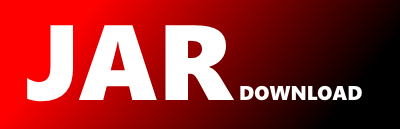
com.amazonaws.services.ecs.AmazonECS Maven / Gradle / Ivy
/*
* Copyright 2010-2016 Amazon.com, Inc. or its affiliates. All Rights
* Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.ecs;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.ecs.model.*;
/**
* Interface for accessing Amazon ECS.
*
*
* Amazon EC2 Container Service (Amazon ECS) is a highly scalable, fast,
* container management service that makes it easy to run, stop, and manage
* Docker containers on a cluster of EC2 instances. Amazon ECS lets you launch
* and stop container-enabled applications with simple API calls, allows you to
* get the state of your cluster from a centralized service, and gives you
* access to many familiar Amazon EC2 features like security groups, Amazon EBS
* volumes, and IAM roles.
*
*
* You can use Amazon ECS to schedule the placement of containers across your
* cluster based on your resource needs, isolation policies, and availability
* requirements. Amazon EC2 Container Service eliminates the need for you to
* operate your own cluster management and configuration management systems or
* worry about scaling your management infrastructure.
*
*/
public interface AmazonECS {
/**
* Overrides the default endpoint for this client
* ("https://ecs.us-east-1.amazonaws.com"). Callers can use this method to
* control which AWS region they want to work with.
*
* Callers can pass in just the endpoint (ex: "ecs.us-east-1.amazonaws.com")
* or a full URL, including the protocol (ex:
* "https://ecs.us-east-1.amazonaws.com"). If the protocol is not specified
* here, the default protocol from this client's {@link ClientConfiguration}
* will be used, which by default is HTTPS.
*
* For more information on using AWS regions with the AWS SDK for Java, and
* a complete list of all available endpoints for all AWS services, see: http://developer.amazonwebservices.com/connect/entry.jspa?externalID=
* 3912
*
* This method is not threadsafe. An endpoint should be configured when
* the client is created and before any service requests are made. Changing
* it afterwards creates inevitable race conditions for any service requests
* in transit or retrying.
*
* @param endpoint
* The endpoint (ex: "ecs.us-east-1.amazonaws.com") or a full URL,
* including the protocol (ex: "https://ecs.us-east-1.amazonaws.com")
* of the region specific AWS endpoint this client will communicate
* with.
*/
void setEndpoint(String endpoint);
/**
* An alternative to {@link AmazonECS#setEndpoint(String)}, sets the
* regional endpoint for this client's service calls. Callers can use this
* method to control which AWS region they want to work with.
*
* By default, all service endpoints in all regions use the https protocol.
* To use http instead, specify it in the {@link ClientConfiguration}
* supplied at construction.
*
* This method is not threadsafe. A region should be configured when the
* client is created and before any service requests are made. Changing it
* afterwards creates inevitable race conditions for any service requests in
* transit or retrying.
*
* @param region
* The region this client will communicate with. See
* {@link Region#getRegion(com.amazonaws.regions.Regions)} for
* accessing a given region. Must not be null and must be a region
* where the service is available.
*
* @see Region#getRegion(com.amazonaws.regions.Regions)
* @see Region#createClient(Class,
* com.amazonaws.auth.AWSCredentialsProvider, ClientConfiguration)
* @see Region#isServiceSupported(String)
*/
void setRegion(Region region);
/**
*
* Creates a new Amazon ECS cluster. By default, your account receives a
* default
cluster when you launch your first container
* instance. However, you can create your own cluster with a unique name
* with the CreateCluster
action.
*
*
* @param createClusterRequest
* @return Result of the CreateCluster operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @sample AmazonECS.CreateCluster
*/
CreateClusterResult createCluster(CreateClusterRequest createClusterRequest);
/**
* Simplified method form for invoking the CreateCluster operation.
