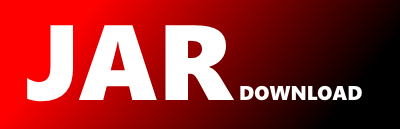
com.amazonaws.services.ecs.AmazonECSAsync Maven / Gradle / Ivy
/*
* Copyright 2010-2016 Amazon.com, Inc. or its affiliates. All Rights
* Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.ecs;
import com.amazonaws.services.ecs.model.*;
/**
* Interface for accessing Amazon ECS asynchronously. Each asynchronous method
* will return a Java Future object representing the asynchronous operation;
* overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
*
* Amazon EC2 Container Service (Amazon ECS) is a highly scalable, fast,
* container management service that makes it easy to run, stop, and manage
* Docker containers on a cluster of EC2 instances. Amazon ECS lets you launch
* and stop container-enabled applications with simple API calls, allows you to
* get the state of your cluster from a centralized service, and gives you
* access to many familiar Amazon EC2 features like security groups, Amazon EBS
* volumes, and IAM roles.
*
*
* You can use Amazon ECS to schedule the placement of containers across your
* cluster based on your resource needs, isolation policies, and availability
* requirements. Amazon EC2 Container Service eliminates the need for you to
* operate your own cluster management and configuration management systems or
* worry about scaling your management infrastructure.
*
*/
public interface AmazonECSAsync extends AmazonECS {
/**
*
* Creates a new Amazon ECS cluster. By default, your account receives a
* default
cluster when you launch your first container
* instance. However, you can create your own cluster with a unique name
* with the CreateCluster
action.
*
*
* @param createClusterRequest
* @return A Java Future containing the result of the CreateCluster
* operation returned by the service.
* @sample AmazonECSAsync.CreateCluster
*/
java.util.concurrent.Future createClusterAsync(
CreateClusterRequest createClusterRequest);
/**
*
* Creates a new Amazon ECS cluster. By default, your account receives a
* default
cluster when you launch your first container
* instance. However, you can create your own cluster with a unique name
* with the CreateCluster
action.
*
*
* @param createClusterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateCluster
* operation returned by the service.
* @sample AmazonECSAsyncHandler.CreateCluster
*/
java.util.concurrent.Future createClusterAsync(
CreateClusterRequest createClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the CreateCluster operation.
*
* @see #createClusterAsync(CreateClusterRequest)
*/
java.util.concurrent.Future createClusterAsync();
/**
* Simplified method form for invoking the CreateCluster operation with an
* AsyncHandler.
*
* @see #createClusterAsync(CreateClusterRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future createClusterAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Runs and maintains a desired number of tasks from a specified task
* definition. If the number of tasks running in a service drops below
* desiredCount
, Amazon ECS spawns another instantiation of the
* task in the specified cluster. To update an existing service, see
* UpdateService.
*
*
* You can optionally specify a deployment configuration for your service.
* During a deployment (which is triggered by changing the task definition
* of a service with an UpdateService operation), the service
* scheduler uses the minimumHealthyPercent
and
* maximumPercent
parameters to determine the deployment
* strategy.
*
*
* If the minimumHealthyPercent
is below 100%, the scheduler
* can ignore the desiredCount
temporarily during a deployment.
* For example, if your service has a desiredCount
of four
* tasks, a minimumHealthyPercent
of 50% allows the scheduler
* to stop two existing tasks before starting two new tasks. Tasks for
* services that do not use a load balancer are considered healthy if
* they are in the RUNNING
state; tasks for services that
* do use a load balancer are considered healthy if they are in the
* RUNNING
state and the container instance it is hosted on is
* reported as healthy by the load balancer. The default value for
* minimumHealthyPercent
is 50% in the console and 100% for the
* AWS CLI, the AWS SDKs, and the APIs.
*
*
* The maximumPercent
parameter represents an upper limit on
* the number of running tasks during a deployment, which enables you to
* define the deployment batch size. For example, if your service has a
* desiredCount
of four tasks, a maximumPercent
* value of 200% starts four new tasks before stopping the four older tasks
* (provided that the cluster resources required to do this are available).
* The default value for maximumPercent
is 200%.
*
*
* When the service scheduler launches new tasks, it attempts to balance
* them across the Availability Zones in your cluster with the following
* logic:
*
*
* -
*
* Determine which of the container instances in your cluster can support
* your service's task definition (for example, they have the required CPU,
* memory, ports, and container instance attributes).
*
*
* -
*
* Sort the valid container instances by the fewest number of running tasks
* for this service in the same Availability Zone as the instance. For
* example, if zone A has one running service task and zones B and C each
* have zero, valid container instances in either zone B or C are considered
* optimal for placement.
*
*
* -
*
* Place the new service task on a valid container instance in an optimal
* Availability Zone (based on the previous steps), favoring container
* instances with the fewest number of running tasks for this service.
*
*
*
*
* @param createServiceRequest
* @return A Java Future containing the result of the CreateService
* operation returned by the service.
* @sample AmazonECSAsync.CreateService
*/
java.util.concurrent.Future createServiceAsync(
CreateServiceRequest createServiceRequest);
/**
*
* Runs and maintains a desired number of tasks from a specified task
* definition. If the number of tasks running in a service drops below
* desiredCount
, Amazon ECS spawns another instantiation of the
* task in the specified cluster. To update an existing service, see
* UpdateService.
