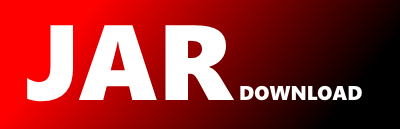
com.amazonaws.services.ecs.model.CapacityProvider Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ecs Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ecs.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The details of a capacity provider.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CapacityProvider implements Serializable, Cloneable, StructuredPojo {
/**
*
* The Amazon Resource Name (ARN) that identifies the capacity provider.
*
*/
private String capacityProviderArn;
/**
*
* The name of the capacity provider.
*
*/
private String name;
/**
*
* The current status of the capacity provider. Only capacity providers in an ACTIVE
state can be used
* in a cluster.
*
*/
private String status;
/**
*
* The Auto Scaling group settings for the capacity provider.
*
*/
private AutoScalingGroupProvider autoScalingGroupProvider;
/**
*
* The metadata that you apply to the capacity provider to help you categorize and organize it. Each tag consists of
* a key and an optional value, both of which you define.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* either keys or values as it is reserved for AWS use. You cannot edit or delete tag keys or values with this
* prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*
*/
private com.amazonaws.internal.SdkInternalList tags;
/**
*
* The Amazon Resource Name (ARN) that identifies the capacity provider.
*
*
* @param capacityProviderArn
* The Amazon Resource Name (ARN) that identifies the capacity provider.
*/
public void setCapacityProviderArn(String capacityProviderArn) {
this.capacityProviderArn = capacityProviderArn;
}
/**
*
* The Amazon Resource Name (ARN) that identifies the capacity provider.
*
*
* @return The Amazon Resource Name (ARN) that identifies the capacity provider.
*/
public String getCapacityProviderArn() {
return this.capacityProviderArn;
}
/**
*
* The Amazon Resource Name (ARN) that identifies the capacity provider.
*
*
* @param capacityProviderArn
* The Amazon Resource Name (ARN) that identifies the capacity provider.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CapacityProvider withCapacityProviderArn(String capacityProviderArn) {
setCapacityProviderArn(capacityProviderArn);
return this;
}
/**
*
* The name of the capacity provider.
*
*
* @param name
* The name of the capacity provider.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the capacity provider.
*
*
* @return The name of the capacity provider.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the capacity provider.
*
*
* @param name
* The name of the capacity provider.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CapacityProvider withName(String name) {
setName(name);
return this;
}
/**
*
* The current status of the capacity provider. Only capacity providers in an ACTIVE
state can be used
* in a cluster.
*
*
* @param status
* The current status of the capacity provider. Only capacity providers in an ACTIVE
state can
* be used in a cluster.
* @see CapacityProviderStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The current status of the capacity provider. Only capacity providers in an ACTIVE
state can be used
* in a cluster.
*
*
* @return The current status of the capacity provider. Only capacity providers in an ACTIVE
state can
* be used in a cluster.
* @see CapacityProviderStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The current status of the capacity provider. Only capacity providers in an ACTIVE
state can be used
* in a cluster.
*
*
* @param status
* The current status of the capacity provider. Only capacity providers in an ACTIVE
state can
* be used in a cluster.
* @return Returns a reference to this object so that method calls can be chained together.
* @see CapacityProviderStatus
*/
public CapacityProvider withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The current status of the capacity provider. Only capacity providers in an ACTIVE
state can be used
* in a cluster.
*
*
* @param status
* The current status of the capacity provider. Only capacity providers in an ACTIVE
state can
* be used in a cluster.
* @return Returns a reference to this object so that method calls can be chained together.
* @see CapacityProviderStatus
*/
public CapacityProvider withStatus(CapacityProviderStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The Auto Scaling group settings for the capacity provider.
*
*
* @param autoScalingGroupProvider
* The Auto Scaling group settings for the capacity provider.
*/
public void setAutoScalingGroupProvider(AutoScalingGroupProvider autoScalingGroupProvider) {
this.autoScalingGroupProvider = autoScalingGroupProvider;
}
/**
*
* The Auto Scaling group settings for the capacity provider.