*
* @see #createCluster(CreateClusterRequest)
*/
CreateClusterResult createCluster();
/**
*
* Runs and maintains a desired number of tasks from a specified task
* definition. If the number of tasks running in a service drops below
* desiredCount
, Amazon ECS spawns another instantiation of the
* task in the specified cluster. To update an existing service, see
* UpdateService.
*
*
* In addition to maintaining the desired count of tasks in your service,
* you can optionally run your service behind a load balancer. The load
* balancer distributes traffic across the tasks that are associated with
* the service.
*
*
* You can optionally specify a deployment configuration for your service.
* During a deployment (which is triggered by changing the task definition
* of a service with an UpdateService operation), the service
* scheduler uses the minimumHealthyPercent
and
* maximumPercent
parameters to determine the deployment
* strategy.
*
*
* If the minimumHealthyPercent
is below 100%, the scheduler
* can ignore the desiredCount
temporarily during a deployment.
* For example, if your service has a desiredCount
of four
* tasks, a minimumHealthyPercent
of 50% allows the scheduler
* to stop two existing tasks before starting two new tasks. Tasks for
* services that do not use a load balancer are considered healthy if
* they are in the RUNNING
state; tasks for services that
* do use a load balancer are considered healthy if they are in the
* RUNNING
state and the container instance it is hosted on is
* reported as healthy by the load balancer. The default value for
* minimumHealthyPercent
is 50% in the console and 100% for the
* AWS CLI, the AWS SDKs, and the APIs.
*
*
* The maximumPercent
parameter represents an upper limit on
* the number of running tasks during a deployment, which enables you to
* define the deployment batch size. For example, if your service has a
* desiredCount
of four tasks, a maximumPercent
* value of 200% starts four new tasks before stopping the four older tasks
* (provided that the cluster resources required to do this are available).
* The default value for maximumPercent
is 200%.
*
*
* When the service scheduler launches new tasks, it attempts to balance
* them across the Availability Zones in your cluster with the following
* logic:
*
*
* -
*
* Determine which of the container instances in your cluster can support
* your service's task definition (for example, they have the required CPU,
* memory, ports, and container instance attributes).
*
*
* -
*
* Sort the valid container instances by the fewest number of running tasks
* for this service in the same Availability Zone as the instance. For
* example, if zone A has one running service task and zones B and C each
* have zero, valid container instances in either zone B or C are considered
* optimal for placement.
*
*
* -
*
* Place the new service task on a valid container instance in an optimal
* Availability Zone (based on the previous steps), favoring container
* instances with the fewest number of running tasks for this service.
*
*
*
*
* @param createServiceRequest
* @return Result of the CreateService operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster could not be found. You can view your
* available clusters with ListClusters. Amazon ECS clusters
* are region-specific.
* @sample AmazonECS.CreateService
*/
CreateServiceResult createService(CreateServiceRequest createServiceRequest);
/**
*
* Deletes the specified cluster. You must deregister all container
* instances from this cluster before you may delete it. You can list the
* container instances in a cluster with ListContainerInstances and
* deregister them with DeregisterContainerInstance.
*
*
* @param deleteClusterRequest
* @return Result of the DeleteCluster operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster could not be found. You can view your
* available clusters with ListClusters. Amazon ECS clusters
* are region-specific.
* @throws ClusterContainsContainerInstancesException
* You cannot delete a cluster that has registered container
* instances. You must first deregister the container instances
* before you can delete the cluster. For more information, see
* DeregisterContainerInstance.
* @throws ClusterContainsServicesException
* You cannot delete a cluster that contains services. You must
* first update the service to reduce its desired task count to 0
* and then delete the service. For more information, see
* UpdateService and DeleteService.
* @sample AmazonECS.DeleteCluster
*/
DeleteClusterResult deleteCluster(DeleteClusterRequest deleteClusterRequest);
/**
*
* Deletes a specified service within a cluster. You can delete a service if
* you have no running tasks in it and the desired task count is zero. If
* the service is actively maintaining tasks, you cannot delete it, and you
* must update the service to a desired task count of zero. For more
* information, see UpdateService.
