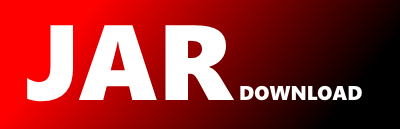
com.amazonaws.services.ecs.model.RunTaskRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ecs Show documentation
/*
* Copyright 2016-2021 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ecs.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class RunTaskRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The capacity provider strategy to use for the task.
*
*
* If a capacityProviderStrategy
is specified, the launchType
parameter must be omitted.
* If no capacityProviderStrategy
or launchType
is specified, the
* defaultCapacityProviderStrategy
for the cluster is used.
*
*/
private com.amazonaws.internal.SdkInternalList capacityProviderStrategy;
/**
*
* The short name or full Amazon Resource Name (ARN) of the cluster on which to run your task. If you do not specify
* a cluster, the default cluster is assumed.
*
*/
private String cluster;
/**
*
* The number of instantiations of the specified task to place on your cluster. You can specify up to 10 tasks per
* call.
*
*/
private Integer count;
/**
*
* Specifies whether to enable Amazon ECS managed tags for the task. For more information, see Tagging Your Amazon ECS
* Resources in the Amazon Elastic Container Service Developer Guide.
*
*/
private Boolean enableECSManagedTags;
/**
*
* Whether or not to enable the execute command functionality for the containers in this task. If true
,
* this enables execute command functionality on all containers in the task.
*
*/
private Boolean enableExecuteCommand;
/**
*
* The name of the task group to associate with the task. The default value is the family name of the task
* definition (for example, family:my-family-name).
*
*/
private String group;
/**
*
* The launch type on which to run your task. The accepted values are FARGATE
and EC2
. For
* more information, see Amazon ECS Launch Types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* When a value of FARGATE
is specified, your tasks are launched on AWS Fargate On-Demand
* infrastructure. To use Fargate Spot, you must use a capacity provider strategy with the FARGATE_SPOT
* capacity provider.
*
*
* When a value of EC2
is specified, your tasks are launched on Amazon EC2 instances registered to your
* cluster.
*
*
* If a launchType
is specified, the capacityProviderStrategy
parameter must be omitted.
*
*/
private String launchType;
/**
*
* The network configuration for the task. This parameter is required for task definitions that use the
* awsvpc
network mode to receive their own elastic network interface, and it is not supported for
* other network modes. For more information, see Task Networking in
* the Amazon Elastic Container Service Developer Guide.
*
*/
private NetworkConfiguration networkConfiguration;
/**
*
* A list of container overrides in JSON format that specify the name of a container in the specified task
* definition and the overrides it should receive. You can override the default command for a container (that is
* specified in the task definition or Docker image) with a command
override. You can also override
* existing environment variables (that are specified in the task definition or Docker image) on a container or add
* new environment variables to it with an environment
override.
*
*
*
* A total of 8192 characters are allowed for overrides. This limit includes the JSON formatting characters of the
* override structure.
*
*
*/
private TaskOverride overrides;
/**
*
* An array of placement constraint objects to use for the task. You can specify up to 10 constraints per task
* (including constraints in the task definition and those specified at runtime).
*
*/
private com.amazonaws.internal.SdkInternalList placementConstraints;
/**
*
* The placement strategy objects to use for the task. You can specify a maximum of five strategy rules per task.
*
*/
private com.amazonaws.internal.SdkInternalList placementStrategy;
/**
*
* The platform version the task should run. A platform version is only specified for tasks using the Fargate launch
* type. If one is not specified, the LATEST
platform version is used by default. For more information,
* see AWS Fargate
* Platform Versions in the Amazon Elastic Container Service Developer Guide.
*
*/
private String platformVersion;
/**
*
* Specifies whether to propagate the tags from the task definition to the task. If no value is specified, the tags
* are not propagated. Tags can only be propagated to the task during task creation. To add tags to a task after
* task creation, use the TagResource API action.
*
*
*
* An error will be received if you specify the SERVICE
option when running a task.
*
*
*/
private String propagateTags;
/**
*
* The reference ID to use for the task.
*
*/
private String referenceId;
/**
*
* An optional tag specified when a task is started. For example, if you automatically trigger a task to run a batch
* process job, you could apply a unique identifier for that job to your task with the startedBy
* parameter. You can then identify which tasks belong to that job by filtering the results of a ListTasks
* call with the startedBy
value. Up to 36 letters (uppercase and lowercase), numbers, hyphens, and
* underscores are allowed.
*
*
* If a task is started by an Amazon ECS service, then the startedBy
parameter contains the deployment
* ID of the service that starts it.
*
*/
private String startedBy;
/**
*
* The metadata that you apply to the task to help you categorize and organize them. Each tag consists of a key and
* an optional value, both of which you define.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* either keys or values as it is reserved for AWS use. You cannot edit or delete tag keys or values with this
* prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*
*/
private com.amazonaws.internal.SdkInternalList tags;
/**
*
* The family
and revision
(family:revision
) or full ARN of the task
* definition to run. If a revision
is not specified, the latest ACTIVE
revision is used.
*
*/
private String taskDefinition;
/**
*
* The capacity provider strategy to use for the task.
*
*
* If a capacityProviderStrategy
is specified, the launchType
parameter must be omitted.
* If no capacityProviderStrategy
or launchType
is specified, the
* defaultCapacityProviderStrategy
for the cluster is used.
*
*
* @return The capacity provider strategy to use for the task.
*
* If a capacityProviderStrategy
is specified, the launchType
parameter must be
* omitted. If no capacityProviderStrategy
or launchType
is specified, the
* defaultCapacityProviderStrategy
for the cluster is used.
*/
public java.util.List getCapacityProviderStrategy() {
if (capacityProviderStrategy == null) {
capacityProviderStrategy = new com.amazonaws.internal.SdkInternalList();
}
return capacityProviderStrategy;
}
/**
*
* The capacity provider strategy to use for the task.
*
*
* If a capacityProviderStrategy
is specified, the launchType
parameter must be omitted.
