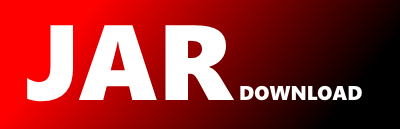
com.amazonaws.services.ec2.model.ModifyVpcEndpointServiceConfigurationRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ec2 Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ec2.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
import com.amazonaws.Request;
import com.amazonaws.services.ec2.model.transform.ModifyVpcEndpointServiceConfigurationRequestMarshaller;
/**
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ModifyVpcEndpointServiceConfigurationRequest extends AmazonWebServiceRequest implements Serializable, Cloneable,
DryRunSupportedRequest {
/**
*
* The ID of the service.
*
*/
private String serviceId;
/**
*
* (Interface endpoint configuration) The private DNS name to assign to the endpoint service.
*
*/
private String privateDnsName;
/**
*
* (Interface endpoint configuration) Removes the private DNS name of the endpoint service.
*
*/
private Boolean removePrivateDnsName;
/**
*
* Indicates whether requests to create an endpoint to your service must be accepted.
*
*/
private Boolean acceptanceRequired;
/**
*
* The Amazon Resource Names (ARNs) of Network Load Balancers to add to your service configuration.
*
*/
private com.amazonaws.internal.SdkInternalList addNetworkLoadBalancerArns;
/**
*
* The Amazon Resource Names (ARNs) of Network Load Balancers to remove from your service configuration.
*
*/
private com.amazonaws.internal.SdkInternalList removeNetworkLoadBalancerArns;
/**
*
* The Amazon Resource Names (ARNs) of Gateway Load Balancers to add to your service configuration.
*
*/
private com.amazonaws.internal.SdkInternalList addGatewayLoadBalancerArns;
/**
*
* The Amazon Resource Names (ARNs) of Gateway Load Balancers to remove from your service configuration.
*
*/
private com.amazonaws.internal.SdkInternalList removeGatewayLoadBalancerArns;
/**
*
* The IP address types to add to your service configuration.
*
*/
private com.amazonaws.internal.SdkInternalList addSupportedIpAddressTypes;
/**
*
* The IP address types to remove from your service configuration.
*
*/
private com.amazonaws.internal.SdkInternalList removeSupportedIpAddressTypes;
/**
*
* The ID of the service.
*
*
* @param serviceId
* The ID of the service.
*/
public void setServiceId(String serviceId) {
this.serviceId = serviceId;
}
/**
*
* The ID of the service.
*
*
* @return The ID of the service.
*/
public String getServiceId() {
return this.serviceId;
}
/**
*
* The ID of the service.
*
*
* @param serviceId
* The ID of the service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyVpcEndpointServiceConfigurationRequest withServiceId(String serviceId) {
setServiceId(serviceId);
return this;
}
/**
*
* (Interface endpoint configuration) The private DNS name to assign to the endpoint service.
*
*
* @param privateDnsName
* (Interface endpoint configuration) The private DNS name to assign to the endpoint service.
*/
public void setPrivateDnsName(String privateDnsName) {
this.privateDnsName = privateDnsName;
}
/**
*
* (Interface endpoint configuration) The private DNS name to assign to the endpoint service.
*
*
* @return (Interface endpoint configuration) The private DNS name to assign to the endpoint service.
*/
public String getPrivateDnsName() {
return this.privateDnsName;
}
/**
*
* (Interface endpoint configuration) The private DNS name to assign to the endpoint service.
*
*
* @param privateDnsName
* (Interface endpoint configuration) The private DNS name to assign to the endpoint service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyVpcEndpointServiceConfigurationRequest withPrivateDnsName(String privateDnsName) {
setPrivateDnsName(privateDnsName);
return this;
}
/**
*
* (Interface endpoint configuration) Removes the private DNS name of the endpoint service.
*
*
* @param removePrivateDnsName
* (Interface endpoint configuration) Removes the private DNS name of the endpoint service.
