Guide.
*
*
* By default, you can associate up to 2 Elastic IP addresses per public NAT gateway. You can increase the limit by
* requesting a quota adjustment. For more information, see Elastic IP address
* quotas in the Amazon Virtual Private Cloud User Guide.
*
*
* @param associateNatGatewayAddressRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateNatGatewayAddress operation returned by the service.
* @sample AmazonEC2AsyncHandler.AssociateNatGatewayAddress
* @see AWS
* API Documentation
*/
java.util.concurrent.Future associateNatGatewayAddressAsync(
AssociateNatGatewayAddressRequest associateNatGatewayAddressRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates a subnet in your VPC or an internet gateway or virtual private gateway attached to your VPC with a
* route table in your VPC. This association causes traffic from the subnet or gateway to be routed according to the
* routes in the route table. The action returns an association ID, which you need in order to disassociate the
* route table later. A route table can be associated with multiple subnets.
*
*
* For more information, see Route
* tables in the Amazon Virtual Private Cloud User Guide.
*
*
* @param associateRouteTableRequest
* @return A Java Future containing the result of the AssociateRouteTable operation returned by the service.
* @sample AmazonEC2Async.AssociateRouteTable
* @see AWS API
* Documentation
*/
java.util.concurrent.Future associateRouteTableAsync(AssociateRouteTableRequest associateRouteTableRequest);
/**
*
* Associates a subnet in your VPC or an internet gateway or virtual private gateway attached to your VPC with a
* route table in your VPC. This association causes traffic from the subnet or gateway to be routed according to the
* routes in the route table. The action returns an association ID, which you need in order to disassociate the
* route table later. A route table can be associated with multiple subnets.
*
*
* For more information, see Route
* tables in the Amazon Virtual Private Cloud User Guide.
*
*
* @param associateRouteTableRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateRouteTable operation returned by the service.
* @sample AmazonEC2AsyncHandler.AssociateRouteTable
* @see AWS API
* Documentation
*/
java.util.concurrent.Future associateRouteTableAsync(AssociateRouteTableRequest associateRouteTableRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates a CIDR block with your subnet. You can only associate a single IPv6 CIDR block with your subnet. An
* IPv6 CIDR block must have a prefix length of /64.
*
*
* @param associateSubnetCidrBlockRequest
* @return A Java Future containing the result of the AssociateSubnetCidrBlock operation returned by the service.
* @sample AmazonEC2Async.AssociateSubnetCidrBlock
* @see AWS
* API Documentation
*/
java.util.concurrent.Future associateSubnetCidrBlockAsync(AssociateSubnetCidrBlockRequest associateSubnetCidrBlockRequest);
/**
*
* Associates a CIDR block with your subnet. You can only associate a single IPv6 CIDR block with your subnet. An
* IPv6 CIDR block must have a prefix length of /64.
*
*
* @param associateSubnetCidrBlockRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateSubnetCidrBlock operation returned by the service.
* @sample AmazonEC2AsyncHandler.AssociateSubnetCidrBlock
* @see AWS
* API Documentation
*/
java.util.concurrent.Future associateSubnetCidrBlockAsync(AssociateSubnetCidrBlockRequest associateSubnetCidrBlockRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates the specified subnets and transit gateway attachments with the specified transit gateway multicast
* domain.
*
*
* The transit gateway attachment must be in the available state before you can add a resource. Use DescribeTransitGatewayAttachments to see the state of the attachment.
*
*
* @param associateTransitGatewayMulticastDomainRequest
* @return A Java Future containing the result of the AssociateTransitGatewayMulticastDomain operation returned by
* the service.
* @sample AmazonEC2Async.AssociateTransitGatewayMulticastDomain
* @see AWS API Documentation
*/
java.util.concurrent.Future associateTransitGatewayMulticastDomainAsync(
AssociateTransitGatewayMulticastDomainRequest associateTransitGatewayMulticastDomainRequest);
/**
*
* Associates the specified subnets and transit gateway attachments with the specified transit gateway multicast
* domain.
*
*
* The transit gateway attachment must be in the available state before you can add a resource. Use DescribeTransitGatewayAttachments to see the state of the attachment.
*
*
* @param associateTransitGatewayMulticastDomainRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateTransitGatewayMulticastDomain operation returned by
* the service.
* @sample AmazonEC2AsyncHandler.AssociateTransitGatewayMulticastDomain
* @see AWS API Documentation
*/
java.util.concurrent.Future associateTransitGatewayMulticastDomainAsync(
AssociateTransitGatewayMulticastDomainRequest associateTransitGatewayMulticastDomainRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates the specified transit gateway attachment with a transit gateway policy table.
*
*
* @param associateTransitGatewayPolicyTableRequest
* @return A Java Future containing the result of the AssociateTransitGatewayPolicyTable operation returned by the
* service.
* @sample AmazonEC2Async.AssociateTransitGatewayPolicyTable
* @see AWS API Documentation
*/
java.util.concurrent.Future associateTransitGatewayPolicyTableAsync(
AssociateTransitGatewayPolicyTableRequest associateTransitGatewayPolicyTableRequest);
/**
*
* Associates the specified transit gateway attachment with a transit gateway policy table.
*
*
* @param associateTransitGatewayPolicyTableRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateTransitGatewayPolicyTable operation returned by the
* service.
* @sample AmazonEC2AsyncHandler.AssociateTransitGatewayPolicyTable
* @see AWS API Documentation
*/
java.util.concurrent.Future associateTransitGatewayPolicyTableAsync(
AssociateTransitGatewayPolicyTableRequest associateTransitGatewayPolicyTableRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates the specified attachment with the specified transit gateway route table. You can associate only one
* route table with an attachment.
*
*
* @param associateTransitGatewayRouteTableRequest
* @return A Java Future containing the result of the AssociateTransitGatewayRouteTable operation returned by the
* service.
* @sample AmazonEC2Async.AssociateTransitGatewayRouteTable
* @see AWS API Documentation
*/
java.util.concurrent.Future associateTransitGatewayRouteTableAsync(
AssociateTransitGatewayRouteTableRequest associateTransitGatewayRouteTableRequest);
/**
*
* Associates the specified attachment with the specified transit gateway route table. You can associate only one
* route table with an attachment.
*
*
* @param associateTransitGatewayRouteTableRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateTransitGatewayRouteTable operation returned by the
* service.
* @sample AmazonEC2AsyncHandler.AssociateTransitGatewayRouteTable
* @see AWS API Documentation
*/
java.util.concurrent.Future associateTransitGatewayRouteTableAsync(
AssociateTransitGatewayRouteTableRequest associateTransitGatewayRouteTableRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
*
* This API action is currently in limited preview only. If you are interested in using this feature, contact
* your account manager.
*
*
*
* Associates a branch network interface with a trunk network interface.
*
*
* Before you create the association, run the create-network-interface command and set --interface-type
to trunk
. You must also
* create a network interface for each branch network interface that you want to associate with the trunk network
* interface.
*
*
* @param associateTrunkInterfaceRequest
* @return A Java Future containing the result of the AssociateTrunkInterface operation returned by the service.
* @sample AmazonEC2Async.AssociateTrunkInterface
* @see AWS
* API Documentation
*/
java.util.concurrent.Future associateTrunkInterfaceAsync(AssociateTrunkInterfaceRequest associateTrunkInterfaceRequest);
/**
*
*
* This API action is currently in limited preview only. If you are interested in using this feature, contact
* your account manager.
*
*
*
* Associates a branch network interface with a trunk network interface.
*
*
* Before you create the association, run the create-network-interface command and set --interface-type
to trunk
. You must also
* create a network interface for each branch network interface that you want to associate with the trunk network
* interface.
*
*
* @param associateTrunkInterfaceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateTrunkInterface operation returned by the service.
* @sample AmazonEC2AsyncHandler.AssociateTrunkInterface
* @see AWS
* API Documentation
*/
java.util.concurrent.Future associateTrunkInterfaceAsync(AssociateTrunkInterfaceRequest associateTrunkInterfaceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates a CIDR block with your VPC. You can associate a secondary IPv4 CIDR block, an Amazon-provided IPv6
* CIDR block, or an IPv6 CIDR block from an IPv6 address pool that you provisioned through bring your own IP
* addresses (BYOIP). The IPv6 CIDR
* block size is fixed at /56.
*
*
* You must specify one of the following in the request: an IPv4 CIDR block, an IPv6 pool, or an Amazon-provided
* IPv6 CIDR block.
*
*
* For more information about associating CIDR blocks with your VPC and applicable restrictions, see VPC and subnet sizing in
* the Amazon Virtual Private Cloud User Guide.
*
*
* @param associateVpcCidrBlockRequest
* @return A Java Future containing the result of the AssociateVpcCidrBlock operation returned by the service.
* @sample AmazonEC2Async.AssociateVpcCidrBlock
* @see AWS API
* Documentation
*/
java.util.concurrent.Future associateVpcCidrBlockAsync(AssociateVpcCidrBlockRequest associateVpcCidrBlockRequest);
/**
*
* Associates a CIDR block with your VPC. You can associate a secondary IPv4 CIDR block, an Amazon-provided IPv6
* CIDR block, or an IPv6 CIDR block from an IPv6 address pool that you provisioned through bring your own IP
* addresses (BYOIP). The IPv6 CIDR
* block size is fixed at /56.
*
*
* You must specify one of the following in the request: an IPv4 CIDR block, an IPv6 pool, or an Amazon-provided
* IPv6 CIDR block.
*
*
* For more information about associating CIDR blocks with your VPC and applicable restrictions, see VPC and subnet sizing in
* the Amazon Virtual Private Cloud User Guide.
*
*
* @param associateVpcCidrBlockRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateVpcCidrBlock operation returned by the service.
* @sample AmazonEC2AsyncHandler.AssociateVpcCidrBlock
* @see AWS API
* Documentation
*/
java.util.concurrent.Future associateVpcCidrBlockAsync(AssociateVpcCidrBlockRequest associateVpcCidrBlockRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
*
* We are retiring EC2-Classic. We recommend that you migrate from EC2-Classic to a VPC. For more information, see
* Migrate from EC2-Classic to a
* VPC in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* Links an EC2-Classic instance to a ClassicLink-enabled VPC through one or more of the VPC's security groups. You
* cannot link an EC2-Classic instance to more than one VPC at a time. You can only link an instance that's in the
* running
state. An instance is automatically unlinked from a VPC when it's stopped - you can link it
* to the VPC again when you restart it.
