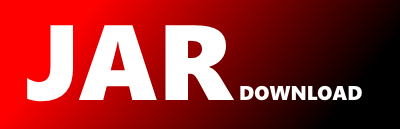
com.amazonaws.services.ecs.model.Cluster Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ecs Show documentation
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ecs.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* A regional grouping of one or more container instances on which you can run task requests. Each account receives a
* default cluster the first time you use the Amazon ECS service, but you may also create other clusters. Clusters may
* contain more than one instance type simultaneously.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Cluster implements Serializable, Cloneable, StructuredPojo {
/**
*
* The Amazon Resource Name (ARN) that identifies the cluster. The ARN contains the arn:aws:ecs
* namespace, followed by the region of the cluster, the AWS account ID of the cluster owner, the
* cluster
namespace, and then the cluster name. For example,
* arn:aws:ecs:region:012345678910:cluster/test
..
*
*/
private String clusterArn;
/**
*
* A user-generated string that you use to identify your cluster.
*
*/
private String clusterName;
/**
*
* The status of the cluster. The valid values are ACTIVE
or INACTIVE
. ACTIVE
* indicates that you can register container instances with the cluster and the associated instances can accept
* tasks.
*
*/
private String status;
/**
*
* The number of container instances registered into the cluster.
*
*/
private Integer registeredContainerInstancesCount;
/**
*
* The number of tasks in the cluster that are in the RUNNING
state.
*
*/
private Integer runningTasksCount;
/**
*
* The number of tasks in the cluster that are in the PENDING
state.
*
*/
private Integer pendingTasksCount;
/**
*
* The number of services that are running on the cluster in an ACTIVE
state. You can view these
* services with ListServices.
*
*/
private Integer activeServicesCount;
/**
*
* The Amazon Resource Name (ARN) that identifies the cluster. The ARN contains the arn:aws:ecs
* namespace, followed by the region of the cluster, the AWS account ID of the cluster owner, the
* cluster
namespace, and then the cluster name. For example,
* arn:aws:ecs:region:012345678910:cluster/test
..
*
*
* @param clusterArn
* The Amazon Resource Name (ARN) that identifies the cluster. The ARN contains the arn:aws:ecs
* namespace, followed by the region of the cluster, the AWS account ID of the cluster owner, the
* cluster
namespace, and then the cluster name. For example,
* arn:aws:ecs:region:012345678910:cluster/test
..
*/
public void setClusterArn(String clusterArn) {
this.clusterArn = clusterArn;
}
/**
*
* The Amazon Resource Name (ARN) that identifies the cluster. The ARN contains the arn:aws:ecs
* namespace, followed by the region of the cluster, the AWS account ID of the cluster owner, the
* cluster
namespace, and then the cluster name. For example,
* arn:aws:ecs:region:012345678910:cluster/test
..
*
*
* @return The Amazon Resource Name (ARN) that identifies the cluster. The ARN contains the arn:aws:ecs
* namespace, followed by the region of the cluster, the AWS account ID of the cluster owner, the
* cluster
namespace, and then the cluster name. For example,
* arn:aws:ecs:region:012345678910:cluster/test
..
*/
public String getClusterArn() {
return this.clusterArn;
}
/**
*
* The Amazon Resource Name (ARN) that identifies the cluster. The ARN contains the arn:aws:ecs
* namespace, followed by the region of the cluster, the AWS account ID of the cluster owner, the
* cluster
namespace, and then the cluster name. For example,
* arn:aws:ecs:region:012345678910:cluster/test
..
*
*
* @param clusterArn
* The Amazon Resource Name (ARN) that identifies the cluster. The ARN contains the arn:aws:ecs
* namespace, followed by the region of the cluster, the AWS account ID of the cluster owner, the
* cluster
namespace, and then the cluster name. For example,
* arn:aws:ecs:region:012345678910:cluster/test
..
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Cluster withClusterArn(String clusterArn) {
setClusterArn(clusterArn);
return this;
}
/**
*
* A user-generated string that you use to identify your cluster.
*
*
* @param clusterName
* A user-generated string that you use to identify your cluster.