* If no capacityProviderStrategy
or launchType
is specified, the
* defaultCapacityProviderStrategy
for the cluster is used.
*
*
* @param capacityProviderStrategy
* The capacity provider strategy to use for the task.
*
* If a capacityProviderStrategy
is specified, the launchType
parameter must be
* omitted. If no capacityProviderStrategy
or launchType
is specified, the
* defaultCapacityProviderStrategy
for the cluster is used.
*/
public void setCapacityProviderStrategy(java.util.Collection capacityProviderStrategy) {
if (capacityProviderStrategy == null) {
this.capacityProviderStrategy = null;
return;
}
this.capacityProviderStrategy = new com.amazonaws.internal.SdkInternalList(capacityProviderStrategy);
}
/**
*
* The capacity provider strategy to use for the task.
*
*
* If a capacityProviderStrategy
is specified, the launchType
parameter must be omitted.
* If no capacityProviderStrategy
or launchType
is specified, the
* defaultCapacityProviderStrategy
for the cluster is used.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCapacityProviderStrategy(java.util.Collection)} or
* {@link #withCapacityProviderStrategy(java.util.Collection)} if you want to override the existing values.
*
*
* @param capacityProviderStrategy
* The capacity provider strategy to use for the task.
*
* If a capacityProviderStrategy
is specified, the launchType
parameter must be
* omitted. If no capacityProviderStrategy
or launchType
is specified, the
* defaultCapacityProviderStrategy
for the cluster is used.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunTaskRequest withCapacityProviderStrategy(CapacityProviderStrategyItem... capacityProviderStrategy) {
if (this.capacityProviderStrategy == null) {
setCapacityProviderStrategy(new com.amazonaws.internal.SdkInternalList(capacityProviderStrategy.length));
}
for (CapacityProviderStrategyItem ele : capacityProviderStrategy) {
this.capacityProviderStrategy.add(ele);
}
return this;
}
/**
*
* The capacity provider strategy to use for the task.
*
*
* If a capacityProviderStrategy
is specified, the launchType
parameter must be omitted.
* If no capacityProviderStrategy
or launchType
is specified, the
* defaultCapacityProviderStrategy
for the cluster is used.
*
*
* @param capacityProviderStrategy
* The capacity provider strategy to use for the task.
*
* If a capacityProviderStrategy
is specified, the launchType
parameter must be
* omitted. If no capacityProviderStrategy
or launchType
is specified, the
* defaultCapacityProviderStrategy
for the cluster is used.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunTaskRequest withCapacityProviderStrategy(java.util.Collection capacityProviderStrategy) {
setCapacityProviderStrategy(capacityProviderStrategy);
return this;
}
/**
*
* The short name or full Amazon Resource Name (ARN) of the cluster on which to run your task. If you do not specify
* a cluster, the default cluster is assumed.
*
*
* @param cluster
* The short name or full Amazon Resource Name (ARN) of the cluster on which to run your task. If you do not
* specify a cluster, the default cluster is assumed.
*/
public void setCluster(String cluster) {
this.cluster = cluster;
}
/**
*
* The short name or full Amazon Resource Name (ARN) of the cluster on which to run your task. If you do not specify
* a cluster, the default cluster is assumed.
*
*
* @return The short name or full Amazon Resource Name (ARN) of the cluster on which to run your task. If you do not
* specify a cluster, the default cluster is assumed.
*/
public String getCluster() {
return this.cluster;
}
/**
*
* The short name or full Amazon Resource Name (ARN) of the cluster on which to run your task. If you do not specify
* a cluster, the default cluster is assumed.
*
*
* @param cluster
* The short name or full Amazon Resource Name (ARN) of the cluster on which to run your task. If you do not
* specify a cluster, the default cluster is assumed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunTaskRequest withCluster(String cluster) {
setCluster(cluster);
return this;
}
/**
*
* The number of instantiations of the specified task to place on your cluster. You can specify up to 10 tasks per
* call.
*
*
* @param count
* The number of instantiations of the specified task to place on your cluster. You can specify up to 10
* tasks per call.
*/
public void setCount(Integer count) {
this.count = count;
}
/**
*
* The number of instantiations of the specified task to place on your cluster. You can specify up to 10 tasks per
* call.
*
*
* @return The number of instantiations of the specified task to place on your cluster. You can specify up to 10
* tasks per call.
*/
public Integer getCount() {
return this.count;
}
/**
*
* The number of instantiations of the specified task to place on your cluster. You can specify up to 10 tasks per
* call.
*
*
* @param count
* The number of instantiations of the specified task to place on your cluster. You can specify up to 10
* tasks per call.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunTaskRequest withCount(Integer count) {
setCount(count);
return this;
}
/**
*
* Specifies whether to enable Amazon ECS managed tags for the task. For more information, see Tagging Your Amazon ECS
* Resources in the Amazon Elastic Container Service Developer Guide.
*
*
* @param enableECSManagedTags
* Specifies whether to enable Amazon ECS managed tags for the task. For more information, see Tagging Your Amazon
* ECS Resources in the Amazon Elastic Container Service Developer Guide.
*/
public void setEnableECSManagedTags(Boolean enableECSManagedTags) {
this.enableECSManagedTags = enableECSManagedTags;
}
/**
*
* Specifies whether to enable Amazon ECS managed tags for the task. For more information, see Tagging Your Amazon ECS
* Resources in the Amazon Elastic Container Service Developer Guide.
*
*
* @return Specifies whether to enable Amazon ECS managed tags for the task. For more information, see Tagging Your
* Amazon ECS Resources in the Amazon Elastic Container Service Developer Guide.
*/
public Boolean getEnableECSManagedTags() {
return this.enableECSManagedTags;
}
/**
*
* Specifies whether to enable Amazon ECS managed tags for the task. For more information, see Tagging Your Amazon ECS
* Resources in the Amazon Elastic Container Service Developer Guide.