*
*
*
* When you delete a service, if there are still running tasks that require
* cleanup, the service status moves from ACTIVE
to
* DRAINING
, and the service is no longer visible in the
* console or in ListServices API operations. After the tasks have
* stopped, then the service status moves from DRAINING
to
* INACTIVE
. Services in the DRAINING
or
* INACTIVE
status can still be viewed with
* DescribeServices API operations; however, in the future,
* INACTIVE
services may be cleaned up and purged from Amazon
* ECS record keeping, and DescribeServices API operations on those
* services will return a ServiceNotFoundException
error.
*
*
*
* @param deleteServiceRequest
* @return Result of the DeleteService operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster could not be found. You can view your
* available clusters with ListClusters. Amazon ECS clusters
* are region-specific.
* @throws ServiceNotFoundException
* The specified service could not be found. You can view your
* available services with ListServices. Amazon ECS services
* are cluster-specific and region-specific.
* @sample AmazonECS.DeleteService
*/
DeleteServiceResult deleteService(DeleteServiceRequest deleteServiceRequest);
/**
*
* Deregisters an Amazon ECS container instance from the specified cluster.
* This instance is no longer available to run tasks.
*
*
* If you intend to use the container instance for some other purpose after
* deregistration, you should stop all of the tasks running on the container
* instance before deregistration to avoid any orphaned tasks from consuming
* resources.
*
*
* Deregistering a container instance removes the instance from a cluster,
* but it does not terminate the EC2 instance; if you are finished using the
* instance, be sure to terminate it in the Amazon EC2 console to stop
* billing.
*
*
*
* If you terminate a running container instance with a connected Amazon ECS
* container agent, the agent automatically deregisters the instance from
* your cluster (stopped container instances or instances with disconnected
* agents are not automatically deregistered when terminated).
*
*
*
* @param deregisterContainerInstanceRequest
* @return Result of the DeregisterContainerInstance operation returned by
* the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster could not be found. You can view your
* available clusters with ListClusters. Amazon ECS clusters
* are region-specific.
* @sample AmazonECS.DeregisterContainerInstance
*/
DeregisterContainerInstanceResult deregisterContainerInstance(
DeregisterContainerInstanceRequest deregisterContainerInstanceRequest);
/**
*
* Deregisters the specified task definition by family and revision. Upon
* deregistration, the task definition is marked as INACTIVE
.
* Existing tasks and services that reference an INACTIVE
task
* definition continue to run without disruption. Existing services that
* reference an INACTIVE
task definition can still scale up or
* down by modifying the service's desired count.
*
*
* You cannot use an INACTIVE
task definition to run new tasks
* or create new services, and you cannot update an existing service to
* reference an INACTIVE
task definition (although there may be
* up to a 10 minute window following deregistration where these
* restrictions have not yet taken effect).
*
*
* @param deregisterTaskDefinitionRequest
* @return Result of the DeregisterTaskDefinition operation returned by the
* service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @sample AmazonECS.DeregisterTaskDefinition
*/
DeregisterTaskDefinitionResult deregisterTaskDefinition(
DeregisterTaskDefinitionRequest deregisterTaskDefinitionRequest);
/**
*
* Describes one or more of your clusters.
*
*
* @param describeClustersRequest
* @return Result of the DescribeClusters operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @sample AmazonECS.DescribeClusters
*/
DescribeClustersResult describeClusters(
DescribeClustersRequest describeClustersRequest);
/**
* Simplified method form for invoking the DescribeClusters operation.
*
* @see #describeClusters(DescribeClustersRequest)
*/
DescribeClustersResult describeClusters();
/**
*
* Describes Amazon EC2 Container Service container instances. Returns
* metadata about registered and remaining resources on each container
* instance requested.
*
*
* @param describeContainerInstancesRequest
* @return Result of the DescribeContainerInstances operation returned by
* the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster could not be found. You can view your
* available clusters with ListClusters. Amazon ECS clusters
* are region-specific.