*/
public void setClusterName(String clusterName) {
this.clusterName = clusterName;
}
/**
*
* A user-generated string that you use to identify your cluster.
*
*
* @return A user-generated string that you use to identify your cluster.
*/
public String getClusterName() {
return this.clusterName;
}
/**
*
* A user-generated string that you use to identify your cluster.
*
*
* @param clusterName
* A user-generated string that you use to identify your cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Cluster withClusterName(String clusterName) {
setClusterName(clusterName);
return this;
}
/**
*
* The status of the cluster. The valid values are ACTIVE
or INACTIVE
. ACTIVE
* indicates that you can register container instances with the cluster and the associated instances can accept
* tasks.
*
*
* @param status
* The status of the cluster. The valid values are ACTIVE
or INACTIVE
.
* ACTIVE
indicates that you can register container instances with the cluster and the
* associated instances can accept tasks.
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the cluster. The valid values are ACTIVE
or INACTIVE
. ACTIVE
* indicates that you can register container instances with the cluster and the associated instances can accept
* tasks.
*
*
* @return The status of the cluster. The valid values are ACTIVE
or INACTIVE
.
* ACTIVE
indicates that you can register container instances with the cluster and the
* associated instances can accept tasks.
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of the cluster. The valid values are ACTIVE
or INACTIVE
. ACTIVE
* indicates that you can register container instances with the cluster and the associated instances can accept
* tasks.
*
*
* @param status
* The status of the cluster. The valid values are ACTIVE
or INACTIVE
.
* ACTIVE
indicates that you can register container instances with the cluster and the
* associated instances can accept tasks.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Cluster withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The number of container instances registered into the cluster.
*
*
* @param registeredContainerInstancesCount
* The number of container instances registered into the cluster.
*/
public void setRegisteredContainerInstancesCount(Integer registeredContainerInstancesCount) {
this.registeredContainerInstancesCount = registeredContainerInstancesCount;
}
/**
*
* The number of container instances registered into the cluster.
*
*
* @return The number of container instances registered into the cluster.
*/
public Integer getRegisteredContainerInstancesCount() {
return this.registeredContainerInstancesCount;
}
/**
*
* The number of container instances registered into the cluster.
*
*
* @param registeredContainerInstancesCount
* The number of container instances registered into the cluster.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Cluster withRegisteredContainerInstancesCount(Integer registeredContainerInstancesCount) {
setRegisteredContainerInstancesCount(registeredContainerInstancesCount);
return this;
}
/**
*
* The number of tasks in the cluster that are in the RUNNING
state.
*
*
* @param runningTasksCount
* The number of tasks in the cluster that are in the RUNNING
state.
*/
public void setRunningTasksCount(Integer runningTasksCount) {
this.runningTasksCount = runningTasksCount;
}
/**
*
* The number of tasks in the cluster that are in the RUNNING
state.
*
*
* @return The number of tasks in the cluster that are in the RUNNING
state.
*/
public Integer getRunningTasksCount() {
return this.runningTasksCount;
}
/**
*
* The number of tasks in the cluster that are in the RUNNING
state.
*
*
* @param runningTasksCount
* The number of tasks in the cluster that are in the RUNNING
state.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Cluster withRunningTasksCount(Integer runningTasksCount) {
setRunningTasksCount(runningTasksCount);
return this;
}
/**
*
* The number of tasks in the cluster that are in the PENDING
state.
*
*
* @param pendingTasksCount
* The number of tasks in the cluster that are in the PENDING
state.
*/
public void setPendingTasksCount(Integer pendingTasksCount) {
this.pendingTasksCount = pendingTasksCount;
}
/**
*
* The number of tasks in the cluster that are in the PENDING
state.
*
*
* @return The number of tasks in the cluster that are in the PENDING
state.
*/
public Integer getPendingTasksCount() {
return this.pendingTasksCount;
}
/**
*
* The number of tasks in the cluster that are in the PENDING
state.
