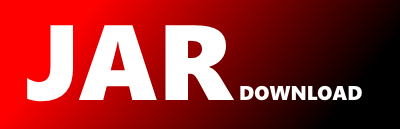
com.amazonaws.services.ecs.model.Resource Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ecs Show documentation
/*
* Copyright 2010-2016 Amazon.com, Inc. or its affiliates. All Rights
* Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.ecs.model;
import java.io.Serializable;
/**
*
* Describes the resources available for a container instance.
*
*/
public class Resource implements Serializable, Cloneable {
/**
*
* The name of the resource, such as CPU
, MEMORY
,
* PORTS
, or a user-defined resource.
*
*/
private String name;
/**
*
* The type of the resource, such as INTEGER
,
* DOUBLE
, LONG
, or STRINGSET
.
*
*/
private String type;
/**
*
* When the doubleValue
type is set, the value of the resource
* must be a double precision floating-point type.
*
*/
private Double doubleValue;
/**
*
* When the longValue
type is set, the value of the resource
* must be an extended precision floating-point type.
*
*/
private Long longValue;
/**
*
* When the integerValue
type is set, the value of the resource
* must be an integer.
*
*/
private Integer integerValue;
/**
*
* When the stringSetValue
type is set, the value of the
* resource must be a string type.
*
*/
private com.amazonaws.internal.SdkInternalList stringSetValue;
/**
*
* The name of the resource, such as CPU
, MEMORY
,
* PORTS
, or a user-defined resource.
*
*
* @param name
* The name of the resource, such as CPU
,
* MEMORY
, PORTS
, or a user-defined
* resource.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the resource, such as CPU
, MEMORY
,
* PORTS
, or a user-defined resource.
*
*
* @return The name of the resource, such as CPU
,
* MEMORY
, PORTS
, or a user-defined
* resource.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the resource, such as CPU
, MEMORY
,
* PORTS
, or a user-defined resource.
*
*
* @param name
* The name of the resource, such as CPU
,
* MEMORY
, PORTS
, or a user-defined
* resource.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public Resource withName(String name) {
setName(name);
return this;
}
/**
*
* The type of the resource, such as INTEGER
,
* DOUBLE
, LONG
, or STRINGSET
.
*
*
* @param type
* The type of the resource, such as INTEGER
,
* DOUBLE
, LONG
, or STRINGSET
.
*/
public void setType(String type) {
this.type = type;
}
/**
*
* The type of the resource, such as INTEGER
,
* DOUBLE
, LONG
, or STRINGSET
.
*
*
* @return The type of the resource, such as INTEGER
,
* DOUBLE
, LONG
, or STRINGSET
* .
*/
public String getType() {
return this.type;
}
/**
*
* The type of the resource, such as INTEGER
,
* DOUBLE
, LONG
, or STRINGSET
.
*
*
* @param type
* The type of the resource, such as INTEGER
,
* DOUBLE
, LONG
, or STRINGSET
.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public Resource withType(String type) {
setType(type);
return this;
}
/**
*
* When the doubleValue
type is set, the value of the resource
* must be a double precision floating-point type.
*
*
* @param doubleValue
* When the doubleValue
type is set, the value of the
* resource must be a double precision floating-point type.
*/
public void setDoubleValue(Double doubleValue) {
this.doubleValue = doubleValue;
}
/**
*
* When the doubleValue
type is set, the value of the resource
* must be a double precision floating-point type.
*
*
* @return When the doubleValue
type is set, the value of the
* resource must be a double precision floating-point type.
*/
public Double getDoubleValue() {
return this.doubleValue;
}
/**
*
* When the doubleValue
type is set, the value of the resource
* must be a double precision floating-point type.
*
*
* @param doubleValue
* When the doubleValue
type is set, the value of the
* resource must be a double precision floating-point type.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public Resource withDoubleValue(Double doubleValue) {
setDoubleValue(doubleValue);
return this;
}
/**
*
* When the longValue
type is set, the value of the resource
* must be an extended precision floating-point type.
*
*
* @param longValue
* When the longValue
type is set, the value of the
* resource must be an extended precision floating-point type.
*/
public void setLongValue(Long longValue) {
this.longValue = longValue;
}
/**
*
* When the longValue
type is set, the value of the resource
* must be an extended precision floating-point type.
*
*
* @return When the longValue
type is set, the value of the
* resource must be an extended precision floating-point type.
*/
public Long getLongValue() {
return this.longValue;
}
/**
*
* When the longValue
type is set, the value of the resource
* must be an extended precision floating-point type.
*
*
* @param longValue
* When the longValue
type is set, the value of the
* resource must be an extended precision floating-point type.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public Resource withLongValue(Long longValue) {
setLongValue(longValue);
return this;
}
/**
*
* When the integerValue
type is set, the value of the resource
* must be an integer.