*
*
* After you've linked an instance, you cannot change the VPC security groups that are associated with it. To change
* the security groups, you must first unlink the instance, and then link it again.
*
*
* Linking your instance to a VPC is sometimes referred to as attaching your instance.
*
*
* @param attachClassicLinkVpcRequest
* @return A Java Future containing the result of the AttachClassicLinkVpc operation returned by the service.
* @sample AmazonEC2Async.AttachClassicLinkVpc
* @see AWS API
* Documentation
*/
java.util.concurrent.Future attachClassicLinkVpcAsync(AttachClassicLinkVpcRequest attachClassicLinkVpcRequest);
/**
*
*
* We are retiring EC2-Classic. We recommend that you migrate from EC2-Classic to a VPC. For more information, see
* Migrate from EC2-Classic to a
* VPC in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* Links an EC2-Classic instance to a ClassicLink-enabled VPC through one or more of the VPC's security groups. You
* cannot link an EC2-Classic instance to more than one VPC at a time. You can only link an instance that's in the
* running
state. An instance is automatically unlinked from a VPC when it's stopped - you can link it
* to the VPC again when you restart it.
*
*
* After you've linked an instance, you cannot change the VPC security groups that are associated with it. To change
* the security groups, you must first unlink the instance, and then link it again.
*
*
* Linking your instance to a VPC is sometimes referred to as attaching your instance.
*
*
* @param attachClassicLinkVpcRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AttachClassicLinkVpc operation returned by the service.
* @sample AmazonEC2AsyncHandler.AttachClassicLinkVpc
* @see AWS API
* Documentation
*/
java.util.concurrent.Future attachClassicLinkVpcAsync(AttachClassicLinkVpcRequest attachClassicLinkVpcRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Attaches an internet gateway or a virtual private gateway to a VPC, enabling connectivity between the internet
* and the VPC. For more information about your VPC and internet gateway, see the Amazon Virtual Private Cloud User Guide.
*
*
* @param attachInternetGatewayRequest
* @return A Java Future containing the result of the AttachInternetGateway operation returned by the service.
* @sample AmazonEC2Async.AttachInternetGateway
* @see AWS API
* Documentation
*/
java.util.concurrent.Future attachInternetGatewayAsync(AttachInternetGatewayRequest attachInternetGatewayRequest);
/**
*
* Attaches an internet gateway or a virtual private gateway to a VPC, enabling connectivity between the internet
* and the VPC. For more information about your VPC and internet gateway, see the Amazon Virtual Private Cloud User Guide.
*
*
* @param attachInternetGatewayRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AttachInternetGateway operation returned by the service.
* @sample AmazonEC2AsyncHandler.AttachInternetGateway
* @see AWS API
* Documentation
*/
java.util.concurrent.Future attachInternetGatewayAsync(AttachInternetGatewayRequest attachInternetGatewayRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Attaches a network interface to an instance.
*
*
* @param attachNetworkInterfaceRequest
* Contains the parameters for AttachNetworkInterface.
* @return A Java Future containing the result of the AttachNetworkInterface operation returned by the service.
* @sample AmazonEC2Async.AttachNetworkInterface
* @see AWS API
* Documentation
*/
java.util.concurrent.Future attachNetworkInterfaceAsync(AttachNetworkInterfaceRequest attachNetworkInterfaceRequest);
/**
*
* Attaches a network interface to an instance.
*
*
* @param attachNetworkInterfaceRequest
* Contains the parameters for AttachNetworkInterface.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AttachNetworkInterface operation returned by the service.
* @sample AmazonEC2AsyncHandler.AttachNetworkInterface
* @see AWS API
* Documentation
*/
java.util.concurrent.Future attachNetworkInterfaceAsync(AttachNetworkInterfaceRequest attachNetworkInterfaceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Attaches the specified Amazon Web Services Verified Access trust provider to the specified Amazon Web Services
* Verified Access instance.
*
*
* @param attachVerifiedAccessTrustProviderRequest
* @return A Java Future containing the result of the AttachVerifiedAccessTrustProvider operation returned by the
* service.
* @sample AmazonEC2Async.AttachVerifiedAccessTrustProvider
* @see AWS API Documentation
*/
java.util.concurrent.Future attachVerifiedAccessTrustProviderAsync(
AttachVerifiedAccessTrustProviderRequest attachVerifiedAccessTrustProviderRequest);
/**
*
* Attaches the specified Amazon Web Services Verified Access trust provider to the specified Amazon Web Services
* Verified Access instance.
*
*
* @param attachVerifiedAccessTrustProviderRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AttachVerifiedAccessTrustProvider operation returned by the
* service.
* @sample AmazonEC2AsyncHandler.AttachVerifiedAccessTrustProvider
* @see AWS API Documentation
*/
java.util.concurrent.Future attachVerifiedAccessTrustProviderAsync(
AttachVerifiedAccessTrustProviderRequest attachVerifiedAccessTrustProviderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Attaches an EBS volume to a running or stopped instance and exposes it to the instance with the specified device
* name.
*
*
* Encrypted EBS volumes must be attached to instances that support Amazon EBS encryption. For more information, see
* Amazon EBS encryption in the
* Amazon Elastic Compute Cloud User Guide.
*
*
* After you attach an EBS volume, you must make it available. For more information, see Make an EBS volume available
* for use.
*
*
* If a volume has an Amazon Web Services Marketplace product code:
*
*
* -
*
* The volume can be attached only to a stopped instance.
*
*
* -
*
* Amazon Web Services Marketplace product codes are copied from the volume to the instance.
*
*
* -
*
* You must be subscribed to the product.
*
*
* -
*
* The instance type and operating system of the instance must support the product. For example, you can't detach a
* volume from a Windows instance and attach it to a Linux instance.
*
*
*
*
* For more information, see Attach an Amazon EBS volume
* to an instance in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param attachVolumeRequest
* @return A Java Future containing the result of the AttachVolume operation returned by the service.
* @sample AmazonEC2Async.AttachVolume
* @see AWS API
* Documentation
*/
java.util.concurrent.Future attachVolumeAsync(AttachVolumeRequest attachVolumeRequest);
/**
*
* Attaches an EBS volume to a running or stopped instance and exposes it to the instance with the specified device
* name.
*
*
* Encrypted EBS volumes must be attached to instances that support Amazon EBS encryption. For more information, see
* Amazon EBS encryption in the
* Amazon Elastic Compute Cloud User Guide.
*
*
* After you attach an EBS volume, you must make it available. For more information, see Make an EBS volume available
* for use.
*
*
* If a volume has an Amazon Web Services Marketplace product code:
*
*
* -
*
* The volume can be attached only to a stopped instance.
*
*
* -
*
* Amazon Web Services Marketplace product codes are copied from the volume to the instance.
*
*
* -
*
* You must be subscribed to the product.
*
*
* -
*
* The instance type and operating system of the instance must support the product. For example, you can't detach a
* volume from a Windows instance and attach it to a Linux instance.
*
*
*
*
* For more information, see Attach an Amazon EBS volume
* to an instance in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param attachVolumeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AttachVolume operation returned by the service.
* @sample AmazonEC2AsyncHandler.AttachVolume
* @see AWS API
* Documentation
*/
java.util.concurrent.Future attachVolumeAsync(AttachVolumeRequest attachVolumeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Attaches a virtual private gateway to a VPC. You can attach one virtual private gateway to one VPC at a time.
*
*
* For more information, see Amazon Web
* Services Site-to-Site VPN in the Amazon Web Services Site-to-Site VPN User Guide.
*
*
* @param attachVpnGatewayRequest
* Contains the parameters for AttachVpnGateway.
* @return A Java Future containing the result of the AttachVpnGateway operation returned by the service.
* @sample AmazonEC2Async.AttachVpnGateway
* @see AWS API
* Documentation
*/
java.util.concurrent.Future attachVpnGatewayAsync(AttachVpnGatewayRequest attachVpnGatewayRequest);
/**
*
* Attaches a virtual private gateway to a VPC. You can attach one virtual private gateway to one VPC at a time.
*
*
* For more information, see Amazon Web
* Services Site-to-Site VPN in the Amazon Web Services Site-to-Site VPN User Guide.
*
*
* @param attachVpnGatewayRequest
* Contains the parameters for AttachVpnGateway.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AttachVpnGateway operation returned by the service.
* @sample AmazonEC2AsyncHandler.AttachVpnGateway
* @see AWS API
* Documentation
*/
java.util.concurrent.Future attachVpnGatewayAsync(AttachVpnGatewayRequest attachVpnGatewayRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds an ingress authorization rule to a Client VPN endpoint. Ingress authorization rules act as firewall rules
* that grant access to networks. You must configure ingress authorization rules to enable clients to access
* resources in Amazon Web Services or on-premises networks.
*
*
* @param authorizeClientVpnIngressRequest
* @return A Java Future containing the result of the AuthorizeClientVpnIngress operation returned by the service.
* @sample AmazonEC2Async.AuthorizeClientVpnIngress
* @see AWS
* API Documentation
*/
java.util.concurrent.Future authorizeClientVpnIngressAsync(
AuthorizeClientVpnIngressRequest authorizeClientVpnIngressRequest);
/**
*
* Adds an ingress authorization rule to a Client VPN endpoint. Ingress authorization rules act as firewall rules
* that grant access to networks. You must configure ingress authorization rules to enable clients to access
* resources in Amazon Web Services or on-premises networks.
*
*
* @param authorizeClientVpnIngressRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AuthorizeClientVpnIngress operation returned by the service.
* @sample AmazonEC2AsyncHandler.AuthorizeClientVpnIngress
* @see AWS
* API Documentation
*/
java.util.concurrent.Future authorizeClientVpnIngressAsync(
AuthorizeClientVpnIngressRequest authorizeClientVpnIngressRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* [VPC only] Adds the specified outbound (egress) rules to a security group for use with a VPC.
*
*
* An outbound rule permits instances to send traffic to the specified IPv4 or IPv6 CIDR address ranges, or to the
* instances that are associated with the specified source security groups. When specifying an outbound rule for
* your security group in a VPC, the IpPermissions
must include a destination for the traffic.
*
*
* You specify a protocol for each rule (for example, TCP). For the TCP and UDP protocols, you must also specify the
* destination port or port range. For the ICMP protocol, you must also specify the ICMP type and code. You can use
* -1 for the type or code to mean all types or all codes.
*
*
* Rule changes are propagated to affected instances as quickly as possible. However, a small delay might occur.
*
*
* For information about VPC security group quotas, see Amazon VPC quotas.
*
*
* @param authorizeSecurityGroupEgressRequest
* @return A Java Future containing the result of the AuthorizeSecurityGroupEgress operation returned by the
* service.
