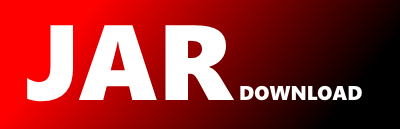
com.amazonaws.services.ecs.model.ServiceConnectService Maven / Gradle / Ivy
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ecs.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The Service Connect service object configuration. For more information, see Service Connect in the
* Amazon Elastic Container Service Developer Guide.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ServiceConnectService implements Serializable, Cloneable, StructuredPojo {
/**
*
* The portName
must match the name of one of the portMappings
from all the containers in
* the task definition of this Amazon ECS service.
*
*/
private String portName;
/**
*
* The discoveryName
is the name of the new Cloud Map service that Amazon ECS creates for this Amazon
* ECS service. This must be unique within the Cloud Map namespace. The name can contain up to 64 characters. The
* name can include lowercase letters, numbers, underscores (_), and hyphens (-). The name can't start with a
* hyphen.
*
*
* If the discoveryName
isn't specified, the port mapping name from the task definition is used in
* portName.namespace
.
*
*/
private String discoveryName;
/**
*
* The list of client aliases for this Service Connect service. You use these to assign names that can be used by
* client applications. The maximum number of client aliases that you can have in this list is 1.
*
*
* Each alias ("endpoint") is a fully-qualified name and port number that other Amazon ECS tasks ("clients") can use
* to connect to this service.
*
*
* Each name and port mapping must be unique within the namespace.
*
*
* For each ServiceConnectService
, you must provide at least one clientAlias
with one
* port
.
*
*/
private com.amazonaws.internal.SdkInternalList clientAliases;
/**
*
* The port number for the Service Connect proxy to listen on.
*
*
* Use the value of this field to bypass the proxy for traffic on the port number specified in the named
* portMapping
in the task definition of this application, and then use it in your VPC security groups
* to allow traffic into the proxy for this Amazon ECS service.
*
*
* In awsvpc
mode and Fargate, the default value is the container port number. The container port
* number is in the portMapping
in the task definition. In bridge mode, the default value is the
* ephemeral port of the Service Connect proxy.
*
*/
private Integer ingressPortOverride;
/**
*
* The portName
must match the name of one of the portMappings
from all the containers in
* the task definition of this Amazon ECS service.
*
*
* @param portName
* The portName
must match the name of one of the portMappings
from all the
* containers in the task definition of this Amazon ECS service.
*/
public void setPortName(String portName) {
this.portName = portName;
}
/**
*
* The portName
must match the name of one of the portMappings
from all the containers in
* the task definition of this Amazon ECS service.
*
*
* @return The portName
must match the name of one of the portMappings
from all the
* containers in the task definition of this Amazon ECS service.
*/
public String getPortName() {
return this.portName;
}
/**
*
* The portName
must match the name of one of the portMappings
from all the containers in
* the task definition of this Amazon ECS service.
*
*
* @param portName
* The portName
must match the name of one of the portMappings
from all the
* containers in the task definition of this Amazon ECS service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceConnectService withPortName(String portName) {
setPortName(portName);
return this;
}
/**
*
* The discoveryName
is the name of the new Cloud Map service that Amazon ECS creates for this Amazon
* ECS service. This must be unique within the Cloud Map namespace. The name can contain up to 64 characters. The
* name can include lowercase letters, numbers, underscores (_), and hyphens (-). The name can't start with a
* hyphen.
*
*
* If the discoveryName
isn't specified, the port mapping name from the task definition is used in
* portName.namespace
.
*
*
* @param discoveryName
* The discoveryName
is the name of the new Cloud Map service that Amazon ECS creates for this
* Amazon ECS service. This must be unique within the Cloud Map namespace. The name can contain up to 64
* characters. The name can include lowercase letters, numbers, underscores (_), and hyphens (-). The name
* can't start with a hyphen.
*
* If the discoveryName
isn't specified, the port mapping name from the task definition is used
* in portName.namespace
.
