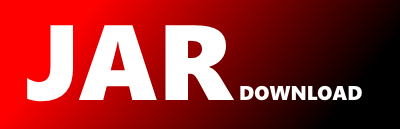
com.amazonaws.services.ecs.model.ContainerDefinition Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ecs Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ecs.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Container definitions are used in task definitions to describe the different containers that are launched as part of
* a task.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ContainerDefinition implements Serializable, Cloneable, StructuredPojo {
/**
*
* The name of a container. If you're linking multiple containers together in a task definition, the
* name
of one container can be entered in the links
of another container to connect the
* containers. Up to 255 letters (uppercase and lowercase), numbers, underscores, and hyphens are allowed. This
* parameter maps to name
in the Create a container section of the
* Docker Remote API and the --name
option to
* docker run.
*
*/
private String name;
/**
*
* The image used to start a container. This string is passed directly to the Docker daemon. By default, images in
* the Docker Hub registry are available. Other repositories are specified with either
* repository-url/image:tag
or
* repository-url/image@digest
. Up to 255 letters (uppercase and lowercase),
* numbers, hyphens, underscores, colons, periods, forward slashes, and number signs are allowed. This parameter
* maps to Image
in the Create a container section of the
* Docker Remote API and the IMAGE
parameter of
* docker run.
*
*
* -
*
* When a new task starts, the Amazon ECS container agent pulls the latest version of the specified image and tag
* for the container to use. However, subsequent updates to a repository image aren't propagated to already running
* tasks.
*
*
* -
*
* Images in Amazon ECR repositories can be specified by either using the full registry/repository:tag
* or registry/repository@digest
. For example,
* 012345678910.dkr.ecr.<region-name>.amazonaws.com/<repository-name>:latest
or
* 012345678910.dkr.ecr.<region-name>.amazonaws.com/<repository-name>@sha256:94afd1f2e64d908bc90dbca0035a5b567EXAMPLE
* .
*
*
* -
*
* Images in official repositories on Docker Hub use a single name (for example, ubuntu
or
* mongo
).
*
*
* -
*
* Images in other repositories on Docker Hub are qualified with an organization name (for example,
* amazon/amazon-ecs-agent
).
*
*
* -
*
* Images in other online repositories are qualified further by a domain name (for example,
* quay.io/assemblyline/ubuntu
).
*
*
*
*/
private String image;
/**
*
* The private repository authentication credentials to use.
*
*/
private RepositoryCredentials repositoryCredentials;
/**
*
* The number of cpu
units reserved for the container. This parameter maps to CpuShares
in
* the Create a container section
* of the Docker Remote API and the
* --cpu-shares
option to docker run.
*
*
* This field is optional for tasks using the Fargate launch type, and the only requirement is that the total amount
* of CPU reserved for all containers within a task be lower than the task-level cpu
value.
*
*
*
* You can determine the number of CPU units that are available per EC2 instance type by multiplying the vCPUs
* listed for that instance type on the Amazon EC2 Instances
* detail page by 1,024.
*
*
*
* Linux containers share unallocated CPU units with other containers on the container instance with the same ratio
* as their allocated amount. For example, if you run a single-container task on a single-core instance type with
* 512 CPU units specified for that container, and that's the only task running on the container instance, that
* container could use the full 1,024 CPU unit share at any given time. However, if you launched another copy of the
* same task on that container instance, each task is guaranteed a minimum of 512 CPU units when needed. Moreover,
* each container could float to higher CPU usage if the other container was not using it. If both tasks were 100%
* active all of the time, they would be limited to 512 CPU units.
*
*
* On Linux container instances, the Docker daemon on the container instance uses the CPU value to calculate the
* relative CPU share ratios for running containers. For more information, see CPU share constraint in the Docker
* documentation. The minimum valid CPU share value that the Linux kernel allows is 2. However, the CPU parameter
* isn't required, and you can use CPU values below 2 in your container definitions. For CPU values below 2
* (including null), the behavior varies based on your Amazon ECS container agent version:
*
*
* -
*
* Agent versions less than or equal to 1.1.0: Null and zero CPU values are passed to Docker as 0, which
* Docker then converts to 1,024 CPU shares. CPU values of 1 are passed to Docker as 1, which the Linux kernel
* converts to two CPU shares.
*
*
* -
*
* Agent versions greater than or equal to 1.2.0: Null, zero, and CPU values of 1 are passed to Docker as 2.
*
*
*
*
* On Windows container instances, the CPU limit is enforced as an absolute limit, or a quota. Windows containers
* only have access to the specified amount of CPU that's described in the task definition. A null or zero CPU value
* is passed to Docker as 0
, which Windows interprets as 1% of one CPU.
*
*/
private Integer cpu;
/**
*
* The amount (in MiB) of memory to present to the container. If your container attempts to exceed the memory
* specified here, the container is killed. The total amount of memory reserved for all containers within a task
* must be lower than the task memory
value, if one is specified. This parameter maps to
* Memory
in the Create a
* container section of the Docker Remote API and the
* --memory
option to docker run.
*
*
* If using the Fargate launch type, this parameter is optional.
*
*
* If using the EC2 launch type, you must specify either a task-level memory value or a container-level memory
* value. If you specify both a container-level memory
and memoryReservation
value,
* memory
must be greater than memoryReservation
. If you specify
* memoryReservation
, then that value is subtracted from the available memory resources for the
* container instance where the container is placed. Otherwise, the value of memory
is used.
*
*
* The Docker 20.10.0 or later daemon reserves a minimum of 6 MiB of memory for a container. So, don't specify less
* than 6 MiB of memory for your containers.
*
*
* The Docker 19.03.13-ce or earlier daemon reserves a minimum of 4 MiB of memory for a container. So, don't specify
* less than 4 MiB of memory for your containers.
*
*/
private Integer memory;
/**
*
* The soft limit (in MiB) of memory to reserve for the container. When system memory is under heavy contention,
* Docker attempts to keep the container memory to this soft limit. However, your container can consume more memory
* when it needs to, up to either the hard limit specified with the memory
parameter (if applicable),
* or all of the available memory on the container instance, whichever comes first. This parameter maps to
* MemoryReservation
in the Create a container section of the
* Docker Remote API and the
* --memory-reservation
option to docker run.
*
*
* If a task-level memory value is not specified, you must specify a non-zero integer for one or both of
* memory
or memoryReservation
in a container definition. If you specify both,
* memory
must be greater than memoryReservation
. If you specify
* memoryReservation
, then that value is subtracted from the available memory resources for the
* container instance where the container is placed. Otherwise, the value of memory
is used.
*
*
* For example, if your container normally uses 128 MiB of memory, but occasionally bursts to 256 MiB of memory for
* short periods of time, you can set a memoryReservation
of 128 MiB, and a memory
hard
* limit of 300 MiB. This configuration would allow the container to only reserve 128 MiB of memory from the
* remaining resources on the container instance, but also allow the container to consume more memory resources when
* needed.
*
*
* The Docker 20.10.0 or later daemon reserves a minimum of 6 MiB of memory for a container. So, don't specify less
* than 6 MiB of memory for your containers.
*
*
* The Docker 19.03.13-ce or earlier daemon reserves a minimum of 4 MiB of memory for a container. So, don't specify
* less than 4 MiB of memory for your containers.
*
*/
private Integer memoryReservation;
/**
*
* The links
parameter allows containers to communicate with each other without the need for port
* mappings. This parameter is only supported if the network mode of a task definition is bridge
. The
* name:internalName
construct is analogous to name:alias
in Docker links. Up to 255
* letters (uppercase and lowercase), numbers, underscores, and hyphens are allowed. For more information about
* linking Docker containers, go to Legacy container links in
* the Docker documentation. This parameter maps to Links
in the Create a container section of the
* Docker Remote API and the --link
option to
* docker run.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* Containers that are collocated on a single container instance may be able to communicate with each other without
* requiring links or host port mappings. Network isolation is achieved on the container instance using security
* groups and VPC settings.
*
*
*/
private com.amazonaws.internal.SdkInternalList links;
/**
*
* The list of port mappings for the container. Port mappings allow containers to access ports on the host container
* instance to send or receive traffic.
*
*
* For task definitions that use the awsvpc
network mode, only specify the containerPort
.
* The hostPort
can be left blank or it must be the same value as the containerPort
.
*
*
* Port mappings on Windows use the NetNAT
gateway address rather than localhost
. There's
* no loopback for port mappings on Windows, so you can't access a container's mapped port from the host itself.
*
*
* This parameter maps to PortBindings
in the Create a container section of the
* Docker Remote API and the --publish
option
* to docker run. If the network
* mode of a task definition is set to none
, then you can't specify port mappings. If the network mode
* of a task definition is set to host
, then host ports must either be undefined or they must match the
* container port in the port mapping.
*
*
*
* After a task reaches the RUNNING
status, manual and automatic host and container port assignments
* are visible in the Network Bindings section of a container description for a selected task in the Amazon
* ECS console. The assignments are also visible in the networkBindings
section DescribeTasks
* responses.
*
*
*/
private com.amazonaws.internal.SdkInternalList portMappings;
/**
*
* If the essential
parameter of a container is marked as true
, and that container fails
* or stops for any reason, all other containers that are part of the task are stopped. If the
* essential
parameter of a container is marked as false
, its failure doesn't affect the
* rest of the containers in a task. If this parameter is omitted, a container is assumed to be essential.
*
*
* All tasks must have at least one essential container. If you have an application that's composed of multiple
* containers, group containers that are used for a common purpose into components, and separate the different
* components into multiple task definitions. For more information, see Application
* Architecture in the Amazon Elastic Container Service Developer Guide.
*
*/
private Boolean essential;
/**
*
*
* Early versions of the Amazon ECS container agent don't properly handle entryPoint
parameters. If you
* have problems using entryPoint
, update your container agent or enter your commands and arguments as
* command
array items instead.
*
*
*
* The entry point that's passed to the container. This parameter maps to Entrypoint
in the Create a container section of the
* Docker Remote API and the --entrypoint
* option to docker run. For more
* information, see https://docs.docker.com/engine
* /reference/builder/#entrypoint.
*
*/
private com.amazonaws.internal.SdkInternalList entryPoint;
/**
*
* The command that's passed to the container. This parameter maps to Cmd
in the Create a container section of the
* Docker Remote API and the COMMAND
parameter
* to docker run. For more
* information, see https://docs.docker.com/engine/reference
* /builder/#cmd. If there are multiple arguments, each argument is a separated string in the array.
*
*/
private com.amazonaws.internal.SdkInternalList command;
/**
*
* The environment variables to pass to a container. This parameter maps to Env
in the Create a container section of the
* Docker Remote API and the --env
option to docker run.
*
*
*
* We don't recommend that you use plaintext environment variables for sensitive information, such as credential
* data.
*
*
*/
private com.amazonaws.internal.SdkInternalList environment;
/**
*
* A list of files containing the environment variables to pass to a container. This parameter maps to the
* --env-file
option to docker run.
*
*
* You can specify up to ten environment files. The file must have a .env
file extension. Each line in
* an environment file contains an environment variable in VARIABLE=VALUE
format. Lines beginning with
* #
are treated as comments and are ignored. For more information about the environment variable file
* syntax, see Declare default environment variables in
* file.
*
*
* If there are environment variables specified using the environment
parameter in a container
* definition, they take precedence over the variables contained within an environment file. If multiple environment
* files are specified that contain the same variable, they're processed from the top down. We recommend that you
* use unique variable names. For more information, see Specifying Environment
* Variables in the Amazon Elastic Container Service Developer Guide.
*
*/
private com.amazonaws.internal.SdkInternalList environmentFiles;
/**
*
* The mount points for data volumes in your container.
*
*
* This parameter maps to Volumes
in the Create a container section of the
* Docker Remote API and the --volume
option to
* docker run.
*
*
* Windows containers can mount whole directories on the same drive as $env:ProgramData
. Windows
* containers can't mount directories on a different drive, and mount point can't be across drives.
*
*/
private com.amazonaws.internal.SdkInternalList mountPoints;
/**
*
* Data volumes to mount from another container. This parameter maps to VolumesFrom
in the Create a container section of the
* Docker Remote API and the --volumes-from
* option to docker run.
*
*/
private com.amazonaws.internal.SdkInternalList volumesFrom;
/**
*
* Linux-specific modifications that are applied to the container, such as Linux kernel capabilities. For more
* information see KernelCapabilities.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*/
private LinuxParameters linuxParameters;
/**
*
* The secrets to pass to the container. For more information, see Specifying
* Sensitive Data in the Amazon Elastic Container Service Developer Guide.
*
*/
private com.amazonaws.internal.SdkInternalList secrets;
/**
*
* The dependencies defined for container startup and shutdown. A container can contain multiple dependencies on
* other containers in a task definition. When a dependency is defined for container startup, for container shutdown
* it is reversed.
*
*
* For tasks using the EC2 launch type, the container instances require at least version 1.26.0 of the container
* agent to turn on container dependencies. However, we recommend using the latest container agent version. For
* information about checking your agent version and updating to the latest version, see Updating the Amazon ECS
* Container Agent in the Amazon Elastic Container Service Developer Guide. If you're using an Amazon
* ECS-optimized Linux AMI, your instance needs at least version 1.26.0-1 of the ecs-init
package. If
* your container instances are launched from version 20190301
or later, then they contain the required
* versions of the container agent and ecs-init
. For more information, see Amazon ECS-optimized
* Linux AMI in the Amazon Elastic Container Service Developer Guide.
*
*
* For tasks using the Fargate launch type, the task or service requires the following platforms:
*
*
* -
*
* Linux platform version 1.3.0
or later.
*
*
* -
*
* Windows platform version 1.0.0
or later.
*
*
*
*/
private com.amazonaws.internal.SdkInternalList dependsOn;
/**
*
* Time duration (in seconds) to wait before giving up on resolving dependencies for a container. For example, you
* specify two containers in a task definition with containerA having a dependency on containerB reaching a
* COMPLETE
, SUCCESS
, or HEALTHY
status. If a startTimeout
value
* is specified for containerB and it doesn't reach the desired status within that time then containerA gives up and
* not start. This results in the task transitioning to a STOPPED
state.