*
*
* You can optionally specify a deployment configuration for your service.
* During a deployment (which is triggered by changing the task definition
* of a service with an UpdateService operation), the service
* scheduler uses the minimumHealthyPercent
and
* maximumPercent
parameters to determine the deployment
* strategy.
*
*
* If the minimumHealthyPercent
is below 100%, the scheduler
* can ignore the desiredCount
temporarily during a deployment.
* For example, if your service has a desiredCount
of four
* tasks, a minimumHealthyPercent
of 50% allows the scheduler
* to stop two existing tasks before starting two new tasks. Tasks for
* services that do not use a load balancer are considered healthy if
* they are in the RUNNING
state; tasks for services that
* do use a load balancer are considered healthy if they are in the
* RUNNING
state and the container instance it is hosted on is
* reported as healthy by the load balancer. The default value for
* minimumHealthyPercent
is 50% in the console and 100% for the
* AWS CLI, the AWS SDKs, and the APIs.
*
*
* The maximumPercent
parameter represents an upper limit on
* the number of running tasks during a deployment, which enables you to
* define the deployment batch size. For example, if your service has a
* desiredCount
of four tasks, a maximumPercent
* value of 200% starts four new tasks before stopping the four older tasks
* (provided that the cluster resources required to do this are available).
* The default value for maximumPercent
is 200%.
*
*
* When the service scheduler launches new tasks, it attempts to balance
* them across the Availability Zones in your cluster with the following
* logic:
*
*
* -
*
* Determine which of the container instances in your cluster can support
* your service's task definition (for example, they have the required CPU,
* memory, ports, and container instance attributes).
*
*
* -
*
* Sort the valid container instances by the fewest number of running tasks
* for this service in the same Availability Zone as the instance. For
* example, if zone A has one running service task and zones B and C each
* have zero, valid container instances in either zone B or C are considered
* optimal for placement.
*
*
* -
*
* Place the new service task on a valid container instance in an optimal
* Availability Zone (based on the previous steps), favoring container
* instances with the fewest number of running tasks for this service.
*
*
*
*
* @param createServiceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateService
* operation returned by the service.
* @sample AmazonECSAsyncHandler.CreateService
*/
java.util.concurrent.Future createServiceAsync(
CreateServiceRequest createServiceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified cluster. You must deregister all container
* instances from this cluster before you may delete it. You can list the
* container instances in a cluster with ListContainerInstances and
* deregister them with DeregisterContainerInstance.
*
*
* @param deleteClusterRequest
* @return A Java Future containing the result of the DeleteCluster
* operation returned by the service.
* @sample AmazonECSAsync.DeleteCluster
*/
java.util.concurrent.Future deleteClusterAsync(
DeleteClusterRequest deleteClusterRequest);
/**
*
* Deletes the specified cluster. You must deregister all container
* instances from this cluster before you may delete it. You can list the
* container instances in a cluster with ListContainerInstances and
* deregister them with DeregisterContainerInstance.
*
*
* @param deleteClusterRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteCluster
* operation returned by the service.
* @sample AmazonECSAsyncHandler.DeleteCluster
*/
java.util.concurrent.Future deleteClusterAsync(
DeleteClusterRequest deleteClusterRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a specified service within a cluster. You can delete a service if
* you have no running tasks in it and the desired task count is zero. If
* the service is actively maintaining tasks, you cannot delete it, and you
* must update the service to a desired task count of zero. For more
* information, see UpdateService.
*
*
*
* When you delete a service, if there are still running tasks that require
* cleanup, the service status moves from ACTIVE
to
* DRAINING
, and the service is no longer visible in the
* console or in ListServices API operations. After the tasks have
* stopped, then the service status moves from DRAINING
to
* INACTIVE
. Services in the DRAINING
or
* INACTIVE
status can still be viewed with
* DescribeServices API operations; however, in the future,
* INACTIVE
services may be cleaned up and purged from Amazon
* ECS record keeping, and DescribeServices API operations on those
* services will return a ServiceNotFoundException
error.
*
*
*
* @param deleteServiceRequest
* @return A Java Future containing the result of the DeleteService
* operation returned by the service.
* @sample AmazonECSAsync.DeleteService
*/
java.util.concurrent.Future deleteServiceAsync(
DeleteServiceRequest deleteServiceRequest);
/**
*
* Deletes a specified service within a cluster. You can delete a service if
* you have no running tasks in it and the desired task count is zero. If
* the service is actively maintaining tasks, you cannot delete it, and you
* must update the service to a desired task count of zero. For more
* information, see UpdateService.
*
*
*
* When you delete a service, if there are still running tasks that require
* cleanup, the service status moves from ACTIVE
to
* DRAINING
, and the service is no longer visible in the
* console or in ListServices API operations. After the tasks have
* stopped, then the service status moves from DRAINING
to
* INACTIVE
. Services in the DRAINING
or
* INACTIVE
status can still be viewed with
* DescribeServices API operations; however, in the future,
* INACTIVE
services may be cleaned up and purged from Amazon
* ECS record keeping, and DescribeServices API operations on those
* services will return a ServiceNotFoundException
error.
*
*
*
* @param deleteServiceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteService
* operation returned by the service.