*/
public void setRemovePrivateDnsName(Boolean removePrivateDnsName) {
this.removePrivateDnsName = removePrivateDnsName;
}
/**
*
* (Interface endpoint configuration) Removes the private DNS name of the endpoint service.
*
*
* @return (Interface endpoint configuration) Removes the private DNS name of the endpoint service.
*/
public Boolean getRemovePrivateDnsName() {
return this.removePrivateDnsName;
}
/**
*
* (Interface endpoint configuration) Removes the private DNS name of the endpoint service.
*
*
* @param removePrivateDnsName
* (Interface endpoint configuration) Removes the private DNS name of the endpoint service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyVpcEndpointServiceConfigurationRequest withRemovePrivateDnsName(Boolean removePrivateDnsName) {
setRemovePrivateDnsName(removePrivateDnsName);
return this;
}
/**
*
* (Interface endpoint configuration) Removes the private DNS name of the endpoint service.
*
*
* @return (Interface endpoint configuration) Removes the private DNS name of the endpoint service.
*/
public Boolean isRemovePrivateDnsName() {
return this.removePrivateDnsName;
}
/**
*
* Indicates whether requests to create an endpoint to your service must be accepted.
*
*
* @param acceptanceRequired
* Indicates whether requests to create an endpoint to your service must be accepted.
*/
public void setAcceptanceRequired(Boolean acceptanceRequired) {
this.acceptanceRequired = acceptanceRequired;
}
/**
*
* Indicates whether requests to create an endpoint to your service must be accepted.
*
*
* @return Indicates whether requests to create an endpoint to your service must be accepted.
*/
public Boolean getAcceptanceRequired() {
return this.acceptanceRequired;
}
/**
*
* Indicates whether requests to create an endpoint to your service must be accepted.
*
*
* @param acceptanceRequired
* Indicates whether requests to create an endpoint to your service must be accepted.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyVpcEndpointServiceConfigurationRequest withAcceptanceRequired(Boolean acceptanceRequired) {
setAcceptanceRequired(acceptanceRequired);
return this;
}
/**
*
* Indicates whether requests to create an endpoint to your service must be accepted.
*
*
* @return Indicates whether requests to create an endpoint to your service must be accepted.
*/
public Boolean isAcceptanceRequired() {
return this.acceptanceRequired;
}
/**
*
* The Amazon Resource Names (ARNs) of Network Load Balancers to add to your service configuration.
*
*
* @return The Amazon Resource Names (ARNs) of Network Load Balancers to add to your service configuration.
*/
public java.util.List getAddNetworkLoadBalancerArns() {
if (addNetworkLoadBalancerArns == null) {
addNetworkLoadBalancerArns = new com.amazonaws.internal.SdkInternalList();
}
return addNetworkLoadBalancerArns;
}
/**
*
* The Amazon Resource Names (ARNs) of Network Load Balancers to add to your service configuration.
*
*
* @param addNetworkLoadBalancerArns
* The Amazon Resource Names (ARNs) of Network Load Balancers to add to your service configuration.
*/
public void setAddNetworkLoadBalancerArns(java.util.Collection addNetworkLoadBalancerArns) {
if (addNetworkLoadBalancerArns == null) {
this.addNetworkLoadBalancerArns = null;
return;
}
this.addNetworkLoadBalancerArns = new com.amazonaws.internal.SdkInternalList(addNetworkLoadBalancerArns);
}
/**
*
* The Amazon Resource Names (ARNs) of Network Load Balancers to add to your service configuration.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAddNetworkLoadBalancerArns(java.util.Collection)} or
* {@link #withAddNetworkLoadBalancerArns(java.util.Collection)} if you want to override the existing values.