*
*
*
* When the ECS_CONTAINER_START_TIMEOUT
container agent configuration variable is used, it's enforced
* independently from this start timeout value.
*
*
*
* For tasks using the Fargate launch type, the task or service requires the following platforms:
*
*
* -
*
* Linux platform version 1.3.0
or later.
*
*
* -
*
* Windows platform version 1.0.0
or later.
*
*
*
*
* For tasks using the EC2 launch type, your container instances require at least version 1.26.0
of the
* container agent to use a container start timeout value. However, we recommend using the latest container agent
* version. For information about checking your agent version and updating to the latest version, see Updating the Amazon ECS
* Container Agent in the Amazon Elastic Container Service Developer Guide. If you're using an Amazon
* ECS-optimized Linux AMI, your instance needs at least version 1.26.0-1
of the ecs-init
* package. If your container instances are launched from version 20190301
or later, then they contain
* the required versions of the container agent and ecs-init
. For more information, see Amazon ECS-optimized
* Linux AMI in the Amazon Elastic Container Service Developer Guide.
*
*
* The valid values are 2-120 seconds.
*
*/
private Integer startTimeout;
/**
*
* Time duration (in seconds) to wait before the container is forcefully killed if it doesn't exit normally on its
* own.
*
*
* For tasks using the Fargate launch type, the task or service requires the following platforms:
*
*
* -
*
* Linux platform version 1.3.0
or later.
*
*
* -
*
* Windows platform version 1.0.0
or later.
*
*
*
*
* The max stop timeout value is 120 seconds and if the parameter is not specified, the default value of 30 seconds
* is used.
*
*
* For tasks that use the EC2 launch type, if the stopTimeout
parameter isn't specified, the value set
* for the Amazon ECS container agent configuration variable ECS_CONTAINER_STOP_TIMEOUT
is used. If
* neither the stopTimeout
parameter or the ECS_CONTAINER_STOP_TIMEOUT
agent configuration
* variable are set, then the default values of 30 seconds for Linux containers and 30 seconds on Windows containers
* are used. Your container instances require at least version 1.26.0 of the container agent to use a container stop
* timeout value. However, we recommend using the latest container agent version. For information about checking
* your agent version and updating to the latest version, see Updating the Amazon ECS
* Container Agent in the Amazon Elastic Container Service Developer Guide. If you're using an Amazon
* ECS-optimized Linux AMI, your instance needs at least version 1.26.0-1 of the ecs-init
package. If
* your container instances are launched from version 20190301
or later, then they contain the required
* versions of the container agent and ecs-init
. For more information, see Amazon ECS-optimized
* Linux AMI in the Amazon Elastic Container Service Developer Guide.
*
*
* The valid values are 2-120 seconds.
*
*/
private Integer stopTimeout;
/**
*
* The hostname to use for your container. This parameter maps to Hostname
in the Create a container section of the
* Docker Remote API and the --hostname
option
* to docker run.
*
*
*
* The hostname
parameter is not supported if you're using the awsvpc
network mode.
*
*
*/
private String hostname;
/**
*
* The user to use inside the container. This parameter maps to User
in the Create a container section of the
* Docker Remote API and the --user
option to
* docker run.
*
*
*
* When running tasks using the host
network mode, don't run containers using the root user (UID 0). We
* recommend using a non-root user for better security.
*
*
*
* You can specify the user
using the following formats. If specifying a UID or GID, you must specify
* it as a positive integer.
*
*
* -
*
* user
*
*
* -
*
* user:group
*
*
* -
*
* uid
*
*
* -
*
* uid:gid
*
*
* -
*
* user:gid
*
*
* -
*
* uid:group
*
*
*
*
*
* This parameter is not supported for Windows containers.
*
*
*/
private String user;
/**
*
* The working directory to run commands inside the container in. This parameter maps to WorkingDir
in
* the Create a container section
* of the Docker Remote API and the --workdir
* option to docker run.
*
*/
private String workingDirectory;
/**
*
* When this parameter is true, networking is off within the container. This parameter maps to
* NetworkDisabled
in the Create a container section of the
* Docker Remote API.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*/
private Boolean disableNetworking;
/**
*
* When this parameter is true, the container is given elevated privileges on the host container instance (similar
* to the root
user). This parameter maps to Privileged
in the Create a container section of the
* Docker Remote API and the --privileged
* option to docker run.
*
*
*
* This parameter is not supported for Windows containers or tasks run on Fargate.
*
*
*/
private Boolean privileged;
/**
*
* When this parameter is true, the container is given read-only access to its root file system. This parameter maps
* to ReadonlyRootfs
in the Create a container section of the
* Docker Remote API and the --read-only
option
* to docker run.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*/
private Boolean readonlyRootFilesystem;
/**
*
* A list of DNS servers that are presented to the container. This parameter maps to Dns
in the Create a container section of the
* Docker Remote API and the --dns
option to docker run.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*/
private com.amazonaws.internal.SdkInternalList dnsServers;
/**
*
* A list of DNS search domains that are presented to the container. This parameter maps to DnsSearch
* in the Create a container
* section of the Docker Remote API and the
* --dns-search
option to docker run.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*/
private com.amazonaws.internal.SdkInternalList dnsSearchDomains;
/**
*
* A list of hostnames and IP address mappings to append to the /etc/hosts
file on the container. This
* parameter maps to ExtraHosts
in the Create a container section of the
* Docker Remote API and the --add-host
option
* to docker run.
*
*
*
* This parameter isn't supported for Windows containers or tasks that use the awsvpc
network mode.
*
*
*/
private com.amazonaws.internal.SdkInternalList extraHosts;
/**
*
* A list of strings to provide custom configuration for multiple security systems. For more information about valid
* values, see Docker Run Security
* Configuration. This field isn't valid for containers in tasks using the Fargate launch type.
*
*
* For Linux tasks on EC2, this parameter can be used to reference custom labels for SELinux and AppArmor
* multi-level security systems.
*
*
* For any tasks on EC2, this parameter can be used to reference a credential spec file that configures a container
* for Active Directory authentication. For more information, see Using gMSAs for Windows
* Containers and Using
* gMSAs for Linux Containers in the Amazon Elastic Container Service Developer Guide.
*
*
* This parameter maps to SecurityOpt
in the Create a container section of the
* Docker Remote API and the --security-opt
* option to docker run.
*
*
*
* The Amazon ECS container agent running on a container instance must register with the
* ECS_SELINUX_CAPABLE=true
or ECS_APPARMOR_CAPABLE=true
environment variables before
* containers placed on that instance can use these security options. For more information, see Amazon ECS Container
* Agent Configuration in the Amazon Elastic Container Service Developer Guide.
*
*
*
* For more information about valid values, see Docker Run Security
* Configuration.
*
*
* Valid values: "no-new-privileges" | "apparmor:PROFILE" | "label:value" | "credentialspec:CredentialSpecFilePath"
*
*/
private com.amazonaws.internal.SdkInternalList dockerSecurityOptions;
/**
*
* When this parameter is true
, you can deploy containerized applications that require
* stdin
or a tty
to be allocated. This parameter maps to OpenStdin
in the Create a container section of the
* Docker Remote API and the --interactive
* option to docker run.
*
*/
private Boolean interactive;
/**
*
* When this parameter is true
, a TTY is allocated. This parameter maps to Tty
in the Create a container section of the
* Docker Remote API and the --tty
option to docker run.
*
*/
private Boolean pseudoTerminal;
/**
*
* A key/value map of labels to add to the container. This parameter maps to Labels
in the Create a container section of the
* Docker Remote API and the --label
option to
* docker run. This parameter
* requires version 1.18 of the Docker Remote API or greater on your container instance. To check the Docker Remote
* API version on your container instance, log in to your container instance and run the following command:
* sudo docker version --format '{{.Server.APIVersion}}'
*
*/
private java.util.Map dockerLabels;
/**
*
* A list of ulimits
to set in the container. If a ulimit
value is specified in a task
* definition, it overrides the default values set by Docker. This parameter maps to Ulimits
in the Create a container section of the
* Docker Remote API and the --ulimit
option to
* docker run. Valid naming
* values are displayed in the Ulimit data type.
*
*
* Amazon ECS tasks hosted on Fargate use the default resource limit values set by the operating system with the
* exception of the nofile
resource limit parameter which Fargate overrides. The nofile
* resource limit sets a restriction on the number of open files that a container can use. The default
* nofile
soft limit is 1024
and the default hard limit is 4096
.
*
*
* This parameter requires version 1.18 of the Docker Remote API or greater on your container instance. To check the
* Docker Remote API version on your container instance, log in to your container instance and run the following
* command: sudo docker version --format '{{.Server.APIVersion}}'
*
*
*
* This parameter is not supported for Windows containers.
*
*
*/
private com.amazonaws.internal.SdkInternalList ulimits;
/**
*
* The log configuration specification for the container.
*
*
* This parameter maps to LogConfig
in the Create a container section of the
* Docker Remote API and the --log-driver
* option to docker run. By
* default, containers use the same logging driver that the Docker daemon uses. However the container can use a
* different logging driver than the Docker daemon by specifying a log driver with this parameter in the container
* definition. To use a different logging driver for a container, the log system must be configured properly on the
* container instance (or on a different log server for remote logging options). For more information about the
* options for different supported log drivers, see Configure logging drivers in the Docker
* documentation.
*
*
*
* Amazon ECS currently supports a subset of the logging drivers available to the Docker daemon (shown in the
* LogConfiguration data type). Additional log drivers may be available in future releases of the Amazon ECS
* container agent.
*
*
*
* This parameter requires version 1.18 of the Docker Remote API or greater on your container instance. To check the
* Docker Remote API version on your container instance, log in to your container instance and run the following
* command: sudo docker version --format '{{.Server.APIVersion}}'
*
*
*
* The Amazon ECS container agent running on a container instance must register the logging drivers available on
* that instance with the ECS_AVAILABLE_LOGGING_DRIVERS
environment variable before containers placed
* on that instance can use these log configuration options. For more information, see Amazon ECS Container
* Agent Configuration in the Amazon Elastic Container Service Developer Guide.
*
*
*/
private LogConfiguration logConfiguration;
/**
*
* The container health check command and associated configuration parameters for the container. This parameter maps
* to HealthCheck
in the Create a container section of the
* Docker Remote API and the HEALTHCHECK
* parameter of docker run.
*
*/
private HealthCheck healthCheck;
/**
*
* A list of namespaced kernel parameters to set in the container. This parameter maps to Sysctls
in
* the Create a container section
* of the Docker Remote API and the --sysctl
* option to docker run. For
* example, you can configure net.ipv4.tcp_keepalive_time
setting to maintain longer lived connections.
*
*
*
* We don't recommended that you specify network-related systemControls
parameters for multiple
* containers in a single task that also uses either the awsvpc
or host
network modes. For
* tasks that use the awsvpc
network mode, the container that's started last determines which
* systemControls
parameters take effect. For tasks that use the host
network mode, it
* changes the container instance's namespaced kernel parameters as well as the containers.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* This parameter is only supported for tasks that are hosted on Fargate if the tasks are using platform version
* 1.4.0
or later (Linux). This isn't supported for Windows containers on Fargate.
*
*
*/
private com.amazonaws.internal.SdkInternalList systemControls;
/**
*
* The type and amount of a resource to assign to a container. The only supported resource is a GPU.
*
*/
private com.amazonaws.internal.SdkInternalList resourceRequirements;
/**
*
* The FireLens configuration for the container. This is used to specify and configure a log router for container
* logs. For more information, see Custom Log Routing in
* the Amazon Elastic Container Service Developer Guide.
*
*/
private FirelensConfiguration firelensConfiguration;
/**
*
* A list of ARNs in SSM or Amazon S3 to a credential spec (CredSpec
) file that configures the
* container for Active Directory authentication. We recommend that you use this parameter instead of the
* dockerSecurityOptions
. The maximum number of ARNs is 1.
*
*
* There are two formats for each ARN.
*
*
* - credentialspecdomainless:MyARN
* -
*
* You use credentialspecdomainless:MyARN
to provide a CredSpec
with an additional section
* for a secret in Secrets Manager. You provide the login credentials to the domain in the secret.
*
*
* Each task that runs on any container instance can join different domains.
*
*
* You can use this format without joining the container instance to a domain.
*
*
* - credentialspec:MyARN
* -
*
* You use credentialspec:MyARN
to provide a CredSpec
for a single domain.
*
*
* You must join the container instance to the domain before you start any tasks that use this task definition.
*
*
*
*
* In both formats, replace MyARN
with the ARN in SSM or Amazon S3.
*
*
* If you provide a credentialspecdomainless:MyARN
, the credspec
must provide a ARN in
* Secrets Manager for a secret containing the username, password, and the domain to connect to. For better
* security, the instance isn't joined to the domain for domainless authentication. Other applications on the
* instance can't use the domainless credentials. You can use this parameter to run tasks on the same instance, even
* it the tasks need to join different domains. For more information, see Using gMSAs for Windows
* Containers and Using
* gMSAs for Linux Containers.
*
*/
private com.amazonaws.internal.SdkInternalList credentialSpecs;
/**
*
* The name of a container. If you're linking multiple containers together in a task definition, the
* name
of one container can be entered in the links
of another container to connect the
* containers. Up to 255 letters (uppercase and lowercase), numbers, underscores, and hyphens are allowed. This
* parameter maps to name
in the Create a container section of the
* Docker Remote API and the --name
option to
* docker run.
*
*
* @param name
* The name of a container. If you're linking multiple containers together in a task definition, the
* name
of one container can be entered in the links
of another container to
* connect the containers. Up to 255 letters (uppercase and lowercase), numbers, underscores, and hyphens are
* allowed. This parameter maps to name
in the Create a container section
* of the Docker Remote API and the
* --name
option to docker run.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of a container. If you're linking multiple containers together in a task definition, the
* name
of one container can be entered in the links
of another container to connect the
* containers. Up to 255 letters (uppercase and lowercase), numbers, underscores, and hyphens are allowed. This
* parameter maps to name
in the Create a container section of the
* Docker Remote API and the --name
option to
* docker run.