*
*
* @param enableECSManagedTags
* Specifies whether to enable Amazon ECS managed tags for the task. For more information, see Tagging Your Amazon
* ECS Resources in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunTaskRequest withEnableECSManagedTags(Boolean enableECSManagedTags) {
setEnableECSManagedTags(enableECSManagedTags);
return this;
}
/**
*
* Specifies whether to enable Amazon ECS managed tags for the task. For more information, see Tagging Your Amazon ECS
* Resources in the Amazon Elastic Container Service Developer Guide.
*
*
* @return Specifies whether to enable Amazon ECS managed tags for the task. For more information, see Tagging Your
* Amazon ECS Resources in the Amazon Elastic Container Service Developer Guide.
*/
public Boolean isEnableECSManagedTags() {
return this.enableECSManagedTags;
}
/**
*
* Whether or not to enable the execute command functionality for the containers in this task. If true
,
* this enables execute command functionality on all containers in the task.
*
*
* @param enableExecuteCommand
* Whether or not to enable the execute command functionality for the containers in this task. If
* true
, this enables execute command functionality on all containers in the task.
*/
public void setEnableExecuteCommand(Boolean enableExecuteCommand) {
this.enableExecuteCommand = enableExecuteCommand;
}
/**
*
* Whether or not to enable the execute command functionality for the containers in this task. If true
,
* this enables execute command functionality on all containers in the task.
*
*
* @return Whether or not to enable the execute command functionality for the containers in this task. If
* true
, this enables execute command functionality on all containers in the task.
*/
public Boolean getEnableExecuteCommand() {
return this.enableExecuteCommand;
}
/**
*
* Whether or not to enable the execute command functionality for the containers in this task. If true
,
* this enables execute command functionality on all containers in the task.
*
*
* @param enableExecuteCommand
* Whether or not to enable the execute command functionality for the containers in this task. If
* true
, this enables execute command functionality on all containers in the task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunTaskRequest withEnableExecuteCommand(Boolean enableExecuteCommand) {
setEnableExecuteCommand(enableExecuteCommand);
return this;
}
/**
*
* Whether or not to enable the execute command functionality for the containers in this task. If true
,
* this enables execute command functionality on all containers in the task.
*
*
* @return Whether or not to enable the execute command functionality for the containers in this task. If
* true
, this enables execute command functionality on all containers in the task.
*/
public Boolean isEnableExecuteCommand() {
return this.enableExecuteCommand;
}
/**
*
* The name of the task group to associate with the task. The default value is the family name of the task
* definition (for example, family:my-family-name).
*
*
* @param group
* The name of the task group to associate with the task. The default value is the family name of the task
* definition (for example, family:my-family-name).
*/
public void setGroup(String group) {
this.group = group;
}
/**
*
* The name of the task group to associate with the task. The default value is the family name of the task
* definition (for example, family:my-family-name).
*
*
* @return The name of the task group to associate with the task. The default value is the family name of the task
* definition (for example, family:my-family-name).
*/
public String getGroup() {
return this.group;
}
/**
*
* The name of the task group to associate with the task. The default value is the family name of the task
* definition (for example, family:my-family-name).
*
*
* @param group
* The name of the task group to associate with the task. The default value is the family name of the task
* definition (for example, family:my-family-name).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunTaskRequest withGroup(String group) {
setGroup(group);
return this;
}
/**
*
* The launch type on which to run your task. The accepted values are FARGATE
and EC2
. For
* more information, see Amazon ECS Launch Types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* When a value of FARGATE
is specified, your tasks are launched on AWS Fargate On-Demand
* infrastructure. To use Fargate Spot, you must use a capacity provider strategy with the FARGATE_SPOT
* capacity provider.
*
*
* When a value of EC2
is specified, your tasks are launched on Amazon EC2 instances registered to your
* cluster.
*
*
* If a launchType
is specified, the capacityProviderStrategy
parameter must be omitted.
*
*
* @param launchType
* The launch type on which to run your task. The accepted values are FARGATE
and
* EC2
. For more information, see Amazon ECS Launch
* Types in the Amazon Elastic Container Service Developer Guide.
*
* When a value of FARGATE
is specified, your tasks are launched on AWS Fargate On-Demand
* infrastructure. To use Fargate Spot, you must use a capacity provider strategy with the
* FARGATE_SPOT
capacity provider.
*
*
* When a value of EC2
is specified, your tasks are launched on Amazon EC2 instances registered
* to your cluster.
*
*
* If a launchType
is specified, the capacityProviderStrategy
parameter must be
* omitted.
* @see LaunchType
*/
public void setLaunchType(String launchType) {
this.launchType = launchType;
}
/**
*
* The launch type on which to run your task. The accepted values are FARGATE
and EC2
. For
* more information, see Amazon ECS Launch Types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* When a value of FARGATE
is specified, your tasks are launched on AWS Fargate On-Demand
* infrastructure. To use Fargate Spot, you must use a capacity provider strategy with the FARGATE_SPOT
* capacity provider.
*
*
* When a value of EC2
is specified, your tasks are launched on Amazon EC2 instances registered to your
* cluster.
*
*
* If a launchType
is specified, the capacityProviderStrategy
parameter must be omitted.
*
*
* @return The launch type on which to run your task. The accepted values are FARGATE
and
* EC2
. For more information, see Amazon ECS Launch
* Types in the Amazon Elastic Container Service Developer Guide.
*
* When a value of FARGATE
is specified, your tasks are launched on AWS Fargate On-Demand
* infrastructure. To use Fargate Spot, you must use a capacity provider strategy with the
* FARGATE_SPOT
capacity provider.
*
*
* When a value of EC2
is specified, your tasks are launched on Amazon EC2 instances registered
* to your cluster.
*
*
* If a launchType
is specified, the capacityProviderStrategy
parameter must be
* omitted.
* @see LaunchType
*/
public String getLaunchType() {
return this.launchType;
}
/**
*
* The launch type on which to run your task. The accepted values are FARGATE
and EC2
. For
* more information, see Amazon ECS Launch Types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* When a value of FARGATE
is specified, your tasks are launched on AWS Fargate On-Demand
* infrastructure. To use Fargate Spot, you must use a capacity provider strategy with the FARGATE_SPOT
* capacity provider.
