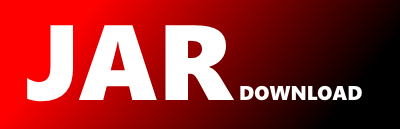
com.amazonaws.services.ecs.AmazonECSClient Maven / Gradle / Ivy
/*
* Copyright 2010-2016 Amazon.com, Inc. or its affiliates. All Rights
* Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.ecs;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import java.util.Map.Entry;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.util.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.services.ecs.model.*;
import com.amazonaws.services.ecs.model.transform.*;
/**
* Client for accessing Amazon ECS. All service calls made using this client are
* blocking, and will not return until the service call completes.
*
*
* Amazon EC2 Container Service (Amazon ECS) is a highly scalable, fast,
* container management service that makes it easy to run, stop, and manage
* Docker containers on a cluster of EC2 instances. Amazon ECS lets you launch
* and stop container-enabled applications with simple API calls, allows you to
* get the state of your cluster from a centralized service, and gives you
* access to many familiar Amazon EC2 features like security groups, Amazon EBS
* volumes, and IAM roles.
*
*
* You can use Amazon ECS to schedule the placement of containers across your
* cluster based on your resource needs, isolation policies, and availability
* requirements. Amazon EC2 Container Service eliminates the need for you to
* operate your own cluster management and configuration management systems or
* worry about scaling your management infrastructure.
*
*/
@ThreadSafe
public class AmazonECSClient extends AmazonWebServiceClient implements
AmazonECS {
/** Provider for AWS credentials. */
private AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AmazonECS.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "ecs";
/** The region metadata service name for computing region endpoints. */
private static final String DEFAULT_ENDPOINT_PREFIX = "ecs";
/**
* Client configuration factory providing ClientConfigurations tailored to
* this client
*/
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
/**
* List of exception unmarshallers for all Amazon ECS exceptions.
*/
protected List jsonErrorUnmarshallers = new ArrayList();
/**
* Constructs a new client to invoke service methods on Amazon ECS. A
* credentials provider chain will be used that searches for credentials in
* this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2
* metadata service
*
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @see DefaultAWSCredentialsProviderChain
*/
public AmazonECSClient() {
this(new DefaultAWSCredentialsProviderChain(), configFactory
.getConfig());
}
/**
* Constructs a new client to invoke service methods on Amazon ECS. A
* credentials provider chain will be used that searches for credentials in
* this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2
* metadata service
*
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param clientConfiguration
* The client configuration options controlling how this client
* connects to Amazon ECS (ex: proxy settings, retry counts, etc.).
*
* @see DefaultAWSCredentialsProviderChain
*/
public AmazonECSClient(ClientConfiguration clientConfiguration) {
this(new DefaultAWSCredentialsProviderChain(), clientConfiguration);
}
/**
* Constructs a new client to invoke service methods on Amazon ECS using the
* specified AWS account credentials.
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when
* authenticating with AWS services.
*/
public AmazonECSClient(AWSCredentials awsCredentials) {
this(awsCredentials, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on Amazon ECS using the
* specified AWS account credentials and client configuration options.
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when
* authenticating with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client
* connects to Amazon ECS (ex: proxy settings, retry counts, etc.).
*/
public AmazonECSClient(AWSCredentials awsCredentials,
ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.awsCredentialsProvider = new StaticCredentialsProvider(
awsCredentials);
init();
}
/**
* Constructs a new client to invoke service methods on Amazon ECS using the
* specified AWS account credentials provider.
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with AWS services.
*/
public AmazonECSClient(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on Amazon ECS using the
* specified AWS account credentials provider and client configuration
* options.
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client
* connects to Amazon ECS (ex: proxy settings, retry counts, etc.).
*/
public AmazonECSClient(AWSCredentialsProvider awsCredentialsProvider,
ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, null);
}
/**
* Constructs a new client to invoke service methods on Amazon ECS using the
* specified AWS account credentials provider, client configuration options,
* and request metric collector.
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client
* connects to Amazon ECS (ex: proxy settings, retry counts, etc.).