*
*
* @return The name of a container. If you're linking multiple containers together in a task definition, the
* name
of one container can be entered in the links
of another container to
* connect the containers. Up to 255 letters (uppercase and lowercase), numbers, underscores, and hyphens
* are allowed. This parameter maps to name
in the Create a container section
* of the Docker Remote API and the
* --name
option to docker run.
*/
public String getName() {
return this.name;
}
/**
*
* The name of a container. If you're linking multiple containers together in a task definition, the
* name
of one container can be entered in the links
of another container to connect the
* containers. Up to 255 letters (uppercase and lowercase), numbers, underscores, and hyphens are allowed. This
* parameter maps to name
in the Create a container section of the
* Docker Remote API and the --name
option to
* docker run.
*
*
* @param name
* The name of a container. If you're linking multiple containers together in a task definition, the
* name
of one container can be entered in the links
of another container to
* connect the containers. Up to 255 letters (uppercase and lowercase), numbers, underscores, and hyphens are
* allowed. This parameter maps to name
in the Create a container section
* of the Docker Remote API and the
* --name
option to docker run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withName(String name) {
setName(name);
return this;
}
/**
*
* The image used to start a container. This string is passed directly to the Docker daemon. By default, images in
* the Docker Hub registry are available. Other repositories are specified with either
* repository-url/image:tag
or
* repository-url/image@digest
. Up to 255 letters (uppercase and lowercase),
* numbers, hyphens, underscores, colons, periods, forward slashes, and number signs are allowed. This parameter
* maps to Image
in the Create a container section of the
* Docker Remote API and the IMAGE
parameter of
* docker run.
*
*
* -
*
* When a new task starts, the Amazon ECS container agent pulls the latest version of the specified image and tag
* for the container to use. However, subsequent updates to a repository image aren't propagated to already running
* tasks.
*
*
* -
*
* Images in Amazon ECR repositories can be specified by either using the full registry/repository:tag
* or registry/repository@digest
. For example,
* 012345678910.dkr.ecr.<region-name>.amazonaws.com/<repository-name>:latest
or
* 012345678910.dkr.ecr.<region-name>.amazonaws.com/<repository-name>@sha256:94afd1f2e64d908bc90dbca0035a5b567EXAMPLE
* .
*
*
* -
*
* Images in official repositories on Docker Hub use a single name (for example, ubuntu
or
* mongo
).
*
*
* -
*
* Images in other repositories on Docker Hub are qualified with an organization name (for example,
* amazon/amazon-ecs-agent
).
*
*
* -
*
* Images in other online repositories are qualified further by a domain name (for example,
* quay.io/assemblyline/ubuntu
).
*
*
*
*
* @param image
* The image used to start a container. This string is passed directly to the Docker daemon. By default,
* images in the Docker Hub registry are available. Other repositories are specified with either
* repository-url/image:tag
or
* repository-url/image@digest
. Up to 255 letters (uppercase and
* lowercase), numbers, hyphens, underscores, colons, periods, forward slashes, and number signs are allowed.
* This parameter maps to Image
in the Create a container section
* of the Docker Remote API and the
* IMAGE
parameter of docker run.
*
* -
*
* When a new task starts, the Amazon ECS container agent pulls the latest version of the specified image and
* tag for the container to use. However, subsequent updates to a repository image aren't propagated to
* already running tasks.
*
*
* -
*
* Images in Amazon ECR repositories can be specified by either using the full
* registry/repository:tag
or registry/repository@digest
. For example,
* 012345678910.dkr.ecr.<region-name>.amazonaws.com/<repository-name>:latest
or
* 012345678910.dkr.ecr.<region-name>.amazonaws.com/<repository-name>@sha256:94afd1f2e64d908bc90dbca0035a5b567EXAMPLE
* .
*
*
* -
*
* Images in official repositories on Docker Hub use a single name (for example, ubuntu
or
* mongo
).
*
*
* -
*
* Images in other repositories on Docker Hub are qualified with an organization name (for example,
* amazon/amazon-ecs-agent
).
*
*
* -
*
* Images in other online repositories are qualified further by a domain name (for example,
* quay.io/assemblyline/ubuntu
).
*
*
*/
public void setImage(String image) {
this.image = image;
}
/**
*
* The image used to start a container. This string is passed directly to the Docker daemon. By default, images in
* the Docker Hub registry are available. Other repositories are specified with either
* repository-url/image:tag
or
* repository-url/image@digest
. Up to 255 letters (uppercase and lowercase),
* numbers, hyphens, underscores, colons, periods, forward slashes, and number signs are allowed. This parameter
* maps to Image
in the Create a container section of the
* Docker Remote API and the IMAGE
parameter of
* docker run.
*
*
* -
*
* When a new task starts, the Amazon ECS container agent pulls the latest version of the specified image and tag
* for the container to use. However, subsequent updates to a repository image aren't propagated to already running
* tasks.
*
*
* -
*
* Images in Amazon ECR repositories can be specified by either using the full registry/repository:tag
* or registry/repository@digest
. For example,
* 012345678910.dkr.ecr.<region-name>.amazonaws.com/<repository-name>:latest
or
* 012345678910.dkr.ecr.<region-name>.amazonaws.com/<repository-name>@sha256:94afd1f2e64d908bc90dbca0035a5b567EXAMPLE
* .
*
*
* -
*
* Images in official repositories on Docker Hub use a single name (for example, ubuntu
or
* mongo
).
*
*
* -
*
* Images in other repositories on Docker Hub are qualified with an organization name (for example,
* amazon/amazon-ecs-agent
).
*
*
* -
*
* Images in other online repositories are qualified further by a domain name (for example,
* quay.io/assemblyline/ubuntu
).
*
*
*
*
* @return The image used to start a container. This string is passed directly to the Docker daemon. By default,
* images in the Docker Hub registry are available. Other repositories are specified with either
* repository-url/image:tag
or
* repository-url/image@digest
. Up to 255 letters (uppercase and
* lowercase), numbers, hyphens, underscores, colons, periods, forward slashes, and number signs are
* allowed. This parameter maps to Image
in the Create a container section
* of the Docker Remote API and the
* IMAGE
parameter of docker run.
*
* -
*
* When a new task starts, the Amazon ECS container agent pulls the latest version of the specified image
* and tag for the container to use. However, subsequent updates to a repository image aren't propagated to
* already running tasks.
*
*
* -
*
* Images in Amazon ECR repositories can be specified by either using the full
* registry/repository:tag
or registry/repository@digest
. For example,
* 012345678910.dkr.ecr.<region-name>.amazonaws.com/<repository-name>:latest
or
* 012345678910.dkr.ecr.<region-name>.amazonaws.com/<repository-name>@sha256:94afd1f2e64d908bc90dbca0035a5b567EXAMPLE
* .
*
*
* -
*
* Images in official repositories on Docker Hub use a single name (for example, ubuntu
or
* mongo
).
*
*
* -
*
* Images in other repositories on Docker Hub are qualified with an organization name (for example,
* amazon/amazon-ecs-agent
).
*
*
* -
*
* Images in other online repositories are qualified further by a domain name (for example,
* quay.io/assemblyline/ubuntu
).
*
*
*/
public String getImage() {
return this.image;
}
/**
*
* The image used to start a container. This string is passed directly to the Docker daemon. By default, images in
* the Docker Hub registry are available. Other repositories are specified with either
* repository-url/image:tag
or
* repository-url/image@digest
. Up to 255 letters (uppercase and lowercase),
* numbers, hyphens, underscores, colons, periods, forward slashes, and number signs are allowed. This parameter
* maps to Image
in the Create a container section of the
* Docker Remote API and the IMAGE
parameter of
* docker run.
*
*
* -
*
* When a new task starts, the Amazon ECS container agent pulls the latest version of the specified image and tag
* for the container to use. However, subsequent updates to a repository image aren't propagated to already running
* tasks.
*
*
* -
*
* Images in Amazon ECR repositories can be specified by either using the full registry/repository:tag
* or registry/repository@digest
. For example,
* 012345678910.dkr.ecr.<region-name>.amazonaws.com/<repository-name>:latest
or
* 012345678910.dkr.ecr.<region-name>.amazonaws.com/<repository-name>@sha256:94afd1f2e64d908bc90dbca0035a5b567EXAMPLE
* .
*
*
* -
*
* Images in official repositories on Docker Hub use a single name (for example, ubuntu
or
* mongo
).
*
*
* -
*
* Images in other repositories on Docker Hub are qualified with an organization name (for example,
* amazon/amazon-ecs-agent
).
*
*
* -
*
* Images in other online repositories are qualified further by a domain name (for example,
* quay.io/assemblyline/ubuntu
).
*
*
*
*
* @param image
* The image used to start a container. This string is passed directly to the Docker daemon. By default,
* images in the Docker Hub registry are available. Other repositories are specified with either
* repository-url/image:tag
or
* repository-url/image@digest
. Up to 255 letters (uppercase and
* lowercase), numbers, hyphens, underscores, colons, periods, forward slashes, and number signs are allowed.
* This parameter maps to Image
in the Create a container section
* of the Docker Remote API and the
* IMAGE
parameter of docker run.
*
* -
*
* When a new task starts, the Amazon ECS container agent pulls the latest version of the specified image and
* tag for the container to use. However, subsequent updates to a repository image aren't propagated to
* already running tasks.
*
*
* -
*
* Images in Amazon ECR repositories can be specified by either using the full
* registry/repository:tag
or registry/repository@digest
. For example,
* 012345678910.dkr.ecr.<region-name>.amazonaws.com/<repository-name>:latest
or
* 012345678910.dkr.ecr.<region-name>.amazonaws.com/<repository-name>@sha256:94afd1f2e64d908bc90dbca0035a5b567EXAMPLE
* .
*
*
* -
*
* Images in official repositories on Docker Hub use a single name (for example, ubuntu
or
* mongo
).
*
*
* -
*
* Images in other repositories on Docker Hub are qualified with an organization name (for example,
* amazon/amazon-ecs-agent
).
*
*
* -
*
* Images in other online repositories are qualified further by a domain name (for example,
* quay.io/assemblyline/ubuntu
).
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withImage(String image) {
setImage(image);
return this;
}
/**
*
* The private repository authentication credentials to use.
*
*
* @param repositoryCredentials
* The private repository authentication credentials to use.
*/
public void setRepositoryCredentials(RepositoryCredentials repositoryCredentials) {
this.repositoryCredentials = repositoryCredentials;
}
/**
*
* The private repository authentication credentials to use.
*
*
* @return The private repository authentication credentials to use.
*/
public RepositoryCredentials getRepositoryCredentials() {
return this.repositoryCredentials;
}
/**
*
* The private repository authentication credentials to use.
*
*
* @param repositoryCredentials
* The private repository authentication credentials to use.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withRepositoryCredentials(RepositoryCredentials repositoryCredentials) {
setRepositoryCredentials(repositoryCredentials);
return this;
}
/**
*
* The number of cpu
units reserved for the container. This parameter maps to CpuShares
in
* the Create a container section
* of the Docker Remote API and the
* --cpu-shares
option to docker run.
*
*
* This field is optional for tasks using the Fargate launch type, and the only requirement is that the total amount
* of CPU reserved for all containers within a task be lower than the task-level cpu
value.
*
*
*
* You can determine the number of CPU units that are available per EC2 instance type by multiplying the vCPUs
* listed for that instance type on the Amazon EC2 Instances
* detail page by 1,024.
*
*
*
* Linux containers share unallocated CPU units with other containers on the container instance with the same ratio
* as their allocated amount. For example, if you run a single-container task on a single-core instance type with
* 512 CPU units specified for that container, and that's the only task running on the container instance, that
* container could use the full 1,024 CPU unit share at any given time. However, if you launched another copy of the
* same task on that container instance, each task is guaranteed a minimum of 512 CPU units when needed. Moreover,
* each container could float to higher CPU usage if the other container was not using it. If both tasks were 100%
* active all of the time, they would be limited to 512 CPU units.
*
*
* On Linux container instances, the Docker daemon on the container instance uses the CPU value to calculate the
* relative CPU share ratios for running containers. For more information, see CPU share constraint in the Docker
* documentation. The minimum valid CPU share value that the Linux kernel allows is 2. However, the CPU parameter
* isn't required, and you can use CPU values below 2 in your container definitions. For CPU values below 2
* (including null), the behavior varies based on your Amazon ECS container agent version:
*
*
* -
*
* Agent versions less than or equal to 1.1.0: Null and zero CPU values are passed to Docker as 0, which
* Docker then converts to 1,024 CPU shares. CPU values of 1 are passed to Docker as 1, which the Linux kernel
* converts to two CPU shares.
*
*
* -
*
* Agent versions greater than or equal to 1.2.0: Null, zero, and CPU values of 1 are passed to Docker as 2.
*
*
*
*
* On Windows container instances, the CPU limit is enforced as an absolute limit, or a quota. Windows containers
* only have access to the specified amount of CPU that's described in the task definition. A null or zero CPU value
* is passed to Docker as 0
, which Windows interprets as 1% of one CPU.
*
*
* @param cpu
* The number of cpu
units reserved for the container. This parameter maps to
* CpuShares
in the Create a container section
* of the Docker Remote API and the
* --cpu-shares
option to docker run.
*
* This field is optional for tasks using the Fargate launch type, and the only requirement is that the total
* amount of CPU reserved for all containers within a task be lower than the task-level cpu
* value.
*
*
*
* You can determine the number of CPU units that are available per EC2 instance type by multiplying the
* vCPUs listed for that instance type on the Amazon EC2
* Instances detail page by 1,024.