*
*
* @param addNetworkLoadBalancerArns
* The Amazon Resource Names (ARNs) of Network Load Balancers to add to your service configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyVpcEndpointServiceConfigurationRequest withAddNetworkLoadBalancerArns(String... addNetworkLoadBalancerArns) {
if (this.addNetworkLoadBalancerArns == null) {
setAddNetworkLoadBalancerArns(new com.amazonaws.internal.SdkInternalList(addNetworkLoadBalancerArns.length));
}
for (String ele : addNetworkLoadBalancerArns) {
this.addNetworkLoadBalancerArns.add(ele);
}
return this;
}
/**
*
* The Amazon Resource Names (ARNs) of Network Load Balancers to add to your service configuration.
*
*
* @param addNetworkLoadBalancerArns
* The Amazon Resource Names (ARNs) of Network Load Balancers to add to your service configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyVpcEndpointServiceConfigurationRequest withAddNetworkLoadBalancerArns(java.util.Collection addNetworkLoadBalancerArns) {
setAddNetworkLoadBalancerArns(addNetworkLoadBalancerArns);
return this;
}
/**
*
* The Amazon Resource Names (ARNs) of Network Load Balancers to remove from your service configuration.
*
*
* @return The Amazon Resource Names (ARNs) of Network Load Balancers to remove from your service configuration.
*/
public java.util.List getRemoveNetworkLoadBalancerArns() {
if (removeNetworkLoadBalancerArns == null) {
removeNetworkLoadBalancerArns = new com.amazonaws.internal.SdkInternalList();
}
return removeNetworkLoadBalancerArns;
}
/**
*
* The Amazon Resource Names (ARNs) of Network Load Balancers to remove from your service configuration.
*
*
* @param removeNetworkLoadBalancerArns
* The Amazon Resource Names (ARNs) of Network Load Balancers to remove from your service configuration.
*/
public void setRemoveNetworkLoadBalancerArns(java.util.Collection removeNetworkLoadBalancerArns) {
if (removeNetworkLoadBalancerArns == null) {
this.removeNetworkLoadBalancerArns = null;
return;
}
this.removeNetworkLoadBalancerArns = new com.amazonaws.internal.SdkInternalList(removeNetworkLoadBalancerArns);
}
/**
*
* The Amazon Resource Names (ARNs) of Network Load Balancers to remove from your service configuration.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRemoveNetworkLoadBalancerArns(java.util.Collection)} or
* {@link #withRemoveNetworkLoadBalancerArns(java.util.Collection)} if you want to override the existing values.
*
*
* @param removeNetworkLoadBalancerArns
* The Amazon Resource Names (ARNs) of Network Load Balancers to remove from your service configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyVpcEndpointServiceConfigurationRequest withRemoveNetworkLoadBalancerArns(String... removeNetworkLoadBalancerArns) {
if (this.removeNetworkLoadBalancerArns == null) {
setRemoveNetworkLoadBalancerArns(new com.amazonaws.internal.SdkInternalList(removeNetworkLoadBalancerArns.length));
}
for (String ele : removeNetworkLoadBalancerArns) {
this.removeNetworkLoadBalancerArns.add(ele);
}
return this;
}
/**
*
* The Amazon Resource Names (ARNs) of Network Load Balancers to remove from your service configuration.
*
*
* @param removeNetworkLoadBalancerArns
* The Amazon Resource Names (ARNs) of Network Load Balancers to remove from your service configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyVpcEndpointServiceConfigurationRequest withRemoveNetworkLoadBalancerArns(java.util.Collection removeNetworkLoadBalancerArns) {
setRemoveNetworkLoadBalancerArns(removeNetworkLoadBalancerArns);
return this;
}
/**
*
* The Amazon Resource Names (ARNs) of Gateway Load Balancers to add to your service configuration.
*
*
* @return The Amazon Resource Names (ARNs) of Gateway Load Balancers to add to your service configuration.