* @sample AmazonECS.DescribeContainerInstances
*/
DescribeContainerInstancesResult describeContainerInstances(
DescribeContainerInstancesRequest describeContainerInstancesRequest);
/**
*
* Describes the specified services running in your cluster.
*
*
* @param describeServicesRequest
* @return Result of the DescribeServices operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster could not be found. You can view your
* available clusters with ListClusters. Amazon ECS clusters
* are region-specific.
* @sample AmazonECS.DescribeServices
*/
DescribeServicesResult describeServices(
DescribeServicesRequest describeServicesRequest);
/**
*
* Describes a task definition. You can specify a family
and
* revision
to find information about a specific task
* definition, or you can simply specify the family to find the latest
* ACTIVE
revision in that family.
*
*
*
* You can only describe INACTIVE
task definitions while an
* active task or service references them.
*
*
*
* @param describeTaskDefinitionRequest
* @return Result of the DescribeTaskDefinition operation returned by the
* service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @sample AmazonECS.DescribeTaskDefinition
*/
DescribeTaskDefinitionResult describeTaskDefinition(
DescribeTaskDefinitionRequest describeTaskDefinitionRequest);
/**
*
* Describes a specified task or tasks.
*
*
* @param describeTasksRequest
* @return Result of the DescribeTasks operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster could not be found. You can view your
* available clusters with ListClusters. Amazon ECS clusters
* are region-specific.
* @sample AmazonECS.DescribeTasks
*/
DescribeTasksResult describeTasks(DescribeTasksRequest describeTasksRequest);
/**
*
*
* This action is only used by the Amazon EC2 Container Service agent, and
* it is not intended for use outside of the agent.
*
*
*
* Returns an endpoint for the Amazon EC2 Container Service agent to poll
* for updates.
*
*
* @param discoverPollEndpointRequest
* @return Result of the DiscoverPollEndpoint operation returned by the
* service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @sample AmazonECS.DiscoverPollEndpoint
*/
DiscoverPollEndpointResult discoverPollEndpoint(
DiscoverPollEndpointRequest discoverPollEndpointRequest);
/**
* Simplified method form for invoking the DiscoverPollEndpoint operation.
*
* @see #discoverPollEndpoint(DiscoverPollEndpointRequest)
*/
DiscoverPollEndpointResult discoverPollEndpoint();
/**
*
* Returns a list of existing clusters.
*
*
* @param listClustersRequest
* @return Result of the ListClusters operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @sample AmazonECS.ListClusters
*/
ListClustersResult listClusters(ListClustersRequest listClustersRequest);
/**
* Simplified method form for invoking the ListClusters operation.
*
* @see #listClusters(ListClustersRequest)
*/
ListClustersResult listClusters();
/**
*
* Returns a list of container instances in a specified cluster.
*
*
* @param listContainerInstancesRequest
* @return Result of the ListContainerInstances operation returned by the
* service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster could not be found. You can view your
* available clusters with ListClusters. Amazon ECS clusters
* are region-specific.
* @sample AmazonECS.ListContainerInstances
*/
ListContainerInstancesResult listContainerInstances(
ListContainerInstancesRequest listContainerInstancesRequest);
/**
* Simplified method form for invoking the ListContainerInstances operation.
*
* @see #listContainerInstances(ListContainerInstancesRequest)
*/
ListContainerInstancesResult listContainerInstances();
/**
*
* Lists the services that are running in a specified cluster.
*
*
* @param listServicesRequest
* @return Result of the ListServices operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster could not be found. You can view your
* available clusters with ListClusters. Amazon ECS clusters
* are region-specific.
* @sample AmazonECS.ListServices
*/
ListServicesResult listServices(ListServicesRequest listServicesRequest);
/**
* Simplified method form for invoking the ListServices operation.
*
* @see #listServices(ListServicesRequest)
*/
ListServicesResult listServices();
/**
*
* Returns a list of task definition families that are registered to your
* account (which may include task definition families that no longer have
* any ACTIVE
task definitions). You can filter the results
* with the familyPrefix
parameter.
