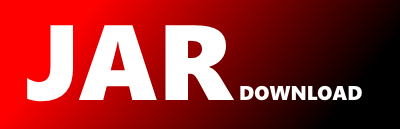
com.amazonaws.services.ecs.model.DockerVolumeConfiguration Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ecs Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ecs.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* This parameter is specified when you're using Docker volumes. Docker volumes are only supported when you're using the
* EC2 launch type. Windows containers only support the use of the local
driver. To use bind mounts,
* specify a host
instead.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DockerVolumeConfiguration implements Serializable, Cloneable, StructuredPojo {
/**
*
* The scope for the Docker volume that determines its lifecycle. Docker volumes that are scoped to a
* task
are automatically provisioned when the task starts and destroyed when the task stops. Docker
* volumes that are scoped as shared
persist after the task stops.
*
*/
private String scope;
/**
*
* If this value is true
, the Docker volume is created if it doesn't already exist.
*
*
*
* This field is only used if the scope
is shared
.
*
*
*/
private Boolean autoprovision;
/**
*
* The Docker volume driver to use. The driver value must match the driver name provided by Docker because it is
* used for task placement. If the driver was installed using the Docker plugin CLI, use
* docker plugin ls
to retrieve the driver name from your container instance. If the driver was
* installed using another method, use Docker plugin discovery to retrieve the driver name. For more information,
* see Docker plugin discovery.
* This parameter maps to Driver
in the Create a volume section of the Docker Remote API and the xxdriver
option to docker volume create.
*
*/
private String driver;
/**
*
* A map of Docker driver-specific options passed through. This parameter maps to DriverOpts
in the Create a volume section of the Docker Remote API and the xxopt
option to docker volume create.
*
*/
private java.util.Map driverOpts;
/**
*
* Custom metadata to add to your Docker volume. This parameter maps to Labels
in the Create a volume section of the Docker Remote API and the xxlabel
option to docker volume create.
*
*/
private java.util.Map labels;
/**
*
* The scope for the Docker volume that determines its lifecycle. Docker volumes that are scoped to a
* task
are automatically provisioned when the task starts and destroyed when the task stops. Docker
* volumes that are scoped as shared
persist after the task stops.
*
*
* @param scope
* The scope for the Docker volume that determines its lifecycle. Docker volumes that are scoped to a
* task
are automatically provisioned when the task starts and destroyed when the task stops.
* Docker volumes that are scoped as shared
persist after the task stops.
* @see Scope
*/
public void setScope(String scope) {
this.scope = scope;
}
/**
*
* The scope for the Docker volume that determines its lifecycle. Docker volumes that are scoped to a
* task
are automatically provisioned when the task starts and destroyed when the task stops. Docker
* volumes that are scoped as shared
persist after the task stops.
*
*
* @return The scope for the Docker volume that determines its lifecycle. Docker volumes that are scoped to a
* task
are automatically provisioned when the task starts and destroyed when the task stops.
* Docker volumes that are scoped as shared
persist after the task stops.
* @see Scope
*/
public String getScope() {
return this.scope;
}
/**
*
* The scope for the Docker volume that determines its lifecycle. Docker volumes that are scoped to a
* task
are automatically provisioned when the task starts and destroyed when the task stops. Docker
* volumes that are scoped as shared
persist after the task stops.
*
*
* @param scope
* The scope for the Docker volume that determines its lifecycle. Docker volumes that are scoped to a
* task
are automatically provisioned when the task starts and destroyed when the task stops.
* Docker volumes that are scoped as shared
persist after the task stops.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Scope
*/
public DockerVolumeConfiguration withScope(String scope) {
setScope(scope);
return this;
}
/**
*
* The scope for the Docker volume that determines its lifecycle. Docker volumes that are scoped to a
* task
are automatically provisioned when the task starts and destroyed when the task stops. Docker
* volumes that are scoped as shared
persist after the task stops.
*
*
* @param scope
* The scope for the Docker volume that determines its lifecycle. Docker volumes that are scoped to a
* task
are automatically provisioned when the task starts and destroyed when the task stops.
* Docker volumes that are scoped as shared
persist after the task stops.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Scope
*/
public DockerVolumeConfiguration withScope(Scope scope) {
this.scope = scope.toString();
return this;
}
/**
*
* If this value is true
, the Docker volume is created if it doesn't already exist.
*
*
*
* This field is only used if the scope
is shared
.
*
*
*
* @param autoprovision
* If this value is true
, the Docker volume is created if it doesn't already exist.
*
* This field is only used if the scope
is shared
.
*
*/
public void setAutoprovision(Boolean autoprovision) {
this.autoprovision = autoprovision;
}
/**
*
* If this value is true
, the Docker volume is created if it doesn't already exist.
*
*
*
* This field is only used if the scope
is shared
.
*
*
*
* @return If this value is true
, the Docker volume is created if it doesn't already exist.
*
* This field is only used if the scope
is shared
.
