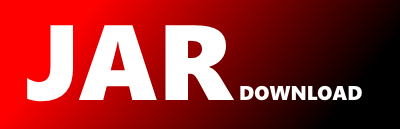
com.amazonaws.services.ec2.model.RunInstancesRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ec2 Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ec2.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
import com.amazonaws.Request;
import com.amazonaws.services.ec2.model.transform.RunInstancesRequestMarshaller;
/**
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class RunInstancesRequest extends AmazonWebServiceRequest implements Serializable, Cloneable, DryRunSupportedRequest {
/**
*
* The block device mapping, which defines the EBS volumes and instance store volumes to attach to the instance at
* launch. For more information, see Block device
* mappings in the Amazon EC2 User Guide.
*
*/
private com.amazonaws.internal.SdkInternalList blockDeviceMappings;
/**
*
* The ID of the AMI. An AMI ID is required to launch an instance and must be specified here or in a launch
* template.
*
*/
private String imageId;
/**
*
* The instance type. For more information, see Instance types in the
* Amazon EC2 User Guide.
*
*/
private String instanceType;
/**
*
* The number of IPv6 addresses to associate with the primary network interface. Amazon EC2 chooses the IPv6
* addresses from the range of your subnet. You cannot specify this option and the option to assign specific IPv6
* addresses in the same request. You can specify this option if you've specified a minimum number of instances to
* launch.
*
*
* You cannot specify this option and the network interfaces option in the same request.
*
*/
private Integer ipv6AddressCount;
/**
*
* The IPv6 addresses from the range of the subnet to associate with the primary network interface. You cannot
* specify this option and the option to assign a number of IPv6 addresses in the same request. You cannot specify
* this option if you've specified a minimum number of instances to launch.
*
*
* You cannot specify this option and the network interfaces option in the same request.
*
*/
private com.amazonaws.internal.SdkInternalList ipv6Addresses;
/**
*
* The ID of the kernel.
*
*
*
* We recommend that you use PV-GRUB instead of kernels and RAM disks. For more information, see PV-GRUB in the Amazon
* EC2 User Guide.
*
*
*/
private String kernelId;
/**
*
* The name of the key pair. You can create a key pair using CreateKeyPair or ImportKeyPair.
*
*
*
* If you do not specify a key pair, you can't connect to the instance unless you choose an AMI that is configured
* to allow users another way to log in.
*
*
*/
private String keyName;
/**
*
* The maximum number of instances to launch. If you specify more instances than Amazon EC2 can launch in the target
* Availability Zone, Amazon EC2 launches the largest possible number of instances above MinCount
.
*
*
* Constraints: Between 1 and the maximum number you're allowed for the specified instance type. For more
* information about the default limits, and how to request an increase, see How many instances can I run in
* Amazon EC2 in the Amazon EC2 FAQ.
*
*/
private Integer maxCount;
/**
*
* The minimum number of instances to launch. If you specify a minimum that is more instances than Amazon EC2 can
* launch in the target Availability Zone, Amazon EC2 launches no instances.
*
*
* Constraints: Between 1 and the maximum number you're allowed for the specified instance type. For more
* information about the default limits, and how to request an increase, see How many instances can I run in
* Amazon EC2 in the Amazon EC2 General FAQ.
*
*/
private Integer minCount;
/**
*
* Specifies whether detailed monitoring is enabled for the instance.
*
*/
private Boolean monitoring;
/**
*
* The placement for the instance.
*
*/
private Placement placement;
/**
*
* The ID of the RAM disk to select. Some kernels require additional drivers at launch. Check the kernel
* requirements for information about whether you need to specify a RAM disk. To find kernel requirements, go to the
* Amazon Web Services Resource Center and search for the kernel ID.
*
*
*
* We recommend that you use PV-GRUB instead of kernels and RAM disks. For more information, see PV-GRUB in the Amazon
* EC2 User Guide.
*
*
*/
private String ramdiskId;
/**
*
* The IDs of the security groups. You can create a security group using CreateSecurityGroup.
*
*
* If you specify a network interface, you must specify any security groups as part of the network interface.
*
*/
private com.amazonaws.internal.SdkInternalList securityGroupIds;
/**
*
* [Default VPC] The names of the security groups.
*
*
* If you specify a network interface, you must specify any security groups as part of the network interface.
*
*
* Default: Amazon EC2 uses the default security group.
*
*/
private com.amazonaws.internal.SdkInternalList securityGroups;
/**
*
* The ID of the subnet to launch the instance into.
*
*
* If you specify a network interface, you must specify any subnets as part of the network interface.
*
*/
private String subnetId;
/**
*
* The user data script to make available to the instance. For more information, see Run commands on your Linux instance at
* launch and Run
* commands on your Windows instance at launch. If you are using a command line tool, base64-encoding is
* performed for you, and you can load the text from a file. Otherwise, you must provide base64-encoded text. User
* data is limited to 16 KB.
*
*/
private String userData;
/**
*
* Reserved.
*
*/
private String additionalInfo;
/**
*
* Unique, case-sensitive identifier you provide to ensure the idempotency of the request. If you do not specify a
* client token, a randomly generated token is used for the request to ensure idempotency.
*
*
* For more information, see Ensuring
* Idempotency.
*
*
* Constraints: Maximum 64 ASCII characters
*
*/
private String clientToken;
/**
*
* If you set this parameter to true
, you can't terminate the instance using the Amazon EC2 console,
* CLI, or API; otherwise, you can. To change this attribute after launch, use ModifyInstanceAttribute. Alternatively, if you set InstanceInitiatedShutdownBehavior
to
* terminate
, you can terminate the instance by running the shutdown command from the instance.
*
*
* Default: false
*
*/
private Boolean disableApiTermination;
/**
*
* Indicates whether the instance is optimized for Amazon EBS I/O. This optimization provides dedicated throughput
* to Amazon EBS and an optimized configuration stack to provide optimal Amazon EBS I/O performance. This
* optimization isn't available with all instance types. Additional usage charges apply when using an EBS-optimized
* instance.
*
*
* Default: false
*
*/
private Boolean ebsOptimized;
/**
*
* The name or Amazon Resource Name (ARN) of an IAM instance profile.
*
*/
private IamInstanceProfileSpecification iamInstanceProfile;
/**
*
* Indicates whether an instance stops or terminates when you initiate shutdown from the instance (using the
* operating system command for system shutdown).
*
*
* Default: stop
*
*/
private String instanceInitiatedShutdownBehavior;
/**
*
* The network interfaces to associate with the instance. If you specify a network interface, you must specify any
* security groups and subnets as part of the network interface.
*
*/
private com.amazonaws.internal.SdkInternalList networkInterfaces;
/**
*
* The primary IPv4 address. You must specify a value from the IPv4 address range of the subnet.
*
*
* Only one private IP address can be designated as primary. You can't specify this option if you've specified the
* option to designate a private IP address as the primary IP address in a network interface specification. You
* cannot specify this option if you're launching more than one instance in the request.
*
*
* You cannot specify this option and the network interfaces option in the same request.
*
*/
private String privateIpAddress;
/**
*
* An elastic GPU to associate with the instance. An Elastic GPU is a GPU resource that you can attach to your
* Windows instance to accelerate the graphics performance of your applications. For more information, see Amazon EC2 Elastic GPUs
* in the Amazon EC2 User Guide.
*
*/
private com.amazonaws.internal.SdkInternalList elasticGpuSpecification;
/**
*
* An elastic inference accelerator to associate with the instance. Elastic inference accelerators are a resource
* you can attach to your Amazon EC2 instances to accelerate your Deep Learning (DL) inference workloads.
*
*
* You cannot specify accelerators from different generations in the same request.
*
*
*
* Starting April 15, 2023, Amazon Web Services will not onboard new customers to Amazon Elastic Inference (EI), and
* will help current customers migrate their workloads to options that offer better price and performance. After
* April 15, 2023, new customers will not be able to launch instances with Amazon EI accelerators in Amazon
* SageMaker, Amazon ECS, or Amazon EC2. However, customers who have used Amazon EI at least once during the past
* 30-day period are considered current customers and will be able to continue using the service.
*
*
*/
private com.amazonaws.internal.SdkInternalList elasticInferenceAccelerators;
/**
*
* The tags to apply to the resources that are created during instance launch.
*
*
* You can specify tags for the following resources only:
*
*
* -
*
* Instances
*
*
* -
*
* Volumes
*
*
* -
*
* Elastic graphics
*
*
* -
*
* Spot Instance requests
*
*
* -
*
* Network interfaces
*
*
*
*
* To tag a resource after it has been created, see CreateTags.
*
*/
private com.amazonaws.internal.SdkInternalList tagSpecifications;
/**
*
* The launch template to use to launch the instances. Any parameters that you specify in RunInstances
* override the same parameters in the launch template. You can specify either the name or ID of a launch template,
* but not both.
*
*/
private LaunchTemplateSpecification launchTemplate;
/**
*
* The market (purchasing) option for the instances.
*
*
* For RunInstances, persistent Spot Instance requests are only supported when
* InstanceInterruptionBehavior is set to either hibernate
or stop
.
*
*/
private InstanceMarketOptionsRequest instanceMarketOptions;
/**
*
* The credit option for CPU usage of the burstable performance instance. Valid values are standard
and
* unlimited
. To change this attribute after launch, use
* ModifyInstanceCreditSpecification. For more information, see Burstable
* performance instances in the Amazon EC2 User Guide.
*
*
* Default: standard
(T2 instances) or unlimited
(T3/T3a/T4g instances)
*
*
* For T3 instances with host
tenancy, only standard
is supported.
*
*/
private CreditSpecificationRequest creditSpecification;
/**
*
* The CPU options for the instance. For more information, see Optimize CPU options in
* the Amazon EC2 User Guide.
*
*/
private CpuOptionsRequest cpuOptions;
/**
*
* Information about the Capacity Reservation targeting option. If you do not specify this parameter, the instance's
* Capacity Reservation preference defaults to open
, which enables it to run in any open Capacity
* Reservation that has matching attributes (instance type, platform, Availability Zone).
*
*/
private CapacityReservationSpecification capacityReservationSpecification;
/**
*
* Indicates whether an instance is enabled for hibernation. This parameter is valid only if the instance meets the
* hibernation
* prerequisites. For more information, see Hibernate your instance in the
* Amazon EC2 User Guide.
*
*
* You can't enable hibernation and Amazon Web Services Nitro Enclaves on the same instance.
*
*/
private HibernationOptionsRequest hibernationOptions;
/**
*
* The license configurations.
*
*/
private com.amazonaws.internal.SdkInternalList licenseSpecifications;
/**
*
* The metadata options for the instance. For more information, see Instance metadata and user
* data.
*
*/
private InstanceMetadataOptionsRequest metadataOptions;
/**
*
* Indicates whether the instance is enabled for Amazon Web Services Nitro Enclaves. For more information, see What is Amazon Web Services Nitro
* Enclaves? in the Amazon Web Services Nitro Enclaves User Guide.
*
*
* You can't enable Amazon Web Services Nitro Enclaves and hibernation on the same instance.
*
*/
private EnclaveOptionsRequest enclaveOptions;
/**
*
* The options for the instance hostname. The default values are inherited from the subnet. Applies only if creating
* a network interface, not attaching an existing one.
*
*/
private PrivateDnsNameOptionsRequest privateDnsNameOptions;
/**
*
* The maintenance and recovery options for the instance.
*
*/
private InstanceMaintenanceOptionsRequest maintenanceOptions;
/**
*
* Indicates whether an instance is enabled for stop protection. For more information, see Stop
* protection.
*
*/
private Boolean disableApiStop;
/**
*
* If you’re launching an instance into a dual-stack or IPv6-only subnet, you can enable assigning a primary IPv6
* address. A primary IPv6 address is an IPv6 GUA address associated with an ENI that you have enabled to use a
* primary IPv6 address. Use this option if an instance relies on its IPv6 address not changing. When you launch the
* instance, Amazon Web Services will automatically assign an IPv6 address associated with the ENI attached to your
* instance to be the primary IPv6 address. Once you enable an IPv6 GUA address to be a primary IPv6, you cannot
* disable it. When you enable an IPv6 GUA address to be a primary IPv6, the first IPv6 GUA will be made the primary
* IPv6 address until the instance is terminated or the network interface is detached. If you have multiple IPv6
* addresses associated with an ENI attached to your instance and you enable a primary IPv6 address, the first IPv6
* GUA address associated with the ENI becomes the primary IPv6 address.
*
*/
private Boolean enablePrimaryIpv6;
/**
* Default constructor for RunInstancesRequest object. Callers should use the setter or fluent setter (with...)
* methods to initialize the object after creating it.
*/
public RunInstancesRequest() {
}
/**
* Constructs a new RunInstancesRequest object. Callers should use the setter or fluent setter (with...) methods to
* initialize any additional object members.
*
* @param imageId
* The ID of the AMI. An AMI ID is required to launch an instance and must be specified here or in a launch
* template.
* @param minCount
* The minimum number of instances to launch. If you specify a minimum that is more instances than Amazon EC2
* can launch in the target Availability Zone, Amazon EC2 launches no instances.
*
* Constraints: Between 1 and the maximum number you're allowed for the specified instance type. For more
* information about the default limits, and how to request an increase, see How many instances can I
* run in Amazon EC2 in the Amazon EC2 General FAQ.
* @param maxCount
* The maximum number of instances to launch. If you specify more instances than Amazon EC2 can launch in the
* target Availability Zone, Amazon EC2 launches the largest possible number of instances above
* MinCount
.
*
*
* Constraints: Between 1 and the maximum number you're allowed for the specified instance type. For more
* information about the default limits, and how to request an increase, see How many instances can I
* run in Amazon EC2 in the Amazon EC2 FAQ.
*/
public RunInstancesRequest(String imageId, Integer minCount, Integer maxCount) {
setImageId(imageId);
setMinCount(minCount);
setMaxCount(maxCount);
}
/**
*
* The block device mapping, which defines the EBS volumes and instance store volumes to attach to the instance at
* launch. For more information, see Block device
* mappings in the Amazon EC2 User Guide.
*
*
* @return The block device mapping, which defines the EBS volumes and instance store volumes to attach to the
* instance at launch. For more information, see Block
* device mappings in the Amazon EC2 User Guide.
*/
public java.util.List getBlockDeviceMappings() {
if (blockDeviceMappings == null) {
blockDeviceMappings = new com.amazonaws.internal.SdkInternalList();
}
return blockDeviceMappings;
}
/**
*
* The block device mapping, which defines the EBS volumes and instance store volumes to attach to the instance at
* launch. For more information, see Block device
* mappings in the Amazon EC2 User Guide.
*
*
* @param blockDeviceMappings
* The block device mapping, which defines the EBS volumes and instance store volumes to attach to the
* instance at launch. For more information, see Block device
* mappings in the Amazon EC2 User Guide.