* @sample AmazonECSAsyncHandler.DeleteService
*/
java.util.concurrent.Future deleteServiceAsync(
DeleteServiceRequest deleteServiceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deregisters an Amazon ECS container instance from the specified cluster.
* This instance is no longer available to run tasks.
*
*
* If you intend to use the container instance for some other purpose after
* deregistration, you should stop all of the tasks running on the container
* instance before deregistration to avoid any orphaned tasks from consuming
* resources.
*
*
* Deregistering a container instance removes the instance from a cluster,
* but it does not terminate the EC2 instance; if you are finished using the
* instance, be sure to terminate it in the Amazon EC2 console to stop
* billing.
*
*
*
* When you terminate a container instance, it is automatically deregistered
* from your cluster.
*
*
*
* @param deregisterContainerInstanceRequest
* @return A Java Future containing the result of the
* DeregisterContainerInstance operation returned by the service.
* @sample AmazonECSAsync.DeregisterContainerInstance
*/
java.util.concurrent.Future deregisterContainerInstanceAsync(
DeregisterContainerInstanceRequest deregisterContainerInstanceRequest);
/**
*
* Deregisters an Amazon ECS container instance from the specified cluster.
* This instance is no longer available to run tasks.
*
*
* If you intend to use the container instance for some other purpose after
* deregistration, you should stop all of the tasks running on the container
* instance before deregistration to avoid any orphaned tasks from consuming
* resources.
*
*
* Deregistering a container instance removes the instance from a cluster,
* but it does not terminate the EC2 instance; if you are finished using the
* instance, be sure to terminate it in the Amazon EC2 console to stop
* billing.
*
*
*
* When you terminate a container instance, it is automatically deregistered
* from your cluster.
*
*
*
* @param deregisterContainerInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DeregisterContainerInstance operation returned by the service.
* @sample AmazonECSAsyncHandler.DeregisterContainerInstance
*/
java.util.concurrent.Future deregisterContainerInstanceAsync(
DeregisterContainerInstanceRequest deregisterContainerInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deregisters the specified task definition by family and revision. Upon
* deregistration, the task definition is marked as INACTIVE
.
* Existing tasks and services that reference an INACTIVE
task
* definition continue to run without disruption. Existing services that
* reference an INACTIVE
task definition can still scale up or
* down by modifying the service's desired count.
*
*
* You cannot use an INACTIVE
task definition to run new tasks
* or create new services, and you cannot update an existing service to
* reference an INACTIVE
task definition (although there may be
* up to a 10 minute window following deregistration where these
* restrictions have not yet taken effect).
*
*
* @param deregisterTaskDefinitionRequest
* @return A Java Future containing the result of the
* DeregisterTaskDefinition operation returned by the service.
* @sample AmazonECSAsync.DeregisterTaskDefinition
*/
java.util.concurrent.Future deregisterTaskDefinitionAsync(
DeregisterTaskDefinitionRequest deregisterTaskDefinitionRequest);
/**
*
* Deregisters the specified task definition by family and revision. Upon
* deregistration, the task definition is marked as INACTIVE
.
* Existing tasks and services that reference an INACTIVE
task
* definition continue to run without disruption. Existing services that
* reference an INACTIVE
task definition can still scale up or
* down by modifying the service's desired count.
*
*
* You cannot use an INACTIVE
task definition to run new tasks
* or create new services, and you cannot update an existing service to
* reference an INACTIVE
task definition (although there may be
* up to a 10 minute window following deregistration where these
* restrictions have not yet taken effect).
*
*
* @param deregisterTaskDefinitionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DeregisterTaskDefinition operation returned by the service.
* @sample AmazonECSAsyncHandler.DeregisterTaskDefinition
*/
java.util.concurrent.Future deregisterTaskDefinitionAsync(
DeregisterTaskDefinitionRequest deregisterTaskDefinitionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes one or more of your clusters.
*
*
* @param describeClustersRequest
* @return A Java Future containing the result of the DescribeClusters
* operation returned by the service.
* @sample AmazonECSAsync.DescribeClusters
*/
java.util.concurrent.Future describeClustersAsync(
DescribeClustersRequest describeClustersRequest);
/**
*
* Describes one or more of your clusters.
*
*
* @param describeClustersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeClusters
* operation returned by the service.
* @sample AmazonECSAsyncHandler.DescribeClusters
*/
java.util.concurrent.Future describeClustersAsync(
DescribeClustersRequest describeClustersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeClusters operation.
*
* @see #describeClustersAsync(DescribeClustersRequest)
*/
java.util.concurrent.Future describeClustersAsync();
/**
* Simplified method form for invoking the DescribeClusters operation with
* an AsyncHandler.
*
* @see #describeClustersAsync(DescribeClustersRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeClustersAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes Amazon EC2 Container Service container instances. Returns
* metadata about registered and remaining resources on each container
* instance requested.
*
*
* @param describeContainerInstancesRequest
* @return A Java Future containing the result of the
* DescribeContainerInstances operation returned by the service.
* @sample AmazonECSAsync.DescribeContainerInstances
*/
java.util.concurrent.Future describeContainerInstancesAsync(
DescribeContainerInstancesRequest describeContainerInstancesRequest);
/**
*
* Describes Amazon EC2 Container Service container instances. Returns
* metadata about registered and remaining resources on each container
* instance requested.