*
*
* @param listTaskDefinitionFamiliesRequest
* @return Result of the ListTaskDefinitionFamilies operation returned by
* the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @sample AmazonECS.ListTaskDefinitionFamilies
*/
ListTaskDefinitionFamiliesResult listTaskDefinitionFamilies(
ListTaskDefinitionFamiliesRequest listTaskDefinitionFamiliesRequest);
/**
* Simplified method form for invoking the ListTaskDefinitionFamilies
* operation.
*
* @see #listTaskDefinitionFamilies(ListTaskDefinitionFamiliesRequest)
*/
ListTaskDefinitionFamiliesResult listTaskDefinitionFamilies();
/**
*
* Returns a list of task definitions that are registered to your account.
* You can filter the results by family name with the
* familyPrefix
parameter or by status with the
* status
parameter.
*
*
* @param listTaskDefinitionsRequest
* @return Result of the ListTaskDefinitions operation returned by the
* service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @sample AmazonECS.ListTaskDefinitions
*/
ListTaskDefinitionsResult listTaskDefinitions(
ListTaskDefinitionsRequest listTaskDefinitionsRequest);
/**
* Simplified method form for invoking the ListTaskDefinitions operation.
*
* @see #listTaskDefinitions(ListTaskDefinitionsRequest)
*/
ListTaskDefinitionsResult listTaskDefinitions();
/**
*
* Returns a list of tasks for a specified cluster. You can filter the
* results by family name, by a particular container instance, or by the
* desired status of the task with the family
,
* containerInstance
, and desiredStatus
* parameters.
*
*
* @param listTasksRequest
* @return Result of the ListTasks operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster could not be found. You can view your
* available clusters with ListClusters. Amazon ECS clusters
* are region-specific.
* @throws ServiceNotFoundException
* The specified service could not be found. You can view your
* available services with ListServices. Amazon ECS services
* are cluster-specific and region-specific.
* @sample AmazonECS.ListTasks
*/
ListTasksResult listTasks(ListTasksRequest listTasksRequest);
/**
* Simplified method form for invoking the ListTasks operation.
*
* @see #listTasks(ListTasksRequest)
*/
ListTasksResult listTasks();
/**
*
*
* This action is only used by the Amazon EC2 Container Service agent, and
* it is not intended for use outside of the agent.
*
*
*
* Registers an EC2 instance into the specified cluster. This instance
* becomes available to place containers on.
*
*
* @param registerContainerInstanceRequest
* @return Result of the RegisterContainerInstance operation returned by the
* service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @sample AmazonECS.RegisterContainerInstance
*/
RegisterContainerInstanceResult registerContainerInstance(
RegisterContainerInstanceRequest registerContainerInstanceRequest);
/**
*
* Registers a new task definition from the supplied family
and
* containerDefinitions
. Optionally, you can add data volumes
* to your containers with the volumes
parameter. For more
* information about task definition parameters and defaults, see Amazon ECS Task Definitions in the Amazon EC2 Container Service
* Developer Guide.
*
*
* @param registerTaskDefinitionRequest
* @return Result of the RegisterTaskDefinition operation returned by the
* service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @sample AmazonECS.RegisterTaskDefinition
*/
RegisterTaskDefinitionResult registerTaskDefinition(
RegisterTaskDefinitionRequest registerTaskDefinitionRequest);
/**
*
* Start a task using random placement and the default Amazon ECS scheduler.
* To use your own scheduler or place a task on a specific container
* instance, use StartTask
instead.
*
*
*
* The count
parameter is limited to 10 tasks per call.
*
*
*
* @param runTaskRequest
* @return Result of the RunTask operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster could not be found. You can view your
* available clusters with ListClusters. Amazon ECS clusters
* are region-specific.
