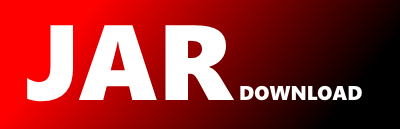
com.amazonaws.services.ec2.model.AllocateIpamPoolCidrRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ec2 Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ec2.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
import com.amazonaws.Request;
import com.amazonaws.services.ec2.model.transform.AllocateIpamPoolCidrRequestMarshaller;
/**
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AllocateIpamPoolCidrRequest extends AmazonWebServiceRequest implements Serializable, Cloneable,
DryRunSupportedRequest {
/**
*
* The ID of the IPAM pool from which you would like to allocate a CIDR.
*
*/
private String ipamPoolId;
/**
*
* The CIDR you would like to allocate from the IPAM pool. Note the following:
*
*
* -
*
* If there is no DefaultNetmaskLength allocation rule set on the pool, you must specify either the NetmaskLength or
* the CIDR.
*
*
* -
*
* If the DefaultNetmaskLength allocation rule is set on the pool, you can specify either the NetmaskLength or the
* CIDR and the DefaultNetmaskLength allocation rule will be ignored.
*
*
*
*
* Possible values: Any available IPv4 or IPv6 CIDR.
*
*/
private String cidr;
/**
*
* The netmask length of the CIDR you would like to allocate from the IPAM pool. Note the following:
*
*
* -
*
* If there is no DefaultNetmaskLength allocation rule set on the pool, you must specify either the NetmaskLength or
* the CIDR.
*
*
* -
*
* If the DefaultNetmaskLength allocation rule is set on the pool, you can specify either the NetmaskLength or the
* CIDR and the DefaultNetmaskLength allocation rule will be ignored.
*
*
*
*
* Possible netmask lengths for IPv4 addresses are 0 - 32. Possible netmask lengths for IPv6 addresses are 0 - 128.
*
*/
private Integer netmaskLength;
/**
*
* A unique, case-sensitive identifier that you provide to ensure the idempotency of the request. For more
* information, see Ensuring
* idempotency.
*
*/
private String clientToken;
/**
*
* A description for the allocation.
*
*/
private String description;
/**
*
* A preview of the next available CIDR in a pool.
*
*/
private Boolean previewNextCidr;
/**
*
* Include a particular CIDR range that can be returned by the pool. Allowed CIDRs are only allowed if using netmask
* length for allocation.
*
*/
private com.amazonaws.internal.SdkInternalList allowedCidrs;
/**
*
* Exclude a particular CIDR range from being returned by the pool. Disallowed CIDRs are only allowed if using
* netmask length for allocation.
*
*/
private com.amazonaws.internal.SdkInternalList disallowedCidrs;
/**
*
* The ID of the IPAM pool from which you would like to allocate a CIDR.
*
*
* @param ipamPoolId
* The ID of the IPAM pool from which you would like to allocate a CIDR.
*/
public void setIpamPoolId(String ipamPoolId) {
this.ipamPoolId = ipamPoolId;
}
/**
*
* The ID of the IPAM pool from which you would like to allocate a CIDR.
*
*
* @return The ID of the IPAM pool from which you would like to allocate a CIDR.
*/
public String getIpamPoolId() {
return this.ipamPoolId;
}
/**
*
* The ID of the IPAM pool from which you would like to allocate a CIDR.
*
*
* @param ipamPoolId
* The ID of the IPAM pool from which you would like to allocate a CIDR.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocateIpamPoolCidrRequest withIpamPoolId(String ipamPoolId) {
setIpamPoolId(ipamPoolId);
return this;
}
/**
*
* The CIDR you would like to allocate from the IPAM pool. Note the following:
*
*
* -
*
* If there is no DefaultNetmaskLength allocation rule set on the pool, you must specify either the NetmaskLength or
* the CIDR.
*
*
* -
*
* If the DefaultNetmaskLength allocation rule is set on the pool, you can specify either the NetmaskLength or the
* CIDR and the DefaultNetmaskLength allocation rule will be ignored.
*
*
*
*
* Possible values: Any available IPv4 or IPv6 CIDR.
*
*
* @param cidr
* The CIDR you would like to allocate from the IPAM pool. Note the following:
*
* -
*
* If there is no DefaultNetmaskLength allocation rule set on the pool, you must specify either the
* NetmaskLength or the CIDR.