*/
public void setBlockDeviceMappings(java.util.Collection blockDeviceMappings) {
if (blockDeviceMappings == null) {
this.blockDeviceMappings = null;
return;
}
this.blockDeviceMappings = new com.amazonaws.internal.SdkInternalList(blockDeviceMappings);
}
/**
*
* The block device mapping, which defines the EBS volumes and instance store volumes to attach to the instance at
* launch. For more information, see Block device
* mappings in the Amazon EC2 User Guide.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setBlockDeviceMappings(java.util.Collection)} or {@link #withBlockDeviceMappings(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param blockDeviceMappings
* The block device mapping, which defines the EBS volumes and instance store volumes to attach to the
* instance at launch. For more information, see Block device
* mappings in the Amazon EC2 User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withBlockDeviceMappings(BlockDeviceMapping... blockDeviceMappings) {
if (this.blockDeviceMappings == null) {
setBlockDeviceMappings(new com.amazonaws.internal.SdkInternalList(blockDeviceMappings.length));
}
for (BlockDeviceMapping ele : blockDeviceMappings) {
this.blockDeviceMappings.add(ele);
}
return this;
}
/**
*
* The block device mapping, which defines the EBS volumes and instance store volumes to attach to the instance at
* launch. For more information, see Block device
* mappings in the Amazon EC2 User Guide.
*
*
* @param blockDeviceMappings
* The block device mapping, which defines the EBS volumes and instance store volumes to attach to the
* instance at launch. For more information, see Block device
* mappings in the Amazon EC2 User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withBlockDeviceMappings(java.util.Collection blockDeviceMappings) {
setBlockDeviceMappings(blockDeviceMappings);
return this;
}
/**
*
* The ID of the AMI. An AMI ID is required to launch an instance and must be specified here or in a launch
* template.
*
*
* @param imageId
* The ID of the AMI. An AMI ID is required to launch an instance and must be specified here or in a launch
* template.
*/
public void setImageId(String imageId) {
this.imageId = imageId;
}
/**
*
* The ID of the AMI. An AMI ID is required to launch an instance and must be specified here or in a launch
* template.
*
*
* @return The ID of the AMI. An AMI ID is required to launch an instance and must be specified here or in a launch
* template.
*/
public String getImageId() {
return this.imageId;
}
/**
*
* The ID of the AMI. An AMI ID is required to launch an instance and must be specified here or in a launch
* template.
*
*
* @param imageId
* The ID of the AMI. An AMI ID is required to launch an instance and must be specified here or in a launch
* template.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withImageId(String imageId) {
setImageId(imageId);
return this;
}
/**
*
* The instance type. For more information, see Instance types in the
* Amazon EC2 User Guide.
*
*
* @param instanceType
* The instance type. For more information, see Instance types in the
* Amazon EC2 User Guide.
* @see InstanceType
*/
public void setInstanceType(String instanceType) {
this.instanceType = instanceType;
}
/**
*
* The instance type. For more information, see Instance types in the
* Amazon EC2 User Guide.
*
*
* @return The instance type. For more information, see Instance types in the
* Amazon EC2 User Guide.
* @see InstanceType
*/
public String getInstanceType() {
return this.instanceType;
}
/**
*
* The instance type. For more information, see Instance types in the
* Amazon EC2 User Guide.
*
*
* @param instanceType
* The instance type. For more information, see Instance types in the
* Amazon EC2 User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see InstanceType
*/
public RunInstancesRequest withInstanceType(String instanceType) {
setInstanceType(instanceType);
return this;
}
/**
*
* The instance type. For more information, see Instance types in the
* Amazon EC2 User Guide.
*
*
* @param instanceType
* The instance type. For more information, see Instance types in the
* Amazon EC2 User Guide.
* @see InstanceType
*/
public void setInstanceType(InstanceType instanceType) {
withInstanceType(instanceType);
}
/**
*
* The instance type. For more information, see Instance types in the
* Amazon EC2 User Guide.
*
*
* @param instanceType
* The instance type. For more information, see Instance types in the
* Amazon EC2 User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see InstanceType
*/
public RunInstancesRequest withInstanceType(InstanceType instanceType) {
this.instanceType = instanceType.toString();
return this;
}
/**
*
* The number of IPv6 addresses to associate with the primary network interface. Amazon EC2 chooses the IPv6
* addresses from the range of your subnet. You cannot specify this option and the option to assign specific IPv6
* addresses in the same request. You can specify this option if you've specified a minimum number of instances to
* launch.
*
*
* You cannot specify this option and the network interfaces option in the same request.
*
*
* @param ipv6AddressCount
* The number of IPv6 addresses to associate with the primary network interface. Amazon EC2 chooses the IPv6
* addresses from the range of your subnet. You cannot specify this option and the option to assign specific
* IPv6 addresses in the same request. You can specify this option if you've specified a minimum number of
* instances to launch.
*
* You cannot specify this option and the network interfaces option in the same request.
*/
public void setIpv6AddressCount(Integer ipv6AddressCount) {
this.ipv6AddressCount = ipv6AddressCount;
}
/**
*
* The number of IPv6 addresses to associate with the primary network interface. Amazon EC2 chooses the IPv6
* addresses from the range of your subnet. You cannot specify this option and the option to assign specific IPv6
* addresses in the same request. You can specify this option if you've specified a minimum number of instances to
* launch.
*
*
* You cannot specify this option and the network interfaces option in the same request.
*
*
* @return The number of IPv6 addresses to associate with the primary network interface. Amazon EC2 chooses the IPv6
* addresses from the range of your subnet. You cannot specify this option and the option to assign specific
* IPv6 addresses in the same request. You can specify this option if you've specified a minimum number of
* instances to launch.
*
* You cannot specify this option and the network interfaces option in the same request.
*/
public Integer getIpv6AddressCount() {
return this.ipv6AddressCount;
}
/**
*
* The number of IPv6 addresses to associate with the primary network interface. Amazon EC2 chooses the IPv6
* addresses from the range of your subnet. You cannot specify this option and the option to assign specific IPv6
* addresses in the same request. You can specify this option if you've specified a minimum number of instances to
* launch.
*
*
* You cannot specify this option and the network interfaces option in the same request.
*
*
* @param ipv6AddressCount
* The number of IPv6 addresses to associate with the primary network interface. Amazon EC2 chooses the IPv6
* addresses from the range of your subnet. You cannot specify this option and the option to assign specific
* IPv6 addresses in the same request. You can specify this option if you've specified a minimum number of
* instances to launch.
*
* You cannot specify this option and the network interfaces option in the same request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withIpv6AddressCount(Integer ipv6AddressCount) {
setIpv6AddressCount(ipv6AddressCount);
return this;
}
/**
*
* The IPv6 addresses from the range of the subnet to associate with the primary network interface. You cannot
* specify this option and the option to assign a number of IPv6 addresses in the same request. You cannot specify
* this option if you've specified a minimum number of instances to launch.
*
*
* You cannot specify this option and the network interfaces option in the same request.
*
*
* @return The IPv6 addresses from the range of the subnet to associate with the primary network interface. You
* cannot specify this option and the option to assign a number of IPv6 addresses in the same request. You
* cannot specify this option if you've specified a minimum number of instances to launch.
*
* You cannot specify this option and the network interfaces option in the same request.
*/
public java.util.List getIpv6Addresses() {
if (ipv6Addresses == null) {
ipv6Addresses = new com.amazonaws.internal.SdkInternalList();
}
return ipv6Addresses;
}
/**
*
* The IPv6 addresses from the range of the subnet to associate with the primary network interface. You cannot
* specify this option and the option to assign a number of IPv6 addresses in the same request. You cannot specify
* this option if you've specified a minimum number of instances to launch.
*
*
* You cannot specify this option and the network interfaces option in the same request.
*
*
* @param ipv6Addresses
* The IPv6 addresses from the range of the subnet to associate with the primary network interface. You
* cannot specify this option and the option to assign a number of IPv6 addresses in the same request. You
* cannot specify this option if you've specified a minimum number of instances to launch.
*
* You cannot specify this option and the network interfaces option in the same request.
*/
public void setIpv6Addresses(java.util.Collection ipv6Addresses) {
if (ipv6Addresses == null) {
this.ipv6Addresses = null;
return;
}
this.ipv6Addresses = new com.amazonaws.internal.SdkInternalList(ipv6Addresses);
}
/**
*
* The IPv6 addresses from the range of the subnet to associate with the primary network interface. You cannot
* specify this option and the option to assign a number of IPv6 addresses in the same request. You cannot specify
* this option if you've specified a minimum number of instances to launch.
*
*
* You cannot specify this option and the network interfaces option in the same request.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setIpv6Addresses(java.util.Collection)} or {@link #withIpv6Addresses(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param ipv6Addresses
* The IPv6 addresses from the range of the subnet to associate with the primary network interface. You
* cannot specify this option and the option to assign a number of IPv6 addresses in the same request. You
* cannot specify this option if you've specified a minimum number of instances to launch.
*
* You cannot specify this option and the network interfaces option in the same request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withIpv6Addresses(InstanceIpv6Address... ipv6Addresses) {
if (this.ipv6Addresses == null) {
setIpv6Addresses(new com.amazonaws.internal.SdkInternalList(ipv6Addresses.length));
}
for (InstanceIpv6Address ele : ipv6Addresses) {
this.ipv6Addresses.add(ele);
}
return this;
}
/**
*
* The IPv6 addresses from the range of the subnet to associate with the primary network interface. You cannot
* specify this option and the option to assign a number of IPv6 addresses in the same request. You cannot specify
* this option if you've specified a minimum number of instances to launch.
*
*
* You cannot specify this option and the network interfaces option in the same request.
*
*
* @param ipv6Addresses
* The IPv6 addresses from the range of the subnet to associate with the primary network interface. You
* cannot specify this option and the option to assign a number of IPv6 addresses in the same request. You
* cannot specify this option if you've specified a minimum number of instances to launch.
*
* You cannot specify this option and the network interfaces option in the same request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withIpv6Addresses(java.util.Collection ipv6Addresses) {
setIpv6Addresses(ipv6Addresses);
return this;
}
/**
*
* The ID of the kernel.
*
*
*
* We recommend that you use PV-GRUB instead of kernels and RAM disks. For more information, see PV-GRUB in the Amazon
* EC2 User Guide.
*
*
*
* @param kernelId
* The ID of the kernel.
*
* We recommend that you use PV-GRUB instead of kernels and RAM disks. For more information, see PV-GRUB in the
* Amazon EC2 User Guide.
*
*/
public void setKernelId(String kernelId) {
this.kernelId = kernelId;
}
/**
*
* The ID of the kernel.
*
*
*
* We recommend that you use PV-GRUB instead of kernels and RAM disks. For more information, see PV-GRUB in the Amazon
* EC2 User Guide.
*
*
*
* @return The ID of the kernel.
*
* We recommend that you use PV-GRUB instead of kernels and RAM disks. For more information, see PV-GRUB in the
* Amazon EC2 User Guide.
*
*/
public String getKernelId() {
return this.kernelId;
}
/**
*
* The ID of the kernel.
*
*
*
* We recommend that you use PV-GRUB instead of kernels and RAM disks. For more information, see PV-GRUB in the Amazon
* EC2 User Guide.
*
*
*
* @param kernelId
* The ID of the kernel.
*
* We recommend that you use PV-GRUB instead of kernels and RAM disks. For more information, see PV-GRUB in the
* Amazon EC2 User Guide.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withKernelId(String kernelId) {
setKernelId(kernelId);
return this;
}
/**
*
* The name of the key pair. You can create a key pair using CreateKeyPair or ImportKeyPair.
*
*
*
* If you do not specify a key pair, you can't connect to the instance unless you choose an AMI that is configured
* to allow users another way to log in.
*
*
*
* @param keyName
* The name of the key pair. You can create a key pair using CreateKeyPair or
* ImportKeyPair.<
* /p>
*
* If you do not specify a key pair, you can't connect to the instance unless you choose an AMI that is
* configured to allow users another way to log in.
*
*/
public void setKeyName(String keyName) {
this.keyName = keyName;
}
/**
*
* The name of the key pair. You can create a key pair using CreateKeyPair or ImportKeyPair.
*
*
*
* If you do not specify a key pair, you can't connect to the instance unless you choose an AMI that is configured
* to allow users another way to log in.
*
*
*
* @return The name of the key pair. You can create a key pair using CreateKeyPair or
* ImportKeyPair.
*
*
* If you do not specify a key pair, you can't connect to the instance unless you choose an AMI that is
* configured to allow users another way to log in.
*
*/
public String getKeyName() {
return this.keyName;
}
/**
*
* The name of the key pair. You can create a key pair using CreateKeyPair or ImportKeyPair.
*
*
*
* If you do not specify a key pair, you can't connect to the instance unless you choose an AMI that is configured
* to allow users another way to log in.
*
*
*
* @param keyName
* The name of the key pair. You can create a key pair using CreateKeyPair or
* ImportKeyPair.<
* /p>
*
* If you do not specify a key pair, you can't connect to the instance unless you choose an AMI that is
* configured to allow users another way to log in.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withKeyName(String keyName) {
setKeyName(keyName);
return this;
}
/**
*
* The maximum number of instances to launch. If you specify more instances than Amazon EC2 can launch in the target
* Availability Zone, Amazon EC2 launches the largest possible number of instances above MinCount
.
*
*
* Constraints: Between 1 and the maximum number you're allowed for the specified instance type. For more
* information about the default limits, and how to request an increase, see How many instances can I run in
* Amazon EC2 in the Amazon EC2 FAQ.
*
*
* @param maxCount
* The maximum number of instances to launch. If you specify more instances than Amazon EC2 can launch in the
* target Availability Zone, Amazon EC2 launches the largest possible number of instances above
* MinCount
.
*
* Constraints: Between 1 and the maximum number you're allowed for the specified instance type. For more
* information about the default limits, and how to request an increase, see How many instances can I
* run in Amazon EC2 in the Amazon EC2 FAQ.
*/
public void setMaxCount(Integer maxCount) {
this.maxCount = maxCount;
}
/**
*
* The maximum number of instances to launch. If you specify more instances than Amazon EC2 can launch in the target
* Availability Zone, Amazon EC2 launches the largest possible number of instances above MinCount
.
*
*
* Constraints: Between 1 and the maximum number you're allowed for the specified instance type. For more
* information about the default limits, and how to request an increase, see How many instances can I run in
* Amazon EC2 in the Amazon EC2 FAQ.
*
*
* @return The maximum number of instances to launch. If you specify more instances than Amazon EC2 can launch in
* the target Availability Zone, Amazon EC2 launches the largest possible number of instances above
* MinCount
.
*
* Constraints: Between 1 and the maximum number you're allowed for the specified instance type. For more
* information about the default limits, and how to request an increase, see How many instances can
* I run in Amazon EC2 in the Amazon EC2 FAQ.
*/
public Integer getMaxCount() {
return this.maxCount;
}
/**
*
* The maximum number of instances to launch. If you specify more instances than Amazon EC2 can launch in the target
* Availability Zone, Amazon EC2 launches the largest possible number of instances above MinCount
.
*
*
* Constraints: Between 1 and the maximum number you're allowed for the specified instance type. For more
* information about the default limits, and how to request an increase, see How many instances can I run in
* Amazon EC2 in the Amazon EC2 FAQ.
*
*
* @param maxCount
* The maximum number of instances to launch. If you specify more instances than Amazon EC2 can launch in the
* target Availability Zone, Amazon EC2 launches the largest possible number of instances above
* MinCount
.
*
* Constraints: Between 1 and the maximum number you're allowed for the specified instance type. For more
* information about the default limits, and how to request an increase, see How many instances can I
* run in Amazon EC2 in the Amazon EC2 FAQ.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withMaxCount(Integer maxCount) {
setMaxCount(maxCount);
return this;
}
/**
*
* The minimum number of instances to launch. If you specify a minimum that is more instances than Amazon EC2 can
* launch in the target Availability Zone, Amazon EC2 launches no instances.