*/
public java.util.List getAddGatewayLoadBalancerArns() {
if (addGatewayLoadBalancerArns == null) {
addGatewayLoadBalancerArns = new com.amazonaws.internal.SdkInternalList();
}
return addGatewayLoadBalancerArns;
}
/**
*
* The Amazon Resource Names (ARNs) of Gateway Load Balancers to add to your service configuration.
*
*
* @param addGatewayLoadBalancerArns
* The Amazon Resource Names (ARNs) of Gateway Load Balancers to add to your service configuration.
*/
public void setAddGatewayLoadBalancerArns(java.util.Collection addGatewayLoadBalancerArns) {
if (addGatewayLoadBalancerArns == null) {
this.addGatewayLoadBalancerArns = null;
return;
}
this.addGatewayLoadBalancerArns = new com.amazonaws.internal.SdkInternalList(addGatewayLoadBalancerArns);
}
/**
*
* The Amazon Resource Names (ARNs) of Gateway Load Balancers to add to your service configuration.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAddGatewayLoadBalancerArns(java.util.Collection)} or
* {@link #withAddGatewayLoadBalancerArns(java.util.Collection)} if you want to override the existing values.
*
*
* @param addGatewayLoadBalancerArns
* The Amazon Resource Names (ARNs) of Gateway Load Balancers to add to your service configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyVpcEndpointServiceConfigurationRequest withAddGatewayLoadBalancerArns(String... addGatewayLoadBalancerArns) {
if (this.addGatewayLoadBalancerArns == null) {
setAddGatewayLoadBalancerArns(new com.amazonaws.internal.SdkInternalList(addGatewayLoadBalancerArns.length));
}
for (String ele : addGatewayLoadBalancerArns) {
this.addGatewayLoadBalancerArns.add(ele);
}
return this;
}
/**
*
* The Amazon Resource Names (ARNs) of Gateway Load Balancers to add to your service configuration.
*
*
* @param addGatewayLoadBalancerArns
* The Amazon Resource Names (ARNs) of Gateway Load Balancers to add to your service configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyVpcEndpointServiceConfigurationRequest withAddGatewayLoadBalancerArns(java.util.Collection addGatewayLoadBalancerArns) {
setAddGatewayLoadBalancerArns(addGatewayLoadBalancerArns);
return this;
}
/**
*
* The Amazon Resource Names (ARNs) of Gateway Load Balancers to remove from your service configuration.
*
*
* @return The Amazon Resource Names (ARNs) of Gateway Load Balancers to remove from your service configuration.
*/
public java.util.List getRemoveGatewayLoadBalancerArns() {
if (removeGatewayLoadBalancerArns == null) {
removeGatewayLoadBalancerArns = new com.amazonaws.internal.SdkInternalList();
}
return removeGatewayLoadBalancerArns;
}
/**
*
* The Amazon Resource Names (ARNs) of Gateway Load Balancers to remove from your service configuration.
*
*
* @param removeGatewayLoadBalancerArns
* The Amazon Resource Names (ARNs) of Gateway Load Balancers to remove from your service configuration.
*/
public void setRemoveGatewayLoadBalancerArns(java.util.Collection removeGatewayLoadBalancerArns) {
if (removeGatewayLoadBalancerArns == null) {
this.removeGatewayLoadBalancerArns = null;
return;
}
this.removeGatewayLoadBalancerArns = new com.amazonaws.internal.SdkInternalList(removeGatewayLoadBalancerArns);
}
/**
*
* The Amazon Resource Names (ARNs) of Gateway Load Balancers to remove from your service configuration.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRemoveGatewayLoadBalancerArns(java.util.Collection)} or
* {@link #withRemoveGatewayLoadBalancerArns(java.util.Collection)} if you want to override the existing values.
