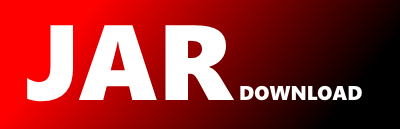
com.amazonaws.services.ecs.model.ContainerDefinition Maven / Gradle / Ivy
/*
* Copyright 2010-2016 Amazon.com, Inc. or its affiliates. All Rights
* Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.ecs.model;
import java.io.Serializable;
/**
*
* Container definitions are used in task definitions to describe the different
* containers that are launched as part of a task.
*
*/
public class ContainerDefinition implements Serializable, Cloneable {
/**
*
* The name of a container. If you are linking multiple containers together
* in a task definition, the name
of one container can be
* entered in the links
of another container to connect the
* containers. Up to 255 letters (uppercase and lowercase), numbers,
* hyphens, and underscores are allowed. This parameter maps to
* name
in the Create a container section of the Docker Remote API and the --name
option to docker run.
*
*/
private String name;
/**
*
* The image used to start a container. This string is passed directly to
* the Docker daemon. Images in the Docker Hub registry are available by
* default. Other repositories are specified with
* repository-url/image:tag
. Up to 255
* letters (uppercase and lowercase), numbers, hyphens, underscores, colons,
* periods, forward slashes, and number signs are allowed. This parameter
* maps to Image
in the Create a container section of the Docker Remote API and the IMAGE
parameter of docker run.
*
*
* -
*
* Images in official repositories on Docker Hub use a single name (for
* example, ubuntu
or mongo
).
*
*
* -
*
* Images in other repositories on Docker Hub are qualified with an
* organization name (for example, amazon/amazon-ecs-agent
).
*
*
* -
*
* Images in other online repositories are qualified further by a domain
* name (for example, quay.io/assemblyline/ubuntu
).
*
*
*
*/
private String image;
/**
*
* The number of cpu
units reserved for the container. A
* container instance has 1,024 cpu
units for every CPU core.
* This parameter specifies the minimum amount of CPU to reserve for a
* container, and containers share unallocated CPU units with other
* containers on the instance with the same ratio as their allocated amount.
* This parameter maps to CpuShares
in the Create a container section of the Docker Remote API and the --cpu-shares
option to docker run.
*
*
*
* You can determine the number of CPU units that are available per EC2
* instance type by multiplying the vCPUs listed for that instance type on
* the Amazon EC2
* Instances detail page by 1,024.
*
*
*
* For example, if you run a single-container task on a single-core instance
* type with 512 CPU units specified for that container, and that is the
* only task running on the container instance, that container could use the
* full 1,024 CPU unit share at any given time. However, if you launched
* another copy of the same task on that container instance, each task would
* be guaranteed a minimum of 512 CPU units when needed, and each container
* could float to higher CPU usage if the other container was not using it,
* but if both tasks were 100% active all of the time, they would be limited
* to 512 CPU units.
*
*
* The Docker daemon on the container instance uses the CPU value to
* calculate the relative CPU share ratios for running containers. For more
* information, see CPU
* share constraint in the Docker documentation. The minimum valid CPU
* share value that the Linux kernel allows is 2; however, the CPU parameter
* is not required, and you can use CPU values below 2 in your container
* definitions. For CPU values below 2 (including null), the behavior varies
* based on your Amazon ECS container agent version:
*
*
* -
*
* Agent versions less than or equal to 1.1.0: Null and zero CPU
* values are passed to Docker as 0, which Docker then converts to 1,024 CPU
* shares. CPU values of 1 are passed to Docker as 1, which the Linux kernel
* converts to 2 CPU shares.
*
*
* -
*
* Agent versions greater than or equal to 1.2.0: Null, zero, and CPU
* values of 1 are passed to Docker as 2.
*
*
*
*/
private Integer cpu;
/**
*
* The number of MiB of memory to reserve for the container. You must
* specify a non-zero integer for this parameter; the Docker daemon reserves
* a minimum of 4 MiB of memory for a container, so you should not specify
* fewer than 4 MiB of memory for your containers. If your container
* attempts to exceed the memory allocated here, the container is killed.
* This parameter maps to Memory
in the Create a container section of the Docker Remote API and the --memory
option to docker run.
*
*/
private Integer memory;
/**
*
* The link
parameter allows containers to communicate with
* each other without the need for port mappings, using the
* name
parameter and optionally, an alias
for the
* link. This construct is analogous to name:alias
in Docker
* links. Up to 255 letters (uppercase and lowercase), numbers, hyphens, and
* underscores are allowed for each name
and alias
* . For more information on linking Docker containers, see https://docs.docker.com/userguide/dockerlinks/. This parameter maps
* to Links
in the Create a container section of the Docker Remote API and the --link
option to
* docker run
.
*
*
*
* Containers that are collocated on a single container instance may be able
* to communicate with each other without requiring links or host port
* mappings. Network isolation is achieved on the container instance using
* security groups and VPC settings.
*
*
*/
private com.amazonaws.internal.SdkInternalList links;
/**
*
* The list of port mappings for the container. Port mappings allow
* containers to access ports on the host container instance to send or
* receive traffic. This parameter maps to PortBindings
in the
* Create a container section of the Docker Remote API and the --publish
option to docker run.
*
*
*
* After a task reaches the RUNNING
status, manual and
* automatic host and container port assignments are visible in the
* Network Bindings section of a container description of a selected
* task in the Amazon ECS console, or the networkBindings
* section DescribeTasks responses.
*
*
*/
private com.amazonaws.internal.SdkInternalList portMappings;
/**
*
* If the essential
parameter of a container is marked as
* true
, and that container fails or stops for any reason, all
* other containers that are part of the task are stopped. If the
* essential
parameter of a container is marked as
* false
, then its failure does not affect the rest of the
* containers in a task. If this parameter is omitted, a container is
* assumed to be essential.
*
*
* All tasks must have at least one essential container. If you have an
* application that is composed of multiple containers, you should group
* containers that are used for a common purpose into components, and
* separate the different components into multiple task definitions. For
* more information, see Application Architecture in the Amazon EC2 Container Service
* Developer Guide.
*
*/
private Boolean essential;
/**
*
*
* Early versions of the Amazon ECS container agent do not properly handle
* entryPoint
parameters. If you have problems using
* entryPoint
, update your container agent or enter your
* commands and arguments as command
array items instead.
*
*
*
* The entry point that is passed to the container. This parameter maps to
* Entrypoint
in the Create a container section of the Docker Remote API and the --entrypoint
option to docker run.
* For more information, see https://docs.docker.com/reference/builder/#entrypoint.
*
*/
private com.amazonaws.internal.SdkInternalList entryPoint;
/**
*
* The command that is passed to the container. This parameter maps to
* Cmd
in the Create a container section of the Docker Remote API and the COMMAND
parameter to docker run.
* For more information, see https://docs.docker.com/reference/builder/#cmd.
*
*/
private com.amazonaws.internal.SdkInternalList command;
/**
*
* The environment variables to pass to a container. This parameter maps to
* Env
in the Create a container section of the Docker Remote API and the --env
option to docker run.
*
*
*
* We do not recommend using plain text environment variables for sensitive
* information, such as credential data.
*
*
*/
private com.amazonaws.internal.SdkInternalList environment;
/**
*
* The mount points for data volumes in your container. This parameter maps
* to Volumes
in the Create a container section of the Docker Remote API and the --volume
option to docker run.
*
*/
private com.amazonaws.internal.SdkInternalList mountPoints;
/**
*
* Data volumes to mount from another container. This parameter maps to
* VolumesFrom
in the Create a container section of the Docker Remote API and the --volumes-from
option to docker run.
*
*/
private com.amazonaws.internal.SdkInternalList volumesFrom;
/**
*
* The hostname to use for your container. This parameter maps to
* Hostname
in the Create a container section of the Docker Remote API and the --hostname
option to docker run.
*
*/
private String hostname;
/**
*
* The user name to use inside the container. This parameter maps to
* User
in the Create a container section of the Docker Remote API and the --user
option to docker run.
*
*/
private String user;
/**
*
* The working directory in which to run commands inside the container. This
* parameter maps to WorkingDir
in the Create a container section of the Docker Remote API and the --workdir
option to docker run.
*
*/
private String workingDirectory;
/**
*
* When this parameter is true, networking is disabled within the container.
* This parameter maps to NetworkDisabled
in the Create a container section of the Docker Remote API.
*
*/
private Boolean disableNetworking;
/**
*
* When this parameter is true, the container is given elevated privileges
* on the host container instance (similar to the root
user).
* This parameter maps to Privileged
in the Create a container section of the Docker Remote API and the --privileged
option to docker run.
*
*/
private Boolean privileged;
/**
*
* When this parameter is true, the container is given read-only access to
* its root file system. This parameter maps to ReadonlyRootfs
* in the Create a container section of the Docker Remote API and the --read-only
option to
* docker run
.
*
*/
private Boolean readonlyRootFilesystem;
/**
*
* A list of DNS servers that are presented to the container. This parameter
* maps to Dns
in the Create a container section of the Docker Remote API and the --dns
option to docker run.
*
*/
private com.amazonaws.internal.SdkInternalList dnsServers;
/**
*
* A list of DNS search domains that are presented to the container. This
* parameter maps to DnsSearch
in the Create a container section of the Docker Remote API and the --dns-search
option to docker run.
*
*/
private com.amazonaws.internal.SdkInternalList dnsSearchDomains;
/**
*
* A list of hostnames and IP address mappings to append to the
* /etc/hosts
file on the container. This parameter maps to
* ExtraHosts
in the Create a container section of the Docker Remote API and the --add-host
option to docker run.
*
*/
private com.amazonaws.internal.SdkInternalList extraHosts;
/**
*
* A list of strings to provide custom labels for SELinux and AppArmor
* multi-level security systems. This parameter maps to
* SecurityOpt
in the Create a container section of the Docker Remote API and the --security-opt
option to docker run.
*
*
*
* The Amazon ECS container agent running on a container instance must
* register with the ECS_SELINUX_CAPABLE=true
or
* ECS_APPARMOR_CAPABLE=true
environment variables before
* containers placed on that instance can use these security options. For
* more information, see Amazon ECS Container Agent Configuration in the Amazon EC2
* Container Service Developer Guide.
*
*
*/
private com.amazonaws.internal.SdkInternalList dockerSecurityOptions;
/**
*
* A key/value map of labels to add to the container. This parameter maps to
* Labels
in the Create a container section of the Docker Remote API and the --label
option to docker run.
* This parameter requires version 1.18 of the Docker Remote API or greater
* on your container instance. To check the Docker Remote API version on
* your container instance, log into your container instance and run the
* following command:
* sudo docker version | grep "Server API version"
*
*/
private java.util.Map dockerLabels;
/**
*
* A list of ulimits
to set in the container. This parameter
* maps to Ulimits
in the Create a container section of the Docker Remote API and the --ulimit
option to docker run.
* Valid naming values are displayed in the Ulimit data type. This
* parameter requires version 1.18 of the Docker Remote API or greater on
* your container instance. To check the Docker Remote API version on your
* container instance, log into your container instance and run the
* following command:
* sudo docker version | grep "Server API version"
*
*/
private com.amazonaws.internal.SdkInternalList ulimits;
/**
*
* The log configuration specification for the container. This parameter
* maps to LogConfig
in the Create a container section of the Docker Remote API and the --log-driver
option to docker run.
* By default, containers use the same logging driver that the Docker daemon
* uses; however the container may use a different logging driver than the
* Docker daemon by specifying a log driver with this parameter in the
* container definition. To use a different logging driver for a container,
* the log system must be configured properly on the container instance (or
* on a different log server for remote logging options). For more
* information on the options for different supported log drivers, see Configure
* logging drivers in the Docker documentation.
*
*
*
* Amazon ECS currently supports a subset of the logging drivers available
* to the Docker daemon (shown in the LogConfiguration data type).
* Currently unsupported log drivers may be available in future releases of
* the Amazon ECS container agent.
*
*
*
* This parameter requires version 1.18 of the Docker Remote API or greater
* on your container instance. To check the Docker Remote API version on
* your container instance, log into your container instance and run the
* following command:
* sudo docker version | grep "Server API version"
*
*
*
* The Amazon ECS container agent running on a container instance must
* register the logging drivers available on that instance with the
* ECS_AVAILABLE_LOGGING_DRIVERS
environment variable before
* containers placed on that instance can use these log configuration
* options. For more information, see Amazon ECS Container Agent Configuration in the Amazon EC2
* Container Service Developer Guide.
*
*
*/
private LogConfiguration logConfiguration;
/**
*
* The name of a container. If you are linking multiple containers together
* in a task definition, the name
of one container can be
* entered in the links
of another container to connect the
* containers. Up to 255 letters (uppercase and lowercase), numbers,
* hyphens, and underscores are allowed. This parameter maps to
* name
in the Create a container section of the Docker Remote API and the --name
option to docker run.
*
*
* @param name
* The name of a container. If you are linking multiple containers
* together in a task definition, the name
of one
* container can be entered in the links
of another
* container to connect the containers. Up to 255 letters (uppercase
* and lowercase), numbers, hyphens, and underscores are allowed.
* This parameter maps to name
in the Create a container section of the Docker Remote API and the --name
option to docker
* run.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of a container. If you are linking multiple containers together
* in a task definition, the name
of one container can be
* entered in the links
of another container to connect the
* containers. Up to 255 letters (uppercase and lowercase), numbers,
* hyphens, and underscores are allowed. This parameter maps to
* name
in the Create a container section of the Docker Remote API and the --name
option to docker run.
*
*
* @return The name of a container. If you are linking multiple containers
* together in a task definition, the name
of one
* container can be entered in the links
of another
* container to connect the containers. Up to 255 letters (uppercase
* and lowercase), numbers, hyphens, and underscores are allowed.
* This parameter maps to name
in the Create a container section of the Docker Remote API and the --name
option to docker
* run.
