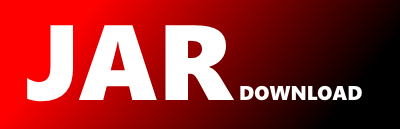
com.amazonaws.services.ecs.model.StartTaskRequest Maven / Gradle / Ivy
/*
* Copyright 2010-2016 Amazon.com, Inc. or its affiliates. All Rights
* Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.ecs.model;
import java.io.Serializable;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
*/
public class StartTaskRequest extends AmazonWebServiceRequest implements
Serializable, Cloneable {
/**
*
* The short name or full Amazon Resource Name (ARN) of the cluster on which
* to start your task. If you do not specify a cluster, the default cluster
* is assumed..
*
*/
private String cluster;
/**
*
* The family
and revision
(
* family:revision
) or full Amazon Resource Name (ARN) of the
* task definition to start. If a revision
is not specified,
* the latest ACTIVE
revision is used.
*
*/
private String taskDefinition;
/**
*
* A list of container overrides in JSON format that specify the name of a
* container in the specified task definition and the overrides it should
* receive. You can override the default command for a container (that is
* specified in the task definition or Docker image) with a
* command
override. You can also override existing environment
* variables (that are specified in the task definition or Docker image) on
* a container or add new environment variables to it with an
* environment
override.
*
*
*
* A total of 8192 characters are allowed for overrides. This limit includes
* the JSON formatting characters of the override structure.
*
*
*/
private TaskOverride overrides;
/**
*
* The container instance IDs or full Amazon Resource Name (ARN) entries for
* the container instances on which you would like to place your task.
*
*
*
* The list of container instances to start tasks on is limited to 10.
*
*
*/
private com.amazonaws.internal.SdkInternalList containerInstances;
/**
*
* An optional tag specified when a task is started. For example if you
* automatically trigger a task to run a batch process job, you could apply
* a unique identifier for that job to your task with the
* startedBy
parameter. You can then identify which tasks
* belong to that job by filtering the results of a ListTasks call
* with the startedBy
value.
*
*
* If a task is started by an Amazon ECS service, then the
* startedBy
parameter contains the deployment ID of the
* service that starts it.
*
*/
private String startedBy;
/**
*
* The short name or full Amazon Resource Name (ARN) of the cluster on which
* to start your task. If you do not specify a cluster, the default cluster
* is assumed..
*
*
* @param cluster
* The short name or full Amazon Resource Name (ARN) of the cluster
* on which to start your task. If you do not specify a cluster, the
* default cluster is assumed..
*/
public void setCluster(String cluster) {
this.cluster = cluster;
}
/**
*
* The short name or full Amazon Resource Name (ARN) of the cluster on which
* to start your task. If you do not specify a cluster, the default cluster
* is assumed..
*
*
* @return The short name or full Amazon Resource Name (ARN) of the cluster
* on which to start your task. If you do not specify a cluster, the
* default cluster is assumed..
*/
public String getCluster() {
return this.cluster;
}
/**
*
* The short name or full Amazon Resource Name (ARN) of the cluster on which
* to start your task. If you do not specify a cluster, the default cluster
* is assumed..
*
*
* @param cluster
* The short name or full Amazon Resource Name (ARN) of the cluster
* on which to start your task. If you do not specify a cluster, the
* default cluster is assumed..
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public StartTaskRequest withCluster(String cluster) {
setCluster(cluster);
return this;
}
/**
*
* The family
and revision
(
* family:revision
) or full Amazon Resource Name (ARN) of the
* task definition to start. If a revision
is not specified,
* the latest ACTIVE
revision is used.
*
*
* @param taskDefinition
* The family
and revision
(
* family:revision
) or full Amazon Resource Name (ARN)
* of the task definition to start. If a revision
is not
* specified, the latest ACTIVE
revision is used.