*
*
* @param removeGatewayLoadBalancerArns
* The Amazon Resource Names (ARNs) of Gateway Load Balancers to remove from your service configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyVpcEndpointServiceConfigurationRequest withRemoveGatewayLoadBalancerArns(String... removeGatewayLoadBalancerArns) {
if (this.removeGatewayLoadBalancerArns == null) {
setRemoveGatewayLoadBalancerArns(new com.amazonaws.internal.SdkInternalList(removeGatewayLoadBalancerArns.length));
}
for (String ele : removeGatewayLoadBalancerArns) {
this.removeGatewayLoadBalancerArns.add(ele);
}
return this;
}
/**
*
* The Amazon Resource Names (ARNs) of Gateway Load Balancers to remove from your service configuration.
*
*
* @param removeGatewayLoadBalancerArns
* The Amazon Resource Names (ARNs) of Gateway Load Balancers to remove from your service configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyVpcEndpointServiceConfigurationRequest withRemoveGatewayLoadBalancerArns(java.util.Collection removeGatewayLoadBalancerArns) {
setRemoveGatewayLoadBalancerArns(removeGatewayLoadBalancerArns);
return this;
}
/**
*
* The IP address types to add to your service configuration.
*
*
* @return The IP address types to add to your service configuration.
*/
public java.util.List getAddSupportedIpAddressTypes() {
if (addSupportedIpAddressTypes == null) {
addSupportedIpAddressTypes = new com.amazonaws.internal.SdkInternalList();
}
return addSupportedIpAddressTypes;
}
/**
*
* The IP address types to add to your service configuration.
*
*
* @param addSupportedIpAddressTypes
* The IP address types to add to your service configuration.
*/
public void setAddSupportedIpAddressTypes(java.util.Collection addSupportedIpAddressTypes) {
if (addSupportedIpAddressTypes == null) {
this.addSupportedIpAddressTypes = null;
return;
}
this.addSupportedIpAddressTypes = new com.amazonaws.internal.SdkInternalList(addSupportedIpAddressTypes);
}
/**
*
* The IP address types to add to your service configuration.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAddSupportedIpAddressTypes(java.util.Collection)} or
* {@link #withAddSupportedIpAddressTypes(java.util.Collection)} if you want to override the existing values.
*
*
* @param addSupportedIpAddressTypes
* The IP address types to add to your service configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyVpcEndpointServiceConfigurationRequest withAddSupportedIpAddressTypes(String... addSupportedIpAddressTypes) {
if (this.addSupportedIpAddressTypes == null) {
setAddSupportedIpAddressTypes(new com.amazonaws.internal.SdkInternalList(addSupportedIpAddressTypes.length));
}
for (String ele : addSupportedIpAddressTypes) {
this.addSupportedIpAddressTypes.add(ele);
}
return this;
}
/**
*
* The IP address types to add to your service configuration.
*
*
* @param addSupportedIpAddressTypes
* The IP address types to add to your service configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyVpcEndpointServiceConfigurationRequest withAddSupportedIpAddressTypes(java.util.Collection addSupportedIpAddressTypes) {
setAddSupportedIpAddressTypes(addSupportedIpAddressTypes);
return this;
}
/**
*
* The IP address types to remove from your service configuration.
*
*
* @return The IP address types to remove from your service configuration.
*/
public java.util.List getRemoveSupportedIpAddressTypes() {
if (removeSupportedIpAddressTypes == null) {
removeSupportedIpAddressTypes = new com.amazonaws.internal.SdkInternalList();
}
return removeSupportedIpAddressTypes;
}
/**
*
* The IP address types to remove from your service configuration.
*
*
* @param removeSupportedIpAddressTypes
* The IP address types to remove from your service configuration.
*/
public void setRemoveSupportedIpAddressTypes(java.util.Collection removeSupportedIpAddressTypes) {
if (removeSupportedIpAddressTypes == null) {
this.removeSupportedIpAddressTypes = null;
return;
}
this.removeSupportedIpAddressTypes = new com.amazonaws.internal.SdkInternalList(removeSupportedIpAddressTypes);
}
/**
*
* The IP address types to remove from your service configuration.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRemoveSupportedIpAddressTypes(java.util.Collection)} or
* {@link #withRemoveSupportedIpAddressTypes(java.util.Collection)} if you want to override the existing values.