* @sample AmazonEC2Async.AuthorizeSecurityGroupEgress
* @see AWS API Documentation
*/
java.util.concurrent.Future authorizeSecurityGroupEgressAsync(
AuthorizeSecurityGroupEgressRequest authorizeSecurityGroupEgressRequest);
/**
*
* [VPC only] Adds the specified outbound (egress) rules to a security group for use with a VPC.
*
*
* An outbound rule permits instances to send traffic to the specified IPv4 or IPv6 CIDR address ranges, or to the
* instances that are associated with the specified source security groups. When specifying an outbound rule for
* your security group in a VPC, the IpPermissions
must include a destination for the traffic.
*
*
* You specify a protocol for each rule (for example, TCP). For the TCP and UDP protocols, you must also specify the
* destination port or port range. For the ICMP protocol, you must also specify the ICMP type and code. You can use
* -1 for the type or code to mean all types or all codes.
*
*
* Rule changes are propagated to affected instances as quickly as possible. However, a small delay might occur.
*
*
* For information about VPC security group quotas, see Amazon VPC quotas.
*
*
* @param authorizeSecurityGroupEgressRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AuthorizeSecurityGroupEgress operation returned by the
* service.
* @sample AmazonEC2AsyncHandler.AuthorizeSecurityGroupEgress
* @see AWS API Documentation
*/
java.util.concurrent.Future authorizeSecurityGroupEgressAsync(
AuthorizeSecurityGroupEgressRequest authorizeSecurityGroupEgressRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds the specified inbound (ingress) rules to a security group.
*
*
* An inbound rule permits instances to receive traffic from the specified IPv4 or IPv6 CIDR address range, or from
* the instances that are associated with the specified destination security groups. When specifying an inbound rule
* for your security group in a VPC, the IpPermissions
must include a source for the traffic.
*
*
* You specify a protocol for each rule (for example, TCP). For TCP and UDP, you must also specify the destination
* port or port range. For ICMP/ICMPv6, you must also specify the ICMP/ICMPv6 type and code. You can use -1 to mean
* all types or all codes.
*
*
* Rule changes are propagated to instances within the security group as quickly as possible. However, a small delay
* might occur.
*
*
* For more information about VPC security group quotas, see Amazon VPC quotas.
*
*
*
* We are retiring EC2-Classic. We recommend that you migrate from EC2-Classic to a VPC. For more information, see
* Migrate from EC2-Classic to a
* VPC in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* @param authorizeSecurityGroupIngressRequest
* @return A Java Future containing the result of the AuthorizeSecurityGroupIngress operation returned by the
* service.
* @sample AmazonEC2Async.AuthorizeSecurityGroupIngress
* @see AWS API Documentation
*/
java.util.concurrent.Future authorizeSecurityGroupIngressAsync(
AuthorizeSecurityGroupIngressRequest authorizeSecurityGroupIngressRequest);
/**
*
* Adds the specified inbound (ingress) rules to a security group.
*
*
* An inbound rule permits instances to receive traffic from the specified IPv4 or IPv6 CIDR address range, or from
* the instances that are associated with the specified destination security groups. When specifying an inbound rule
* for your security group in a VPC, the IpPermissions
must include a source for the traffic.
*
*
* You specify a protocol for each rule (for example, TCP). For TCP and UDP, you must also specify the destination
* port or port range. For ICMP/ICMPv6, you must also specify the ICMP/ICMPv6 type and code. You can use -1 to mean
* all types or all codes.
*
*
* Rule changes are propagated to instances within the security group as quickly as possible. However, a small delay
* might occur.
*
*
* For more information about VPC security group quotas, see Amazon VPC quotas.
*
*
*
* We are retiring EC2-Classic. We recommend that you migrate from EC2-Classic to a VPC. For more information, see
* Migrate from EC2-Classic to a
* VPC in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* @param authorizeSecurityGroupIngressRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AuthorizeSecurityGroupIngress operation returned by the
* service.
* @sample AmazonEC2AsyncHandler.AuthorizeSecurityGroupIngress
* @see AWS API Documentation
*/
java.util.concurrent.Future authorizeSecurityGroupIngressAsync(
AuthorizeSecurityGroupIngressRequest authorizeSecurityGroupIngressRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Bundles an Amazon instance store-backed Windows instance.
*
*
* During bundling, only the root device volume (C:\) is bundled. Data on other instance store volumes is not
* preserved.
*
*
*
* This action is not applicable for Linux/Unix instances or Windows instances that are backed by Amazon EBS.
*
*
*
* @param bundleInstanceRequest
* Contains the parameters for BundleInstance.
* @return A Java Future containing the result of the BundleInstance operation returned by the service.
* @sample AmazonEC2Async.BundleInstance
* @see AWS API
* Documentation
*/
java.util.concurrent.Future bundleInstanceAsync(BundleInstanceRequest bundleInstanceRequest);
/**
*
* Bundles an Amazon instance store-backed Windows instance.
*
*
* During bundling, only the root device volume (C:\) is bundled. Data on other instance store volumes is not
* preserved.
*
*
*
* This action is not applicable for Linux/Unix instances or Windows instances that are backed by Amazon EBS.
*
*
*
* @param bundleInstanceRequest
* Contains the parameters for BundleInstance.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BundleInstance operation returned by the service.
* @sample AmazonEC2AsyncHandler.BundleInstance
* @see AWS API
* Documentation
*/
java.util.concurrent.Future bundleInstanceAsync(BundleInstanceRequest bundleInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels a bundling operation for an instance store-backed Windows instance.
*
*
* @param cancelBundleTaskRequest
* Contains the parameters for CancelBundleTask.
* @return A Java Future containing the result of the CancelBundleTask operation returned by the service.
* @sample AmazonEC2Async.CancelBundleTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelBundleTaskAsync(CancelBundleTaskRequest cancelBundleTaskRequest);
/**
*
* Cancels a bundling operation for an instance store-backed Windows instance.
*
*
* @param cancelBundleTaskRequest
* Contains the parameters for CancelBundleTask.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelBundleTask operation returned by the service.
* @sample AmazonEC2AsyncHandler.CancelBundleTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelBundleTaskAsync(CancelBundleTaskRequest cancelBundleTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels the specified Capacity Reservation, releases the reserved capacity, and changes the Capacity
* Reservation's state to cancelled
.
*
*
* Instances running in the reserved capacity continue running until you stop them. Stopped instances that target
* the Capacity Reservation can no longer launch. Modify these instances to either target a different Capacity
* Reservation, launch On-Demand Instance capacity, or run in any open Capacity Reservation that has matching
* attributes and sufficient capacity.
*
*
* @param cancelCapacityReservationRequest
* @return A Java Future containing the result of the CancelCapacityReservation operation returned by the service.
* @sample AmazonEC2Async.CancelCapacityReservation
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelCapacityReservationAsync(
CancelCapacityReservationRequest cancelCapacityReservationRequest);
/**
*
* Cancels the specified Capacity Reservation, releases the reserved capacity, and changes the Capacity
* Reservation's state to cancelled
.
*
*
* Instances running in the reserved capacity continue running until you stop them. Stopped instances that target
* the Capacity Reservation can no longer launch. Modify these instances to either target a different Capacity
* Reservation, launch On-Demand Instance capacity, or run in any open Capacity Reservation that has matching
* attributes and sufficient capacity.
*
*
* @param cancelCapacityReservationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelCapacityReservation operation returned by the service.
* @sample AmazonEC2AsyncHandler.CancelCapacityReservation
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelCapacityReservationAsync(
CancelCapacityReservationRequest cancelCapacityReservationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels one or more Capacity Reservation Fleets. When you cancel a Capacity Reservation Fleet, the following
* happens:
*
*
* -
*
* The Capacity Reservation Fleet's status changes to cancelled
.
*
*
* -
*
* The individual Capacity Reservations in the Fleet are cancelled. Instances running in the Capacity Reservations
* at the time of cancelling the Fleet continue to run in shared capacity.
*
*
* -
*
* The Fleet stops creating new Capacity Reservations.
*
*
*
*
* @param cancelCapacityReservationFleetsRequest
* @return A Java Future containing the result of the CancelCapacityReservationFleets operation returned by the
* service.
* @sample AmazonEC2Async.CancelCapacityReservationFleets
* @see AWS API Documentation
*/
java.util.concurrent.Future cancelCapacityReservationFleetsAsync(
CancelCapacityReservationFleetsRequest cancelCapacityReservationFleetsRequest);
/**
*
* Cancels one or more Capacity Reservation Fleets. When you cancel a Capacity Reservation Fleet, the following
* happens:
*
*
* -
*
* The Capacity Reservation Fleet's status changes to cancelled
.
*
*
* -
*
* The individual Capacity Reservations in the Fleet are cancelled. Instances running in the Capacity Reservations
* at the time of cancelling the Fleet continue to run in shared capacity.
*
*
* -
*
* The Fleet stops creating new Capacity Reservations.
*
*
*
*
* @param cancelCapacityReservationFleetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelCapacityReservationFleets operation returned by the
* service.
* @sample AmazonEC2AsyncHandler.CancelCapacityReservationFleets
* @see AWS API Documentation
*/
java.util.concurrent.Future cancelCapacityReservationFleetsAsync(
CancelCapacityReservationFleetsRequest cancelCapacityReservationFleetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels an active conversion task. The task can be the import of an instance or volume. The action removes all
* artifacts of the conversion, including a partially uploaded volume or instance. If the conversion is complete or
* is in the process of transferring the final disk image, the command fails and returns an exception.
*
*
* For more information, see Importing a
* Virtual Machine Using the Amazon EC2 CLI.
*
*
* @param cancelConversionTaskRequest
* @return A Java Future containing the result of the CancelConversionTask operation returned by the service.
* @sample AmazonEC2Async.CancelConversionTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelConversionTaskAsync(CancelConversionTaskRequest cancelConversionTaskRequest);
/**
*
* Cancels an active conversion task. The task can be the import of an instance or volume. The action removes all
* artifacts of the conversion, including a partially uploaded volume or instance. If the conversion is complete or
* is in the process of transferring the final disk image, the command fails and returns an exception.
*
*
* For more information, see Importing a
* Virtual Machine Using the Amazon EC2 CLI.
*
*
* @param cancelConversionTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelConversionTask operation returned by the service.
* @sample AmazonEC2AsyncHandler.CancelConversionTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelConversionTaskAsync(CancelConversionTaskRequest cancelConversionTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels an active export task. The request removes all artifacts of the export, including any partially-created
* Amazon S3 objects. If the export task is complete or is in the process of transferring the final disk image, the
* command fails and returns an error.