*/
public String getName() {
return this.name;
}
/**
*
* The name of a container. If you are linking multiple containers together
* in a task definition, the name
of one container can be
* entered in the links
of another container to connect the
* containers. Up to 255 letters (uppercase and lowercase), numbers,
* hyphens, and underscores are allowed. This parameter maps to
* name
in the Create a container section of the Docker Remote API and the --name
option to docker run.
*
*
* @param name
* The name of a container. If you are linking multiple containers
* together in a task definition, the name
of one
* container can be entered in the links
of another
* container to connect the containers. Up to 255 letters (uppercase
* and lowercase), numbers, hyphens, and underscores are allowed.
* This parameter maps to name
in the Create a container section of the Docker Remote API and the --name
option to docker
* run.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withName(String name) {
setName(name);
return this;
}
/**
*
* The image used to start a container. This string is passed directly to
* the Docker daemon. Images in the Docker Hub registry are available by
* default. Other repositories are specified with
* repository-url/image:tag
. Up to 255
* letters (uppercase and lowercase), numbers, hyphens, underscores, colons,
* periods, forward slashes, and number signs are allowed. This parameter
* maps to Image
in the Create a container section of the Docker Remote API and the IMAGE
parameter of docker run.
*
*
* -
*
* Images in official repositories on Docker Hub use a single name (for
* example, ubuntu
or mongo
).
*
*
* -
*
* Images in other repositories on Docker Hub are qualified with an
* organization name (for example, amazon/amazon-ecs-agent
).
*
*
* -
*
* Images in other online repositories are qualified further by a domain
* name (for example, quay.io/assemblyline/ubuntu
).
*
*
*
*
* @param image
* The image used to start a container. This string is passed
* directly to the Docker daemon. Images in the Docker Hub registry
* are available by default. Other repositories are specified with
* repository-url/image:tag
. Up
* to 255 letters (uppercase and lowercase), numbers, hyphens,
* underscores, colons, periods, forward slashes, and number signs
* are allowed. This parameter maps to Image
in the Create a container section of the Docker Remote API and the IMAGE
parameter of docker
* run.
*
* -
*
* Images in official repositories on Docker Hub use a single name
* (for example, ubuntu
or mongo
).
*
*
* -
*
* Images in other repositories on Docker Hub are qualified with an
* organization name (for example,
* amazon/amazon-ecs-agent
).
*
*
* -
*
* Images in other online repositories are qualified further by a
* domain name (for example, quay.io/assemblyline/ubuntu
* ).
*
*
*/
public void setImage(String image) {
this.image = image;
}
/**
*
* The image used to start a container. This string is passed directly to
* the Docker daemon. Images in the Docker Hub registry are available by
* default. Other repositories are specified with
* repository-url/image:tag
. Up to 255
* letters (uppercase and lowercase), numbers, hyphens, underscores, colons,
* periods, forward slashes, and number signs are allowed. This parameter
* maps to Image
in the Create a container section of the Docker Remote API and the IMAGE
parameter of docker run.
*
*
* -
*
* Images in official repositories on Docker Hub use a single name (for
* example, ubuntu
or mongo
).
*
*
* -
*
* Images in other repositories on Docker Hub are qualified with an
* organization name (for example, amazon/amazon-ecs-agent
).
*
*
* -
*
* Images in other online repositories are qualified further by a domain
* name (for example, quay.io/assemblyline/ubuntu
).
*
*
*
*
* @return The image used to start a container. This string is passed
* directly to the Docker daemon. Images in the Docker Hub registry
* are available by default. Other repositories are specified with
* repository-url/image:tag
. Up
* to 255 letters (uppercase and lowercase), numbers, hyphens,
* underscores, colons, periods, forward slashes, and number signs
* are allowed. This parameter maps to Image
in the Create a container section of the Docker Remote API and the IMAGE
parameter of docker
* run.
*
* -
*
* Images in official repositories on Docker Hub use a single name
* (for example, ubuntu
or mongo
).
*
*
* -
*
* Images in other repositories on Docker Hub are qualified with an
* organization name (for example,
* amazon/amazon-ecs-agent
).
*
*
* -
*
* Images in other online repositories are qualified further by a
* domain name (for example,
* quay.io/assemblyline/ubuntu
).
*
*
*/
public String getImage() {
return this.image;
}
/**
*
* The image used to start a container. This string is passed directly to
* the Docker daemon. Images in the Docker Hub registry are available by
* default. Other repositories are specified with
* repository-url/image:tag
. Up to 255
* letters (uppercase and lowercase), numbers, hyphens, underscores, colons,
* periods, forward slashes, and number signs are allowed. This parameter
* maps to Image
in the Create a container section of the Docker Remote API and the IMAGE
parameter of docker run.
*
*
* -
*
* Images in official repositories on Docker Hub use a single name (for
* example, ubuntu
or mongo
).
*
*
* -
*
* Images in other repositories on Docker Hub are qualified with an
* organization name (for example, amazon/amazon-ecs-agent
).
*
*
* -
*
* Images in other online repositories are qualified further by a domain
* name (for example, quay.io/assemblyline/ubuntu
).
*
*
*
*
* @param image
* The image used to start a container. This string is passed
* directly to the Docker daemon. Images in the Docker Hub registry
* are available by default. Other repositories are specified with
* repository-url/image:tag
. Up
* to 255 letters (uppercase and lowercase), numbers, hyphens,
* underscores, colons, periods, forward slashes, and number signs
* are allowed. This parameter maps to Image
in the Create a container section of the Docker Remote API and the IMAGE
parameter of docker
* run.
*
* -
*
* Images in official repositories on Docker Hub use a single name
* (for example, ubuntu
or mongo
).
*
*
* -
*
* Images in other repositories on Docker Hub are qualified with an
* organization name (for example,
* amazon/amazon-ecs-agent
).
*
*
* -
*
* Images in other online repositories are qualified further by a
* domain name (for example, quay.io/assemblyline/ubuntu
* ).
*
*
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withImage(String image) {
setImage(image);
return this;
}
/**
*
* The number of cpu
units reserved for the container. A
* container instance has 1,024 cpu
units for every CPU core.
* This parameter specifies the minimum amount of CPU to reserve for a
* container, and containers share unallocated CPU units with other
* containers on the instance with the same ratio as their allocated amount.
* This parameter maps to CpuShares
in the Create a container section of the Docker Remote API and the --cpu-shares
option to docker run.
*
*
*
* You can determine the number of CPU units that are available per EC2
* instance type by multiplying the vCPUs listed for that instance type on
* the Amazon EC2
* Instances detail page by 1,024.
*
*
*
* For example, if you run a single-container task on a single-core instance
* type with 512 CPU units specified for that container, and that is the
* only task running on the container instance, that container could use the
* full 1,024 CPU unit share at any given time. However, if you launched
* another copy of the same task on that container instance, each task would
* be guaranteed a minimum of 512 CPU units when needed, and each container
* could float to higher CPU usage if the other container was not using it,
* but if both tasks were 100% active all of the time, they would be limited
* to 512 CPU units.
*
*
* The Docker daemon on the container instance uses the CPU value to
* calculate the relative CPU share ratios for running containers. For more
* information, see CPU
* share constraint in the Docker documentation. The minimum valid CPU
* share value that the Linux kernel allows is 2; however, the CPU parameter
* is not required, and you can use CPU values below 2 in your container
* definitions. For CPU values below 2 (including null), the behavior varies
* based on your Amazon ECS container agent version:
*
*
* -
*
* Agent versions less than or equal to 1.1.0: Null and zero CPU
* values are passed to Docker as 0, which Docker then converts to 1,024 CPU
* shares. CPU values of 1 are passed to Docker as 1, which the Linux kernel
* converts to 2 CPU shares.
*
*
* -
*
* Agent versions greater than or equal to 1.2.0: Null, zero, and CPU
* values of 1 are passed to Docker as 2.
*
*
*
*
* @param cpu
* The number of cpu
units reserved for the container. A
* container instance has 1,024 cpu
units for every CPU
* core. This parameter specifies the minimum amount of CPU to
* reserve for a container, and containers share unallocated CPU
* units with other containers on the instance with the same ratio as
* their allocated amount. This parameter maps to
* CpuShares
in the Create a container section of the Docker Remote API and the --cpu-shares
option to
* docker
* run.
*
* You can determine the number of CPU units that are available per
* EC2 instance type by multiplying the vCPUs listed for that
* instance type on the Amazon EC2
* Instances detail page by 1,024.
*
*
*
* For example, if you run a single-container task on a single-core
* instance type with 512 CPU units specified for that container, and
* that is the only task running on the container instance, that
* container could use the full 1,024 CPU unit share at any given
* time. However, if you launched another copy of the same task on
* that container instance, each task would be guaranteed a minimum
* of 512 CPU units when needed, and each container could float to
* higher CPU usage if the other container was not using it, but if
* both tasks were 100% active all of the time, they would be limited
* to 512 CPU units.
*
*
* The Docker daemon on the container instance uses the CPU value to
* calculate the relative CPU share ratios for running containers.
* For more information, see CPU
* share constraint in the Docker documentation. The minimum
* valid CPU share value that the Linux kernel allows is 2; however,
* the CPU parameter is not required, and you can use CPU values
* below 2 in your container definitions. For CPU values below 2
* (including null), the behavior varies based on your Amazon ECS
* container agent version:
*
*
* -
*
* Agent versions less than or equal to 1.1.0: Null and zero
* CPU values are passed to Docker as 0, which Docker then converts
* to 1,024 CPU shares. CPU values of 1 are passed to Docker as 1,
* which the Linux kernel converts to 2 CPU shares.
*
*
* -
*
* Agent versions greater than or equal to 1.2.0: Null, zero,
* and CPU values of 1 are passed to Docker as 2.
*
*
*/
public void setCpu(Integer cpu) {
this.cpu = cpu;
}
/**
*
* The number of cpu
units reserved for the container. A
* container instance has 1,024 cpu
units for every CPU core.
* This parameter specifies the minimum amount of CPU to reserve for a
* container, and containers share unallocated CPU units with other
* containers on the instance with the same ratio as their allocated amount.
* This parameter maps to CpuShares
in the Create a container section of the Docker Remote API and the --cpu-shares
option to docker run.
*
*
*
* You can determine the number of CPU units that are available per EC2
* instance type by multiplying the vCPUs listed for that instance type on
* the Amazon EC2
* Instances detail page by 1,024.
*
*
*
* For example, if you run a single-container task on a single-core instance
* type with 512 CPU units specified for that container, and that is the
* only task running on the container instance, that container could use the
* full 1,024 CPU unit share at any given time. However, if you launched
* another copy of the same task on that container instance, each task would
* be guaranteed a minimum of 512 CPU units when needed, and each container
* could float to higher CPU usage if the other container was not using it,
* but if both tasks were 100% active all of the time, they would be limited
* to 512 CPU units.
*
*
* The Docker daemon on the container instance uses the CPU value to
* calculate the relative CPU share ratios for running containers. For more
* information, see CPU
* share constraint in the Docker documentation. The minimum valid CPU
* share value that the Linux kernel allows is 2; however, the CPU parameter
* is not required, and you can use CPU values below 2 in your container
* definitions. For CPU values below 2 (including null), the behavior varies
* based on your Amazon ECS container agent version:
*
*
* -
*
* Agent versions less than or equal to 1.1.0: Null and zero CPU
* values are passed to Docker as 0, which Docker then converts to 1,024 CPU
* shares. CPU values of 1 are passed to Docker as 1, which the Linux kernel
* converts to 2 CPU shares.
*
*
* -
*
* Agent versions greater than or equal to 1.2.0: Null, zero, and CPU
* values of 1 are passed to Docker as 2.
*
*
*
*
* @return The number of cpu
units reserved for the container.
* A container instance has 1,024 cpu
units for every
* CPU core. This parameter specifies the minimum amount of CPU to
* reserve for a container, and containers share unallocated CPU
* units with other containers on the instance with the same ratio
* as their allocated amount. This parameter maps to
* CpuShares
in the Create a container section of the Docker Remote API and the --cpu-shares
option
* to
* docker run.
*
* You can determine the number of CPU units that are available per
* EC2 instance type by multiplying the vCPUs listed for that
* instance type on the Amazon EC2
* Instances detail page by 1,024.
*
*
*
* For example, if you run a single-container task on a single-core
* instance type with 512 CPU units specified for that container,
* and that is the only task running on the container instance, that
* container could use the full 1,024 CPU unit share at any given
* time. However, if you launched another copy of the same task on
* that container instance, each task would be guaranteed a minimum
* of 512 CPU units when needed, and each container could float to
* higher CPU usage if the other container was not using it, but if
* both tasks were 100% active all of the time, they would be
* limited to 512 CPU units.
*
*
* The Docker daemon on the container instance uses the CPU value to
* calculate the relative CPU share ratios for running containers.
* For more information, see CPU
* share constraint in the Docker documentation. The minimum
* valid CPU share value that the Linux kernel allows is 2; however,
* the CPU parameter is not required, and you can use CPU values
* below 2 in your container definitions. For CPU values below 2
* (including null), the behavior varies based on your Amazon ECS
* container agent version:
*
*
* -
*
* Agent versions less than or equal to 1.1.0: Null and zero
* CPU values are passed to Docker as 0, which Docker then converts
* to 1,024 CPU shares. CPU values of 1 are passed to Docker as 1,
* which the Linux kernel converts to 2 CPU shares.
*
*
* -
*
* Agent versions greater than or equal to 1.2.0: Null, zero,
* and CPU values of 1 are passed to Docker as 2.
*
*
*/
public Integer getCpu() {
return this.cpu;
}
/**
*
* The number of cpu
units reserved for the container. A
* container instance has 1,024 cpu
units for every CPU core.
* This parameter specifies the minimum amount of CPU to reserve for a
* container, and containers share unallocated CPU units with other
* containers on the instance with the same ratio as their allocated amount.
* This parameter maps to CpuShares
in the Create a container section of the Docker Remote API and the --cpu-shares
option to docker run.
*
*
*
* You can determine the number of CPU units that are available per EC2
* instance type by multiplying the vCPUs listed for that instance type on
* the Amazon EC2
* Instances detail page by 1,024.