*
*
* @param removeSupportedIpAddressTypes
* The IP address types to remove from your service configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyVpcEndpointServiceConfigurationRequest withRemoveSupportedIpAddressTypes(String... removeSupportedIpAddressTypes) {
if (this.removeSupportedIpAddressTypes == null) {
setRemoveSupportedIpAddressTypes(new com.amazonaws.internal.SdkInternalList(removeSupportedIpAddressTypes.length));
}
for (String ele : removeSupportedIpAddressTypes) {
this.removeSupportedIpAddressTypes.add(ele);
}
return this;
}
/**
*
* The IP address types to remove from your service configuration.
*
*
* @param removeSupportedIpAddressTypes
* The IP address types to remove from your service configuration.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ModifyVpcEndpointServiceConfigurationRequest withRemoveSupportedIpAddressTypes(java.util.Collection removeSupportedIpAddressTypes) {
setRemoveSupportedIpAddressTypes(removeSupportedIpAddressTypes);
return this;
}
/**
* This method is intended for internal use only. Returns the marshaled request configured with additional
* parameters to enable operation dry-run.
*/
@Override
public Request getDryRunRequest() {
Request request = new ModifyVpcEndpointServiceConfigurationRequestMarshaller().marshall(this);
request.addParameter("DryRun", Boolean.toString(true));
return request;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getServiceId() != null)
sb.append("ServiceId: ").append(getServiceId()).append(",");
if (getPrivateDnsName() != null)
sb.append("PrivateDnsName: ").append(getPrivateDnsName()).append(",");
if (getRemovePrivateDnsName() != null)
sb.append("RemovePrivateDnsName: ").append(getRemovePrivateDnsName()).append(",");
if (getAcceptanceRequired() != null)
sb.append("AcceptanceRequired: ").append(getAcceptanceRequired()).append(",");
if (getAddNetworkLoadBalancerArns() != null)
sb.append("AddNetworkLoadBalancerArns: ").append(getAddNetworkLoadBalancerArns()).append(",");
if (getRemoveNetworkLoadBalancerArns() != null)
sb.append("RemoveNetworkLoadBalancerArns: ").append(getRemoveNetworkLoadBalancerArns()).append(",");
if (getAddGatewayLoadBalancerArns() != null)
sb.append("AddGatewayLoadBalancerArns: ").append(getAddGatewayLoadBalancerArns()).append(",");
if (getRemoveGatewayLoadBalancerArns() != null)
sb.append("RemoveGatewayLoadBalancerArns: ").append(getRemoveGatewayLoadBalancerArns()).append(",");
if (getAddSupportedIpAddressTypes() != null)
sb.append("AddSupportedIpAddressTypes: ").append(getAddSupportedIpAddressTypes()).append(",");
if (getRemoveSupportedIpAddressTypes() != null)
sb.append("RemoveSupportedIpAddressTypes: ").append(getRemoveSupportedIpAddressTypes());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ModifyVpcEndpointServiceConfigurationRequest == false)
return false;
ModifyVpcEndpointServiceConfigurationRequest other = (ModifyVpcEndpointServiceConfigurationRequest) obj;
if (other.getServiceId() == null ^ this.getServiceId() == null)
return false;
if (other.getServiceId() != null && other.getServiceId().equals(this.getServiceId()) == false)
return false;
if (other.getPrivateDnsName() == null ^ this.getPrivateDnsName() == null)
return false;
if (other.getPrivateDnsName() != null && other.getPrivateDnsName().equals(this.getPrivateDnsName()) == false)
return false;
if (other.getRemovePrivateDnsName() == null ^ this.getRemovePrivateDnsName() == null)