*
*
* @param cancelExportTaskRequest
* @return A Java Future containing the result of the CancelExportTask operation returned by the service.
* @sample AmazonEC2Async.CancelExportTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelExportTaskAsync(CancelExportTaskRequest cancelExportTaskRequest);
/**
*
* Cancels an active export task. The request removes all artifacts of the export, including any partially-created
* Amazon S3 objects. If the export task is complete or is in the process of transferring the final disk image, the
* command fails and returns an error.
*
*
* @param cancelExportTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelExportTask operation returned by the service.
* @sample AmazonEC2AsyncHandler.CancelExportTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelExportTaskAsync(CancelExportTaskRequest cancelExportTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes your Amazon Web Services account from the launch permissions for the specified AMI. For more information,
* see Cancel having an
* AMI shared with your Amazon Web Services account in the Amazon EC2 User Guide.
*
*
* @param cancelImageLaunchPermissionRequest
* @return A Java Future containing the result of the CancelImageLaunchPermission operation returned by the service.
* @sample AmazonEC2Async.CancelImageLaunchPermission
* @see AWS API Documentation
*/
java.util.concurrent.Future cancelImageLaunchPermissionAsync(
CancelImageLaunchPermissionRequest cancelImageLaunchPermissionRequest);
/**
*
* Removes your Amazon Web Services account from the launch permissions for the specified AMI. For more information,
* see Cancel having an
* AMI shared with your Amazon Web Services account in the Amazon EC2 User Guide.
*
*
* @param cancelImageLaunchPermissionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelImageLaunchPermission operation returned by the service.
* @sample AmazonEC2AsyncHandler.CancelImageLaunchPermission
* @see AWS API Documentation
*/
java.util.concurrent.Future cancelImageLaunchPermissionAsync(
CancelImageLaunchPermissionRequest cancelImageLaunchPermissionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels an in-process import virtual machine or import snapshot task.
*
*
* @param cancelImportTaskRequest
* @return A Java Future containing the result of the CancelImportTask operation returned by the service.
* @sample AmazonEC2Async.CancelImportTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelImportTaskAsync(CancelImportTaskRequest cancelImportTaskRequest);
/**
*
* Cancels an in-process import virtual machine or import snapshot task.
*
*
* @param cancelImportTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelImportTask operation returned by the service.
* @sample AmazonEC2AsyncHandler.CancelImportTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelImportTaskAsync(CancelImportTaskRequest cancelImportTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the CancelImportTask operation.
*
* @see #cancelImportTaskAsync(CancelImportTaskRequest)
*/
java.util.concurrent.Future cancelImportTaskAsync();
/**
* Simplified method form for invoking the CancelImportTask operation with an AsyncHandler.
*
* @see #cancelImportTaskAsync(CancelImportTaskRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future cancelImportTaskAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels the specified Reserved Instance listing in the Reserved Instance Marketplace.
*
*
* For more information, see Reserved Instance
* Marketplace in the Amazon EC2 User Guide.
*
*
* @param cancelReservedInstancesListingRequest
* Contains the parameters for CancelReservedInstancesListing.
* @return A Java Future containing the result of the CancelReservedInstancesListing operation returned by the
* service.
* @sample AmazonEC2Async.CancelReservedInstancesListing
* @see AWS API Documentation
*/
java.util.concurrent.Future cancelReservedInstancesListingAsync(
CancelReservedInstancesListingRequest cancelReservedInstancesListingRequest);
/**
*
* Cancels the specified Reserved Instance listing in the Reserved Instance Marketplace.
*
*
* For more information, see Reserved Instance
* Marketplace in the Amazon EC2 User Guide.
*
*
* @param cancelReservedInstancesListingRequest
* Contains the parameters for CancelReservedInstancesListing.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelReservedInstancesListing operation returned by the
* service.
* @sample AmazonEC2AsyncHandler.CancelReservedInstancesListing
* @see AWS API Documentation
*/
java.util.concurrent.Future cancelReservedInstancesListingAsync(
CancelReservedInstancesListingRequest cancelReservedInstancesListingRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels the specified Spot Fleet requests.
*
*
* After you cancel a Spot Fleet request, the Spot Fleet launches no new instances.
*
*
* You must also specify whether a canceled Spot Fleet request should terminate its instances. If you choose to
* terminate the instances, the Spot Fleet request enters the cancelled_terminating
state. Otherwise,
* the Spot Fleet request enters the cancelled_running
state and the instances continue to run until
* they are interrupted or you terminate them manually.
*
*
* @param cancelSpotFleetRequestsRequest
* Contains the parameters for CancelSpotFleetRequests.
* @return A Java Future containing the result of the CancelSpotFleetRequests operation returned by the service.
* @sample AmazonEC2Async.CancelSpotFleetRequests
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelSpotFleetRequestsAsync(CancelSpotFleetRequestsRequest cancelSpotFleetRequestsRequest);
/**
*
* Cancels the specified Spot Fleet requests.
*
*
* After you cancel a Spot Fleet request, the Spot Fleet launches no new instances.
*
*
* You must also specify whether a canceled Spot Fleet request should terminate its instances. If you choose to
* terminate the instances, the Spot Fleet request enters the cancelled_terminating
state. Otherwise,
* the Spot Fleet request enters the cancelled_running
state and the instances continue to run until
* they are interrupted or you terminate them manually.
*
*
* @param cancelSpotFleetRequestsRequest
* Contains the parameters for CancelSpotFleetRequests.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelSpotFleetRequests operation returned by the service.
* @sample AmazonEC2AsyncHandler.CancelSpotFleetRequests
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelSpotFleetRequestsAsync(CancelSpotFleetRequestsRequest cancelSpotFleetRequestsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels one or more Spot Instance requests.
*
*
*
* Canceling a Spot Instance request does not terminate running Spot Instances associated with the request.
*
*
*
* @param cancelSpotInstanceRequestsRequest
* Contains the parameters for CancelSpotInstanceRequests.
* @return A Java Future containing the result of the CancelSpotInstanceRequests operation returned by the service.
* @sample AmazonEC2Async.CancelSpotInstanceRequests
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelSpotInstanceRequestsAsync(
CancelSpotInstanceRequestsRequest cancelSpotInstanceRequestsRequest);
/**
*
* Cancels one or more Spot Instance requests.
*
*
*
* Canceling a Spot Instance request does not terminate running Spot Instances associated with the request.
*
*
*
* @param cancelSpotInstanceRequestsRequest
* Contains the parameters for CancelSpotInstanceRequests.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelSpotInstanceRequests operation returned by the service.
* @sample AmazonEC2AsyncHandler.CancelSpotInstanceRequests
* @see AWS
* API Documentation
*/
java.util.concurrent.Future cancelSpotInstanceRequestsAsync(
CancelSpotInstanceRequestsRequest cancelSpotInstanceRequestsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Determines whether a product code is associated with an instance. This action can only be used by the owner of
* the product code. It is useful when a product code owner must verify whether another user's instance is eligible
* for support.
*
*
* @param confirmProductInstanceRequest
* @return A Java Future containing the result of the ConfirmProductInstance operation returned by the service.
* @sample AmazonEC2Async.ConfirmProductInstance
* @see AWS API
* Documentation
*/
java.util.concurrent.Future confirmProductInstanceAsync(ConfirmProductInstanceRequest confirmProductInstanceRequest);
/**
*
* Determines whether a product code is associated with an instance. This action can only be used by the owner of
* the product code. It is useful when a product code owner must verify whether another user's instance is eligible
* for support.
*
*
* @param confirmProductInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ConfirmProductInstance operation returned by the service.
* @sample AmazonEC2AsyncHandler.ConfirmProductInstance
* @see AWS API
* Documentation
*/
java.util.concurrent.Future confirmProductInstanceAsync(ConfirmProductInstanceRequest confirmProductInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Copies the specified Amazon FPGA Image (AFI) to the current Region.
*
*
* @param copyFpgaImageRequest
* @return A Java Future containing the result of the CopyFpgaImage operation returned by the service.
* @sample AmazonEC2Async.CopyFpgaImage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future copyFpgaImageAsync(CopyFpgaImageRequest copyFpgaImageRequest);
/**
*
* Copies the specified Amazon FPGA Image (AFI) to the current Region.
*
*
* @param copyFpgaImageRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CopyFpgaImage operation returned by the service.
* @sample AmazonEC2AsyncHandler.CopyFpgaImage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future copyFpgaImageAsync(CopyFpgaImageRequest copyFpgaImageRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Initiates the copy of an AMI. You can copy an AMI from one Region to another, or from a Region to an Outpost. You
* can't copy an AMI from an Outpost to a Region, from one Outpost to another, or within the same Outpost. To copy
* an AMI to another partition, see CreateStoreImageTask.
*
*
* To copy an AMI from one Region to another, specify the source Region using the SourceRegion parameter, and
* specify the destination Region using its endpoint. Copies of encrypted backing snapshots for the AMI are
* encrypted. Copies of unencrypted backing snapshots remain unencrypted, unless you set Encrypted
* during the copy operation. You cannot create an unencrypted copy of an encrypted backing snapshot.
*
*
* To copy an AMI from a Region to an Outpost, specify the source Region using the SourceRegion parameter,
* and specify the ARN of the destination Outpost using DestinationOutpostArn. Backing snapshots copied to an
* Outpost are encrypted by default using the default encryption key for the Region, or a different key that you
* specify in the request using KmsKeyId. Outposts do not support unencrypted snapshots. For more
* information, Amazon
* EBS local snapshots on Outposts in the Amazon EC2 User Guide.
*
*
* For more information about the prerequisites and limits when copying an AMI, see Copy an AMI in the Amazon EC2
* User Guide.
*
*
* @param copyImageRequest
* Contains the parameters for CopyImage.
* @return A Java Future containing the result of the CopyImage operation returned by the service.
* @sample AmazonEC2Async.CopyImage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future copyImageAsync(CopyImageRequest copyImageRequest);
/**
*
* Initiates the copy of an AMI. You can copy an AMI from one Region to another, or from a Region to an Outpost. You
* can't copy an AMI from an Outpost to a Region, from one Outpost to another, or within the same Outpost. To copy
* an AMI to another partition, see CreateStoreImageTask.
*
*
* To copy an AMI from one Region to another, specify the source Region using the SourceRegion parameter, and
* specify the destination Region using its endpoint. Copies of encrypted backing snapshots for the AMI are
* encrypted. Copies of unencrypted backing snapshots remain unencrypted, unless you set Encrypted
* during the copy operation. You cannot create an unencrypted copy of an encrypted backing snapshot.