*/
public void setTaskDefinition(String taskDefinition) {
this.taskDefinition = taskDefinition;
}
/**
*
* The family
and revision
(
* family:revision
) or full Amazon Resource Name (ARN) of the
* task definition to start. If a revision
is not specified,
* the latest ACTIVE
revision is used.
*
*
* @return The family
and revision
(
* family:revision
) or full Amazon Resource Name (ARN)
* of the task definition to start. If a revision
is
* not specified, the latest ACTIVE
revision is used.
*/
public String getTaskDefinition() {
return this.taskDefinition;
}
/**
*
* The family
and revision
(
* family:revision
) or full Amazon Resource Name (ARN) of the
* task definition to start. If a revision
is not specified,
* the latest ACTIVE
revision is used.
*
*
* @param taskDefinition
* The family
and revision
(
* family:revision
) or full Amazon Resource Name (ARN)
* of the task definition to start. If a revision
is not
* specified, the latest ACTIVE
revision is used.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public StartTaskRequest withTaskDefinition(String taskDefinition) {
setTaskDefinition(taskDefinition);
return this;
}
/**
*
* A list of container overrides in JSON format that specify the name of a
* container in the specified task definition and the overrides it should
* receive. You can override the default command for a container (that is
* specified in the task definition or Docker image) with a
* command
override. You can also override existing environment
* variables (that are specified in the task definition or Docker image) on
* a container or add new environment variables to it with an
* environment
override.
*
*
*
* A total of 8192 characters are allowed for overrides. This limit includes
* the JSON formatting characters of the override structure.
*
*
*
* @param overrides
* A list of container overrides in JSON format that specify the name
* of a container in the specified task definition and the overrides
* it should receive. You can override the default command for a
* container (that is specified in the task definition or Docker
* image) with a command
override. You can also override
* existing environment variables (that are specified in the task
* definition or Docker image) on a container or add new environment
* variables to it with an environment
override.
*
*
* A total of 8192 characters are allowed for overrides. This limit
* includes the JSON formatting characters of the override structure.
*
*/
public void setOverrides(TaskOverride overrides) {
this.overrides = overrides;
}
/**
*
* A list of container overrides in JSON format that specify the name of a
* container in the specified task definition and the overrides it should
* receive. You can override the default command for a container (that is
* specified in the task definition or Docker image) with a
* command
override. You can also override existing environment
* variables (that are specified in the task definition or Docker image) on
* a container or add new environment variables to it with an
* environment
override.
*
*
*
* A total of 8192 characters are allowed for overrides. This limit includes
* the JSON formatting characters of the override structure.
*
*
*
* @return A list of container overrides in JSON format that specify the
* name of a container in the specified task definition and the
* overrides it should receive. You can override the default command
* for a container (that is specified in the task definition or
* Docker image) with a command
override. You can also
* override existing environment variables (that are specified in
* the task definition or Docker image) on a container or add new
* environment variables to it with an environment
* override.
*
* A total of 8192 characters are allowed for overrides. This limit
* includes the JSON formatting characters of the override
* structure.
*
*/
public TaskOverride getOverrides() {
return this.overrides;
}
/**
*
* A list of container overrides in JSON format that specify the name of a
* container in the specified task definition and the overrides it should
* receive. You can override the default command for a container (that is
* specified in the task definition or Docker image) with a
* command
override. You can also override existing environment
* variables (that are specified in the task definition or Docker image) on
* a container or add new environment variables to it with an
* environment
override.
*
*
*
* A total of 8192 characters are allowed for overrides. This limit includes
* the JSON formatting characters of the override structure.
*
*
*
* @param overrides
* A list of container overrides in JSON format that specify the name
* of a container in the specified task definition and the overrides
* it should receive. You can override the default command for a
* container (that is specified in the task definition or Docker
* image) with a command
override. You can also override
* existing environment variables (that are specified in the task
* definition or Docker image) on a container or add new environment
* variables to it with an environment
override.