*
*
* @param describeContainerInstancesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* DescribeContainerInstances operation returned by the service.
* @sample AmazonECSAsyncHandler.DescribeContainerInstances
*/
java.util.concurrent.Future describeContainerInstancesAsync(
DescribeContainerInstancesRequest describeContainerInstancesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes the specified services running in your cluster.
*
*
* @param describeServicesRequest
* @return A Java Future containing the result of the DescribeServices
* operation returned by the service.
* @sample AmazonECSAsync.DescribeServices
*/
java.util.concurrent.Future describeServicesAsync(
DescribeServicesRequest describeServicesRequest);
/**
*
* Describes the specified services running in your cluster.
*
*
* @param describeServicesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeServices
* operation returned by the service.
* @sample AmazonECSAsyncHandler.DescribeServices
*/
java.util.concurrent.Future describeServicesAsync(
DescribeServicesRequest describeServicesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a task definition. You can specify a family
and
* revision
to find information about a specific task
* definition, or you can simply specify the family to find the latest
* ACTIVE
revision in that family.
*
*
*
* You can only describe INACTIVE
task definitions while an
* active task or service references them.
*
*
*
* @param describeTaskDefinitionRequest
* @return A Java Future containing the result of the DescribeTaskDefinition
* operation returned by the service.
* @sample AmazonECSAsync.DescribeTaskDefinition
*/
java.util.concurrent.Future describeTaskDefinitionAsync(
DescribeTaskDefinitionRequest describeTaskDefinitionRequest);
/**
*
* Describes a task definition. You can specify a family
and
* revision
to find information about a specific task
* definition, or you can simply specify the family to find the latest
* ACTIVE
revision in that family.
*
*
*
* You can only describe INACTIVE
task definitions while an
* active task or service references them.
*
*
*
* @param describeTaskDefinitionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeTaskDefinition
* operation returned by the service.
* @sample AmazonECSAsyncHandler.DescribeTaskDefinition
*/
java.util.concurrent.Future describeTaskDefinitionAsync(
DescribeTaskDefinitionRequest describeTaskDefinitionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a specified task or tasks.
*
*
* @param describeTasksRequest
* @return A Java Future containing the result of the DescribeTasks
* operation returned by the service.
* @sample AmazonECSAsync.DescribeTasks
*/
java.util.concurrent.Future describeTasksAsync(
DescribeTasksRequest describeTasksRequest);
/**
*
* Describes a specified task or tasks.
*
*
* @param describeTasksRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeTasks
* operation returned by the service.
* @sample AmazonECSAsyncHandler.DescribeTasks
*/
java.util.concurrent.Future describeTasksAsync(
DescribeTasksRequest describeTasksRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
*
* This action is only used by the Amazon EC2 Container Service agent, and
* it is not intended for use outside of the agent.
*
*
*
* Returns an endpoint for the Amazon EC2 Container Service agent to poll
* for updates.
*
*
* @param discoverPollEndpointRequest
* @return A Java Future containing the result of the DiscoverPollEndpoint
* operation returned by the service.
* @sample AmazonECSAsync.DiscoverPollEndpoint
*/
java.util.concurrent.Future discoverPollEndpointAsync(
DiscoverPollEndpointRequest discoverPollEndpointRequest);
/**
*
*
* This action is only used by the Amazon EC2 Container Service agent, and
* it is not intended for use outside of the agent.
*
*
*
* Returns an endpoint for the Amazon EC2 Container Service agent to poll
* for updates.
*
*
* @param discoverPollEndpointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DiscoverPollEndpoint
* operation returned by the service.
* @sample AmazonECSAsyncHandler.DiscoverPollEndpoint
*/
java.util.concurrent.Future discoverPollEndpointAsync(
DiscoverPollEndpointRequest discoverPollEndpointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DiscoverPollEndpoint operation.
*
* @see #discoverPollEndpointAsync(DiscoverPollEndpointRequest)
*/
java.util.concurrent.Future discoverPollEndpointAsync();
/**
* Simplified method form for invoking the DiscoverPollEndpoint operation
* with an AsyncHandler.
*
* @see #discoverPollEndpointAsync(DiscoverPollEndpointRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future discoverPollEndpointAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of existing clusters.
*
*
* @param listClustersRequest
* @return A Java Future containing the result of the ListClusters operation
* returned by the service.
* @sample AmazonECSAsync.ListClusters
*/
java.util.concurrent.Future listClustersAsync(
ListClustersRequest listClustersRequest);
/**
*
* Returns a list of existing clusters.
*
*
* @param listClustersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListClusters operation
* returned by the service.
* @sample AmazonECSAsyncHandler.ListClusters
*/
java.util.concurrent.Future listClustersAsync(
ListClustersRequest listClustersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the ListClusters operation.
*
* @see #listClustersAsync(ListClustersRequest)
*/
java.util.concurrent.Future listClustersAsync();
/**
* Simplified method form for invoking the ListClusters operation with an
* AsyncHandler.
*
* @see #listClustersAsync(ListClustersRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future listClustersAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of container instances in a specified cluster.
*
*
* @param listContainerInstancesRequest
* @return A Java Future containing the result of the ListContainerInstances
* operation returned by the service.