*
*
* Constraints: Between 1 and the maximum number you're allowed for the specified instance type. For more
* information about the default limits, and how to request an increase, see How many instances can I run in
* Amazon EC2 in the Amazon EC2 General FAQ.
*
*
* @param minCount
* The minimum number of instances to launch. If you specify a minimum that is more instances than Amazon EC2
* can launch in the target Availability Zone, Amazon EC2 launches no instances.
*
* Constraints: Between 1 and the maximum number you're allowed for the specified instance type. For more
* information about the default limits, and how to request an increase, see How many instances can I
* run in Amazon EC2 in the Amazon EC2 General FAQ.
*/
public void setMinCount(Integer minCount) {
this.minCount = minCount;
}
/**
*
* The minimum number of instances to launch. If you specify a minimum that is more instances than Amazon EC2 can
* launch in the target Availability Zone, Amazon EC2 launches no instances.
*
*
* Constraints: Between 1 and the maximum number you're allowed for the specified instance type. For more
* information about the default limits, and how to request an increase, see How many instances can I run in
* Amazon EC2 in the Amazon EC2 General FAQ.
*
*
* @return The minimum number of instances to launch. If you specify a minimum that is more instances than Amazon
* EC2 can launch in the target Availability Zone, Amazon EC2 launches no instances.
*
* Constraints: Between 1 and the maximum number you're allowed for the specified instance type. For more
* information about the default limits, and how to request an increase, see How many instances can
* I run in Amazon EC2 in the Amazon EC2 General FAQ.
*/
public Integer getMinCount() {
return this.minCount;
}
/**
*
* The minimum number of instances to launch. If you specify a minimum that is more instances than Amazon EC2 can
* launch in the target Availability Zone, Amazon EC2 launches no instances.
*
*
* Constraints: Between 1 and the maximum number you're allowed for the specified instance type. For more
* information about the default limits, and how to request an increase, see How many instances can I run in
* Amazon EC2 in the Amazon EC2 General FAQ.
*
*
* @param minCount
* The minimum number of instances to launch. If you specify a minimum that is more instances than Amazon EC2
* can launch in the target Availability Zone, Amazon EC2 launches no instances.
*
* Constraints: Between 1 and the maximum number you're allowed for the specified instance type. For more
* information about the default limits, and how to request an increase, see How many instances can I
* run in Amazon EC2 in the Amazon EC2 General FAQ.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withMinCount(Integer minCount) {
setMinCount(minCount);
return this;
}
/**
*
* Specifies whether detailed monitoring is enabled for the instance.
*
*
* @param monitoring
* Specifies whether detailed monitoring is enabled for the instance.
*/
public void setMonitoring(Boolean monitoring) {
this.monitoring = monitoring;
}
/**
*
* Specifies whether detailed monitoring is enabled for the instance.
*
*
* @return Specifies whether detailed monitoring is enabled for the instance.
*/
public Boolean getMonitoring() {
return this.monitoring;
}
/**
*
* Specifies whether detailed monitoring is enabled for the instance.
*
*
* @param monitoring
* Specifies whether detailed monitoring is enabled for the instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withMonitoring(Boolean monitoring) {
setMonitoring(monitoring);
return this;
}
/**
*
* Specifies whether detailed monitoring is enabled for the instance.
*
*
* @return Specifies whether detailed monitoring is enabled for the instance.
*/
public Boolean isMonitoring() {
return this.monitoring;
}
/**
*
* The placement for the instance.
*
*
* @param placement
* The placement for the instance.
*/
public void setPlacement(Placement placement) {
this.placement = placement;
}
/**
*
* The placement for the instance.
*
*
* @return The placement for the instance.
*/
public Placement getPlacement() {
return this.placement;
}
/**
*
* The placement for the instance.
*
*
* @param placement
* The placement for the instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withPlacement(Placement placement) {
setPlacement(placement);
return this;
}
/**
*
* The ID of the RAM disk to select. Some kernels require additional drivers at launch. Check the kernel
* requirements for information about whether you need to specify a RAM disk. To find kernel requirements, go to the
* Amazon Web Services Resource Center and search for the kernel ID.
*
*
*
* We recommend that you use PV-GRUB instead of kernels and RAM disks. For more information, see PV-GRUB in the Amazon
* EC2 User Guide.
*
*
*
* @param ramdiskId
* The ID of the RAM disk to select. Some kernels require additional drivers at launch. Check the kernel
* requirements for information about whether you need to specify a RAM disk. To find kernel requirements, go
* to the Amazon Web Services Resource Center and search for the kernel ID.
*
* We recommend that you use PV-GRUB instead of kernels and RAM disks. For more information, see PV-GRUB in the
* Amazon EC2 User Guide.
*
*/
public void setRamdiskId(String ramdiskId) {
this.ramdiskId = ramdiskId;
}
/**
*
* The ID of the RAM disk to select. Some kernels require additional drivers at launch. Check the kernel
* requirements for information about whether you need to specify a RAM disk. To find kernel requirements, go to the
* Amazon Web Services Resource Center and search for the kernel ID.
*
*
*
* We recommend that you use PV-GRUB instead of kernels and RAM disks. For more information, see PV-GRUB in the Amazon
* EC2 User Guide.
*
*
*
* @return The ID of the RAM disk to select. Some kernels require additional drivers at launch. Check the kernel
* requirements for information about whether you need to specify a RAM disk. To find kernel requirements,
* go to the Amazon Web Services Resource Center and search for the kernel ID.
*
* We recommend that you use PV-GRUB instead of kernels and RAM disks. For more information, see PV-GRUB in the
* Amazon EC2 User Guide.
*
*/
public String getRamdiskId() {
return this.ramdiskId;
}
/**
*
* The ID of the RAM disk to select. Some kernels require additional drivers at launch. Check the kernel
* requirements for information about whether you need to specify a RAM disk. To find kernel requirements, go to the
* Amazon Web Services Resource Center and search for the kernel ID.
*
*
*
* We recommend that you use PV-GRUB instead of kernels and RAM disks. For more information, see PV-GRUB in the Amazon
* EC2 User Guide.
*
*
*
* @param ramdiskId
* The ID of the RAM disk to select. Some kernels require additional drivers at launch. Check the kernel
* requirements for information about whether you need to specify a RAM disk. To find kernel requirements, go
* to the Amazon Web Services Resource Center and search for the kernel ID.
*
* We recommend that you use PV-GRUB instead of kernels and RAM disks. For more information, see PV-GRUB in the
* Amazon EC2 User Guide.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withRamdiskId(String ramdiskId) {
setRamdiskId(ramdiskId);
return this;
}
/**
*
* The IDs of the security groups. You can create a security group using CreateSecurityGroup.
*
*
* If you specify a network interface, you must specify any security groups as part of the network interface.
*
*
* @return The IDs of the security groups. You can create a security group using CreateSecurityGroup.
*
* If you specify a network interface, you must specify any security groups as part of the network
* interface.
*/
public java.util.List getSecurityGroupIds() {
if (securityGroupIds == null) {
securityGroupIds = new com.amazonaws.internal.SdkInternalList();
}
return securityGroupIds;
}
/**
*
* The IDs of the security groups. You can create a security group using CreateSecurityGroup.
*
*
* If you specify a network interface, you must specify any security groups as part of the network interface.
*
*
* @param securityGroupIds
* The IDs of the security groups. You can create a security group using CreateSecurityGroup.
*
* If you specify a network interface, you must specify any security groups as part of the network interface.
*/
public void setSecurityGroupIds(java.util.Collection securityGroupIds) {
if (securityGroupIds == null) {
this.securityGroupIds = null;
return;
}
this.securityGroupIds = new com.amazonaws.internal.SdkInternalList(securityGroupIds);
}
/**
*
* The IDs of the security groups. You can create a security group using CreateSecurityGroup.
*
*
* If you specify a network interface, you must specify any security groups as part of the network interface.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSecurityGroupIds(java.util.Collection)} or {@link #withSecurityGroupIds(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param securityGroupIds
* The IDs of the security groups. You can create a security group using CreateSecurityGroup.
*
* If you specify a network interface, you must specify any security groups as part of the network interface.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withSecurityGroupIds(String... securityGroupIds) {
if (this.securityGroupIds == null) {
setSecurityGroupIds(new com.amazonaws.internal.SdkInternalList(securityGroupIds.length));
}
for (String ele : securityGroupIds) {
this.securityGroupIds.add(ele);
}
return this;
}
/**
*
* The IDs of the security groups. You can create a security group using CreateSecurityGroup.
*
*
* If you specify a network interface, you must specify any security groups as part of the network interface.
*
*
* @param securityGroupIds
* The IDs of the security groups. You can create a security group using CreateSecurityGroup.
*
* If you specify a network interface, you must specify any security groups as part of the network interface.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withSecurityGroupIds(java.util.Collection securityGroupIds) {
setSecurityGroupIds(securityGroupIds);
return this;
}
/**
*
* [Default VPC] The names of the security groups.
*
*
* If you specify a network interface, you must specify any security groups as part of the network interface.
*
*
* Default: Amazon EC2 uses the default security group.
*
*
* @return [Default VPC] The names of the security groups.
*
* If you specify a network interface, you must specify any security groups as part of the network
* interface.
*
*
* Default: Amazon EC2 uses the default security group.
*/
public java.util.List getSecurityGroups() {
if (securityGroups == null) {
securityGroups = new com.amazonaws.internal.SdkInternalList();
}
return securityGroups;
}
/**
*
* [Default VPC] The names of the security groups.
*
*
* If you specify a network interface, you must specify any security groups as part of the network interface.
*
*
* Default: Amazon EC2 uses the default security group.
*
*
* @param securityGroups
* [Default VPC] The names of the security groups.
*
* If you specify a network interface, you must specify any security groups as part of the network interface.
*
*
* Default: Amazon EC2 uses the default security group.
*/
public void setSecurityGroups(java.util.Collection securityGroups) {
if (securityGroups == null) {
this.securityGroups = null;
return;
}
this.securityGroups = new com.amazonaws.internal.SdkInternalList(securityGroups);
}
/**
*
* [Default VPC] The names of the security groups.
*
*
* If you specify a network interface, you must specify any security groups as part of the network interface.
*
*
* Default: Amazon EC2 uses the default security group.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSecurityGroups(java.util.Collection)} or {@link #withSecurityGroups(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param securityGroups
* [Default VPC] The names of the security groups.
*
* If you specify a network interface, you must specify any security groups as part of the network interface.
*
*
* Default: Amazon EC2 uses the default security group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withSecurityGroups(String... securityGroups) {
if (this.securityGroups == null) {
setSecurityGroups(new com.amazonaws.internal.SdkInternalList(securityGroups.length));
}
for (String ele : securityGroups) {
this.securityGroups.add(ele);
}
return this;
}
/**
*
* [Default VPC] The names of the security groups.
*
*
* If you specify a network interface, you must specify any security groups as part of the network interface.
*
*
* Default: Amazon EC2 uses the default security group.
*
*
* @param securityGroups
* [Default VPC] The names of the security groups.
*
* If you specify a network interface, you must specify any security groups as part of the network interface.
*
*
* Default: Amazon EC2 uses the default security group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withSecurityGroups(java.util.Collection securityGroups) {
setSecurityGroups(securityGroups);
return this;
}
/**
*
* The ID of the subnet to launch the instance into.
*
*
* If you specify a network interface, you must specify any subnets as part of the network interface.
*
*
* @param subnetId
* The ID of the subnet to launch the instance into.
*
* If you specify a network interface, you must specify any subnets as part of the network interface.
*/
public void setSubnetId(String subnetId) {
this.subnetId = subnetId;
}
/**
*
* The ID of the subnet to launch the instance into.
*
*
* If you specify a network interface, you must specify any subnets as part of the network interface.
*
*
* @return The ID of the subnet to launch the instance into.
*
* If you specify a network interface, you must specify any subnets as part of the network interface.
*/
public String getSubnetId() {
return this.subnetId;
}
/**
*
* The ID of the subnet to launch the instance into.
*
*
* If you specify a network interface, you must specify any subnets as part of the network interface.
*
*
* @param subnetId
* The ID of the subnet to launch the instance into.
*
* If you specify a network interface, you must specify any subnets as part of the network interface.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withSubnetId(String subnetId) {
setSubnetId(subnetId);
return this;
}
/**
*
* The user data script to make available to the instance. For more information, see Run commands on your Linux instance at
* launch and Run
* commands on your Windows instance at launch. If you are using a command line tool, base64-encoding is
* performed for you, and you can load the text from a file. Otherwise, you must provide base64-encoded text. User
* data is limited to 16 KB.
*
*
* @param userData
* The user data script to make available to the instance. For more information, see Run commands on your Linux
* instance at launch and Run commands on
* your Windows instance at launch. If you are using a command line tool, base64-encoding is performed
* for you, and you can load the text from a file. Otherwise, you must provide base64-encoded text. User data
* is limited to 16 KB.
*/
public void setUserData(String userData) {
this.userData = userData;
}
/**
*
* The user data script to make available to the instance. For more information, see Run commands on your Linux instance at
* launch and Run
* commands on your Windows instance at launch. If you are using a command line tool, base64-encoding is
* performed for you, and you can load the text from a file. Otherwise, you must provide base64-encoded text. User
* data is limited to 16 KB.
*
*
* @return The user data script to make available to the instance. For more information, see Run commands on your Linux
* instance at launch and Run commands on
* your Windows instance at launch. If you are using a command line tool, base64-encoding is performed
* for you, and you can load the text from a file. Otherwise, you must provide base64-encoded text. User
* data is limited to 16 KB.
*/
public String getUserData() {
return this.userData;
}
/**
*
* The user data script to make available to the instance. For more information, see Run commands on your Linux instance at
* launch and Run
* commands on your Windows instance at launch. If you are using a command line tool, base64-encoding is
* performed for you, and you can load the text from a file. Otherwise, you must provide base64-encoded text. User
* data is limited to 16 KB.
*
*
* @param userData
* The user data script to make available to the instance. For more information, see Run commands on your Linux
* instance at launch and Run commands on
* your Windows instance at launch. If you are using a command line tool, base64-encoding is performed
* for you, and you can load the text from a file. Otherwise, you must provide base64-encoded text. User data
* is limited to 16 KB.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withUserData(String userData) {
setUserData(userData);
return this;
}
/**
*
* Reserved.
*
*
* @param additionalInfo
* Reserved.
*/
public void setAdditionalInfo(String additionalInfo) {
this.additionalInfo = additionalInfo;
}
/**
*
* Reserved.
*
*
* @return Reserved.
*/
public String getAdditionalInfo() {
return this.additionalInfo;
}
/**
*
* Reserved.
*
*
* @param additionalInfo
* Reserved.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withAdditionalInfo(String additionalInfo) {
setAdditionalInfo(additionalInfo);
return this;
}
/**
*
* Unique, case-sensitive identifier you provide to ensure the idempotency of the request. If you do not specify a
* client token, a randomly generated token is used for the request to ensure idempotency.
*
*
* For more information, see Ensuring
* Idempotency.
*
*
* Constraints: Maximum 64 ASCII characters
*
*
* @param clientToken
* Unique, case-sensitive identifier you provide to ensure the idempotency of the request. If you do not
* specify a client token, a randomly generated token is used for the request to ensure idempotency.
*
* For more information, see Ensuring
* Idempotency.
