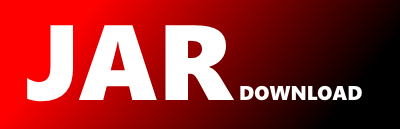
com.amazonaws.services.ecs.model.TaskSet Maven / Gradle / Ivy
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ecs.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Information about a set of Amazon ECS tasks in either an AWS CodeDeploy or an EXTERNAL
deployment. An
* Amazon ECS task set includes details such as the desired number of tasks, how many tasks are running, and whether the
* task set serves production traffic.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class TaskSet implements Serializable, Cloneable, StructuredPojo {
/**
*
* The ID of the task set.
*
*/
private String id;
/**
*
* The Amazon Resource Name (ARN) of the task set.
*
*/
private String taskSetArn;
/**
*
* The Amazon Resource Name (ARN) of the service the task set exists in.
*
*/
private String serviceArn;
/**
*
* The Amazon Resource Name (ARN) of the cluster that the service that hosts the task set exists in.
*
*/
private String clusterArn;
/**
*
* The tag specified when a task set is started. If the task set is created by an AWS CodeDeploy deployment, the
* startedBy
parameter is CODE_DEPLOY
. For a task set created for an external deployment,
* the startedBy field isn't used.
*
*/
private String startedBy;
/**
*
* The external ID associated with the task set.
*
*
* If a task set is created by an AWS CodeDeploy deployment, the externalId
parameter contains the AWS
* CodeDeploy deployment ID.
*
*
* If a task set is created for an external deployment and is associated with a service discovery registry, the
* externalId
parameter contains the ECS_TASK_SET_EXTERNAL_ID
AWS Cloud Map attribute.
*
*/
private String externalId;
/**
*
* The status of the task set. The following describes each state:
*
*
* - PRIMARY
* -
*
* The task set is serving production traffic.
*
*
* - ACTIVE
* -
*
* The task set is not serving production traffic.
*
*
* - DRAINING
* -
*
* The tasks in the task set are being stopped and their corresponding targets are being deregistered from their
* target group.
*
*
*
*/
private String status;
/**
*
* The task definition the task set is using.
*
*/
private String taskDefinition;
/**
*
* The computed desired count for the task set. This is calculated by multiplying the service's
* desiredCount
by the task set's scale
percentage. The result is always rounded up. For
* example, if the computed desired count is 1.2, it rounds up to 2 tasks.
*
*/
private Integer computedDesiredCount;
/**
*
* The number of tasks in the task set that are in the PENDING
status during a deployment. A task in
* the PENDING
state is preparing to enter the RUNNING
state. A task set enters the
* PENDING
status when it launches for the first time or when it is restarted after being in the
* STOPPED
state.
*
*/
private Integer pendingCount;
/**
*
* The number of tasks in the task set that are in the RUNNING
status during a deployment. A task in
* the RUNNING
state is running and ready for use.
*
*/
private Integer runningCount;
/**
*
* The Unix timestamp for when the task set was created.
*
*/
private java.util.Date createdAt;
/**
*
* The Unix timestamp for when the task set was last updated.
*
*/
private java.util.Date updatedAt;
/**
*
* The launch type the tasks in the task set are using. For more information, see Amazon ECS Launch Types
* in the Amazon Elastic Container Service Developer Guide.
*
*/
private String launchType;
/**
*
* The capacity provider strategy associated with the task set.
*
*/
private com.amazonaws.internal.SdkInternalList capacityProviderStrategy;
/**
*
* The platform version on which the tasks in the task set are running. A platform version is only specified for
* tasks using the Fargate launch type. If one is not specified, the LATEST
platform version is used by
* default. For more information, see AWS Fargate Platform
* Versions in the Amazon Elastic Container Service Developer Guide.
*
*/
private String platformVersion;
/**
*
* The network configuration for the task set.
*
*/
private NetworkConfiguration networkConfiguration;
/**
*
* Details on a load balancer that is used with a task set.
*
*/
private com.amazonaws.internal.SdkInternalList loadBalancers;
/**
*
* The details of the service discovery registries to assign to this task set. For more information, see Service Discovery.
*
*/
private com.amazonaws.internal.SdkInternalList serviceRegistries;
/**
*
* A floating-point percentage of the desired number of tasks to place and keep running in the task set.
*
*/
private Scale scale;
/**
*
* The stability status, which indicates whether the task set has reached a steady state. If the following
* conditions are met, the task set will be in STEADY_STATE
:
*
*
* -
*
* The task runningCount
is equal to the computedDesiredCount
.
*
*
* -
*
* The pendingCount
is 0
.
*
*
* -
*
* There are no tasks running on container instances in the DRAINING
status.
*
*
* -
*
* All tasks are reporting a healthy status from the load balancers, service discovery, and container health checks.
*
*
*
*
* If any of those conditions are not met, the stability status returns STABILIZING
.
*
*/
private String stabilityStatus;
/**
*
* The Unix timestamp for when the task set stability status was retrieved.
*
*/
private java.util.Date stabilityStatusAt;
/**
*
* The ID of the task set.
*
*
* @param id
* The ID of the task set.
*/
public void setId(String id) {
this.id = id;
}
/**
*
* The ID of the task set.
*
*
* @return The ID of the task set.
*/
public String getId() {
return this.id;
}
/**
*
* The ID of the task set.
*
*
* @param id
* The ID of the task set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskSet withId(String id) {
setId(id);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the task set.
*
*
* @param taskSetArn
* The Amazon Resource Name (ARN) of the task set.
*/
public void setTaskSetArn(String taskSetArn) {
this.taskSetArn = taskSetArn;
}
/**
*
* The Amazon Resource Name (ARN) of the task set.