*
*
* To copy an AMI from a Region to an Outpost, specify the source Region using the SourceRegion parameter,
* and specify the ARN of the destination Outpost using DestinationOutpostArn. Backing snapshots copied to an
* Outpost are encrypted by default using the default encryption key for the Region, or a different key that you
* specify in the request using KmsKeyId. Outposts do not support unencrypted snapshots. For more
* information, Amazon
* EBS local snapshots on Outposts in the Amazon EC2 User Guide.
*
*
* For more information about the prerequisites and limits when copying an AMI, see Copy an AMI in the Amazon EC2
* User Guide.
*
*
* @param copyImageRequest
* Contains the parameters for CopyImage.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CopyImage operation returned by the service.
* @sample AmazonEC2AsyncHandler.CopyImage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future copyImageAsync(CopyImageRequest copyImageRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Copies a point-in-time snapshot of an EBS volume and stores it in Amazon S3. You can copy a snapshot within the
* same Region, from one Region to another, or from a Region to an Outpost. You can't copy a snapshot from an
* Outpost to a Region, from one Outpost to another, or within the same Outpost.
*
*
* You can use the snapshot to create EBS volumes or Amazon Machine Images (AMIs).
*
*
* When copying snapshots to a Region, copies of encrypted EBS snapshots remain encrypted. Copies of unencrypted
* snapshots remain unencrypted, unless you enable encryption for the snapshot copy operation. By default, encrypted
* snapshot copies use the default Key Management Service (KMS) KMS key; however, you can specify a different KMS
* key. To copy an encrypted snapshot that has been shared from another account, you must have permissions for the
* KMS key used to encrypt the snapshot.
*
*
* Snapshots copied to an Outpost are encrypted by default using the default encryption key for the Region, or a
* different key that you specify in the request using KmsKeyId. Outposts do not support unencrypted
* snapshots. For more information, Amazon EBS local
* snapshots on Outposts in the Amazon Elastic Compute Cloud User Guide.
*
*
* Snapshots created by copying another snapshot have an arbitrary volume ID that should not be used for any
* purpose.
*
*
* For more information, see Copy an Amazon EBS snapshot
* in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param copySnapshotRequest
* @return A Java Future containing the result of the CopySnapshot operation returned by the service.
* @sample AmazonEC2Async.CopySnapshot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future copySnapshotAsync(CopySnapshotRequest copySnapshotRequest);
/**
*
* Copies a point-in-time snapshot of an EBS volume and stores it in Amazon S3. You can copy a snapshot within the
* same Region, from one Region to another, or from a Region to an Outpost. You can't copy a snapshot from an
* Outpost to a Region, from one Outpost to another, or within the same Outpost.
*
*
* You can use the snapshot to create EBS volumes or Amazon Machine Images (AMIs).
*
*
* When copying snapshots to a Region, copies of encrypted EBS snapshots remain encrypted. Copies of unencrypted
* snapshots remain unencrypted, unless you enable encryption for the snapshot copy operation. By default, encrypted
* snapshot copies use the default Key Management Service (KMS) KMS key; however, you can specify a different KMS
* key. To copy an encrypted snapshot that has been shared from another account, you must have permissions for the
* KMS key used to encrypt the snapshot.
*
*
* Snapshots copied to an Outpost are encrypted by default using the default encryption key for the Region, or a
* different key that you specify in the request using KmsKeyId. Outposts do not support unencrypted
* snapshots. For more information, Amazon EBS local
* snapshots on Outposts in the Amazon Elastic Compute Cloud User Guide.
*
*
* Snapshots created by copying another snapshot have an arbitrary volume ID that should not be used for any
* purpose.
*
*
* For more information, see Copy an Amazon EBS snapshot
* in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param copySnapshotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CopySnapshot operation returned by the service.
* @sample AmazonEC2AsyncHandler.CopySnapshot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future copySnapshotAsync(CopySnapshotRequest copySnapshotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new Capacity Reservation with the specified attributes.
*
*
* Capacity Reservations enable you to reserve capacity for your Amazon EC2 instances in a specific Availability
* Zone for any duration. This gives you the flexibility to selectively add capacity reservations and still get the
* Regional RI discounts for that usage. By creating Capacity Reservations, you ensure that you always have access
* to Amazon EC2 capacity when you need it, for as long as you need it. For more information, see Capacity
* Reservations in the Amazon EC2 User Guide.
*
*
* Your request to create a Capacity Reservation could fail if Amazon EC2 does not have sufficient capacity to
* fulfill the request. If your request fails due to Amazon EC2 capacity constraints, either try again at a later
* time, try in a different Availability Zone, or request a smaller capacity reservation. If your application is
* flexible across instance types and sizes, try to create a Capacity Reservation with different instance
* attributes.
*
*
* Your request could also fail if the requested quantity exceeds your On-Demand Instance limit for the selected
* instance type. If your request fails due to limit constraints, increase your On-Demand Instance limit for the
* required instance type and try again. For more information about increasing your instance limits, see Amazon EC2 Service Quotas
* in the Amazon EC2 User Guide.
*
*
* @param createCapacityReservationRequest
* @return A Java Future containing the result of the CreateCapacityReservation operation returned by the service.
* @sample AmazonEC2Async.CreateCapacityReservation
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createCapacityReservationAsync(
CreateCapacityReservationRequest createCapacityReservationRequest);
/**
*
* Creates a new Capacity Reservation with the specified attributes.
*
*
* Capacity Reservations enable you to reserve capacity for your Amazon EC2 instances in a specific Availability
* Zone for any duration. This gives you the flexibility to selectively add capacity reservations and still get the
* Regional RI discounts for that usage. By creating Capacity Reservations, you ensure that you always have access
* to Amazon EC2 capacity when you need it, for as long as you need it. For more information, see Capacity
* Reservations in the Amazon EC2 User Guide.
*
*
* Your request to create a Capacity Reservation could fail if Amazon EC2 does not have sufficient capacity to
* fulfill the request. If your request fails due to Amazon EC2 capacity constraints, either try again at a later
* time, try in a different Availability Zone, or request a smaller capacity reservation. If your application is
* flexible across instance types and sizes, try to create a Capacity Reservation with different instance
* attributes.
*
*
* Your request could also fail if the requested quantity exceeds your On-Demand Instance limit for the selected
* instance type. If your request fails due to limit constraints, increase your On-Demand Instance limit for the
* required instance type and try again. For more information about increasing your instance limits, see Amazon EC2 Service Quotas
* in the Amazon EC2 User Guide.
*
*
* @param createCapacityReservationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateCapacityReservation operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateCapacityReservation
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createCapacityReservationAsync(
CreateCapacityReservationRequest createCapacityReservationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a Capacity Reservation Fleet. For more information, see Create a
* Capacity Reservation Fleet in the Amazon EC2 User Guide.
*
*
* @param createCapacityReservationFleetRequest
* @return A Java Future containing the result of the CreateCapacityReservationFleet operation returned by the
* service.
* @sample AmazonEC2Async.CreateCapacityReservationFleet
* @see AWS API Documentation
*/
java.util.concurrent.Future createCapacityReservationFleetAsync(
CreateCapacityReservationFleetRequest createCapacityReservationFleetRequest);
/**
*
* Creates a Capacity Reservation Fleet. For more information, see Create a
* Capacity Reservation Fleet in the Amazon EC2 User Guide.
*
*
* @param createCapacityReservationFleetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateCapacityReservationFleet operation returned by the
* service.
* @sample AmazonEC2AsyncHandler.CreateCapacityReservationFleet
* @see AWS API Documentation
*/
java.util.concurrent.Future createCapacityReservationFleetAsync(
CreateCapacityReservationFleetRequest createCapacityReservationFleetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a carrier gateway. For more information about carrier gateways, see Carrier gateways in the Amazon Web Services Wavelength Developer Guide.
*
*
* @param createCarrierGatewayRequest
* @return A Java Future containing the result of the CreateCarrierGateway operation returned by the service.
* @sample AmazonEC2Async.CreateCarrierGateway
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createCarrierGatewayAsync(CreateCarrierGatewayRequest createCarrierGatewayRequest);
/**
*
* Creates a carrier gateway. For more information about carrier gateways, see Carrier gateways in the Amazon Web Services Wavelength Developer Guide.
*
*
* @param createCarrierGatewayRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateCarrierGateway operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateCarrierGateway
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createCarrierGatewayAsync(CreateCarrierGatewayRequest createCarrierGatewayRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a Client VPN endpoint. A Client VPN endpoint is the resource you create and configure to enable and
* manage client VPN sessions. It is the destination endpoint at which all client VPN sessions are terminated.
*
*
* @param createClientVpnEndpointRequest
* @return A Java Future containing the result of the CreateClientVpnEndpoint operation returned by the service.
* @sample AmazonEC2Async.CreateClientVpnEndpoint
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createClientVpnEndpointAsync(CreateClientVpnEndpointRequest createClientVpnEndpointRequest);
/**
*
* Creates a Client VPN endpoint. A Client VPN endpoint is the resource you create and configure to enable and
* manage client VPN sessions. It is the destination endpoint at which all client VPN sessions are terminated.
*
*
* @param createClientVpnEndpointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateClientVpnEndpoint operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateClientVpnEndpoint
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createClientVpnEndpointAsync(CreateClientVpnEndpointRequest createClientVpnEndpointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds a route to a network to a Client VPN endpoint. Each Client VPN endpoint has a route table that describes the
* available destination network routes. Each route in the route table specifies the path for traffic to specific
* resources or networks.
*
*
* @param createClientVpnRouteRequest
* @return A Java Future containing the result of the CreateClientVpnRoute operation returned by the service.
* @sample AmazonEC2Async.CreateClientVpnRoute
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createClientVpnRouteAsync(CreateClientVpnRouteRequest createClientVpnRouteRequest);
/**
*
* Adds a route to a network to a Client VPN endpoint. Each Client VPN endpoint has a route table that describes the
* available destination network routes. Each route in the route table specifies the path for traffic to specific
* resources or networks.
*
*
* @param createClientVpnRouteRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateClientVpnRoute operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateClientVpnRoute
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createClientVpnRouteAsync(CreateClientVpnRouteRequest createClientVpnRouteRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a range of customer-owned IP addresses.
*
*
* @param createCoipCidrRequest
* @return A Java Future containing the result of the CreateCoipCidr operation returned by the service.