*
*
* -
*
* If the DefaultNetmaskLength allocation rule is set on the pool, you can specify either the NetmaskLength
* or the CIDR and the DefaultNetmaskLength allocation rule will be ignored.
*
*
*
*
* Possible values: Any available IPv4 or IPv6 CIDR.
*/
public void setCidr(String cidr) {
this.cidr = cidr;
}
/**
*
* The CIDR you would like to allocate from the IPAM pool. Note the following:
*
*
* -
*
* If there is no DefaultNetmaskLength allocation rule set on the pool, you must specify either the NetmaskLength or
* the CIDR.
*
*
* -
*
* If the DefaultNetmaskLength allocation rule is set on the pool, you can specify either the NetmaskLength or the
* CIDR and the DefaultNetmaskLength allocation rule will be ignored.
*
*
*
*
* Possible values: Any available IPv4 or IPv6 CIDR.
*
*
* @return The CIDR you would like to allocate from the IPAM pool. Note the following:
*
* -
*
* If there is no DefaultNetmaskLength allocation rule set on the pool, you must specify either the
* NetmaskLength or the CIDR.
*
*
* -
*
* If the DefaultNetmaskLength allocation rule is set on the pool, you can specify either the NetmaskLength
* or the CIDR and the DefaultNetmaskLength allocation rule will be ignored.
*
*
*
*
* Possible values: Any available IPv4 or IPv6 CIDR.
*/
public String getCidr() {
return this.cidr;
}
/**
*
* The CIDR you would like to allocate from the IPAM pool. Note the following:
*
*
* -
*
* If there is no DefaultNetmaskLength allocation rule set on the pool, you must specify either the NetmaskLength or
* the CIDR.
*
*
* -
*
* If the DefaultNetmaskLength allocation rule is set on the pool, you can specify either the NetmaskLength or the
* CIDR and the DefaultNetmaskLength allocation rule will be ignored.
*
*
*
*
* Possible values: Any available IPv4 or IPv6 CIDR.
*
*
* @param cidr
* The CIDR you would like to allocate from the IPAM pool. Note the following:
*
* -
*
* If there is no DefaultNetmaskLength allocation rule set on the pool, you must specify either the
* NetmaskLength or the CIDR.
*
*
* -
*
* If the DefaultNetmaskLength allocation rule is set on the pool, you can specify either the NetmaskLength
* or the CIDR and the DefaultNetmaskLength allocation rule will be ignored.
*
*
*
*
* Possible values: Any available IPv4 or IPv6 CIDR.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocateIpamPoolCidrRequest withCidr(String cidr) {
setCidr(cidr);
return this;
}
/**
*
* The netmask length of the CIDR you would like to allocate from the IPAM pool. Note the following:
*
*
* -
*
* If there is no DefaultNetmaskLength allocation rule set on the pool, you must specify either the NetmaskLength or
* the CIDR.
*
*
* -
*
* If the DefaultNetmaskLength allocation rule is set on the pool, you can specify either the NetmaskLength or the
* CIDR and the DefaultNetmaskLength allocation rule will be ignored.
*
*
*
*
* Possible netmask lengths for IPv4 addresses are 0 - 32. Possible netmask lengths for IPv6 addresses are 0 - 128.
*
*
* @param netmaskLength
* The netmask length of the CIDR you would like to allocate from the IPAM pool. Note the following:
*
* -
*
* If there is no DefaultNetmaskLength allocation rule set on the pool, you must specify either the
* NetmaskLength or the CIDR.
*
*
* -
*
* If the DefaultNetmaskLength allocation rule is set on the pool, you can specify either the NetmaskLength
* or the CIDR and the DefaultNetmaskLength allocation rule will be ignored.
*
*
*
*
* Possible netmask lengths for IPv4 addresses are 0 - 32. Possible netmask lengths for IPv6 addresses are 0
* - 128.
*/
public void setNetmaskLength(Integer netmaskLength) {
this.netmaskLength = netmaskLength;
}
/**
*
* The netmask length of the CIDR you would like to allocate from the IPAM pool. Note the following:
*
*
* -
*
* If there is no DefaultNetmaskLength allocation rule set on the pool, you must specify either the NetmaskLength or
* the CIDR.