*
*
*
* Linux containers share unallocated CPU units with other containers on the container instance with the same
* ratio as their allocated amount. For example, if you run a single-container task on a single-core instance
* type with 512 CPU units specified for that container, and that's the only task running on the container
* instance, that container could use the full 1,024 CPU unit share at any given time. However, if you
* launched another copy of the same task on that container instance, each task is guaranteed a minimum of
* 512 CPU units when needed. Moreover, each container could float to higher CPU usage if the other container
* was not using it. If both tasks were 100% active all of the time, they would be limited to 512 CPU units.
*
*
* On Linux container instances, the Docker daemon on the container instance uses the CPU value to calculate
* the relative CPU share ratios for running containers. For more information, see CPU share constraint in the
* Docker documentation. The minimum valid CPU share value that the Linux kernel allows is 2. However, the
* CPU parameter isn't required, and you can use CPU values below 2 in your container definitions. For CPU
* values below 2 (including null), the behavior varies based on your Amazon ECS container agent version:
*
*
* -
*
* Agent versions less than or equal to 1.1.0: Null and zero CPU values are passed to Docker as 0,
* which Docker then converts to 1,024 CPU shares. CPU values of 1 are passed to Docker as 1, which the Linux
* kernel converts to two CPU shares.
*
*
* -
*
* Agent versions greater than or equal to 1.2.0: Null, zero, and CPU values of 1 are passed to Docker
* as 2.
*
*
*
*
* On Windows container instances, the CPU limit is enforced as an absolute limit, or a quota. Windows
* containers only have access to the specified amount of CPU that's described in the task definition. A null
* or zero CPU value is passed to Docker as 0
, which Windows interprets as 1% of one CPU.
*/
public void setCpu(Integer cpu) {
this.cpu = cpu;
}
/**
*
* The number of cpu
units reserved for the container. This parameter maps to CpuShares
in
* the Create a container section
* of the Docker Remote API and the
* --cpu-shares
option to docker run.
*
*
* This field is optional for tasks using the Fargate launch type, and the only requirement is that the total amount
* of CPU reserved for all containers within a task be lower than the task-level cpu
value.
*
*
*
* You can determine the number of CPU units that are available per EC2 instance type by multiplying the vCPUs
* listed for that instance type on the Amazon EC2 Instances
* detail page by 1,024.
*
*
*
* Linux containers share unallocated CPU units with other containers on the container instance with the same ratio
* as their allocated amount. For example, if you run a single-container task on a single-core instance type with
* 512 CPU units specified for that container, and that's the only task running on the container instance, that
* container could use the full 1,024 CPU unit share at any given time. However, if you launched another copy of the
* same task on that container instance, each task is guaranteed a minimum of 512 CPU units when needed. Moreover,
* each container could float to higher CPU usage if the other container was not using it. If both tasks were 100%
* active all of the time, they would be limited to 512 CPU units.
*
*
* On Linux container instances, the Docker daemon on the container instance uses the CPU value to calculate the
* relative CPU share ratios for running containers. For more information, see CPU share constraint in the Docker
* documentation. The minimum valid CPU share value that the Linux kernel allows is 2. However, the CPU parameter
* isn't required, and you can use CPU values below 2 in your container definitions. For CPU values below 2
* (including null), the behavior varies based on your Amazon ECS container agent version:
*
*
* -
*
* Agent versions less than or equal to 1.1.0: Null and zero CPU values are passed to Docker as 0, which
* Docker then converts to 1,024 CPU shares. CPU values of 1 are passed to Docker as 1, which the Linux kernel
* converts to two CPU shares.
*
*
* -
*
* Agent versions greater than or equal to 1.2.0: Null, zero, and CPU values of 1 are passed to Docker as 2.
*
*
*
*
* On Windows container instances, the CPU limit is enforced as an absolute limit, or a quota. Windows containers
* only have access to the specified amount of CPU that's described in the task definition. A null or zero CPU value
* is passed to Docker as 0
, which Windows interprets as 1% of one CPU.
*
*
* @return The number of cpu
units reserved for the container. This parameter maps to
* CpuShares
in the Create a container section
* of the Docker Remote API and the
* --cpu-shares
option to docker run.
*
* This field is optional for tasks using the Fargate launch type, and the only requirement is that the
* total amount of CPU reserved for all containers within a task be lower than the task-level
* cpu
value.
*
*
*
* You can determine the number of CPU units that are available per EC2 instance type by multiplying the
* vCPUs listed for that instance type on the Amazon EC2
* Instances detail page by 1,024.
*
*
*
* Linux containers share unallocated CPU units with other containers on the container instance with the
* same ratio as their allocated amount. For example, if you run a single-container task on a single-core
* instance type with 512 CPU units specified for that container, and that's the only task running on the
* container instance, that container could use the full 1,024 CPU unit share at any given time. However, if
* you launched another copy of the same task on that container instance, each task is guaranteed a minimum
* of 512 CPU units when needed. Moreover, each container could float to higher CPU usage if the other
* container was not using it. If both tasks were 100% active all of the time, they would be limited to 512
* CPU units.
*
*
* On Linux container instances, the Docker daemon on the container instance uses the CPU value to calculate
* the relative CPU share ratios for running containers. For more information, see CPU share constraint in the
* Docker documentation. The minimum valid CPU share value that the Linux kernel allows is 2. However, the
* CPU parameter isn't required, and you can use CPU values below 2 in your container definitions. For CPU
* values below 2 (including null), the behavior varies based on your Amazon ECS container agent version:
*
*
* -
*
* Agent versions less than or equal to 1.1.0: Null and zero CPU values are passed to Docker as 0,
* which Docker then converts to 1,024 CPU shares. CPU values of 1 are passed to Docker as 1, which the
* Linux kernel converts to two CPU shares.
*
*
* -
*
* Agent versions greater than or equal to 1.2.0: Null, zero, and CPU values of 1 are passed to
* Docker as 2.
*
*
*
*
* On Windows container instances, the CPU limit is enforced as an absolute limit, or a quota. Windows
* containers only have access to the specified amount of CPU that's described in the task definition. A
* null or zero CPU value is passed to Docker as 0
, which Windows interprets as 1% of one CPU.
*/
public Integer getCpu() {
return this.cpu;
}
/**
*
* The number of cpu
units reserved for the container. This parameter maps to CpuShares
in
* the Create a container section
* of the Docker Remote API and the
* --cpu-shares
option to docker run.
*
*
* This field is optional for tasks using the Fargate launch type, and the only requirement is that the total amount
* of CPU reserved for all containers within a task be lower than the task-level cpu
value.
*
*
*
* You can determine the number of CPU units that are available per EC2 instance type by multiplying the vCPUs
* listed for that instance type on the Amazon EC2 Instances
* detail page by 1,024.
*
*
*
* Linux containers share unallocated CPU units with other containers on the container instance with the same ratio
* as their allocated amount. For example, if you run a single-container task on a single-core instance type with
* 512 CPU units specified for that container, and that's the only task running on the container instance, that
* container could use the full 1,024 CPU unit share at any given time. However, if you launched another copy of the
* same task on that container instance, each task is guaranteed a minimum of 512 CPU units when needed. Moreover,
* each container could float to higher CPU usage if the other container was not using it. If both tasks were 100%
* active all of the time, they would be limited to 512 CPU units.
*
*
* On Linux container instances, the Docker daemon on the container instance uses the CPU value to calculate the
* relative CPU share ratios for running containers. For more information, see CPU share constraint in the Docker
* documentation. The minimum valid CPU share value that the Linux kernel allows is 2. However, the CPU parameter
* isn't required, and you can use CPU values below 2 in your container definitions. For CPU values below 2
* (including null), the behavior varies based on your Amazon ECS container agent version:
*
*
* -
*
* Agent versions less than or equal to 1.1.0: Null and zero CPU values are passed to Docker as 0, which
* Docker then converts to 1,024 CPU shares. CPU values of 1 are passed to Docker as 1, which the Linux kernel
* converts to two CPU shares.
*
*
* -
*
* Agent versions greater than or equal to 1.2.0: Null, zero, and CPU values of 1 are passed to Docker as 2.
*
*
*
*
* On Windows container instances, the CPU limit is enforced as an absolute limit, or a quota. Windows containers
* only have access to the specified amount of CPU that's described in the task definition. A null or zero CPU value
* is passed to Docker as 0
, which Windows interprets as 1% of one CPU.
*
*
* @param cpu
* The number of cpu
units reserved for the container. This parameter maps to
* CpuShares
in the Create a container section
* of the Docker Remote API and the
* --cpu-shares
option to docker run.
*
* This field is optional for tasks using the Fargate launch type, and the only requirement is that the total
* amount of CPU reserved for all containers within a task be lower than the task-level cpu
* value.
*
*
*
* You can determine the number of CPU units that are available per EC2 instance type by multiplying the
* vCPUs listed for that instance type on the Amazon EC2
* Instances detail page by 1,024.
*
*
*
* Linux containers share unallocated CPU units with other containers on the container instance with the same
* ratio as their allocated amount. For example, if you run a single-container task on a single-core instance
* type with 512 CPU units specified for that container, and that's the only task running on the container
* instance, that container could use the full 1,024 CPU unit share at any given time. However, if you
* launched another copy of the same task on that container instance, each task is guaranteed a minimum of
* 512 CPU units when needed. Moreover, each container could float to higher CPU usage if the other container
* was not using it. If both tasks were 100% active all of the time, they would be limited to 512 CPU units.
*
*
* On Linux container instances, the Docker daemon on the container instance uses the CPU value to calculate
* the relative CPU share ratios for running containers. For more information, see CPU share constraint in the
* Docker documentation. The minimum valid CPU share value that the Linux kernel allows is 2. However, the
* CPU parameter isn't required, and you can use CPU values below 2 in your container definitions. For CPU
* values below 2 (including null), the behavior varies based on your Amazon ECS container agent version:
*
*
* -
*
* Agent versions less than or equal to 1.1.0: Null and zero CPU values are passed to Docker as 0,
* which Docker then converts to 1,024 CPU shares. CPU values of 1 are passed to Docker as 1, which the Linux
* kernel converts to two CPU shares.
*
*
* -
*
* Agent versions greater than or equal to 1.2.0: Null, zero, and CPU values of 1 are passed to Docker
* as 2.
*
*
*
*
* On Windows container instances, the CPU limit is enforced as an absolute limit, or a quota. Windows
* containers only have access to the specified amount of CPU that's described in the task definition. A null
* or zero CPU value is passed to Docker as 0
, which Windows interprets as 1% of one CPU.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withCpu(Integer cpu) {
setCpu(cpu);
return this;
}
/**
*
* The amount (in MiB) of memory to present to the container. If your container attempts to exceed the memory
* specified here, the container is killed. The total amount of memory reserved for all containers within a task
* must be lower than the task memory
value, if one is specified. This parameter maps to
* Memory
in the Create a
* container section of the Docker Remote API and the
* --memory
option to docker run.
*
*
* If using the Fargate launch type, this parameter is optional.
*
*
* If using the EC2 launch type, you must specify either a task-level memory value or a container-level memory
* value. If you specify both a container-level memory
and memoryReservation
value,
* memory
must be greater than memoryReservation
. If you specify
* memoryReservation
, then that value is subtracted from the available memory resources for the
* container instance where the container is placed. Otherwise, the value of memory
is used.
*
*
* The Docker 20.10.0 or later daemon reserves a minimum of 6 MiB of memory for a container. So, don't specify less
* than 6 MiB of memory for your containers.
*
*
* The Docker 19.03.13-ce or earlier daemon reserves a minimum of 4 MiB of memory for a container. So, don't specify
* less than 4 MiB of memory for your containers.
*
*
* @param memory
* The amount (in MiB) of memory to present to the container. If your container attempts to exceed the memory
* specified here, the container is killed. The total amount of memory reserved for all containers within a
* task must be lower than the task memory
value, if one is specified. This parameter maps to
* Memory
in the Create a container section
* of the Docker Remote API and the
* --memory
option to docker run.
*
* If using the Fargate launch type, this parameter is optional.
*
*
* If using the EC2 launch type, you must specify either a task-level memory value or a container-level
* memory value. If you specify both a container-level memory
and memoryReservation
* value, memory
must be greater than memoryReservation
. If you specify
* memoryReservation
, then that value is subtracted from the available memory resources for the
* container instance where the container is placed. Otherwise, the value of memory
is used.
*
*
* The Docker 20.10.0 or later daemon reserves a minimum of 6 MiB of memory for a container. So, don't
* specify less than 6 MiB of memory for your containers.
*
*
* The Docker 19.03.13-ce or earlier daemon reserves a minimum of 4 MiB of memory for a container. So, don't
* specify less than 4 MiB of memory for your containers.
*/
public void setMemory(Integer memory) {
this.memory = memory;
}
/**
*
* The amount (in MiB) of memory to present to the container. If your container attempts to exceed the memory
* specified here, the container is killed. The total amount of memory reserved for all containers within a task
* must be lower than the task memory
value, if one is specified. This parameter maps to
* Memory
in the Create a
* container section of the Docker Remote API and the
* --memory
option to docker run.
*
*
* If using the Fargate launch type, this parameter is optional.
*
*
* If using the EC2 launch type, you must specify either a task-level memory value or a container-level memory
* value. If you specify both a container-level memory
and memoryReservation
value,
* memory
must be greater than memoryReservation
. If you specify
* memoryReservation
, then that value is subtracted from the available memory resources for the
* container instance where the container is placed. Otherwise, the value of memory
is used.
*
*
* The Docker 20.10.0 or later daemon reserves a minimum of 6 MiB of memory for a container. So, don't specify less
* than 6 MiB of memory for your containers.
*
*
* The Docker 19.03.13-ce or earlier daemon reserves a minimum of 4 MiB of memory for a container. So, don't specify
* less than 4 MiB of memory for your containers.
*
*
* @return The amount (in MiB) of memory to present to the container. If your container attempts to exceed the
* memory specified here, the container is killed. The total amount of memory reserved for all containers
* within a task must be lower than the task memory
value, if one is specified. This parameter
* maps to Memory
in the Create a container section
* of the Docker Remote API and the
* --memory
option to docker run.