* @param requestMetricCollector
* optional request metric collector
*/
public AmazonECSClient(AWSCredentialsProvider awsCredentialsProvider,
ClientConfiguration clientConfiguration,
RequestMetricCollector requestMetricCollector) {
super(clientConfiguration, requestMetricCollector);
this.awsCredentialsProvider = awsCredentialsProvider;
init();
}
private void init() {
jsonErrorUnmarshallers
.add(new JsonErrorUnmarshallerV2(
com.amazonaws.services.ecs.model.ClusterContainsServicesException.class,
"ClusterContainsServicesException"));
jsonErrorUnmarshallers.add(new JsonErrorUnmarshallerV2(
com.amazonaws.services.ecs.model.ServerException.class,
"ServerException"));
jsonErrorUnmarshallers
.add(new JsonErrorUnmarshallerV2(
com.amazonaws.services.ecs.model.NoUpdateAvailableException.class,
"NoUpdateAvailableException"));
jsonErrorUnmarshallers
.add(new JsonErrorUnmarshallerV2(
com.amazonaws.services.ecs.model.ClusterNotFoundException.class,
"ClusterNotFoundException"));
jsonErrorUnmarshallers
.add(new JsonErrorUnmarshallerV2(
com.amazonaws.services.ecs.model.UpdateInProgressException.class,
"UpdateInProgressException"));
jsonErrorUnmarshallers
.add(new JsonErrorUnmarshallerV2(
com.amazonaws.services.ecs.model.ServiceNotFoundException.class,
"ServiceNotFoundException"));
jsonErrorUnmarshallers
.add(new JsonErrorUnmarshallerV2(
com.amazonaws.services.ecs.model.ServiceNotActiveException.class,
"ServiceNotActiveException"));
jsonErrorUnmarshallers.add(new JsonErrorUnmarshallerV2(
com.amazonaws.services.ecs.model.MissingVersionException.class,
"MissingVersionException"));
jsonErrorUnmarshallers
.add(new JsonErrorUnmarshallerV2(
com.amazonaws.services.ecs.model.InvalidParameterException.class,
"InvalidParameterException"));
jsonErrorUnmarshallers
.add(new JsonErrorUnmarshallerV2(
com.amazonaws.services.ecs.model.ClusterContainsContainerInstancesException.class,
"ClusterContainsContainerInstancesException"));
jsonErrorUnmarshallers.add(new JsonErrorUnmarshallerV2(
com.amazonaws.services.ecs.model.ClientException.class,
"ClientException"));
jsonErrorUnmarshallers
.add(JsonErrorUnmarshallerV2.DEFAULT_UNMARSHALLER);
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(DEFAULT_ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("https://ecs.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s
.addAll(chainFactory
.newRequestHandlerChain("/com/amazonaws/services/ecs/request.handlers"));
requestHandler2s
.addAll(chainFactory
.newRequestHandler2Chain("/com/amazonaws/services/ecs/request.handler2s"));
}
/**
*
* Creates a new Amazon ECS cluster. By default, your account receives a
* default
cluster when you launch your first container
* instance. However, you can create your own cluster with a unique name
* with the CreateCluster
action.
*
*
* @param createClusterRequest
* @return Result of the CreateCluster operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @sample AmazonECS.CreateCluster
*/
@Override
public CreateClusterResult createCluster(
CreateClusterRequest createClusterRequest) {
ExecutionContext executionContext = createExecutionContext(createClusterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateClusterRequestMarshaller().marshall(super
.beforeMarshalling(createClusterRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = SdkJsonProtocolFactory
.createResponseHandler(
new CreateClusterResultJsonUnmarshaller(), false);
responseHandler.setIsPayloadJson(true);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public CreateClusterResult createCluster() {
return createCluster(new CreateClusterRequest());
}
/**
*
* Runs and maintains a desired number of tasks from a specified task
* definition. If the number of tasks running in a service drops below
* desiredCount
, Amazon ECS spawns another instantiation of the
* task in the specified cluster. To update an existing service, see
* UpdateService.
*
*
* In addition to maintaining the desired count of tasks in your service,
* you can optionally run your service behind a load balancer. The load
* balancer distributes traffic across the tasks that are associated with
* the service.
*
*
* You can optionally specify a deployment configuration for your service.
* During a deployment (which is triggered by changing the task definition
* of a service with an UpdateService operation), the service
* scheduler uses the minimumHealthyPercent
and
* maximumPercent
parameters to determine the deployment
* strategy.
*
*
* If the minimumHealthyPercent
is below 100%, the scheduler
* can ignore the desiredCount
temporarily during a deployment.
* For example, if your service has a desiredCount
of four
* tasks, a minimumHealthyPercent
of 50% allows the scheduler
* to stop two existing tasks before starting two new tasks. Tasks for
* services that do not use a load balancer are considered healthy if
* they are in the RUNNING
state; tasks for services that
* do use a load balancer are considered healthy if they are in the
* RUNNING
state and the container instance it is hosted on is
* reported as healthy by the load balancer. The default value for
* minimumHealthyPercent
is 50% in the console and 100% for the
* AWS CLI, the AWS SDKs, and the APIs.
*
*
* The maximumPercent
parameter represents an upper limit on
* the number of running tasks during a deployment, which enables you to
* define the deployment batch size. For example, if your service has a
* desiredCount
of four tasks, a maximumPercent
* value of 200% starts four new tasks before stopping the four older tasks
* (provided that the cluster resources required to do this are available).
* The default value for maximumPercent
is 200%.
*
*
* When the service scheduler launches new tasks, it attempts to balance
* them across the Availability Zones in your cluster with the following
* logic:
*
*
* -
*
* Determine which of the container instances in your cluster can support
* your service's task definition (for example, they have the required CPU,
* memory, ports, and container instance attributes).
*
*
* -
*
* Sort the valid container instances by the fewest number of running tasks
* for this service in the same Availability Zone as the instance. For
* example, if zone A has one running service task and zones B and C each
* have zero, valid container instances in either zone B or C are considered
* optimal for placement.
*
*
* -
*
* Place the new service task on a valid container instance in an optimal
* Availability Zone (based on the previous steps), favoring container
* instances with the fewest number of running tasks for this service.
