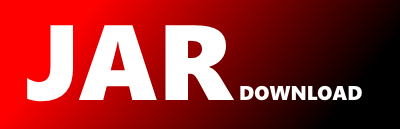
com.amazonaws.services.ecs.model.ServiceRegistry Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ecs Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ecs.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The details for the service registry.
*
*
* Each service may be associated with one service registry. Multiple service registries for each service are not
* supported.
*
*
* When you add, update, or remove the service registries configuration, Amazon ECS starts a new deployment. New tasks
* are registered and deregistered to the updated service registry configuration.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ServiceRegistry implements Serializable, Cloneable, StructuredPojo {
/**
*
* The Amazon Resource Name (ARN) of the service registry. The currently supported service registry is Cloud Map.
* For more information, see CreateService.
*
*/
private String registryArn;
/**
*
* The port value used if your service discovery service specified an SRV record. This field might be used if both
* the awsvpc
network mode and SRV records are used.
*
*/
private Integer port;
/**
*
* The container name value to be used for your service discovery service. It's already specified in the task
* definition. If the task definition that your service task specifies uses the bridge
or
* host
network mode, you must specify a containerName
and containerPort
* combination from the task definition. If the task definition that your service task specifies uses the
* awsvpc
network mode and a type SRV DNS record is used, you must specify either a
* containerName
and containerPort
combination or a port
value. However, you
* can't specify both.
*
*/
private String containerName;
/**
*
* The port value to be used for your service discovery service. It's already specified in the task definition. If
* the task definition your service task specifies uses the bridge
or host
network mode,
* you must specify a containerName
and containerPort
combination from the task
* definition. If the task definition your service task specifies uses the awsvpc
network mode and a
* type SRV DNS record is used, you must specify either a containerName
and containerPort
* combination or a port
value. However, you can't specify both.
*
*/
private Integer containerPort;
/**
*
* The Amazon Resource Name (ARN) of the service registry. The currently supported service registry is Cloud Map.
* For more information, see CreateService.
*
*
* @param registryArn
* The Amazon Resource Name (ARN) of the service registry. The currently supported service registry is Cloud
* Map. For more information, see CreateService.
*/
public void setRegistryArn(String registryArn) {
this.registryArn = registryArn;
}
/**
*
* The Amazon Resource Name (ARN) of the service registry. The currently supported service registry is Cloud Map.
* For more information, see CreateService.
*
*
* @return The Amazon Resource Name (ARN) of the service registry. The currently supported service registry is Cloud
* Map. For more information, see CreateService.
*/
public String getRegistryArn() {
return this.registryArn;
}
/**
*
* The Amazon Resource Name (ARN) of the service registry. The currently supported service registry is Cloud Map.
* For more information, see CreateService.
*
*
* @param registryArn
* The Amazon Resource Name (ARN) of the service registry. The currently supported service registry is Cloud
* Map. For more information, see CreateService.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceRegistry withRegistryArn(String registryArn) {
setRegistryArn(registryArn);
return this;
}
/**
*
* The port value used if your service discovery service specified an SRV record. This field might be used if both
* the awsvpc
network mode and SRV records are used.
*
*
* @param port
* The port value used if your service discovery service specified an SRV record. This field might be used if
* both the awsvpc
network mode and SRV records are used.
*/
public void setPort(Integer port) {
this.port = port;
}
/**
*
* The port value used if your service discovery service specified an SRV record. This field might be used if both
* the awsvpc
network mode and SRV records are used.
*
*
* @return The port value used if your service discovery service specified an SRV record. This field might be used
* if both the awsvpc
network mode and SRV records are used.
*/
public Integer getPort() {
return this.port;
}
/**
*
* The port value used if your service discovery service specified an SRV record. This field might be used if both
* the awsvpc
network mode and SRV records are used.
*
*
* @param port
* The port value used if your service discovery service specified an SRV record. This field might be used if
* both the awsvpc
network mode and SRV records are used.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceRegistry withPort(Integer port) {
setPort(port);
return this;
}
/**
*
* The container name value to be used for your service discovery service. It's already specified in the task
* definition. If the task definition that your service task specifies uses the bridge
or
* host
network mode, you must specify a containerName
and containerPort
* combination from the task definition. If the task definition that your service task specifies uses the
* awsvpc
network mode and a type SRV DNS record is used, you must specify either a
* containerName
and containerPort
combination or a port
value. However, you
* can't specify both.
*
*
* @param containerName
* The container name value to be used for your service discovery service. It's already specified in the task
* definition. If the task definition that your service task specifies uses the bridge
or
* host
network mode, you must specify a containerName
and
* containerPort
combination from the task definition. If the task definition that your service
* task specifies uses the awsvpc
network mode and a type SRV DNS record is used, you must
* specify either a containerName
and containerPort
combination or a
* port
value. However, you can't specify both.
*/
public void setContainerName(String containerName) {
this.containerName = containerName;
}
/**
*
* The container name value to be used for your service discovery service. It's already specified in the task
* definition. If the task definition that your service task specifies uses the bridge
or
* host
network mode, you must specify a containerName
and containerPort
* combination from the task definition. If the task definition that your service task specifies uses the
* awsvpc
network mode and a type SRV DNS record is used, you must specify either a
* containerName
and containerPort
combination or a port
value. However, you
* can't specify both.
*
*
* @return The container name value to be used for your service discovery service. It's already specified in the
* task definition. If the task definition that your service task specifies uses the bridge
or
* host
network mode, you must specify a containerName
and
* containerPort
combination from the task definition. If the task definition that your service
* task specifies uses the awsvpc
network mode and a type SRV DNS record is used, you must
* specify either a containerName
and containerPort
combination or a
* port
value. However, you can't specify both.