* @sample AmazonECSAsync.ListContainerInstances
*/
java.util.concurrent.Future listContainerInstancesAsync(
ListContainerInstancesRequest listContainerInstancesRequest);
/**
*
* Returns a list of container instances in a specified cluster.
*
*
* @param listContainerInstancesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListContainerInstances
* operation returned by the service.
* @sample AmazonECSAsyncHandler.ListContainerInstances
*/
java.util.concurrent.Future listContainerInstancesAsync(
ListContainerInstancesRequest listContainerInstancesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the ListContainerInstances operation.
*
* @see #listContainerInstancesAsync(ListContainerInstancesRequest)
*/
java.util.concurrent.Future listContainerInstancesAsync();
/**
* Simplified method form for invoking the ListContainerInstances operation
* with an AsyncHandler.
*
* @see #listContainerInstancesAsync(ListContainerInstancesRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future listContainerInstancesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the services that are running in a specified cluster.
*
*
* @param listServicesRequest
* @return A Java Future containing the result of the ListServices operation
* returned by the service.
* @sample AmazonECSAsync.ListServices
*/
java.util.concurrent.Future listServicesAsync(
ListServicesRequest listServicesRequest);
/**
*
* Lists the services that are running in a specified cluster.
*
*
* @param listServicesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListServices operation
* returned by the service.
* @sample AmazonECSAsyncHandler.ListServices
*/
java.util.concurrent.Future listServicesAsync(
ListServicesRequest listServicesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the ListServices operation.
*
* @see #listServicesAsync(ListServicesRequest)
*/
java.util.concurrent.Future listServicesAsync();
/**
* Simplified method form for invoking the ListServices operation with an
* AsyncHandler.
*
* @see #listServicesAsync(ListServicesRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future listServicesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of task definition families that are registered to your
* account (which may include task definition families that no longer have
* any ACTIVE
task definitions). You can filter the results
* with the familyPrefix
parameter.
*
*
* @param listTaskDefinitionFamiliesRequest
* @return A Java Future containing the result of the
* ListTaskDefinitionFamilies operation returned by the service.
* @sample AmazonECSAsync.ListTaskDefinitionFamilies
*/
java.util.concurrent.Future listTaskDefinitionFamiliesAsync(
ListTaskDefinitionFamiliesRequest listTaskDefinitionFamiliesRequest);
/**
*
* Returns a list of task definition families that are registered to your
* account (which may include task definition families that no longer have
* any ACTIVE
task definitions). You can filter the results
* with the familyPrefix
parameter.
*
*
* @param listTaskDefinitionFamiliesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* ListTaskDefinitionFamilies operation returned by the service.
* @sample AmazonECSAsyncHandler.ListTaskDefinitionFamilies
*/
java.util.concurrent.Future listTaskDefinitionFamiliesAsync(
ListTaskDefinitionFamiliesRequest listTaskDefinitionFamiliesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the ListTaskDefinitionFamilies
* operation.
*
* @see #listTaskDefinitionFamiliesAsync(ListTaskDefinitionFamiliesRequest)
*/
java.util.concurrent.Future listTaskDefinitionFamiliesAsync();
/**
* Simplified method form for invoking the ListTaskDefinitionFamilies
* operation with an AsyncHandler.
*
* @see #listTaskDefinitionFamiliesAsync(ListTaskDefinitionFamiliesRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future listTaskDefinitionFamiliesAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of task definitions that are registered to your account.
* You can filter the results by family name with the
* familyPrefix
parameter or by status with the
* status
parameter.
*
*
* @param listTaskDefinitionsRequest
* @return A Java Future containing the result of the ListTaskDefinitions
* operation returned by the service.
* @sample AmazonECSAsync.ListTaskDefinitions
*/
java.util.concurrent.Future listTaskDefinitionsAsync(
ListTaskDefinitionsRequest listTaskDefinitionsRequest);
/**
*
* Returns a list of task definitions that are registered to your account.
* You can filter the results by family name with the
* familyPrefix
parameter or by status with the
* status
parameter.
*
*
* @param listTaskDefinitionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTaskDefinitions
* operation returned by the service.
* @sample AmazonECSAsyncHandler.ListTaskDefinitions
*/
java.util.concurrent.Future listTaskDefinitionsAsync(
ListTaskDefinitionsRequest listTaskDefinitionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the ListTaskDefinitions operation.
*
* @see #listTaskDefinitionsAsync(ListTaskDefinitionsRequest)
*/
java.util.concurrent.Future listTaskDefinitionsAsync();
/**
* Simplified method form for invoking the ListTaskDefinitions operation
* with an AsyncHandler.
*
* @see #listTaskDefinitionsAsync(ListTaskDefinitionsRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future listTaskDefinitionsAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of tasks for a specified cluster. You can filter the
* results by family name, by a particular container instance, or by the
* desired status of the task with the family
,
* containerInstance
, and desiredStatus
* parameters.
*
*
* @param listTasksRequest
* @return A Java Future containing the result of the ListTasks operation
* returned by the service.
* @sample AmazonECSAsync.ListTasks
*/
java.util.concurrent.Future listTasksAsync(
ListTasksRequest listTasksRequest);
/**
*
* Returns a list of tasks for a specified cluster. You can filter the
* results by family name, by a particular container instance, or by the
* desired status of the task with the family
,
* containerInstance
, and desiredStatus
* parameters.