*
*
* When a value of EC2
is specified, your tasks are launched on Amazon EC2 instances registered to your
* cluster.
*
*
* If a launchType
is specified, the capacityProviderStrategy
parameter must be omitted.
*
*
* @param launchType
* The launch type on which to run your task. The accepted values are FARGATE
and
* EC2
. For more information, see Amazon ECS Launch
* Types in the Amazon Elastic Container Service Developer Guide.
*
* When a value of FARGATE
is specified, your tasks are launched on AWS Fargate On-Demand
* infrastructure. To use Fargate Spot, you must use a capacity provider strategy with the
* FARGATE_SPOT
capacity provider.
*
*
* When a value of EC2
is specified, your tasks are launched on Amazon EC2 instances registered
* to your cluster.
*
*
* If a launchType
is specified, the capacityProviderStrategy
parameter must be
* omitted.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LaunchType
*/
public RunTaskRequest withLaunchType(String launchType) {
setLaunchType(launchType);
return this;
}
/**
*
* The launch type on which to run your task. The accepted values are FARGATE
and EC2
. For
* more information, see Amazon ECS Launch Types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* When a value of FARGATE
is specified, your tasks are launched on AWS Fargate On-Demand
* infrastructure. To use Fargate Spot, you must use a capacity provider strategy with the FARGATE_SPOT
* capacity provider.
*
*
* When a value of EC2
is specified, your tasks are launched on Amazon EC2 instances registered to your
* cluster.
*
*
* If a launchType
is specified, the capacityProviderStrategy
parameter must be omitted.
*
*
* @param launchType
* The launch type on which to run your task. The accepted values are FARGATE
and
* EC2
. For more information, see Amazon ECS Launch
* Types in the Amazon Elastic Container Service Developer Guide.
*
* When a value of FARGATE
is specified, your tasks are launched on AWS Fargate On-Demand
* infrastructure. To use Fargate Spot, you must use a capacity provider strategy with the
* FARGATE_SPOT
capacity provider.
*
*
* When a value of EC2
is specified, your tasks are launched on Amazon EC2 instances registered
* to your cluster.
*
*
* If a launchType
is specified, the capacityProviderStrategy
parameter must be
* omitted.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LaunchType
*/
public RunTaskRequest withLaunchType(LaunchType launchType) {
this.launchType = launchType.toString();
return this;
}
/**
*
* The network configuration for the task. This parameter is required for task definitions that use the
* awsvpc
network mode to receive their own elastic network interface, and it is not supported for
* other network modes. For more information, see Task Networking in
* the Amazon Elastic Container Service Developer Guide.
*
*
* @param networkConfiguration
* The network configuration for the task. This parameter is required for task definitions that use the
* awsvpc
network mode to receive their own elastic network interface, and it is not supported
* for other network modes. For more information, see Task
* Networking in the Amazon Elastic Container Service Developer Guide.
*/
public void setNetworkConfiguration(NetworkConfiguration networkConfiguration) {
this.networkConfiguration = networkConfiguration;
}
/**
*
* The network configuration for the task. This parameter is required for task definitions that use the
* awsvpc
network mode to receive their own elastic network interface, and it is not supported for
* other network modes. For more information, see Task Networking in
* the Amazon Elastic Container Service Developer Guide.
*
*
* @return The network configuration for the task. This parameter is required for task definitions that use the
* awsvpc
network mode to receive their own elastic network interface, and it is not supported
* for other network modes. For more information, see Task
* Networking in the Amazon Elastic Container Service Developer Guide.
*/
public NetworkConfiguration getNetworkConfiguration() {
return this.networkConfiguration;
}
/**
*
* The network configuration for the task. This parameter is required for task definitions that use the
* awsvpc
network mode to receive their own elastic network interface, and it is not supported for
* other network modes. For more information, see Task Networking in
* the Amazon Elastic Container Service Developer Guide.
*
*
* @param networkConfiguration
* The network configuration for the task. This parameter is required for task definitions that use the
* awsvpc
network mode to receive their own elastic network interface, and it is not supported
* for other network modes. For more information, see Task
* Networking in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunTaskRequest withNetworkConfiguration(NetworkConfiguration networkConfiguration) {
setNetworkConfiguration(networkConfiguration);
return this;
}
/**
*
* A list of container overrides in JSON format that specify the name of a container in the specified task
* definition and the overrides it should receive. You can override the default command for a container (that is
* specified in the task definition or Docker image) with a command
override. You can also override
* existing environment variables (that are specified in the task definition or Docker image) on a container or add
* new environment variables to it with an environment
override.
*
*
*
* A total of 8192 characters are allowed for overrides. This limit includes the JSON formatting characters of the
* override structure.
*
*
*
* @param overrides
* A list of container overrides in JSON format that specify the name of a container in the specified task
* definition and the overrides it should receive. You can override the default command for a container (that
* is specified in the task definition or Docker image) with a command
override. You can also
* override existing environment variables (that are specified in the task definition or Docker image) on a
* container or add new environment variables to it with an environment
override.
*
* A total of 8192 characters are allowed for overrides. This limit includes the JSON formatting characters
* of the override structure.
*
*/
public void setOverrides(TaskOverride overrides) {
this.overrides = overrides;
}
/**
*
* A list of container overrides in JSON format that specify the name of a container in the specified task
* definition and the overrides it should receive. You can override the default command for a container (that is
* specified in the task definition or Docker image) with a command
override. You can also override
* existing environment variables (that are specified in the task definition or Docker image) on a container or add
* new environment variables to it with an environment
override.
*
*
*
* A total of 8192 characters are allowed for overrides. This limit includes the JSON formatting characters of the
* override structure.
*
*
*
* @return A list of container overrides in JSON format that specify the name of a container in the specified task
* definition and the overrides it should receive. You can override the default command for a container
* (that is specified in the task definition or Docker image) with a command
override. You can
* also override existing environment variables (that are specified in the task definition or Docker image)
* on a container or add new environment variables to it with an environment
override.
*
*
* A total of 8192 characters are allowed for overrides. This limit includes the JSON formatting characters
* of the override structure.