*
*
* -
*
* If the DefaultNetmaskLength allocation rule is set on the pool, you can specify either the NetmaskLength or the
* CIDR and the DefaultNetmaskLength allocation rule will be ignored.
*
*
*
*
* Possible netmask lengths for IPv4 addresses are 0 - 32. Possible netmask lengths for IPv6 addresses are 0 - 128.
*
*
* @return The netmask length of the CIDR you would like to allocate from the IPAM pool. Note the following:
*
* -
*
* If there is no DefaultNetmaskLength allocation rule set on the pool, you must specify either the
* NetmaskLength or the CIDR.
*
*
* -
*
* If the DefaultNetmaskLength allocation rule is set on the pool, you can specify either the NetmaskLength
* or the CIDR and the DefaultNetmaskLength allocation rule will be ignored.
*
*
*
*
* Possible netmask lengths for IPv4 addresses are 0 - 32. Possible netmask lengths for IPv6 addresses are 0
* - 128.
*/
public Integer getNetmaskLength() {
return this.netmaskLength;
}
/**
*
* The netmask length of the CIDR you would like to allocate from the IPAM pool. Note the following:
*
*
* -
*
* If there is no DefaultNetmaskLength allocation rule set on the pool, you must specify either the NetmaskLength or
* the CIDR.
*
*
* -
*
* If the DefaultNetmaskLength allocation rule is set on the pool, you can specify either the NetmaskLength or the
* CIDR and the DefaultNetmaskLength allocation rule will be ignored.
*
*
*
*
* Possible netmask lengths for IPv4 addresses are 0 - 32. Possible netmask lengths for IPv6 addresses are 0 - 128.
*
*
* @param netmaskLength
* The netmask length of the CIDR you would like to allocate from the IPAM pool. Note the following:
*
* -
*
* If there is no DefaultNetmaskLength allocation rule set on the pool, you must specify either the
* NetmaskLength or the CIDR.
*
*
* -
*
* If the DefaultNetmaskLength allocation rule is set on the pool, you can specify either the NetmaskLength
* or the CIDR and the DefaultNetmaskLength allocation rule will be ignored.
*
*
*
*
* Possible netmask lengths for IPv4 addresses are 0 - 32. Possible netmask lengths for IPv6 addresses are 0
* - 128.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocateIpamPoolCidrRequest withNetmaskLength(Integer netmaskLength) {
setNetmaskLength(netmaskLength);
return this;
}
/**
*
* A unique, case-sensitive identifier that you provide to ensure the idempotency of the request. For more
* information, see Ensuring
* idempotency.
*
*
* @param clientToken
* A unique, case-sensitive identifier that you provide to ensure the idempotency of the request. For more
* information, see Ensuring idempotency.
*/
public void setClientToken(String clientToken) {
this.clientToken = clientToken;
}
/**
*
* A unique, case-sensitive identifier that you provide to ensure the idempotency of the request. For more
* information, see Ensuring
* idempotency.
*
*
* @return A unique, case-sensitive identifier that you provide to ensure the idempotency of the request. For more
* information, see Ensuring idempotency.
*/
public String getClientToken() {
return this.clientToken;
}
/**
*
* A unique, case-sensitive identifier that you provide to ensure the idempotency of the request. For more
* information, see Ensuring
* idempotency.
*
*
* @param clientToken
* A unique, case-sensitive identifier that you provide to ensure the idempotency of the request. For more
* information, see Ensuring idempotency.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocateIpamPoolCidrRequest withClientToken(String clientToken) {
setClientToken(clientToken);
return this;
}
/**
*
* A description for the allocation.
*
*
* @param description
* A description for the allocation.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A description for the allocation.
*
*
* @return A description for the allocation.
*/
public String getDescription() {
return this.description;
}
/**
*
* A description for the allocation.
*
*
* @param description
* A description for the allocation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocateIpamPoolCidrRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* A preview of the next available CIDR in a pool.
*
*
* @param previewNextCidr
* A preview of the next available CIDR in a pool.
*/
public void setPreviewNextCidr(Boolean previewNextCidr) {
this.previewNextCidr = previewNextCidr;
}
/**
*
* A preview of the next available CIDR in a pool.
*
*
* @return A preview of the next available CIDR in a pool.
*/
public Boolean getPreviewNextCidr() {
return this.previewNextCidr;
}
/**
*
* A preview of the next available CIDR in a pool.
