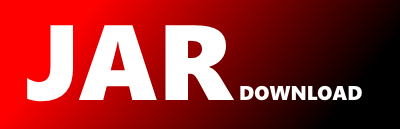
com.amazonaws.services.ecs.model.Service Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ecs Show documentation
/*
* Copyright 2011-2016 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ecs.model;
import java.io.Serializable;
/**
*
* Details on a service within a cluster
*
*/
public class Service implements Serializable, Cloneable {
/**
*
* The Amazon Resource Name (ARN) that identifies the service. The ARN contains the arn:aws:ecs
* namespace, followed by the region of the service, the AWS account ID of the service owner, the
* service
namespace, and then the service name. For example,
* arn:aws:ecs:region:012345678910:service/my-service
.
*
*/
private String serviceArn;
/**
*
* The name of your service. Up to 255 letters (uppercase and lowercase), numbers, hyphens, and underscores are
* allowed. Service names must be unique within a cluster, but you can have similarly named services in multiple
* clusters within a region or across multiple regions.
*
*/
private String serviceName;
/**
*
* The Amazon Resource Name (ARN) of the cluster that hosts the service.
*
*/
private String clusterArn;
/**
*
* A list of Elastic Load Balancing load balancer objects, containing the load balancer name, the container name (as
* it appears in a container definition), and the container port to access from the load balancer.
*
*/
private com.amazonaws.internal.SdkInternalList loadBalancers;
/**
*
* The status of the service. The valid values are ACTIVE
, DRAINING
, or
* INACTIVE
.
*
*/
private String status;
/**
*
* The desired number of instantiations of the task definition to keep running on the service. This value is
* specified when the service is created with CreateService, and it can be modified with
* UpdateService.
*
*/
private Integer desiredCount;
/**
*
* The number of tasks in the cluster that are in the RUNNING
state.
*
*/
private Integer runningCount;
/**
*
* The number of tasks in the cluster that are in the PENDING
state.
*
*/
private Integer pendingCount;
/**
*
* The task definition to use for tasks in the service. This value is specified when the service is created with
* CreateService, and it can be modified with UpdateService.
*
*/
private String taskDefinition;
/**
*
* Optional deployment parameters that control how many tasks run during the deployment and the ordering of stopping
* and starting tasks.
*
*/
private DeploymentConfiguration deploymentConfiguration;
/**
*
* The current state of deployments for the service.
*
*/
private com.amazonaws.internal.SdkInternalList deployments;
/**
*
* The Amazon Resource Name (ARN) of the IAM role associated with the service that allows the Amazon ECS container
* agent to register container instances with an Elastic Load Balancing load balancer.
*
*/
private String roleArn;
/**
*
* The event stream for your service. A maximum of 100 of the latest events are displayed.
*
*/
private com.amazonaws.internal.SdkInternalList events;
/**
*
* The Unix timestamp for when the service was created.
*
*/
private java.util.Date createdAt;
/**
*
* The Amazon Resource Name (ARN) that identifies the service. The ARN contains the arn:aws:ecs
* namespace, followed by the region of the service, the AWS account ID of the service owner, the
* service
namespace, and then the service name. For example,
* arn:aws:ecs:region:012345678910:service/my-service
.
*
*
* @param serviceArn
* The Amazon Resource Name (ARN) that identifies the service. The ARN contains the arn:aws:ecs
* namespace, followed by the region of the service, the AWS account ID of the service owner, the
* service
namespace, and then the service name. For example,
* arn:aws:ecs:region:012345678910:service/my-service
.
*/
public void setServiceArn(String serviceArn) {
this.serviceArn = serviceArn;
}
/**
*
* The Amazon Resource Name (ARN) that identifies the service. The ARN contains the arn:aws:ecs
* namespace, followed by the region of the service, the AWS account ID of the service owner, the
* service
namespace, and then the service name. For example,
* arn:aws:ecs:region:012345678910:service/my-service
.
*
*
* @return The Amazon Resource Name (ARN) that identifies the service. The ARN contains the arn:aws:ecs
* namespace, followed by the region of the service, the AWS account ID of the service owner, the
* service
namespace, and then the service name. For example,
* arn:aws:ecs:region:012345678910:service/my-service
.