*
*
* @return The Amazon Resource Name (ARN) of the task set.
*/
public String getTaskSetArn() {
return this.taskSetArn;
}
/**
*
* The Amazon Resource Name (ARN) of the task set.
*
*
* @param taskSetArn
* The Amazon Resource Name (ARN) of the task set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskSet withTaskSetArn(String taskSetArn) {
setTaskSetArn(taskSetArn);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the service the task set exists in.
*
*
* @param serviceArn
* The Amazon Resource Name (ARN) of the service the task set exists in.
*/
public void setServiceArn(String serviceArn) {
this.serviceArn = serviceArn;
}
/**
*
* The Amazon Resource Name (ARN) of the service the task set exists in.
*
*
* @return The Amazon Resource Name (ARN) of the service the task set exists in.
*/
public String getServiceArn() {
return this.serviceArn;
}
/**
*
* The Amazon Resource Name (ARN) of the service the task set exists in.
*
*
* @param serviceArn
* The Amazon Resource Name (ARN) of the service the task set exists in.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskSet withServiceArn(String serviceArn) {
setServiceArn(serviceArn);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the cluster that the service that hosts the task set exists in.
*
*
* @param clusterArn
* The Amazon Resource Name (ARN) of the cluster that the service that hosts the task set exists in.
*/
public void setClusterArn(String clusterArn) {
this.clusterArn = clusterArn;
}
/**
*
* The Amazon Resource Name (ARN) of the cluster that the service that hosts the task set exists in.
*
*
* @return The Amazon Resource Name (ARN) of the cluster that the service that hosts the task set exists in.
*/
public String getClusterArn() {
return this.clusterArn;
}
/**
*
* The Amazon Resource Name (ARN) of the cluster that the service that hosts the task set exists in.
*
*
* @param clusterArn
* The Amazon Resource Name (ARN) of the cluster that the service that hosts the task set exists in.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskSet withClusterArn(String clusterArn) {
setClusterArn(clusterArn);
return this;
}
/**
*
* The tag specified when a task set is started. If the task set is created by an AWS CodeDeploy deployment, the
* startedBy
parameter is CODE_DEPLOY
. For a task set created for an external deployment,
* the startedBy field isn't used.
*
*
* @param startedBy
* The tag specified when a task set is started. If the task set is created by an AWS CodeDeploy deployment,
* the startedBy
parameter is CODE_DEPLOY
. For a task set created for an external
* deployment, the startedBy field isn't used.
*/
public void setStartedBy(String startedBy) {
this.startedBy = startedBy;
}
/**
*
* The tag specified when a task set is started. If the task set is created by an AWS CodeDeploy deployment, the
* startedBy
parameter is CODE_DEPLOY
. For a task set created for an external deployment,
* the startedBy field isn't used.
*
*
* @return The tag specified when a task set is started. If the task set is created by an AWS CodeDeploy deployment,
* the startedBy
parameter is CODE_DEPLOY
. For a task set created for an external
* deployment, the startedBy field isn't used.
*/
public String getStartedBy() {
return this.startedBy;
}
/**
*
* The tag specified when a task set is started. If the task set is created by an AWS CodeDeploy deployment, the
* startedBy
parameter is CODE_DEPLOY
. For a task set created for an external deployment,
* the startedBy field isn't used.
*
*
* @param startedBy
* The tag specified when a task set is started. If the task set is created by an AWS CodeDeploy deployment,
* the startedBy
parameter is CODE_DEPLOY
. For a task set created for an external
* deployment, the startedBy field isn't used.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskSet withStartedBy(String startedBy) {
setStartedBy(startedBy);
return this;
}
/**
*
* The external ID associated with the task set.
*
*
* If a task set is created by an AWS CodeDeploy deployment, the externalId
parameter contains the AWS
* CodeDeploy deployment ID.
*
*
* If a task set is created for an external deployment and is associated with a service discovery registry, the
* externalId
parameter contains the ECS_TASK_SET_EXTERNAL_ID
AWS Cloud Map attribute.
*
*
* @param externalId
* The external ID associated with the task set.
*
* If a task set is created by an AWS CodeDeploy deployment, the externalId
parameter contains
* the AWS CodeDeploy deployment ID.
*
*
* If a task set is created for an external deployment and is associated with a service discovery registry,
* the externalId
parameter contains the ECS_TASK_SET_EXTERNAL_ID
AWS Cloud Map
* attribute.
*/
public void setExternalId(String externalId) {
this.externalId = externalId;
}
/**
*
* The external ID associated with the task set.
*
*
* If a task set is created by an AWS CodeDeploy deployment, the externalId
parameter contains the AWS
* CodeDeploy deployment ID.
*
*
* If a task set is created for an external deployment and is associated with a service discovery registry, the
* externalId
parameter contains the ECS_TASK_SET_EXTERNAL_ID
AWS Cloud Map attribute.
*
*
* @return The external ID associated with the task set.
*
* If a task set is created by an AWS CodeDeploy deployment, the externalId
parameter contains
* the AWS CodeDeploy deployment ID.
*
*
* If a task set is created for an external deployment and is associated with a service discovery registry,
* the externalId
parameter contains the ECS_TASK_SET_EXTERNAL_ID
AWS Cloud Map
* attribute.
*/
public String getExternalId() {
return this.externalId;
}
/**
*
* The external ID associated with the task set.
*
*
* If a task set is created by an AWS CodeDeploy deployment, the externalId
parameter contains the AWS
* CodeDeploy deployment ID.
*
*
* If a task set is created for an external deployment and is associated with a service discovery registry, the
* externalId
parameter contains the ECS_TASK_SET_EXTERNAL_ID
AWS Cloud Map attribute.
*
*
* @param externalId
* The external ID associated with the task set.