*
*
* @param previewNextCidr
* A preview of the next available CIDR in a pool.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocateIpamPoolCidrRequest withPreviewNextCidr(Boolean previewNextCidr) {
setPreviewNextCidr(previewNextCidr);
return this;
}
/**
*
* A preview of the next available CIDR in a pool.
*
*
* @return A preview of the next available CIDR in a pool.
*/
public Boolean isPreviewNextCidr() {
return this.previewNextCidr;
}
/**
*
* Include a particular CIDR range that can be returned by the pool. Allowed CIDRs are only allowed if using netmask
* length for allocation.
*
*
* @return Include a particular CIDR range that can be returned by the pool. Allowed CIDRs are only allowed if using
* netmask length for allocation.
*/
public java.util.List getAllowedCidrs() {
if (allowedCidrs == null) {
allowedCidrs = new com.amazonaws.internal.SdkInternalList();
}
return allowedCidrs;
}
/**
*
* Include a particular CIDR range that can be returned by the pool. Allowed CIDRs are only allowed if using netmask
* length for allocation.
*
*
* @param allowedCidrs
* Include a particular CIDR range that can be returned by the pool. Allowed CIDRs are only allowed if using
* netmask length for allocation.
*/
public void setAllowedCidrs(java.util.Collection allowedCidrs) {
if (allowedCidrs == null) {
this.allowedCidrs = null;
return;
}
this.allowedCidrs = new com.amazonaws.internal.SdkInternalList(allowedCidrs);
}
/**
*
* Include a particular CIDR range that can be returned by the pool. Allowed CIDRs are only allowed if using netmask
* length for allocation.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAllowedCidrs(java.util.Collection)} or {@link #withAllowedCidrs(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param allowedCidrs
* Include a particular CIDR range that can be returned by the pool. Allowed CIDRs are only allowed if using
* netmask length for allocation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocateIpamPoolCidrRequest withAllowedCidrs(String... allowedCidrs) {
if (this.allowedCidrs == null) {
setAllowedCidrs(new com.amazonaws.internal.SdkInternalList(allowedCidrs.length));
}
for (String ele : allowedCidrs) {
this.allowedCidrs.add(ele);
}
return this;
}
/**
*
* Include a particular CIDR range that can be returned by the pool. Allowed CIDRs are only allowed if using netmask
* length for allocation.
*
*
* @param allowedCidrs
* Include a particular CIDR range that can be returned by the pool. Allowed CIDRs are only allowed if using
* netmask length for allocation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocateIpamPoolCidrRequest withAllowedCidrs(java.util.Collection allowedCidrs) {
setAllowedCidrs(allowedCidrs);
return this;
}
/**
*
* Exclude a particular CIDR range from being returned by the pool. Disallowed CIDRs are only allowed if using
* netmask length for allocation.
*
*
* @return Exclude a particular CIDR range from being returned by the pool. Disallowed CIDRs are only allowed if
* using netmask length for allocation.
*/
public java.util.List getDisallowedCidrs() {
if (disallowedCidrs == null) {
disallowedCidrs = new com.amazonaws.internal.SdkInternalList();
}
return disallowedCidrs;
}
/**
*
* Exclude a particular CIDR range from being returned by the pool. Disallowed CIDRs are only allowed if using
* netmask length for allocation.
*
*
* @param disallowedCidrs
* Exclude a particular CIDR range from being returned by the pool. Disallowed CIDRs are only allowed if
* using netmask length for allocation.
*/
public void setDisallowedCidrs(java.util.Collection disallowedCidrs) {
if (disallowedCidrs == null) {
this.disallowedCidrs = null;
return;
}
this.disallowedCidrs = new com.amazonaws.internal.SdkInternalList(disallowedCidrs);
}
/**
*
* Exclude a particular CIDR range from being returned by the pool. Disallowed CIDRs are only allowed if using
* netmask length for allocation.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDisallowedCidrs(java.util.Collection)} or {@link #withDisallowedCidrs(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param disallowedCidrs
* Exclude a particular CIDR range from being returned by the pool. Disallowed CIDRs are only allowed if
* using netmask length for allocation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocateIpamPoolCidrRequest withDisallowedCidrs(String... disallowedCidrs) {
if (this.disallowedCidrs == null) {
setDisallowedCidrs(new com.amazonaws.internal.SdkInternalList(disallowedCidrs.length));
}
for (String ele : disallowedCidrs) {
this.disallowedCidrs.add(ele);
}
return this;
}
/**
*
* Exclude a particular CIDR range from being returned by the pool. Disallowed CIDRs are only allowed if using
* netmask length for allocation.