*/
public String getServiceArn() {
return this.serviceArn;
}
/**
*
* The Amazon Resource Name (ARN) that identifies the service. The ARN contains the arn:aws:ecs
* namespace, followed by the region of the service, the AWS account ID of the service owner, the
* service
namespace, and then the service name. For example,
* arn:aws:ecs:region:012345678910:service/my-service
.
*
*
* @param serviceArn
* The Amazon Resource Name (ARN) that identifies the service. The ARN contains the arn:aws:ecs
* namespace, followed by the region of the service, the AWS account ID of the service owner, the
* service
namespace, and then the service name. For example,
* arn:aws:ecs:region:012345678910:service/my-service
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withServiceArn(String serviceArn) {
setServiceArn(serviceArn);
return this;
}
/**
*
* The name of your service. Up to 255 letters (uppercase and lowercase), numbers, hyphens, and underscores are
* allowed. Service names must be unique within a cluster, but you can have similarly named services in multiple
* clusters within a region or across multiple regions.
*
*
* @param serviceName
* The name of your service. Up to 255 letters (uppercase and lowercase), numbers, hyphens, and underscores
* are allowed. Service names must be unique within a cluster, but you can have similarly named services in
* multiple clusters within a region or across multiple regions.
*/
public void setServiceName(String serviceName) {
this.serviceName = serviceName;
}
/**
*
* The name of your service. Up to 255 letters (uppercase and lowercase), numbers, hyphens, and underscores are
* allowed. Service names must be unique within a cluster, but you can have similarly named services in multiple
* clusters within a region or across multiple regions.
*
*
* @return The name of your service. Up to 255 letters (uppercase and lowercase), numbers, hyphens, and underscores
* are allowed. Service names must be unique within a cluster, but you can have similarly named services in
* multiple clusters within a region or across multiple regions.
*/
public String getServiceName() {
return this.serviceName;
}
/**
*
* The name of your service. Up to 255 letters (uppercase and lowercase), numbers, hyphens, and underscores are
* allowed. Service names must be unique within a cluster, but you can have similarly named services in multiple
* clusters within a region or across multiple regions.
*
*
* @param serviceName
* The name of your service. Up to 255 letters (uppercase and lowercase), numbers, hyphens, and underscores
* are allowed. Service names must be unique within a cluster, but you can have similarly named services in
* multiple clusters within a region or across multiple regions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withServiceName(String serviceName) {
setServiceName(serviceName);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the cluster that hosts the service.
*
*
* @param clusterArn
* The Amazon Resource Name (ARN) of the cluster that hosts the service.
*/
public void setClusterArn(String clusterArn) {
this.clusterArn = clusterArn;
}
/**
*
* The Amazon Resource Name (ARN) of the cluster that hosts the service.
*
*
* @return The Amazon Resource Name (ARN) of the cluster that hosts the service.
*/
public String getClusterArn() {
return this.clusterArn;
}
/**
*
* The Amazon Resource Name (ARN) of the cluster that hosts the service.
*
*
* @param clusterArn
* The Amazon Resource Name (ARN) of the cluster that hosts the service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withClusterArn(String clusterArn) {
setClusterArn(clusterArn);
return this;
}
/**
*
* A list of Elastic Load Balancing load balancer objects, containing the load balancer name, the container name (as
* it appears in a container definition), and the container port to access from the load balancer.
*
*
* @return A list of Elastic Load Balancing load balancer objects, containing the load balancer name, the container
* name (as it appears in a container definition), and the container port to access from the load balancer.
*/
public java.util.List getLoadBalancers() {
if (loadBalancers == null) {
loadBalancers = new com.amazonaws.internal.SdkInternalList();
}
return loadBalancers;
}
/**
*
* A list of Elastic Load Balancing load balancer objects, containing the load balancer name, the container name (as
* it appears in a container definition), and the container port to access from the load balancer.
*
*
* @param loadBalancers
* A list of Elastic Load Balancing load balancer objects, containing the load balancer name, the container
* name (as it appears in a container definition), and the container port to access from the load balancer.