*
* If using the Fargate launch type, this parameter is optional.
*
*
* If using the EC2 launch type, you must specify either a task-level memory value or a container-level
* memory value. If you specify both a container-level memory
and
* memoryReservation
value, memory
must be greater than
* memoryReservation
. If you specify memoryReservation
, then that value is
* subtracted from the available memory resources for the container instance where the container is placed.
* Otherwise, the value of memory
is used.
*
*
* The Docker 20.10.0 or later daemon reserves a minimum of 6 MiB of memory for a container. So, don't
* specify less than 6 MiB of memory for your containers.
*
*
* The Docker 19.03.13-ce or earlier daemon reserves a minimum of 4 MiB of memory for a container. So, don't
* specify less than 4 MiB of memory for your containers.
*/
public Integer getMemory() {
return this.memory;
}
/**
*
* The amount (in MiB) of memory to present to the container. If your container attempts to exceed the memory
* specified here, the container is killed. The total amount of memory reserved for all containers within a task
* must be lower than the task memory
value, if one is specified. This parameter maps to
* Memory
in the Create a
* container section of the Docker Remote API and the
* --memory
option to docker run.
*
*
* If using the Fargate launch type, this parameter is optional.
*
*
* If using the EC2 launch type, you must specify either a task-level memory value or a container-level memory
* value. If you specify both a container-level memory
and memoryReservation
value,
* memory
must be greater than memoryReservation
. If you specify
* memoryReservation
, then that value is subtracted from the available memory resources for the
* container instance where the container is placed. Otherwise, the value of memory
is used.
*
*
* The Docker 20.10.0 or later daemon reserves a minimum of 6 MiB of memory for a container. So, don't specify less
* than 6 MiB of memory for your containers.
*
*
* The Docker 19.03.13-ce or earlier daemon reserves a minimum of 4 MiB of memory for a container. So, don't specify
* less than 4 MiB of memory for your containers.
*
*
* @param memory
* The amount (in MiB) of memory to present to the container. If your container attempts to exceed the memory
* specified here, the container is killed. The total amount of memory reserved for all containers within a
* task must be lower than the task memory
value, if one is specified. This parameter maps to
* Memory
in the Create a container section
* of the Docker Remote API and the
* --memory
option to docker run.
*
* If using the Fargate launch type, this parameter is optional.
*
*
* If using the EC2 launch type, you must specify either a task-level memory value or a container-level
* memory value. If you specify both a container-level memory
and memoryReservation
* value, memory
must be greater than memoryReservation
. If you specify
* memoryReservation
, then that value is subtracted from the available memory resources for the
* container instance where the container is placed. Otherwise, the value of memory
is used.
*
*
* The Docker 20.10.0 or later daemon reserves a minimum of 6 MiB of memory for a container. So, don't
* specify less than 6 MiB of memory for your containers.
*
*
* The Docker 19.03.13-ce or earlier daemon reserves a minimum of 4 MiB of memory for a container. So, don't
* specify less than 4 MiB of memory for your containers.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withMemory(Integer memory) {
setMemory(memory);
return this;
}
/**
*
* The soft limit (in MiB) of memory to reserve for the container. When system memory is under heavy contention,
* Docker attempts to keep the container memory to this soft limit. However, your container can consume more memory
* when it needs to, up to either the hard limit specified with the memory
parameter (if applicable),
* or all of the available memory on the container instance, whichever comes first. This parameter maps to
* MemoryReservation
in the Create a container section of the
* Docker Remote API and the
* --memory-reservation
option to docker run.
*
*
* If a task-level memory value is not specified, you must specify a non-zero integer for one or both of
* memory
or memoryReservation
in a container definition. If you specify both,
* memory
must be greater than memoryReservation
. If you specify
* memoryReservation
, then that value is subtracted from the available memory resources for the
* container instance where the container is placed. Otherwise, the value of memory
is used.
*
*
* For example, if your container normally uses 128 MiB of memory, but occasionally bursts to 256 MiB of memory for
* short periods of time, you can set a memoryReservation
of 128 MiB, and a memory
hard
* limit of 300 MiB. This configuration would allow the container to only reserve 128 MiB of memory from the
* remaining resources on the container instance, but also allow the container to consume more memory resources when
* needed.
*
*
* The Docker 20.10.0 or later daemon reserves a minimum of 6 MiB of memory for a container. So, don't specify less
* than 6 MiB of memory for your containers.
*
*
* The Docker 19.03.13-ce or earlier daemon reserves a minimum of 4 MiB of memory for a container. So, don't specify
* less than 4 MiB of memory for your containers.
*
*
* @param memoryReservation
* The soft limit (in MiB) of memory to reserve for the container. When system memory is under heavy
* contention, Docker attempts to keep the container memory to this soft limit. However, your container can
* consume more memory when it needs to, up to either the hard limit specified with the memory
* parameter (if applicable), or all of the available memory on the container instance, whichever comes
* first. This parameter maps to MemoryReservation
in the Create a container section
* of the Docker Remote API and the
* --memory-reservation
option to docker run.
*
* If a task-level memory value is not specified, you must specify a non-zero integer for one or both of
* memory
or memoryReservation
in a container definition. If you specify both,
* memory
must be greater than memoryReservation
. If you specify
* memoryReservation
, then that value is subtracted from the available memory resources for the
* container instance where the container is placed. Otherwise, the value of memory
is used.
*
*
* For example, if your container normally uses 128 MiB of memory, but occasionally bursts to 256 MiB of
* memory for short periods of time, you can set a memoryReservation
of 128 MiB, and a
* memory
hard limit of 300 MiB. This configuration would allow the container to only reserve
* 128 MiB of memory from the remaining resources on the container instance, but also allow the container to
* consume more memory resources when needed.
*
*
* The Docker 20.10.0 or later daemon reserves a minimum of 6 MiB of memory for a container. So, don't
* specify less than 6 MiB of memory for your containers.
*
*
* The Docker 19.03.13-ce or earlier daemon reserves a minimum of 4 MiB of memory for a container. So, don't
* specify less than 4 MiB of memory for your containers.
*/
public void setMemoryReservation(Integer memoryReservation) {
this.memoryReservation = memoryReservation;
}
/**
*
* The soft limit (in MiB) of memory to reserve for the container. When system memory is under heavy contention,
* Docker attempts to keep the container memory to this soft limit. However, your container can consume more memory
* when it needs to, up to either the hard limit specified with the memory
parameter (if applicable),
* or all of the available memory on the container instance, whichever comes first. This parameter maps to
* MemoryReservation
in the Create a container section of the
* Docker Remote API and the
* --memory-reservation
option to docker run.
*
*
* If a task-level memory value is not specified, you must specify a non-zero integer for one or both of
* memory
or memoryReservation
in a container definition. If you specify both,
* memory
must be greater than memoryReservation
. If you specify
* memoryReservation
, then that value is subtracted from the available memory resources for the
* container instance where the container is placed. Otherwise, the value of memory
is used.
*
*
* For example, if your container normally uses 128 MiB of memory, but occasionally bursts to 256 MiB of memory for
* short periods of time, you can set a memoryReservation
of 128 MiB, and a memory
hard
* limit of 300 MiB. This configuration would allow the container to only reserve 128 MiB of memory from the
* remaining resources on the container instance, but also allow the container to consume more memory resources when
* needed.
*
*
* The Docker 20.10.0 or later daemon reserves a minimum of 6 MiB of memory for a container. So, don't specify less
* than 6 MiB of memory for your containers.
*
*
* The Docker 19.03.13-ce or earlier daemon reserves a minimum of 4 MiB of memory for a container. So, don't specify
* less than 4 MiB of memory for your containers.
*
*
* @return The soft limit (in MiB) of memory to reserve for the container. When system memory is under heavy
* contention, Docker attempts to keep the container memory to this soft limit. However, your container can
* consume more memory when it needs to, up to either the hard limit specified with the memory
* parameter (if applicable), or all of the available memory on the container instance, whichever comes
* first. This parameter maps to MemoryReservation
in the Create a container section
* of the Docker Remote API and the
* --memory-reservation
option to docker run.
*
* If a task-level memory value is not specified, you must specify a non-zero integer for one or both of
* memory
or memoryReservation
in a container definition. If you specify both,
* memory
must be greater than memoryReservation
. If you specify
* memoryReservation
, then that value is subtracted from the available memory resources for the
* container instance where the container is placed. Otherwise, the value of memory
is used.
*
*
* For example, if your container normally uses 128 MiB of memory, but occasionally bursts to 256 MiB of
* memory for short periods of time, you can set a memoryReservation
of 128 MiB, and a
* memory
hard limit of 300 MiB. This configuration would allow the container to only reserve
* 128 MiB of memory from the remaining resources on the container instance, but also allow the container to
* consume more memory resources when needed.
*
*
* The Docker 20.10.0 or later daemon reserves a minimum of 6 MiB of memory for a container. So, don't
* specify less than 6 MiB of memory for your containers.
*
*
* The Docker 19.03.13-ce or earlier daemon reserves a minimum of 4 MiB of memory for a container. So, don't
* specify less than 4 MiB of memory for your containers.
*/
public Integer getMemoryReservation() {
return this.memoryReservation;
}
/**
*
* The soft limit (in MiB) of memory to reserve for the container. When system memory is under heavy contention,
* Docker attempts to keep the container memory to this soft limit. However, your container can consume more memory
* when it needs to, up to either the hard limit specified with the memory
parameter (if applicable),
* or all of the available memory on the container instance, whichever comes first. This parameter maps to
* MemoryReservation
in the Create a container section of the
* Docker Remote API and the
* --memory-reservation
option to docker run.
*
*
* If a task-level memory value is not specified, you must specify a non-zero integer for one or both of
* memory
or memoryReservation
in a container definition. If you specify both,
* memory
must be greater than memoryReservation
. If you specify
* memoryReservation
, then that value is subtracted from the available memory resources for the
* container instance where the container is placed. Otherwise, the value of memory
is used.
*
*
* For example, if your container normally uses 128 MiB of memory, but occasionally bursts to 256 MiB of memory for
* short periods of time, you can set a memoryReservation
of 128 MiB, and a memory
hard
* limit of 300 MiB. This configuration would allow the container to only reserve 128 MiB of memory from the
* remaining resources on the container instance, but also allow the container to consume more memory resources when
* needed.
*
*
* The Docker 20.10.0 or later daemon reserves a minimum of 6 MiB of memory for a container. So, don't specify less
* than 6 MiB of memory for your containers.
*
*
* The Docker 19.03.13-ce or earlier daemon reserves a minimum of 4 MiB of memory for a container. So, don't specify
* less than 4 MiB of memory for your containers.
*
*
* @param memoryReservation
* The soft limit (in MiB) of memory to reserve for the container. When system memory is under heavy
* contention, Docker attempts to keep the container memory to this soft limit. However, your container can
* consume more memory when it needs to, up to either the hard limit specified with the memory
* parameter (if applicable), or all of the available memory on the container instance, whichever comes
* first. This parameter maps to MemoryReservation
in the Create a container section
* of the Docker Remote API and the
* --memory-reservation
option to docker run.
*
* If a task-level memory value is not specified, you must specify a non-zero integer for one or both of
* memory
or memoryReservation
in a container definition. If you specify both,
* memory
must be greater than memoryReservation
. If you specify
* memoryReservation
, then that value is subtracted from the available memory resources for the
* container instance where the container is placed. Otherwise, the value of memory
is used.
*
*
* For example, if your container normally uses 128 MiB of memory, but occasionally bursts to 256 MiB of
* memory for short periods of time, you can set a memoryReservation
of 128 MiB, and a
* memory
hard limit of 300 MiB. This configuration would allow the container to only reserve
* 128 MiB of memory from the remaining resources on the container instance, but also allow the container to
* consume more memory resources when needed.
*
*
* The Docker 20.10.0 or later daemon reserves a minimum of 6 MiB of memory for a container. So, don't
* specify less than 6 MiB of memory for your containers.
*
*
* The Docker 19.03.13-ce or earlier daemon reserves a minimum of 4 MiB of memory for a container. So, don't
* specify less than 4 MiB of memory for your containers.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withMemoryReservation(Integer memoryReservation) {
setMemoryReservation(memoryReservation);
return this;
}
/**
*
* The links
parameter allows containers to communicate with each other without the need for port
* mappings. This parameter is only supported if the network mode of a task definition is bridge
. The
* name:internalName
construct is analogous to name:alias
in Docker links. Up to 255
* letters (uppercase and lowercase), numbers, underscores, and hyphens are allowed. For more information about
* linking Docker containers, go to Legacy container links in
* the Docker documentation. This parameter maps to Links
in the Create a container section of the
* Docker Remote API and the --link
option to
* docker run.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* Containers that are collocated on a single container instance may be able to communicate with each other without
* requiring links or host port mappings. Network isolation is achieved on the container instance using security
* groups and VPC settings.
*
*
*
* @return The links
parameter allows containers to communicate with each other without the need for
* port mappings. This parameter is only supported if the network mode of a task definition is
* bridge
. The name:internalName
construct is analogous to name:alias
* in Docker links. Up to 255 letters (uppercase and lowercase), numbers, underscores, and hyphens are
* allowed. For more information about linking Docker containers, go to Legacy container links in the Docker documentation.
* This parameter maps to Links
in the Create a container section
* of the Docker Remote API and the
* --link
option to docker run.
*
* This parameter is not supported for Windows containers.
*
*
*
* Containers that are collocated on a single container instance may be able to communicate with each other
* without requiring links or host port mappings. Network isolation is achieved on the container instance
* using security groups and VPC settings.