*
*
* A total of 8192 characters are allowed for overrides. This limit
* includes the JSON formatting characters of the override structure.
*
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public StartTaskRequest withOverrides(TaskOverride overrides) {
setOverrides(overrides);
return this;
}
/**
*
* The container instance IDs or full Amazon Resource Name (ARN) entries for
* the container instances on which you would like to place your task.
*
*
*
* The list of container instances to start tasks on is limited to 10.
*
*
*
* @return The container instance IDs or full Amazon Resource Name (ARN)
* entries for the container instances on which you would like to
* place your task.
*
* The list of container instances to start tasks on is limited to
* 10.
*
*/
public java.util.List getContainerInstances() {
if (containerInstances == null) {
containerInstances = new com.amazonaws.internal.SdkInternalList();
}
return containerInstances;
}
/**
*
* The container instance IDs or full Amazon Resource Name (ARN) entries for
* the container instances on which you would like to place your task.
*
*
*
* The list of container instances to start tasks on is limited to 10.
*
*
*
* @param containerInstances
* The container instance IDs or full Amazon Resource Name (ARN)
* entries for the container instances on which you would like to
* place your task.
*
* The list of container instances to start tasks on is limited to
* 10.
*
*/
public void setContainerInstances(
java.util.Collection containerInstances) {
if (containerInstances == null) {
this.containerInstances = null;
return;
}
this.containerInstances = new com.amazonaws.internal.SdkInternalList(
containerInstances);
}
/**
*
* The container instance IDs or full Amazon Resource Name (ARN) entries for
* the container instances on which you would like to place your task.
*
*
*
* The list of container instances to start tasks on is limited to 10.
*
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setContainerInstances(java.util.Collection)} or
* {@link #withContainerInstances(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param containerInstances
* The container instance IDs or full Amazon Resource Name (ARN)
* entries for the container instances on which you would like to
* place your task.
*
* The list of container instances to start tasks on is limited to
* 10.
*
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public StartTaskRequest withContainerInstances(String... containerInstances) {
if (this.containerInstances == null) {
setContainerInstances(new com.amazonaws.internal.SdkInternalList(
containerInstances.length));
}
for (String ele : containerInstances) {
this.containerInstances.add(ele);
}
return this;
}
/**
*
* The container instance IDs or full Amazon Resource Name (ARN) entries for
* the container instances on which you would like to place your task.
*
*
*
* The list of container instances to start tasks on is limited to 10.
*
*
*
* @param containerInstances
* The container instance IDs or full Amazon Resource Name (ARN)
* entries for the container instances on which you would like to
* place your task.
*
* The list of container instances to start tasks on is limited to
* 10.
*
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public StartTaskRequest withContainerInstances(
java.util.Collection containerInstances) {
setContainerInstances(containerInstances);
return this;
}
/**
*
* An optional tag specified when a task is started. For example if you
* automatically trigger a task to run a batch process job, you could apply
* a unique identifier for that job to your task with the
* startedBy
parameter. You can then identify which tasks
* belong to that job by filtering the results of a ListTasks call
* with the startedBy
value.
*
*
* If a task is started by an Amazon ECS service, then the
* startedBy
parameter contains the deployment ID of the
* service that starts it.
*
*
* @param startedBy
* An optional tag specified when a task is started. For example if
* you automatically trigger a task to run a batch process job, you
* could apply a unique identifier for that job to your task with the
* startedBy
parameter. You can then identify which
* tasks belong to that job by filtering the results of a
* ListTasks call with the startedBy
value.
*
* If a task is started by an Amazon ECS service, then the
* startedBy
parameter contains the deployment ID of the
* service that starts it.
*/
public void setStartedBy(String startedBy) {
this.startedBy = startedBy;
}
/**
*
* An optional tag specified when a task is started. For example if you
* automatically trigger a task to run a batch process job, you could apply
* a unique identifier for that job to your task with the
* startedBy
parameter. You can then identify which tasks
* belong to that job by filtering the results of a ListTasks call
* with the startedBy
value.