*/
public void setDiscoveryName(String discoveryName) {
this.discoveryName = discoveryName;
}
/**
*
* The discoveryName
is the name of the new Cloud Map service that Amazon ECS creates for this Amazon
* ECS service. This must be unique within the Cloud Map namespace. The name can contain up to 64 characters. The
* name can include lowercase letters, numbers, underscores (_), and hyphens (-). The name can't start with a
* hyphen.
*
*
* If the discoveryName
isn't specified, the port mapping name from the task definition is used in
* portName.namespace
.
*
*
* @return The discoveryName
is the name of the new Cloud Map service that Amazon ECS creates for this
* Amazon ECS service. This must be unique within the Cloud Map namespace. The name can contain up to 64
* characters. The name can include lowercase letters, numbers, underscores (_), and hyphens (-). The name
* can't start with a hyphen.
*
* If the discoveryName
isn't specified, the port mapping name from the task definition is used
* in portName.namespace
.
*/
public String getDiscoveryName() {
return this.discoveryName;
}
/**
*
* The discoveryName
is the name of the new Cloud Map service that Amazon ECS creates for this Amazon
* ECS service. This must be unique within the Cloud Map namespace. The name can contain up to 64 characters. The
* name can include lowercase letters, numbers, underscores (_), and hyphens (-). The name can't start with a
* hyphen.
*
*
* If the discoveryName
isn't specified, the port mapping name from the task definition is used in
* portName.namespace
.
*
*
* @param discoveryName
* The discoveryName
is the name of the new Cloud Map service that Amazon ECS creates for this
* Amazon ECS service. This must be unique within the Cloud Map namespace. The name can contain up to 64
* characters. The name can include lowercase letters, numbers, underscores (_), and hyphens (-). The name
* can't start with a hyphen.
*
* If the discoveryName
isn't specified, the port mapping name from the task definition is used
* in portName.namespace
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceConnectService withDiscoveryName(String discoveryName) {
setDiscoveryName(discoveryName);
return this;
}
/**
*
* The list of client aliases for this Service Connect service. You use these to assign names that can be used by
* client applications. The maximum number of client aliases that you can have in this list is 1.
*
*
* Each alias ("endpoint") is a fully-qualified name and port number that other Amazon ECS tasks ("clients") can use
* to connect to this service.
*
*
* Each name and port mapping must be unique within the namespace.
*
*
* For each ServiceConnectService
, you must provide at least one clientAlias
with one
* port
.
*
*
* @return The list of client aliases for this Service Connect service. You use these to assign names that can be
* used by client applications. The maximum number of client aliases that you can have in this list is
* 1.
*
* Each alias ("endpoint") is a fully-qualified name and port number that other Amazon ECS tasks ("clients")
* can use to connect to this service.
*
*
* Each name and port mapping must be unique within the namespace.
*
*
* For each ServiceConnectService
, you must provide at least one clientAlias
with
* one port
.
*/
public java.util.List getClientAliases() {
if (clientAliases == null) {
clientAliases = new com.amazonaws.internal.SdkInternalList();
}
return clientAliases;
}
/**
*
* The list of client aliases for this Service Connect service. You use these to assign names that can be used by
* client applications. The maximum number of client aliases that you can have in this list is 1.
*
*
* Each alias ("endpoint") is a fully-qualified name and port number that other Amazon ECS tasks ("clients") can use
* to connect to this service.
*
*
* Each name and port mapping must be unique within the namespace.
*
*
* For each ServiceConnectService
, you must provide at least one clientAlias
with one
* port
.
*
*
* @param clientAliases
* The list of client aliases for this Service Connect service. You use these to assign names that can be
* used by client applications. The maximum number of client aliases that you can have in this list is 1.
*
* Each alias ("endpoint") is a fully-qualified name and port number that other Amazon ECS tasks ("clients")
* can use to connect to this service.
*
*
* Each name and port mapping must be unique within the namespace.