*
*
*
* For example, if you run a single-container task on a single-core instance
* type with 512 CPU units specified for that container, and that is the
* only task running on the container instance, that container could use the
* full 1,024 CPU unit share at any given time. However, if you launched
* another copy of the same task on that container instance, each task would
* be guaranteed a minimum of 512 CPU units when needed, and each container
* could float to higher CPU usage if the other container was not using it,
* but if both tasks were 100% active all of the time, they would be limited
* to 512 CPU units.
*
*
* The Docker daemon on the container instance uses the CPU value to
* calculate the relative CPU share ratios for running containers. For more
* information, see CPU
* share constraint in the Docker documentation. The minimum valid CPU
* share value that the Linux kernel allows is 2; however, the CPU parameter
* is not required, and you can use CPU values below 2 in your container
* definitions. For CPU values below 2 (including null), the behavior varies
* based on your Amazon ECS container agent version:
*
*
* -
*
* Agent versions less than or equal to 1.1.0: Null and zero CPU
* values are passed to Docker as 0, which Docker then converts to 1,024 CPU
* shares. CPU values of 1 are passed to Docker as 1, which the Linux kernel
* converts to 2 CPU shares.
*
*
* -
*
* Agent versions greater than or equal to 1.2.0: Null, zero, and CPU
* values of 1 are passed to Docker as 2.
*
*
*
*
* @param cpu
* The number of cpu
units reserved for the container. A
* container instance has 1,024 cpu
units for every CPU
* core. This parameter specifies the minimum amount of CPU to
* reserve for a container, and containers share unallocated CPU
* units with other containers on the instance with the same ratio as
* their allocated amount. This parameter maps to
* CpuShares
in the Create a container section of the Docker Remote API and the --cpu-shares
option to
* docker
* run.
*
* You can determine the number of CPU units that are available per
* EC2 instance type by multiplying the vCPUs listed for that
* instance type on the Amazon EC2
* Instances detail page by 1,024.
*
*
*
* For example, if you run a single-container task on a single-core
* instance type with 512 CPU units specified for that container, and
* that is the only task running on the container instance, that
* container could use the full 1,024 CPU unit share at any given
* time. However, if you launched another copy of the same task on
* that container instance, each task would be guaranteed a minimum
* of 512 CPU units when needed, and each container could float to
* higher CPU usage if the other container was not using it, but if
* both tasks were 100% active all of the time, they would be limited
* to 512 CPU units.
*
*
* The Docker daemon on the container instance uses the CPU value to
* calculate the relative CPU share ratios for running containers.
* For more information, see CPU
* share constraint in the Docker documentation. The minimum
* valid CPU share value that the Linux kernel allows is 2; however,
* the CPU parameter is not required, and you can use CPU values
* below 2 in your container definitions. For CPU values below 2
* (including null), the behavior varies based on your Amazon ECS
* container agent version:
*
*
* -
*
* Agent versions less than or equal to 1.1.0: Null and zero
* CPU values are passed to Docker as 0, which Docker then converts
* to 1,024 CPU shares. CPU values of 1 are passed to Docker as 1,
* which the Linux kernel converts to 2 CPU shares.
*
*
* -
*
* Agent versions greater than or equal to 1.2.0: Null, zero,
* and CPU values of 1 are passed to Docker as 2.
*
*
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withCpu(Integer cpu) {
setCpu(cpu);
return this;
}
/**
*
* The number of MiB of memory to reserve for the container. You must
* specify a non-zero integer for this parameter; the Docker daemon reserves
* a minimum of 4 MiB of memory for a container, so you should not specify
* fewer than 4 MiB of memory for your containers. If your container
* attempts to exceed the memory allocated here, the container is killed.
* This parameter maps to Memory
in the Create a container section of the Docker Remote API and the --memory
option to docker run.
*
*
* @param memory
* The number of MiB of memory to reserve for the container. You must
* specify a non-zero integer for this parameter; the Docker daemon
* reserves a minimum of 4 MiB of memory for a container, so you
* should not specify fewer than 4 MiB of memory for your containers.
* If your container attempts to exceed the memory allocated here,
* the container is killed. This parameter maps to
* Memory
in the Create a container section of the Docker Remote API and the --memory
option to docker
* run.
*/
public void setMemory(Integer memory) {
this.memory = memory;
}
/**
*
* The number of MiB of memory to reserve for the container. You must
* specify a non-zero integer for this parameter; the Docker daemon reserves
* a minimum of 4 MiB of memory for a container, so you should not specify
* fewer than 4 MiB of memory for your containers. If your container
* attempts to exceed the memory allocated here, the container is killed.
* This parameter maps to Memory
in the Create a container section of the Docker Remote API and the --memory
option to docker run.
*
*
* @return The number of MiB of memory to reserve for the container. You
* must specify a non-zero integer for this parameter; the Docker
* daemon reserves a minimum of 4 MiB of memory for a container, so
* you should not specify fewer than 4 MiB of memory for your
* containers. If your container attempts to exceed the memory
* allocated here, the container is killed. This parameter maps to
* Memory
in the Create a container section of the Docker Remote API and the --memory
option to docker
* run.
*/
public Integer getMemory() {
return this.memory;
}
/**
*
* The number of MiB of memory to reserve for the container. You must
* specify a non-zero integer for this parameter; the Docker daemon reserves
* a minimum of 4 MiB of memory for a container, so you should not specify
* fewer than 4 MiB of memory for your containers. If your container
* attempts to exceed the memory allocated here, the container is killed.
* This parameter maps to Memory
in the Create a container section of the Docker Remote API and the --memory
option to docker run.
*
*
* @param memory
* The number of MiB of memory to reserve for the container. You must
* specify a non-zero integer for this parameter; the Docker daemon
* reserves a minimum of 4 MiB of memory for a container, so you
* should not specify fewer than 4 MiB of memory for your containers.
* If your container attempts to exceed the memory allocated here,
* the container is killed. This parameter maps to
* Memory
in the Create a container section of the Docker Remote API and the --memory
option to docker
* run.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withMemory(Integer memory) {
setMemory(memory);
return this;
}
/**
*
* The link
parameter allows containers to communicate with
* each other without the need for port mappings, using the
* name
parameter and optionally, an alias
for the
* link. This construct is analogous to name:alias
in Docker
* links. Up to 255 letters (uppercase and lowercase), numbers, hyphens, and
* underscores are allowed for each name
and alias
* . For more information on linking Docker containers, see https://docs.docker.com/userguide/dockerlinks/. This parameter maps
* to Links
in the Create a container section of the Docker Remote API and the --link
option to
* docker run
.
*
*
*
* Containers that are collocated on a single container instance may be able
* to communicate with each other without requiring links or host port
* mappings. Network isolation is achieved on the container instance using
* security groups and VPC settings.
*
*
*
* @return The link
parameter allows containers to communicate
* with each other without the need for port mappings, using the
* name
parameter and optionally, an alias
* for the link. This construct is analogous to
* name:alias
in Docker links. Up to 255 letters
* (uppercase and lowercase), numbers, hyphens, and underscores are
* allowed for each name
and alias
. For
* more information on linking Docker containers, see https://docs.docker.com/userguide/dockerlinks/. This
* parameter maps to Links
in the Create a container section of the Docker Remote API and the --link
option to
* docker run
.
*
* Containers that are collocated on a single container instance may
* be able to communicate with each other without requiring links or
* host port mappings. Network isolation is achieved on the
* container instance using security groups and VPC settings.
*
*/
public java.util.List getLinks() {
if (links == null) {
links = new com.amazonaws.internal.SdkInternalList();
}
return links;
}
/**
*
* The link
parameter allows containers to communicate with
* each other without the need for port mappings, using the
* name
parameter and optionally, an alias
for the
* link. This construct is analogous to name:alias
in Docker
* links. Up to 255 letters (uppercase and lowercase), numbers, hyphens, and
* underscores are allowed for each name
and alias
* . For more information on linking Docker containers, see https://docs.docker.com/userguide/dockerlinks/. This parameter maps
* to Links
in the Create a container section of the Docker Remote API and the --link
option to
* docker run
.
*
*
*
* Containers that are collocated on a single container instance may be able
* to communicate with each other without requiring links or host port
* mappings. Network isolation is achieved on the container instance using
* security groups and VPC settings.
*
*
*
* @param links
* The link
parameter allows containers to communicate
* with each other without the need for port mappings, using the
* name
parameter and optionally, an alias
* for the link. This construct is analogous to
* name:alias
in Docker links. Up to 255 letters
* (uppercase and lowercase), numbers, hyphens, and underscores are
* allowed for each name
and alias
. For
* more information on linking Docker containers, see https://docs.docker.com/userguide/dockerlinks/. This
* parameter maps to Links
in the Create a container section of the Docker Remote API and the --link
option to
* docker run
.
*
* Containers that are collocated on a single container instance may
* be able to communicate with each other without requiring links or
* host port mappings. Network isolation is achieved on the container
* instance using security groups and VPC settings.
*
*/
public void setLinks(java.util.Collection links) {
if (links == null) {
this.links = null;
return;
}
this.links = new com.amazonaws.internal.SdkInternalList(links);
}
/**
*
* The link
parameter allows containers to communicate with
* each other without the need for port mappings, using the
* name
parameter and optionally, an alias
for the
* link. This construct is analogous to name:alias
in Docker
* links. Up to 255 letters (uppercase and lowercase), numbers, hyphens, and
* underscores are allowed for each name
and alias
* . For more information on linking Docker containers, see https://docs.docker.com/userguide/dockerlinks/. This parameter maps
* to Links
in the Create a container section of the Docker Remote API and the --link
option to
* docker run
.
*
*
*
* Containers that are collocated on a single container instance may be able
* to communicate with each other without requiring links or host port
* mappings. Network isolation is achieved on the container instance using
* security groups and VPC settings.
*
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setLinks(java.util.Collection)} or
* {@link #withLinks(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param links
* The link
parameter allows containers to communicate
* with each other without the need for port mappings, using the
* name
parameter and optionally, an alias
* for the link. This construct is analogous to
* name:alias
in Docker links. Up to 255 letters
* (uppercase and lowercase), numbers, hyphens, and underscores are
* allowed for each name
and alias
. For
* more information on linking Docker containers, see https://docs.docker.com/userguide/dockerlinks/. This
* parameter maps to Links
in the Create a container section of the Docker Remote API and the --link
option to
* docker run
.
*
* Containers that are collocated on a single container instance may
* be able to communicate with each other without requiring links or
* host port mappings. Network isolation is achieved on the container
* instance using security groups and VPC settings.
*
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withLinks(String... links) {
if (this.links == null) {
setLinks(new com.amazonaws.internal.SdkInternalList(
links.length));
}
for (String ele : links) {
this.links.add(ele);
}
return this;
}
/**
*
* The link
parameter allows containers to communicate with
* each other without the need for port mappings, using the
* name
parameter and optionally, an alias
for the
* link. This construct is analogous to name:alias
in Docker
* links. Up to 255 letters (uppercase and lowercase), numbers, hyphens, and
* underscores are allowed for each name
and alias
* . For more information on linking Docker containers, see https://docs.docker.com/userguide/dockerlinks/. This parameter maps
* to Links
in the Create a container section of the Docker Remote API and the --link
option to
* docker run
.
*
*
*
* Containers that are collocated on a single container instance may be able
* to communicate with each other without requiring links or host port
* mappings. Network isolation is achieved on the container instance using
* security groups and VPC settings.
*
*
*
* @param links
* The link
parameter allows containers to communicate
* with each other without the need for port mappings, using the
* name
parameter and optionally, an alias
* for the link. This construct is analogous to
* name:alias
in Docker links. Up to 255 letters
* (uppercase and lowercase), numbers, hyphens, and underscores are
* allowed for each name
and alias
. For
* more information on linking Docker containers, see https://docs.docker.com/userguide/dockerlinks/. This
* parameter maps to Links
in the Create a container section of the Docker Remote API and the --link
option to
* docker run
.
*
* Containers that are collocated on a single container instance may
* be able to communicate with each other without requiring links or
* host port mappings. Network isolation is achieved on the container
* instance using security groups and VPC settings.
*
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withLinks(java.util.Collection links) {
setLinks(links);
return this;
}
/**
*
* The list of port mappings for the container. Port mappings allow
* containers to access ports on the host container instance to send or
* receive traffic. This parameter maps to PortBindings
in the
* Create a container section of the Docker Remote API and the --publish
option to docker run.
*
*
*
* After a task reaches the RUNNING
status, manual and
* automatic host and container port assignments are visible in the
* Network Bindings section of a container description of a selected
* task in the Amazon ECS console, or the networkBindings
* section DescribeTasks responses.
*
*
*
* @return The list of port mappings for the container. Port mappings allow
* containers to access ports on the host container instance to send
* or receive traffic. This parameter maps to
* PortBindings
in the Create a container section of the Docker Remote API and the --publish
option to
*
* docker run.
*
* After a task reaches the RUNNING
status, manual and
* automatic host and container port assignments are visible in the
* Network Bindings section of a container description of a
* selected task in the Amazon ECS console, or the
* networkBindings
section DescribeTasks
* responses.
*
*/
public java.util.List getPortMappings() {
if (portMappings == null) {
portMappings = new com.amazonaws.internal.SdkInternalList();
}
return portMappings;
}
/**
*
* The list of port mappings for the container. Port mappings allow
* containers to access ports on the host container instance to send or
* receive traffic. This parameter maps to PortBindings
in the
* Create a container section of the Docker Remote API and the --publish
option to docker run.
*
*
*
* After a task reaches the RUNNING
status, manual and
* automatic host and container port assignments are visible in the
* Network Bindings section of a container description of a selected
* task in the Amazon ECS console, or the networkBindings
* section DescribeTasks responses.
*
*
*
* @param portMappings
* The list of port mappings for the container. Port mappings allow
* containers to access ports on the host container instance to send
* or receive traffic. This parameter maps to
* PortBindings
in the Create a container section of the Docker Remote API and the --publish
option to docker
* run.