*
*
* @param listTasksRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTasks operation
* returned by the service.
* @sample AmazonECSAsyncHandler.ListTasks
*/
java.util.concurrent.Future listTasksAsync(
ListTasksRequest listTasksRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the ListTasks operation.
*
* @see #listTasksAsync(ListTasksRequest)
*/
java.util.concurrent.Future listTasksAsync();
/**
* Simplified method form for invoking the ListTasks operation with an
* AsyncHandler.
*
* @see #listTasksAsync(ListTasksRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future listTasksAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
*
* This action is only used by the Amazon EC2 Container Service agent, and
* it is not intended for use outside of the agent.
*
*
*
* Registers an EC2 instance into the specified cluster. This instance
* becomes available to place containers on.
*
*
* @param registerContainerInstanceRequest
* @return A Java Future containing the result of the
* RegisterContainerInstance operation returned by the service.
* @sample AmazonECSAsync.RegisterContainerInstance
*/
java.util.concurrent.Future registerContainerInstanceAsync(
RegisterContainerInstanceRequest registerContainerInstanceRequest);
/**
*
*
* This action is only used by the Amazon EC2 Container Service agent, and
* it is not intended for use outside of the agent.
*
*
*
* Registers an EC2 instance into the specified cluster. This instance
* becomes available to place containers on.
*
*
* @param registerContainerInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* RegisterContainerInstance operation returned by the service.
* @sample AmazonECSAsyncHandler.RegisterContainerInstance
*/
java.util.concurrent.Future registerContainerInstanceAsync(
RegisterContainerInstanceRequest registerContainerInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Registers a new task definition from the supplied family
and
* containerDefinitions
. Optionally, you can add data volumes
* to your containers with the volumes
parameter. For more
* information about task definition parameters and defaults, see Amazon ECS Task Definitions in the Amazon EC2 Container Service
* Developer Guide.
*
*
* @param registerTaskDefinitionRequest
* @return A Java Future containing the result of the RegisterTaskDefinition
* operation returned by the service.
* @sample AmazonECSAsync.RegisterTaskDefinition
*/
java.util.concurrent.Future registerTaskDefinitionAsync(
RegisterTaskDefinitionRequest registerTaskDefinitionRequest);
/**
*
* Registers a new task definition from the supplied family
and
* containerDefinitions
. Optionally, you can add data volumes
* to your containers with the volumes
parameter. For more
* information about task definition parameters and defaults, see Amazon ECS Task Definitions in the Amazon EC2 Container Service
* Developer Guide.
*
*
* @param registerTaskDefinitionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RegisterTaskDefinition
* operation returned by the service.
* @sample AmazonECSAsyncHandler.RegisterTaskDefinition
*/
java.util.concurrent.Future registerTaskDefinitionAsync(
RegisterTaskDefinitionRequest registerTaskDefinitionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Start a task using random placement and the default Amazon ECS scheduler.
* To use your own scheduler or place a task on a specific container
* instance, use StartTask
instead.
*
*
*
* The count
parameter is limited to 10 tasks per call.
*
*
*
* @param runTaskRequest
* @return A Java Future containing the result of the RunTask operation
* returned by the service.
* @sample AmazonECSAsync.RunTask
*/
java.util.concurrent.Future runTaskAsync(
RunTaskRequest runTaskRequest);
/**
*
* Start a task using random placement and the default Amazon ECS scheduler.
* To use your own scheduler or place a task on a specific container
* instance, use StartTask
instead.
*
*
*
* The count
parameter is limited to 10 tasks per call.
*
*
*
* @param runTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RunTask operation
* returned by the service.
* @sample AmazonECSAsyncHandler.RunTask
*/
java.util.concurrent.Future runTaskAsync(
RunTaskRequest runTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts a new task from the specified task definition on the specified
* container instance or instances. To use the default Amazon ECS scheduler
* to place your task, use RunTask
instead.
*
*
*
* The list of container instances to start tasks on is limited to 10.
*
*
*
* @param startTaskRequest
* @return A Java Future containing the result of the StartTask operation
* returned by the service.
* @sample AmazonECSAsync.StartTask
*/
java.util.concurrent.Future startTaskAsync(
StartTaskRequest startTaskRequest);
/**
*
* Starts a new task from the specified task definition on the specified
* container instance or instances. To use the default Amazon ECS scheduler
* to place your task, use RunTask
instead.
*
*
*
* The list of container instances to start tasks on is limited to 10.
*
*
*
* @param startTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartTask operation
* returned by the service.
* @sample AmazonECSAsyncHandler.StartTask
*/
java.util.concurrent.Future startTaskAsync(
StartTaskRequest startTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Stops a running task.
*
*
* When StopTask is called on a task, the equivalent of
* docker stop
is issued to the containers running in the task.
* This results in a SIGTERM
and a 30-second timeout, after
* which SIGKILL
is sent and the containers are forcibly
* stopped. If the container handles the SIGTERM
gracefully and
* exits within 30 seconds from receiving it, no SIGKILL
is
* sent.
*
*
* @param stopTaskRequest
* @return A Java Future containing the result of the StopTask operation
* returned by the service.