*
* If a task set is created by an AWS CodeDeploy deployment, the externalId
parameter contains
* the AWS CodeDeploy deployment ID.
*
*
* If a task set is created for an external deployment and is associated with a service discovery registry,
* the externalId
parameter contains the ECS_TASK_SET_EXTERNAL_ID
AWS Cloud Map
* attribute.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskSet withExternalId(String externalId) {
setExternalId(externalId);
return this;
}
/**
*
* The status of the task set. The following describes each state:
*
*
* - PRIMARY
* -
*
* The task set is serving production traffic.
*
*
* - ACTIVE
* -
*
* The task set is not serving production traffic.
*
*
* - DRAINING
* -
*
* The tasks in the task set are being stopped and their corresponding targets are being deregistered from their
* target group.
*
*
*
*
* @param status
* The status of the task set. The following describes each state:
*
* - PRIMARY
* -
*
* The task set is serving production traffic.
*
*
* - ACTIVE
* -
*
* The task set is not serving production traffic.
*
*
* - DRAINING
* -
*
* The tasks in the task set are being stopped and their corresponding targets are being deregistered from
* their target group.
*
*
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the task set. The following describes each state:
*
*
* - PRIMARY
* -
*
* The task set is serving production traffic.
*
*
* - ACTIVE
* -
*
* The task set is not serving production traffic.
*
*
* - DRAINING
* -
*
* The tasks in the task set are being stopped and their corresponding targets are being deregistered from their
* target group.
*
*
*
*
* @return The status of the task set. The following describes each state:
*
* - PRIMARY
* -
*
* The task set is serving production traffic.
*
*
* - ACTIVE
* -
*
* The task set is not serving production traffic.
*
*
* - DRAINING
* -
*
* The tasks in the task set are being stopped and their corresponding targets are being deregistered from
* their target group.
*
*
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of the task set. The following describes each state:
*
*
* - PRIMARY
* -
*
* The task set is serving production traffic.
*
*
* - ACTIVE
* -
*
* The task set is not serving production traffic.
*
*
* - DRAINING
* -
*
* The tasks in the task set are being stopped and their corresponding targets are being deregistered from their
* target group.
*
*
*
*
* @param status
* The status of the task set. The following describes each state:
*
* - PRIMARY
* -
*
* The task set is serving production traffic.
*
*
* - ACTIVE
* -
*
* The task set is not serving production traffic.
*
*
* - DRAINING
* -
*
* The tasks in the task set are being stopped and their corresponding targets are being deregistered from
* their target group.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskSet withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The task definition the task set is using.
*
*
* @param taskDefinition
* The task definition the task set is using.
*/
public void setTaskDefinition(String taskDefinition) {
this.taskDefinition = taskDefinition;
}
/**
*
* The task definition the task set is using.
*
*
* @return The task definition the task set is using.
*/
public String getTaskDefinition() {
return this.taskDefinition;
}
/**
*
* The task definition the task set is using.
*
*
* @param taskDefinition
* The task definition the task set is using.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskSet withTaskDefinition(String taskDefinition) {
setTaskDefinition(taskDefinition);
return this;
}
/**
*
* The computed desired count for the task set. This is calculated by multiplying the service's
* desiredCount
by the task set's scale
percentage. The result is always rounded up. For
* example, if the computed desired count is 1.2, it rounds up to 2 tasks.
*
*
* @param computedDesiredCount
* The computed desired count for the task set. This is calculated by multiplying the service's
* desiredCount
by the task set's scale
percentage. The result is always rounded
* up. For example, if the computed desired count is 1.2, it rounds up to 2 tasks.
*/
public void setComputedDesiredCount(Integer computedDesiredCount) {
this.computedDesiredCount = computedDesiredCount;
}
/**
*
* The computed desired count for the task set. This is calculated by multiplying the service's
* desiredCount
by the task set's scale
percentage. The result is always rounded up. For
* example, if the computed desired count is 1.2, it rounds up to 2 tasks.
*
*
* @return The computed desired count for the task set. This is calculated by multiplying the service's
* desiredCount
by the task set's scale
percentage. The result is always rounded
* up. For example, if the computed desired count is 1.2, it rounds up to 2 tasks.
*/
public Integer getComputedDesiredCount() {
return this.computedDesiredCount;
}
/**
*
* The computed desired count for the task set. This is calculated by multiplying the service's
* desiredCount
by the task set's scale
percentage. The result is always rounded up. For
* example, if the computed desired count is 1.2, it rounds up to 2 tasks.
*
*
* @param computedDesiredCount
* The computed desired count for the task set. This is calculated by multiplying the service's
* desiredCount
by the task set's scale
percentage. The result is always rounded
* up. For example, if the computed desired count is 1.2, it rounds up to 2 tasks.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskSet withComputedDesiredCount(Integer computedDesiredCount) {
setComputedDesiredCount(computedDesiredCount);
return this;
}
/**
*
* The number of tasks in the task set that are in the PENDING
status during a deployment. A task in
* the PENDING
state is preparing to enter the RUNNING
state. A task set enters the
* PENDING
status when it launches for the first time or when it is restarted after being in the
* STOPPED
state.
*
*
* @param pendingCount
* The number of tasks in the task set that are in the PENDING
status during a deployment. A
* task in the PENDING
state is preparing to enter the RUNNING
state. A task set
* enters the PENDING
status when it launches for the first time or when it is restarted after
* being in the STOPPED
state.
*/
public void setPendingCount(Integer pendingCount) {
this.pendingCount = pendingCount;
}
/**
*
* The number of tasks in the task set that are in the PENDING
status during a deployment. A task in
* the PENDING
state is preparing to enter the RUNNING
state. A task set enters the
* PENDING
status when it launches for the first time or when it is restarted after being in the
* STOPPED
state.