*
*
* Constraints: Maximum 64 ASCII characters
*/
public void setClientToken(String clientToken) {
this.clientToken = clientToken;
}
/**
*
* Unique, case-sensitive identifier you provide to ensure the idempotency of the request. If you do not specify a
* client token, a randomly generated token is used for the request to ensure idempotency.
*
*
* For more information, see Ensuring
* Idempotency.
*
*
* Constraints: Maximum 64 ASCII characters
*
*
* @return Unique, case-sensitive identifier you provide to ensure the idempotency of the request. If you do not
* specify a client token, a randomly generated token is used for the request to ensure idempotency.
*
* For more information, see Ensuring
* Idempotency.
*
*
* Constraints: Maximum 64 ASCII characters
*/
public String getClientToken() {
return this.clientToken;
}
/**
*
* Unique, case-sensitive identifier you provide to ensure the idempotency of the request. If you do not specify a
* client token, a randomly generated token is used for the request to ensure idempotency.
*
*
* For more information, see Ensuring
* Idempotency.
*
*
* Constraints: Maximum 64 ASCII characters
*
*
* @param clientToken
* Unique, case-sensitive identifier you provide to ensure the idempotency of the request. If you do not
* specify a client token, a randomly generated token is used for the request to ensure idempotency.
*
* For more information, see Ensuring
* Idempotency.
*
*
* Constraints: Maximum 64 ASCII characters
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withClientToken(String clientToken) {
setClientToken(clientToken);
return this;
}
/**
*
* If you set this parameter to true
, you can't terminate the instance using the Amazon EC2 console,
* CLI, or API; otherwise, you can. To change this attribute after launch, use ModifyInstanceAttribute. Alternatively, if you set InstanceInitiatedShutdownBehavior
to
* terminate
, you can terminate the instance by running the shutdown command from the instance.
*
*
* Default: false
*
*
* @param disableApiTermination
* If you set this parameter to true
, you can't terminate the instance using the Amazon EC2
* console, CLI, or API; otherwise, you can. To change this attribute after launch, use ModifyInstanceAttribute. Alternatively, if you set InstanceInitiatedShutdownBehavior
to
* terminate
, you can terminate the instance by running the shutdown command from the
* instance.
*
* Default: false
*/
public void setDisableApiTermination(Boolean disableApiTermination) {
this.disableApiTermination = disableApiTermination;
}
/**
*
* If you set this parameter to true
, you can't terminate the instance using the Amazon EC2 console,
* CLI, or API; otherwise, you can. To change this attribute after launch, use ModifyInstanceAttribute. Alternatively, if you set InstanceInitiatedShutdownBehavior
to
* terminate
, you can terminate the instance by running the shutdown command from the instance.
*
*
* Default: false
*
*
* @return If you set this parameter to true
, you can't terminate the instance using the Amazon EC2
* console, CLI, or API; otherwise, you can. To change this attribute after launch, use ModifyInstanceAttribute. Alternatively, if you set InstanceInitiatedShutdownBehavior
to
* terminate
, you can terminate the instance by running the shutdown command from the
* instance.
*
* Default: false
*/
public Boolean getDisableApiTermination() {
return this.disableApiTermination;
}
/**
*
* If you set this parameter to true
, you can't terminate the instance using the Amazon EC2 console,
* CLI, or API; otherwise, you can. To change this attribute after launch, use ModifyInstanceAttribute. Alternatively, if you set InstanceInitiatedShutdownBehavior
to
* terminate
, you can terminate the instance by running the shutdown command from the instance.
*
*
* Default: false
*
*
* @param disableApiTermination
* If you set this parameter to true
, you can't terminate the instance using the Amazon EC2
* console, CLI, or API; otherwise, you can. To change this attribute after launch, use ModifyInstanceAttribute. Alternatively, if you set InstanceInitiatedShutdownBehavior
to
* terminate
, you can terminate the instance by running the shutdown command from the
* instance.
*
* Default: false
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withDisableApiTermination(Boolean disableApiTermination) {
setDisableApiTermination(disableApiTermination);
return this;
}
/**
*
* If you set this parameter to true
, you can't terminate the instance using the Amazon EC2 console,
* CLI, or API; otherwise, you can. To change this attribute after launch, use ModifyInstanceAttribute. Alternatively, if you set InstanceInitiatedShutdownBehavior
to
* terminate
, you can terminate the instance by running the shutdown command from the instance.
*
*
* Default: false
*
*
* @return If you set this parameter to true
, you can't terminate the instance using the Amazon EC2
* console, CLI, or API; otherwise, you can. To change this attribute after launch, use ModifyInstanceAttribute. Alternatively, if you set InstanceInitiatedShutdownBehavior
to
* terminate
, you can terminate the instance by running the shutdown command from the
* instance.
*
* Default: false
*/
public Boolean isDisableApiTermination() {
return this.disableApiTermination;
}
/**
*
* Indicates whether the instance is optimized for Amazon EBS I/O. This optimization provides dedicated throughput
* to Amazon EBS and an optimized configuration stack to provide optimal Amazon EBS I/O performance. This
* optimization isn't available with all instance types. Additional usage charges apply when using an EBS-optimized
* instance.
*
*
* Default: false
*
*
* @param ebsOptimized
* Indicates whether the instance is optimized for Amazon EBS I/O. This optimization provides dedicated
* throughput to Amazon EBS and an optimized configuration stack to provide optimal Amazon EBS I/O
* performance. This optimization isn't available with all instance types. Additional usage charges apply
* when using an EBS-optimized instance.
*
* Default: false
*/
public void setEbsOptimized(Boolean ebsOptimized) {
this.ebsOptimized = ebsOptimized;
}
/**
*
* Indicates whether the instance is optimized for Amazon EBS I/O. This optimization provides dedicated throughput
* to Amazon EBS and an optimized configuration stack to provide optimal Amazon EBS I/O performance. This
* optimization isn't available with all instance types. Additional usage charges apply when using an EBS-optimized
* instance.
*
*
* Default: false
*
*
* @return Indicates whether the instance is optimized for Amazon EBS I/O. This optimization provides dedicated
* throughput to Amazon EBS and an optimized configuration stack to provide optimal Amazon EBS I/O
* performance. This optimization isn't available with all instance types. Additional usage charges apply
* when using an EBS-optimized instance.
*
* Default: false
*/
public Boolean getEbsOptimized() {
return this.ebsOptimized;
}
/**
*
* Indicates whether the instance is optimized for Amazon EBS I/O. This optimization provides dedicated throughput
* to Amazon EBS and an optimized configuration stack to provide optimal Amazon EBS I/O performance. This
* optimization isn't available with all instance types. Additional usage charges apply when using an EBS-optimized
* instance.
*
*
* Default: false
*
*
* @param ebsOptimized
* Indicates whether the instance is optimized for Amazon EBS I/O. This optimization provides dedicated
* throughput to Amazon EBS and an optimized configuration stack to provide optimal Amazon EBS I/O
* performance. This optimization isn't available with all instance types. Additional usage charges apply
* when using an EBS-optimized instance.
*
* Default: false
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withEbsOptimized(Boolean ebsOptimized) {
setEbsOptimized(ebsOptimized);
return this;
}
/**
*
* Indicates whether the instance is optimized for Amazon EBS I/O. This optimization provides dedicated throughput
* to Amazon EBS and an optimized configuration stack to provide optimal Amazon EBS I/O performance. This
* optimization isn't available with all instance types. Additional usage charges apply when using an EBS-optimized
* instance.
*
*
* Default: false
*
*
* @return Indicates whether the instance is optimized for Amazon EBS I/O. This optimization provides dedicated
* throughput to Amazon EBS and an optimized configuration stack to provide optimal Amazon EBS I/O
* performance. This optimization isn't available with all instance types. Additional usage charges apply
* when using an EBS-optimized instance.
*
* Default: false
*/
public Boolean isEbsOptimized() {
return this.ebsOptimized;
}
/**
*
* The name or Amazon Resource Name (ARN) of an IAM instance profile.
*
*
* @param iamInstanceProfile
* The name or Amazon Resource Name (ARN) of an IAM instance profile.
*/
public void setIamInstanceProfile(IamInstanceProfileSpecification iamInstanceProfile) {
this.iamInstanceProfile = iamInstanceProfile;
}
/**
*
* The name or Amazon Resource Name (ARN) of an IAM instance profile.
*
*
* @return The name or Amazon Resource Name (ARN) of an IAM instance profile.
*/
public IamInstanceProfileSpecification getIamInstanceProfile() {
return this.iamInstanceProfile;
}
/**
*
* The name or Amazon Resource Name (ARN) of an IAM instance profile.
*
*
* @param iamInstanceProfile
* The name or Amazon Resource Name (ARN) of an IAM instance profile.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withIamInstanceProfile(IamInstanceProfileSpecification iamInstanceProfile) {
setIamInstanceProfile(iamInstanceProfile);
return this;
}
/**
*
* Indicates whether an instance stops or terminates when you initiate shutdown from the instance (using the
* operating system command for system shutdown).
*
*
* Default: stop
*
*
* @param instanceInitiatedShutdownBehavior
* Indicates whether an instance stops or terminates when you initiate shutdown from the instance (using the
* operating system command for system shutdown).
*
* Default: stop
* @see ShutdownBehavior
*/
public void setInstanceInitiatedShutdownBehavior(String instanceInitiatedShutdownBehavior) {
this.instanceInitiatedShutdownBehavior = instanceInitiatedShutdownBehavior;
}
/**
*
* Indicates whether an instance stops or terminates when you initiate shutdown from the instance (using the
* operating system command for system shutdown).
*
*
* Default: stop
*
*
* @return Indicates whether an instance stops or terminates when you initiate shutdown from the instance (using the
* operating system command for system shutdown).
*
* Default: stop
* @see ShutdownBehavior
*/
public String getInstanceInitiatedShutdownBehavior() {
return this.instanceInitiatedShutdownBehavior;
}
/**
*
* Indicates whether an instance stops or terminates when you initiate shutdown from the instance (using the
* operating system command for system shutdown).
*
*
* Default: stop
*
*
* @param instanceInitiatedShutdownBehavior
* Indicates whether an instance stops or terminates when you initiate shutdown from the instance (using the
* operating system command for system shutdown).
*
* Default: stop
* @return Returns a reference to this object so that method calls can be chained together.
* @see ShutdownBehavior
*/
public RunInstancesRequest withInstanceInitiatedShutdownBehavior(String instanceInitiatedShutdownBehavior) {
setInstanceInitiatedShutdownBehavior(instanceInitiatedShutdownBehavior);
return this;
}
/**
*
* Indicates whether an instance stops or terminates when you initiate shutdown from the instance (using the
* operating system command for system shutdown).
*
*
* Default: stop
*
*
* @param instanceInitiatedShutdownBehavior
* Indicates whether an instance stops or terminates when you initiate shutdown from the instance (using the
* operating system command for system shutdown).
*
* Default: stop
* @see ShutdownBehavior
*/
public void setInstanceInitiatedShutdownBehavior(ShutdownBehavior instanceInitiatedShutdownBehavior) {
withInstanceInitiatedShutdownBehavior(instanceInitiatedShutdownBehavior);
}
/**
*
* Indicates whether an instance stops or terminates when you initiate shutdown from the instance (using the
* operating system command for system shutdown).
*
*
* Default: stop
*
*
* @param instanceInitiatedShutdownBehavior
* Indicates whether an instance stops or terminates when you initiate shutdown from the instance (using the
* operating system command for system shutdown).
*
* Default: stop
* @return Returns a reference to this object so that method calls can be chained together.
* @see ShutdownBehavior
*/
public RunInstancesRequest withInstanceInitiatedShutdownBehavior(ShutdownBehavior instanceInitiatedShutdownBehavior) {
this.instanceInitiatedShutdownBehavior = instanceInitiatedShutdownBehavior.toString();
return this;
}
/**
*
* The network interfaces to associate with the instance. If you specify a network interface, you must specify any
* security groups and subnets as part of the network interface.
*
*
* @return The network interfaces to associate with the instance. If you specify a network interface, you must
* specify any security groups and subnets as part of the network interface.
*/
public java.util.List getNetworkInterfaces() {
if (networkInterfaces == null) {
networkInterfaces = new com.amazonaws.internal.SdkInternalList();
}
return networkInterfaces;
}
/**
*
* The network interfaces to associate with the instance. If you specify a network interface, you must specify any
* security groups and subnets as part of the network interface.
*
*
* @param networkInterfaces
* The network interfaces to associate with the instance. If you specify a network interface, you must
* specify any security groups and subnets as part of the network interface.
*/
public void setNetworkInterfaces(java.util.Collection networkInterfaces) {
if (networkInterfaces == null) {
this.networkInterfaces = null;
return;
}
this.networkInterfaces = new com.amazonaws.internal.SdkInternalList(networkInterfaces);
}
/**
*
* The network interfaces to associate with the instance. If you specify a network interface, you must specify any
* security groups and subnets as part of the network interface.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setNetworkInterfaces(java.util.Collection)} or {@link #withNetworkInterfaces(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param networkInterfaces
* The network interfaces to associate with the instance. If you specify a network interface, you must
* specify any security groups and subnets as part of the network interface.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withNetworkInterfaces(InstanceNetworkInterfaceSpecification... networkInterfaces) {
if (this.networkInterfaces == null) {
setNetworkInterfaces(new com.amazonaws.internal.SdkInternalList(networkInterfaces.length));
}
for (InstanceNetworkInterfaceSpecification ele : networkInterfaces) {
this.networkInterfaces.add(ele);
}
return this;
}
/**
*
* The network interfaces to associate with the instance. If you specify a network interface, you must specify any
* security groups and subnets as part of the network interface.
*
*
* @param networkInterfaces
* The network interfaces to associate with the instance. If you specify a network interface, you must
* specify any security groups and subnets as part of the network interface.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withNetworkInterfaces(java.util.Collection networkInterfaces) {
setNetworkInterfaces(networkInterfaces);
return this;
}
/**
*
* The primary IPv4 address. You must specify a value from the IPv4 address range of the subnet.
*
*
* Only one private IP address can be designated as primary. You can't specify this option if you've specified the
* option to designate a private IP address as the primary IP address in a network interface specification. You
* cannot specify this option if you're launching more than one instance in the request.
*
*
* You cannot specify this option and the network interfaces option in the same request.
*
*
* @param privateIpAddress
* The primary IPv4 address. You must specify a value from the IPv4 address range of the subnet.
*
* Only one private IP address can be designated as primary. You can't specify this option if you've
* specified the option to designate a private IP address as the primary IP address in a network interface
* specification. You cannot specify this option if you're launching more than one instance in the request.
*
*
* You cannot specify this option and the network interfaces option in the same request.
*/
public void setPrivateIpAddress(String privateIpAddress) {
this.privateIpAddress = privateIpAddress;
}
/**
*
* The primary IPv4 address. You must specify a value from the IPv4 address range of the subnet.
*
*
* Only one private IP address can be designated as primary. You can't specify this option if you've specified the
* option to designate a private IP address as the primary IP address in a network interface specification. You
* cannot specify this option if you're launching more than one instance in the request.
*
*
* You cannot specify this option and the network interfaces option in the same request.
*
*
* @return The primary IPv4 address. You must specify a value from the IPv4 address range of the subnet.