*
* After a task reaches the RUNNING
status, manual and
* automatic host and container port assignments are visible in the
* Network Bindings section of a container description of a
* selected task in the Amazon ECS console, or the
* networkBindings
section DescribeTasks
* responses.
*
*/
public void setPortMappings(java.util.Collection portMappings) {
if (portMappings == null) {
this.portMappings = null;
return;
}
this.portMappings = new com.amazonaws.internal.SdkInternalList(
portMappings);
}
/**
*
* The list of port mappings for the container. Port mappings allow
* containers to access ports on the host container instance to send or
* receive traffic. This parameter maps to PortBindings
in the
* Create a container section of the Docker Remote API and the --publish
option to docker run.
*
*
*
* After a task reaches the RUNNING
status, manual and
* automatic host and container port assignments are visible in the
* Network Bindings section of a container description of a selected
* task in the Amazon ECS console, or the networkBindings
* section DescribeTasks responses.
*
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setPortMappings(java.util.Collection)} or
* {@link #withPortMappings(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param portMappings
* The list of port mappings for the container. Port mappings allow
* containers to access ports on the host container instance to send
* or receive traffic. This parameter maps to
* PortBindings
in the Create a container section of the Docker Remote API and the --publish
option to docker
* run.
*
* After a task reaches the RUNNING
status, manual and
* automatic host and container port assignments are visible in the
* Network Bindings section of a container description of a
* selected task in the Amazon ECS console, or the
* networkBindings
section DescribeTasks
* responses.
*
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withPortMappings(PortMapping... portMappings) {
if (this.portMappings == null) {
setPortMappings(new com.amazonaws.internal.SdkInternalList(
portMappings.length));
}
for (PortMapping ele : portMappings) {
this.portMappings.add(ele);
}
return this;
}
/**
*
* The list of port mappings for the container. Port mappings allow
* containers to access ports on the host container instance to send or
* receive traffic. This parameter maps to PortBindings
in the
* Create a container section of the Docker Remote API and the --publish
option to docker run.
*
*
*
* After a task reaches the RUNNING
status, manual and
* automatic host and container port assignments are visible in the
* Network Bindings section of a container description of a selected
* task in the Amazon ECS console, or the networkBindings
* section DescribeTasks responses.
*
*
*
* @param portMappings
* The list of port mappings for the container. Port mappings allow
* containers to access ports on the host container instance to send
* or receive traffic. This parameter maps to
* PortBindings
in the Create a container section of the Docker Remote API and the --publish
option to docker
* run.
*
* After a task reaches the RUNNING
status, manual and
* automatic host and container port assignments are visible in the
* Network Bindings section of a container description of a
* selected task in the Amazon ECS console, or the
* networkBindings
section DescribeTasks
* responses.
*
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withPortMappings(
java.util.Collection portMappings) {
setPortMappings(portMappings);
return this;
}
/**
*
* If the essential
parameter of a container is marked as
* true
, and that container fails or stops for any reason, all
* other containers that are part of the task are stopped. If the
* essential
parameter of a container is marked as
* false
, then its failure does not affect the rest of the
* containers in a task. If this parameter is omitted, a container is
* assumed to be essential.
*
*
* All tasks must have at least one essential container. If you have an
* application that is composed of multiple containers, you should group
* containers that are used for a common purpose into components, and
* separate the different components into multiple task definitions. For
* more information, see Application Architecture in the Amazon EC2 Container Service
* Developer Guide.
*
*
* @param essential
* If the essential
parameter of a container is marked
* as true
, and that container fails or stops for any
* reason, all other containers that are part of the task are
* stopped. If the essential
parameter of a container is
* marked as false
, then its failure does not affect the
* rest of the containers in a task. If this parameter is omitted, a
* container is assumed to be essential.
*
* All tasks must have at least one essential container. If you have
* an application that is composed of multiple containers, you should
* group containers that are used for a common purpose into
* components, and separate the different components into multiple
* task definitions. For more information, see Application Architecture in the Amazon EC2 Container
* Service Developer Guide.
*/
public void setEssential(Boolean essential) {
this.essential = essential;
}
/**
*
* If the essential
parameter of a container is marked as
* true
, and that container fails or stops for any reason, all
* other containers that are part of the task are stopped. If the
* essential
parameter of a container is marked as
* false
, then its failure does not affect the rest of the
* containers in a task. If this parameter is omitted, a container is
* assumed to be essential.
*
*
* All tasks must have at least one essential container. If you have an
* application that is composed of multiple containers, you should group
* containers that are used for a common purpose into components, and
* separate the different components into multiple task definitions. For
* more information, see Application Architecture in the Amazon EC2 Container Service
* Developer Guide.
*
*
* @return If the essential
parameter of a container is marked
* as true
, and that container fails or stops for any
* reason, all other containers that are part of the task are
* stopped. If the essential
parameter of a container
* is marked as false
, then its failure does not affect
* the rest of the containers in a task. If this parameter is
* omitted, a container is assumed to be essential.
*
* All tasks must have at least one essential container. If you have
* an application that is composed of multiple containers, you
* should group containers that are used for a common purpose into
* components, and separate the different components into multiple
* task definitions. For more information, see Application Architecture in the Amazon EC2 Container
* Service Developer Guide.
*/
public Boolean getEssential() {
return this.essential;
}
/**
*
* If the essential
parameter of a container is marked as
* true
, and that container fails or stops for any reason, all
* other containers that are part of the task are stopped. If the
* essential
parameter of a container is marked as
* false
, then its failure does not affect the rest of the
* containers in a task. If this parameter is omitted, a container is
* assumed to be essential.
*
*
* All tasks must have at least one essential container. If you have an
* application that is composed of multiple containers, you should group
* containers that are used for a common purpose into components, and
* separate the different components into multiple task definitions. For
* more information, see Application Architecture in the Amazon EC2 Container Service
* Developer Guide.
*
*
* @param essential
* If the essential
parameter of a container is marked
* as true
, and that container fails or stops for any
* reason, all other containers that are part of the task are
* stopped. If the essential
parameter of a container is
* marked as false
, then its failure does not affect the
* rest of the containers in a task. If this parameter is omitted, a
* container is assumed to be essential.
*
* All tasks must have at least one essential container. If you have
* an application that is composed of multiple containers, you should
* group containers that are used for a common purpose into
* components, and separate the different components into multiple
* task definitions. For more information, see Application Architecture in the Amazon EC2 Container
* Service Developer Guide.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withEssential(Boolean essential) {
setEssential(essential);
return this;
}
/**
*
* If the essential
parameter of a container is marked as
* true
, and that container fails or stops for any reason, all
* other containers that are part of the task are stopped. If the
* essential
parameter of a container is marked as
* false
, then its failure does not affect the rest of the
* containers in a task. If this parameter is omitted, a container is
* assumed to be essential.
*
*
* All tasks must have at least one essential container. If you have an
* application that is composed of multiple containers, you should group
* containers that are used for a common purpose into components, and
* separate the different components into multiple task definitions. For
* more information, see Application Architecture in the Amazon EC2 Container Service
* Developer Guide.
*
*
* @return If the essential
parameter of a container is marked
* as true
, and that container fails or stops for any
* reason, all other containers that are part of the task are
* stopped. If the essential
parameter of a container
* is marked as false
, then its failure does not affect
* the rest of the containers in a task. If this parameter is
* omitted, a container is assumed to be essential.
*
* All tasks must have at least one essential container. If you have
* an application that is composed of multiple containers, you
* should group containers that are used for a common purpose into
* components, and separate the different components into multiple
* task definitions. For more information, see Application Architecture in the Amazon EC2 Container
* Service Developer Guide.
*/
public Boolean isEssential() {
return this.essential;
}
/**
*
*
* Early versions of the Amazon ECS container agent do not properly handle
* entryPoint
parameters. If you have problems using
* entryPoint
, update your container agent or enter your
* commands and arguments as command
array items instead.
*
*
*
* The entry point that is passed to the container. This parameter maps to
* Entrypoint
in the Create a container section of the Docker Remote API and the --entrypoint
option to docker run.
* For more information, see https://docs.docker.com/reference/builder/#entrypoint.
*
*
* @return
* Early versions of the Amazon ECS container agent do not properly
* handle entryPoint
parameters. If you have problems
* using entryPoint
, update your container agent or
* enter your commands and arguments as command
array
* items instead.
*
*
*
* The entry point that is passed to the container. This parameter
* maps to Entrypoint
in the Create a container section of the Docker Remote API and the --entrypoint
option
* to
* docker run. For more information, see https://docs.docker.com/reference/builder/#entrypoint.
*/
public java.util.List getEntryPoint() {
if (entryPoint == null) {
entryPoint = new com.amazonaws.internal.SdkInternalList();
}
return entryPoint;
}
/**
*
*
* Early versions of the Amazon ECS container agent do not properly handle
* entryPoint
parameters. If you have problems using
* entryPoint
, update your container agent or enter your
* commands and arguments as command
array items instead.
*
*
*
* The entry point that is passed to the container. This parameter maps to
* Entrypoint
in the Create a container section of the Docker Remote API and the --entrypoint
option to docker run.
* For more information, see https://docs.docker.com/reference/builder/#entrypoint.
*
*
* @param entryPoint
*
* Early versions of the Amazon ECS container agent do not properly
* handle entryPoint
parameters. If you have problems
* using entryPoint
, update your container agent or
* enter your commands and arguments as command
array
* items instead.
*
*
*
* The entry point that is passed to the container. This parameter
* maps to Entrypoint
in the Create a container section of the Docker Remote API and the --entrypoint
option to
* docker
* run. For more information, see https://docs.docker.com/reference/builder/#entrypoint.
*/
public void setEntryPoint(java.util.Collection entryPoint) {
if (entryPoint == null) {
this.entryPoint = null;
return;
}
this.entryPoint = new com.amazonaws.internal.SdkInternalList(
entryPoint);
}
/**
*
*
* Early versions of the Amazon ECS container agent do not properly handle
* entryPoint
parameters. If you have problems using
* entryPoint
, update your container agent or enter your
* commands and arguments as command
array items instead.
*
*
*
* The entry point that is passed to the container. This parameter maps to
* Entrypoint
in the Create a container section of the Docker Remote API and the --entrypoint
option to docker run.
* For more information, see https://docs.docker.com/reference/builder/#entrypoint.
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setEntryPoint(java.util.Collection)} or
* {@link #withEntryPoint(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param entryPoint
*
* Early versions of the Amazon ECS container agent do not properly
* handle entryPoint
parameters. If you have problems
* using entryPoint
, update your container agent or
* enter your commands and arguments as command
array
* items instead.
*
*
*
* The entry point that is passed to the container. This parameter
* maps to Entrypoint
in the Create a container section of the Docker Remote API and the --entrypoint
option to
* docker
* run. For more information, see https://docs.docker.com/reference/builder/#entrypoint.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withEntryPoint(String... entryPoint) {
if (this.entryPoint == null) {
setEntryPoint(new com.amazonaws.internal.SdkInternalList(
entryPoint.length));
}
for (String ele : entryPoint) {
this.entryPoint.add(ele);
}
return this;
}
/**
*
*
* Early versions of the Amazon ECS container agent do not properly handle
* entryPoint
parameters. If you have problems using
* entryPoint
, update your container agent or enter your
* commands and arguments as command
array items instead.
*
*
*
* The entry point that is passed to the container. This parameter maps to
* Entrypoint
in the Create a container section of the Docker Remote API and the --entrypoint
option to docker run.
* For more information, see https://docs.docker.com/reference/builder/#entrypoint.
*
*
* @param entryPoint
*
* Early versions of the Amazon ECS container agent do not properly
* handle entryPoint
parameters. If you have problems
* using entryPoint
, update your container agent or
* enter your commands and arguments as command
array
* items instead.
*
*
*
* The entry point that is passed to the container. This parameter
* maps to Entrypoint
in the Create a container section of the Docker Remote API and the --entrypoint
option to
* docker
* run. For more information, see https://docs.docker.com/reference/builder/#entrypoint.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withEntryPoint(
java.util.Collection entryPoint) {
setEntryPoint(entryPoint);
return this;
}
/**
*
* The command that is passed to the container. This parameter maps to
* Cmd
in the Create a container section of the Docker Remote API and the COMMAND
parameter to docker run.
* For more information, see https://docs.docker.com/reference/builder/#cmd.
*
*
* @return The command that is passed to the container. This parameter maps
* to Cmd
in the Create a container section of the Docker Remote API and the COMMAND
parameter to
*
* docker run. For more information, see https://docs.docker.com/reference/builder/#cmd.
*/
public java.util.List getCommand() {
if (command == null) {
command = new com.amazonaws.internal.SdkInternalList();
}
return command;
}
/**
*
* The command that is passed to the container. This parameter maps to
* Cmd
in the Create a container section of the Docker Remote API and the COMMAND
parameter to docker run.
* For more information, see https://docs.docker.com/reference/builder/#cmd.
*
*
* @param command
* The command that is passed to the container. This parameter maps
* to Cmd
in the Create a container section of the Docker Remote API and the COMMAND
parameter to
* docker
* run. For more information, see https://docs.docker.com/reference/builder/#cmd.
*/
public void setCommand(java.util.Collection command) {
if (command == null) {
this.command = null;
return;
}
this.command = new com.amazonaws.internal.SdkInternalList(
command);
}
/**
*
* The command that is passed to the container. This parameter maps to
* Cmd
in the Create a container section of the Docker Remote API and the COMMAND
parameter to docker run.
* For more information, see https://docs.docker.com/reference/builder/#cmd.
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setCommand(java.util.Collection)} or
* {@link #withCommand(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param command
* The command that is passed to the container. This parameter maps
* to Cmd
in the Create a container section of the Docker Remote API and the COMMAND
parameter to
* docker
* run. For more information, see https://docs.docker.com/reference/builder/#cmd.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withCommand(String... command) {
if (this.command == null) {
setCommand(new com.amazonaws.internal.SdkInternalList(
command.length));
}
for (String ele : command) {
this.command.add(ele);
}
return this;
}
/**
*
* The command that is passed to the container. This parameter maps to
* Cmd
in the Create a container section of the Docker Remote API and the COMMAND
parameter to docker run.