*
*
* @return The number of tasks in the task set that are in the PENDING
status during a deployment. A
* task in the PENDING
state is preparing to enter the RUNNING
state. A task set
* enters the PENDING
status when it launches for the first time or when it is restarted after
* being in the STOPPED
state.
*/
public Integer getPendingCount() {
return this.pendingCount;
}
/**
*
* The number of tasks in the task set that are in the PENDING
status during a deployment. A task in
* the PENDING
state is preparing to enter the RUNNING
state. A task set enters the
* PENDING
status when it launches for the first time or when it is restarted after being in the
* STOPPED
state.
*
*
* @param pendingCount
* The number of tasks in the task set that are in the PENDING
status during a deployment. A
* task in the PENDING
state is preparing to enter the RUNNING
state. A task set
* enters the PENDING
status when it launches for the first time or when it is restarted after
* being in the STOPPED
state.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskSet withPendingCount(Integer pendingCount) {
setPendingCount(pendingCount);
return this;
}
/**
*
* The number of tasks in the task set that are in the RUNNING
status during a deployment. A task in
* the RUNNING
state is running and ready for use.
*
*
* @param runningCount
* The number of tasks in the task set that are in the RUNNING
status during a deployment. A
* task in the RUNNING
state is running and ready for use.
*/
public void setRunningCount(Integer runningCount) {
this.runningCount = runningCount;
}
/**
*
* The number of tasks in the task set that are in the RUNNING
status during a deployment. A task in
* the RUNNING
state is running and ready for use.
*
*
* @return The number of tasks in the task set that are in the RUNNING
status during a deployment. A
* task in the RUNNING
state is running and ready for use.
*/
public Integer getRunningCount() {
return this.runningCount;
}
/**
*
* The number of tasks in the task set that are in the RUNNING
status during a deployment. A task in
* the RUNNING
state is running and ready for use.
*
*
* @param runningCount
* The number of tasks in the task set that are in the RUNNING
status during a deployment. A
* task in the RUNNING
state is running and ready for use.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskSet withRunningCount(Integer runningCount) {
setRunningCount(runningCount);
return this;
}
/**
*
* The Unix timestamp for when the task set was created.
*
*
* @param createdAt
* The Unix timestamp for when the task set was created.
*/
public void setCreatedAt(java.util.Date createdAt) {
this.createdAt = createdAt;
}
/**
*
* The Unix timestamp for when the task set was created.
*
*
* @return The Unix timestamp for when the task set was created.
*/
public java.util.Date getCreatedAt() {
return this.createdAt;
}
/**
*
* The Unix timestamp for when the task set was created.
*
*
* @param createdAt
* The Unix timestamp for when the task set was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskSet withCreatedAt(java.util.Date createdAt) {
setCreatedAt(createdAt);
return this;
}
/**
*
* The Unix timestamp for when the task set was last updated.
*
*
* @param updatedAt
* The Unix timestamp for when the task set was last updated.
*/
public void setUpdatedAt(java.util.Date updatedAt) {
this.updatedAt = updatedAt;
}
/**
*
* The Unix timestamp for when the task set was last updated.
*
*
* @return The Unix timestamp for when the task set was last updated.
*/
public java.util.Date getUpdatedAt() {
return this.updatedAt;
}
/**
*
* The Unix timestamp for when the task set was last updated.
*
*
* @param updatedAt
* The Unix timestamp for when the task set was last updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskSet withUpdatedAt(java.util.Date updatedAt) {
setUpdatedAt(updatedAt);
return this;
}
/**
*
* The launch type the tasks in the task set are using. For more information, see Amazon ECS Launch Types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* @param launchType
* The launch type the tasks in the task set are using. For more information, see Amazon ECS Launch
* Types in the Amazon Elastic Container Service Developer Guide.
* @see LaunchType
*/
public void setLaunchType(String launchType) {
this.launchType = launchType;
}
/**
*
* The launch type the tasks in the task set are using. For more information, see Amazon ECS Launch Types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* @return The launch type the tasks in the task set are using. For more information, see Amazon ECS Launch
* Types in the Amazon Elastic Container Service Developer Guide.
* @see LaunchType
*/
public String getLaunchType() {
return this.launchType;
}
/**
*
* The launch type the tasks in the task set are using. For more information, see Amazon ECS Launch Types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* @param launchType
* The launch type the tasks in the task set are using. For more information, see Amazon ECS Launch
* Types in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LaunchType
*/
public TaskSet withLaunchType(String launchType) {
setLaunchType(launchType);
return this;
}
/**
*
* The launch type the tasks in the task set are using. For more information, see Amazon ECS Launch Types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* @param launchType
* The launch type the tasks in the task set are using. For more information, see Amazon ECS Launch
* Types in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LaunchType
*/
public TaskSet withLaunchType(LaunchType launchType) {
this.launchType = launchType.toString();
return this;
}
/**
*
* The capacity provider strategy associated with the task set.
*
*
* @return The capacity provider strategy associated with the task set.
*/
public java.util.List getCapacityProviderStrategy() {
if (capacityProviderStrategy == null) {
capacityProviderStrategy = new com.amazonaws.internal.SdkInternalList();
}
return capacityProviderStrategy;
}
/**
*
* The capacity provider strategy associated with the task set.
*
*
* @param capacityProviderStrategy
* The capacity provider strategy associated with the task set.
*/
public void setCapacityProviderStrategy(java.util.Collection capacityProviderStrategy) {
if (capacityProviderStrategy == null) {
this.capacityProviderStrategy = null;
return;
}
this.capacityProviderStrategy = new com.amazonaws.internal.SdkInternalList(capacityProviderStrategy);
}
/**
*
* The capacity provider strategy associated with the task set.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCapacityProviderStrategy(java.util.Collection)} or
* {@link #withCapacityProviderStrategy(java.util.Collection)} if you want to override the existing values.