*/
public void setLoadBalancers(java.util.Collection loadBalancers) {
if (loadBalancers == null) {
this.loadBalancers = null;
return;
}
this.loadBalancers = new com.amazonaws.internal.SdkInternalList(loadBalancers);
}
/**
*
* A list of Elastic Load Balancing load balancer objects, containing the load balancer name, the container name (as
* it appears in a container definition), and the container port to access from the load balancer.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setLoadBalancers(java.util.Collection)} or {@link #withLoadBalancers(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param loadBalancers
* A list of Elastic Load Balancing load balancer objects, containing the load balancer name, the container
* name (as it appears in a container definition), and the container port to access from the load balancer.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withLoadBalancers(LoadBalancer... loadBalancers) {
if (this.loadBalancers == null) {
setLoadBalancers(new com.amazonaws.internal.SdkInternalList(loadBalancers.length));
}
for (LoadBalancer ele : loadBalancers) {
this.loadBalancers.add(ele);
}
return this;
}
/**
*
* A list of Elastic Load Balancing load balancer objects, containing the load balancer name, the container name (as
* it appears in a container definition), and the container port to access from the load balancer.
*
*
* @param loadBalancers
* A list of Elastic Load Balancing load balancer objects, containing the load balancer name, the container
* name (as it appears in a container definition), and the container port to access from the load balancer.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withLoadBalancers(java.util.Collection loadBalancers) {
setLoadBalancers(loadBalancers);
return this;
}
/**
*
* The status of the service. The valid values are ACTIVE
, DRAINING
, or
* INACTIVE
.
*
*
* @param status
* The status of the service. The valid values are ACTIVE
, DRAINING
, or
* INACTIVE
.
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the service. The valid values are ACTIVE
, DRAINING
, or
* INACTIVE
.
*
*
* @return The status of the service. The valid values are ACTIVE
, DRAINING
, or
* INACTIVE
.
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of the service. The valid values are ACTIVE
, DRAINING
, or
* INACTIVE
.
*
*
* @param status
* The status of the service. The valid values are ACTIVE
, DRAINING
, or
* INACTIVE
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The desired number of instantiations of the task definition to keep running on the service. This value is
* specified when the service is created with CreateService, and it can be modified with
* UpdateService.
*
*
* @param desiredCount
* The desired number of instantiations of the task definition to keep running on the service. This value is
* specified when the service is created with CreateService, and it can be modified with
* UpdateService.
*/
public void setDesiredCount(Integer desiredCount) {
this.desiredCount = desiredCount;
}
/**
*
* The desired number of instantiations of the task definition to keep running on the service. This value is
* specified when the service is created with CreateService, and it can be modified with
* UpdateService.
*
*
* @return The desired number of instantiations of the task definition to keep running on the service. This value is
* specified when the service is created with CreateService, and it can be modified with
* UpdateService.
*/
public Integer getDesiredCount() {
return this.desiredCount;
}
/**
*
* The desired number of instantiations of the task definition to keep running on the service. This value is
* specified when the service is created with CreateService, and it can be modified with
* UpdateService.
*
*
* @param desiredCount
* The desired number of instantiations of the task definition to keep running on the service. This value is
* specified when the service is created with CreateService, and it can be modified with
* UpdateService.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withDesiredCount(Integer desiredCount) {
setDesiredCount(desiredCount);
return this;
}
/**
*
* The number of tasks in the cluster that are in the RUNNING
state.
*
*
* @param runningCount
* The number of tasks in the cluster that are in the RUNNING
state.
*/
public void setRunningCount(Integer runningCount) {
this.runningCount = runningCount;
}
/**
*
* The number of tasks in the cluster that are in the RUNNING
state.
*
*
* @return The number of tasks in the cluster that are in the RUNNING
state.
*/
public Integer getRunningCount() {
return this.runningCount;
}
/**
*
* The number of tasks in the cluster that are in the RUNNING
state.
*
*
* @param runningCount
* The number of tasks in the cluster that are in the RUNNING
state.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withRunningCount(Integer runningCount) {
setRunningCount(runningCount);
return this;
}
/**
*
* The number of tasks in the cluster that are in the PENDING
state.
*
*
* @param pendingCount
* The number of tasks in the cluster that are in the PENDING
state.
*/
public void setPendingCount(Integer pendingCount) {
this.pendingCount = pendingCount;
}
/**
*
* The number of tasks in the cluster that are in the PENDING
state.