* @sample AmazonEC2Async.CreateCoipCidr
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createCoipCidrAsync(CreateCoipCidrRequest createCoipCidrRequest);
/**
*
* Creates a range of customer-owned IP addresses.
*
*
* @param createCoipCidrRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateCoipCidr operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateCoipCidr
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createCoipCidrAsync(CreateCoipCidrRequest createCoipCidrRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a pool of customer-owned IP (CoIP) addresses.
*
*
* @param createCoipPoolRequest
* @return A Java Future containing the result of the CreateCoipPool operation returned by the service.
* @sample AmazonEC2Async.CreateCoipPool
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createCoipPoolAsync(CreateCoipPoolRequest createCoipPoolRequest);
/**
*
* Creates a pool of customer-owned IP (CoIP) addresses.
*
*
* @param createCoipPoolRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateCoipPool operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateCoipPool
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createCoipPoolAsync(CreateCoipPoolRequest createCoipPoolRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Provides information to Amazon Web Services about your customer gateway device. The customer gateway device is
* the appliance at your end of the VPN connection. You must provide the IP address of the customer gateway device’s
* external interface. The IP address must be static and can be behind a device performing network address
* translation (NAT).
*
*
* For devices that use Border Gateway Protocol (BGP), you can also provide the device's BGP Autonomous System
* Number (ASN). You can use an existing ASN assigned to your network. If you don't have an ASN already, you can use
* a private ASN. For more information, see Customer gateway options for your
* Site-to-Site VPN connection in the Amazon Web Services Site-to-Site VPN User Guide.
*
*
* To create more than one customer gateway with the same VPN type, IP address, and BGP ASN, specify a unique device
* name for each customer gateway. An identical request returns information about the existing customer gateway; it
* doesn't create a new customer gateway.
*
*
* @param createCustomerGatewayRequest
* Contains the parameters for CreateCustomerGateway.
* @return A Java Future containing the result of the CreateCustomerGateway operation returned by the service.
* @sample AmazonEC2Async.CreateCustomerGateway
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createCustomerGatewayAsync(CreateCustomerGatewayRequest createCustomerGatewayRequest);
/**
*
* Provides information to Amazon Web Services about your customer gateway device. The customer gateway device is
* the appliance at your end of the VPN connection. You must provide the IP address of the customer gateway device’s
* external interface. The IP address must be static and can be behind a device performing network address
* translation (NAT).
*
*
* For devices that use Border Gateway Protocol (BGP), you can also provide the device's BGP Autonomous System
* Number (ASN). You can use an existing ASN assigned to your network. If you don't have an ASN already, you can use
* a private ASN. For more information, see Customer gateway options for your
* Site-to-Site VPN connection in the Amazon Web Services Site-to-Site VPN User Guide.
*
*
* To create more than one customer gateway with the same VPN type, IP address, and BGP ASN, specify a unique device
* name for each customer gateway. An identical request returns information about the existing customer gateway; it
* doesn't create a new customer gateway.
*
*
* @param createCustomerGatewayRequest
* Contains the parameters for CreateCustomerGateway.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateCustomerGateway operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateCustomerGateway
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createCustomerGatewayAsync(CreateCustomerGatewayRequest createCustomerGatewayRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a default subnet with a size /20
IPv4 CIDR block in the specified Availability Zone in your
* default VPC. You can have only one default subnet per Availability Zone. For more information, see Creating a default
* subnet in the Amazon Virtual Private Cloud User Guide.
*
*
* @param createDefaultSubnetRequest
* @return A Java Future containing the result of the CreateDefaultSubnet operation returned by the service.
* @sample AmazonEC2Async.CreateDefaultSubnet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDefaultSubnetAsync(CreateDefaultSubnetRequest createDefaultSubnetRequest);
/**
*
* Creates a default subnet with a size /20
IPv4 CIDR block in the specified Availability Zone in your
* default VPC. You can have only one default subnet per Availability Zone. For more information, see Creating a default
* subnet in the Amazon Virtual Private Cloud User Guide.
*
*
* @param createDefaultSubnetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDefaultSubnet operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateDefaultSubnet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDefaultSubnetAsync(CreateDefaultSubnetRequest createDefaultSubnetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a default VPC with a size /16
IPv4 CIDR block and a default subnet in each Availability
* Zone. For more information about the components of a default VPC, see Default VPC and default subnets in
* the Amazon Virtual Private Cloud User Guide. You cannot specify the components of the default VPC
* yourself.
*
*
* If you deleted your previous default VPC, you can create a default VPC. You cannot have more than one default VPC
* per Region.
*
*
* If your account supports EC2-Classic, you cannot use this action to create a default VPC in a Region that
* supports EC2-Classic. If you want a default VPC in a Region that supports EC2-Classic, see
* "I really want a default VPC for my existing EC2 account. Is that possible?" in the Default VPCs FAQ.
*
*
*
* We are retiring EC2-Classic. We recommend that you migrate from EC2-Classic to a VPC. For more information, see
* Migrate from EC2-Classic to a
* VPC in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* @param createDefaultVpcRequest
* @return A Java Future containing the result of the CreateDefaultVpc operation returned by the service.
* @sample AmazonEC2Async.CreateDefaultVpc
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDefaultVpcAsync(CreateDefaultVpcRequest createDefaultVpcRequest);
/**
*
* Creates a default VPC with a size /16
IPv4 CIDR block and a default subnet in each Availability
* Zone. For more information about the components of a default VPC, see Default VPC and default subnets in
* the Amazon Virtual Private Cloud User Guide. You cannot specify the components of the default VPC
* yourself.
*
*
* If you deleted your previous default VPC, you can create a default VPC. You cannot have more than one default VPC
* per Region.
*
*
* If your account supports EC2-Classic, you cannot use this action to create a default VPC in a Region that
* supports EC2-Classic. If you want a default VPC in a Region that supports EC2-Classic, see
* "I really want a default VPC for my existing EC2 account. Is that possible?" in the Default VPCs FAQ.
*
*
*
* We are retiring EC2-Classic. We recommend that you migrate from EC2-Classic to a VPC. For more information, see
* Migrate from EC2-Classic to a
* VPC in the Amazon Elastic Compute Cloud User Guide.
*
*
*
* @param createDefaultVpcRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDefaultVpc operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateDefaultVpc
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDefaultVpcAsync(CreateDefaultVpcRequest createDefaultVpcRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a set of DHCP options for your VPC. After creating the set, you must associate it with the VPC, causing
* all existing and new instances that you launch in the VPC to use this set of DHCP options. The following are the
* individual DHCP options you can specify. For more information about the options, see RFC 2132.
*
*
* -
*
* domain-name-servers
- The IP addresses of up to four domain name servers, or AmazonProvidedDNS. The
* default DHCP option set specifies AmazonProvidedDNS. If specifying more than one domain name server, specify the
* IP addresses in a single parameter, separated by commas. To have your instance receive a custom DNS hostname as
* specified in domain-name
, you must set domain-name-servers
to a custom DNS server.
*
*
* -
*
* domain-name
- If you're using AmazonProvidedDNS in us-east-1
, specify
* ec2.internal
. If you're using AmazonProvidedDNS in another Region, specify
* region.compute.internal
(for example, ap-northeast-1.compute.internal
). Otherwise,
* specify a domain name (for example, ExampleCompany.com
). This value is used to complete unqualified
* DNS hostnames. Important: Some Linux operating systems accept multiple domain names separated by spaces.
* However, Windows and other Linux operating systems treat the value as a single domain, which results in
* unexpected behavior. If your DHCP options set is associated with a VPC that has instances with multiple operating
* systems, specify only one domain name.
*
*
* -
*
* ntp-servers
- The IP addresses of up to four Network Time Protocol (NTP) servers.
*
*
* -
*
* netbios-name-servers
- The IP addresses of up to four NetBIOS name servers.
*
*
* -
*
* netbios-node-type
- The NetBIOS node type (1, 2, 4, or 8). We recommend that you specify 2
* (broadcast and multicast are not currently supported). For more information about these node types, see RFC 2132.
*
*
*
*
* Your VPC automatically starts out with a set of DHCP options that includes only a DNS server that we provide
* (AmazonProvidedDNS). If you create a set of options, and if your VPC has an internet gateway, make sure to set
* the domain-name-servers
option either to AmazonProvidedDNS
or to a domain name server
* of your choice. For more information, see DHCP options sets in the
* Amazon Virtual Private Cloud User Guide.
*
*
* @param createDhcpOptionsRequest
* @return A Java Future containing the result of the CreateDhcpOptions operation returned by the service.
* @sample AmazonEC2Async.CreateDhcpOptions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDhcpOptionsAsync(CreateDhcpOptionsRequest createDhcpOptionsRequest);
/**
*
* Creates a set of DHCP options for your VPC. After creating the set, you must associate it with the VPC, causing
* all existing and new instances that you launch in the VPC to use this set of DHCP options. The following are the
* individual DHCP options you can specify. For more information about the options, see RFC 2132.
*
*
* -
*
* domain-name-servers
- The IP addresses of up to four domain name servers, or AmazonProvidedDNS. The
* default DHCP option set specifies AmazonProvidedDNS. If specifying more than one domain name server, specify the
* IP addresses in a single parameter, separated by commas. To have your instance receive a custom DNS hostname as
* specified in domain-name
, you must set domain-name-servers
to a custom DNS server.
*
*
* -
*
* domain-name
- If you're using AmazonProvidedDNS in us-east-1
, specify
* ec2.internal
. If you're using AmazonProvidedDNS in another Region, specify
* region.compute.internal
(for example, ap-northeast-1.compute.internal
). Otherwise,
* specify a domain name (for example, ExampleCompany.com
). This value is used to complete unqualified
* DNS hostnames. Important: Some Linux operating systems accept multiple domain names separated by spaces.
* However, Windows and other Linux operating systems treat the value as a single domain, which results in
* unexpected behavior. If your DHCP options set is associated with a VPC that has instances with multiple operating
* systems, specify only one domain name.
*
*
* -
*
* ntp-servers
- The IP addresses of up to four Network Time Protocol (NTP) servers.
*
*
* -
*
* netbios-name-servers
- The IP addresses of up to four NetBIOS name servers.
*
*
* -
*
* netbios-node-type
- The NetBIOS node type (1, 2, 4, or 8). We recommend that you specify 2
* (broadcast and multicast are not currently supported). For more information about these node types, see RFC 2132.