*
*/
public TaskOverride getOverrides() {
return this.overrides;
}
/**
*
* A list of container overrides in JSON format that specify the name of a container in the specified task
* definition and the overrides it should receive. You can override the default command for a container (that is
* specified in the task definition or Docker image) with a command
override. You can also override
* existing environment variables (that are specified in the task definition or Docker image) on a container or add
* new environment variables to it with an environment
override.
*
*
*
* A total of 8192 characters are allowed for overrides. This limit includes the JSON formatting characters of the
* override structure.
*
*
*
* @param overrides
* A list of container overrides in JSON format that specify the name of a container in the specified task
* definition and the overrides it should receive. You can override the default command for a container (that
* is specified in the task definition or Docker image) with a command
override. You can also
* override existing environment variables (that are specified in the task definition or Docker image) on a
* container or add new environment variables to it with an environment
override.
*
* A total of 8192 characters are allowed for overrides. This limit includes the JSON formatting characters
* of the override structure.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunTaskRequest withOverrides(TaskOverride overrides) {
setOverrides(overrides);
return this;
}
/**
*
* An array of placement constraint objects to use for the task. You can specify up to 10 constraints per task
* (including constraints in the task definition and those specified at runtime).
*
*
* @return An array of placement constraint objects to use for the task. You can specify up to 10 constraints per
* task (including constraints in the task definition and those specified at runtime).
*/
public java.util.List getPlacementConstraints() {
if (placementConstraints == null) {
placementConstraints = new com.amazonaws.internal.SdkInternalList();
}
return placementConstraints;
}
/**
*
* An array of placement constraint objects to use for the task. You can specify up to 10 constraints per task
* (including constraints in the task definition and those specified at runtime).
*
*
* @param placementConstraints
* An array of placement constraint objects to use for the task. You can specify up to 10 constraints per
* task (including constraints in the task definition and those specified at runtime).
*/
public void setPlacementConstraints(java.util.Collection placementConstraints) {
if (placementConstraints == null) {
this.placementConstraints = null;
return;
}
this.placementConstraints = new com.amazonaws.internal.SdkInternalList(placementConstraints);
}
/**
*
* An array of placement constraint objects to use for the task. You can specify up to 10 constraints per task
* (including constraints in the task definition and those specified at runtime).
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPlacementConstraints(java.util.Collection)} or {@link #withPlacementConstraints(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param placementConstraints
* An array of placement constraint objects to use for the task. You can specify up to 10 constraints per
* task (including constraints in the task definition and those specified at runtime).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunTaskRequest withPlacementConstraints(PlacementConstraint... placementConstraints) {
if (this.placementConstraints == null) {
setPlacementConstraints(new com.amazonaws.internal.SdkInternalList(placementConstraints.length));
}
for (PlacementConstraint ele : placementConstraints) {
this.placementConstraints.add(ele);
}
return this;
}
/**
*
* An array of placement constraint objects to use for the task. You can specify up to 10 constraints per task
* (including constraints in the task definition and those specified at runtime).
*
*
* @param placementConstraints
* An array of placement constraint objects to use for the task. You can specify up to 10 constraints per
* task (including constraints in the task definition and those specified at runtime).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunTaskRequest withPlacementConstraints(java.util.Collection placementConstraints) {
setPlacementConstraints(placementConstraints);
return this;
}
/**
*
* The placement strategy objects to use for the task. You can specify a maximum of five strategy rules per task.
*
*
* @return The placement strategy objects to use for the task. You can specify a maximum of five strategy rules per
* task.
*/
public java.util.List getPlacementStrategy() {
if (placementStrategy == null) {
placementStrategy = new com.amazonaws.internal.SdkInternalList();
}
return placementStrategy;
}
/**
*
* The placement strategy objects to use for the task. You can specify a maximum of five strategy rules per task.
*
*
* @param placementStrategy
* The placement strategy objects to use for the task. You can specify a maximum of five strategy rules per
* task.
*/
public void setPlacementStrategy(java.util.Collection placementStrategy) {
if (placementStrategy == null) {
this.placementStrategy = null;
return;
}
this.placementStrategy = new com.amazonaws.internal.SdkInternalList(placementStrategy);
}
/**
*
* The placement strategy objects to use for the task. You can specify a maximum of five strategy rules per task.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPlacementStrategy(java.util.Collection)} or {@link #withPlacementStrategy(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param placementStrategy
* The placement strategy objects to use for the task. You can specify a maximum of five strategy rules per
* task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunTaskRequest withPlacementStrategy(PlacementStrategy... placementStrategy) {
if (this.placementStrategy == null) {
setPlacementStrategy(new com.amazonaws.internal.SdkInternalList(placementStrategy.length));
}
for (PlacementStrategy ele : placementStrategy) {
this.placementStrategy.add(ele);
}
return this;
}
/**
*
* The placement strategy objects to use for the task. You can specify a maximum of five strategy rules per task.
*
*
* @param placementStrategy
* The placement strategy objects to use for the task. You can specify a maximum of five strategy rules per
* task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunTaskRequest withPlacementStrategy(java.util.Collection placementStrategy) {
setPlacementStrategy(placementStrategy);
return this;
}
/**
*
* The platform version the task should run. A platform version is only specified for tasks using the Fargate launch
* type. If one is not specified, the LATEST
platform version is used by default. For more information,
* see AWS Fargate
* Platform Versions in the Amazon Elastic Container Service Developer Guide.
*
*
* @param platformVersion
* The platform version the task should run. A platform version is only specified for tasks using the Fargate
* launch type. If one is not specified, the LATEST
platform version is used by default. For
* more information, see AWS Fargate
* Platform Versions in the Amazon Elastic Container Service Developer Guide.
*/
public void setPlatformVersion(String platformVersion) {
this.platformVersion = platformVersion;
}
/**
*
* The platform version the task should run. A platform version is only specified for tasks using the Fargate launch
* type. If one is not specified, the LATEST
platform version is used by default. For more information,
* see AWS Fargate
* Platform Versions in the Amazon Elastic Container Service Developer Guide.