*
* Only one private IP address can be designated as primary. You can't specify this option if you've
* specified the option to designate a private IP address as the primary IP address in a network interface
* specification. You cannot specify this option if you're launching more than one instance in the request.
*
*
* You cannot specify this option and the network interfaces option in the same request.
*/
public String getPrivateIpAddress() {
return this.privateIpAddress;
}
/**
*
* The primary IPv4 address. You must specify a value from the IPv4 address range of the subnet.
*
*
* Only one private IP address can be designated as primary. You can't specify this option if you've specified the
* option to designate a private IP address as the primary IP address in a network interface specification. You
* cannot specify this option if you're launching more than one instance in the request.
*
*
* You cannot specify this option and the network interfaces option in the same request.
*
*
* @param privateIpAddress
* The primary IPv4 address. You must specify a value from the IPv4 address range of the subnet.
*
* Only one private IP address can be designated as primary. You can't specify this option if you've
* specified the option to designate a private IP address as the primary IP address in a network interface
* specification. You cannot specify this option if you're launching more than one instance in the request.
*
*
* You cannot specify this option and the network interfaces option in the same request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withPrivateIpAddress(String privateIpAddress) {
setPrivateIpAddress(privateIpAddress);
return this;
}
/**
*
* An elastic GPU to associate with the instance. An Elastic GPU is a GPU resource that you can attach to your
* Windows instance to accelerate the graphics performance of your applications. For more information, see Amazon EC2 Elastic GPUs
* in the Amazon EC2 User Guide.
*
*
* @return An elastic GPU to associate with the instance. An Elastic GPU is a GPU resource that you can attach to
* your Windows instance to accelerate the graphics performance of your applications. For more information,
* see Amazon EC2
* Elastic GPUs in the Amazon EC2 User Guide.
*/
public java.util.List getElasticGpuSpecification() {
if (elasticGpuSpecification == null) {
elasticGpuSpecification = new com.amazonaws.internal.SdkInternalList();
}
return elasticGpuSpecification;
}
/**
*
* An elastic GPU to associate with the instance. An Elastic GPU is a GPU resource that you can attach to your
* Windows instance to accelerate the graphics performance of your applications. For more information, see Amazon EC2 Elastic GPUs
* in the Amazon EC2 User Guide.
*
*
* @param elasticGpuSpecification
* An elastic GPU to associate with the instance. An Elastic GPU is a GPU resource that you can attach to
* your Windows instance to accelerate the graphics performance of your applications. For more information,
* see Amazon EC2
* Elastic GPUs in the Amazon EC2 User Guide.
*/
public void setElasticGpuSpecification(java.util.Collection elasticGpuSpecification) {
if (elasticGpuSpecification == null) {
this.elasticGpuSpecification = null;
return;
}
this.elasticGpuSpecification = new com.amazonaws.internal.SdkInternalList(elasticGpuSpecification);
}
/**
*
* An elastic GPU to associate with the instance. An Elastic GPU is a GPU resource that you can attach to your
* Windows instance to accelerate the graphics performance of your applications. For more information, see Amazon EC2 Elastic GPUs
* in the Amazon EC2 User Guide.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setElasticGpuSpecification(java.util.Collection)} or
* {@link #withElasticGpuSpecification(java.util.Collection)} if you want to override the existing values.
*
*
* @param elasticGpuSpecification
* An elastic GPU to associate with the instance. An Elastic GPU is a GPU resource that you can attach to
* your Windows instance to accelerate the graphics performance of your applications. For more information,
* see Amazon EC2
* Elastic GPUs in the Amazon EC2 User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withElasticGpuSpecification(ElasticGpuSpecification... elasticGpuSpecification) {
if (this.elasticGpuSpecification == null) {
setElasticGpuSpecification(new com.amazonaws.internal.SdkInternalList(elasticGpuSpecification.length));
}
for (ElasticGpuSpecification ele : elasticGpuSpecification) {
this.elasticGpuSpecification.add(ele);
}
return this;
}
/**
*
* An elastic GPU to associate with the instance. An Elastic GPU is a GPU resource that you can attach to your
* Windows instance to accelerate the graphics performance of your applications. For more information, see Amazon EC2 Elastic GPUs
* in the Amazon EC2 User Guide.
*
*
* @param elasticGpuSpecification
* An elastic GPU to associate with the instance. An Elastic GPU is a GPU resource that you can attach to
* your Windows instance to accelerate the graphics performance of your applications. For more information,
* see Amazon EC2
* Elastic GPUs in the Amazon EC2 User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withElasticGpuSpecification(java.util.Collection elasticGpuSpecification) {
setElasticGpuSpecification(elasticGpuSpecification);
return this;
}
/**
*
* An elastic inference accelerator to associate with the instance. Elastic inference accelerators are a resource
* you can attach to your Amazon EC2 instances to accelerate your Deep Learning (DL) inference workloads.
*
*
* You cannot specify accelerators from different generations in the same request.
*
*
*
* Starting April 15, 2023, Amazon Web Services will not onboard new customers to Amazon Elastic Inference (EI), and
* will help current customers migrate their workloads to options that offer better price and performance. After
* April 15, 2023, new customers will not be able to launch instances with Amazon EI accelerators in Amazon
* SageMaker, Amazon ECS, or Amazon EC2. However, customers who have used Amazon EI at least once during the past
* 30-day period are considered current customers and will be able to continue using the service.
*
*
*
* @return An elastic inference accelerator to associate with the instance. Elastic inference accelerators are a
* resource you can attach to your Amazon EC2 instances to accelerate your Deep Learning (DL) inference
* workloads.
*
* You cannot specify accelerators from different generations in the same request.
*
*
*
* Starting April 15, 2023, Amazon Web Services will not onboard new customers to Amazon Elastic Inference
* (EI), and will help current customers migrate their workloads to options that offer better price and
* performance. After April 15, 2023, new customers will not be able to launch instances with Amazon EI
* accelerators in Amazon SageMaker, Amazon ECS, or Amazon EC2. However, customers who have used Amazon EI
* at least once during the past 30-day period are considered current customers and will be able to continue
* using the service.
*
*/
public java.util.List getElasticInferenceAccelerators() {
if (elasticInferenceAccelerators == null) {
elasticInferenceAccelerators = new com.amazonaws.internal.SdkInternalList();
}
return elasticInferenceAccelerators;
}
/**
*
* An elastic inference accelerator to associate with the instance. Elastic inference accelerators are a resource
* you can attach to your Amazon EC2 instances to accelerate your Deep Learning (DL) inference workloads.
*
*
* You cannot specify accelerators from different generations in the same request.
*
*
*
* Starting April 15, 2023, Amazon Web Services will not onboard new customers to Amazon Elastic Inference (EI), and
* will help current customers migrate their workloads to options that offer better price and performance. After
* April 15, 2023, new customers will not be able to launch instances with Amazon EI accelerators in Amazon
* SageMaker, Amazon ECS, or Amazon EC2. However, customers who have used Amazon EI at least once during the past
* 30-day period are considered current customers and will be able to continue using the service.
*
*
*
* @param elasticInferenceAccelerators
* An elastic inference accelerator to associate with the instance. Elastic inference accelerators are a
* resource you can attach to your Amazon EC2 instances to accelerate your Deep Learning (DL) inference
* workloads.
*
* You cannot specify accelerators from different generations in the same request.
*
*
*
* Starting April 15, 2023, Amazon Web Services will not onboard new customers to Amazon Elastic Inference
* (EI), and will help current customers migrate their workloads to options that offer better price and
* performance. After April 15, 2023, new customers will not be able to launch instances with Amazon EI
* accelerators in Amazon SageMaker, Amazon ECS, or Amazon EC2. However, customers who have used Amazon EI at
* least once during the past 30-day period are considered current customers and will be able to continue
* using the service.
*
*/
public void setElasticInferenceAccelerators(java.util.Collection elasticInferenceAccelerators) {
if (elasticInferenceAccelerators == null) {
this.elasticInferenceAccelerators = null;
return;
}
this.elasticInferenceAccelerators = new com.amazonaws.internal.SdkInternalList(elasticInferenceAccelerators);
}
/**
*
* An elastic inference accelerator to associate with the instance. Elastic inference accelerators are a resource
* you can attach to your Amazon EC2 instances to accelerate your Deep Learning (DL) inference workloads.
*
*
* You cannot specify accelerators from different generations in the same request.
*
*
*
* Starting April 15, 2023, Amazon Web Services will not onboard new customers to Amazon Elastic Inference (EI), and
* will help current customers migrate their workloads to options that offer better price and performance. After
* April 15, 2023, new customers will not be able to launch instances with Amazon EI accelerators in Amazon
* SageMaker, Amazon ECS, or Amazon EC2. However, customers who have used Amazon EI at least once during the past
* 30-day period are considered current customers and will be able to continue using the service.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setElasticInferenceAccelerators(java.util.Collection)} or
* {@link #withElasticInferenceAccelerators(java.util.Collection)} if you want to override the existing values.
*
*
* @param elasticInferenceAccelerators
* An elastic inference accelerator to associate with the instance. Elastic inference accelerators are a
* resource you can attach to your Amazon EC2 instances to accelerate your Deep Learning (DL) inference
* workloads.
*
* You cannot specify accelerators from different generations in the same request.
*
*
*
* Starting April 15, 2023, Amazon Web Services will not onboard new customers to Amazon Elastic Inference
* (EI), and will help current customers migrate their workloads to options that offer better price and
* performance. After April 15, 2023, new customers will not be able to launch instances with Amazon EI
* accelerators in Amazon SageMaker, Amazon ECS, or Amazon EC2. However, customers who have used Amazon EI at
* least once during the past 30-day period are considered current customers and will be able to continue
* using the service.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withElasticInferenceAccelerators(ElasticInferenceAccelerator... elasticInferenceAccelerators) {
if (this.elasticInferenceAccelerators == null) {
setElasticInferenceAccelerators(new com.amazonaws.internal.SdkInternalList(elasticInferenceAccelerators.length));
}
for (ElasticInferenceAccelerator ele : elasticInferenceAccelerators) {
this.elasticInferenceAccelerators.add(ele);
}
return this;
}
/**
*
* An elastic inference accelerator to associate with the instance. Elastic inference accelerators are a resource
* you can attach to your Amazon EC2 instances to accelerate your Deep Learning (DL) inference workloads.
*
*
* You cannot specify accelerators from different generations in the same request.
*
*
*
* Starting April 15, 2023, Amazon Web Services will not onboard new customers to Amazon Elastic Inference (EI), and
* will help current customers migrate their workloads to options that offer better price and performance. After
* April 15, 2023, new customers will not be able to launch instances with Amazon EI accelerators in Amazon
* SageMaker, Amazon ECS, or Amazon EC2. However, customers who have used Amazon EI at least once during the past
* 30-day period are considered current customers and will be able to continue using the service.
*
*
*
* @param elasticInferenceAccelerators
* An elastic inference accelerator to associate with the instance. Elastic inference accelerators are a
* resource you can attach to your Amazon EC2 instances to accelerate your Deep Learning (DL) inference
* workloads.
*
* You cannot specify accelerators from different generations in the same request.
*
*
*
* Starting April 15, 2023, Amazon Web Services will not onboard new customers to Amazon Elastic Inference
* (EI), and will help current customers migrate their workloads to options that offer better price and
* performance. After April 15, 2023, new customers will not be able to launch instances with Amazon EI
* accelerators in Amazon SageMaker, Amazon ECS, or Amazon EC2. However, customers who have used Amazon EI at
* least once during the past 30-day period are considered current customers and will be able to continue
* using the service.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withElasticInferenceAccelerators(java.util.Collection elasticInferenceAccelerators) {
setElasticInferenceAccelerators(elasticInferenceAccelerators);
return this;
}
/**
*
* The tags to apply to the resources that are created during instance launch.
*
*
* You can specify tags for the following resources only:
*
*
* -
*
* Instances
*
*
* -
*
* Volumes
*
*
* -
*
* Elastic graphics
*
*
* -
*
* Spot Instance requests
*
*
* -
*
* Network interfaces
*
*
*
*
* To tag a resource after it has been created, see CreateTags.
*
*
* @return The tags to apply to the resources that are created during instance launch.
*
* You can specify tags for the following resources only:
*
*
* -
*
* Instances
*
*
* -
*
* Volumes
*
*
* -
*
* Elastic graphics
*
*
* -
*
* Spot Instance requests
*
*
* -
*
* Network interfaces
*
*
*
*
* To tag a resource after it has been created, see CreateTags.
*/
public java.util.List getTagSpecifications() {
if (tagSpecifications == null) {
tagSpecifications = new com.amazonaws.internal.SdkInternalList();
}
return tagSpecifications;
}
/**
*
* The tags to apply to the resources that are created during instance launch.
*
*
* You can specify tags for the following resources only:
*
*
* -
*
* Instances
*
*
* -
*
* Volumes
*
*
* -
*
* Elastic graphics
*
*
* -
*
* Spot Instance requests
*
*
* -
*
* Network interfaces
*
*
*
*
* To tag a resource after it has been created, see CreateTags.
*
*
* @param tagSpecifications
* The tags to apply to the resources that are created during instance launch.
*
* You can specify tags for the following resources only:
*
*
* -
*
* Instances
*
*
* -
*
* Volumes
*
*
* -
*
* Elastic graphics
*
*
* -
*
* Spot Instance requests
*
*
* -
*
* Network interfaces
*
*
*
*
* To tag a resource after it has been created, see CreateTags.
*/
public void setTagSpecifications(java.util.Collection tagSpecifications) {
if (tagSpecifications == null) {
this.tagSpecifications = null;
return;
}
this.tagSpecifications = new com.amazonaws.internal.SdkInternalList(tagSpecifications);
}
/**
*
* The tags to apply to the resources that are created during instance launch.
*
*
* You can specify tags for the following resources only:
*
*
* -
*
* Instances
*
*
* -
*
* Volumes
*
*
* -
*
* Elastic graphics
*
*
* -
*
* Spot Instance requests
*
*
* -
*
* Network interfaces
*
*
*
*
* To tag a resource after it has been created, see CreateTags.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTagSpecifications(java.util.Collection)} or {@link #withTagSpecifications(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param tagSpecifications
* The tags to apply to the resources that are created during instance launch.
*
* You can specify tags for the following resources only:
*
*
* -
*
* Instances
*
*
* -
*
* Volumes
*
*
* -
*
* Elastic graphics
*
*
* -
*
* Spot Instance requests
*
*
* -
*
* Network interfaces
*
*
*
*
* To tag a resource after it has been created, see CreateTags.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withTagSpecifications(TagSpecification... tagSpecifications) {
if (this.tagSpecifications == null) {
setTagSpecifications(new com.amazonaws.internal.SdkInternalList(tagSpecifications.length));
}
for (TagSpecification ele : tagSpecifications) {
this.tagSpecifications.add(ele);
}
return this;
}
/**
*
* The tags to apply to the resources that are created during instance launch.
*
*
* You can specify tags for the following resources only:
*
*
* -
*
* Instances
*
*
* -
*
* Volumes
*
*
* -
*
* Elastic graphics
*
*
* -
*
* Spot Instance requests
*
*
* -
*
* Network interfaces
*
*
*
*
* To tag a resource after it has been created, see CreateTags.
*
*
* @param tagSpecifications
* The tags to apply to the resources that are created during instance launch.