*
*
*
*
* @param createServiceRequest
* @return Result of the CreateService operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster could not be found. You can view your
* available clusters with ListClusters. Amazon ECS clusters
* are region-specific.
* @sample AmazonECS.CreateService
*/
@Override
public CreateServiceResult createService(
CreateServiceRequest createServiceRequest) {
ExecutionContext executionContext = createExecutionContext(createServiceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateServiceRequestMarshaller().marshall(super
.beforeMarshalling(createServiceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = SdkJsonProtocolFactory
.createResponseHandler(
new CreateServiceResultJsonUnmarshaller(), false);
responseHandler.setIsPayloadJson(true);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified cluster. You must deregister all container
* instances from this cluster before you may delete it. You can list the
* container instances in a cluster with ListContainerInstances and
* deregister them with DeregisterContainerInstance.
*
*
* @param deleteClusterRequest
* @return Result of the DeleteCluster operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster could not be found. You can view your
* available clusters with ListClusters. Amazon ECS clusters
* are region-specific.
* @throws ClusterContainsContainerInstancesException
* You cannot delete a cluster that has registered container
* instances. You must first deregister the container instances
* before you can delete the cluster. For more information, see
* DeregisterContainerInstance.
* @throws ClusterContainsServicesException
* You cannot delete a cluster that contains services. You must
* first update the service to reduce its desired task count to 0
* and then delete the service. For more information, see
* UpdateService and DeleteService.
* @sample AmazonECS.DeleteCluster
*/
@Override
public DeleteClusterResult deleteCluster(
DeleteClusterRequest deleteClusterRequest) {
ExecutionContext executionContext = createExecutionContext(deleteClusterRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteClusterRequestMarshaller().marshall(super
.beforeMarshalling(deleteClusterRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = SdkJsonProtocolFactory
.createResponseHandler(
new DeleteClusterResultJsonUnmarshaller(), false);
responseHandler.setIsPayloadJson(true);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a specified service within a cluster. You can delete a service if
* you have no running tasks in it and the desired task count is zero. If
* the service is actively maintaining tasks, you cannot delete it, and you
* must update the service to a desired task count of zero. For more
* information, see UpdateService.
*
*
*
* When you delete a service, if there are still running tasks that require
* cleanup, the service status moves from ACTIVE
to
* DRAINING
, and the service is no longer visible in the
* console or in ListServices API operations. After the tasks have
* stopped, then the service status moves from DRAINING
to
* INACTIVE
. Services in the DRAINING
or
* INACTIVE
status can still be viewed with
* DescribeServices API operations; however, in the future,
* INACTIVE
services may be cleaned up and purged from Amazon
* ECS record keeping, and DescribeServices API operations on those
* services will return a ServiceNotFoundException
error.
*
*
*
* @param deleteServiceRequest
* @return Result of the DeleteService operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster could not be found. You can view your
* available clusters with ListClusters. Amazon ECS clusters
* are region-specific.
* @throws ServiceNotFoundException
* The specified service could not be found. You can view your
* available services with ListServices. Amazon ECS services
* are cluster-specific and region-specific.
* @sample AmazonECS.DeleteService
*/
@Override
public DeleteServiceResult deleteService(
DeleteServiceRequest deleteServiceRequest) {
ExecutionContext executionContext = createExecutionContext(deleteServiceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteServiceRequestMarshaller().marshall(super
.beforeMarshalling(deleteServiceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = SdkJsonProtocolFactory
.createResponseHandler(
new DeleteServiceResultJsonUnmarshaller(), false);
responseHandler.setIsPayloadJson(true);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deregisters an Amazon ECS container instance from the specified cluster.
* This instance is no longer available to run tasks.
*
*
* If you intend to use the container instance for some other purpose after
* deregistration, you should stop all of the tasks running on the container
* instance before deregistration to avoid any orphaned tasks from consuming
* resources.
*
*
* Deregistering a container instance removes the instance from a cluster,
* but it does not terminate the EC2 instance; if you are finished using the
* instance, be sure to terminate it in the Amazon EC2 console to stop
* billing.
*
*
*
* If you terminate a running container instance with a connected Amazon ECS
* container agent, the agent automatically deregisters the instance from
* your cluster (stopped container instances or instances with disconnected
* agents are not automatically deregistered when terminated).
*
*
*
* @param deregisterContainerInstanceRequest
* @return Result of the DeregisterContainerInstance operation returned by
* the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster could not be found. You can view your
* available clusters with ListClusters. Amazon ECS clusters
* are region-specific.
* @sample AmazonECS.DeregisterContainerInstance
*/
@Override
public DeregisterContainerInstanceResult deregisterContainerInstance(
DeregisterContainerInstanceRequest deregisterContainerInstanceRequest) {
ExecutionContext executionContext = createExecutionContext(deregisterContainerInstanceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeregisterContainerInstanceRequestMarshaller()
.marshall(super
.beforeMarshalling(deregisterContainerInstanceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = SdkJsonProtocolFactory
.createResponseHandler(
new DeregisterContainerInstanceResultJsonUnmarshaller(),
false);
responseHandler.setIsPayloadJson(true);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deregisters the specified task definition by family and revision. Upon
* deregistration, the task definition is marked as INACTIVE
.