* @sample AmazonECS.RunTask
*/
RunTaskResult runTask(RunTaskRequest runTaskRequest);
/**
*
* Starts a new task from the specified task definition on the specified
* container instance or instances. To use the default Amazon ECS scheduler
* to place your task, use RunTask
instead.
*
*
*
* The list of container instances to start tasks on is limited to 10.
*
*
*
* @param startTaskRequest
* @return Result of the StartTask operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster could not be found. You can view your
* available clusters with ListClusters. Amazon ECS clusters
* are region-specific.
* @sample AmazonECS.StartTask
*/
StartTaskResult startTask(StartTaskRequest startTaskRequest);
/**
*
* Stops a running task.
*
*
* When StopTask is called on a task, the equivalent of
* docker stop
is issued to the containers running in the task.
* This results in a SIGTERM
and a 30-second timeout, after
* which SIGKILL
is sent and the containers are forcibly
* stopped. If the container handles the SIGTERM
gracefully and
* exits within 30 seconds from receiving it, no SIGKILL
is
* sent.
*
*
* @param stopTaskRequest
* @return Result of the StopTask operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster could not be found. You can view your
* available clusters with ListClusters. Amazon ECS clusters
* are region-specific.
* @sample AmazonECS.StopTask
*/
StopTaskResult stopTask(StopTaskRequest stopTaskRequest);
/**
*
*
* This action is only used by the Amazon EC2 Container Service agent, and
* it is not intended for use outside of the agent.
*
*
*
* Sent to acknowledge that a container changed states.
*
*
* @param submitContainerStateChangeRequest
* @return Result of the SubmitContainerStateChange operation returned by
* the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @sample AmazonECS.SubmitContainerStateChange
*/
SubmitContainerStateChangeResult submitContainerStateChange(
SubmitContainerStateChangeRequest submitContainerStateChangeRequest);
/**
* Simplified method form for invoking the SubmitContainerStateChange
* operation.
*
* @see #submitContainerStateChange(SubmitContainerStateChangeRequest)
*/
SubmitContainerStateChangeResult submitContainerStateChange();
/**
*
*
* This action is only used by the Amazon EC2 Container Service agent, and
* it is not intended for use outside of the agent.
*
*
*
* Sent to acknowledge that a task changed states.
*
*
* @param submitTaskStateChangeRequest
* @return Result of the SubmitTaskStateChange operation returned by the
* service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @sample AmazonECS.SubmitTaskStateChange
*/
SubmitTaskStateChangeResult submitTaskStateChange(
SubmitTaskStateChangeRequest submitTaskStateChangeRequest);
/**
*
* Updates the Amazon ECS container agent on a specified container instance.
* Updating the Amazon ECS container agent does not interrupt running tasks
* or services on the container instance. The process for updating the agent
* differs depending on whether your container instance was launched with
* the Amazon ECS-optimized AMI or another operating system.
*
*
* UpdateContainerAgent
requires the Amazon ECS-optimized AMI
* or Amazon Linux with the ecs-init
service installed and
* running. For help updating the Amazon ECS container agent on other
* operating systems, see Manually Updating the Amazon ECS Container Agent in the Amazon
* EC2 Container Service Developer Guide.
*
*
* @param updateContainerAgentRequest
* @return Result of the UpdateContainerAgent operation returned by the
* service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster could not be found. You can view your
* available clusters with ListClusters. Amazon ECS clusters
* are region-specific.
* @throws UpdateInProgressException
* There is already a current Amazon ECS container agent update in
* progress on the specified container instance. If the container
* agent becomes disconnected while it is in a transitional stage,
* such as PENDING
or STAGING
, the update
* process can get stuck in that state. However, when the agent
* reconnects, it resumes where it stopped previously.
* @throws NoUpdateAvailableException
* There is no update available for this Amazon ECS container agent.
* This could be because the agent is already running the latest
* version, or it is so old that there is no update path to the
* current version.
* @throws MissingVersionException
* Amazon ECS is unable to determine the current version of the
* Amazon ECS container agent on the container instance and does not
* have enough information to proceed with an update. This could be
* because the agent running on the container instance is an older
* or custom version that does not use our version information.