*
*/
public java.util.List getLinks() {
if (links == null) {
links = new com.amazonaws.internal.SdkInternalList();
}
return links;
}
/**
*
* The links
parameter allows containers to communicate with each other without the need for port
* mappings. This parameter is only supported if the network mode of a task definition is bridge
. The
* name:internalName
construct is analogous to name:alias
in Docker links. Up to 255
* letters (uppercase and lowercase), numbers, underscores, and hyphens are allowed. For more information about
* linking Docker containers, go to Legacy container links in
* the Docker documentation. This parameter maps to Links
in the Create a container section of the
* Docker Remote API and the --link
option to
* docker run.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* Containers that are collocated on a single container instance may be able to communicate with each other without
* requiring links or host port mappings. Network isolation is achieved on the container instance using security
* groups and VPC settings.
*
*
*
* @param links
* The links
parameter allows containers to communicate with each other without the need for
* port mappings. This parameter is only supported if the network mode of a task definition is
* bridge
. The name:internalName
construct is analogous to name:alias
* in Docker links. Up to 255 letters (uppercase and lowercase), numbers, underscores, and hyphens are
* allowed. For more information about linking Docker containers, go to Legacy container links in the Docker documentation. This
* parameter maps to Links
in the Create a container section
* of the Docker Remote API and the
* --link
option to docker run.
*
* This parameter is not supported for Windows containers.
*
*
*
* Containers that are collocated on a single container instance may be able to communicate with each other
* without requiring links or host port mappings. Network isolation is achieved on the container instance
* using security groups and VPC settings.
*
*/
public void setLinks(java.util.Collection links) {
if (links == null) {
this.links = null;
return;
}
this.links = new com.amazonaws.internal.SdkInternalList(links);
}
/**
*
* The links
parameter allows containers to communicate with each other without the need for port
* mappings. This parameter is only supported if the network mode of a task definition is bridge
. The
* name:internalName
construct is analogous to name:alias
in Docker links. Up to 255
* letters (uppercase and lowercase), numbers, underscores, and hyphens are allowed. For more information about
* linking Docker containers, go to Legacy container links in
* the Docker documentation. This parameter maps to Links
in the Create a container section of the
* Docker Remote API and the --link
option to
* docker run.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* Containers that are collocated on a single container instance may be able to communicate with each other without
* requiring links or host port mappings. Network isolation is achieved on the container instance using security
* groups and VPC settings.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setLinks(java.util.Collection)} or {@link #withLinks(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param links
* The links
parameter allows containers to communicate with each other without the need for
* port mappings. This parameter is only supported if the network mode of a task definition is
* bridge
. The name:internalName
construct is analogous to name:alias
* in Docker links. Up to 255 letters (uppercase and lowercase), numbers, underscores, and hyphens are
* allowed. For more information about linking Docker containers, go to Legacy container links in the Docker documentation. This
* parameter maps to Links
in the Create a container section
* of the Docker Remote API and the
* --link
option to docker run.
*
* This parameter is not supported for Windows containers.
*
*
*
* Containers that are collocated on a single container instance may be able to communicate with each other
* without requiring links or host port mappings. Network isolation is achieved on the container instance
* using security groups and VPC settings.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withLinks(String... links) {
if (this.links == null) {
setLinks(new com.amazonaws.internal.SdkInternalList(links.length));
}
for (String ele : links) {
this.links.add(ele);
}
return this;
}
/**
*
* The links
parameter allows containers to communicate with each other without the need for port
* mappings. This parameter is only supported if the network mode of a task definition is bridge
. The
* name:internalName
construct is analogous to name:alias
in Docker links. Up to 255
* letters (uppercase and lowercase), numbers, underscores, and hyphens are allowed. For more information about
* linking Docker containers, go to Legacy container links in
* the Docker documentation. This parameter maps to Links
in the Create a container section of the
* Docker Remote API and the --link
option to
* docker run.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* Containers that are collocated on a single container instance may be able to communicate with each other without
* requiring links or host port mappings. Network isolation is achieved on the container instance using security
* groups and VPC settings.
*
*
*
* @param links
* The links
parameter allows containers to communicate with each other without the need for
* port mappings. This parameter is only supported if the network mode of a task definition is
* bridge
. The name:internalName
construct is analogous to name:alias
* in Docker links. Up to 255 letters (uppercase and lowercase), numbers, underscores, and hyphens are
* allowed. For more information about linking Docker containers, go to Legacy container links in the Docker documentation. This
* parameter maps to Links
in the Create a container section
* of the Docker Remote API and the
* --link
option to docker run.
*
* This parameter is not supported for Windows containers.
*
*
*
* Containers that are collocated on a single container instance may be able to communicate with each other
* without requiring links or host port mappings. Network isolation is achieved on the container instance
* using security groups and VPC settings.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withLinks(java.util.Collection links) {
setLinks(links);
return this;
}
/**
*
* The list of port mappings for the container. Port mappings allow containers to access ports on the host container
* instance to send or receive traffic.
*
*
* For task definitions that use the awsvpc
network mode, only specify the containerPort
.
* The hostPort
can be left blank or it must be the same value as the containerPort
.
*
*
* Port mappings on Windows use the NetNAT
gateway address rather than localhost
. There's
* no loopback for port mappings on Windows, so you can't access a container's mapped port from the host itself.
*
*
* This parameter maps to PortBindings
in the Create a container section of the
* Docker Remote API and the --publish
option
* to docker run. If the network
* mode of a task definition is set to none
, then you can't specify port mappings. If the network mode
* of a task definition is set to host
, then host ports must either be undefined or they must match the
* container port in the port mapping.
*
*
*
* After a task reaches the RUNNING
status, manual and automatic host and container port assignments
* are visible in the Network Bindings section of a container description for a selected task in the Amazon
* ECS console. The assignments are also visible in the networkBindings
section DescribeTasks
* responses.
*
*
*
* @return The list of port mappings for the container. Port mappings allow containers to access ports on the host
* container instance to send or receive traffic.
*
* For task definitions that use the awsvpc
network mode, only specify the
* containerPort
. The hostPort
can be left blank or it must be the same value as
* the containerPort
.
*
*
* Port mappings on Windows use the NetNAT
gateway address rather than localhost
.
* There's no loopback for port mappings on Windows, so you can't access a container's mapped port from the
* host itself.
*
*
* This parameter maps to PortBindings
in the Create a container section
* of the Docker Remote API and the
* --publish
option to docker run. If the
* network mode of a task definition is set to none
, then you can't specify port mappings. If
* the network mode of a task definition is set to host
, then host ports must either be
* undefined or they must match the container port in the port mapping.
*
*
*
* After a task reaches the RUNNING
status, manual and automatic host and container port
* assignments are visible in the Network Bindings section of a container description for a selected
* task in the Amazon ECS console. The assignments are also visible in the networkBindings
* section DescribeTasks responses.
*
*/
public java.util.List getPortMappings() {
if (portMappings == null) {
portMappings = new com.amazonaws.internal.SdkInternalList();
}
return portMappings;
}
/**
*
* The list of port mappings for the container. Port mappings allow containers to access ports on the host container
* instance to send or receive traffic.
*
*
* For task definitions that use the awsvpc
network mode, only specify the containerPort
.
* The hostPort
can be left blank or it must be the same value as the containerPort
.
*
*
* Port mappings on Windows use the NetNAT
gateway address rather than localhost
. There's
* no loopback for port mappings on Windows, so you can't access a container's mapped port from the host itself.
*
*
* This parameter maps to PortBindings
in the Create a container section of the
* Docker Remote API and the --publish
option
* to docker run. If the network
* mode of a task definition is set to none
, then you can't specify port mappings. If the network mode
* of a task definition is set to host
, then host ports must either be undefined or they must match the
* container port in the port mapping.
*
*
*
* After a task reaches the RUNNING
status, manual and automatic host and container port assignments
* are visible in the Network Bindings section of a container description for a selected task in the Amazon
* ECS console. The assignments are also visible in the networkBindings
section DescribeTasks
* responses.
*
*
*
* @param portMappings
* The list of port mappings for the container. Port mappings allow containers to access ports on the host
* container instance to send or receive traffic.
*
* For task definitions that use the awsvpc
network mode, only specify the
* containerPort
. The hostPort
can be left blank or it must be the same value as
* the containerPort
.
*
*
* Port mappings on Windows use the NetNAT
gateway address rather than localhost
.
* There's no loopback for port mappings on Windows, so you can't access a container's mapped port from the
* host itself.
*
*
* This parameter maps to PortBindings
in the Create a container section
* of the Docker Remote API and the
* --publish
option to docker run. If the network
* mode of a task definition is set to none
, then you can't specify port mappings. If the
* network mode of a task definition is set to host
, then host ports must either be undefined or
* they must match the container port in the port mapping.
*
*
*
* After a task reaches the RUNNING
status, manual and automatic host and container port
* assignments are visible in the Network Bindings section of a container description for a selected
* task in the Amazon ECS console. The assignments are also visible in the networkBindings
* section DescribeTasks responses.
*
*/
public void setPortMappings(java.util.Collection portMappings) {
if (portMappings == null) {
this.portMappings = null;
return;
}
this.portMappings = new com.amazonaws.internal.SdkInternalList(portMappings);
}
/**
*
* The list of port mappings for the container. Port mappings allow containers to access ports on the host container
* instance to send or receive traffic.
*
*
* For task definitions that use the awsvpc
network mode, only specify the containerPort
.
* The hostPort
can be left blank or it must be the same value as the containerPort
.
*
*
* Port mappings on Windows use the NetNAT
gateway address rather than localhost
. There's
* no loopback for port mappings on Windows, so you can't access a container's mapped port from the host itself.
*
*
* This parameter maps to PortBindings
in the Create a container section of the
* Docker Remote API and the --publish
option
* to docker run. If the network
* mode of a task definition is set to none
, then you can't specify port mappings. If the network mode
* of a task definition is set to host
, then host ports must either be undefined or they must match the
* container port in the port mapping.
*
*
*
* After a task reaches the RUNNING
status, manual and automatic host and container port assignments
* are visible in the Network Bindings section of a container description for a selected task in the Amazon
* ECS console. The assignments are also visible in the networkBindings
section DescribeTasks
* responses.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPortMappings(java.util.Collection)} or {@link #withPortMappings(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param portMappings
* The list of port mappings for the container. Port mappings allow containers to access ports on the host
* container instance to send or receive traffic.
*
* For task definitions that use the awsvpc
network mode, only specify the
* containerPort
. The hostPort
can be left blank or it must be the same value as
* the containerPort
.
*
*
* Port mappings on Windows use the NetNAT
gateway address rather than localhost
.
* There's no loopback for port mappings on Windows, so you can't access a container's mapped port from the
* host itself.
*
*
* This parameter maps to PortBindings
in the Create a container section
* of the Docker Remote API and the
* --publish
option to docker run. If the network
* mode of a task definition is set to none
, then you can't specify port mappings. If the
* network mode of a task definition is set to host
, then host ports must either be undefined or
* they must match the container port in the port mapping.
*
*
*
* After a task reaches the RUNNING
status, manual and automatic host and container port
* assignments are visible in the Network Bindings section of a container description for a selected
* task in the Amazon ECS console. The assignments are also visible in the networkBindings
* section DescribeTasks responses.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withPortMappings(PortMapping... portMappings) {
if (this.portMappings == null) {
setPortMappings(new com.amazonaws.internal.SdkInternalList(portMappings.length));
}
for (PortMapping ele : portMappings) {
this.portMappings.add(ele);
}
return this;
}
/**
*
* The list of port mappings for the container. Port mappings allow containers to access ports on the host container
* instance to send or receive traffic.
*
*
* For task definitions that use the awsvpc
network mode, only specify the containerPort
.
* The hostPort
can be left blank or it must be the same value as the containerPort
.
*
*
* Port mappings on Windows use the NetNAT
gateway address rather than localhost
. There's
* no loopback for port mappings on Windows, so you can't access a container's mapped port from the host itself.
*
*
* This parameter maps to PortBindings
in the Create a container section of the
* Docker Remote API and the --publish
option
* to docker run. If the network
* mode of a task definition is set to none
, then you can't specify port mappings. If the network mode
* of a task definition is set to host
, then host ports must either be undefined or they must match the
* container port in the port mapping.
*
*
*
* After a task reaches the RUNNING
status, manual and automatic host and container port assignments
* are visible in the Network Bindings section of a container description for a selected task in the Amazon
* ECS console. The assignments are also visible in the networkBindings
section DescribeTasks
* responses.
*
*
*
* @param portMappings
* The list of port mappings for the container. Port mappings allow containers to access ports on the host
* container instance to send or receive traffic.
*
* For task definitions that use the awsvpc
network mode, only specify the
* containerPort
. The hostPort
can be left blank or it must be the same value as
* the containerPort
.
*
*
* Port mappings on Windows use the NetNAT
gateway address rather than localhost
.
* There's no loopback for port mappings on Windows, so you can't access a container's mapped port from the
* host itself.
*
*
* This parameter maps to PortBindings
in the Create a container section
* of the Docker Remote API and the
* --publish
option to docker run. If the network
* mode of a task definition is set to none
, then you can't specify port mappings. If the
* network mode of a task definition is set to host
, then host ports must either be undefined or
* they must match the container port in the port mapping.
*
*
*
* After a task reaches the RUNNING
status, manual and automatic host and container port
* assignments are visible in the Network Bindings section of a container description for a selected
* task in the Amazon ECS console. The assignments are also visible in the networkBindings
* section DescribeTasks responses.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withPortMappings(java.util.Collection portMappings) {
setPortMappings(portMappings);
return this;
}
/**
*
* If the essential
parameter of a container is marked as true
, and that container fails
* or stops for any reason, all other containers that are part of the task are stopped. If the
* essential
parameter of a container is marked as false
, its failure doesn't affect the
* rest of the containers in a task. If this parameter is omitted, a container is assumed to be essential.
*
*
* All tasks must have at least one essential container. If you have an application that's composed of multiple
* containers, group containers that are used for a common purpose into components, and separate the different
* components into multiple task definitions. For more information, see Application
* Architecture in the Amazon Elastic Container Service Developer Guide.