* For more information, see https://docs.docker.com/reference/builder/#cmd.
*
*
* @param command
* The command that is passed to the container. This parameter maps
* to Cmd
in the Create a container section of the Docker Remote API and the COMMAND
parameter to
* docker
* run. For more information, see https://docs.docker.com/reference/builder/#cmd.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withCommand(java.util.Collection command) {
setCommand(command);
return this;
}
/**
*
* The environment variables to pass to a container. This parameter maps to
* Env
in the Create a container section of the Docker Remote API and the --env
option to docker run.
*
*
*
* We do not recommend using plain text environment variables for sensitive
* information, such as credential data.
*
*
*
* @return The environment variables to pass to a container. This parameter
* maps to Env
in the Create a container section of the Docker Remote API and the --env
option to docker
* run.
*
* We do not recommend using plain text environment variables for
* sensitive information, such as credential data.
*
*/
public java.util.List getEnvironment() {
if (environment == null) {
environment = new com.amazonaws.internal.SdkInternalList();
}
return environment;
}
/**
*
* The environment variables to pass to a container. This parameter maps to
* Env
in the Create a container section of the Docker Remote API and the --env
option to docker run.
*
*
*
* We do not recommend using plain text environment variables for sensitive
* information, such as credential data.
*
*
*
* @param environment
* The environment variables to pass to a container. This parameter
* maps to Env
in the Create a container section of the Docker Remote API and the --env
option to docker
* run.
*
* We do not recommend using plain text environment variables for
* sensitive information, such as credential data.
*
*/
public void setEnvironment(java.util.Collection environment) {
if (environment == null) {
this.environment = null;
return;
}
this.environment = new com.amazonaws.internal.SdkInternalList(
environment);
}
/**
*
* The environment variables to pass to a container. This parameter maps to
* Env
in the Create a container section of the Docker Remote API and the --env
option to docker run.
*
*
*
* We do not recommend using plain text environment variables for sensitive
* information, such as credential data.
*
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setEnvironment(java.util.Collection)} or
* {@link #withEnvironment(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param environment
* The environment variables to pass to a container. This parameter
* maps to Env
in the Create a container section of the Docker Remote API and the --env
option to docker
* run.
*
* We do not recommend using plain text environment variables for
* sensitive information, such as credential data.
*
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withEnvironment(KeyValuePair... environment) {
if (this.environment == null) {
setEnvironment(new com.amazonaws.internal.SdkInternalList(
environment.length));
}
for (KeyValuePair ele : environment) {
this.environment.add(ele);
}
return this;
}
/**
*
* The environment variables to pass to a container. This parameter maps to
* Env
in the Create a container section of the Docker Remote API and the --env
option to docker run.
*
*
*
* We do not recommend using plain text environment variables for sensitive
* information, such as credential data.
*
*
*
* @param environment
* The environment variables to pass to a container. This parameter
* maps to Env
in the Create a container section of the Docker Remote API and the --env
option to docker
* run.
*
* We do not recommend using plain text environment variables for
* sensitive information, such as credential data.
*
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withEnvironment(
java.util.Collection environment) {
setEnvironment(environment);
return this;
}
/**
*
* The mount points for data volumes in your container. This parameter maps
* to Volumes
in the Create a container section of the Docker Remote API and the --volume
option to docker run.
*
*
* @return The mount points for data volumes in your container. This
* parameter maps to Volumes
in the Create a container section of the Docker Remote API and the --volume
option to docker
* run.
*/
public java.util.List getMountPoints() {
if (mountPoints == null) {
mountPoints = new com.amazonaws.internal.SdkInternalList();
}
return mountPoints;
}
/**
*
* The mount points for data volumes in your container. This parameter maps
* to Volumes
in the Create a container section of the Docker Remote API and the --volume
option to docker run.
*
*
* @param mountPoints
* The mount points for data volumes in your container. This
* parameter maps to Volumes
in the Create a container section of the Docker Remote API and the --volume
option to docker
* run.
*/
public void setMountPoints(java.util.Collection mountPoints) {
if (mountPoints == null) {
this.mountPoints = null;
return;
}
this.mountPoints = new com.amazonaws.internal.SdkInternalList(
mountPoints);
}
/**
*
* The mount points for data volumes in your container. This parameter maps
* to Volumes
in the Create a container section of the Docker Remote API and the --volume
option to docker run.
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setMountPoints(java.util.Collection)} or
* {@link #withMountPoints(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param mountPoints
* The mount points for data volumes in your container. This
* parameter maps to Volumes
in the Create a container section of the Docker Remote API and the --volume
option to docker
* run.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withMountPoints(MountPoint... mountPoints) {
if (this.mountPoints == null) {
setMountPoints(new com.amazonaws.internal.SdkInternalList(
mountPoints.length));
}
for (MountPoint ele : mountPoints) {
this.mountPoints.add(ele);
}
return this;
}
/**
*
* The mount points for data volumes in your container. This parameter maps
* to Volumes
in the Create a container section of the Docker Remote API and the --volume
option to docker run.
*
*
* @param mountPoints
* The mount points for data volumes in your container. This
* parameter maps to Volumes
in the Create a container section of the Docker Remote API and the --volume
option to docker
* run.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withMountPoints(
java.util.Collection mountPoints) {
setMountPoints(mountPoints);
return this;
}
/**
*
* Data volumes to mount from another container. This parameter maps to
* VolumesFrom
in the Create a container section of the Docker Remote API and the --volumes-from
option to docker run.
*
*
* @return Data volumes to mount from another container. This parameter maps
* to VolumesFrom
in the Create a container section of the Docker Remote API and the --volumes-from
option
* to
* docker run.
*/
public java.util.List getVolumesFrom() {
if (volumesFrom == null) {
volumesFrom = new com.amazonaws.internal.SdkInternalList();
}
return volumesFrom;
}
/**
*
* Data volumes to mount from another container. This parameter maps to
* VolumesFrom
in the Create a container section of the Docker Remote API and the --volumes-from
option to docker run.
*
*
* @param volumesFrom
* Data volumes to mount from another container. This parameter maps
* to VolumesFrom
in the Create a container section of the Docker Remote API and the --volumes-from
option
* to docker
* run.
*/
public void setVolumesFrom(java.util.Collection volumesFrom) {
if (volumesFrom == null) {
this.volumesFrom = null;
return;
}
this.volumesFrom = new com.amazonaws.internal.SdkInternalList(
volumesFrom);
}
/**
*
* Data volumes to mount from another container. This parameter maps to
* VolumesFrom
in the Create a container section of the Docker Remote API and the --volumes-from
option to docker run.
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setVolumesFrom(java.util.Collection)} or
* {@link #withVolumesFrom(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param volumesFrom
* Data volumes to mount from another container. This parameter maps
* to VolumesFrom
in the Create a container section of the Docker Remote API and the --volumes-from
option
* to docker
* run.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withVolumesFrom(VolumeFrom... volumesFrom) {
if (this.volumesFrom == null) {
setVolumesFrom(new com.amazonaws.internal.SdkInternalList(
volumesFrom.length));
}
for (VolumeFrom ele : volumesFrom) {
this.volumesFrom.add(ele);
}
return this;
}
/**
*
* Data volumes to mount from another container. This parameter maps to
* VolumesFrom
in the Create a container section of the Docker Remote API and the --volumes-from
option to docker run.
*
*
* @param volumesFrom
* Data volumes to mount from another container. This parameter maps
* to VolumesFrom
in the Create a container section of the Docker Remote API and the --volumes-from
option
* to docker
* run.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withVolumesFrom(
java.util.Collection volumesFrom) {
setVolumesFrom(volumesFrom);
return this;
}
/**
*
* The hostname to use for your container. This parameter maps to
* Hostname
in the Create a container section of the Docker Remote API and the --hostname
option to docker run.
*
*
* @param hostname
* The hostname to use for your container. This parameter maps to
* Hostname
in the Create a container section of the Docker Remote API and the --hostname
option to
* docker
* run.
*/
public void setHostname(String hostname) {
this.hostname = hostname;
}
/**
*
* The hostname to use for your container. This parameter maps to
* Hostname
in the Create a container section of the Docker Remote API and the --hostname
option to docker run.
*
*
* @return The hostname to use for your container. This parameter maps to
* Hostname
in the Create a container section of the Docker Remote API and the --hostname
option to
*
* docker run.
*/
public String getHostname() {
return this.hostname;
}
/**
*
* The hostname to use for your container. This parameter maps to
* Hostname
in the Create a container section of the Docker Remote API and the --hostname
option to docker run.
*
*
* @param hostname
* The hostname to use for your container. This parameter maps to
* Hostname
in the Create a container section of the Docker Remote API and the --hostname
option to
* docker
* run.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withHostname(String hostname) {
setHostname(hostname);
return this;
}
/**
*
* The user name to use inside the container. This parameter maps to
* User
in the Create a container section of the Docker Remote API and the --user
option to docker run.
*
*
* @param user
* The user name to use inside the container. This parameter maps to
* User
in the Create a container section of the Docker Remote API and the --user
option to docker
* run.
*/
public void setUser(String user) {
this.user = user;
}
/**
*
* The user name to use inside the container. This parameter maps to
* User
in the Create a container section of the Docker Remote API and the --user
option to docker run.
*
*
* @return The user name to use inside the container. This parameter maps to
* User
in the Create a container section of the Docker Remote API and the --user
option to docker
* run.
*/
public String getUser() {
return this.user;
}
/**
*
* The user name to use inside the container. This parameter maps to
* User
in the Create a container section of the Docker Remote API and the --user
option to docker run.
*
*
* @param user
* The user name to use inside the container. This parameter maps to
* User
in the Create a container section of the Docker Remote API and the --user
option to docker
* run.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withUser(String user) {
setUser(user);
return this;
}
/**
*
* The working directory in which to run commands inside the container. This
* parameter maps to WorkingDir
in the Create a container section of the Docker Remote API and the --workdir
option to docker run.
*
*
* @param workingDirectory
* The working directory in which to run commands inside the
* container. This parameter maps to WorkingDir
in the
* Create a container section of the Docker Remote API and the --workdir
option to docker
* run.
*/
public void setWorkingDirectory(String workingDirectory) {
this.workingDirectory = workingDirectory;
}
/**
*
* The working directory in which to run commands inside the container. This
* parameter maps to WorkingDir
in the Create a container section of the Docker Remote API and the --workdir
option to docker run.
*
*
* @return The working directory in which to run commands inside the
* container. This parameter maps to WorkingDir
in the
* Create a container section of the Docker Remote API and the --workdir
option to
*
* docker run.
*/
public String getWorkingDirectory() {
return this.workingDirectory;
}
/**
*
* The working directory in which to run commands inside the container. This
* parameter maps to WorkingDir
in the Create a container section of the Docker Remote API and the --workdir
option to docker run.
*
*
* @param workingDirectory
* The working directory in which to run commands inside the
* container. This parameter maps to WorkingDir
in the
* Create a container section of the Docker Remote API and the --workdir
option to docker
* run.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withWorkingDirectory(String workingDirectory) {
setWorkingDirectory(workingDirectory);
return this;
}
/**
*
* When this parameter is true, networking is disabled within the container.
* This parameter maps to NetworkDisabled
in the Create a container section of the Docker Remote API.
*
*
* @param disableNetworking
* When this parameter is true, networking is disabled within the
* container. This parameter maps to NetworkDisabled
in
* the Create a container section of the Docker Remote API.
*/
public void setDisableNetworking(Boolean disableNetworking) {
this.disableNetworking = disableNetworking;
}
/**
*
* When this parameter is true, networking is disabled within the container.
* This parameter maps to NetworkDisabled
in the Create a container section of the Docker Remote API.
*
*
* @return When this parameter is true, networking is disabled within the
* container. This parameter maps to NetworkDisabled
in
* the Create a container section of the Docker Remote API.
*/
public Boolean getDisableNetworking() {
return this.disableNetworking;
}
/**
*
* When this parameter is true, networking is disabled within the container.
* This parameter maps to NetworkDisabled
in the Create a container section of the Docker Remote API.
*
*
* @param disableNetworking
* When this parameter is true, networking is disabled within the
* container. This parameter maps to NetworkDisabled
in
* the Create a container section of the Docker Remote API.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withDisableNetworking(Boolean disableNetworking) {
setDisableNetworking(disableNetworking);
return this;
}
/**
*
* When this parameter is true, networking is disabled within the container.
* This parameter maps to NetworkDisabled
in the Create a container section of the Docker Remote API.
*
*
* @return When this parameter is true, networking is disabled within the
* container. This parameter maps to NetworkDisabled
in
* the Create a container section of the Docker Remote API.
*/
public Boolean isDisableNetworking() {
return this.disableNetworking;
}
/**
*
* When this parameter is true, the container is given elevated privileges
* on the host container instance (similar to the root
user).
* This parameter maps to Privileged
in the Create a container section of the Docker Remote API and the --privileged
option to docker run.
*
*
* @param privileged
* When this parameter is true, the container is given elevated
* privileges on the host container instance (similar to the
* root
user). This parameter maps to
* Privileged
in the Create a container section of the Docker Remote API and the --privileged
option to
* docker
* run.
*/
public void setPrivileged(Boolean privileged) {
this.privileged = privileged;
}
/**
*
* When this parameter is true, the container is given elevated privileges
* on the host container instance (similar to the root
user).
* This parameter maps to Privileged
in the Create a container section of the Docker Remote API and the --privileged
option to docker run.
*
*
* @return When this parameter is true, the container is given elevated
* privileges on the host container instance (similar to the
* root
user). This parameter maps to
* Privileged
in the Create a container section of the Docker Remote API and the --privileged
option
* to
* docker run.
*/
public Boolean getPrivileged() {
return this.privileged;
}
/**
*
* When this parameter is true, the container is given elevated privileges
* on the host container instance (similar to the root
user).