* @sample AmazonECSAsync.StopTask
*/
java.util.concurrent.Future stopTaskAsync(
StopTaskRequest stopTaskRequest);
/**
*
* Stops a running task.
*
*
* When StopTask is called on a task, the equivalent of
* docker stop
is issued to the containers running in the task.
* This results in a SIGTERM
and a 30-second timeout, after
* which SIGKILL
is sent and the containers are forcibly
* stopped. If the container handles the SIGTERM
gracefully and
* exits within 30 seconds from receiving it, no SIGKILL
is
* sent.
*
*
* @param stopTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StopTask operation
* returned by the service.
* @sample AmazonECSAsyncHandler.StopTask
*/
java.util.concurrent.Future stopTaskAsync(
StopTaskRequest stopTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
*
* This action is only used by the Amazon EC2 Container Service agent, and
* it is not intended for use outside of the agent.
*
*
*
* Sent to acknowledge that a container changed states.
*
*
* @param submitContainerStateChangeRequest
* @return A Java Future containing the result of the
* SubmitContainerStateChange operation returned by the service.
* @sample AmazonECSAsync.SubmitContainerStateChange
*/
java.util.concurrent.Future submitContainerStateChangeAsync(
SubmitContainerStateChangeRequest submitContainerStateChangeRequest);
/**
*
*
* This action is only used by the Amazon EC2 Container Service agent, and
* it is not intended for use outside of the agent.
*
*
*
* Sent to acknowledge that a container changed states.
*
*
* @param submitContainerStateChangeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the
* SubmitContainerStateChange operation returned by the service.
* @sample AmazonECSAsyncHandler.SubmitContainerStateChange
*/
java.util.concurrent.Future submitContainerStateChangeAsync(
SubmitContainerStateChangeRequest submitContainerStateChangeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the SubmitContainerStateChange
* operation.
*
* @see #submitContainerStateChangeAsync(SubmitContainerStateChangeRequest)
*/
java.util.concurrent.Future submitContainerStateChangeAsync();
/**
* Simplified method form for invoking the SubmitContainerStateChange
* operation with an AsyncHandler.
*
* @see #submitContainerStateChangeAsync(SubmitContainerStateChangeRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future submitContainerStateChangeAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
*
* This action is only used by the Amazon EC2 Container Service agent, and
* it is not intended for use outside of the agent.
*
*
*
* Sent to acknowledge that a task changed states.
*
*
* @param submitTaskStateChangeRequest
* @return A Java Future containing the result of the SubmitTaskStateChange
* operation returned by the service.
* @sample AmazonECSAsync.SubmitTaskStateChange
*/
java.util.concurrent.Future submitTaskStateChangeAsync(
SubmitTaskStateChangeRequest submitTaskStateChangeRequest);
/**
*
*
* This action is only used by the Amazon EC2 Container Service agent, and
* it is not intended for use outside of the agent.
*
*
*
* Sent to acknowledge that a task changed states.
*
*
* @param submitTaskStateChangeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SubmitTaskStateChange
* operation returned by the service.
* @sample AmazonECSAsyncHandler.SubmitTaskStateChange
*/
java.util.concurrent.Future submitTaskStateChangeAsync(
SubmitTaskStateChangeRequest submitTaskStateChangeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the Amazon ECS container agent on a specified container instance.
* Updating the Amazon ECS container agent does not interrupt running tasks
* or services on the container instance. The process for updating the agent
* differs depending on whether your container instance was launched with
* the Amazon ECS-optimized AMI or another operating system.
*
*
* UpdateContainerAgent
requires the Amazon ECS-optimized AMI
* or Amazon Linux with the ecs-init
service installed and
* running. For help updating the Amazon ECS container agent on other
* operating systems, see Manually Updating the Amazon ECS Container Agent in the Amazon
* EC2 Container Service Developer Guide.
*
*
* @param updateContainerAgentRequest
* @return A Java Future containing the result of the UpdateContainerAgent
* operation returned by the service.
* @sample AmazonECSAsync.UpdateContainerAgent
*/
java.util.concurrent.Future updateContainerAgentAsync(
UpdateContainerAgentRequest updateContainerAgentRequest);
/**
*
* Updates the Amazon ECS container agent on a specified container instance.
* Updating the Amazon ECS container agent does not interrupt running tasks
* or services on the container instance. The process for updating the agent
* differs depending on whether your container instance was launched with
* the Amazon ECS-optimized AMI or another operating system.
*
*
* UpdateContainerAgent
requires the Amazon ECS-optimized AMI
* or Amazon Linux with the ecs-init
service installed and
* running. For help updating the Amazon ECS container agent on other
* operating systems, see Manually Updating the Amazon ECS Container Agent in the Amazon
* EC2 Container Service Developer Guide.
*
*
* @param updateContainerAgentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateContainerAgent
* operation returned by the service.
* @sample AmazonECSAsyncHandler.UpdateContainerAgent
*/
java.util.concurrent.Future updateContainerAgentAsync(
UpdateContainerAgentRequest updateContainerAgentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Modifies the desired count, deployment configuration, or task definition
* used in a service.
*
*
* You can add to or subtract from the number of instantiations of a task
* definition in a service by specifying the cluster that the service is
* running in and a new desiredCount
parameter.
*
*
* You can use UpdateService to modify your task definition and
* deploy a new version of your service.