* Existing tasks and services that reference an INACTIVE
task
* definition continue to run without disruption. Existing services that
* reference an INACTIVE
task definition can still scale up or
* down by modifying the service's desired count.
*
*
* You cannot use an INACTIVE
task definition to run new tasks
* or create new services, and you cannot update an existing service to
* reference an INACTIVE
task definition (although there may be
* up to a 10 minute window following deregistration where these
* restrictions have not yet taken effect).
*
*
* @param deregisterTaskDefinitionRequest
* @return Result of the DeregisterTaskDefinition operation returned by the
* service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @sample AmazonECS.DeregisterTaskDefinition
*/
@Override
public DeregisterTaskDefinitionResult deregisterTaskDefinition(
DeregisterTaskDefinitionRequest deregisterTaskDefinitionRequest) {
ExecutionContext executionContext = createExecutionContext(deregisterTaskDefinitionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeregisterTaskDefinitionRequestMarshaller()
.marshall(super
.beforeMarshalling(deregisterTaskDefinitionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = SdkJsonProtocolFactory
.createResponseHandler(
new DeregisterTaskDefinitionResultJsonUnmarshaller(),
false);
responseHandler.setIsPayloadJson(true);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes one or more of your clusters.
*
*
* @param describeClustersRequest
* @return Result of the DescribeClusters operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @sample AmazonECS.DescribeClusters
*/
@Override
public DescribeClustersResult describeClusters(
DescribeClustersRequest describeClustersRequest) {
ExecutionContext executionContext = createExecutionContext(describeClustersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeClustersRequestMarshaller()
.marshall(super
.beforeMarshalling(describeClustersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = SdkJsonProtocolFactory
.createResponseHandler(
new DescribeClustersResultJsonUnmarshaller(), false);
responseHandler.setIsPayloadJson(true);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeClustersResult describeClusters() {
return describeClusters(new DescribeClustersRequest());
}
/**
*
* Describes Amazon EC2 Container Service container instances. Returns
* metadata about registered and remaining resources on each container
* instance requested.
*
*
* @param describeContainerInstancesRequest
* @return Result of the DescribeContainerInstances operation returned by
* the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster could not be found. You can view your
* available clusters with ListClusters. Amazon ECS clusters
* are region-specific.
* @sample AmazonECS.DescribeContainerInstances
*/
@Override
public DescribeContainerInstancesResult describeContainerInstances(
DescribeContainerInstancesRequest describeContainerInstancesRequest) {
ExecutionContext executionContext = createExecutionContext(describeContainerInstancesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeContainerInstancesRequestMarshaller()
.marshall(super
.beforeMarshalling(describeContainerInstancesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = SdkJsonProtocolFactory
.createResponseHandler(
new DescribeContainerInstancesResultJsonUnmarshaller(),
false);
responseHandler.setIsPayloadJson(true);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes the specified services running in your cluster.
*
*
* @param describeServicesRequest
* @return Result of the DescribeServices operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster could not be found. You can view your
* available clusters with ListClusters. Amazon ECS clusters
* are region-specific.
* @sample AmazonECS.DescribeServices
*/
@Override
public DescribeServicesResult describeServices(
DescribeServicesRequest describeServicesRequest) {
ExecutionContext executionContext = createExecutionContext(describeServicesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeServicesRequestMarshaller()
.marshall(super
.beforeMarshalling(describeServicesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = SdkJsonProtocolFactory
.createResponseHandler(
new DescribeServicesResultJsonUnmarshaller(), false);
responseHandler.setIsPayloadJson(true);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes a task definition. You can specify a family
and
* revision
to find information about a specific task
* definition, or you can simply specify the family to find the latest
* ACTIVE
revision in that family.
*
*
*
* You can only describe INACTIVE
task definitions while an
* active task or service references them.
*
*
*
* @param describeTaskDefinitionRequest
* @return Result of the DescribeTaskDefinition operation returned by the
* service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @sample AmazonECS.DescribeTaskDefinition
*/
@Override
public DescribeTaskDefinitionResult describeTaskDefinition(
DescribeTaskDefinitionRequest describeTaskDefinitionRequest) {
ExecutionContext executionContext = createExecutionContext(describeTaskDefinitionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeTaskDefinitionRequestMarshaller()
.marshall(super
.beforeMarshalling(describeTaskDefinitionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = SdkJsonProtocolFactory
.createResponseHandler(
new DescribeTaskDefinitionResultJsonUnmarshaller(),
false);
responseHandler.setIsPayloadJson(true);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Describes a specified task or tasks.
*
*
* @param describeTasksRequest
* @return Result of the DescribeTasks operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster could not be found. You can view your
* available clusters with ListClusters. Amazon ECS clusters
* are region-specific.
* @sample AmazonECS.DescribeTasks
*/
@Override
public DescribeTasksResult describeTasks(
DescribeTasksRequest describeTasksRequest) {
ExecutionContext executionContext = createExecutionContext(describeTasksRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeTasksRequestMarshaller().marshall(super
.beforeMarshalling(describeTasksRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = SdkJsonProtocolFactory
.createResponseHandler(
new DescribeTasksResultJsonUnmarshaller(), false);
responseHandler.setIsPayloadJson(true);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
*
* This action is only used by the Amazon EC2 Container Service agent, and
* it is not intended for use outside of the agent.