*
*
* @return The Auto Scaling group settings for the capacity provider.
*/
public AutoScalingGroupProvider getAutoScalingGroupProvider() {
return this.autoScalingGroupProvider;
}
/**
*
* The Auto Scaling group settings for the capacity provider.
*
*
* @param autoScalingGroupProvider
* The Auto Scaling group settings for the capacity provider.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CapacityProvider withAutoScalingGroupProvider(AutoScalingGroupProvider autoScalingGroupProvider) {
setAutoScalingGroupProvider(autoScalingGroupProvider);
return this;
}
/**
*
* The metadata that you apply to the capacity provider to help you categorize and organize it. Each tag consists of
* a key and an optional value, both of which you define.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* either keys or values as it is reserved for AWS use. You cannot edit or delete tag keys or values with this
* prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*
*
* @return The metadata that you apply to the capacity provider to help you categorize and organize it. Each tag
* consists of a key and an optional value, both of which you define.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may
* have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a
* prefix for either keys or values as it is reserved for AWS use. You cannot edit or delete tag keys or
* values with this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*/
public java.util.List getTags() {
if (tags == null) {
tags = new com.amazonaws.internal.SdkInternalList();
}
return tags;
}
/**
*
* The metadata that you apply to the capacity provider to help you categorize and organize it. Each tag consists of
* a key and an optional value, both of which you define.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* either keys or values as it is reserved for AWS use. You cannot edit or delete tag keys or values with this
* prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*
*
* @param tags
* The metadata that you apply to the capacity provider to help you categorize and organize it. Each tag
* consists of a key and an optional value, both of which you define.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may
* have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix
* for either keys or values as it is reserved for AWS use. You cannot edit or delete tag keys or values with
* this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new com.amazonaws.internal.SdkInternalList(tags);
}
/**
*
* The metadata that you apply to the capacity provider to help you categorize and organize it. Each tag consists of
* a key and an optional value, both of which you define.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* either keys or values as it is reserved for AWS use. You cannot edit or delete tag keys or values with this
* prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* The metadata that you apply to the capacity provider to help you categorize and organize it. Each tag
* consists of a key and an optional value, both of which you define.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may
* have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix
* for either keys or values as it is reserved for AWS use. You cannot edit or delete tag keys or values with
* this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CapacityProvider withTags(Tag... tags) {
if (this.tags == null) {
setTags(new com.amazonaws.internal.SdkInternalList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* The metadata that you apply to the capacity provider to help you categorize and organize it. Each tag consists of
* a key and an optional value, both of which you define.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* either keys or values as it is reserved for AWS use. You cannot edit or delete tag keys or values with this
* prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*
*
* @param tags
* The metadata that you apply to the capacity provider to help you categorize and organize it. Each tag
* consists of a key and an optional value, both of which you define.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may
* have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix
* for either keys or values as it is reserved for AWS use. You cannot edit or delete tag keys or values with
* this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CapacityProvider withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCapacityProviderArn() != null)
sb.append("CapacityProviderArn: ").append(getCapacityProviderArn()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getAutoScalingGroupProvider() != null)
sb.append("AutoScalingGroupProvider: ").append(getAutoScalingGroupProvider()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CapacityProvider == false)
return false;
CapacityProvider other = (CapacityProvider) obj;
if (other.getCapacityProviderArn() == null ^ this.getCapacityProviderArn() == null)
return false;
if (other.getCapacityProviderArn() != null && other.getCapacityProviderArn().equals(this.getCapacityProviderArn()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getAutoScalingGroupProvider() == null ^ this.getAutoScalingGroupProvider() == null)
return false;
if (other.getAutoScalingGroupProvider() != null && other.getAutoScalingGroupProvider().equals(this.getAutoScalingGroupProvider()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCapacityProviderArn() == null) ? 0 : getCapacityProviderArn().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getAutoScalingGroupProvider() == null) ? 0 : getAutoScalingGroupProvider().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public CapacityProvider clone() {
try {
return (CapacityProvider) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.ecs.model.transform.CapacityProviderMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}