* This parameter maps to Privileged
in the Create a container section of the Docker Remote API and the --privileged
option to docker run.
*
*
* @param privileged
* When this parameter is true, the container is given elevated
* privileges on the host container instance (similar to the
* root
user). This parameter maps to
* Privileged
in the Create a container section of the Docker Remote API and the --privileged
option to
* docker
* run.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withPrivileged(Boolean privileged) {
setPrivileged(privileged);
return this;
}
/**
*
* When this parameter is true, the container is given elevated privileges
* on the host container instance (similar to the root
user).
* This parameter maps to Privileged
in the Create a container section of the Docker Remote API and the --privileged
option to docker run.
*
*
* @return When this parameter is true, the container is given elevated
* privileges on the host container instance (similar to the
* root
user). This parameter maps to
* Privileged
in the Create a container section of the Docker Remote API and the --privileged
option
* to
* docker run.
*/
public Boolean isPrivileged() {
return this.privileged;
}
/**
*
* When this parameter is true, the container is given read-only access to
* its root file system. This parameter maps to ReadonlyRootfs
* in the Create a container section of the Docker Remote API and the --read-only
option to
* docker run
.
*
*
* @param readonlyRootFilesystem
* When this parameter is true, the container is given read-only
* access to its root file system. This parameter maps to
* ReadonlyRootfs
in the Create a container section of the Docker Remote API and the --read-only
option to
* docker run
.
*/
public void setReadonlyRootFilesystem(Boolean readonlyRootFilesystem) {
this.readonlyRootFilesystem = readonlyRootFilesystem;
}
/**
*
* When this parameter is true, the container is given read-only access to
* its root file system. This parameter maps to ReadonlyRootfs
* in the Create a container section of the Docker Remote API and the --read-only
option to
* docker run
.
*
*
* @return When this parameter is true, the container is given read-only
* access to its root file system. This parameter maps to
* ReadonlyRootfs
in the Create a container section of the Docker Remote API and the --read-only
option to
* docker run
.
*/
public Boolean getReadonlyRootFilesystem() {
return this.readonlyRootFilesystem;
}
/**
*
* When this parameter is true, the container is given read-only access to
* its root file system. This parameter maps to ReadonlyRootfs
* in the Create a container section of the Docker Remote API and the --read-only
option to
* docker run
.
*
*
* @param readonlyRootFilesystem
* When this parameter is true, the container is given read-only
* access to its root file system. This parameter maps to
* ReadonlyRootfs
in the Create a container section of the Docker Remote API and the --read-only
option to
* docker run
.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withReadonlyRootFilesystem(
Boolean readonlyRootFilesystem) {
setReadonlyRootFilesystem(readonlyRootFilesystem);
return this;
}
/**
*
* When this parameter is true, the container is given read-only access to
* its root file system. This parameter maps to ReadonlyRootfs
* in the Create a container section of the Docker Remote API and the --read-only
option to
* docker run
.
*
*
* @return When this parameter is true, the container is given read-only
* access to its root file system. This parameter maps to
* ReadonlyRootfs
in the Create a container section of the Docker Remote API and the --read-only
option to
* docker run
.
*/
public Boolean isReadonlyRootFilesystem() {
return this.readonlyRootFilesystem;
}
/**
*
* A list of DNS servers that are presented to the container. This parameter
* maps to Dns
in the Create a container section of the Docker Remote API and the --dns
option to docker run.
*
*
* @return A list of DNS servers that are presented to the container. This
* parameter maps to Dns
in the Create a container section of the Docker Remote API and the --dns
option to docker
* run.
*/
public java.util.List getDnsServers() {
if (dnsServers == null) {
dnsServers = new com.amazonaws.internal.SdkInternalList();
}
return dnsServers;
}
/**
*
* A list of DNS servers that are presented to the container. This parameter
* maps to Dns
in the Create a container section of the Docker Remote API and the --dns
option to docker run.
*
*
* @param dnsServers
* A list of DNS servers that are presented to the container. This
* parameter maps to Dns
in the Create a container section of the Docker Remote API and the --dns
option to docker
* run.
*/
public void setDnsServers(java.util.Collection dnsServers) {
if (dnsServers == null) {
this.dnsServers = null;
return;
}
this.dnsServers = new com.amazonaws.internal.SdkInternalList(
dnsServers);
}
/**
*
* A list of DNS servers that are presented to the container. This parameter
* maps to Dns
in the Create a container section of the Docker Remote API and the --dns
option to docker run.
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setDnsServers(java.util.Collection)} or
* {@link #withDnsServers(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param dnsServers
* A list of DNS servers that are presented to the container. This
* parameter maps to Dns
in the Create a container section of the Docker Remote API and the --dns
option to docker
* run.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withDnsServers(String... dnsServers) {
if (this.dnsServers == null) {
setDnsServers(new com.amazonaws.internal.SdkInternalList(
dnsServers.length));
}
for (String ele : dnsServers) {
this.dnsServers.add(ele);
}
return this;
}
/**
*
* A list of DNS servers that are presented to the container. This parameter
* maps to Dns
in the Create a container section of the Docker Remote API and the --dns
option to docker run.
*
*
* @param dnsServers
* A list of DNS servers that are presented to the container. This
* parameter maps to Dns
in the Create a container section of the Docker Remote API and the --dns
option to docker
* run.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withDnsServers(
java.util.Collection dnsServers) {
setDnsServers(dnsServers);
return this;
}
/**
*
* A list of DNS search domains that are presented to the container. This
* parameter maps to DnsSearch
in the Create a container section of the Docker Remote API and the --dns-search
option to docker run.
*
*
* @return A list of DNS search domains that are presented to the container.
* This parameter maps to DnsSearch
in the Create a container section of the Docker Remote API and the --dns-search
option
* to
* docker run.
*/
public java.util.List getDnsSearchDomains() {
if (dnsSearchDomains == null) {
dnsSearchDomains = new com.amazonaws.internal.SdkInternalList();
}
return dnsSearchDomains;
}
/**
*
* A list of DNS search domains that are presented to the container. This
* parameter maps to DnsSearch
in the Create a container section of the Docker Remote API and the --dns-search
option to docker run.
*
*
* @param dnsSearchDomains
* A list of DNS search domains that are presented to the container.
* This parameter maps to DnsSearch
in the Create a container section of the Docker Remote API and the --dns-search
option to
* docker
* run.
*/
public void setDnsSearchDomains(
java.util.Collection dnsSearchDomains) {
if (dnsSearchDomains == null) {
this.dnsSearchDomains = null;
return;
}
this.dnsSearchDomains = new com.amazonaws.internal.SdkInternalList(
dnsSearchDomains);
}
/**
*
* A list of DNS search domains that are presented to the container. This
* parameter maps to DnsSearch
in the Create a container section of the Docker Remote API and the --dns-search
option to docker run.
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setDnsSearchDomains(java.util.Collection)} or
* {@link #withDnsSearchDomains(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param dnsSearchDomains
* A list of DNS search domains that are presented to the container.
* This parameter maps to DnsSearch
in the Create a container section of the Docker Remote API and the --dns-search
option to
* docker
* run.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withDnsSearchDomains(String... dnsSearchDomains) {
if (this.dnsSearchDomains == null) {
setDnsSearchDomains(new com.amazonaws.internal.SdkInternalList(
dnsSearchDomains.length));
}
for (String ele : dnsSearchDomains) {
this.dnsSearchDomains.add(ele);
}
return this;
}
/**
*
* A list of DNS search domains that are presented to the container. This
* parameter maps to DnsSearch
in the Create a container section of the Docker Remote API and the --dns-search
option to docker run.
*
*
* @param dnsSearchDomains
* A list of DNS search domains that are presented to the container.
* This parameter maps to DnsSearch
in the Create a container section of the Docker Remote API and the --dns-search
option to
* docker
* run.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withDnsSearchDomains(
java.util.Collection dnsSearchDomains) {
setDnsSearchDomains(dnsSearchDomains);
return this;
}
/**
*
* A list of hostnames and IP address mappings to append to the
* /etc/hosts
file on the container. This parameter maps to
* ExtraHosts
in the Create a container section of the Docker Remote API and the --add-host
option to docker run.
*
*
* @return A list of hostnames and IP address mappings to append to the
* /etc/hosts
file on the container. This parameter
* maps to ExtraHosts
in the Create a container section of the Docker Remote API and the --add-host
option to
*
* docker run.
*/
public java.util.List getExtraHosts() {
if (extraHosts == null) {
extraHosts = new com.amazonaws.internal.SdkInternalList();
}
return extraHosts;
}
/**
*
* A list of hostnames and IP address mappings to append to the
* /etc/hosts
file on the container. This parameter maps to
* ExtraHosts
in the Create a container section of the Docker Remote API and the --add-host
option to docker run.
*
*
* @param extraHosts
* A list of hostnames and IP address mappings to append to the
* /etc/hosts
file on the container. This parameter maps
* to ExtraHosts
in the Create a container section of the Docker Remote API and the --add-host
option to
* docker
* run.
*/
public void setExtraHosts(java.util.Collection extraHosts) {
if (extraHosts == null) {
this.extraHosts = null;
return;
}
this.extraHosts = new com.amazonaws.internal.SdkInternalList(
extraHosts);
}
/**
*
* A list of hostnames and IP address mappings to append to the
* /etc/hosts
file on the container. This parameter maps to
* ExtraHosts
in the Create a container section of the Docker Remote API and the --add-host
option to docker run.
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setExtraHosts(java.util.Collection)} or
* {@link #withExtraHosts(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param extraHosts
* A list of hostnames and IP address mappings to append to the
* /etc/hosts
file on the container. This parameter maps
* to ExtraHosts
in the Create a container section of the Docker Remote API and the --add-host
option to
* docker
* run.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withExtraHosts(HostEntry... extraHosts) {
if (this.extraHosts == null) {
setExtraHosts(new com.amazonaws.internal.SdkInternalList(
extraHosts.length));
}
for (HostEntry ele : extraHosts) {
this.extraHosts.add(ele);
}
return this;
}
/**
*
* A list of hostnames and IP address mappings to append to the
* /etc/hosts
file on the container. This parameter maps to
* ExtraHosts
in the Create a container section of the Docker Remote API and the --add-host
option to docker run.
*
*
* @param extraHosts
* A list of hostnames and IP address mappings to append to the
* /etc/hosts
file on the container. This parameter maps
* to ExtraHosts
in the Create a container section of the Docker Remote API and the --add-host
option to
* docker
* run.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withExtraHosts(
java.util.Collection extraHosts) {
setExtraHosts(extraHosts);
return this;
}
/**
*
* A list of strings to provide custom labels for SELinux and AppArmor
* multi-level security systems. This parameter maps to
* SecurityOpt
in the Create a container section of the Docker Remote API and the --security-opt
option to docker run.
*
*
*
* The Amazon ECS container agent running on a container instance must
* register with the ECS_SELINUX_CAPABLE=true
or
* ECS_APPARMOR_CAPABLE=true
environment variables before
* containers placed on that instance can use these security options. For
* more information, see Amazon ECS Container Agent Configuration in the Amazon EC2
* Container Service Developer Guide.
*
*
*
* @return A list of strings to provide custom labels for SELinux and
* AppArmor multi-level security systems. This parameter maps to
* SecurityOpt
in the Create a container section of the Docker Remote API and the --security-opt
option
* to
* docker run.
*
* The Amazon ECS container agent running on a container instance
* must register with the ECS_SELINUX_CAPABLE=true
or
* ECS_APPARMOR_CAPABLE=true
environment variables
* before containers placed on that instance can use these security
* options. For more information, see Amazon ECS Container Agent Configuration in the Amazon
* EC2 Container Service Developer Guide.
*
*/
public java.util.List getDockerSecurityOptions() {
if (dockerSecurityOptions == null) {
dockerSecurityOptions = new com.amazonaws.internal.SdkInternalList();
}
return dockerSecurityOptions;
}
/**
*
* A list of strings to provide custom labels for SELinux and AppArmor
* multi-level security systems. This parameter maps to
* SecurityOpt
in the Create a container section of the Docker Remote API and the --security-opt
option to docker run.
*
*
*
* The Amazon ECS container agent running on a container instance must
* register with the ECS_SELINUX_CAPABLE=true
or
* ECS_APPARMOR_CAPABLE=true
environment variables before
* containers placed on that instance can use these security options. For
* more information, see Amazon ECS Container Agent Configuration in the Amazon EC2
* Container Service Developer Guide.
*
*
*
* @param dockerSecurityOptions
* A list of strings to provide custom labels for SELinux and
* AppArmor multi-level security systems. This parameter maps to
* SecurityOpt
in the Create a container section of the Docker Remote API and the --security-opt
option
* to docker
* run.
*
* The Amazon ECS container agent running on a container instance
* must register with the ECS_SELINUX_CAPABLE=true
or
* ECS_APPARMOR_CAPABLE=true
environment variables
* before containers placed on that instance can use these security
* options. For more information, see Amazon ECS Container Agent Configuration in the Amazon EC2
* Container Service Developer Guide.
*
*/
public void setDockerSecurityOptions(
java.util.Collection dockerSecurityOptions) {
if (dockerSecurityOptions == null) {
this.dockerSecurityOptions = null;
return;
}
this.dockerSecurityOptions = new com.amazonaws.internal.SdkInternalList(
dockerSecurityOptions);
}
/**
*
* A list of strings to provide custom labels for SELinux and AppArmor
* multi-level security systems. This parameter maps to
* SecurityOpt
in the Create a container section of the Docker Remote API and the --security-opt
option to docker run.
*
*
*
* The Amazon ECS container agent running on a container instance must
* register with the ECS_SELINUX_CAPABLE=true
or
* ECS_APPARMOR_CAPABLE=true
environment variables before
* containers placed on that instance can use these security options. For
* more information, see Amazon ECS Container Agent Configuration in the Amazon EC2
* Container Service Developer Guide.