*
*
* @param capacityProviderStrategy
* The capacity provider strategy associated with the task set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskSet withCapacityProviderStrategy(CapacityProviderStrategyItem... capacityProviderStrategy) {
if (this.capacityProviderStrategy == null) {
setCapacityProviderStrategy(new com.amazonaws.internal.SdkInternalList(capacityProviderStrategy.length));
}
for (CapacityProviderStrategyItem ele : capacityProviderStrategy) {
this.capacityProviderStrategy.add(ele);
}
return this;
}
/**
*
* The capacity provider strategy associated with the task set.
*
*
* @param capacityProviderStrategy
* The capacity provider strategy associated with the task set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskSet withCapacityProviderStrategy(java.util.Collection capacityProviderStrategy) {
setCapacityProviderStrategy(capacityProviderStrategy);
return this;
}
/**
*
* The platform version on which the tasks in the task set are running. A platform version is only specified for
* tasks using the Fargate launch type. If one is not specified, the LATEST
platform version is used by
* default. For more information, see AWS Fargate Platform
* Versions in the Amazon Elastic Container Service Developer Guide.
*
*
* @param platformVersion
* The platform version on which the tasks in the task set are running. A platform version is only specified
* for tasks using the Fargate launch type. If one is not specified, the LATEST
platform version
* is used by default. For more information, see AWS Fargate
* Platform Versions in the Amazon Elastic Container Service Developer Guide.
*/
public void setPlatformVersion(String platformVersion) {
this.platformVersion = platformVersion;
}
/**
*
* The platform version on which the tasks in the task set are running. A platform version is only specified for
* tasks using the Fargate launch type. If one is not specified, the LATEST
platform version is used by
* default. For more information, see AWS Fargate Platform
* Versions in the Amazon Elastic Container Service Developer Guide.
*
*
* @return The platform version on which the tasks in the task set are running. A platform version is only specified
* for tasks using the Fargate launch type. If one is not specified, the LATEST
platform
* version is used by default. For more information, see AWS Fargate
* Platform Versions in the Amazon Elastic Container Service Developer Guide.
*/
public String getPlatformVersion() {
return this.platformVersion;
}
/**
*
* The platform version on which the tasks in the task set are running. A platform version is only specified for
* tasks using the Fargate launch type. If one is not specified, the LATEST
platform version is used by
* default. For more information, see AWS Fargate Platform
* Versions in the Amazon Elastic Container Service Developer Guide.
*
*
* @param platformVersion
* The platform version on which the tasks in the task set are running. A platform version is only specified
* for tasks using the Fargate launch type. If one is not specified, the LATEST
platform version
* is used by default. For more information, see AWS Fargate
* Platform Versions in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskSet withPlatformVersion(String platformVersion) {
setPlatformVersion(platformVersion);
return this;
}
/**
*
* The network configuration for the task set.
*
*
* @param networkConfiguration
* The network configuration for the task set.
*/
public void setNetworkConfiguration(NetworkConfiguration networkConfiguration) {
this.networkConfiguration = networkConfiguration;
}
/**
*
* The network configuration for the task set.
*
*
* @return The network configuration for the task set.
*/
public NetworkConfiguration getNetworkConfiguration() {
return this.networkConfiguration;
}
/**
*
* The network configuration for the task set.
*
*
* @param networkConfiguration
* The network configuration for the task set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskSet withNetworkConfiguration(NetworkConfiguration networkConfiguration) {
setNetworkConfiguration(networkConfiguration);
return this;
}
/**
*
* Details on a load balancer that is used with a task set.
*
*
* @return Details on a load balancer that is used with a task set.
*/
public java.util.List getLoadBalancers() {
if (loadBalancers == null) {
loadBalancers = new com.amazonaws.internal.SdkInternalList();
}
return loadBalancers;
}
/**
*
* Details on a load balancer that is used with a task set.
*
*
* @param loadBalancers
* Details on a load balancer that is used with a task set.
*/
public void setLoadBalancers(java.util.Collection loadBalancers) {
if (loadBalancers == null) {
this.loadBalancers = null;
return;
}
this.loadBalancers = new com.amazonaws.internal.SdkInternalList(loadBalancers);
}
/**
*
* Details on a load balancer that is used with a task set.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setLoadBalancers(java.util.Collection)} or {@link #withLoadBalancers(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param loadBalancers
* Details on a load balancer that is used with a task set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskSet withLoadBalancers(LoadBalancer... loadBalancers) {
if (this.loadBalancers == null) {
setLoadBalancers(new com.amazonaws.internal.SdkInternalList(loadBalancers.length));
}
for (LoadBalancer ele : loadBalancers) {
this.loadBalancers.add(ele);
}
return this;
}
/**
*
* Details on a load balancer that is used with a task set.
*
*
* @param loadBalancers
* Details on a load balancer that is used with a task set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskSet withLoadBalancers(java.util.Collection loadBalancers) {
setLoadBalancers(loadBalancers);
return this;
}
/**
*
* The details of the service discovery registries to assign to this task set. For more information, see Service Discovery.
*
*
* @return The details of the service discovery registries to assign to this task set. For more information, see Service
* Discovery.
*/
public java.util.List getServiceRegistries() {
if (serviceRegistries == null) {
serviceRegistries = new com.amazonaws.internal.SdkInternalList();
}
return serviceRegistries;
}
/**
*
* The details of the service discovery registries to assign to this task set. For more information, see Service Discovery.
*
*
* @param serviceRegistries
* The details of the service discovery registries to assign to this task set. For more information, see Service
* Discovery.