*
*
*
* Returns an endpoint for the Amazon EC2 Container Service agent to poll
* for updates.
*
*
* @param discoverPollEndpointRequest
* @return Result of the DiscoverPollEndpoint operation returned by the
* service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @sample AmazonECS.DiscoverPollEndpoint
*/
@Override
public DiscoverPollEndpointResult discoverPollEndpoint(
DiscoverPollEndpointRequest discoverPollEndpointRequest) {
ExecutionContext executionContext = createExecutionContext(discoverPollEndpointRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DiscoverPollEndpointRequestMarshaller()
.marshall(super
.beforeMarshalling(discoverPollEndpointRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = SdkJsonProtocolFactory
.createResponseHandler(
new DiscoverPollEndpointResultJsonUnmarshaller(),
false);
responseHandler.setIsPayloadJson(true);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DiscoverPollEndpointResult discoverPollEndpoint() {
return discoverPollEndpoint(new DiscoverPollEndpointRequest());
}
/**
*
* Returns a list of existing clusters.
*
*
* @param listClustersRequest
* @return Result of the ListClusters operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @sample AmazonECS.ListClusters
*/
@Override
public ListClustersResult listClusters(
ListClustersRequest listClustersRequest) {
ExecutionContext executionContext = createExecutionContext(listClustersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListClustersRequestMarshaller().marshall(super
.beforeMarshalling(listClustersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = SdkJsonProtocolFactory
.createResponseHandler(
new ListClustersResultJsonUnmarshaller(), false);
responseHandler.setIsPayloadJson(true);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public ListClustersResult listClusters() {
return listClusters(new ListClustersRequest());
}
/**
*
* Returns a list of container instances in a specified cluster.
*
*
* @param listContainerInstancesRequest
* @return Result of the ListContainerInstances operation returned by the
* service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster could not be found. You can view your
* available clusters with ListClusters. Amazon ECS clusters
* are region-specific.
* @sample AmazonECS.ListContainerInstances
*/
@Override
public ListContainerInstancesResult listContainerInstances(
ListContainerInstancesRequest listContainerInstancesRequest) {
ExecutionContext executionContext = createExecutionContext(listContainerInstancesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListContainerInstancesRequestMarshaller()
.marshall(super
.beforeMarshalling(listContainerInstancesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = SdkJsonProtocolFactory
.createResponseHandler(
new ListContainerInstancesResultJsonUnmarshaller(),
false);
responseHandler.setIsPayloadJson(true);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public ListContainerInstancesResult listContainerInstances() {
return listContainerInstances(new ListContainerInstancesRequest());
}
/**
*
* Lists the services that are running in a specified cluster.
*
*
* @param listServicesRequest
* @return Result of the ListServices operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster could not be found. You can view your
* available clusters with ListClusters. Amazon ECS clusters
* are region-specific.
* @sample AmazonECS.ListServices
*/
@Override
public ListServicesResult listServices(
ListServicesRequest listServicesRequest) {
ExecutionContext executionContext = createExecutionContext(listServicesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListServicesRequestMarshaller().marshall(super
.beforeMarshalling(listServicesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = SdkJsonProtocolFactory
.createResponseHandler(
new ListServicesResultJsonUnmarshaller(), false);
responseHandler.setIsPayloadJson(true);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public ListServicesResult listServices() {
return listServices(new ListServicesRequest());
}
/**
*
* Returns a list of task definition families that are registered to your
* account (which may include task definition families that no longer have
* any ACTIVE
task definitions). You can filter the results
* with the familyPrefix
parameter.
*
*
* @param listTaskDefinitionFamiliesRequest
* @return Result of the ListTaskDefinitionFamilies operation returned by
* the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @sample AmazonECS.ListTaskDefinitionFamilies
*/
@Override
public ListTaskDefinitionFamiliesResult listTaskDefinitionFamilies(
ListTaskDefinitionFamiliesRequest listTaskDefinitionFamiliesRequest) {
ExecutionContext executionContext = createExecutionContext(listTaskDefinitionFamiliesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTaskDefinitionFamiliesRequestMarshaller()
.marshall(super
.beforeMarshalling(listTaskDefinitionFamiliesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = SdkJsonProtocolFactory
.createResponseHandler(
new ListTaskDefinitionFamiliesResultJsonUnmarshaller(),
false);
responseHandler.setIsPayloadJson(true);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public ListTaskDefinitionFamiliesResult listTaskDefinitionFamilies() {
return listTaskDefinitionFamilies(new ListTaskDefinitionFamiliesRequest());
}
/**
*
* Returns a list of task definitions that are registered to your account.
* You can filter the results by family name with the
* familyPrefix
parameter or by status with the
* status
parameter.