*
*
* @param essential
* If the essential
parameter of a container is marked as true
, and that container
* fails or stops for any reason, all other containers that are part of the task are stopped. If the
* essential
parameter of a container is marked as false
, its failure doesn't
* affect the rest of the containers in a task. If this parameter is omitted, a container is assumed to be
* essential.
*
* All tasks must have at least one essential container. If you have an application that's composed of
* multiple containers, group containers that are used for a common purpose into components, and separate the
* different components into multiple task definitions. For more information, see Application Architecture in the Amazon Elastic Container Service Developer Guide.
*/
public void setEssential(Boolean essential) {
this.essential = essential;
}
/**
*
* If the essential
parameter of a container is marked as true
, and that container fails
* or stops for any reason, all other containers that are part of the task are stopped. If the
* essential
parameter of a container is marked as false
, its failure doesn't affect the
* rest of the containers in a task. If this parameter is omitted, a container is assumed to be essential.
*
*
* All tasks must have at least one essential container. If you have an application that's composed of multiple
* containers, group containers that are used for a common purpose into components, and separate the different
* components into multiple task definitions. For more information, see Application
* Architecture in the Amazon Elastic Container Service Developer Guide.
*
*
* @return If the essential
parameter of a container is marked as true
, and that container
* fails or stops for any reason, all other containers that are part of the task are stopped. If the
* essential
parameter of a container is marked as false
, its failure doesn't
* affect the rest of the containers in a task. If this parameter is omitted, a container is assumed to be
* essential.
*
* All tasks must have at least one essential container. If you have an application that's composed of
* multiple containers, group containers that are used for a common purpose into components, and separate
* the different components into multiple task definitions. For more information, see Application Architecture in the Amazon Elastic Container Service Developer Guide.
*/
public Boolean getEssential() {
return this.essential;
}
/**
*
* If the essential
parameter of a container is marked as true
, and that container fails
* or stops for any reason, all other containers that are part of the task are stopped. If the
* essential
parameter of a container is marked as false
, its failure doesn't affect the
* rest of the containers in a task. If this parameter is omitted, a container is assumed to be essential.
*
*
* All tasks must have at least one essential container. If you have an application that's composed of multiple
* containers, group containers that are used for a common purpose into components, and separate the different
* components into multiple task definitions. For more information, see Application
* Architecture in the Amazon Elastic Container Service Developer Guide.
*
*
* @param essential
* If the essential
parameter of a container is marked as true
, and that container
* fails or stops for any reason, all other containers that are part of the task are stopped. If the
* essential
parameter of a container is marked as false
, its failure doesn't
* affect the rest of the containers in a task. If this parameter is omitted, a container is assumed to be
* essential.
*
* All tasks must have at least one essential container. If you have an application that's composed of
* multiple containers, group containers that are used for a common purpose into components, and separate the
* different components into multiple task definitions. For more information, see Application Architecture in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withEssential(Boolean essential) {
setEssential(essential);
return this;
}
/**
*
* If the essential
parameter of a container is marked as true
, and that container fails
* or stops for any reason, all other containers that are part of the task are stopped. If the
* essential
parameter of a container is marked as false
, its failure doesn't affect the
* rest of the containers in a task. If this parameter is omitted, a container is assumed to be essential.
*
*
* All tasks must have at least one essential container. If you have an application that's composed of multiple
* containers, group containers that are used for a common purpose into components, and separate the different
* components into multiple task definitions. For more information, see Application
* Architecture in the Amazon Elastic Container Service Developer Guide.
*
*
* @return If the essential
parameter of a container is marked as true
, and that container
* fails or stops for any reason, all other containers that are part of the task are stopped. If the
* essential
parameter of a container is marked as false
, its failure doesn't
* affect the rest of the containers in a task. If this parameter is omitted, a container is assumed to be
* essential.
*
* All tasks must have at least one essential container. If you have an application that's composed of
* multiple containers, group containers that are used for a common purpose into components, and separate
* the different components into multiple task definitions. For more information, see Application Architecture in the Amazon Elastic Container Service Developer Guide.
*/
public Boolean isEssential() {
return this.essential;
}
/**
*
*
* Early versions of the Amazon ECS container agent don't properly handle entryPoint
parameters. If you
* have problems using entryPoint
, update your container agent or enter your commands and arguments as
* command
array items instead.
*
*
*
* The entry point that's passed to the container. This parameter maps to Entrypoint
in the Create a container section of the
* Docker Remote API and the --entrypoint
* option to docker run. For more
* information, see https://docs.docker.com/engine
* /reference/builder/#entrypoint.
*
*
* @return
* Early versions of the Amazon ECS container agent don't properly handle entryPoint
* parameters. If you have problems using entryPoint
, update your container agent or enter your
* commands and arguments as command
array items instead.
*
*
*
* The entry point that's passed to the container. This parameter maps to Entrypoint
in the Create a container section
* of the Docker Remote API and the
* --entrypoint
option to docker run. For more
* information, see https://docs.docker
* .com/engine/reference/builder/#entrypoint.
*/
public java.util.List getEntryPoint() {
if (entryPoint == null) {
entryPoint = new com.amazonaws.internal.SdkInternalList();
}
return entryPoint;
}
/**
*
*
* Early versions of the Amazon ECS container agent don't properly handle entryPoint
parameters. If you
* have problems using entryPoint
, update your container agent or enter your commands and arguments as
* command
array items instead.
*
*
*
* The entry point that's passed to the container. This parameter maps to Entrypoint
in the Create a container section of the
* Docker Remote API and the --entrypoint
* option to docker run. For more
* information, see https://docs.docker.com/engine
* /reference/builder/#entrypoint.
*
*
* @param entryPoint
*
* Early versions of the Amazon ECS container agent don't properly handle entryPoint
parameters.
* If you have problems using entryPoint
, update your container agent or enter your commands and
* arguments as command
array items instead.
*
*
*
* The entry point that's passed to the container. This parameter maps to Entrypoint
in the Create a container section
* of the Docker Remote API and the
* --entrypoint
option to docker run. For more
* information, see https://docs.docker
* .com/engine/reference/builder/#entrypoint.
*/
public void setEntryPoint(java.util.Collection entryPoint) {
if (entryPoint == null) {
this.entryPoint = null;
return;
}
this.entryPoint = new com.amazonaws.internal.SdkInternalList(entryPoint);
}
/**
*
*
* Early versions of the Amazon ECS container agent don't properly handle entryPoint
parameters. If you
* have problems using entryPoint
, update your container agent or enter your commands and arguments as
* command
array items instead.
*
*
*
* The entry point that's passed to the container. This parameter maps to Entrypoint
in the Create a container section of the
* Docker Remote API and the --entrypoint
* option to docker run. For more
* information, see https://docs.docker.com/engine
* /reference/builder/#entrypoint.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setEntryPoint(java.util.Collection)} or {@link #withEntryPoint(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param entryPoint
*
* Early versions of the Amazon ECS container agent don't properly handle entryPoint
parameters.
* If you have problems using entryPoint
, update your container agent or enter your commands and
* arguments as command
array items instead.
*
*
*
* The entry point that's passed to the container. This parameter maps to Entrypoint
in the Create a container section
* of the Docker Remote API and the
* --entrypoint
option to docker run. For more
* information, see https://docs.docker
* .com/engine/reference/builder/#entrypoint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withEntryPoint(String... entryPoint) {
if (this.entryPoint == null) {
setEntryPoint(new com.amazonaws.internal.SdkInternalList(entryPoint.length));
}
for (String ele : entryPoint) {
this.entryPoint.add(ele);
}
return this;
}
/**
*
*
* Early versions of the Amazon ECS container agent don't properly handle entryPoint
parameters. If you
* have problems using entryPoint
, update your container agent or enter your commands and arguments as
* command
array items instead.
*
*
*
* The entry point that's passed to the container. This parameter maps to Entrypoint
in the Create a container section of the
* Docker Remote API and the --entrypoint
* option to docker run. For more
* information, see https://docs.docker.com/engine
* /reference/builder/#entrypoint.
*
*
* @param entryPoint
*
* Early versions of the Amazon ECS container agent don't properly handle entryPoint
parameters.
* If you have problems using entryPoint
, update your container agent or enter your commands and
* arguments as command
array items instead.
*
*
*
* The entry point that's passed to the container. This parameter maps to Entrypoint
in the Create a container section
* of the Docker Remote API and the
* --entrypoint
option to docker run. For more
* information, see https://docs.docker
* .com/engine/reference/builder/#entrypoint.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withEntryPoint(java.util.Collection entryPoint) {
setEntryPoint(entryPoint);
return this;
}
/**
*
* The command that's passed to the container. This parameter maps to Cmd
in the Create a container section of the
* Docker Remote API and the COMMAND
parameter
* to docker run. For more
* information, see https://docs.docker.com/engine/reference
* /builder/#cmd. If there are multiple arguments, each argument is a separated string in the array.
*
*
* @return The command that's passed to the container. This parameter maps to Cmd
in the Create a container section
* of the Docker Remote API and the
* COMMAND
parameter to docker run. For more
* information, see https://docs.docker.com/engine
* /reference/builder/#cmd. If there are multiple arguments, each argument is a separated string in the
* array.
*/
public java.util.List getCommand() {
if (command == null) {
command = new com.amazonaws.internal.SdkInternalList();
}
return command;
}
/**
*
* The command that's passed to the container. This parameter maps to Cmd
in the Create a container section of the
* Docker Remote API and the COMMAND
parameter
* to docker run. For more
* information, see https://docs.docker.com/engine/reference
* /builder/#cmd. If there are multiple arguments, each argument is a separated string in the array.
*
*
* @param command
* The command that's passed to the container. This parameter maps to Cmd
in the Create a container section
* of the Docker Remote API and the
* COMMAND
parameter to docker run. For more
* information, see https://docs.docker.com/engine
* /reference/builder/#cmd. If there are multiple arguments, each argument is a separated string in the
* array.
*/
public void setCommand(java.util.Collection command) {
if (command == null) {
this.command = null;
return;
}
this.command = new com.amazonaws.internal.SdkInternalList(command);
}
/**
*
* The command that's passed to the container. This parameter maps to Cmd
in the Create a container section of the
* Docker Remote API and the COMMAND
parameter
* to docker run. For more
* information, see https://docs.docker.com/engine/reference
* /builder/#cmd. If there are multiple arguments, each argument is a separated string in the array.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCommand(java.util.Collection)} or {@link #withCommand(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param command
* The command that's passed to the container. This parameter maps to Cmd
in the Create a container section
* of the Docker Remote API and the
* COMMAND
parameter to docker run. For more
* information, see https://docs.docker.com/engine
* /reference/builder/#cmd. If there are multiple arguments, each argument is a separated string in the
* array.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withCommand(String... command) {
if (this.command == null) {
setCommand(new com.amazonaws.internal.SdkInternalList(command.length));
}
for (String ele : command) {
this.command.add(ele);
}
return this;
}
/**
*
* The command that's passed to the container. This parameter maps to Cmd
in the Create a container section of the
* Docker Remote API and the COMMAND
parameter
* to docker run. For more
* information, see https://docs.docker.com/engine/reference
* /builder/#cmd. If there are multiple arguments, each argument is a separated string in the array.
*
*
* @param command
* The command that's passed to the container. This parameter maps to Cmd
in the Create a container section
* of the Docker Remote API and the
* COMMAND
parameter to docker run. For more
* information, see https://docs.docker.com/engine
* /reference/builder/#cmd. If there are multiple arguments, each argument is a separated string in the
* array.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withCommand(java.util.Collection command) {
setCommand(command);
return this;
}
/**
*
* The environment variables to pass to a container. This parameter maps to Env
in the Create a container section of the
* Docker Remote API and the --env
option to docker run.
*
*
*
* We don't recommend that you use plaintext environment variables for sensitive information, such as credential
* data.
*
*
*
* @return The environment variables to pass to a container. This parameter maps to Env
in the Create a container section
* of the Docker Remote API and the
* --env
option to docker run.
*
*
* We don't recommend that you use plaintext environment variables for sensitive information, such as
* credential data.
*
*/
public java.util.List getEnvironment() {
if (environment == null) {
environment = new com.amazonaws.internal.SdkInternalList();
}
return environment;
}
/**
*
* The environment variables to pass to a container. This parameter maps to Env
in the Create a container section of the
* Docker Remote API and the --env
option to docker run.
*
*
*
* We don't recommend that you use plaintext environment variables for sensitive information, such as credential
* data.
*
*
*
* @param environment
* The environment variables to pass to a container. This parameter maps to Env
in the Create a container section
* of the Docker Remote API and the
* --env
option to docker run.
*
*
* We don't recommend that you use plaintext environment variables for sensitive information, such as
* credential data.
*
*/
public void setEnvironment(java.util.Collection environment) {
if (environment == null) {
this.environment = null;
return;
}
this.environment = new com.amazonaws.internal.SdkInternalList(environment);
}
/**
*
* The environment variables to pass to a container. This parameter maps to Env
in the Create a container section of the
* Docker Remote API and the --env
option to docker run.
*
*
*
* We don't recommend that you use plaintext environment variables for sensitive information, such as credential
* data.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setEnvironment(java.util.Collection)} or {@link #withEnvironment(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param environment
* The environment variables to pass to a container. This parameter maps to Env
in the Create a container section
* of the Docker Remote API and the
* --env
option to docker run.
*
*
* We don't recommend that you use plaintext environment variables for sensitive information, such as
* credential data.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withEnvironment(KeyValuePair... environment) {
if (this.environment == null) {
setEnvironment(new com.amazonaws.internal.SdkInternalList(environment.length));
}
for (KeyValuePair ele : environment) {
this.environment.add(ele);
}
return this;
}
/**
*
* The environment variables to pass to a container. This parameter maps to Env
in the Create a container section of the
* Docker Remote API and the --env
option to docker run.
*
*
*
* We don't recommend that you use plaintext environment variables for sensitive information, such as credential
* data.
*
*
*
* @param environment
* The environment variables to pass to a container. This parameter maps to Env
in the Create a container section
* of the Docker Remote API and the
* --env
option to docker run.