*
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setDockerSecurityOptions(java.util.Collection)} or
* {@link #withDockerSecurityOptions(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param dockerSecurityOptions
* A list of strings to provide custom labels for SELinux and
* AppArmor multi-level security systems. This parameter maps to
* SecurityOpt
in the Create a container section of the Docker Remote API and the --security-opt
option
* to docker
* run.
*
* The Amazon ECS container agent running on a container instance
* must register with the ECS_SELINUX_CAPABLE=true
or
* ECS_APPARMOR_CAPABLE=true
environment variables
* before containers placed on that instance can use these security
* options. For more information, see Amazon ECS Container Agent Configuration in the Amazon EC2
* Container Service Developer Guide.
*
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withDockerSecurityOptions(
String... dockerSecurityOptions) {
if (this.dockerSecurityOptions == null) {
setDockerSecurityOptions(new com.amazonaws.internal.SdkInternalList(
dockerSecurityOptions.length));
}
for (String ele : dockerSecurityOptions) {
this.dockerSecurityOptions.add(ele);
}
return this;
}
/**
*
* A list of strings to provide custom labels for SELinux and AppArmor
* multi-level security systems. This parameter maps to
* SecurityOpt
in the Create a container section of the Docker Remote API and the --security-opt
option to docker run.
*
*
*
* The Amazon ECS container agent running on a container instance must
* register with the ECS_SELINUX_CAPABLE=true
or
* ECS_APPARMOR_CAPABLE=true
environment variables before
* containers placed on that instance can use these security options. For
* more information, see Amazon ECS Container Agent Configuration in the Amazon EC2
* Container Service Developer Guide.
*
*
*
* @param dockerSecurityOptions
* A list of strings to provide custom labels for SELinux and
* AppArmor multi-level security systems. This parameter maps to
* SecurityOpt
in the Create a container section of the Docker Remote API and the --security-opt
option
* to docker
* run.
*
* The Amazon ECS container agent running on a container instance
* must register with the ECS_SELINUX_CAPABLE=true
or
* ECS_APPARMOR_CAPABLE=true
environment variables
* before containers placed on that instance can use these security
* options. For more information, see Amazon ECS Container Agent Configuration in the Amazon EC2
* Container Service Developer Guide.
*
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withDockerSecurityOptions(
java.util.Collection dockerSecurityOptions) {
setDockerSecurityOptions(dockerSecurityOptions);
return this;
}
/**
*
* A key/value map of labels to add to the container. This parameter maps to
* Labels
in the Create a container section of the Docker Remote API and the --label
option to docker run.
* This parameter requires version 1.18 of the Docker Remote API or greater
* on your container instance. To check the Docker Remote API version on
* your container instance, log into your container instance and run the
* following command:
* sudo docker version | grep "Server API version"
*
*
* @return A key/value map of labels to add to the container. This parameter
* maps to Labels
in the Create a container section of the Docker Remote API and the --label
option to docker
* run. This parameter requires version 1.18 of the Docker
* Remote API or greater on your container instance. To check the
* Docker Remote API version on your container instance, log into
* your container instance and run the following command:
* sudo docker version | grep "Server API version"
*/
public java.util.Map getDockerLabels() {
return dockerLabels;
}
/**
*
* A key/value map of labels to add to the container. This parameter maps to
* Labels
in the Create a container section of the Docker Remote API and the --label
option to docker run.
* This parameter requires version 1.18 of the Docker Remote API or greater
* on your container instance. To check the Docker Remote API version on
* your container instance, log into your container instance and run the
* following command:
* sudo docker version | grep "Server API version"
*
*
* @param dockerLabels
* A key/value map of labels to add to the container. This parameter
* maps to Labels
in the Create a container section of the Docker Remote API and the --label
option to docker
* run. This parameter requires version 1.18 of the Docker Remote
* API or greater on your container instance. To check the Docker
* Remote API version on your container instance, log into your
* container instance and run the following command:
* sudo docker version | grep "Server API version"
*/
public void setDockerLabels(java.util.Map dockerLabels) {
this.dockerLabels = dockerLabels;
}
/**
*
* A key/value map of labels to add to the container. This parameter maps to
* Labels
in the Create a container section of the Docker Remote API and the --label
option to docker run.
* This parameter requires version 1.18 of the Docker Remote API or greater
* on your container instance. To check the Docker Remote API version on
* your container instance, log into your container instance and run the
* following command:
* sudo docker version | grep "Server API version"
*
*
* @param dockerLabels
* A key/value map of labels to add to the container. This parameter
* maps to Labels
in the Create a container section of the Docker Remote API and the --label
option to docker
* run. This parameter requires version 1.18 of the Docker Remote
* API or greater on your container instance. To check the Docker
* Remote API version on your container instance, log into your
* container instance and run the following command:
* sudo docker version | grep "Server API version"
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withDockerLabels(
java.util.Map dockerLabels) {
setDockerLabels(dockerLabels);
return this;
}
public ContainerDefinition addDockerLabelsEntry(String key, String value) {
if (null == this.dockerLabels) {
this.dockerLabels = new java.util.HashMap();
}
if (this.dockerLabels.containsKey(key))
throw new IllegalArgumentException("Duplicated keys ("
+ key.toString() + ") are provided.");
this.dockerLabels.put(key, value);
return this;
}
/**
* Removes all the entries added into DockerLabels. <p> Returns a
* reference to this object so that method calls can be chained together.
*/
public ContainerDefinition clearDockerLabelsEntries() {
this.dockerLabels = null;
return this;
}
/**
*
* A list of ulimits
to set in the container. This parameter
* maps to Ulimits
in the Create a container section of the Docker Remote API and the --ulimit
option to docker run.
* Valid naming values are displayed in the Ulimit data type. This
* parameter requires version 1.18 of the Docker Remote API or greater on
* your container instance. To check the Docker Remote API version on your
* container instance, log into your container instance and run the
* following command:
* sudo docker version | grep "Server API version"
*
*
* @return A list of ulimits
to set in the container. This
* parameter maps to Ulimits
in the Create a container section of the Docker Remote API and the --ulimit
option to docker
* run. Valid naming values are displayed in the Ulimit
* data type. This parameter requires version 1.18 of the Docker
* Remote API or greater on your container instance. To check the
* Docker Remote API version on your container instance, log into
* your container instance and run the following command:
* sudo docker version | grep "Server API version"
*/
public java.util.List getUlimits() {
if (ulimits == null) {
ulimits = new com.amazonaws.internal.SdkInternalList();
}
return ulimits;
}
/**
*
* A list of ulimits
to set in the container. This parameter
* maps to Ulimits
in the Create a container section of the Docker Remote API and the --ulimit
option to docker run.
* Valid naming values are displayed in the Ulimit data type. This
* parameter requires version 1.18 of the Docker Remote API or greater on
* your container instance. To check the Docker Remote API version on your
* container instance, log into your container instance and run the
* following command:
* sudo docker version | grep "Server API version"
*
*
* @param ulimits
* A list of ulimits
to set in the container. This
* parameter maps to Ulimits
in the Create a container section of the Docker Remote API and the --ulimit
option to docker
* run. Valid naming values are displayed in the Ulimit
* data type. This parameter requires version 1.18 of the Docker
* Remote API or greater on your container instance. To check the
* Docker Remote API version on your container instance, log into
* your container instance and run the following command:
* sudo docker version | grep "Server API version"
*/
public void setUlimits(java.util.Collection ulimits) {
if (ulimits == null) {
this.ulimits = null;
return;
}
this.ulimits = new com.amazonaws.internal.SdkInternalList(
ulimits);
}
/**
*
* A list of ulimits
to set in the container. This parameter
* maps to Ulimits
in the Create a container section of the Docker Remote API and the --ulimit
option to docker run.
* Valid naming values are displayed in the Ulimit data type. This
* parameter requires version 1.18 of the Docker Remote API or greater on
* your container instance. To check the Docker Remote API version on your
* container instance, log into your container instance and run the
* following command:
* sudo docker version | grep "Server API version"
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setUlimits(java.util.Collection)} or
* {@link #withUlimits(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param ulimits
* A list of ulimits
to set in the container. This
* parameter maps to Ulimits
in the Create a container section of the Docker Remote API and the --ulimit
option to docker
* run. Valid naming values are displayed in the Ulimit
* data type. This parameter requires version 1.18 of the Docker
* Remote API or greater on your container instance. To check the
* Docker Remote API version on your container instance, log into
* your container instance and run the following command:
* sudo docker version | grep "Server API version"
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withUlimits(Ulimit... ulimits) {
if (this.ulimits == null) {
setUlimits(new com.amazonaws.internal.SdkInternalList(
ulimits.length));
}
for (Ulimit ele : ulimits) {
this.ulimits.add(ele);
}
return this;
}
/**
*
* A list of ulimits
to set in the container. This parameter
* maps to Ulimits
in the Create a container section of the Docker Remote API and the --ulimit
option to docker run.
* Valid naming values are displayed in the Ulimit data type. This
* parameter requires version 1.18 of the Docker Remote API or greater on
* your container instance. To check the Docker Remote API version on your
* container instance, log into your container instance and run the
* following command:
* sudo docker version | grep "Server API version"
*
*
* @param ulimits
* A list of ulimits
to set in the container. This
* parameter maps to Ulimits
in the Create a container section of the Docker Remote API and the --ulimit
option to docker
* run. Valid naming values are displayed in the Ulimit
* data type. This parameter requires version 1.18 of the Docker
* Remote API or greater on your container instance. To check the
* Docker Remote API version on your container instance, log into
* your container instance and run the following command:
* sudo docker version | grep "Server API version"
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withUlimits(java.util.Collection ulimits) {
setUlimits(ulimits);
return this;
}
/**
*
* The log configuration specification for the container. This parameter
* maps to LogConfig
in the Create a container section of the Docker Remote API and the --log-driver
option to docker run.
* By default, containers use the same logging driver that the Docker daemon
* uses; however the container may use a different logging driver than the
* Docker daemon by specifying a log driver with this parameter in the
* container definition. To use a different logging driver for a container,
* the log system must be configured properly on the container instance (or
* on a different log server for remote logging options). For more
* information on the options for different supported log drivers, see Configure
* logging drivers in the Docker documentation.
*
*
*
* Amazon ECS currently supports a subset of the logging drivers available
* to the Docker daemon (shown in the LogConfiguration data type).
* Currently unsupported log drivers may be available in future releases of
* the Amazon ECS container agent.
*
*
*
* This parameter requires version 1.18 of the Docker Remote API or greater
* on your container instance. To check the Docker Remote API version on
* your container instance, log into your container instance and run the
* following command:
* sudo docker version | grep "Server API version"
*
*
*
* The Amazon ECS container agent running on a container instance must
* register the logging drivers available on that instance with the
* ECS_AVAILABLE_LOGGING_DRIVERS
environment variable before
* containers placed on that instance can use these log configuration
* options. For more information, see Amazon ECS Container Agent Configuration in the Amazon EC2
* Container Service Developer Guide.
*
*
*
* @param logConfiguration
* The log configuration specification for the container. This
* parameter maps to LogConfig
in the Create a container section of the Docker Remote API and the --log-driver
option to
* docker
* run. By default, containers use the same logging driver that
* the Docker daemon uses; however the container may use a different
* logging driver than the Docker daemon by specifying a log driver
* with this parameter in the container definition. To use a
* different logging driver for a container, the log system must be
* configured properly on the container instance (or on a different
* log server for remote logging options). For more information on
* the options for different supported log drivers, see Configure logging drivers in the Docker documentation.
*
*
* Amazon ECS currently supports a subset of the logging drivers
* available to the Docker daemon (shown in the
* LogConfiguration data type). Currently unsupported log
* drivers may be available in future releases of the Amazon ECS
* container agent.
*
*
*
* This parameter requires version 1.18 of the Docker Remote API or
* greater on your container instance. To check the Docker Remote API
* version on your container instance, log into your container
* instance and run the following command:
* sudo docker version | grep "Server API version"
*
*
*
* The Amazon ECS container agent running on a container instance
* must register the logging drivers available on that instance with
* the ECS_AVAILABLE_LOGGING_DRIVERS
environment
* variable before containers placed on that instance can use these
* log configuration options. For more information, see Amazon ECS Container Agent Configuration in the Amazon EC2
* Container Service Developer Guide.
*
*/
public void setLogConfiguration(LogConfiguration logConfiguration) {
this.logConfiguration = logConfiguration;
}
/**
*
* The log configuration specification for the container. This parameter
* maps to LogConfig
in the Create a container section of the Docker Remote API and the --log-driver
option to docker run.
* By default, containers use the same logging driver that the Docker daemon
* uses; however the container may use a different logging driver than the
* Docker daemon by specifying a log driver with this parameter in the
* container definition. To use a different logging driver for a container,
* the log system must be configured properly on the container instance (or
* on a different log server for remote logging options). For more
* information on the options for different supported log drivers, see Configure
* logging drivers in the Docker documentation.
*
*
*
* Amazon ECS currently supports a subset of the logging drivers available
* to the Docker daemon (shown in the LogConfiguration data type).
* Currently unsupported log drivers may be available in future releases of
* the Amazon ECS container agent.
*
*
*
* This parameter requires version 1.18 of the Docker Remote API or greater
* on your container instance. To check the Docker Remote API version on
* your container instance, log into your container instance and run the
* following command:
* sudo docker version | grep "Server API version"
*
*
*
* The Amazon ECS container agent running on a container instance must
* register the logging drivers available on that instance with the
* ECS_AVAILABLE_LOGGING_DRIVERS
environment variable before
* containers placed on that instance can use these log configuration
* options. For more information, see Amazon ECS Container Agent Configuration in the Amazon EC2
* Container Service Developer Guide.
*
*
*
* @return The log configuration specification for the container. This
* parameter maps to LogConfig
in the Create a container section of the Docker Remote API and the --log-driver
option
* to
* docker run. By default, containers use the same logging
* driver that the Docker daemon uses; however the container may use
* a different logging driver than the Docker daemon by specifying a
* log driver with this parameter in the container definition. To
* use a different logging driver for a container, the log system
* must be configured properly on the container instance (or on a
* different log server for remote logging options). For more
* information on the options for different supported log drivers,
* see Configure logging drivers in the Docker documentation.