*
* You can specify tags for the following resources only:
*
*
* -
*
* Instances
*
*
* -
*
* Volumes
*
*
* -
*
* Elastic graphics
*
*
* -
*
* Spot Instance requests
*
*
* -
*
* Network interfaces
*
*
*
*
* To tag a resource after it has been created, see CreateTags.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withTagSpecifications(java.util.Collection tagSpecifications) {
setTagSpecifications(tagSpecifications);
return this;
}
/**
*
* The launch template to use to launch the instances. Any parameters that you specify in RunInstances
* override the same parameters in the launch template. You can specify either the name or ID of a launch template,
* but not both.
*
*
* @param launchTemplate
* The launch template to use to launch the instances. Any parameters that you specify in RunInstances
* override the same parameters in the launch template. You can specify either the name or ID of a launch
* template, but not both.
*/
public void setLaunchTemplate(LaunchTemplateSpecification launchTemplate) {
this.launchTemplate = launchTemplate;
}
/**
*
* The launch template to use to launch the instances. Any parameters that you specify in RunInstances
* override the same parameters in the launch template. You can specify either the name or ID of a launch template,
* but not both.
*
*
* @return The launch template to use to launch the instances. Any parameters that you specify in
* RunInstances override the same parameters in the launch template. You can specify either the name
* or ID of a launch template, but not both.
*/
public LaunchTemplateSpecification getLaunchTemplate() {
return this.launchTemplate;
}
/**
*
* The launch template to use to launch the instances. Any parameters that you specify in RunInstances
* override the same parameters in the launch template. You can specify either the name or ID of a launch template,
* but not both.
*
*
* @param launchTemplate
* The launch template to use to launch the instances. Any parameters that you specify in RunInstances
* override the same parameters in the launch template. You can specify either the name or ID of a launch
* template, but not both.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withLaunchTemplate(LaunchTemplateSpecification launchTemplate) {
setLaunchTemplate(launchTemplate);
return this;
}
/**
*
* The market (purchasing) option for the instances.
*
*
* For RunInstances, persistent Spot Instance requests are only supported when
* InstanceInterruptionBehavior is set to either hibernate
or stop
.
*
*
* @param instanceMarketOptions
* The market (purchasing) option for the instances.
*
* For RunInstances, persistent Spot Instance requests are only supported when
* InstanceInterruptionBehavior is set to either hibernate
or stop
.
*/
public void setInstanceMarketOptions(InstanceMarketOptionsRequest instanceMarketOptions) {
this.instanceMarketOptions = instanceMarketOptions;
}
/**
*
* The market (purchasing) option for the instances.
*
*
* For RunInstances, persistent Spot Instance requests are only supported when
* InstanceInterruptionBehavior is set to either hibernate
or stop
.
*
*
* @return The market (purchasing) option for the instances.
*
* For RunInstances, persistent Spot Instance requests are only supported when
* InstanceInterruptionBehavior is set to either hibernate
or stop
.
*/
public InstanceMarketOptionsRequest getInstanceMarketOptions() {
return this.instanceMarketOptions;
}
/**
*
* The market (purchasing) option for the instances.
*
*
* For RunInstances, persistent Spot Instance requests are only supported when
* InstanceInterruptionBehavior is set to either hibernate
or stop
.
*
*
* @param instanceMarketOptions
* The market (purchasing) option for the instances.
*
* For RunInstances, persistent Spot Instance requests are only supported when
* InstanceInterruptionBehavior is set to either hibernate
or stop
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withInstanceMarketOptions(InstanceMarketOptionsRequest instanceMarketOptions) {
setInstanceMarketOptions(instanceMarketOptions);
return this;
}
/**
*
* The credit option for CPU usage of the burstable performance instance. Valid values are standard
and
* unlimited
. To change this attribute after launch, use
* ModifyInstanceCreditSpecification. For more information, see Burstable
* performance instances in the Amazon EC2 User Guide.
*
*
* Default: standard
(T2 instances) or unlimited
(T3/T3a/T4g instances)
*
*
* For T3 instances with host
tenancy, only standard
is supported.
*
*
* @param creditSpecification
* The credit option for CPU usage of the burstable performance instance. Valid values are
* standard
and unlimited
. To change this attribute after launch, use
* ModifyInstanceCreditSpecification. For more information, see Burstable
* performance instances in the Amazon EC2 User Guide.
*
* Default: standard
(T2 instances) or unlimited
(T3/T3a/T4g instances)
*
*
* For T3 instances with host
tenancy, only standard
is supported.
*/
public void setCreditSpecification(CreditSpecificationRequest creditSpecification) {
this.creditSpecification = creditSpecification;
}
/**
*
* The credit option for CPU usage of the burstable performance instance. Valid values are standard
and
* unlimited
. To change this attribute after launch, use
* ModifyInstanceCreditSpecification. For more information, see Burstable
* performance instances in the Amazon EC2 User Guide.
*
*
* Default: standard
(T2 instances) or unlimited
(T3/T3a/T4g instances)
*
*
* For T3 instances with host
tenancy, only standard
is supported.
*
*
* @return The credit option for CPU usage of the burstable performance instance. Valid values are
* standard
and unlimited
. To change this attribute after launch, use
* ModifyInstanceCreditSpecification. For more information, see Burstable
* performance instances in the Amazon EC2 User Guide.
*
* Default: standard
(T2 instances) or unlimited
(T3/T3a/T4g instances)
*
*
* For T3 instances with host
tenancy, only standard
is supported.
*/
public CreditSpecificationRequest getCreditSpecification() {
return this.creditSpecification;
}
/**
*
* The credit option for CPU usage of the burstable performance instance. Valid values are standard
and
* unlimited
. To change this attribute after launch, use
* ModifyInstanceCreditSpecification. For more information, see Burstable
* performance instances in the Amazon EC2 User Guide.
*
*
* Default: standard
(T2 instances) or unlimited
(T3/T3a/T4g instances)
*
*
* For T3 instances with host
tenancy, only standard
is supported.
*
*
* @param creditSpecification
* The credit option for CPU usage of the burstable performance instance. Valid values are
* standard
and unlimited
. To change this attribute after launch, use
* ModifyInstanceCreditSpecification. For more information, see Burstable
* performance instances in the Amazon EC2 User Guide.
*
* Default: standard
(T2 instances) or unlimited
(T3/T3a/T4g instances)
*
*
* For T3 instances with host
tenancy, only standard
is supported.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withCreditSpecification(CreditSpecificationRequest creditSpecification) {
setCreditSpecification(creditSpecification);
return this;
}
/**
*
* The CPU options for the instance. For more information, see Optimize CPU options in
* the Amazon EC2 User Guide.
*
*
* @param cpuOptions
* The CPU options for the instance. For more information, see Optimize CPU
* options in the Amazon EC2 User Guide.
*/
public void setCpuOptions(CpuOptionsRequest cpuOptions) {
this.cpuOptions = cpuOptions;
}
/**
*
* The CPU options for the instance. For more information, see Optimize CPU options in
* the Amazon EC2 User Guide.
*
*
* @return The CPU options for the instance. For more information, see Optimize CPU
* options in the Amazon EC2 User Guide.
*/
public CpuOptionsRequest getCpuOptions() {
return this.cpuOptions;
}
/**
*
* The CPU options for the instance. For more information, see Optimize CPU options in
* the Amazon EC2 User Guide.
*
*
* @param cpuOptions
* The CPU options for the instance. For more information, see Optimize CPU
* options in the Amazon EC2 User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withCpuOptions(CpuOptionsRequest cpuOptions) {
setCpuOptions(cpuOptions);
return this;
}
/**
*
* Information about the Capacity Reservation targeting option. If you do not specify this parameter, the instance's
* Capacity Reservation preference defaults to open
, which enables it to run in any open Capacity
* Reservation that has matching attributes (instance type, platform, Availability Zone).
*
*
* @param capacityReservationSpecification
* Information about the Capacity Reservation targeting option. If you do not specify this parameter, the
* instance's Capacity Reservation preference defaults to open
, which enables it to run in any
* open Capacity Reservation that has matching attributes (instance type, platform, Availability Zone).
*/
public void setCapacityReservationSpecification(CapacityReservationSpecification capacityReservationSpecification) {
this.capacityReservationSpecification = capacityReservationSpecification;
}
/**
*
* Information about the Capacity Reservation targeting option. If you do not specify this parameter, the instance's
* Capacity Reservation preference defaults to open
, which enables it to run in any open Capacity
* Reservation that has matching attributes (instance type, platform, Availability Zone).
*
*
* @return Information about the Capacity Reservation targeting option. If you do not specify this parameter, the
* instance's Capacity Reservation preference defaults to open
, which enables it to run in any
* open Capacity Reservation that has matching attributes (instance type, platform, Availability Zone).
*/
public CapacityReservationSpecification getCapacityReservationSpecification() {
return this.capacityReservationSpecification;
}
/**
*
* Information about the Capacity Reservation targeting option. If you do not specify this parameter, the instance's
* Capacity Reservation preference defaults to open
, which enables it to run in any open Capacity
* Reservation that has matching attributes (instance type, platform, Availability Zone).
*
*
* @param capacityReservationSpecification
* Information about the Capacity Reservation targeting option. If you do not specify this parameter, the
* instance's Capacity Reservation preference defaults to open
, which enables it to run in any
* open Capacity Reservation that has matching attributes (instance type, platform, Availability Zone).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withCapacityReservationSpecification(CapacityReservationSpecification capacityReservationSpecification) {
setCapacityReservationSpecification(capacityReservationSpecification);
return this;
}
/**
*
* Indicates whether an instance is enabled for hibernation. This parameter is valid only if the instance meets the
* hibernation
* prerequisites. For more information, see Hibernate your instance in the
* Amazon EC2 User Guide.
*
*
* You can't enable hibernation and Amazon Web Services Nitro Enclaves on the same instance.
*
*
* @param hibernationOptions
* Indicates whether an instance is enabled for hibernation. This parameter is valid only if the instance
* meets the hibernation
* prerequisites. For more information, see Hibernate your instance in
* the Amazon EC2 User Guide.
*
* You can't enable hibernation and Amazon Web Services Nitro Enclaves on the same instance.
*/
public void setHibernationOptions(HibernationOptionsRequest hibernationOptions) {
this.hibernationOptions = hibernationOptions;
}
/**
*
* Indicates whether an instance is enabled for hibernation. This parameter is valid only if the instance meets the
* hibernation
* prerequisites. For more information, see Hibernate your instance in the
* Amazon EC2 User Guide.
*
*
* You can't enable hibernation and Amazon Web Services Nitro Enclaves on the same instance.
*
*
* @return Indicates whether an instance is enabled for hibernation. This parameter is valid only if the instance
* meets the hibernation
* prerequisites. For more information, see Hibernate your instance in
* the Amazon EC2 User Guide.
*
* You can't enable hibernation and Amazon Web Services Nitro Enclaves on the same instance.
*/
public HibernationOptionsRequest getHibernationOptions() {
return this.hibernationOptions;
}
/**
*
* Indicates whether an instance is enabled for hibernation. This parameter is valid only if the instance meets the
* hibernation
* prerequisites. For more information, see Hibernate your instance in the
* Amazon EC2 User Guide.
*
*
* You can't enable hibernation and Amazon Web Services Nitro Enclaves on the same instance.
*
*
* @param hibernationOptions
* Indicates whether an instance is enabled for hibernation. This parameter is valid only if the instance
* meets the hibernation
* prerequisites. For more information, see Hibernate your instance in
* the Amazon EC2 User Guide.
*
* You can't enable hibernation and Amazon Web Services Nitro Enclaves on the same instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withHibernationOptions(HibernationOptionsRequest hibernationOptions) {
setHibernationOptions(hibernationOptions);
return this;
}
/**
*
* The license configurations.
*
*
* @return The license configurations.
*/
public java.util.List getLicenseSpecifications() {
if (licenseSpecifications == null) {
licenseSpecifications = new com.amazonaws.internal.SdkInternalList();
}
return licenseSpecifications;
}
/**
*
* The license configurations.
*
*
* @param licenseSpecifications
* The license configurations.
*/
public void setLicenseSpecifications(java.util.Collection licenseSpecifications) {
if (licenseSpecifications == null) {
this.licenseSpecifications = null;
return;
}
this.licenseSpecifications = new com.amazonaws.internal.SdkInternalList(licenseSpecifications);
}
/**
*
* The license configurations.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setLicenseSpecifications(java.util.Collection)} or
* {@link #withLicenseSpecifications(java.util.Collection)} if you want to override the existing values.
*
*
* @param licenseSpecifications
* The license configurations.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withLicenseSpecifications(LicenseConfigurationRequest... licenseSpecifications) {
if (this.licenseSpecifications == null) {
setLicenseSpecifications(new com.amazonaws.internal.SdkInternalList(licenseSpecifications.length));
}
for (LicenseConfigurationRequest ele : licenseSpecifications) {
this.licenseSpecifications.add(ele);
}
return this;
}
/**
*
* The license configurations.
*
*
* @param licenseSpecifications
* The license configurations.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withLicenseSpecifications(java.util.Collection licenseSpecifications) {
setLicenseSpecifications(licenseSpecifications);
return this;
}
/**
*
* The metadata options for the instance. For more information, see Instance metadata and user
* data.
*
*
* @param metadataOptions
* The metadata options for the instance. For more information, see Instance metadata
* and user data.
*/
public void setMetadataOptions(InstanceMetadataOptionsRequest metadataOptions) {
this.metadataOptions = metadataOptions;
}
/**
*
* The metadata options for the instance. For more information, see Instance metadata and user
* data.
*
*
* @return The metadata options for the instance. For more information, see Instance metadata
* and user data.
*/
public InstanceMetadataOptionsRequest getMetadataOptions() {
return this.metadataOptions;
}
/**
*
* The metadata options for the instance. For more information, see Instance metadata and user
* data.
*
*
* @param metadataOptions
* The metadata options for the instance. For more information, see Instance metadata
* and user data.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withMetadataOptions(InstanceMetadataOptionsRequest metadataOptions) {
setMetadataOptions(metadataOptions);
return this;
}
/**
*
* Indicates whether the instance is enabled for Amazon Web Services Nitro Enclaves. For more information, see What is Amazon Web Services Nitro
* Enclaves? in the Amazon Web Services Nitro Enclaves User Guide.
*
*
* You can't enable Amazon Web Services Nitro Enclaves and hibernation on the same instance.
*
*
* @param enclaveOptions
* Indicates whether the instance is enabled for Amazon Web Services Nitro Enclaves. For more information,
* see What is Amazon Web
* Services Nitro Enclaves? in the Amazon Web Services Nitro Enclaves User Guide.
*
* You can't enable Amazon Web Services Nitro Enclaves and hibernation on the same instance.
*/
public void setEnclaveOptions(EnclaveOptionsRequest enclaveOptions) {
this.enclaveOptions = enclaveOptions;
}
/**
*
* Indicates whether the instance is enabled for Amazon Web Services Nitro Enclaves. For more information, see What is Amazon Web Services Nitro
* Enclaves? in the Amazon Web Services Nitro Enclaves User Guide.
*
*
* You can't enable Amazon Web Services Nitro Enclaves and hibernation on the same instance.
*
*
* @return Indicates whether the instance is enabled for Amazon Web Services Nitro Enclaves. For more information,
* see What is Amazon Web
* Services Nitro Enclaves? in the Amazon Web Services Nitro Enclaves User Guide.
*
* You can't enable Amazon Web Services Nitro Enclaves and hibernation on the same instance.