*
*
* @return The number of tasks in the cluster that are in the PENDING
state.
*/
public Integer getPendingCount() {
return this.pendingCount;
}
/**
*
* The number of tasks in the cluster that are in the PENDING
state.
*
*
* @param pendingCount
* The number of tasks in the cluster that are in the PENDING
state.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withPendingCount(Integer pendingCount) {
setPendingCount(pendingCount);
return this;
}
/**
*
* The task definition to use for tasks in the service. This value is specified when the service is created with
* CreateService, and it can be modified with UpdateService.
*
*
* @param taskDefinition
* The task definition to use for tasks in the service. This value is specified when the service is created
* with CreateService, and it can be modified with UpdateService.
*/
public void setTaskDefinition(String taskDefinition) {
this.taskDefinition = taskDefinition;
}
/**
*
* The task definition to use for tasks in the service. This value is specified when the service is created with
* CreateService, and it can be modified with UpdateService.
*
*
* @return The task definition to use for tasks in the service. This value is specified when the service is created
* with CreateService, and it can be modified with UpdateService.
*/
public String getTaskDefinition() {
return this.taskDefinition;
}
/**
*
* The task definition to use for tasks in the service. This value is specified when the service is created with
* CreateService, and it can be modified with UpdateService.
*
*
* @param taskDefinition
* The task definition to use for tasks in the service. This value is specified when the service is created
* with CreateService, and it can be modified with UpdateService.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withTaskDefinition(String taskDefinition) {
setTaskDefinition(taskDefinition);
return this;
}
/**
*
* Optional deployment parameters that control how many tasks run during the deployment and the ordering of stopping
* and starting tasks.
*
*
* @param deploymentConfiguration
* Optional deployment parameters that control how many tasks run during the deployment and the ordering of
* stopping and starting tasks.
*/
public void setDeploymentConfiguration(DeploymentConfiguration deploymentConfiguration) {
this.deploymentConfiguration = deploymentConfiguration;
}
/**
*
* Optional deployment parameters that control how many tasks run during the deployment and the ordering of stopping
* and starting tasks.
*
*
* @return Optional deployment parameters that control how many tasks run during the deployment and the ordering of
* stopping and starting tasks.
*/
public DeploymentConfiguration getDeploymentConfiguration() {
return this.deploymentConfiguration;
}
/**
*
* Optional deployment parameters that control how many tasks run during the deployment and the ordering of stopping
* and starting tasks.
*
*
* @param deploymentConfiguration
* Optional deployment parameters that control how many tasks run during the deployment and the ordering of
* stopping and starting tasks.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withDeploymentConfiguration(DeploymentConfiguration deploymentConfiguration) {
setDeploymentConfiguration(deploymentConfiguration);
return this;
}
/**
*
* The current state of deployments for the service.
*
*
* @return The current state of deployments for the service.
*/
public java.util.List getDeployments() {
if (deployments == null) {
deployments = new com.amazonaws.internal.SdkInternalList();
}
return deployments;
}
/**
*
* The current state of deployments for the service.
*
*
* @param deployments
* The current state of deployments for the service.
*/
public void setDeployments(java.util.Collection deployments) {
if (deployments == null) {
this.deployments = null;
return;
}
this.deployments = new com.amazonaws.internal.SdkInternalList(deployments);
}
/**
*
* The current state of deployments for the service.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDeployments(java.util.Collection)} or {@link #withDeployments(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param deployments
* The current state of deployments for the service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withDeployments(Deployment... deployments) {
if (this.deployments == null) {
setDeployments(new com.amazonaws.internal.SdkInternalList(deployments.length));
}
for (Deployment ele : deployments) {
this.deployments.add(ele);
}
return this;
}
/**
*
* The current state of deployments for the service.
*
*
* @param deployments
* The current state of deployments for the service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withDeployments(java.util.Collection deployments) {
setDeployments(deployments);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role associated with the service that allows the Amazon ECS container
* agent to register container instances with an Elastic Load Balancing load balancer.
*
*
* @param roleArn
* The Amazon Resource Name (ARN) of the IAM role associated with the service that allows the Amazon ECS
* container agent to register container instances with an Elastic Load Balancing load balancer.