*
*
* @param listTaskDefinitionsRequest
* @return Result of the ListTaskDefinitions operation returned by the
* service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @sample AmazonECS.ListTaskDefinitions
*/
@Override
public ListTaskDefinitionsResult listTaskDefinitions(
ListTaskDefinitionsRequest listTaskDefinitionsRequest) {
ExecutionContext executionContext = createExecutionContext(listTaskDefinitionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTaskDefinitionsRequestMarshaller()
.marshall(super
.beforeMarshalling(listTaskDefinitionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = SdkJsonProtocolFactory
.createResponseHandler(
new ListTaskDefinitionsResultJsonUnmarshaller(),
false);
responseHandler.setIsPayloadJson(true);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public ListTaskDefinitionsResult listTaskDefinitions() {
return listTaskDefinitions(new ListTaskDefinitionsRequest());
}
/**
*
* Returns a list of tasks for a specified cluster. You can filter the
* results by family name, by a particular container instance, or by the
* desired status of the task with the family
,
* containerInstance
, and desiredStatus
* parameters.
*
*
* @param listTasksRequest
* @return Result of the ListTasks operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster could not be found. You can view your
* available clusters with ListClusters. Amazon ECS clusters
* are region-specific.
* @throws ServiceNotFoundException
* The specified service could not be found. You can view your
* available services with ListServices. Amazon ECS services
* are cluster-specific and region-specific.
* @sample AmazonECS.ListTasks
*/
@Override
public ListTasksResult listTasks(ListTasksRequest listTasksRequest) {
ExecutionContext executionContext = createExecutionContext(listTasksRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTasksRequestMarshaller().marshall(super
.beforeMarshalling(listTasksRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = SdkJsonProtocolFactory
.createResponseHandler(
new ListTasksResultJsonUnmarshaller(), false);
responseHandler.setIsPayloadJson(true);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public ListTasksResult listTasks() {
return listTasks(new ListTasksRequest());
}
/**
*
*
* This action is only used by the Amazon EC2 Container Service agent, and
* it is not intended for use outside of the agent.
*
*
*
* Registers an EC2 instance into the specified cluster. This instance
* becomes available to place containers on.
*
*
* @param registerContainerInstanceRequest
* @return Result of the RegisterContainerInstance operation returned by the
* service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @sample AmazonECS.RegisterContainerInstance
*/
@Override
public RegisterContainerInstanceResult registerContainerInstance(
RegisterContainerInstanceRequest registerContainerInstanceRequest) {
ExecutionContext executionContext = createExecutionContext(registerContainerInstanceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RegisterContainerInstanceRequestMarshaller()
.marshall(super
.beforeMarshalling(registerContainerInstanceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = SdkJsonProtocolFactory
.createResponseHandler(
new RegisterContainerInstanceResultJsonUnmarshaller(),
false);
responseHandler.setIsPayloadJson(true);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Registers a new task definition from the supplied family
and
* containerDefinitions
. Optionally, you can add data volumes
* to your containers with the volumes
parameter. For more
* information about task definition parameters and defaults, see Amazon ECS Task Definitions in the Amazon EC2 Container Service
* Developer Guide.
*
*
* @param registerTaskDefinitionRequest
* @return Result of the RegisterTaskDefinition operation returned by the
* service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @sample AmazonECS.RegisterTaskDefinition
*/
@Override
public RegisterTaskDefinitionResult registerTaskDefinition(
RegisterTaskDefinitionRequest registerTaskDefinitionRequest) {
ExecutionContext executionContext = createExecutionContext(registerTaskDefinitionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RegisterTaskDefinitionRequestMarshaller()
.marshall(super
.beforeMarshalling(registerTaskDefinitionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = SdkJsonProtocolFactory
.createResponseHandler(
new RegisterTaskDefinitionResultJsonUnmarshaller(),
false);
responseHandler.setIsPayloadJson(true);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Start a task using random placement and the default Amazon ECS scheduler.
* To use your own scheduler or place a task on a specific container
* instance, use StartTask
instead.
*
*
*
* The count
parameter is limited to 10 tasks per call.
*
*
*
* @param runTaskRequest
* @return Result of the RunTask operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster could not be found. You can view your
* available clusters with ListClusters. Amazon ECS clusters
* are region-specific.
* @sample AmazonECS.RunTask
*/
@Override
public RunTaskResult runTask(RunTaskRequest runTaskRequest) {
ExecutionContext executionContext = createExecutionContext(runTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RunTaskRequestMarshaller().marshall(super
.beforeMarshalling(runTaskRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = SdkJsonProtocolFactory
.createResponseHandler(new RunTaskResultJsonUnmarshaller(),
false);
responseHandler.setIsPayloadJson(true);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Starts a new task from the specified task definition on the specified
* container instance or instances. To use the default Amazon ECS scheduler
* to place your task, use RunTask
instead.
*
*
*
* The list of container instances to start tasks on is limited to 10.
*
*
*
* @param startTaskRequest
* @return Result of the StartTask operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster could not be found. You can view your
* available clusters with ListClusters. Amazon ECS clusters
* are region-specific.
* @sample AmazonECS.StartTask
*/
@Override
public StartTaskResult startTask(StartTaskRequest startTaskRequest) {
ExecutionContext executionContext = createExecutionContext(startTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartTaskRequestMarshaller().marshall(super
.beforeMarshalling(startTaskRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = SdkJsonProtocolFactory
.createResponseHandler(
new StartTaskResultJsonUnmarshaller(), false);
responseHandler.setIsPayloadJson(true);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Stops a running task.