*
*
* @param pendingTasksCount
* The number of tasks in the cluster that are in the PENDING
state.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Cluster withPendingTasksCount(Integer pendingTasksCount) {
setPendingTasksCount(pendingTasksCount);
return this;
}
/**
*
* The number of services that are running on the cluster in an ACTIVE
state. You can view these
* services with ListServices.
*
*
* @param activeServicesCount
* The number of services that are running on the cluster in an ACTIVE
state. You can view these
* services with ListServices.
*/
public void setActiveServicesCount(Integer activeServicesCount) {
this.activeServicesCount = activeServicesCount;
}
/**
*
* The number of services that are running on the cluster in an ACTIVE
state. You can view these
* services with ListServices.
*
*
* @return The number of services that are running on the cluster in an ACTIVE
state. You can view
* these services with ListServices.
*/
public Integer getActiveServicesCount() {
return this.activeServicesCount;
}
/**
*
* The number of services that are running on the cluster in an ACTIVE
state. You can view these
* services with ListServices.
*
*
* @param activeServicesCount
* The number of services that are running on the cluster in an ACTIVE
state. You can view these
* services with ListServices.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Cluster withActiveServicesCount(Integer activeServicesCount) {
setActiveServicesCount(activeServicesCount);
return this;
}
/**
* Returns a string representation of this object; useful for testing and debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getClusterArn() != null)
sb.append("ClusterArn: ").append(getClusterArn()).append(",");
if (getClusterName() != null)
sb.append("ClusterName: ").append(getClusterName()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getRegisteredContainerInstancesCount() != null)
sb.append("RegisteredContainerInstancesCount: ").append(getRegisteredContainerInstancesCount()).append(",");
if (getRunningTasksCount() != null)
sb.append("RunningTasksCount: ").append(getRunningTasksCount()).append(",");
if (getPendingTasksCount() != null)
sb.append("PendingTasksCount: ").append(getPendingTasksCount()).append(",");
if (getActiveServicesCount() != null)
sb.append("ActiveServicesCount: ").append(getActiveServicesCount());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Cluster == false)
return false;
Cluster other = (Cluster) obj;
if (other.getClusterArn() == null ^ this.getClusterArn() == null)
return false;
if (other.getClusterArn() != null && other.getClusterArn().equals(this.getClusterArn()) == false)
return false;
if (other.getClusterName() == null ^ this.getClusterName() == null)
return false;
if (other.getClusterName() != null && other.getClusterName().equals(this.getClusterName()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getRegisteredContainerInstancesCount() == null ^ this.getRegisteredContainerInstancesCount() == null)
return false;
if (other.getRegisteredContainerInstancesCount() != null
&& other.getRegisteredContainerInstancesCount().equals(this.getRegisteredContainerInstancesCount()) == false)
return false;
if (other.getRunningTasksCount() == null ^ this.getRunningTasksCount() == null)
return false;
if (other.getRunningTasksCount() != null && other.getRunningTasksCount().equals(this.getRunningTasksCount()) == false)
return false;
if (other.getPendingTasksCount() == null ^ this.getPendingTasksCount() == null)
return false;
if (other.getPendingTasksCount() != null && other.getPendingTasksCount().equals(this.getPendingTasksCount()) == false)
return false;
if (other.getActiveServicesCount() == null ^ this.getActiveServicesCount() == null)
return false;
if (other.getActiveServicesCount() != null && other.getActiveServicesCount().equals(this.getActiveServicesCount()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getClusterArn() == null) ? 0 : getClusterArn().hashCode());
hashCode = prime * hashCode + ((getClusterName() == null) ? 0 : getClusterName().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getRegisteredContainerInstancesCount() == null) ? 0 : getRegisteredContainerInstancesCount().hashCode());
hashCode = prime * hashCode + ((getRunningTasksCount() == null) ? 0 : getRunningTasksCount().hashCode());
hashCode = prime * hashCode + ((getPendingTasksCount() == null) ? 0 : getPendingTasksCount().hashCode());
hashCode = prime * hashCode + ((getActiveServicesCount() == null) ? 0 : getActiveServicesCount().hashCode());
return hashCode;
}
@Override
public Cluster clone() {
try {
return (Cluster) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.ecs.model.transform.ClusterMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}