*
*/
public Boolean getAutoprovision() {
return this.autoprovision;
}
/**
*
* If this value is true
, the Docker volume is created if it doesn't already exist.
*
*
*
* This field is only used if the scope
is shared
.
*
*
*
* @param autoprovision
* If this value is true
, the Docker volume is created if it doesn't already exist.
*
* This field is only used if the scope
is shared
.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DockerVolumeConfiguration withAutoprovision(Boolean autoprovision) {
setAutoprovision(autoprovision);
return this;
}
/**
*
* If this value is true
, the Docker volume is created if it doesn't already exist.
*
*
*
* This field is only used if the scope
is shared
.
*
*
*
* @return If this value is true
, the Docker volume is created if it doesn't already exist.
*
* This field is only used if the scope
is shared
.
*
*/
public Boolean isAutoprovision() {
return this.autoprovision;
}
/**
*
* The Docker volume driver to use. The driver value must match the driver name provided by Docker because it is
* used for task placement. If the driver was installed using the Docker plugin CLI, use
* docker plugin ls
to retrieve the driver name from your container instance. If the driver was
* installed using another method, use Docker plugin discovery to retrieve the driver name. For more information,
* see Docker plugin discovery.
* This parameter maps to Driver
in the Create a volume section of the Docker Remote API and the xxdriver
option to docker volume create.
*
*
* @param driver
* The Docker volume driver to use. The driver value must match the driver name provided by Docker because it
* is used for task placement. If the driver was installed using the Docker plugin CLI, use
* docker plugin ls
to retrieve the driver name from your container instance. If the driver was
* installed using another method, use Docker plugin discovery to retrieve the driver name. For more
* information, see Docker
* plugin discovery. This parameter maps to Driver
in the Create a volume section of the
* Docker Remote API and the xxdriver
* option to docker volume
* create.
*/
public void setDriver(String driver) {
this.driver = driver;
}
/**
*
* The Docker volume driver to use. The driver value must match the driver name provided by Docker because it is
* used for task placement. If the driver was installed using the Docker plugin CLI, use
* docker plugin ls
to retrieve the driver name from your container instance. If the driver was
* installed using another method, use Docker plugin discovery to retrieve the driver name. For more information,
* see Docker plugin discovery.
* This parameter maps to Driver
in the Create a volume section of the Docker Remote API and the xxdriver
option to docker volume create.
*
*
* @return The Docker volume driver to use. The driver value must match the driver name provided by Docker because
* it is used for task placement. If the driver was installed using the Docker plugin CLI, use
* docker plugin ls
to retrieve the driver name from your container instance. If the driver was
* installed using another method, use Docker plugin discovery to retrieve the driver name. For more
* information, see Docker
* plugin discovery. This parameter maps to Driver
in the Create a volume section of
* the Docker Remote API and the
* xxdriver
option to docker volume create.
*/
public String getDriver() {
return this.driver;
}
/**
*
* The Docker volume driver to use. The driver value must match the driver name provided by Docker because it is
* used for task placement. If the driver was installed using the Docker plugin CLI, use
* docker plugin ls
to retrieve the driver name from your container instance. If the driver was
* installed using another method, use Docker plugin discovery to retrieve the driver name. For more information,
* see Docker plugin discovery.
* This parameter maps to Driver
in the Create a volume section of the Docker Remote API and the xxdriver
option to docker volume create.
*
*
* @param driver
* The Docker volume driver to use. The driver value must match the driver name provided by Docker because it
* is used for task placement. If the driver was installed using the Docker plugin CLI, use
* docker plugin ls
to retrieve the driver name from your container instance. If the driver was
* installed using another method, use Docker plugin discovery to retrieve the driver name. For more
* information, see Docker
* plugin discovery. This parameter maps to Driver
in the Create a volume section of the
* Docker Remote API and the xxdriver
* option to docker volume
* create.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DockerVolumeConfiguration withDriver(String driver) {
setDriver(driver);
return this;
}
/**
*
* A map of Docker driver-specific options passed through. This parameter maps to DriverOpts
in the Create a volume section of the Docker Remote API and the xxopt
option to docker volume create.
*
*
* @return A map of Docker driver-specific options passed through. This parameter maps to DriverOpts
in
* the Create a volume
* section of the Docker Remote API and the
* xxopt
option to docker volume create.
*/
public java.util.Map getDriverOpts() {
return driverOpts;
}
/**
*
* A map of Docker driver-specific options passed through. This parameter maps to DriverOpts
in the Create a volume section of the Docker Remote API and the xxopt
option to docker volume create.
*
*
* @param driverOpts
* A map of Docker driver-specific options passed through. This parameter maps to DriverOpts
in
* the Create a volume section
* of the Docker Remote API and the
* xxopt
option to docker volume create.
*/
public void setDriverOpts(java.util.Map driverOpts) {
this.driverOpts = driverOpts;
}
/**
*
* A map of Docker driver-specific options passed through. This parameter maps to DriverOpts
in the Create a volume section of the Docker Remote API and the xxopt
option to docker volume create.