*
*
* When StopTask is called on a task, the equivalent of
* docker stop
is issued to the containers running in the task.
* This results in a SIGTERM
and a 30-second timeout, after
* which SIGKILL
is sent and the containers are forcibly
* stopped. If the container handles the SIGTERM
gracefully and
* exits within 30 seconds from receiving it, no SIGKILL
is
* sent.
*
*
* @param stopTaskRequest
* @return Result of the StopTask operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster could not be found. You can view your
* available clusters with ListClusters. Amazon ECS clusters
* are region-specific.
* @sample AmazonECS.StopTask
*/
@Override
public StopTaskResult stopTask(StopTaskRequest stopTaskRequest) {
ExecutionContext executionContext = createExecutionContext(stopTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StopTaskRequestMarshaller().marshall(super
.beforeMarshalling(stopTaskRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = SdkJsonProtocolFactory
.createResponseHandler(
new StopTaskResultJsonUnmarshaller(), false);
responseHandler.setIsPayloadJson(true);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
*
* This action is only used by the Amazon EC2 Container Service agent, and
* it is not intended for use outside of the agent.
*
*
*
* Sent to acknowledge that a container changed states.
*
*
* @param submitContainerStateChangeRequest
* @return Result of the SubmitContainerStateChange operation returned by
* the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @sample AmazonECS.SubmitContainerStateChange
*/
@Override
public SubmitContainerStateChangeResult submitContainerStateChange(
SubmitContainerStateChangeRequest submitContainerStateChangeRequest) {
ExecutionContext executionContext = createExecutionContext(submitContainerStateChangeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SubmitContainerStateChangeRequestMarshaller()
.marshall(super
.beforeMarshalling(submitContainerStateChangeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = SdkJsonProtocolFactory
.createResponseHandler(
new SubmitContainerStateChangeResultJsonUnmarshaller(),
false);
responseHandler.setIsPayloadJson(true);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public SubmitContainerStateChangeResult submitContainerStateChange() {
return submitContainerStateChange(new SubmitContainerStateChangeRequest());
}
/**
*
*
* This action is only used by the Amazon EC2 Container Service agent, and
* it is not intended for use outside of the agent.
*
*
*
* Sent to acknowledge that a task changed states.
*
*
* @param submitTaskStateChangeRequest
* @return Result of the SubmitTaskStateChange operation returned by the
* service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @sample AmazonECS.SubmitTaskStateChange
*/
@Override
public SubmitTaskStateChangeResult submitTaskStateChange(
SubmitTaskStateChangeRequest submitTaskStateChangeRequest) {
ExecutionContext executionContext = createExecutionContext(submitTaskStateChangeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SubmitTaskStateChangeRequestMarshaller()
.marshall(super
.beforeMarshalling(submitTaskStateChangeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = SdkJsonProtocolFactory
.createResponseHandler(
new SubmitTaskStateChangeResultJsonUnmarshaller(),
false);
responseHandler.setIsPayloadJson(true);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the Amazon ECS container agent on a specified container instance.
* Updating the Amazon ECS container agent does not interrupt running tasks
* or services on the container instance. The process for updating the agent
* differs depending on whether your container instance was launched with
* the Amazon ECS-optimized AMI or another operating system.
*
*
* UpdateContainerAgent
requires the Amazon ECS-optimized AMI
* or Amazon Linux with the ecs-init
service installed and
* running. For help updating the Amazon ECS container agent on other
* operating systems, see Manually Updating the Amazon ECS Container Agent in the Amazon
* EC2 Container Service Developer Guide.
*
*
* @param updateContainerAgentRequest
* @return Result of the UpdateContainerAgent operation returned by the
* service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster could not be found. You can view your
* available clusters with ListClusters. Amazon ECS clusters
* are region-specific.
* @throws UpdateInProgressException
* There is already a current Amazon ECS container agent update in
* progress on the specified container instance. If the container
* agent becomes disconnected while it is in a transitional stage,
* such as PENDING
or STAGING
, the update
* process can get stuck in that state. However, when the agent
* reconnects, it resumes where it stopped previously.
* @throws NoUpdateAvailableException
* There is no update available for this Amazon ECS container agent.
* This could be because the agent is already running the latest
* version, or it is so old that there is no update path to the
* current version.
* @throws MissingVersionException
* Amazon ECS is unable to determine the current version of the
* Amazon ECS container agent on the container instance and does not
* have enough information to proceed with an update. This could be
* because the agent running on the container instance is an older
* or custom version that does not use our version information.
* @sample AmazonECS.UpdateContainerAgent
*/
@Override
public UpdateContainerAgentResult updateContainerAgent(
UpdateContainerAgentRequest updateContainerAgentRequest) {
ExecutionContext executionContext = createExecutionContext(updateContainerAgentRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateContainerAgentRequestMarshaller()
.marshall(super
.beforeMarshalling(updateContainerAgentRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = SdkJsonProtocolFactory
.createResponseHandler(
new UpdateContainerAgentResultJsonUnmarshaller(),
false);
responseHandler.setIsPayloadJson(true);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Modifies the desired count, deployment configuration, or task definition
* used in a service.