return false;
if (other.getRemovePrivateDnsName() != null && other.getRemovePrivateDnsName().equals(this.getRemovePrivateDnsName()) == false)
return false;
if (other.getAcceptanceRequired() == null ^ this.getAcceptanceRequired() == null)
return false;
if (other.getAcceptanceRequired() != null && other.getAcceptanceRequired().equals(this.getAcceptanceRequired()) == false)
return false;
if (other.getAddNetworkLoadBalancerArns() == null ^ this.getAddNetworkLoadBalancerArns() == null)
return false;
if (other.getAddNetworkLoadBalancerArns() != null && other.getAddNetworkLoadBalancerArns().equals(this.getAddNetworkLoadBalancerArns()) == false)
return false;
if (other.getRemoveNetworkLoadBalancerArns() == null ^ this.getRemoveNetworkLoadBalancerArns() == null)
return false;
if (other.getRemoveNetworkLoadBalancerArns() != null
&& other.getRemoveNetworkLoadBalancerArns().equals(this.getRemoveNetworkLoadBalancerArns()) == false)
return false;
if (other.getAddGatewayLoadBalancerArns() == null ^ this.getAddGatewayLoadBalancerArns() == null)
return false;
if (other.getAddGatewayLoadBalancerArns() != null && other.getAddGatewayLoadBalancerArns().equals(this.getAddGatewayLoadBalancerArns()) == false)
return false;
if (other.getRemoveGatewayLoadBalancerArns() == null ^ this.getRemoveGatewayLoadBalancerArns() == null)
return false;
if (other.getRemoveGatewayLoadBalancerArns() != null
&& other.getRemoveGatewayLoadBalancerArns().equals(this.getRemoveGatewayLoadBalancerArns()) == false)
return false;
if (other.getAddSupportedIpAddressTypes() == null ^ this.getAddSupportedIpAddressTypes() == null)
return false;
if (other.getAddSupportedIpAddressTypes() != null && other.getAddSupportedIpAddressTypes().equals(this.getAddSupportedIpAddressTypes()) == false)
return false;
if (other.getRemoveSupportedIpAddressTypes() == null ^ this.getRemoveSupportedIpAddressTypes() == null)
return false;
if (other.getRemoveSupportedIpAddressTypes() != null
&& other.getRemoveSupportedIpAddressTypes().equals(this.getRemoveSupportedIpAddressTypes()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getServiceId() == null) ? 0 : getServiceId().hashCode());
hashCode = prime * hashCode + ((getPrivateDnsName() == null) ? 0 : getPrivateDnsName().hashCode());
hashCode = prime * hashCode + ((getRemovePrivateDnsName() == null) ? 0 : getRemovePrivateDnsName().hashCode());
hashCode = prime * hashCode + ((getAcceptanceRequired() == null) ? 0 : getAcceptanceRequired().hashCode());
hashCode = prime * hashCode + ((getAddNetworkLoadBalancerArns() == null) ? 0 : getAddNetworkLoadBalancerArns().hashCode());
hashCode = prime * hashCode + ((getRemoveNetworkLoadBalancerArns() == null) ? 0 : getRemoveNetworkLoadBalancerArns().hashCode());
hashCode = prime * hashCode + ((getAddGatewayLoadBalancerArns() == null) ? 0 : getAddGatewayLoadBalancerArns().hashCode());
hashCode = prime * hashCode + ((getRemoveGatewayLoadBalancerArns() == null) ? 0 : getRemoveGatewayLoadBalancerArns().hashCode());
hashCode = prime * hashCode + ((getAddSupportedIpAddressTypes() == null) ? 0 : getAddSupportedIpAddressTypes().hashCode());
hashCode = prime * hashCode + ((getRemoveSupportedIpAddressTypes() == null) ? 0 : getRemoveSupportedIpAddressTypes().hashCode());
return hashCode;
}
@Override
public ModifyVpcEndpointServiceConfigurationRequest clone() {
return (ModifyVpcEndpointServiceConfigurationRequest) super.clone();
}
}