*
*
* @param integerValue
* When the integerValue
type is set, the value of the
* resource must be an integer.
*/
public void setIntegerValue(Integer integerValue) {
this.integerValue = integerValue;
}
/**
*
* When the integerValue
type is set, the value of the resource
* must be an integer.
*
*
* @return When the integerValue
type is set, the value of the
* resource must be an integer.
*/
public Integer getIntegerValue() {
return this.integerValue;
}
/**
*
* When the integerValue
type is set, the value of the resource
* must be an integer.
*
*
* @param integerValue
* When the integerValue
type is set, the value of the
* resource must be an integer.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public Resource withIntegerValue(Integer integerValue) {
setIntegerValue(integerValue);
return this;
}
/**
*
* When the stringSetValue
type is set, the value of the
* resource must be a string type.
*
*
* @return When the stringSetValue
type is set, the value of
* the resource must be a string type.
*/
public java.util.List getStringSetValue() {
if (stringSetValue == null) {
stringSetValue = new com.amazonaws.internal.SdkInternalList();
}
return stringSetValue;
}
/**
*
* When the stringSetValue
type is set, the value of the
* resource must be a string type.
*
*
* @param stringSetValue
* When the stringSetValue
type is set, the value of the
* resource must be a string type.
*/
public void setStringSetValue(java.util.Collection stringSetValue) {
if (stringSetValue == null) {
this.stringSetValue = null;
return;
}
this.stringSetValue = new com.amazonaws.internal.SdkInternalList(
stringSetValue);
}
/**
*
* When the stringSetValue
type is set, the value of the
* resource must be a string type.
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setStringSetValue(java.util.Collection)} or
* {@link #withStringSetValue(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param stringSetValue
* When the stringSetValue
type is set, the value of the
* resource must be a string type.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public Resource withStringSetValue(String... stringSetValue) {
if (this.stringSetValue == null) {
setStringSetValue(new com.amazonaws.internal.SdkInternalList(
stringSetValue.length));
}
for (String ele : stringSetValue) {
this.stringSetValue.add(ele);
}
return this;
}
/**
*
* When the stringSetValue
type is set, the value of the
* resource must be a string type.
*
*
* @param stringSetValue
* When the stringSetValue
type is set, the value of the
* resource must be a string type.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public Resource withStringSetValue(
java.util.Collection stringSetValue) {
setStringSetValue(stringSetValue);
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: " + getName() + ",");
if (getType() != null)
sb.append("Type: " + getType() + ",");
if (getDoubleValue() != null)
sb.append("DoubleValue: " + getDoubleValue() + ",");
if (getLongValue() != null)
sb.append("LongValue: " + getLongValue() + ",");
if (getIntegerValue() != null)
sb.append("IntegerValue: " + getIntegerValue() + ",");
if (getStringSetValue() != null)
sb.append("StringSetValue: " + getStringSetValue());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Resource == false)
return false;
Resource other = (Resource) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null
&& other.getName().equals(this.getName()) == false)
return false;
if (other.getType() == null ^ this.getType() == null)
return false;
if (other.getType() != null
&& other.getType().equals(this.getType()) == false)
return false;
if (other.getDoubleValue() == null ^ this.getDoubleValue() == null)
return false;
if (other.getDoubleValue() != null
&& other.getDoubleValue().equals(this.getDoubleValue()) == false)
return false;
if (other.getLongValue() == null ^ this.getLongValue() == null)
return false;
if (other.getLongValue() != null
&& other.getLongValue().equals(this.getLongValue()) == false)
return false;
if (other.getIntegerValue() == null ^ this.getIntegerValue() == null)
return false;
if (other.getIntegerValue() != null
&& other.getIntegerValue().equals(this.getIntegerValue()) == false)
return false;
if (other.getStringSetValue() == null
^ this.getStringSetValue() == null)
return false;
if (other.getStringSetValue() != null
&& other.getStringSetValue().equals(this.getStringSetValue()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode
+ ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode
+ ((getType() == null) ? 0 : getType().hashCode());
hashCode = prime
* hashCode
+ ((getDoubleValue() == null) ? 0 : getDoubleValue().hashCode());
hashCode = prime * hashCode
+ ((getLongValue() == null) ? 0 : getLongValue().hashCode());
hashCode = prime
* hashCode
+ ((getIntegerValue() == null) ? 0 : getIntegerValue()
.hashCode());
hashCode = prime
* hashCode
+ ((getStringSetValue() == null) ? 0 : getStringSetValue()
.hashCode());
return hashCode;
}
@Override
public Resource clone() {
try {
return (Resource) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException(
"Got a CloneNotSupportedException from Object.clone() "
+ "even though we're Cloneable!", e);
}
}
}