*
*
* For each ServiceConnectService
, you must provide at least one clientAlias
with
* one port
.
*/
public void setClientAliases(java.util.Collection clientAliases) {
if (clientAliases == null) {
this.clientAliases = null;
return;
}
this.clientAliases = new com.amazonaws.internal.SdkInternalList(clientAliases);
}
/**
*
* The list of client aliases for this Service Connect service. You use these to assign names that can be used by
* client applications. The maximum number of client aliases that you can have in this list is 1.
*
*
* Each alias ("endpoint") is a fully-qualified name and port number that other Amazon ECS tasks ("clients") can use
* to connect to this service.
*
*
* Each name and port mapping must be unique within the namespace.
*
*
* For each ServiceConnectService
, you must provide at least one clientAlias
with one
* port
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setClientAliases(java.util.Collection)} or {@link #withClientAliases(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param clientAliases
* The list of client aliases for this Service Connect service. You use these to assign names that can be
* used by client applications. The maximum number of client aliases that you can have in this list is 1.
*
* Each alias ("endpoint") is a fully-qualified name and port number that other Amazon ECS tasks ("clients")
* can use to connect to this service.
*
*
* Each name and port mapping must be unique within the namespace.
*
*
* For each ServiceConnectService
, you must provide at least one clientAlias
with
* one port
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceConnectService withClientAliases(ServiceConnectClientAlias... clientAliases) {
if (this.clientAliases == null) {
setClientAliases(new com.amazonaws.internal.SdkInternalList(clientAliases.length));
}
for (ServiceConnectClientAlias ele : clientAliases) {
this.clientAliases.add(ele);
}
return this;
}
/**
*
* The list of client aliases for this Service Connect service. You use these to assign names that can be used by
* client applications. The maximum number of client aliases that you can have in this list is 1.
*
*
* Each alias ("endpoint") is a fully-qualified name and port number that other Amazon ECS tasks ("clients") can use
* to connect to this service.
*
*
* Each name and port mapping must be unique within the namespace.
*
*
* For each ServiceConnectService
, you must provide at least one clientAlias
with one
* port
.
*
*
* @param clientAliases
* The list of client aliases for this Service Connect service. You use these to assign names that can be
* used by client applications. The maximum number of client aliases that you can have in this list is 1.
*
* Each alias ("endpoint") is a fully-qualified name and port number that other Amazon ECS tasks ("clients")
* can use to connect to this service.
*
*
* Each name and port mapping must be unique within the namespace.
*
*
* For each ServiceConnectService
, you must provide at least one clientAlias
with
* one port
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceConnectService withClientAliases(java.util.Collection clientAliases) {
setClientAliases(clientAliases);
return this;
}
/**
*
* The port number for the Service Connect proxy to listen on.
*
*
* Use the value of this field to bypass the proxy for traffic on the port number specified in the named
* portMapping
in the task definition of this application, and then use it in your VPC security groups
* to allow traffic into the proxy for this Amazon ECS service.
*
*
* In awsvpc
mode and Fargate, the default value is the container port number. The container port
* number is in the portMapping
in the task definition. In bridge mode, the default value is the
* ephemeral port of the Service Connect proxy.
*
*
* @param ingressPortOverride
* The port number for the Service Connect proxy to listen on.
*
* Use the value of this field to bypass the proxy for traffic on the port number specified in the named
* portMapping
in the task definition of this application, and then use it in your VPC security
* groups to allow traffic into the proxy for this Amazon ECS service.
*
*
* In awsvpc
mode and Fargate, the default value is the container port number. The container
* port number is in the portMapping
in the task definition. In bridge mode, the default value
* is the ephemeral port of the Service Connect proxy.
*/
public void setIngressPortOverride(Integer ingressPortOverride) {
this.ingressPortOverride = ingressPortOverride;
}
/**
*
* The port number for the Service Connect proxy to listen on.