*/
public void setServiceRegistries(java.util.Collection serviceRegistries) {
if (serviceRegistries == null) {
this.serviceRegistries = null;
return;
}
this.serviceRegistries = new com.amazonaws.internal.SdkInternalList(serviceRegistries);
}
/**
*
* The details of the service discovery registries to assign to this task set. For more information, see Service Discovery.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setServiceRegistries(java.util.Collection)} or {@link #withServiceRegistries(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param serviceRegistries
* The details of the service discovery registries to assign to this task set. For more information, see Service
* Discovery.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskSet withServiceRegistries(ServiceRegistry... serviceRegistries) {
if (this.serviceRegistries == null) {
setServiceRegistries(new com.amazonaws.internal.SdkInternalList(serviceRegistries.length));
}
for (ServiceRegistry ele : serviceRegistries) {
this.serviceRegistries.add(ele);
}
return this;
}
/**
*
* The details of the service discovery registries to assign to this task set. For more information, see Service Discovery.
*
*
* @param serviceRegistries
* The details of the service discovery registries to assign to this task set. For more information, see Service
* Discovery.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskSet withServiceRegistries(java.util.Collection serviceRegistries) {
setServiceRegistries(serviceRegistries);
return this;
}
/**
*
* A floating-point percentage of the desired number of tasks to place and keep running in the task set.
*
*
* @param scale
* A floating-point percentage of the desired number of tasks to place and keep running in the task set.
*/
public void setScale(Scale scale) {
this.scale = scale;
}
/**
*
* A floating-point percentage of the desired number of tasks to place and keep running in the task set.
*
*
* @return A floating-point percentage of the desired number of tasks to place and keep running in the task set.
*/
public Scale getScale() {
return this.scale;
}
/**
*
* A floating-point percentage of the desired number of tasks to place and keep running in the task set.
*
*
* @param scale
* A floating-point percentage of the desired number of tasks to place and keep running in the task set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskSet withScale(Scale scale) {
setScale(scale);
return this;
}
/**
*
* The stability status, which indicates whether the task set has reached a steady state. If the following
* conditions are met, the task set will be in STEADY_STATE
:
*
*
* -
*
* The task runningCount
is equal to the computedDesiredCount
.
*
*
* -
*
* The pendingCount
is 0
.
*
*
* -
*
* There are no tasks running on container instances in the DRAINING
status.
*
*
* -
*
* All tasks are reporting a healthy status from the load balancers, service discovery, and container health checks.
*
*
*
*
* If any of those conditions are not met, the stability status returns STABILIZING
.
*
*
* @param stabilityStatus
* The stability status, which indicates whether the task set has reached a steady state. If the following
* conditions are met, the task set will be in STEADY_STATE
:
*
* -
*
* The task runningCount
is equal to the computedDesiredCount
.
*
*
* -
*
* The pendingCount
is 0
.
*
*
* -
*
* There are no tasks running on container instances in the DRAINING
status.
*
*
* -
*
* All tasks are reporting a healthy status from the load balancers, service discovery, and container health
* checks.
*
*
*
*
* If any of those conditions are not met, the stability status returns STABILIZING
.
* @see StabilityStatus
*/
public void setStabilityStatus(String stabilityStatus) {
this.stabilityStatus = stabilityStatus;
}
/**
*
* The stability status, which indicates whether the task set has reached a steady state. If the following
* conditions are met, the task set will be in STEADY_STATE
:
*
*
* -
*
* The task runningCount
is equal to the computedDesiredCount
.
*
*
* -
*
* The pendingCount
is 0
.
*
*
* -
*
* There are no tasks running on container instances in the DRAINING
status.
*
*
* -
*
* All tasks are reporting a healthy status from the load balancers, service discovery, and container health checks.
*
*
*
*
* If any of those conditions are not met, the stability status returns STABILIZING
.
*
*
* @return The stability status, which indicates whether the task set has reached a steady state. If the following
* conditions are met, the task set will be in STEADY_STATE
:
*
* -
*
* The task runningCount
is equal to the computedDesiredCount
.
*
*
* -
*
* The pendingCount
is 0
.
*
*
* -
*
* There are no tasks running on container instances in the DRAINING
status.
*
*
* -
*
* All tasks are reporting a healthy status from the load balancers, service discovery, and container health
* checks.
*
*
*
*
* If any of those conditions are not met, the stability status returns STABILIZING
.
* @see StabilityStatus
*/
public String getStabilityStatus() {
return this.stabilityStatus;
}
/**
*
* The stability status, which indicates whether the task set has reached a steady state. If the following
* conditions are met, the task set will be in STEADY_STATE
:
*
*
* -
*
* The task runningCount
is equal to the computedDesiredCount
.
*
*
* -
*
* The pendingCount
is 0
.
*
*
* -
*
* There are no tasks running on container instances in the DRAINING
status.
*
*
* -
*
* All tasks are reporting a healthy status from the load balancers, service discovery, and container health checks.
*
*
*
*
* If any of those conditions are not met, the stability status returns STABILIZING
.
*
*
* @param stabilityStatus
* The stability status, which indicates whether the task set has reached a steady state. If the following
* conditions are met, the task set will be in STEADY_STATE
:
*
* -
*
* The task runningCount
is equal to the computedDesiredCount
.
*
*
* -
*
* The pendingCount
is 0
.
*
*
* -
*
* There are no tasks running on container instances in the DRAINING
status.
*
*
* -
*
* All tasks are reporting a healthy status from the load balancers, service discovery, and container health
* checks.