*
*
* @param disallowedCidrs
* Exclude a particular CIDR range from being returned by the pool. Disallowed CIDRs are only allowed if
* using netmask length for allocation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AllocateIpamPoolCidrRequest withDisallowedCidrs(java.util.Collection disallowedCidrs) {
setDisallowedCidrs(disallowedCidrs);
return this;
}
/**
* This method is intended for internal use only. Returns the marshaled request configured with additional
* parameters to enable operation dry-run.
*/
@Override
public Request getDryRunRequest() {
Request request = new AllocateIpamPoolCidrRequestMarshaller().marshall(this);
request.addParameter("DryRun", Boolean.toString(true));
return request;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getIpamPoolId() != null)
sb.append("IpamPoolId: ").append(getIpamPoolId()).append(",");
if (getCidr() != null)
sb.append("Cidr: ").append(getCidr()).append(",");
if (getNetmaskLength() != null)
sb.append("NetmaskLength: ").append(getNetmaskLength()).append(",");
if (getClientToken() != null)
sb.append("ClientToken: ").append(getClientToken()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getPreviewNextCidr() != null)
sb.append("PreviewNextCidr: ").append(getPreviewNextCidr()).append(",");
if (getAllowedCidrs() != null)
sb.append("AllowedCidrs: ").append(getAllowedCidrs()).append(",");
if (getDisallowedCidrs() != null)
sb.append("DisallowedCidrs: ").append(getDisallowedCidrs());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof AllocateIpamPoolCidrRequest == false)
return false;
AllocateIpamPoolCidrRequest other = (AllocateIpamPoolCidrRequest) obj;
if (other.getIpamPoolId() == null ^ this.getIpamPoolId() == null)
return false;
if (other.getIpamPoolId() != null && other.getIpamPoolId().equals(this.getIpamPoolId()) == false)
return false;
if (other.getCidr() == null ^ this.getCidr() == null)
return false;
if (other.getCidr() != null && other.getCidr().equals(this.getCidr()) == false)
return false;
if (other.getNetmaskLength() == null ^ this.getNetmaskLength() == null)
return false;
if (other.getNetmaskLength() != null && other.getNetmaskLength().equals(this.getNetmaskLength()) == false)
return false;
if (other.getClientToken() == null ^ this.getClientToken() == null)
return false;
if (other.getClientToken() != null && other.getClientToken().equals(this.getClientToken()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getPreviewNextCidr() == null ^ this.getPreviewNextCidr() == null)
return false;
if (other.getPreviewNextCidr() != null && other.getPreviewNextCidr().equals(this.getPreviewNextCidr()) == false)
return false;
if (other.getAllowedCidrs() == null ^ this.getAllowedCidrs() == null)
return false;
if (other.getAllowedCidrs() != null && other.getAllowedCidrs().equals(this.getAllowedCidrs()) == false)
return false;
if (other.getDisallowedCidrs() == null ^ this.getDisallowedCidrs() == null)
return false;
if (other.getDisallowedCidrs() != null && other.getDisallowedCidrs().equals(this.getDisallowedCidrs()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getIpamPoolId() == null) ? 0 : getIpamPoolId().hashCode());
hashCode = prime * hashCode + ((getCidr() == null) ? 0 : getCidr().hashCode());
hashCode = prime * hashCode + ((getNetmaskLength() == null) ? 0 : getNetmaskLength().hashCode());
hashCode = prime * hashCode + ((getClientToken() == null) ? 0 : getClientToken().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getPreviewNextCidr() == null) ? 0 : getPreviewNextCidr().hashCode());
hashCode = prime * hashCode + ((getAllowedCidrs() == null) ? 0 : getAllowedCidrs().hashCode());
hashCode = prime * hashCode + ((getDisallowedCidrs() == null) ? 0 : getDisallowedCidrs().hashCode());
return hashCode;
}
@Override
public AllocateIpamPoolCidrRequest clone() {
return (AllocateIpamPoolCidrRequest) super.clone();
}
}