*
*
* Use the value of this field to bypass the proxy for traffic on the port number specified in the named
* portMapping
in the task definition of this application, and then use it in your VPC security groups
* to allow traffic into the proxy for this Amazon ECS service.
*
*
* In awsvpc
mode and Fargate, the default value is the container port number. The container port
* number is in the portMapping
in the task definition. In bridge mode, the default value is the
* ephemeral port of the Service Connect proxy.
*
*
* @return The port number for the Service Connect proxy to listen on.
*
* Use the value of this field to bypass the proxy for traffic on the port number specified in the named
* portMapping
in the task definition of this application, and then use it in your VPC security
* groups to allow traffic into the proxy for this Amazon ECS service.
*
*
* In awsvpc
mode and Fargate, the default value is the container port number. The container
* port number is in the portMapping
in the task definition. In bridge mode, the default value
* is the ephemeral port of the Service Connect proxy.
*/
public Integer getIngressPortOverride() {
return this.ingressPortOverride;
}
/**
*
* The port number for the Service Connect proxy to listen on.
*
*
* Use the value of this field to bypass the proxy for traffic on the port number specified in the named
* portMapping
in the task definition of this application, and then use it in your VPC security groups
* to allow traffic into the proxy for this Amazon ECS service.
*
*
* In awsvpc
mode and Fargate, the default value is the container port number. The container port
* number is in the portMapping
in the task definition. In bridge mode, the default value is the
* ephemeral port of the Service Connect proxy.
*
*
* @param ingressPortOverride
* The port number for the Service Connect proxy to listen on.
*
* Use the value of this field to bypass the proxy for traffic on the port number specified in the named
* portMapping
in the task definition of this application, and then use it in your VPC security
* groups to allow traffic into the proxy for this Amazon ECS service.
*
*
* In awsvpc
mode and Fargate, the default value is the container port number. The container
* port number is in the portMapping
in the task definition. In bridge mode, the default value
* is the ephemeral port of the Service Connect proxy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceConnectService withIngressPortOverride(Integer ingressPortOverride) {
setIngressPortOverride(ingressPortOverride);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getPortName() != null)
sb.append("PortName: ").append(getPortName()).append(",");
if (getDiscoveryName() != null)
sb.append("DiscoveryName: ").append(getDiscoveryName()).append(",");
if (getClientAliases() != null)
sb.append("ClientAliases: ").append(getClientAliases()).append(",");
if (getIngressPortOverride() != null)
sb.append("IngressPortOverride: ").append(getIngressPortOverride());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ServiceConnectService == false)
return false;
ServiceConnectService other = (ServiceConnectService) obj;
if (other.getPortName() == null ^ this.getPortName() == null)
return false;
if (other.getPortName() != null && other.getPortName().equals(this.getPortName()) == false)
return false;
if (other.getDiscoveryName() == null ^ this.getDiscoveryName() == null)
return false;
if (other.getDiscoveryName() != null && other.getDiscoveryName().equals(this.getDiscoveryName()) == false)
return false;
if (other.getClientAliases() == null ^ this.getClientAliases() == null)
return false;
if (other.getClientAliases() != null && other.getClientAliases().equals(this.getClientAliases()) == false)
return false;
if (other.getIngressPortOverride() == null ^ this.getIngressPortOverride() == null)
return false;
if (other.getIngressPortOverride() != null && other.getIngressPortOverride().equals(this.getIngressPortOverride()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getPortName() == null) ? 0 : getPortName().hashCode());
hashCode = prime * hashCode + ((getDiscoveryName() == null) ? 0 : getDiscoveryName().hashCode());
hashCode = prime * hashCode + ((getClientAliases() == null) ? 0 : getClientAliases().hashCode());
hashCode = prime * hashCode + ((getIngressPortOverride() == null) ? 0 : getIngressPortOverride().hashCode());
return hashCode;
}
@Override
public ServiceConnectService clone() {
try {
return (ServiceConnectService) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.ecs.model.transform.ServiceConnectServiceMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}