*
*
*
*
* Your VPC automatically starts out with a set of DHCP options that includes only a DNS server that we provide
* (AmazonProvidedDNS). If you create a set of options, and if your VPC has an internet gateway, make sure to set
* the domain-name-servers
option either to AmazonProvidedDNS
or to a domain name server
* of your choice. For more information, see DHCP options sets in the
* Amazon Virtual Private Cloud User Guide.
*
*
* @param createDhcpOptionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateDhcpOptions operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateDhcpOptions
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createDhcpOptionsAsync(CreateDhcpOptionsRequest createDhcpOptionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* [IPv6 only] Creates an egress-only internet gateway for your VPC. An egress-only internet gateway is used to
* enable outbound communication over IPv6 from instances in your VPC to the internet, and prevents hosts outside of
* your VPC from initiating an IPv6 connection with your instance.
*
*
* @param createEgressOnlyInternetGatewayRequest
* @return A Java Future containing the result of the CreateEgressOnlyInternetGateway operation returned by the
* service.
* @sample AmazonEC2Async.CreateEgressOnlyInternetGateway
* @see AWS API Documentation
*/
java.util.concurrent.Future createEgressOnlyInternetGatewayAsync(
CreateEgressOnlyInternetGatewayRequest createEgressOnlyInternetGatewayRequest);
/**
*
* [IPv6 only] Creates an egress-only internet gateway for your VPC. An egress-only internet gateway is used to
* enable outbound communication over IPv6 from instances in your VPC to the internet, and prevents hosts outside of
* your VPC from initiating an IPv6 connection with your instance.
*
*
* @param createEgressOnlyInternetGatewayRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateEgressOnlyInternetGateway operation returned by the
* service.
* @sample AmazonEC2AsyncHandler.CreateEgressOnlyInternetGateway
* @see AWS API Documentation
*/
java.util.concurrent.Future createEgressOnlyInternetGatewayAsync(
CreateEgressOnlyInternetGatewayRequest createEgressOnlyInternetGatewayRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Launches an EC2 Fleet.
*
*
* You can create a single EC2 Fleet that includes multiple launch specifications that vary by instance type, AMI,
* Availability Zone, or subnet.
*
*
* For more information, see EC2
* Fleet in the Amazon EC2 User Guide.
*
*
* @param createFleetRequest
* @return A Java Future containing the result of the CreateFleet operation returned by the service.
* @sample AmazonEC2Async.CreateFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFleetAsync(CreateFleetRequest createFleetRequest);
/**
*
* Launches an EC2 Fleet.
*
*
* You can create a single EC2 Fleet that includes multiple launch specifications that vary by instance type, AMI,
* Availability Zone, or subnet.
*
*
* For more information, see EC2
* Fleet in the Amazon EC2 User Guide.
*
*
* @param createFleetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateFleet operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateFleet
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFleetAsync(CreateFleetRequest createFleetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates one or more flow logs to capture information about IP traffic for a specific network interface, subnet,
* or VPC.
*
*
* Flow log data for a monitored network interface is recorded as flow log records, which are log events consisting
* of fields that describe the traffic flow. For more information, see Flow log records in
* the Amazon Virtual Private Cloud User Guide.
*
*
* When publishing to CloudWatch Logs, flow log records are published to a log group, and each network interface has
* a unique log stream in the log group. When publishing to Amazon S3, flow log records for all of the monitored
* network interfaces are published to a single log file object that is stored in the specified bucket.
*
*
* For more information, see VPC Flow
* Logs in the Amazon Virtual Private Cloud User Guide.
*
*
* @param createFlowLogsRequest
* @return A Java Future containing the result of the CreateFlowLogs operation returned by the service.
* @sample AmazonEC2Async.CreateFlowLogs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFlowLogsAsync(CreateFlowLogsRequest createFlowLogsRequest);
/**
*
* Creates one or more flow logs to capture information about IP traffic for a specific network interface, subnet,
* or VPC.
*
*
* Flow log data for a monitored network interface is recorded as flow log records, which are log events consisting
* of fields that describe the traffic flow. For more information, see Flow log records in
* the Amazon Virtual Private Cloud User Guide.
*
*
* When publishing to CloudWatch Logs, flow log records are published to a log group, and each network interface has
* a unique log stream in the log group. When publishing to Amazon S3, flow log records for all of the monitored
* network interfaces are published to a single log file object that is stored in the specified bucket.
*
*
* For more information, see VPC Flow
* Logs in the Amazon Virtual Private Cloud User Guide.
*
*
* @param createFlowLogsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateFlowLogs operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateFlowLogs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFlowLogsAsync(CreateFlowLogsRequest createFlowLogsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Amazon FPGA Image (AFI) from the specified design checkpoint (DCP).
*
*
* The create operation is asynchronous. To verify that the AFI is ready for use, check the output logs.
*
*
* An AFI contains the FPGA bitstream that is ready to download to an FPGA. You can securely deploy an AFI on
* multiple FPGA-accelerated instances. For more information, see the Amazon Web Services FPGA Hardware Development Kit.
*
*
* @param createFpgaImageRequest
* @return A Java Future containing the result of the CreateFpgaImage operation returned by the service.
* @sample AmazonEC2Async.CreateFpgaImage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFpgaImageAsync(CreateFpgaImageRequest createFpgaImageRequest);
/**
*
* Creates an Amazon FPGA Image (AFI) from the specified design checkpoint (DCP).
*
*
* The create operation is asynchronous. To verify that the AFI is ready for use, check the output logs.
*
*
* An AFI contains the FPGA bitstream that is ready to download to an FPGA. You can securely deploy an AFI on
* multiple FPGA-accelerated instances. For more information, see the Amazon Web Services FPGA Hardware Development Kit.
*
*
* @param createFpgaImageRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateFpgaImage operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateFpgaImage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createFpgaImageAsync(CreateFpgaImageRequest createFpgaImageRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Amazon EBS-backed AMI from an Amazon EBS-backed instance that is either running or stopped.
*
*
* By default, when Amazon EC2 creates the new AMI, it reboots the instance so that it can take snapshots of the
* attached volumes while data is at rest, in order to ensure a consistent state. You can set the
* NoReboot
parameter to true
in the API request, or use the --no-reboot
* option in the CLI to prevent Amazon EC2 from shutting down and rebooting the instance.
*
*
*
* If you choose to bypass the shutdown and reboot process by setting the NoReboot
parameter to
* true
in the API request, or by using the --no-reboot
option in the CLI, we can't
* guarantee the file system integrity of the created image.
*
*
*
* If you customized your instance with instance store volumes or Amazon EBS volumes in addition to the root device
* volume, the new AMI contains block device mapping information for those volumes. When you launch an instance from
* this new AMI, the instance automatically launches with those additional volumes.
*
*
* For more information, see Create an Amazon EBS-backed
* Linux AMI in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param createImageRequest
* @return A Java Future containing the result of the CreateImage operation returned by the service.
* @sample AmazonEC2Async.CreateImage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createImageAsync(CreateImageRequest createImageRequest);
/**
*
* Creates an Amazon EBS-backed AMI from an Amazon EBS-backed instance that is either running or stopped.
*
*
* By default, when Amazon EC2 creates the new AMI, it reboots the instance so that it can take snapshots of the
* attached volumes while data is at rest, in order to ensure a consistent state. You can set the
* NoReboot
parameter to true
in the API request, or use the --no-reboot
* option in the CLI to prevent Amazon EC2 from shutting down and rebooting the instance.
*
*
*
* If you choose to bypass the shutdown and reboot process by setting the NoReboot
parameter to
* true
in the API request, or by using the --no-reboot
option in the CLI, we can't
* guarantee the file system integrity of the created image.
*
*
*
* If you customized your instance with instance store volumes or Amazon EBS volumes in addition to the root device
* volume, the new AMI contains block device mapping information for those volumes. When you launch an instance from
* this new AMI, the instance automatically launches with those additional volumes.
*
*
* For more information, see Create an Amazon EBS-backed
* Linux AMI in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param createImageRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateImage operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateImage
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createImageAsync(CreateImageRequest createImageRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an event window in which scheduled events for the associated Amazon EC2 instances can run.
*
*
* You can define either a set of time ranges or a cron expression when creating the event window, but not both. All
* event window times are in UTC.
*
*
* You can create up to 200 event windows per Amazon Web Services Region.
*
*
* When you create the event window, targets (instance IDs, Dedicated Host IDs, or tags) are not yet associated with
* it. To ensure that the event window can be used, you must associate one or more targets with it by using the
* AssociateInstanceEventWindow API.
*
*
*
* Event windows are applicable only for scheduled events that stop, reboot, or terminate instances.
*
*
* Event windows are not applicable for:
*
*
* -
*
* Expedited scheduled events and network maintenance events.
*
*
* -
*
* Unscheduled maintenance such as AutoRecovery and unplanned reboots.
*
*
*
*
*
* For more information, see Define
* event windows for scheduled events in the Amazon EC2 User Guide.
*
*
* @param createInstanceEventWindowRequest
* @return A Java Future containing the result of the CreateInstanceEventWindow operation returned by the service.
* @sample AmazonEC2Async.CreateInstanceEventWindow
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createInstanceEventWindowAsync(
CreateInstanceEventWindowRequest createInstanceEventWindowRequest);
/**
*
* Creates an event window in which scheduled events for the associated Amazon EC2 instances can run.
*
*
* You can define either a set of time ranges or a cron expression when creating the event window, but not both. All
* event window times are in UTC.
*
*
* You can create up to 200 event windows per Amazon Web Services Region.
*
*
* When you create the event window, targets (instance IDs, Dedicated Host IDs, or tags) are not yet associated with
* it. To ensure that the event window can be used, you must associate one or more targets with it by using the
* AssociateInstanceEventWindow API.
*
*
*
* Event windows are applicable only for scheduled events that stop, reboot, or terminate instances.
*
*
* Event windows are not applicable for:
*
*
* -
*
* Expedited scheduled events and network maintenance events.
*
*
* -
*
* Unscheduled maintenance such as AutoRecovery and unplanned reboots.
*
*
*
*
*
* For more information, see Define
* event windows for scheduled events in the Amazon EC2 User Guide.
*
*
* @param createInstanceEventWindowRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateInstanceEventWindow operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateInstanceEventWindow
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createInstanceEventWindowAsync(
CreateInstanceEventWindowRequest createInstanceEventWindowRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Exports a running or stopped instance to an Amazon S3 bucket.
*
*
* For information about the supported operating systems, image formats, and known limitations for the types of
* instances you can export, see Exporting an instance as a VM Using
* VM Import/Export in the VM Import/Export User Guide.