*
*
* @return The platform version the task should run. A platform version is only specified for tasks using the
* Fargate launch type. If one is not specified, the LATEST
platform version is used by
* default. For more information, see AWS Fargate
* Platform Versions in the Amazon Elastic Container Service Developer Guide.
*/
public String getPlatformVersion() {
return this.platformVersion;
}
/**
*
* The platform version the task should run. A platform version is only specified for tasks using the Fargate launch
* type. If one is not specified, the LATEST
platform version is used by default. For more information,
* see AWS Fargate
* Platform Versions in the Amazon Elastic Container Service Developer Guide.
*
*
* @param platformVersion
* The platform version the task should run. A platform version is only specified for tasks using the Fargate
* launch type. If one is not specified, the LATEST
platform version is used by default. For
* more information, see AWS Fargate
* Platform Versions in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunTaskRequest withPlatformVersion(String platformVersion) {
setPlatformVersion(platformVersion);
return this;
}
/**
*
* Specifies whether to propagate the tags from the task definition to the task. If no value is specified, the tags
* are not propagated. Tags can only be propagated to the task during task creation. To add tags to a task after
* task creation, use the TagResource API action.
*
*
*
* An error will be received if you specify the SERVICE
option when running a task.
*
*
*
* @param propagateTags
* Specifies whether to propagate the tags from the task definition to the task. If no value is specified,
* the tags are not propagated. Tags can only be propagated to the task during task creation. To add tags to
* a task after task creation, use the TagResource API action.
*
* An error will be received if you specify the SERVICE
option when running a task.
*
* @see PropagateTags
*/
public void setPropagateTags(String propagateTags) {
this.propagateTags = propagateTags;
}
/**
*
* Specifies whether to propagate the tags from the task definition to the task. If no value is specified, the tags
* are not propagated. Tags can only be propagated to the task during task creation. To add tags to a task after
* task creation, use the TagResource API action.
*
*
*
* An error will be received if you specify the SERVICE
option when running a task.
*
*
*
* @return Specifies whether to propagate the tags from the task definition to the task. If no value is specified,
* the tags are not propagated. Tags can only be propagated to the task during task creation. To add tags to
* a task after task creation, use the TagResource API action.
*
* An error will be received if you specify the SERVICE
option when running a task.
*
* @see PropagateTags
*/
public String getPropagateTags() {
return this.propagateTags;
}
/**
*
* Specifies whether to propagate the tags from the task definition to the task. If no value is specified, the tags
* are not propagated. Tags can only be propagated to the task during task creation. To add tags to a task after
* task creation, use the TagResource API action.
*
*
*
* An error will be received if you specify the SERVICE
option when running a task.
*
*
*
* @param propagateTags
* Specifies whether to propagate the tags from the task definition to the task. If no value is specified,
* the tags are not propagated. Tags can only be propagated to the task during task creation. To add tags to
* a task after task creation, use the TagResource API action.
*
* An error will be received if you specify the SERVICE
option when running a task.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see PropagateTags
*/
public RunTaskRequest withPropagateTags(String propagateTags) {
setPropagateTags(propagateTags);
return this;
}
/**
*
* Specifies whether to propagate the tags from the task definition to the task. If no value is specified, the tags
* are not propagated. Tags can only be propagated to the task during task creation. To add tags to a task after
* task creation, use the TagResource API action.
*
*
*
* An error will be received if you specify the SERVICE
option when running a task.
*
*
*
* @param propagateTags
* Specifies whether to propagate the tags from the task definition to the task. If no value is specified,
* the tags are not propagated. Tags can only be propagated to the task during task creation. To add tags to
* a task after task creation, use the TagResource API action.
*
* An error will be received if you specify the SERVICE
option when running a task.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see PropagateTags
*/
public RunTaskRequest withPropagateTags(PropagateTags propagateTags) {
this.propagateTags = propagateTags.toString();
return this;
}
/**
*
* The reference ID to use for the task.
*
*
* @param referenceId
* The reference ID to use for the task.
*/
public void setReferenceId(String referenceId) {
this.referenceId = referenceId;
}
/**
*
* The reference ID to use for the task.
*
*
* @return The reference ID to use for the task.
*/
public String getReferenceId() {
return this.referenceId;
}
/**
*
* The reference ID to use for the task.
*
*
* @param referenceId
* The reference ID to use for the task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunTaskRequest withReferenceId(String referenceId) {
setReferenceId(referenceId);
return this;
}
/**
*
* An optional tag specified when a task is started. For example, if you automatically trigger a task to run a batch
* process job, you could apply a unique identifier for that job to your task with the startedBy
* parameter. You can then identify which tasks belong to that job by filtering the results of a ListTasks
* call with the startedBy
value. Up to 36 letters (uppercase and lowercase), numbers, hyphens, and
* underscores are allowed.
*
*
* If a task is started by an Amazon ECS service, then the startedBy
parameter contains the deployment
* ID of the service that starts it.
*
*
* @param startedBy
* An optional tag specified when a task is started. For example, if you automatically trigger a task to run
* a batch process job, you could apply a unique identifier for that job to your task with the
* startedBy
parameter. You can then identify which tasks belong to that job by filtering the
* results of a ListTasks call with the startedBy
value. Up to 36 letters (uppercase and
* lowercase), numbers, hyphens, and underscores are allowed.
*
* If a task is started by an Amazon ECS service, then the startedBy
parameter contains the
* deployment ID of the service that starts it.
*/
public void setStartedBy(String startedBy) {
this.startedBy = startedBy;
}
/**
*
* An optional tag specified when a task is started. For example, if you automatically trigger a task to run a batch
* process job, you could apply a unique identifier for that job to your task with the startedBy
* parameter. You can then identify which tasks belong to that job by filtering the results of a ListTasks
* call with the startedBy
value. Up to 36 letters (uppercase and lowercase), numbers, hyphens, and
* underscores are allowed.
*
*
* If a task is started by an Amazon ECS service, then the startedBy
parameter contains the deployment
* ID of the service that starts it.