*/
public String getContainerName() {
return this.containerName;
}
/**
*
* The container name value to be used for your service discovery service. It's already specified in the task
* definition. If the task definition that your service task specifies uses the bridge
or
* host
network mode, you must specify a containerName
and containerPort
* combination from the task definition. If the task definition that your service task specifies uses the
* awsvpc
network mode and a type SRV DNS record is used, you must specify either a
* containerName
and containerPort
combination or a port
value. However, you
* can't specify both.
*
*
* @param containerName
* The container name value to be used for your service discovery service. It's already specified in the task
* definition. If the task definition that your service task specifies uses the bridge
or
* host
network mode, you must specify a containerName
and
* containerPort
combination from the task definition. If the task definition that your service
* task specifies uses the awsvpc
network mode and a type SRV DNS record is used, you must
* specify either a containerName
and containerPort
combination or a
* port
value. However, you can't specify both.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceRegistry withContainerName(String containerName) {
setContainerName(containerName);
return this;
}
/**
*
* The port value to be used for your service discovery service. It's already specified in the task definition. If
* the task definition your service task specifies uses the bridge
or host
network mode,
* you must specify a containerName
and containerPort
combination from the task
* definition. If the task definition your service task specifies uses the awsvpc
network mode and a
* type SRV DNS record is used, you must specify either a containerName
and containerPort
* combination or a port
value. However, you can't specify both.
*
*
* @param containerPort
* The port value to be used for your service discovery service. It's already specified in the task
* definition. If the task definition your service task specifies uses the bridge
or
* host
network mode, you must specify a containerName
and
* containerPort
combination from the task definition. If the task definition your service task
* specifies uses the awsvpc
network mode and a type SRV DNS record is used, you must specify
* either a containerName
and containerPort
combination or a port
* value. However, you can't specify both.
*/
public void setContainerPort(Integer containerPort) {
this.containerPort = containerPort;
}
/**
*
* The port value to be used for your service discovery service. It's already specified in the task definition. If
* the task definition your service task specifies uses the bridge
or host
network mode,
* you must specify a containerName
and containerPort
combination from the task
* definition. If the task definition your service task specifies uses the awsvpc
network mode and a
* type SRV DNS record is used, you must specify either a containerName
and containerPort
* combination or a port
value. However, you can't specify both.
*
*
* @return The port value to be used for your service discovery service. It's already specified in the task
* definition. If the task definition your service task specifies uses the bridge
or
* host
network mode, you must specify a containerName
and
* containerPort
combination from the task definition. If the task definition your service task
* specifies uses the awsvpc
network mode and a type SRV DNS record is used, you must specify
* either a containerName
and containerPort
combination or a port
* value. However, you can't specify both.
*/
public Integer getContainerPort() {
return this.containerPort;
}
/**
*
* The port value to be used for your service discovery service. It's already specified in the task definition. If
* the task definition your service task specifies uses the bridge
or host
network mode,
* you must specify a containerName
and containerPort
combination from the task
* definition. If the task definition your service task specifies uses the awsvpc
network mode and a
* type SRV DNS record is used, you must specify either a containerName
and containerPort
* combination or a port
value. However, you can't specify both.
*
*
* @param containerPort
* The port value to be used for your service discovery service. It's already specified in the task
* definition. If the task definition your service task specifies uses the bridge
or
* host
network mode, you must specify a containerName
and
* containerPort
combination from the task definition. If the task definition your service task
* specifies uses the awsvpc
network mode and a type SRV DNS record is used, you must specify
* either a containerName
and containerPort
combination or a port
* value. However, you can't specify both.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceRegistry withContainerPort(Integer containerPort) {
setContainerPort(containerPort);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getRegistryArn() != null)
sb.append("RegistryArn: ").append(getRegistryArn()).append(",");
if (getPort() != null)
sb.append("Port: ").append(getPort()).append(",");
if (getContainerName() != null)
sb.append("ContainerName: ").append(getContainerName()).append(",");
if (getContainerPort() != null)
sb.append("ContainerPort: ").append(getContainerPort());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ServiceRegistry == false)
return false;
ServiceRegistry other = (ServiceRegistry) obj;
if (other.getRegistryArn() == null ^ this.getRegistryArn() == null)
return false;
if (other.getRegistryArn() != null && other.getRegistryArn().equals(this.getRegistryArn()) == false)
return false;
if (other.getPort() == null ^ this.getPort() == null)
return false;
if (other.getPort() != null && other.getPort().equals(this.getPort()) == false)
return false;
if (other.getContainerName() == null ^ this.getContainerName() == null)
return false;
if (other.getContainerName() != null && other.getContainerName().equals(this.getContainerName()) == false)
return false;
if (other.getContainerPort() == null ^ this.getContainerPort() == null)
return false;
if (other.getContainerPort() != null && other.getContainerPort().equals(this.getContainerPort()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getRegistryArn() == null) ? 0 : getRegistryArn().hashCode());
hashCode = prime * hashCode + ((getPort() == null) ? 0 : getPort().hashCode());
hashCode = prime * hashCode + ((getContainerName() == null) ? 0 : getContainerName().hashCode());
hashCode = prime * hashCode + ((getContainerPort() == null) ? 0 : getContainerPort().hashCode());
return hashCode;
}
@Override
public ServiceRegistry clone() {
try {
return (ServiceRegistry) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.ecs.model.transform.ServiceRegistryMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}