*
*
* If a task is started by an Amazon ECS service, then the
* startedBy
parameter contains the deployment ID of the
* service that starts it.
*
*
* @return An optional tag specified when a task is started. For example if
* you automatically trigger a task to run a batch process job, you
* could apply a unique identifier for that job to your task with
* the startedBy
parameter. You can then identify which
* tasks belong to that job by filtering the results of a
* ListTasks call with the startedBy
value.
*
* If a task is started by an Amazon ECS service, then the
* startedBy
parameter contains the deployment ID of
* the service that starts it.
*/
public String getStartedBy() {
return this.startedBy;
}
/**
*
* An optional tag specified when a task is started. For example if you
* automatically trigger a task to run a batch process job, you could apply
* a unique identifier for that job to your task with the
* startedBy
parameter. You can then identify which tasks
* belong to that job by filtering the results of a ListTasks call
* with the startedBy
value.
*
*
* If a task is started by an Amazon ECS service, then the
* startedBy
parameter contains the deployment ID of the
* service that starts it.
*
*
* @param startedBy
* An optional tag specified when a task is started. For example if
* you automatically trigger a task to run a batch process job, you
* could apply a unique identifier for that job to your task with the
* startedBy
parameter. You can then identify which
* tasks belong to that job by filtering the results of a
* ListTasks call with the startedBy
value.
*
* If a task is started by an Amazon ECS service, then the
* startedBy
parameter contains the deployment ID of the
* service that starts it.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public StartTaskRequest withStartedBy(String startedBy) {
setStartedBy(startedBy);
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCluster() != null)
sb.append("Cluster: " + getCluster() + ",");
if (getTaskDefinition() != null)
sb.append("TaskDefinition: " + getTaskDefinition() + ",");
if (getOverrides() != null)
sb.append("Overrides: " + getOverrides() + ",");
if (getContainerInstances() != null)
sb.append("ContainerInstances: " + getContainerInstances() + ",");
if (getStartedBy() != null)
sb.append("StartedBy: " + getStartedBy());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof StartTaskRequest == false)
return false;
StartTaskRequest other = (StartTaskRequest) obj;
if (other.getCluster() == null ^ this.getCluster() == null)
return false;
if (other.getCluster() != null
&& other.getCluster().equals(this.getCluster()) == false)
return false;
if (other.getTaskDefinition() == null
^ this.getTaskDefinition() == null)
return false;
if (other.getTaskDefinition() != null
&& other.getTaskDefinition().equals(this.getTaskDefinition()) == false)
return false;
if (other.getOverrides() == null ^ this.getOverrides() == null)
return false;
if (other.getOverrides() != null
&& other.getOverrides().equals(this.getOverrides()) == false)
return false;
if (other.getContainerInstances() == null
^ this.getContainerInstances() == null)
return false;
if (other.getContainerInstances() != null
&& other.getContainerInstances().equals(
this.getContainerInstances()) == false)
return false;
if (other.getStartedBy() == null ^ this.getStartedBy() == null)
return false;
if (other.getStartedBy() != null
&& other.getStartedBy().equals(this.getStartedBy()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode
+ ((getCluster() == null) ? 0 : getCluster().hashCode());
hashCode = prime
* hashCode
+ ((getTaskDefinition() == null) ? 0 : getTaskDefinition()
.hashCode());
hashCode = prime * hashCode
+ ((getOverrides() == null) ? 0 : getOverrides().hashCode());
hashCode = prime
* hashCode
+ ((getContainerInstances() == null) ? 0
: getContainerInstances().hashCode());
hashCode = prime * hashCode
+ ((getStartedBy() == null) ? 0 : getStartedBy().hashCode());
return hashCode;
}
@Override
public StartTaskRequest clone() {
return (StartTaskRequest) super.clone();
}
}