*
*
* You can add to or subtract from the number of instantiations of a task
* definition in a service by specifying the cluster that the service is
* running in and a new desiredCount
parameter.
*
*
* You can use UpdateService to modify your task definition and
* deploy a new version of your service.
*
*
* You can also update the deployment configuration of a service. When a
* deployment is triggered by updating the task definition of a service, the
* service scheduler uses the deployment configuration parameters,
* minimumHealthyPercent
and maximumPercent
, to
* determine the deployment strategy.
*
*
* If the minimumHealthyPercent
is below 100%, the scheduler
* can ignore the desiredCount
temporarily during a deployment.
* For example, if your service has a desiredCount
of four
* tasks, a minimumHealthyPercent
of 50% allows the scheduler
* to stop two existing tasks before starting two new tasks. Tasks for
* services that do not use a load balancer are considered healthy if
* they are in the RUNNING
state; tasks for services that
* do use a load balancer are considered healthy if they are in the
* RUNNING
state and the container instance it is hosted on is
* reported as healthy by the load balancer.
*
*
* The maximumPercent
parameter represents an upper limit on
* the number of running tasks during a deployment, which enables you to
* define the deployment batch size. For example, if your service has a
* desiredCount
of four tasks, a maximumPercent
* value of 200% starts four new tasks before stopping the four older tasks
* (provided that the cluster resources required to do this are available).
*
*
* When UpdateService stops a task during a deployment, the
* equivalent of docker stop
is issued to the containers
* running in the task. This results in a SIGTERM
and a
* 30-second timeout, after which SIGKILL
is sent and the
* containers are forcibly stopped. If the container handles the
* SIGTERM
gracefully and exits within 30 seconds from
* receiving it, no SIGKILL
is sent.
*
*
* When the service scheduler launches new tasks, it attempts to balance
* them across the Availability Zones in your cluster with the following
* logic:
*
*
* -
*
* Determine which of the container instances in your cluster can support
* your service's task definition (for example, they have the required CPU,
* memory, ports, and container instance attributes).
*
*
* -
*
* Sort the valid container instances by the fewest number of running tasks
* for this service in the same Availability Zone as the instance. For
* example, if zone A has one running service task and zones B and C each
* have zero, valid container instances in either zone B or C are considered
* optimal for placement.
*
*
* -
*
* Place the new service task on a valid container instance in an optimal
* Availability Zone (based on the previous steps), favoring container
* instances with the fewest number of running tasks for this service.
*
*
*
*
* @param updateServiceRequest
* @return Result of the UpdateService operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action, such as using
* an action or resource on behalf of a user that doesn't have
* permission to use the action or resource, or specifying an
* identifier that is not valid.
* @throws InvalidParameterException
* The specified parameter is invalid. Review the available
* parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster could not be found. You can view your
* available clusters with ListClusters. Amazon ECS clusters
* are region-specific.
* @throws ServiceNotFoundException
* The specified service could not be found. You can view your
* available services with ListServices. Amazon ECS services
* are cluster-specific and region-specific.
* @throws ServiceNotActiveException
* The specified service is not active. You cannot update a service
* that is not active. If you have previously deleted a service, you
* can re-create it with CreateService.
* @sample AmazonECS.UpdateService
*/
@Override
public UpdateServiceResult updateService(
UpdateServiceRequest updateServiceRequest) {
ExecutionContext executionContext = createExecutionContext(updateServiceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateServiceRequestMarshaller().marshall(super
.beforeMarshalling(updateServiceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
JsonResponseHandler responseHandler = SdkJsonProtocolFactory
.createResponseHandler(
new UpdateServiceResultJsonUnmarshaller(), false);
responseHandler.setIsPayloadJson(true);
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful,
* request, typically used for debugging issues where a service isn't acting
* as expected. This data isn't considered part of the result data returned
* by an operation, so it's available through this separate, diagnostic
* interface.
*
* Response metadata is only cached for a limited period of time, so if you
* need to access this extra diagnostic information for an executed request,
* you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none
* is available.
*/
public ResponseMetadata getCachedResponseMetadata(
AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be
* overriden at the request level.
**/
private Response invoke(
Request request,
HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
executionContext.setCredentialsProvider(CredentialUtils
.getCredentialsProvider(request.getOriginalRequest(),
awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext);
}
/**
* Invoke with no authentication. Credentials are not required and any
* credentials set on the client or request will be ignored for this
* operation.
**/
private Response anonymousInvoke(
Request request,
HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack
* thereof) have been configured in the ExecutionContext beforehand.
**/
private Response doInvoke(
Request request,
HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
request.setEndpoint(endpoint);
request.setTimeOffset(timeOffset);
JsonErrorResponseHandlerV2 errorResponseHandler = SdkJsonProtocolFactory
.createErrorResponseHandler(jsonErrorUnmarshallers, false);
return client.execute(request, responseHandler, errorResponseHandler,
executionContext);
}
}