* @sample AmazonECS.UpdateContainerAgent
*/
UpdateContainerAgentResult updateContainerAgent(
UpdateContainerAgentRequest updateContainerAgentRequest);
/**
*
* Modifies the desired count, deployment configuration, or task definition
* used in a service.
*
*
* You can add to or subtract from the number of instantiations of a task
* definition in a service by specifying the cluster that the service is
* running in and a new desiredCount
parameter.
*
*
* You can use UpdateService to modify your task definition and
* deploy a new version of your service.
*
*
* You can also update the deployment configuration of a service. When a
* deployment is triggered by updating the task definition of a service, the
* service scheduler uses the deployment configuration parameters,
* minimumHealthyPercent
and maximumPercent
, to
* determine the deployment strategy.
*
*
* If the minimumHealthyPercent
is below 100%, the scheduler
* can ignore the desiredCount
temporarily during a deployment.
* For example, if your service has a desiredCount
of four
* tasks, a minimumHealthyPercent
of 50% allows the scheduler
* to stop two existing tasks before starting two new tasks. Tasks for
* services that do not use a load balancer are considered healthy if
* they are in the RUNNING
state; tasks for services that
* do use a load balancer are considered healthy if they are in the
* RUNNING
state and the container instance it is hosted on is
* reported as healthy by the load balancer.
*
*
* The maximumPercent
parameter represents an upper limit on
* the number of running tasks during a deployment, which enables you to
* define the deployment batch size. For example, if your service has a
* desiredCount
of four tasks, a maximumPercent
* value of 200% starts four new tasks before stopping the four older tasks
* (provided that the cluster resources required to do this are available).
*
*
* When UpdateService stops a task during a deployment, the
* equivalent of docker stop
is issued to the containers
* running in the task. This results in a SIGTERM
and a
* 30-second timeout, after which SIGKILL
is sent and the
* containers are forcibly stopped. If the container handles the
* SIGTERM
gracefully and exits within 30 seconds from
* receiving it, no SIGKILL
is sent.
*
*
* When the service scheduler launches new tasks, it attempts to balance
* them across the Availability Zones in your cluster with the following
* logic:
*
*
* -
*
* Determine which of the container instances in your cluster can support
* your service's task definition (for example, they have the required CPU,
* memory, ports, and container instance attributes).
*
*
* -
*
* Sort the valid container instances by the fewest number of running tasks
* for this service in the same Availability Zone as the instance. For
* example, if zone A has one running service task and zones B and C each
* have zero, valid container instances in either zone B or C are considered
* optimal for placement.
*
*
* -
*
* Place the new service task on a valid container instance in an optimal
* Availability Zone (based on the previous steps), favoring container
* instances with the fewest number of running tasks for this service.
*
*
*
*
* @param updateServiceRequest
* @return Result of the UpdateService operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster could not be found. You can view your
* available clusters with ListClusters. Amazon ECS clusters
* are region-specific.
* @throws ServiceNotFoundException
* The specified service could not be found. You can view your
* available services with ListServices. Amazon ECS services
* are cluster-specific and region-specific.
* @throws ServiceNotActiveException
* The specified service is not active. You cannot update a service
* that is not active. If you have previously deleted a service, you
* can re-create it with CreateService.
* @sample AmazonECS.UpdateService
*/
UpdateServiceResult updateService(UpdateServiceRequest updateServiceRequest);
/**
* Shuts down this client object, releasing any resources that might be held
* open. This is an optional method, and callers are not expected to call
* it, but can if they want to explicitly release any open resources. Once a
* client has been shutdown, it should not be used to make any more
* requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request,
* typically used for debugging issues where a service isn't acting as
* expected. This data isn't considered part of the result data returned by
* an operation, so it's available through this separate, diagnostic
* interface.
*
* Response metadata is only cached for a limited period of time, so if you
* need to access this extra diagnostic information for an executed request,
* you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none
* is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}