*
*
* @return An optional tag specified when a task is started. For example, if you automatically trigger a task to run
* a batch process job, you could apply a unique identifier for that job to your task with the
* startedBy
parameter. You can then identify which tasks belong to that job by filtering the
* results of a ListTasks call with the startedBy
value. Up to 36 letters (uppercase and
* lowercase), numbers, hyphens, and underscores are allowed.
*
* If a task is started by an Amazon ECS service, then the startedBy
parameter contains the
* deployment ID of the service that starts it.
*/
public String getStartedBy() {
return this.startedBy;
}
/**
*
* An optional tag specified when a task is started. For example, if you automatically trigger a task to run a batch
* process job, you could apply a unique identifier for that job to your task with the startedBy
* parameter. You can then identify which tasks belong to that job by filtering the results of a ListTasks
* call with the startedBy
value. Up to 36 letters (uppercase and lowercase), numbers, hyphens, and
* underscores are allowed.
*
*
* If a task is started by an Amazon ECS service, then the startedBy
parameter contains the deployment
* ID of the service that starts it.
*
*
* @param startedBy
* An optional tag specified when a task is started. For example, if you automatically trigger a task to run
* a batch process job, you could apply a unique identifier for that job to your task with the
* startedBy
parameter. You can then identify which tasks belong to that job by filtering the
* results of a ListTasks call with the startedBy
value. Up to 36 letters (uppercase and
* lowercase), numbers, hyphens, and underscores are allowed.
*
* If a task is started by an Amazon ECS service, then the startedBy
parameter contains the
* deployment ID of the service that starts it.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunTaskRequest withStartedBy(String startedBy) {
setStartedBy(startedBy);
return this;
}
/**
*
* The metadata that you apply to the task to help you categorize and organize them. Each tag consists of a key and
* an optional value, both of which you define.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* either keys or values as it is reserved for AWS use. You cannot edit or delete tag keys or values with this
* prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*
*
* @return The metadata that you apply to the task to help you categorize and organize them. Each tag consists of a
* key and an optional value, both of which you define.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may
* have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a
* prefix for either keys or values as it is reserved for AWS use. You cannot edit or delete tag keys or
* values with this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*/
public java.util.List getTags() {
if (tags == null) {
tags = new com.amazonaws.internal.SdkInternalList();
}
return tags;
}
/**
*
* The metadata that you apply to the task to help you categorize and organize them. Each tag consists of a key and
* an optional value, both of which you define.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* either keys or values as it is reserved for AWS use. You cannot edit or delete tag keys or values with this
* prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*
*
* @param tags
* The metadata that you apply to the task to help you categorize and organize them. Each tag consists of a
* key and an optional value, both of which you define.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may
* have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix
* for either keys or values as it is reserved for AWS use. You cannot edit or delete tag keys or values with
* this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new com.amazonaws.internal.SdkInternalList(tags);
}
/**
*
* The metadata that you apply to the task to help you categorize and organize them. Each tag consists of a key and
* an optional value, both of which you define.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* either keys or values as it is reserved for AWS use. You cannot edit or delete tag keys or values with this
* prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* The metadata that you apply to the task to help you categorize and organize them. Each tag consists of a
* key and an optional value, both of which you define.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may
* have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix
* for either keys or values as it is reserved for AWS use. You cannot edit or delete tag keys or values with
* this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunTaskRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new com.amazonaws.internal.SdkInternalList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* The metadata that you apply to the task to help you categorize and organize them. Each tag consists of a key and
* an optional value, both of which you define.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* either keys or values as it is reserved for AWS use. You cannot edit or delete tag keys or values with this
* prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*
*
* @param tags
* The metadata that you apply to the task to help you categorize and organize them. Each tag consists of a
* key and an optional value, both of which you define.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may
* have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix
* for either keys or values as it is reserved for AWS use. You cannot edit or delete tag keys or values with
* this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunTaskRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* The family
and revision
(family:revision
) or full ARN of the task
* definition to run. If a revision
is not specified, the latest ACTIVE
revision is used.
*
*
* @param taskDefinition
* The family
and revision
(family:revision
) or full ARN of the task
* definition to run. If a revision
is not specified, the latest ACTIVE
revision is
* used.
*/
public void setTaskDefinition(String taskDefinition) {
this.taskDefinition = taskDefinition;
}
/**
*
* The family
and revision
(family:revision
) or full ARN of the task
* definition to run. If a revision
is not specified, the latest ACTIVE
revision is used.
*
*
* @return The family
and revision
(family:revision
) or full ARN of the task
* definition to run. If a revision
is not specified, the latest ACTIVE
revision
* is used.
*/
public String getTaskDefinition() {
return this.taskDefinition;
}
/**
*
* The family
and revision
(family:revision
) or full ARN of the task
* definition to run. If a revision
is not specified, the latest ACTIVE
revision is used.