*
*
* @param driverOpts
* A map of Docker driver-specific options passed through. This parameter maps to DriverOpts
in
* the Create a volume section
* of the Docker Remote API and the
* xxopt
option to docker volume create.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DockerVolumeConfiguration withDriverOpts(java.util.Map driverOpts) {
setDriverOpts(driverOpts);
return this;
}
/**
* Add a single DriverOpts entry
*
* @see DockerVolumeConfiguration#withDriverOpts
* @returns a reference to this object so that method calls can be chained together.
*/
public DockerVolumeConfiguration addDriverOptsEntry(String key, String value) {
if (null == this.driverOpts) {
this.driverOpts = new java.util.HashMap();
}
if (this.driverOpts.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.driverOpts.put(key, value);
return this;
}
/**
* Removes all the entries added into DriverOpts.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DockerVolumeConfiguration clearDriverOptsEntries() {
this.driverOpts = null;
return this;
}
/**
*
* Custom metadata to add to your Docker volume. This parameter maps to Labels
in the Create a volume section of the Docker Remote API and the xxlabel
option to docker volume create.
*
*
* @return Custom metadata to add to your Docker volume. This parameter maps to Labels
in the Create a volume section of
* the Docker Remote API and the
* xxlabel
option to docker volume create.
*/
public java.util.Map getLabels() {
return labels;
}
/**
*
* Custom metadata to add to your Docker volume. This parameter maps to Labels
in the Create a volume section of the Docker Remote API and the xxlabel
option to docker volume create.
*
*
* @param labels
* Custom metadata to add to your Docker volume. This parameter maps to Labels
in the Create a volume section of the
* Docker Remote API and the xxlabel
* option to docker volume
* create.
*/
public void setLabels(java.util.Map labels) {
this.labels = labels;
}
/**
*
* Custom metadata to add to your Docker volume. This parameter maps to Labels
in the Create a volume section of the Docker Remote API and the xxlabel
option to docker volume create.
*
*
* @param labels
* Custom metadata to add to your Docker volume. This parameter maps to Labels
in the Create a volume section of the
* Docker Remote API and the xxlabel
* option to docker volume
* create.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DockerVolumeConfiguration withLabels(java.util.Map labels) {
setLabels(labels);
return this;
}
/**
* Add a single Labels entry
*
* @see DockerVolumeConfiguration#withLabels
* @returns a reference to this object so that method calls can be chained together.
*/
public DockerVolumeConfiguration addLabelsEntry(String key, String value) {
if (null == this.labels) {
this.labels = new java.util.HashMap();
}
if (this.labels.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.labels.put(key, value);
return this;
}
/**
* Removes all the entries added into Labels.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DockerVolumeConfiguration clearLabelsEntries() {
this.labels = null;
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getScope() != null)
sb.append("Scope: ").append(getScope()).append(",");
if (getAutoprovision() != null)
sb.append("Autoprovision: ").append(getAutoprovision()).append(",");
if (getDriver() != null)
sb.append("Driver: ").append(getDriver()).append(",");
if (getDriverOpts() != null)
sb.append("DriverOpts: ").append(getDriverOpts()).append(",");
if (getLabels() != null)
sb.append("Labels: ").append(getLabels());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DockerVolumeConfiguration == false)
return false;
DockerVolumeConfiguration other = (DockerVolumeConfiguration) obj;
if (other.getScope() == null ^ this.getScope() == null)
return false;
if (other.getScope() != null && other.getScope().equals(this.getScope()) == false)
return false;
if (other.getAutoprovision() == null ^ this.getAutoprovision() == null)
return false;
if (other.getAutoprovision() != null && other.getAutoprovision().equals(this.getAutoprovision()) == false)
return false;
if (other.getDriver() == null ^ this.getDriver() == null)
return false;
if (other.getDriver() != null && other.getDriver().equals(this.getDriver()) == false)
return false;
if (other.getDriverOpts() == null ^ this.getDriverOpts() == null)
return false;
if (other.getDriverOpts() != null && other.getDriverOpts().equals(this.getDriverOpts()) == false)
return false;
if (other.getLabels() == null ^ this.getLabels() == null)
return false;
if (other.getLabels() != null && other.getLabels().equals(this.getLabels()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getScope() == null) ? 0 : getScope().hashCode());
hashCode = prime * hashCode + ((getAutoprovision() == null) ? 0 : getAutoprovision().hashCode());
hashCode = prime * hashCode + ((getDriver() == null) ? 0 : getDriver().hashCode());
hashCode = prime * hashCode + ((getDriverOpts() == null) ? 0 : getDriverOpts().hashCode());
hashCode = prime * hashCode + ((getLabels() == null) ? 0 : getLabels().hashCode());
return hashCode;
}
@Override
public DockerVolumeConfiguration clone() {
try {
return (DockerVolumeConfiguration) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.ecs.model.transform.DockerVolumeConfigurationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}