*/
public void setRoleArn(String roleArn) {
this.roleArn = roleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role associated with the service that allows the Amazon ECS container
* agent to register container instances with an Elastic Load Balancing load balancer.
*
*
* @return The Amazon Resource Name (ARN) of the IAM role associated with the service that allows the Amazon ECS
* container agent to register container instances with an Elastic Load Balancing load balancer.
*/
public String getRoleArn() {
return this.roleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role associated with the service that allows the Amazon ECS container
* agent to register container instances with an Elastic Load Balancing load balancer.
*
*
* @param roleArn
* The Amazon Resource Name (ARN) of the IAM role associated with the service that allows the Amazon ECS
* container agent to register container instances with an Elastic Load Balancing load balancer.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withRoleArn(String roleArn) {
setRoleArn(roleArn);
return this;
}
/**
*
* The event stream for your service. A maximum of 100 of the latest events are displayed.
*
*
* @return The event stream for your service. A maximum of 100 of the latest events are displayed.
*/
public java.util.List getEvents() {
if (events == null) {
events = new com.amazonaws.internal.SdkInternalList();
}
return events;
}
/**
*
* The event stream for your service. A maximum of 100 of the latest events are displayed.
*
*
* @param events
* The event stream for your service. A maximum of 100 of the latest events are displayed.
*/
public void setEvents(java.util.Collection events) {
if (events == null) {
this.events = null;
return;
}
this.events = new com.amazonaws.internal.SdkInternalList(events);
}
/**
*
* The event stream for your service. A maximum of 100 of the latest events are displayed.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setEvents(java.util.Collection)} or {@link #withEvents(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param events
* The event stream for your service. A maximum of 100 of the latest events are displayed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withEvents(ServiceEvent... events) {
if (this.events == null) {
setEvents(new com.amazonaws.internal.SdkInternalList(events.length));
}
for (ServiceEvent ele : events) {
this.events.add(ele);
}
return this;
}
/**
*
* The event stream for your service. A maximum of 100 of the latest events are displayed.
*
*
* @param events
* The event stream for your service. A maximum of 100 of the latest events are displayed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withEvents(java.util.Collection events) {
setEvents(events);
return this;
}
/**
*
* The Unix timestamp for when the service was created.
*
*
* @param createdAt
* The Unix timestamp for when the service was created.
*/
public void setCreatedAt(java.util.Date createdAt) {
this.createdAt = createdAt;
}
/**
*
* The Unix timestamp for when the service was created.
*
*
* @return The Unix timestamp for when the service was created.
*/
public java.util.Date getCreatedAt() {
return this.createdAt;
}
/**
*
* The Unix timestamp for when the service was created.
*
*
* @param createdAt
* The Unix timestamp for when the service was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withCreatedAt(java.util.Date createdAt) {
setCreatedAt(createdAt);
return this;
}
/**
* Returns a string representation of this object; useful for testing and debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getServiceArn() != null)
sb.append("ServiceArn: " + getServiceArn() + ",");
if (getServiceName() != null)
sb.append("ServiceName: " + getServiceName() + ",");
if (getClusterArn() != null)
sb.append("ClusterArn: " + getClusterArn() + ",");
if (getLoadBalancers() != null)
sb.append("LoadBalancers: " + getLoadBalancers() + ",");
if (getStatus() != null)
sb.append("Status: " + getStatus() + ",");
if (getDesiredCount() != null)
sb.append("DesiredCount: " + getDesiredCount() + ",");
if (getRunningCount() != null)
sb.append("RunningCount: " + getRunningCount() + ",");
if (getPendingCount() != null)
sb.append("PendingCount: " + getPendingCount() + ",");
if (getTaskDefinition() != null)
sb.append("TaskDefinition: " + getTaskDefinition() + ",");
if (getDeploymentConfiguration() != null)
sb.append("DeploymentConfiguration: " + getDeploymentConfiguration() + ",");