*
*
* You can also update the deployment configuration of a service. When a
* deployment is triggered by updating the task definition of a service, the
* service scheduler uses the deployment configuration parameters,
* minimumHealthyPercent
and maximumPercent
, to
* determine the deployment strategy.
*
*
* If the minimumHealthyPercent
is below 100%, the scheduler
* can ignore the desiredCount
temporarily during a deployment.
* For example, if your service has a desiredCount
of four
* tasks, a minimumHealthyPercent
of 50% allows the scheduler
* to stop two existing tasks before starting two new tasks. Tasks for
* services that do not use a load balancer are considered healthy if
* they are in the RUNNING
state; tasks for services that
* do use a load balancer are considered healthy if they are in the
* RUNNING
state and the container instance it is hosted on is
* reported as healthy by the load balancer.
*
*
* The maximumPercent
parameter represents an upper limit on
* the number of running tasks during a deployment, which enables you to
* define the deployment batch size. For example, if your service has a
* desiredCount
of four tasks, a maximumPercent
* value of 200% starts four new tasks before stopping the four older tasks
* (provided that the cluster resources required to do this are available).
*
*
* When UpdateService stops a task during a deployment, the
* equivalent of docker stop
is issued to the containers
* running in the task. This results in a SIGTERM
and a
* 30-second timeout, after which SIGKILL
is sent and the
* containers are forcibly stopped. If the container handles the
* SIGTERM
gracefully and exits within 30 seconds from
* receiving it, no SIGKILL
is sent.
*
*
* When the service scheduler launches new tasks, it attempts to balance
* them across the Availability Zones in your cluster with the following
* logic:
*
*
* -
*
* Determine which of the container instances in your cluster can support
* your service's task definition (for example, they have the required CPU,
* memory, ports, and container instance attributes).
*
*
* -
*
* Sort the valid container instances by the fewest number of running tasks
* for this service in the same Availability Zone as the instance. For
* example, if zone A has one running service task and zones B and C each
* have zero, valid container instances in either zone B or C are considered
* optimal for placement.
*
*
* -
*
* Place the new service task on a valid container instance in an optimal
* Availability Zone (based on the previous steps), favoring container
* instances with the fewest number of running tasks for this service.
*
*
*
*
* @param updateServiceRequest
* @return A Java Future containing the result of the UpdateService
* operation returned by the service.
* @sample AmazonECSAsync.UpdateService
*/
java.util.concurrent.Future updateServiceAsync(
UpdateServiceRequest updateServiceRequest);
/**
*
* Modifies the desired count, deployment configuration, or task definition
* used in a service.
*
*
* You can add to or subtract from the number of instantiations of a task
* definition in a service by specifying the cluster that the service is
* running in and a new desiredCount
parameter.
*
*
* You can use UpdateService to modify your task definition and
* deploy a new version of your service.
*
*
* You can also update the deployment configuration of a service. When a
* deployment is triggered by updating the task definition of a service, the
* service scheduler uses the deployment configuration parameters,
* minimumHealthyPercent
and maximumPercent
, to
* determine the deployment strategy.
*
*
* If the minimumHealthyPercent
is below 100%, the scheduler
* can ignore the desiredCount
temporarily during a deployment.
* For example, if your service has a desiredCount
of four
* tasks, a minimumHealthyPercent
of 50% allows the scheduler
* to stop two existing tasks before starting two new tasks. Tasks for
* services that do not use a load balancer are considered healthy if
* they are in the RUNNING
state; tasks for services that
* do use a load balancer are considered healthy if they are in the
* RUNNING
state and the container instance it is hosted on is
* reported as healthy by the load balancer.
*
*
* The maximumPercent
parameter represents an upper limit on
* the number of running tasks during a deployment, which enables you to
* define the deployment batch size. For example, if your service has a
* desiredCount
of four tasks, a maximumPercent
* value of 200% starts four new tasks before stopping the four older tasks
* (provided that the cluster resources required to do this are available).
*
*
* When UpdateService stops a task during a deployment, the
* equivalent of docker stop
is issued to the containers
* running in the task. This results in a SIGTERM
and a
* 30-second timeout, after which SIGKILL
is sent and the
* containers are forcibly stopped. If the container handles the
* SIGTERM
gracefully and exits within 30 seconds from
* receiving it, no SIGKILL
is sent.
*
*
* When the service scheduler launches new tasks, it attempts to balance
* them across the Availability Zones in your cluster with the following
* logic:
*
*
* -
*
* Determine which of the container instances in your cluster can support
* your service's task definition (for example, they have the required CPU,
* memory, ports, and container instance attributes).
*
*
* -
*
* Sort the valid container instances by the fewest number of running tasks
* for this service in the same Availability Zone as the instance. For
* example, if zone A has one running service task and zones B and C each
* have zero, valid container instances in either zone B or C are considered
* optimal for placement.
*
*
* -
*
* Place the new service task on a valid container instance in an optimal
* Availability Zone (based on the previous steps), favoring container
* instances with the fewest number of running tasks for this service.
*
*
*
*
* @param updateServiceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateService
* operation returned by the service.
* @sample AmazonECSAsyncHandler.UpdateService
*/
java.util.concurrent.Future updateServiceAsync(
UpdateServiceRequest updateServiceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}