*
*
* @param createInstanceExportTaskRequest
* @return A Java Future containing the result of the CreateInstanceExportTask operation returned by the service.
* @sample AmazonEC2Async.CreateInstanceExportTask
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createInstanceExportTaskAsync(CreateInstanceExportTaskRequest createInstanceExportTaskRequest);
/**
*
* Exports a running or stopped instance to an Amazon S3 bucket.
*
*
* For information about the supported operating systems, image formats, and known limitations for the types of
* instances you can export, see Exporting an instance as a VM Using
* VM Import/Export in the VM Import/Export User Guide.
*
*
* @param createInstanceExportTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateInstanceExportTask operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateInstanceExportTask
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createInstanceExportTaskAsync(CreateInstanceExportTaskRequest createInstanceExportTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an internet gateway for use with a VPC. After creating the internet gateway, you attach it to a VPC using
* AttachInternetGateway.
*
*
* For more information about your VPC and internet gateway, see the Amazon Virtual Private Cloud User Guide.
*
*
* @param createInternetGatewayRequest
* @return A Java Future containing the result of the CreateInternetGateway operation returned by the service.
* @sample AmazonEC2Async.CreateInternetGateway
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createInternetGatewayAsync(CreateInternetGatewayRequest createInternetGatewayRequest);
/**
*
* Creates an internet gateway for use with a VPC. After creating the internet gateway, you attach it to a VPC using
* AttachInternetGateway.
*
*
* For more information about your VPC and internet gateway, see the Amazon Virtual Private Cloud User Guide.
*
*
* @param createInternetGatewayRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateInternetGateway operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateInternetGateway
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createInternetGatewayAsync(CreateInternetGatewayRequest createInternetGatewayRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the CreateInternetGateway operation.
*
* @see #createInternetGatewayAsync(CreateInternetGatewayRequest)
*/
java.util.concurrent.Future createInternetGatewayAsync();
/**
* Simplified method form for invoking the CreateInternetGateway operation with an AsyncHandler.
*
* @see #createInternetGatewayAsync(CreateInternetGatewayRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future createInternetGatewayAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create an IPAM. Amazon VPC IP Address Manager (IPAM) is a VPC feature that you can use to automate your IP
* address management workflows including assigning, tracking, troubleshooting, and auditing IP addresses across
* Amazon Web Services Regions and accounts throughout your Amazon Web Services Organization.
*
*
* For more information, see Create an
* IPAM in the Amazon VPC IPAM User Guide.
*
*
* @param createIpamRequest
* @return A Java Future containing the result of the CreateIpam operation returned by the service.
* @sample AmazonEC2Async.CreateIpam
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createIpamAsync(CreateIpamRequest createIpamRequest);
/**
*
* Create an IPAM. Amazon VPC IP Address Manager (IPAM) is a VPC feature that you can use to automate your IP
* address management workflows including assigning, tracking, troubleshooting, and auditing IP addresses across
* Amazon Web Services Regions and accounts throughout your Amazon Web Services Organization.
*
*
* For more information, see Create an
* IPAM in the Amazon VPC IPAM User Guide.
*
*
* @param createIpamRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateIpam operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateIpam
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createIpamAsync(CreateIpamRequest createIpamRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create an IP address pool for Amazon VPC IP Address Manager (IPAM). In IPAM, a pool is a collection of contiguous
* IP addresses CIDRs. Pools enable you to organize your IP addresses according to your routing and security needs.
* For example, if you have separate routing and security needs for development and production applications, you can
* create a pool for each.
*
*
* For more information, see Create a
* top-level pool in the Amazon VPC IPAM User Guide.
*
*
* @param createIpamPoolRequest
* @return A Java Future containing the result of the CreateIpamPool operation returned by the service.
* @sample AmazonEC2Async.CreateIpamPool
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createIpamPoolAsync(CreateIpamPoolRequest createIpamPoolRequest);
/**
*
* Create an IP address pool for Amazon VPC IP Address Manager (IPAM). In IPAM, a pool is a collection of contiguous
* IP addresses CIDRs. Pools enable you to organize your IP addresses according to your routing and security needs.
* For example, if you have separate routing and security needs for development and production applications, you can
* create a pool for each.
*
*
* For more information, see Create a
* top-level pool in the Amazon VPC IPAM User Guide.
*
*
* @param createIpamPoolRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateIpamPool operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateIpamPool
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createIpamPoolAsync(CreateIpamPoolRequest createIpamPoolRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an IPAM resource discovery. A resource discovery is an IPAM component that enables IPAM to manage and
* monitor resources that belong to the owning account.
*
*
* @param createIpamResourceDiscoveryRequest
* @return A Java Future containing the result of the CreateIpamResourceDiscovery operation returned by the service.
* @sample AmazonEC2Async.CreateIpamResourceDiscovery
* @see AWS API Documentation
*/
java.util.concurrent.Future createIpamResourceDiscoveryAsync(
CreateIpamResourceDiscoveryRequest createIpamResourceDiscoveryRequest);
/**
*
* Creates an IPAM resource discovery. A resource discovery is an IPAM component that enables IPAM to manage and
* monitor resources that belong to the owning account.
*
*
* @param createIpamResourceDiscoveryRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateIpamResourceDiscovery operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateIpamResourceDiscovery
* @see AWS API Documentation
*/
java.util.concurrent.Future createIpamResourceDiscoveryAsync(
CreateIpamResourceDiscoveryRequest createIpamResourceDiscoveryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Create an IPAM scope. In IPAM, a scope is the highest-level container within IPAM. An IPAM contains two default
* scopes. Each scope represents the IP space for a single network. The private scope is intended for all private IP
* address space. The public scope is intended for all public IP address space. Scopes enable you to reuse IP
* addresses across multiple unconnected networks without causing IP address overlap or conflict.
*
*
* For more information, see Add a
* scope in the Amazon VPC IPAM User Guide.
*
*
* @param createIpamScopeRequest
* @return A Java Future containing the result of the CreateIpamScope operation returned by the service.
* @sample AmazonEC2Async.CreateIpamScope
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createIpamScopeAsync(CreateIpamScopeRequest createIpamScopeRequest);
/**
*
* Create an IPAM scope. In IPAM, a scope is the highest-level container within IPAM. An IPAM contains two default
* scopes. Each scope represents the IP space for a single network. The private scope is intended for all private IP
* address space. The public scope is intended for all public IP address space. Scopes enable you to reuse IP
* addresses across multiple unconnected networks without causing IP address overlap or conflict.
*
*
* For more information, see Add a
* scope in the Amazon VPC IPAM User Guide.
*
*
* @param createIpamScopeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateIpamScope operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateIpamScope
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createIpamScopeAsync(CreateIpamScopeRequest createIpamScopeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an ED25519 or 2048-bit RSA key pair with the specified name and in the specified PEM or PPK format.
* Amazon EC2 stores the public key and displays the private key for you to save to a file. The private key is
* returned as an unencrypted PEM encoded PKCS#1 private key or an unencrypted PPK formatted private key for use
* with PuTTY. If a key with the specified name already exists, Amazon EC2 returns an error.
*
*
* The key pair returned to you is available only in the Amazon Web Services Region in which you create it. If you
* prefer, you can create your own key pair using a third-party tool and upload it to any Region using
* ImportKeyPair.
*
*
* You can have up to 5,000 key pairs per Amazon Web Services Region.
*
*
* For more information, see Amazon
* EC2 key pairs in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param createKeyPairRequest
* @return A Java Future containing the result of the CreateKeyPair operation returned by the service.
* @sample AmazonEC2Async.CreateKeyPair
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createKeyPairAsync(CreateKeyPairRequest createKeyPairRequest);
/**
*
* Creates an ED25519 or 2048-bit RSA key pair with the specified name and in the specified PEM or PPK format.
* Amazon EC2 stores the public key and displays the private key for you to save to a file. The private key is
* returned as an unencrypted PEM encoded PKCS#1 private key or an unencrypted PPK formatted private key for use
* with PuTTY. If a key with the specified name already exists, Amazon EC2 returns an error.
*
*
* The key pair returned to you is available only in the Amazon Web Services Region in which you create it. If you
* prefer, you can create your own key pair using a third-party tool and upload it to any Region using
* ImportKeyPair.
*
*
* You can have up to 5,000 key pairs per Amazon Web Services Region.
*
*
* For more information, see Amazon
* EC2 key pairs in the Amazon Elastic Compute Cloud User Guide.
*
*
* @param createKeyPairRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateKeyPair operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateKeyPair
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createKeyPairAsync(CreateKeyPairRequest createKeyPairRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a launch template.
*
*
* A launch template contains the parameters to launch an instance. When you launch an instance using
* RunInstances, you can specify a launch template instead of providing the launch parameters in the request.
* For more information, see Launch an instance from a
* launch template in the Amazon Elastic Compute Cloud User Guide.
*
*
* If you want to clone an existing launch template as the basis for creating a new launch template, you can use the
* Amazon EC2 console. The API, SDKs, and CLI do not support cloning a template. For more information, see Create a launch template from an existing launch template in the Amazon Elastic Compute Cloud User
* Guide.
*
*
* @param createLaunchTemplateRequest
* @return A Java Future containing the result of the CreateLaunchTemplate operation returned by the service.
* @sample AmazonEC2Async.CreateLaunchTemplate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createLaunchTemplateAsync(CreateLaunchTemplateRequest createLaunchTemplateRequest);
/**
*
* Creates a launch template.
*
*
* A launch template contains the parameters to launch an instance. When you launch an instance using
* RunInstances, you can specify a launch template instead of providing the launch parameters in the request.
* For more information, see Launch an instance from a
* launch template in the Amazon Elastic Compute Cloud User Guide.
*
*
* If you want to clone an existing launch template as the basis for creating a new launch template, you can use the
* Amazon EC2 console. The API, SDKs, and CLI do not support cloning a template. For more information, see Create a launch template from an existing launch template in the Amazon Elastic Compute Cloud User
* Guide.
*
*
* @param createLaunchTemplateRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateLaunchTemplate operation returned by the service.
* @sample AmazonEC2AsyncHandler.CreateLaunchTemplate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createLaunchTemplateAsync(CreateLaunchTemplateRequest createLaunchTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new version of a launch template. You can specify an existing version of launch template from which to
* base the new version.
*
*
* Launch template versions are numbered in the order in which they are created. You cannot specify, change, or
* replace the numbering of launch template versions.
*
*
* Launch templates are immutable; after you create a launch template, you can't modify it. Instead, you can create
* a new version of the launch template that includes any changes you require.
*
*
* For more information, see