*
*
*
*
* If any of those conditions are not met, the stability status returns STABILIZING
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see StabilityStatus
*/
public TaskSet withStabilityStatus(String stabilityStatus) {
setStabilityStatus(stabilityStatus);
return this;
}
/**
*
* The stability status, which indicates whether the task set has reached a steady state. If the following
* conditions are met, the task set will be in STEADY_STATE
:
*
*
* -
*
* The task runningCount
is equal to the computedDesiredCount
.
*
*
* -
*
* The pendingCount
is 0
.
*
*
* -
*
* There are no tasks running on container instances in the DRAINING
status.
*
*
* -
*
* All tasks are reporting a healthy status from the load balancers, service discovery, and container health checks.
*
*
*
*
* If any of those conditions are not met, the stability status returns STABILIZING
.
*
*
* @param stabilityStatus
* The stability status, which indicates whether the task set has reached a steady state. If the following
* conditions are met, the task set will be in STEADY_STATE
:
*
* -
*
* The task runningCount
is equal to the computedDesiredCount
.
*
*
* -
*
* The pendingCount
is 0
.
*
*
* -
*
* There are no tasks running on container instances in the DRAINING
status.
*
*
* -
*
* All tasks are reporting a healthy status from the load balancers, service discovery, and container health
* checks.
*
*
*
*
* If any of those conditions are not met, the stability status returns STABILIZING
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see StabilityStatus
*/
public TaskSet withStabilityStatus(StabilityStatus stabilityStatus) {
this.stabilityStatus = stabilityStatus.toString();
return this;
}
/**
*
* The Unix timestamp for when the task set stability status was retrieved.
*
*
* @param stabilityStatusAt
* The Unix timestamp for when the task set stability status was retrieved.
*/
public void setStabilityStatusAt(java.util.Date stabilityStatusAt) {
this.stabilityStatusAt = stabilityStatusAt;
}
/**
*
* The Unix timestamp for when the task set stability status was retrieved.
*
*
* @return The Unix timestamp for when the task set stability status was retrieved.
*/
public java.util.Date getStabilityStatusAt() {
return this.stabilityStatusAt;
}
/**
*
* The Unix timestamp for when the task set stability status was retrieved.
*
*
* @param stabilityStatusAt
* The Unix timestamp for when the task set stability status was retrieved.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskSet withStabilityStatusAt(java.util.Date stabilityStatusAt) {
setStabilityStatusAt(stabilityStatusAt);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getId() != null)
sb.append("Id: ").append(getId()).append(",");
if (getTaskSetArn() != null)
sb.append("TaskSetArn: ").append(getTaskSetArn()).append(",");
if (getServiceArn() != null)
sb.append("ServiceArn: ").append(getServiceArn()).append(",");
if (getClusterArn() != null)
sb.append("ClusterArn: ").append(getClusterArn()).append(",");
if (getStartedBy() != null)
sb.append("StartedBy: ").append(getStartedBy()).append(",");
if (getExternalId() != null)
sb.append("ExternalId: ").append(getExternalId()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getTaskDefinition() != null)
sb.append("TaskDefinition: ").append(getTaskDefinition()).append(",");
if (getComputedDesiredCount() != null)
sb.append("ComputedDesiredCount: ").append(getComputedDesiredCount()).append(",");
if (getPendingCount() != null)
sb.append("PendingCount: ").append(getPendingCount()).append(",");
if (getRunningCount() != null)
sb.append("RunningCount: ").append(getRunningCount()).append(",");
if (getCreatedAt() != null)
sb.append("CreatedAt: ").append(getCreatedAt()).append(",");
if (getUpdatedAt() != null)
sb.append("UpdatedAt: ").append(getUpdatedAt()).append(",");
if (getLaunchType() != null)
sb.append("LaunchType: ").append(getLaunchType()).append(",");
if (getCapacityProviderStrategy() != null)
sb.append("CapacityProviderStrategy: ").append(getCapacityProviderStrategy()).append(",");
if (getPlatformVersion() != null)
sb.append("PlatformVersion: ").append(getPlatformVersion()).append(",");
if (getNetworkConfiguration() != null)
sb.append("NetworkConfiguration: ").append(getNetworkConfiguration()).append(",");
if (getLoadBalancers() != null)
sb.append("LoadBalancers: ").append(getLoadBalancers()).append(",");
if (getServiceRegistries() != null)
sb.append("ServiceRegistries: ").append(getServiceRegistries()).append(",");
if (getScale() != null)
sb.append("Scale: ").append(getScale()).append(",");
if (getStabilityStatus() != null)
sb.append("StabilityStatus: ").append(getStabilityStatus()).append(",");
if (getStabilityStatusAt() != null)
sb.append("StabilityStatusAt: ").append(getStabilityStatusAt());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof TaskSet == false)
return false;
TaskSet other = (TaskSet) obj;
if (other.getId() == null ^ this.getId() == null)
return false;
if (other.getId() != null && other.getId().equals(this.getId()) == false)
return false;
if (other.getTaskSetArn() == null ^ this.getTaskSetArn() == null)
return false;
if (other.getTaskSetArn() != null && other.getTaskSetArn().equals(this.getTaskSetArn()) == false)
return false;
if (other.getServiceArn() == null ^ this.getServiceArn() == null)
return false;
if (other.getServiceArn() != null && other.getServiceArn().equals(this.getServiceArn()) == false)
return false;
if (other.getClusterArn() == null ^ this.getClusterArn() == null)
return false;
if (other.getClusterArn() != null && other.getClusterArn().equals(this.getClusterArn()) == false)
return false;
if (other.getStartedBy() == null ^ this.getStartedBy() == null)
return false;