if (getDeployments() != null)
sb.append("Deployments: " + getDeployments() + ",");
if (getRoleArn() != null)
sb.append("RoleArn: " + getRoleArn() + ",");
if (getEvents() != null)
sb.append("Events: " + getEvents() + ",");
if (getCreatedAt() != null)
sb.append("CreatedAt: " + getCreatedAt());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Service == false)
return false;
Service other = (Service) obj;
if (other.getServiceArn() == null ^ this.getServiceArn() == null)
return false;
if (other.getServiceArn() != null && other.getServiceArn().equals(this.getServiceArn()) == false)
return false;
if (other.getServiceName() == null ^ this.getServiceName() == null)
return false;
if (other.getServiceName() != null && other.getServiceName().equals(this.getServiceName()) == false)
return false;
if (other.getClusterArn() == null ^ this.getClusterArn() == null)
return false;
if (other.getClusterArn() != null && other.getClusterArn().equals(this.getClusterArn()) == false)
return false;
if (other.getLoadBalancers() == null ^ this.getLoadBalancers() == null)
return false;
if (other.getLoadBalancers() != null && other.getLoadBalancers().equals(this.getLoadBalancers()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getDesiredCount() == null ^ this.getDesiredCount() == null)
return false;
if (other.getDesiredCount() != null && other.getDesiredCount().equals(this.getDesiredCount()) == false)
return false;
if (other.getRunningCount() == null ^ this.getRunningCount() == null)
return false;
if (other.getRunningCount() != null && other.getRunningCount().equals(this.getRunningCount()) == false)
return false;
if (other.getPendingCount() == null ^ this.getPendingCount() == null)
return false;
if (other.getPendingCount() != null && other.getPendingCount().equals(this.getPendingCount()) == false)
return false;
if (other.getTaskDefinition() == null ^ this.getTaskDefinition() == null)
return false;
if (other.getTaskDefinition() != null && other.getTaskDefinition().equals(this.getTaskDefinition()) == false)
return false;
if (other.getDeploymentConfiguration() == null ^ this.getDeploymentConfiguration() == null)
return false;
if (other.getDeploymentConfiguration() != null && other.getDeploymentConfiguration().equals(this.getDeploymentConfiguration()) == false)
return false;
if (other.getDeployments() == null ^ this.getDeployments() == null)
return false;
if (other.getDeployments() != null && other.getDeployments().equals(this.getDeployments()) == false)
return false;
if (other.getRoleArn() == null ^ this.getRoleArn() == null)
return false;
if (other.getRoleArn() != null && other.getRoleArn().equals(this.getRoleArn()) == false)
return false;
if (other.getEvents() == null ^ this.getEvents() == null)
return false;
if (other.getEvents() != null && other.getEvents().equals(this.getEvents()) == false)
return false;
if (other.getCreatedAt() == null ^ this.getCreatedAt() == null)
return false;
if (other.getCreatedAt() != null && other.getCreatedAt().equals(this.getCreatedAt()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getServiceArn() == null) ? 0 : getServiceArn().hashCode());
hashCode = prime * hashCode + ((getServiceName() == null) ? 0 : getServiceName().hashCode());
hashCode = prime * hashCode + ((getClusterArn() == null) ? 0 : getClusterArn().hashCode());
hashCode = prime * hashCode + ((getLoadBalancers() == null) ? 0 : getLoadBalancers().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getDesiredCount() == null) ? 0 : getDesiredCount().hashCode());
hashCode = prime * hashCode + ((getRunningCount() == null) ? 0 : getRunningCount().hashCode());
hashCode = prime * hashCode + ((getPendingCount() == null) ? 0 : getPendingCount().hashCode());
hashCode = prime * hashCode + ((getTaskDefinition() == null) ? 0 : getTaskDefinition().hashCode());
hashCode = prime * hashCode + ((getDeploymentConfiguration() == null) ? 0 : getDeploymentConfiguration().hashCode());
hashCode = prime * hashCode + ((getDeployments() == null) ? 0 : getDeployments().hashCode());
hashCode = prime * hashCode + ((getRoleArn() == null) ? 0 : getRoleArn().hashCode());
hashCode = prime * hashCode + ((getEvents() == null) ? 0 : getEvents().hashCode());
hashCode = prime * hashCode + ((getCreatedAt() == null) ? 0 : getCreatedAt().hashCode());
return hashCode;
}
@Override
public Service clone() {
try {
return (Service) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}