*
*
* Amazon ECS currently supports a subset of the logging drivers
* available to the Docker daemon (shown in the
* LogConfiguration data type). Currently unsupported log
* drivers may be available in future releases of the Amazon ECS
* container agent.
*
*
*
* This parameter requires version 1.18 of the Docker Remote API or
* greater on your container instance. To check the Docker Remote
* API version on your container instance, log into your container
* instance and run the following command:
* sudo docker version | grep "Server API version"
*
*
*
* The Amazon ECS container agent running on a container instance
* must register the logging drivers available on that instance with
* the ECS_AVAILABLE_LOGGING_DRIVERS
environment
* variable before containers placed on that instance can use these
* log configuration options. For more information, see Amazon ECS Container Agent Configuration in the Amazon
* EC2 Container Service Developer Guide.
*
*/
public LogConfiguration getLogConfiguration() {
return this.logConfiguration;
}
/**
*
* The log configuration specification for the container. This parameter
* maps to LogConfig
in the Create a container section of the Docker Remote API and the --log-driver
option to docker run.
* By default, containers use the same logging driver that the Docker daemon
* uses; however the container may use a different logging driver than the
* Docker daemon by specifying a log driver with this parameter in the
* container definition. To use a different logging driver for a container,
* the log system must be configured properly on the container instance (or
* on a different log server for remote logging options). For more
* information on the options for different supported log drivers, see Configure
* logging drivers in the Docker documentation.
*
*
*
* Amazon ECS currently supports a subset of the logging drivers available
* to the Docker daemon (shown in the LogConfiguration data type).
* Currently unsupported log drivers may be available in future releases of
* the Amazon ECS container agent.
*
*
*
* This parameter requires version 1.18 of the Docker Remote API or greater
* on your container instance. To check the Docker Remote API version on
* your container instance, log into your container instance and run the
* following command:
* sudo docker version | grep "Server API version"
*
*
*
* The Amazon ECS container agent running on a container instance must
* register the logging drivers available on that instance with the
* ECS_AVAILABLE_LOGGING_DRIVERS
environment variable before
* containers placed on that instance can use these log configuration
* options. For more information, see Amazon ECS Container Agent Configuration in the Amazon EC2
* Container Service Developer Guide.
*
*
*
* @param logConfiguration
* The log configuration specification for the container. This
* parameter maps to LogConfig
in the Create a container section of the Docker Remote API and the --log-driver
option to
* docker
* run. By default, containers use the same logging driver that
* the Docker daemon uses; however the container may use a different
* logging driver than the Docker daemon by specifying a log driver
* with this parameter in the container definition. To use a
* different logging driver for a container, the log system must be
* configured properly on the container instance (or on a different
* log server for remote logging options). For more information on
* the options for different supported log drivers, see Configure logging drivers in the Docker documentation.
*
*
* Amazon ECS currently supports a subset of the logging drivers
* available to the Docker daemon (shown in the
* LogConfiguration data type). Currently unsupported log
* drivers may be available in future releases of the Amazon ECS
* container agent.
*
*
*
* This parameter requires version 1.18 of the Docker Remote API or
* greater on your container instance. To check the Docker Remote API
* version on your container instance, log into your container
* instance and run the following command:
* sudo docker version | grep "Server API version"
*
*
*
* The Amazon ECS container agent running on a container instance
* must register the logging drivers available on that instance with
* the ECS_AVAILABLE_LOGGING_DRIVERS
environment
* variable before containers placed on that instance can use these
* log configuration options. For more information, see Amazon ECS Container Agent Configuration in the Amazon EC2
* Container Service Developer Guide.
*
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public ContainerDefinition withLogConfiguration(
LogConfiguration logConfiguration) {
setLogConfiguration(logConfiguration);
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: " + getName() + ",");
if (getImage() != null)
sb.append("Image: " + getImage() + ",");
if (getCpu() != null)
sb.append("Cpu: " + getCpu() + ",");
if (getMemory() != null)
sb.append("Memory: " + getMemory() + ",");
if (getLinks() != null)
sb.append("Links: " + getLinks() + ",");
if (getPortMappings() != null)
sb.append("PortMappings: " + getPortMappings() + ",");
if (getEssential() != null)
sb.append("Essential: " + getEssential() + ",");
if (getEntryPoint() != null)
sb.append("EntryPoint: " + getEntryPoint() + ",");
if (getCommand() != null)
sb.append("Command: " + getCommand() + ",");
if (getEnvironment() != null)
sb.append("Environment: " + getEnvironment() + ",");
if (getMountPoints() != null)
sb.append("MountPoints: " + getMountPoints() + ",");
if (getVolumesFrom() != null)
sb.append("VolumesFrom: " + getVolumesFrom() + ",");
if (getHostname() != null)
sb.append("Hostname: " + getHostname() + ",");
if (getUser() != null)
sb.append("User: " + getUser() + ",");
if (getWorkingDirectory() != null)
sb.append("WorkingDirectory: " + getWorkingDirectory() + ",");
if (getDisableNetworking() != null)
sb.append("DisableNetworking: " + getDisableNetworking() + ",");
if (getPrivileged() != null)
sb.append("Privileged: " + getPrivileged() + ",");
if (getReadonlyRootFilesystem() != null)
sb.append("ReadonlyRootFilesystem: " + getReadonlyRootFilesystem()
+ ",");
if (getDnsServers() != null)
sb.append("DnsServers: " + getDnsServers() + ",");
if (getDnsSearchDomains() != null)
sb.append("DnsSearchDomains: " + getDnsSearchDomains() + ",");
if (getExtraHosts() != null)
sb.append("ExtraHosts: " + getExtraHosts() + ",");
if (getDockerSecurityOptions() != null)
sb.append("DockerSecurityOptions: " + getDockerSecurityOptions()
+ ",");
if (getDockerLabels() != null)
sb.append("DockerLabels: " + getDockerLabels() + ",");
if (getUlimits() != null)
sb.append("Ulimits: " + getUlimits() + ",");
if (getLogConfiguration() != null)
sb.append("LogConfiguration: " + getLogConfiguration());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ContainerDefinition == false)
return false;
ContainerDefinition other = (ContainerDefinition) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null
&& other.getName().equals(this.getName()) == false)
return false;
if (other.getImage() == null ^ this.getImage() == null)
return false;
if (other.getImage() != null
&& other.getImage().equals(this.getImage()) == false)
return false;
if (other.getCpu() == null ^ this.getCpu() == null)
return false;
if (other.getCpu() != null
&& other.getCpu().equals(this.getCpu()) == false)
return false;
if (other.getMemory() == null ^ this.getMemory() == null)
return false;
if (other.getMemory() != null
&& other.getMemory().equals(this.getMemory()) == false)
return false;
if (other.getLinks() == null ^ this.getLinks() == null)
return false;
if (other.getLinks() != null
&& other.getLinks().equals(this.getLinks()) == false)
return false;
if (other.getPortMappings() == null ^ this.getPortMappings() == null)
return false;
if (other.getPortMappings() != null
&& other.getPortMappings().equals(this.getPortMappings()) == false)
return false;
if (other.getEssential() == null ^ this.getEssential() == null)
return false;
if (other.getEssential() != null
&& other.getEssential().equals(this.getEssential()) == false)
return false;
if (other.getEntryPoint() == null ^ this.getEntryPoint() == null)
return false;
if (other.getEntryPoint() != null
&& other.getEntryPoint().equals(this.getEntryPoint()) == false)
return false;
if (other.getCommand() == null ^ this.getCommand() == null)
return false;
if (other.getCommand() != null
&& other.getCommand().equals(this.getCommand()) == false)
return false;
if (other.getEnvironment() == null ^ this.getEnvironment() == null)
return false;
if (other.getEnvironment() != null
&& other.getEnvironment().equals(this.getEnvironment()) == false)
return false;
if (other.getMountPoints() == null ^ this.getMountPoints() == null)
return false;
if (other.getMountPoints() != null
&& other.getMountPoints().equals(this.getMountPoints()) == false)
return false;
if (other.getVolumesFrom() == null ^ this.getVolumesFrom() == null)
return false;
if (other.getVolumesFrom() != null
&& other.getVolumesFrom().equals(this.getVolumesFrom()) == false)
return false;
if (other.getHostname() == null ^ this.getHostname() == null)
return false;
if (other.getHostname() != null
&& other.getHostname().equals(this.getHostname()) == false)
return false;
if (other.getUser() == null ^ this.getUser() == null)
return false;
if (other.getUser() != null
&& other.getUser().equals(this.getUser()) == false)
return false;
if (other.getWorkingDirectory() == null
^ this.getWorkingDirectory() == null)
return false;
if (other.getWorkingDirectory() != null
&& other.getWorkingDirectory().equals(
this.getWorkingDirectory()) == false)
return false;
if (other.getDisableNetworking() == null
^ this.getDisableNetworking() == null)
return false;
if (other.getDisableNetworking() != null
&& other.getDisableNetworking().equals(
this.getDisableNetworking()) == false)
return false;
if (other.getPrivileged() == null ^ this.getPrivileged() == null)
return false;
if (other.getPrivileged() != null
&& other.getPrivileged().equals(this.getPrivileged()) == false)
return false;
if (other.getReadonlyRootFilesystem() == null
^ this.getReadonlyRootFilesystem() == null)
return false;
if (other.getReadonlyRootFilesystem() != null
&& other.getReadonlyRootFilesystem().equals(
this.getReadonlyRootFilesystem()) == false)
return false;
if (other.getDnsServers() == null ^ this.getDnsServers() == null)
return false;
if (other.getDnsServers() != null
&& other.getDnsServers().equals(this.getDnsServers()) == false)
return false;
if (other.getDnsSearchDomains() == null
^ this.getDnsSearchDomains() == null)
return false;
if (other.getDnsSearchDomains() != null
&& other.getDnsSearchDomains().equals(
this.getDnsSearchDomains()) == false)
return false;
if (other.getExtraHosts() == null ^ this.getExtraHosts() == null)
return false;
if (other.getExtraHosts() != null
&& other.getExtraHosts().equals(this.getExtraHosts()) == false)
return false;
if (other.getDockerSecurityOptions() == null
^ this.getDockerSecurityOptions() == null)
return false;
if (other.getDockerSecurityOptions() != null
&& other.getDockerSecurityOptions().equals(
this.getDockerSecurityOptions()) == false)
return false;
if (other.getDockerLabels() == null ^ this.getDockerLabels() == null)
return false;
if (other.getDockerLabels() != null
&& other.getDockerLabels().equals(this.getDockerLabels()) == false)
return false;
if (other.getUlimits() == null ^ this.getUlimits() == null)
return false;
if (other.getUlimits() != null
&& other.getUlimits().equals(this.getUlimits()) == false)
return false;
if (other.getLogConfiguration() == null
^ this.getLogConfiguration() == null)
return false;
if (other.getLogConfiguration() != null
&& other.getLogConfiguration().equals(
this.getLogConfiguration()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode
+ ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode
+ ((getImage() == null) ? 0 : getImage().hashCode());
hashCode = prime * hashCode
+ ((getCpu() == null) ? 0 : getCpu().hashCode());
hashCode = prime * hashCode
+ ((getMemory() == null) ? 0 : getMemory().hashCode());
hashCode = prime * hashCode
+ ((getLinks() == null) ? 0 : getLinks().hashCode());
hashCode = prime
* hashCode
+ ((getPortMappings() == null) ? 0 : getPortMappings()
.hashCode());
hashCode = prime * hashCode
+ ((getEssential() == null) ? 0 : getEssential().hashCode());
hashCode = prime * hashCode
+ ((getEntryPoint() == null) ? 0 : getEntryPoint().hashCode());
hashCode = prime * hashCode
+ ((getCommand() == null) ? 0 : getCommand().hashCode());
hashCode = prime
* hashCode
+ ((getEnvironment() == null) ? 0 : getEnvironment().hashCode());
hashCode = prime
* hashCode
+ ((getMountPoints() == null) ? 0 : getMountPoints().hashCode());
hashCode = prime
* hashCode
+ ((getVolumesFrom() == null) ? 0 : getVolumesFrom().hashCode());
hashCode = prime * hashCode
+ ((getHostname() == null) ? 0 : getHostname().hashCode());
hashCode = prime * hashCode
+ ((getUser() == null) ? 0 : getUser().hashCode());
hashCode = prime
* hashCode
+ ((getWorkingDirectory() == null) ? 0 : getWorkingDirectory()
.hashCode());
hashCode = prime
* hashCode
+ ((getDisableNetworking() == null) ? 0
: getDisableNetworking().hashCode());
hashCode = prime * hashCode
+ ((getPrivileged() == null) ? 0 : getPrivileged().hashCode());
hashCode = prime
* hashCode
+ ((getReadonlyRootFilesystem() == null) ? 0
: getReadonlyRootFilesystem().hashCode());
hashCode = prime * hashCode
+ ((getDnsServers() == null) ? 0 : getDnsServers().hashCode());
hashCode = prime
* hashCode
+ ((getDnsSearchDomains() == null) ? 0 : getDnsSearchDomains()
.hashCode());
hashCode = prime * hashCode
+ ((getExtraHosts() == null) ? 0 : getExtraHosts().hashCode());
hashCode = prime
* hashCode
+ ((getDockerSecurityOptions() == null) ? 0
: getDockerSecurityOptions().hashCode());
hashCode = prime
* hashCode
+ ((getDockerLabels() == null) ? 0 : getDockerLabels()
.hashCode());
hashCode = prime * hashCode
+ ((getUlimits() == null) ? 0 : getUlimits().hashCode());
hashCode = prime
* hashCode
+ ((getLogConfiguration() == null) ? 0 : getLogConfiguration()
.hashCode());
return hashCode;
}
@Override
public ContainerDefinition clone() {
try {
return (ContainerDefinition) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException(
"Got a CloneNotSupportedException from Object.clone() "
+ "even though we're Cloneable!", e);
}
}
}