*
*
* @param taskDefinition
* The family
and revision
(family:revision
) or full ARN of the task
* definition to run. If a revision
is not specified, the latest ACTIVE
revision is
* used.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public RunTaskRequest withTaskDefinition(String taskDefinition) {
setTaskDefinition(taskDefinition);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCapacityProviderStrategy() != null)
sb.append("CapacityProviderStrategy: ").append(getCapacityProviderStrategy()).append(",");
if (getCluster() != null)
sb.append("Cluster: ").append(getCluster()).append(",");
if (getCount() != null)
sb.append("Count: ").append(getCount()).append(",");
if (getEnableECSManagedTags() != null)
sb.append("EnableECSManagedTags: ").append(getEnableECSManagedTags()).append(",");
if (getEnableExecuteCommand() != null)
sb.append("EnableExecuteCommand: ").append(getEnableExecuteCommand()).append(",");
if (getGroup() != null)
sb.append("Group: ").append(getGroup()).append(",");
if (getLaunchType() != null)
sb.append("LaunchType: ").append(getLaunchType()).append(",");
if (getNetworkConfiguration() != null)
sb.append("NetworkConfiguration: ").append(getNetworkConfiguration()).append(",");
if (getOverrides() != null)
sb.append("Overrides: ").append(getOverrides()).append(",");
if (getPlacementConstraints() != null)
sb.append("PlacementConstraints: ").append(getPlacementConstraints()).append(",");
if (getPlacementStrategy() != null)
sb.append("PlacementStrategy: ").append(getPlacementStrategy()).append(",");
if (getPlatformVersion() != null)
sb.append("PlatformVersion: ").append(getPlatformVersion()).append(",");
if (getPropagateTags() != null)
sb.append("PropagateTags: ").append(getPropagateTags()).append(",");
if (getReferenceId() != null)
sb.append("ReferenceId: ").append(getReferenceId()).append(",");
if (getStartedBy() != null)
sb.append("StartedBy: ").append(getStartedBy()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getTaskDefinition() != null)
sb.append("TaskDefinition: ").append(getTaskDefinition());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof RunTaskRequest == false)
return false;
RunTaskRequest other = (RunTaskRequest) obj;
if (other.getCapacityProviderStrategy() == null ^ this.getCapacityProviderStrategy() == null)
return false;
if (other.getCapacityProviderStrategy() != null && other.getCapacityProviderStrategy().equals(this.getCapacityProviderStrategy()) == false)
return false;
if (other.getCluster() == null ^ this.getCluster() == null)
return false;
if (other.getCluster() != null && other.getCluster().equals(this.getCluster()) == false)
return false;
if (other.getCount() == null ^ this.getCount() == null)
return false;
if (other.getCount() != null && other.getCount().equals(this.getCount()) == false)
return false;
if (other.getEnableECSManagedTags() == null ^ this.getEnableECSManagedTags() == null)
return false;
if (other.getEnableECSManagedTags() != null && other.getEnableECSManagedTags().equals(this.getEnableECSManagedTags()) == false)
return false;
if (other.getEnableExecuteCommand() == null ^ this.getEnableExecuteCommand() == null)
return false;
if (other.getEnableExecuteCommand() != null && other.getEnableExecuteCommand().equals(this.getEnableExecuteCommand()) == false)
return false;
if (other.getGroup() == null ^ this.getGroup() == null)
return false;
if (other.getGroup() != null && other.getGroup().equals(this.getGroup()) == false)
return false;
if (other.getLaunchType() == null ^ this.getLaunchType() == null)
return false;
if (other.getLaunchType() != null && other.getLaunchType().equals(this.getLaunchType()) == false)
return false;
if (other.getNetworkConfiguration() == null ^ this.getNetworkConfiguration() == null)
return false;
if (other.getNetworkConfiguration() != null && other.getNetworkConfiguration().equals(this.getNetworkConfiguration()) == false)
return false;
if (other.getOverrides() == null ^ this.getOverrides() == null)
return false;
if (other.getOverrides() != null && other.getOverrides().equals(this.getOverrides()) == false)
return false;
if (other.getPlacementConstraints() == null ^ this.getPlacementConstraints() == null)
return false;
if (other.getPlacementConstraints() != null && other.getPlacementConstraints().equals(this.getPlacementConstraints()) == false)
return false;
if (other.getPlacementStrategy() == null ^ this.getPlacementStrategy() == null)
return false;
if (other.getPlacementStrategy() != null && other.getPlacementStrategy().equals(this.getPlacementStrategy()) == false)
return false;
if (other.getPlatformVersion() == null ^ this.getPlatformVersion() == null)
return false;
if (other.getPlatformVersion() != null && other.getPlatformVersion().equals(this.getPlatformVersion()) == false)
return false;
if (other.getPropagateTags() == null ^ this.getPropagateTags() == null)
return false;
if (other.getPropagateTags() != null && other.getPropagateTags().equals(this.getPropagateTags()) == false)
return false;
if (other.getReferenceId() == null ^ this.getReferenceId() == null)
return false;
if (other.getReferenceId() != null && other.getReferenceId().equals(this.getReferenceId()) == false)
return false;
if (other.getStartedBy() == null ^ this.getStartedBy() == null)
return false;
if (other.getStartedBy() != null && other.getStartedBy().equals(this.getStartedBy()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getTaskDefinition() == null ^ this.getTaskDefinition() == null)
return false;
if (other.getTaskDefinition() != null && other.getTaskDefinition().equals(this.getTaskDefinition()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCapacityProviderStrategy() == null) ? 0 : getCapacityProviderStrategy().hashCode());
hashCode = prime * hashCode + ((getCluster() == null) ? 0 : getCluster().hashCode());
hashCode = prime * hashCode + ((getCount() == null) ? 0 : getCount().hashCode());
hashCode = prime * hashCode + ((getEnableECSManagedTags() == null) ? 0 : getEnableECSManagedTags().hashCode());
hashCode = prime * hashCode + ((getEnableExecuteCommand() == null) ? 0 : getEnableExecuteCommand().hashCode());
hashCode = prime * hashCode + ((getGroup() == null) ? 0 : getGroup().hashCode());
hashCode = prime * hashCode + ((getLaunchType() == null) ? 0 : getLaunchType().hashCode());
hashCode = prime * hashCode + ((getNetworkConfiguration() == null) ? 0 : getNetworkConfiguration().hashCode());
hashCode = prime * hashCode + ((getOverrides() == null) ? 0 : getOverrides().hashCode());
hashCode = prime * hashCode + ((getPlacementConstraints() == null) ? 0 : getPlacementConstraints().hashCode());
hashCode = prime * hashCode + ((getPlacementStrategy() == null) ? 0 : getPlacementStrategy().hashCode());
hashCode = prime * hashCode + ((getPlatformVersion() == null) ? 0 : getPlatformVersion().hashCode());
hashCode = prime * hashCode + ((getPropagateTags() == null) ? 0 : getPropagateTags().hashCode());
hashCode = prime * hashCode + ((getReferenceId() == null) ? 0 : getReferenceId().hashCode());
hashCode = prime * hashCode + ((getStartedBy() == null) ? 0 : getStartedBy().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getTaskDefinition() == null) ? 0 : getTaskDefinition().hashCode());
return hashCode;
}
@Override
public RunTaskRequest clone() {
return (RunTaskRequest) super.clone();
}
}