if (other.getStartedBy() != null && other.getStartedBy().equals(this.getStartedBy()) == false)
return false;
if (other.getExternalId() == null ^ this.getExternalId() == null)
return false;
if (other.getExternalId() != null && other.getExternalId().equals(this.getExternalId()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getTaskDefinition() == null ^ this.getTaskDefinition() == null)
return false;
if (other.getTaskDefinition() != null && other.getTaskDefinition().equals(this.getTaskDefinition()) == false)
return false;
if (other.getComputedDesiredCount() == null ^ this.getComputedDesiredCount() == null)
return false;
if (other.getComputedDesiredCount() != null && other.getComputedDesiredCount().equals(this.getComputedDesiredCount()) == false)
return false;
if (other.getPendingCount() == null ^ this.getPendingCount() == null)
return false;
if (other.getPendingCount() != null && other.getPendingCount().equals(this.getPendingCount()) == false)
return false;
if (other.getRunningCount() == null ^ this.getRunningCount() == null)
return false;
if (other.getRunningCount() != null && other.getRunningCount().equals(this.getRunningCount()) == false)
return false;
if (other.getCreatedAt() == null ^ this.getCreatedAt() == null)
return false;
if (other.getCreatedAt() != null && other.getCreatedAt().equals(this.getCreatedAt()) == false)
return false;
if (other.getUpdatedAt() == null ^ this.getUpdatedAt() == null)
return false;
if (other.getUpdatedAt() != null && other.getUpdatedAt().equals(this.getUpdatedAt()) == false)
return false;
if (other.getLaunchType() == null ^ this.getLaunchType() == null)
return false;
if (other.getLaunchType() != null && other.getLaunchType().equals(this.getLaunchType()) == false)
return false;
if (other.getCapacityProviderStrategy() == null ^ this.getCapacityProviderStrategy() == null)
return false;
if (other.getCapacityProviderStrategy() != null && other.getCapacityProviderStrategy().equals(this.getCapacityProviderStrategy()) == false)
return false;
if (other.getPlatformVersion() == null ^ this.getPlatformVersion() == null)
return false;
if (other.getPlatformVersion() != null && other.getPlatformVersion().equals(this.getPlatformVersion()) == false)
return false;
if (other.getNetworkConfiguration() == null ^ this.getNetworkConfiguration() == null)
return false;
if (other.getNetworkConfiguration() != null && other.getNetworkConfiguration().equals(this.getNetworkConfiguration()) == false)
return false;
if (other.getLoadBalancers() == null ^ this.getLoadBalancers() == null)
return false;
if (other.getLoadBalancers() != null && other.getLoadBalancers().equals(this.getLoadBalancers()) == false)
return false;
if (other.getServiceRegistries() == null ^ this.getServiceRegistries() == null)
return false;
if (other.getServiceRegistries() != null && other.getServiceRegistries().equals(this.getServiceRegistries()) == false)
return false;
if (other.getScale() == null ^ this.getScale() == null)
return false;
if (other.getScale() != null && other.getScale().equals(this.getScale()) == false)
return false;
if (other.getStabilityStatus() == null ^ this.getStabilityStatus() == null)
return false;
if (other.getStabilityStatus() != null && other.getStabilityStatus().equals(this.getStabilityStatus()) == false)
return false;
if (other.getStabilityStatusAt() == null ^ this.getStabilityStatusAt() == null)
return false;
if (other.getStabilityStatusAt() != null && other.getStabilityStatusAt().equals(this.getStabilityStatusAt()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getId() == null) ? 0 : getId().hashCode());
hashCode = prime * hashCode + ((getTaskSetArn() == null) ? 0 : getTaskSetArn().hashCode());
hashCode = prime * hashCode + ((getServiceArn() == null) ? 0 : getServiceArn().hashCode());
hashCode = prime * hashCode + ((getClusterArn() == null) ? 0 : getClusterArn().hashCode());
hashCode = prime * hashCode + ((getStartedBy() == null) ? 0 : getStartedBy().hashCode());
hashCode = prime * hashCode + ((getExternalId() == null) ? 0 : getExternalId().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getTaskDefinition() == null) ? 0 : getTaskDefinition().hashCode());
hashCode = prime * hashCode + ((getComputedDesiredCount() == null) ? 0 : getComputedDesiredCount().hashCode());
hashCode = prime * hashCode + ((getPendingCount() == null) ? 0 : getPendingCount().hashCode());
hashCode = prime * hashCode + ((getRunningCount() == null) ? 0 : getRunningCount().hashCode());
hashCode = prime * hashCode + ((getCreatedAt() == null) ? 0 : getCreatedAt().hashCode());
hashCode = prime * hashCode + ((getUpdatedAt() == null) ? 0 : getUpdatedAt().hashCode());
hashCode = prime * hashCode + ((getLaunchType() == null) ? 0 : getLaunchType().hashCode());
hashCode = prime * hashCode + ((getCapacityProviderStrategy() == null) ? 0 : getCapacityProviderStrategy().hashCode());
hashCode = prime * hashCode + ((getPlatformVersion() == null) ? 0 : getPlatformVersion().hashCode());
hashCode = prime * hashCode + ((getNetworkConfiguration() == null) ? 0 : getNetworkConfiguration().hashCode());
hashCode = prime * hashCode + ((getLoadBalancers() == null) ? 0 : getLoadBalancers().hashCode());
hashCode = prime * hashCode + ((getServiceRegistries() == null) ? 0 : getServiceRegistries().hashCode());
hashCode = prime * hashCode + ((getScale() == null) ? 0 : getScale().hashCode());
hashCode = prime * hashCode + ((getStabilityStatus() == null) ? 0 : getStabilityStatus().hashCode());
hashCode = prime * hashCode + ((getStabilityStatusAt() == null) ? 0 : getStabilityStatusAt().hashCode());
return hashCode;
}
@Override
public TaskSet clone() {
try {
return (TaskSet) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.ecs.model.transform.TaskSetMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}