*/
public EnclaveOptionsRequest getEnclaveOptions() {
return this.enclaveOptions;
}
/**
*
* Indicates whether the instance is enabled for Amazon Web Services Nitro Enclaves. For more information, see What is Amazon Web Services Nitro
* Enclaves? in the Amazon Web Services Nitro Enclaves User Guide.
*
*
* You can't enable Amazon Web Services Nitro Enclaves and hibernation on the same instance.
*
*
* @param enclaveOptions
* Indicates whether the instance is enabled for Amazon Web Services Nitro Enclaves. For more information,
* see What is Amazon Web
* Services Nitro Enclaves? in the Amazon Web Services Nitro Enclaves User Guide.
*
* You can't enable Amazon Web Services Nitro Enclaves and hibernation on the same instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withEnclaveOptions(EnclaveOptionsRequest enclaveOptions) {
setEnclaveOptions(enclaveOptions);
return this;
}
/**
*
* The options for the instance hostname. The default values are inherited from the subnet. Applies only if creating
* a network interface, not attaching an existing one.
*
*
* @param privateDnsNameOptions
* The options for the instance hostname. The default values are inherited from the subnet. Applies only if
* creating a network interface, not attaching an existing one.
*/
public void setPrivateDnsNameOptions(PrivateDnsNameOptionsRequest privateDnsNameOptions) {
this.privateDnsNameOptions = privateDnsNameOptions;
}
/**
*
* The options for the instance hostname. The default values are inherited from the subnet. Applies only if creating
* a network interface, not attaching an existing one.
*
*
* @return The options for the instance hostname. The default values are inherited from the subnet. Applies only if
* creating a network interface, not attaching an existing one.
*/
public PrivateDnsNameOptionsRequest getPrivateDnsNameOptions() {
return this.privateDnsNameOptions;
}
/**
*
* The options for the instance hostname. The default values are inherited from the subnet. Applies only if creating
* a network interface, not attaching an existing one.
*
*
* @param privateDnsNameOptions
* The options for the instance hostname. The default values are inherited from the subnet. Applies only if
* creating a network interface, not attaching an existing one.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withPrivateDnsNameOptions(PrivateDnsNameOptionsRequest privateDnsNameOptions) {
setPrivateDnsNameOptions(privateDnsNameOptions);
return this;
}
/**
*
* The maintenance and recovery options for the instance.
*
*
* @param maintenanceOptions
* The maintenance and recovery options for the instance.
*/
public void setMaintenanceOptions(InstanceMaintenanceOptionsRequest maintenanceOptions) {
this.maintenanceOptions = maintenanceOptions;
}
/**
*
* The maintenance and recovery options for the instance.
*
*
* @return The maintenance and recovery options for the instance.
*/
public InstanceMaintenanceOptionsRequest getMaintenanceOptions() {
return this.maintenanceOptions;
}
/**
*
* The maintenance and recovery options for the instance.
*
*
* @param maintenanceOptions
* The maintenance and recovery options for the instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withMaintenanceOptions(InstanceMaintenanceOptionsRequest maintenanceOptions) {
setMaintenanceOptions(maintenanceOptions);
return this;
}
/**
*
* Indicates whether an instance is enabled for stop protection. For more information, see Stop
* protection.
*
*
* @param disableApiStop
* Indicates whether an instance is enabled for stop protection. For more information, see Stop
* protection.
*/
public void setDisableApiStop(Boolean disableApiStop) {
this.disableApiStop = disableApiStop;
}
/**
*
* Indicates whether an instance is enabled for stop protection. For more information, see Stop
* protection.
*
*
* @return Indicates whether an instance is enabled for stop protection. For more information, see Stop
* protection.
*/
public Boolean getDisableApiStop() {
return this.disableApiStop;
}
/**
*
* Indicates whether an instance is enabled for stop protection. For more information, see Stop
* protection.
*
*
* @param disableApiStop
* Indicates whether an instance is enabled for stop protection. For more information, see Stop
* protection.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withDisableApiStop(Boolean disableApiStop) {
setDisableApiStop(disableApiStop);
return this;
}
/**
*
* Indicates whether an instance is enabled for stop protection. For more information, see Stop
* protection.
*
*
* @return Indicates whether an instance is enabled for stop protection. For more information, see Stop
* protection.
*/
public Boolean isDisableApiStop() {
return this.disableApiStop;
}
/**
*
* If you’re launching an instance into a dual-stack or IPv6-only subnet, you can enable assigning a primary IPv6
* address. A primary IPv6 address is an IPv6 GUA address associated with an ENI that you have enabled to use a
* primary IPv6 address. Use this option if an instance relies on its IPv6 address not changing. When you launch the
* instance, Amazon Web Services will automatically assign an IPv6 address associated with the ENI attached to your
* instance to be the primary IPv6 address. Once you enable an IPv6 GUA address to be a primary IPv6, you cannot
* disable it. When you enable an IPv6 GUA address to be a primary IPv6, the first IPv6 GUA will be made the primary
* IPv6 address until the instance is terminated or the network interface is detached. If you have multiple IPv6
* addresses associated with an ENI attached to your instance and you enable a primary IPv6 address, the first IPv6
* GUA address associated with the ENI becomes the primary IPv6 address.
*
*
* @param enablePrimaryIpv6
* If you’re launching an instance into a dual-stack or IPv6-only subnet, you can enable assigning a primary
* IPv6 address. A primary IPv6 address is an IPv6 GUA address associated with an ENI that you have enabled
* to use a primary IPv6 address. Use this option if an instance relies on its IPv6 address not changing.
* When you launch the instance, Amazon Web Services will automatically assign an IPv6 address associated
* with the ENI attached to your instance to be the primary IPv6 address. Once you enable an IPv6 GUA address
* to be a primary IPv6, you cannot disable it. When you enable an IPv6 GUA address to be a primary IPv6, the
* first IPv6 GUA will be made the primary IPv6 address until the instance is terminated or the network
* interface is detached. If you have multiple IPv6 addresses associated with an ENI attached to your
* instance and you enable a primary IPv6 address, the first IPv6 GUA address associated with the ENI becomes
* the primary IPv6 address.
*/
public void setEnablePrimaryIpv6(Boolean enablePrimaryIpv6) {
this.enablePrimaryIpv6 = enablePrimaryIpv6;
}
/**
*
* If you’re launching an instance into a dual-stack or IPv6-only subnet, you can enable assigning a primary IPv6
* address. A primary IPv6 address is an IPv6 GUA address associated with an ENI that you have enabled to use a
* primary IPv6 address. Use this option if an instance relies on its IPv6 address not changing. When you launch the
* instance, Amazon Web Services will automatically assign an IPv6 address associated with the ENI attached to your
* instance to be the primary IPv6 address. Once you enable an IPv6 GUA address to be a primary IPv6, you cannot
* disable it. When you enable an IPv6 GUA address to be a primary IPv6, the first IPv6 GUA will be made the primary
* IPv6 address until the instance is terminated or the network interface is detached. If you have multiple IPv6
* addresses associated with an ENI attached to your instance and you enable a primary IPv6 address, the first IPv6
* GUA address associated with the ENI becomes the primary IPv6 address.
*
*
* @return If you’re launching an instance into a dual-stack or IPv6-only subnet, you can enable assigning a primary
* IPv6 address. A primary IPv6 address is an IPv6 GUA address associated with an ENI that you have enabled
* to use a primary IPv6 address. Use this option if an instance relies on its IPv6 address not changing.
* When you launch the instance, Amazon Web Services will automatically assign an IPv6 address associated
* with the ENI attached to your instance to be the primary IPv6 address. Once you enable an IPv6 GUA
* address to be a primary IPv6, you cannot disable it. When you enable an IPv6 GUA address to be a primary
* IPv6, the first IPv6 GUA will be made the primary IPv6 address until the instance is terminated or the
* network interface is detached. If you have multiple IPv6 addresses associated with an ENI attached to
* your instance and you enable a primary IPv6 address, the first IPv6 GUA address associated with the ENI
* becomes the primary IPv6 address.
*/
public Boolean getEnablePrimaryIpv6() {
return this.enablePrimaryIpv6;
}
/**
*
* If you’re launching an instance into a dual-stack or IPv6-only subnet, you can enable assigning a primary IPv6
* address. A primary IPv6 address is an IPv6 GUA address associated with an ENI that you have enabled to use a
* primary IPv6 address. Use this option if an instance relies on its IPv6 address not changing. When you launch the
* instance, Amazon Web Services will automatically assign an IPv6 address associated with the ENI attached to your
* instance to be the primary IPv6 address. Once you enable an IPv6 GUA address to be a primary IPv6, you cannot
* disable it. When you enable an IPv6 GUA address to be a primary IPv6, the first IPv6 GUA will be made the primary
* IPv6 address until the instance is terminated or the network interface is detached. If you have multiple IPv6
* addresses associated with an ENI attached to your instance and you enable a primary IPv6 address, the first IPv6
* GUA address associated with the ENI becomes the primary IPv6 address.
*
*
* @param enablePrimaryIpv6
* If you’re launching an instance into a dual-stack or IPv6-only subnet, you can enable assigning a primary
* IPv6 address. A primary IPv6 address is an IPv6 GUA address associated with an ENI that you have enabled
* to use a primary IPv6 address. Use this option if an instance relies on its IPv6 address not changing.
* When you launch the instance, Amazon Web Services will automatically assign an IPv6 address associated
* with the ENI attached to your instance to be the primary IPv6 address. Once you enable an IPv6 GUA address
* to be a primary IPv6, you cannot disable it. When you enable an IPv6 GUA address to be a primary IPv6, the
* first IPv6 GUA will be made the primary IPv6 address until the instance is terminated or the network
* interface is detached. If you have multiple IPv6 addresses associated with an ENI attached to your
* instance and you enable a primary IPv6 address, the first IPv6 GUA address associated with the ENI becomes
* the primary IPv6 address.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunInstancesRequest withEnablePrimaryIpv6(Boolean enablePrimaryIpv6) {
setEnablePrimaryIpv6(enablePrimaryIpv6);
return this;
}
/**
*
* If you’re launching an instance into a dual-stack or IPv6-only subnet, you can enable assigning a primary IPv6
* address. A primary IPv6 address is an IPv6 GUA address associated with an ENI that you have enabled to use a
* primary IPv6 address. Use this option if an instance relies on its IPv6 address not changing. When you launch the
* instance, Amazon Web Services will automatically assign an IPv6 address associated with the ENI attached to your
* instance to be the primary IPv6 address. Once you enable an IPv6 GUA address to be a primary IPv6, you cannot
* disable it. When you enable an IPv6 GUA address to be a primary IPv6, the first IPv6 GUA will be made the primary
* IPv6 address until the instance is terminated or the network interface is detached. If you have multiple IPv6
* addresses associated with an ENI attached to your instance and you enable a primary IPv6 address, the first IPv6
* GUA address associated with the ENI becomes the primary IPv6 address.
*
*
* @return If you’re launching an instance into a dual-stack or IPv6-only subnet, you can enable assigning a primary
* IPv6 address. A primary IPv6 address is an IPv6 GUA address associated with an ENI that you have enabled
* to use a primary IPv6 address. Use this option if an instance relies on its IPv6 address not changing.
* When you launch the instance, Amazon Web Services will automatically assign an IPv6 address associated
* with the ENI attached to your instance to be the primary IPv6 address. Once you enable an IPv6 GUA
* address to be a primary IPv6, you cannot disable it. When you enable an IPv6 GUA address to be a primary
* IPv6, the first IPv6 GUA will be made the primary IPv6 address until the instance is terminated or the
* network interface is detached. If you have multiple IPv6 addresses associated with an ENI attached to
* your instance and you enable a primary IPv6 address, the first IPv6 GUA address associated with the ENI
* becomes the primary IPv6 address.
*/
public Boolean isEnablePrimaryIpv6() {
return this.enablePrimaryIpv6;
}
/**
* This method is intended for internal use only. Returns the marshaled request configured with additional
* parameters to enable operation dry-run.
*/
@Override
public Request getDryRunRequest() {
Request request = new RunInstancesRequestMarshaller().marshall(this);
request.addParameter("DryRun", Boolean.toString(true));
return request;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getBlockDeviceMappings() != null)
sb.append("BlockDeviceMappings: ").append(getBlockDeviceMappings()).append(",");
if (getImageId() != null)
sb.append("ImageId: ").append(getImageId()).append(",");
if (getInstanceType() != null)
sb.append("InstanceType: ").append(getInstanceType()).append(",");
if (getIpv6AddressCount() != null)
sb.append("Ipv6AddressCount: ").append(getIpv6AddressCount()).append(",");
if (getIpv6Addresses() != null)
sb.append("Ipv6Addresses: ").append(getIpv6Addresses()).append(",");
if (getKernelId() != null)
sb.append("KernelId: ").append(getKernelId()).append(",");
if (getKeyName() != null)
sb.append("KeyName: ").append(getKeyName()).append(",");
if (getMaxCount() != null)
sb.append("MaxCount: ").append(getMaxCount()).append(",");
if (getMinCount() != null)
sb.append("MinCount: ").append(getMinCount()).append(",");
if (getMonitoring() != null)
sb.append("Monitoring: ").append(getMonitoring()).append(",");
if (getPlacement() != null)
sb.append("Placement: ").append(getPlacement()).append(",");
if (getRamdiskId() != null)
sb.append("RamdiskId: ").append(getRamdiskId()).append(",");
if (getSecurityGroupIds() != null)
sb.append("SecurityGroupIds: ").append(getSecurityGroupIds()).append(",");
if (getSecurityGroups() != null)
sb.append("SecurityGroups: ").append(getSecurityGroups()).append(",");
if (getSubnetId() != null)
sb.append("SubnetId: ").append(getSubnetId()).append(",");
if (getUserData() != null)
sb.append("UserData: ").append("***Sensitive Data Redacted***").append(",");
if (getAdditionalInfo() != null)
sb.append("AdditionalInfo: ").append(getAdditionalInfo()).append(",");
if (getClientToken() != null)
sb.append("ClientToken: ").append(getClientToken()).append(",");
if (getDisableApiTermination() != null)
sb.append("DisableApiTermination: ").append(getDisableApiTermination()).append(",");
if (getEbsOptimized() != null)
sb.append("EbsOptimized: ").append(getEbsOptimized()).append(",");
if (getIamInstanceProfile() != null)
sb.append("IamInstanceProfile: ").append(getIamInstanceProfile()).append(",");
if (getInstanceInitiatedShutdownBehavior() != null)
sb.append("InstanceInitiatedShutdownBehavior: ").append(getInstanceInitiatedShutdownBehavior()).append(",");
if (getNetworkInterfaces() != null)
sb.append("NetworkInterfaces: ").append(getNetworkInterfaces()).append(",");
if (getPrivateIpAddress() != null)
sb.append("PrivateIpAddress: ").append(getPrivateIpAddress()).append(",");
if (getElasticGpuSpecification() != null)
sb.append("ElasticGpuSpecification: ").append(getElasticGpuSpecification()).append(",");
if (getElasticInferenceAccelerators() != null)
sb.append("ElasticInferenceAccelerators: ").append(getElasticInferenceAccelerators()).append(",");
if (getTagSpecifications() != null)
sb.append("TagSpecifications: ").append(getTagSpecifications()).append(",");
if (getLaunchTemplate() != null)
sb.append("LaunchTemplate: ").append(getLaunchTemplate()).append(",");
if (getInstanceMarketOptions() != null)
sb.append("InstanceMarketOptions: ").append(getInstanceMarketOptions()).append(",");
if (getCreditSpecification() != null)
sb.append("CreditSpecification: ").append(getCreditSpecification()).append(",");
if (getCpuOptions() != null)
sb.append("CpuOptions: ").append(getCpuOptions()).append(",");
if (getCapacityReservationSpecification() != null)
sb.append("CapacityReservationSpecification: ").append(getCapacityReservationSpecification()).append(",");
if (getHibernationOptions() != null)
sb.append("HibernationOptions: ").append(getHibernationOptions()).append(",");
if (getLicenseSpecifications() != null)
sb.append("LicenseSpecifications: ").append(getLicenseSpecifications()).append(",");
if (getMetadataOptions() != null)
sb.append("MetadataOptions: ").append(getMetadataOptions()).append(",");
if (getEnclaveOptions() != null)
sb.append("EnclaveOptions: ").append(getEnclaveOptions()).append(",");
if (getPrivateDnsNameOptions() != null)
sb.append("PrivateDnsNameOptions: ").append(getPrivateDnsNameOptions()).append(",");
if (getMaintenanceOptions() != null)
sb.append("MaintenanceOptions: ").append(getMaintenanceOptions()).append(",");
if (getDisableApiStop() != null)
sb.append("DisableApiStop: ").append(getDisableApiStop()).append(",");
if (getEnablePrimaryIpv6() != null)
sb.append("EnablePrimaryIpv6: ").append(getEnablePrimaryIpv6());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof RunInstancesRequest == false)
return false;
RunInstancesRequest other = (RunInstancesRequest) obj;
if (other.getBlockDeviceMappings() == null ^ this.getBlockDeviceMappings() == null)
return false;
if (other.getBlockDeviceMappings() != null && other.getBlockDeviceMappings().equals(this.getBlockDeviceMappings()) == false)
return false;
if (other.getImageId() == null ^ this.getImageId() == null)
return false;
if (other.getImageId() != null && other.getImageId().equals(this.getImageId()) == false)
return false;
if (other.getInstanceType() == null ^ this.getInstanceType() == null)
return false;
if (other.getInstanceType() != null && other.getInstanceType().equals(this.getInstanceType()) == false)
return false;
if (other.getIpv6AddressCount() == null ^ this.getIpv6AddressCount() == null)
return false;
if (other.getIpv6AddressCount() != null && other.getIpv6AddressCount().equals(this.getIpv6AddressCount()) == false)
return false;
if (other.getIpv6Addresses() == null ^ this.getIpv6Addresses() == null)
return false;
if (other.getIpv6Addresses() != null && other.getIpv6Addresses().equals(this.getIpv6Addresses()) == false)
return false;
if (other.getKernelId() == null ^ this.getKernelId() == null)
return false;
if (other.getKernelId() != null && other.getKernelId().equals(this.getKernelId()) == false)
return false;
if (other.getKeyName() == null ^ this.getKeyName() == null)
return false;
if (other.getKeyName() != null && other.getKeyName().equals(this.getKeyName()) == false)
return false;
if (other.getMaxCount() == null ^ this.getMaxCount() == null)
return false;
if (other.getMaxCount() != null && other.getMaxCount().equals(this.getMaxCount()) == false)
return false;
if (other.getMinCount() == null ^ this.getMinCount() == null)
return false;
if (other.getMinCount() != null && other.getMinCount().equals(this.getMinCount()) == false)
return false;
if (other.getMonitoring() == null ^ this.getMonitoring() == null)
return false;
if (other.getMonitoring() != null && other.getMonitoring().equals(this.getMonitoring()) == false)
return false;
if (other.getPlacement() == null ^ this.getPlacement() == null)
return false;
if (other.getPlacement() != null && other.getPlacement().equals(this.getPlacement()) == false)
return false;
if (other.getRamdiskId() == null ^ this.getRamdiskId() == null)
return false;
if (other.getRamdiskId() != null && other.getRamdiskId().equals(this.getRamdiskId()) == false)
return false;
if (other.getSecurityGroupIds() == null ^ this.getSecurityGroupIds() == null)
return false;
if (other.getSecurityGroupIds() != null && other.getSecurityGroupIds().equals(this.getSecurityGroupIds()) == false)
return false;
if (other.getSecurityGroups() == null ^ this.getSecurityGroups() == null)
return false;
if (other.getSecurityGroups() != null && other.getSecurityGroups().equals(this.getSecurityGroups()) == false)
return false;
if (other.getSubnetId() == null ^ this.getSubnetId() == null)
return false;
if (other.getSubnetId() != null && other.getSubnetId().equals(this.getSubnetId()) == false)
return false;
if (other.getUserData() == null ^ this.getUserData() == null)
return false;
if (other.getUserData() != null && other.getUserData().equals(this.getUserData()) == false)
return false;
if (other.getAdditionalInfo() == null ^ this.getAdditionalInfo() == null)
return false;
if (other.getAdditionalInfo() != null && other.getAdditionalInfo().equals(this.getAdditionalInfo()) == false)
return false;
if (other.getClientToken() == null ^ this.getClientToken() == null)
return false;
if (other.getClientToken() != null && other.getClientToken().equals(this.getClientToken()) == false)
return false;
if (other.getDisableApiTermination() == null ^ this.getDisableApiTermination() == null)
return false;
if (other.getDisableApiTermination() != null && other.getDisableApiTermination().equals(this.getDisableApiTermination()) == false)
return false;
if (other.getEbsOptimized() == null ^ this.getEbsOptimized() == null)
return false;
if (other.getEbsOptimized() != null && other.getEbsOptimized().equals(this.getEbsOptimized()) == false)
return false;
if (other.getIamInstanceProfile() == null ^ this.getIamInstanceProfile() == null)
return false;
if (other.getIamInstanceProfile() != null && other.getIamInstanceProfile().equals(this.getIamInstanceProfile()) == false)
return false;
if (other.getInstanceInitiatedShutdownBehavior() == null ^ this.getInstanceInitiatedShutdownBehavior() == null)
return false;
if (other.getInstanceInitiatedShutdownBehavior() != null
&& other.getInstanceInitiatedShutdownBehavior().equals(this.getInstanceInitiatedShutdownBehavior()) == false)
return false;
if (other.getNetworkInterfaces() == null ^ this.getNetworkInterfaces() == null)
return false;
if (other.getNetworkInterfaces() != null && other.getNetworkInterfaces().equals(this.getNetworkInterfaces()) == false)
return false;
if (other.getPrivateIpAddress() == null ^ this.getPrivateIpAddress() == null)
return false;
if (other.getPrivateIpAddress() != null && other.getPrivateIpAddress().equals(this.getPrivateIpAddress()) == false)
return false;
if (other.getElasticGpuSpecification() == null ^ this.getElasticGpuSpecification() == null)
return false;
if (other.getElasticGpuSpecification() != null && other.getElasticGpuSpecification().equals(this.getElasticGpuSpecification()) == false)
return false;
if (other.getElasticInferenceAccelerators() == null ^ this.getElasticInferenceAccelerators() == null)
return false;
if (other.getElasticInferenceAccelerators() != null && other.getElasticInferenceAccelerators().equals(this.getElasticInferenceAccelerators()) == false)
return false;
if (other.getTagSpecifications() == null ^ this.getTagSpecifications() == null)
return false;
if (other.getTagSpecifications() != null && other.getTagSpecifications().equals(this.getTagSpecifications()) == false)
return false;
if (other.getLaunchTemplate() == null ^ this.getLaunchTemplate() == null)
return false;
if (other.getLaunchTemplate() != null && other.getLaunchTemplate().equals(this.getLaunchTemplate()) == false)
return false;
if (other.getInstanceMarketOptions() == null ^ this.getInstanceMarketOptions() == null)
return false;
if (other.getInstanceMarketOptions() != null && other.getInstanceMarketOptions().equals(this.getInstanceMarketOptions()) == false)
return false;
if (other.getCreditSpecification() == null ^ this.getCreditSpecification() == null)
return false;
if (other.getCreditSpecification() != null && other.getCreditSpecification().equals(this.getCreditSpecification()) == false)
return false;
if (other.getCpuOptions() == null ^ this.getCpuOptions() == null)
return false;
if (other.getCpuOptions() != null && other.getCpuOptions().equals(this.getCpuOptions()) == false)
return false;
if (other.getCapacityReservationSpecification() == null ^ this.getCapacityReservationSpecification() == null)
return false;
if (other.getCapacityReservationSpecification() != null
&& other.getCapacityReservationSpecification().equals(this.getCapacityReservationSpecification()) == false)
return false;
if (other.getHibernationOptions() == null ^ this.getHibernationOptions() == null)
return false;
if (other.getHibernationOptions() != null && other.getHibernationOptions().equals(this.getHibernationOptions()) == false)
return false;
if (other.getLicenseSpecifications() == null ^ this.getLicenseSpecifications() == null)
return false;
if (other.getLicenseSpecifications() != null && other.getLicenseSpecifications().equals(this.getLicenseSpecifications()) == false)
return false;
if (other.getMetadataOptions() == null ^ this.getMetadataOptions() == null)
return false;
if (other.getMetadataOptions() != null && other.getMetadataOptions().equals(this.getMetadataOptions()) == false)
return false;
if (other.getEnclaveOptions() == null ^ this.getEnclaveOptions() == null)
return false;
if (other.getEnclaveOptions() != null && other.getEnclaveOptions().equals(this.getEnclaveOptions()) == false)
return false;
if (other.getPrivateDnsNameOptions() == null ^ this.getPrivateDnsNameOptions() == null)
return false;
if (other.getPrivateDnsNameOptions() != null && other.getPrivateDnsNameOptions().equals(this.getPrivateDnsNameOptions()) == false)
return false;
if (other.getMaintenanceOptions() == null ^ this.getMaintenanceOptions() == null)
return false;
if (other.getMaintenanceOptions() != null && other.getMaintenanceOptions().equals(this.getMaintenanceOptions()) == false)
return false;
if (other.getDisableApiStop() == null ^ this.getDisableApiStop() == null)
return false;
if (other.getDisableApiStop() != null && other.getDisableApiStop().equals(this.getDisableApiStop()) == false)
return false;
if (other.getEnablePrimaryIpv6() == null ^ this.getEnablePrimaryIpv6() == null)
return false;
if (other.getEnablePrimaryIpv6() != null && other.getEnablePrimaryIpv6().equals(this.getEnablePrimaryIpv6()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getBlockDeviceMappings() == null) ? 0 : getBlockDeviceMappings().hashCode());
hashCode = prime * hashCode + ((getImageId() == null) ? 0 : getImageId().hashCode());
hashCode = prime * hashCode + ((getInstanceType() == null) ? 0 : getInstanceType().hashCode());
hashCode = prime * hashCode + ((getIpv6AddressCount() == null) ? 0 : getIpv6AddressCount().hashCode());
hashCode = prime * hashCode + ((getIpv6Addresses() == null) ? 0 : getIpv6Addresses().hashCode());
hashCode = prime * hashCode + ((getKernelId() == null) ? 0 : getKernelId().hashCode());
hashCode = prime * hashCode + ((getKeyName() == null) ? 0 : getKeyName().hashCode());
hashCode = prime * hashCode + ((getMaxCount() == null) ? 0 : getMaxCount().hashCode());
hashCode = prime * hashCode + ((getMinCount() == null) ? 0 : getMinCount().hashCode());
hashCode = prime * hashCode + ((getMonitoring() == null) ? 0 : getMonitoring().hashCode());
hashCode = prime * hashCode + ((getPlacement() == null) ? 0 : getPlacement().hashCode());
hashCode = prime * hashCode + ((getRamdiskId() == null) ? 0 : getRamdiskId().hashCode());
hashCode = prime * hashCode + ((getSecurityGroupIds() == null) ? 0 : getSecurityGroupIds().hashCode());
hashCode = prime * hashCode + ((getSecurityGroups() == null) ? 0 : getSecurityGroups().hashCode());
hashCode = prime * hashCode + ((getSubnetId() == null) ? 0 : getSubnetId().hashCode());
hashCode = prime * hashCode + ((getUserData() == null) ? 0 : getUserData().hashCode());
hashCode = prime * hashCode + ((getAdditionalInfo() == null) ? 0 : getAdditionalInfo().hashCode());
hashCode = prime * hashCode + ((getClientToken() == null) ? 0 : getClientToken().hashCode());
hashCode = prime * hashCode + ((getDisableApiTermination() == null) ? 0 : getDisableApiTermination().hashCode());
hashCode = prime * hashCode + ((getEbsOptimized() == null) ? 0 : getEbsOptimized().hashCode());
hashCode = prime * hashCode + ((getIamInstanceProfile() == null) ? 0 : getIamInstanceProfile().hashCode());
hashCode = prime * hashCode + ((getInstanceInitiatedShutdownBehavior() == null) ? 0 : getInstanceInitiatedShutdownBehavior().hashCode());
hashCode = prime * hashCode + ((getNetworkInterfaces() == null) ? 0 : getNetworkInterfaces().hashCode());
hashCode = prime * hashCode + ((getPrivateIpAddress() == null) ? 0 : getPrivateIpAddress().hashCode());
hashCode = prime * hashCode + ((getElasticGpuSpecification() == null) ? 0 : getElasticGpuSpecification().hashCode());
hashCode = prime * hashCode + ((getElasticInferenceAccelerators() == null) ? 0 : getElasticInferenceAccelerators().hashCode());
hashCode = prime * hashCode + ((getTagSpecifications() == null) ? 0 : getTagSpecifications().hashCode());
hashCode = prime * hashCode + ((getLaunchTemplate() == null) ? 0 : getLaunchTemplate().hashCode());
hashCode = prime * hashCode + ((getInstanceMarketOptions() == null) ? 0 : getInstanceMarketOptions().hashCode());
hashCode = prime * hashCode + ((getCreditSpecification() == null) ? 0 : getCreditSpecification().hashCode());
hashCode = prime * hashCode + ((getCpuOptions() == null) ? 0 : getCpuOptions().hashCode());
hashCode = prime * hashCode + ((getCapacityReservationSpecification() == null) ? 0 : getCapacityReservationSpecification().hashCode());
hashCode = prime * hashCode + ((getHibernationOptions() == null) ? 0 : getHibernationOptions().hashCode());
hashCode = prime * hashCode + ((getLicenseSpecifications() == null) ? 0 : getLicenseSpecifications().hashCode());
hashCode = prime * hashCode + ((getMetadataOptions() == null) ? 0 : getMetadataOptions().hashCode());
hashCode = prime * hashCode + ((getEnclaveOptions() == null) ? 0 : getEnclaveOptions().hashCode());
hashCode = prime * hashCode + ((getPrivateDnsNameOptions() == null) ? 0 : getPrivateDnsNameOptions().hashCode());
hashCode = prime * hashCode + ((getMaintenanceOptions() == null) ? 0 : getMaintenanceOptions().hashCode());
hashCode = prime * hashCode + ((getDisableApiStop() == null) ? 0 : getDisableApiStop().hashCode());
hashCode = prime * hashCode + ((getEnablePrimaryIpv6() == null) ? 0 : getEnablePrimaryIpv6().hashCode());
return hashCode;
}
@Override
public RunInstancesRequest clone() {
return (RunInstancesRequest) super.clone();
}
}