*
*
* We don't recommend that you use plaintext environment variables for sensitive information, such as
* credential data.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withEnvironment(java.util.Collection environment) {
setEnvironment(environment);
return this;
}
/**
*
* A list of files containing the environment variables to pass to a container. This parameter maps to the
* --env-file
option to docker run.
*
*
* You can specify up to ten environment files. The file must have a .env
file extension. Each line in
* an environment file contains an environment variable in VARIABLE=VALUE
format. Lines beginning with
* #
are treated as comments and are ignored. For more information about the environment variable file
* syntax, see Declare default environment variables in
* file.
*
*
* If there are environment variables specified using the environment
parameter in a container
* definition, they take precedence over the variables contained within an environment file. If multiple environment
* files are specified that contain the same variable, they're processed from the top down. We recommend that you
* use unique variable names. For more information, see Specifying Environment
* Variables in the Amazon Elastic Container Service Developer Guide.
*
*
* @return A list of files containing the environment variables to pass to a container. This parameter maps to the
* --env-file
option to docker run.
*
* You can specify up to ten environment files. The file must have a .env
file extension. Each
* line in an environment file contains an environment variable in VARIABLE=VALUE
format. Lines
* beginning with #
are treated as comments and are ignored. For more information about the
* environment variable file syntax, see Declare default
* environment variables in file.
*
*
* If there are environment variables specified using the environment
parameter in a container
* definition, they take precedence over the variables contained within an environment file. If multiple
* environment files are specified that contain the same variable, they're processed from the top down. We
* recommend that you use unique variable names. For more information, see Specifying
* Environment Variables in the Amazon Elastic Container Service Developer Guide.
*/
public java.util.List getEnvironmentFiles() {
if (environmentFiles == null) {
environmentFiles = new com.amazonaws.internal.SdkInternalList();
}
return environmentFiles;
}
/**
*
* A list of files containing the environment variables to pass to a container. This parameter maps to the
* --env-file
option to docker run.
*
*
* You can specify up to ten environment files. The file must have a .env
file extension. Each line in
* an environment file contains an environment variable in VARIABLE=VALUE
format. Lines beginning with
* #
are treated as comments and are ignored. For more information about the environment variable file
* syntax, see Declare default environment variables in
* file.
*
*
* If there are environment variables specified using the environment
parameter in a container
* definition, they take precedence over the variables contained within an environment file. If multiple environment
* files are specified that contain the same variable, they're processed from the top down. We recommend that you
* use unique variable names. For more information, see Specifying Environment
* Variables in the Amazon Elastic Container Service Developer Guide.
*
*
* @param environmentFiles
* A list of files containing the environment variables to pass to a container. This parameter maps to the
* --env-file
option to docker run.
*
* You can specify up to ten environment files. The file must have a .env
file extension. Each
* line in an environment file contains an environment variable in VARIABLE=VALUE
format. Lines
* beginning with #
are treated as comments and are ignored. For more information about the
* environment variable file syntax, see Declare default
* environment variables in file.
*
*
* If there are environment variables specified using the environment
parameter in a container
* definition, they take precedence over the variables contained within an environment file. If multiple
* environment files are specified that contain the same variable, they're processed from the top down. We
* recommend that you use unique variable names. For more information, see Specifying
* Environment Variables in the Amazon Elastic Container Service Developer Guide.
*/
public void setEnvironmentFiles(java.util.Collection environmentFiles) {
if (environmentFiles == null) {
this.environmentFiles = null;
return;
}
this.environmentFiles = new com.amazonaws.internal.SdkInternalList(environmentFiles);
}
/**
*
* A list of files containing the environment variables to pass to a container. This parameter maps to the
* --env-file
option to docker run.
*
*
* You can specify up to ten environment files. The file must have a .env
file extension. Each line in
* an environment file contains an environment variable in VARIABLE=VALUE
format. Lines beginning with
* #
are treated as comments and are ignored. For more information about the environment variable file
* syntax, see Declare default environment variables in
* file.
*
*
* If there are environment variables specified using the environment
parameter in a container
* definition, they take precedence over the variables contained within an environment file. If multiple environment
* files are specified that contain the same variable, they're processed from the top down. We recommend that you
* use unique variable names. For more information, see Specifying Environment
* Variables in the Amazon Elastic Container Service Developer Guide.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setEnvironmentFiles(java.util.Collection)} or {@link #withEnvironmentFiles(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param environmentFiles
* A list of files containing the environment variables to pass to a container. This parameter maps to the
* --env-file
option to docker run.
*
* You can specify up to ten environment files. The file must have a .env
file extension. Each
* line in an environment file contains an environment variable in VARIABLE=VALUE
format. Lines
* beginning with #
are treated as comments and are ignored. For more information about the
* environment variable file syntax, see Declare default
* environment variables in file.
*
*
* If there are environment variables specified using the environment
parameter in a container
* definition, they take precedence over the variables contained within an environment file. If multiple
* environment files are specified that contain the same variable, they're processed from the top down. We
* recommend that you use unique variable names. For more information, see Specifying
* Environment Variables in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withEnvironmentFiles(EnvironmentFile... environmentFiles) {
if (this.environmentFiles == null) {
setEnvironmentFiles(new com.amazonaws.internal.SdkInternalList(environmentFiles.length));
}
for (EnvironmentFile ele : environmentFiles) {
this.environmentFiles.add(ele);
}
return this;
}
/**
*
* A list of files containing the environment variables to pass to a container. This parameter maps to the
* --env-file
option to docker run.
*
*
* You can specify up to ten environment files. The file must have a .env
file extension. Each line in
* an environment file contains an environment variable in VARIABLE=VALUE
format. Lines beginning with
* #
are treated as comments and are ignored. For more information about the environment variable file
* syntax, see Declare default environment variables in
* file.
*
*
* If there are environment variables specified using the environment
parameter in a container
* definition, they take precedence over the variables contained within an environment file. If multiple environment
* files are specified that contain the same variable, they're processed from the top down. We recommend that you
* use unique variable names. For more information, see Specifying Environment
* Variables in the Amazon Elastic Container Service Developer Guide.
*
*
* @param environmentFiles
* A list of files containing the environment variables to pass to a container. This parameter maps to the
* --env-file
option to docker run.
*
* You can specify up to ten environment files. The file must have a .env
file extension. Each
* line in an environment file contains an environment variable in VARIABLE=VALUE
format. Lines
* beginning with #
are treated as comments and are ignored. For more information about the
* environment variable file syntax, see Declare default
* environment variables in file.
*
*
* If there are environment variables specified using the environment
parameter in a container
* definition, they take precedence over the variables contained within an environment file. If multiple
* environment files are specified that contain the same variable, they're processed from the top down. We
* recommend that you use unique variable names. For more information, see Specifying
* Environment Variables in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withEnvironmentFiles(java.util.Collection environmentFiles) {
setEnvironmentFiles(environmentFiles);
return this;
}
/**
*
* The mount points for data volumes in your container.
*
*
* This parameter maps to Volumes
in the Create a container section of the
* Docker Remote API and the --volume
option to
* docker run.
*
*
* Windows containers can mount whole directories on the same drive as $env:ProgramData
. Windows
* containers can't mount directories on a different drive, and mount point can't be across drives.
*
*
* @return The mount points for data volumes in your container.
*
* This parameter maps to Volumes
in the Create a container section
* of the Docker Remote API and the
* --volume
option to docker run.
*
*
* Windows containers can mount whole directories on the same drive as $env:ProgramData
.
* Windows containers can't mount directories on a different drive, and mount point can't be across drives.
*/
public java.util.List getMountPoints() {
if (mountPoints == null) {
mountPoints = new com.amazonaws.internal.SdkInternalList();
}
return mountPoints;
}
/**
*
* The mount points for data volumes in your container.
*
*
* This parameter maps to Volumes
in the Create a container section of the
* Docker Remote API and the --volume
option to
* docker run.
*
*
* Windows containers can mount whole directories on the same drive as $env:ProgramData
. Windows
* containers can't mount directories on a different drive, and mount point can't be across drives.
*
*
* @param mountPoints
* The mount points for data volumes in your container.
*
* This parameter maps to Volumes
in the Create a container section
* of the Docker Remote API and the
* --volume
option to docker run.
*
*
* Windows containers can mount whole directories on the same drive as $env:ProgramData
. Windows
* containers can't mount directories on a different drive, and mount point can't be across drives.
*/
public void setMountPoints(java.util.Collection mountPoints) {
if (mountPoints == null) {
this.mountPoints = null;
return;
}
this.mountPoints = new com.amazonaws.internal.SdkInternalList(mountPoints);
}
/**
*
* The mount points for data volumes in your container.
*
*
* This parameter maps to Volumes
in the Create a container section of the
* Docker Remote API and the --volume
option to
* docker run.
*
*
* Windows containers can mount whole directories on the same drive as $env:ProgramData
. Windows
* containers can't mount directories on a different drive, and mount point can't be across drives.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setMountPoints(java.util.Collection)} or {@link #withMountPoints(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param mountPoints
* The mount points for data volumes in your container.
*
* This parameter maps to Volumes
in the Create a container section
* of the Docker Remote API and the
* --volume
option to docker run.
*
*
* Windows containers can mount whole directories on the same drive as $env:ProgramData
. Windows
* containers can't mount directories on a different drive, and mount point can't be across drives.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withMountPoints(MountPoint... mountPoints) {
if (this.mountPoints == null) {
setMountPoints(new com.amazonaws.internal.SdkInternalList(mountPoints.length));
}
for (MountPoint ele : mountPoints) {
this.mountPoints.add(ele);
}
return this;
}
/**
*
* The mount points for data volumes in your container.
*
*
* This parameter maps to Volumes
in the Create a container section of the
* Docker Remote API and the --volume
option to
* docker run.
*
*
* Windows containers can mount whole directories on the same drive as $env:ProgramData
. Windows
* containers can't mount directories on a different drive, and mount point can't be across drives.
*
*
* @param mountPoints
* The mount points for data volumes in your container.
*
* This parameter maps to Volumes
in the Create a container section
* of the Docker Remote API and the
* --volume
option to docker run.
*
*
* Windows containers can mount whole directories on the same drive as $env:ProgramData
. Windows
* containers can't mount directories on a different drive, and mount point can't be across drives.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withMountPoints(java.util.Collection mountPoints) {
setMountPoints(mountPoints);
return this;
}
/**
*
* Data volumes to mount from another container. This parameter maps to VolumesFrom
in the Create a container section of the
* Docker Remote API and the --volumes-from
* option to docker run.
*
*
* @return Data volumes to mount from another container. This parameter maps to VolumesFrom
in the Create a container section
* of the Docker Remote API and the
* --volumes-from
option to docker run.
*/
public java.util.List getVolumesFrom() {
if (volumesFrom == null) {
volumesFrom = new com.amazonaws.internal.SdkInternalList();
}
return volumesFrom;
}
/**
*
* Data volumes to mount from another container. This parameter maps to VolumesFrom
in the Create a container section of the
* Docker Remote API and the --volumes-from
* option to docker run.
*
*
* @param volumesFrom
* Data volumes to mount from another container. This parameter maps to VolumesFrom
in the Create a container section
* of the Docker Remote API and the
* --volumes-from
option to docker run.
*/
public void setVolumesFrom(java.util.Collection volumesFrom) {
if (volumesFrom == null) {
this.volumesFrom = null;
return;
}
this.volumesFrom = new com.amazonaws.internal.SdkInternalList(volumesFrom);
}
/**
*
* Data volumes to mount from another container. This parameter maps to VolumesFrom
in the Create a container section of the
* Docker Remote API and the --volumes-from
* option to docker run.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setVolumesFrom(java.util.Collection)} or {@link #withVolumesFrom(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param volumesFrom
* Data volumes to mount from another container. This parameter maps to VolumesFrom
in the Create a container section
* of the Docker Remote API and the
* --volumes-from
option to docker run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withVolumesFrom(VolumeFrom... volumesFrom) {
if (this.volumesFrom == null) {
setVolumesFrom(new com.amazonaws.internal.SdkInternalList(volumesFrom.length));
}
for (VolumeFrom ele : volumesFrom) {
this.volumesFrom.add(ele);
}
return this;
}
/**
*
* Data volumes to mount from another container. This parameter maps to VolumesFrom
in the Create a container section of the
* Docker Remote API and the --volumes-from
* option to docker run.
*
*
* @param volumesFrom
* Data volumes to mount from another container. This parameter maps to VolumesFrom
in the Create a container section
* of the Docker Remote API and the
* --volumes-from
option to docker run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withVolumesFrom(java.util.Collection volumesFrom) {
setVolumesFrom(volumesFrom);
return this;
}
/**
*
* Linux-specific modifications that are applied to the container, such as Linux kernel capabilities. For more
* information see KernelCapabilities.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* @param linuxParameters
* Linux-specific modifications that are applied to the container, such as Linux kernel capabilities. For
* more information see KernelCapabilities.
*
* This parameter is not supported for Windows containers.
*
*/
public void setLinuxParameters(LinuxParameters linuxParameters) {
this.linuxParameters = linuxParameters;
}
/**
*
* Linux-specific modifications that are applied to the container, such as Linux kernel capabilities. For more
* information see KernelCapabilities.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* @return Linux-specific modifications that are applied to the container, such as Linux kernel capabilities. For
* more information see KernelCapabilities.
*
* This parameter is not supported for Windows containers.
*
*/
public LinuxParameters getLinuxParameters() {
return this.linuxParameters;
}
/**
*
* Linux-specific modifications that are applied to the container, such as Linux kernel capabilities. For more
* information see KernelCapabilities.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* @param linuxParameters
* Linux-specific modifications that are applied to the container, such as Linux kernel capabilities. For
* more information see KernelCapabilities.
*
* This parameter is not supported for Windows containers.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withLinuxParameters(LinuxParameters linuxParameters) {
setLinuxParameters(linuxParameters);
return this;
}
/**
*
* The secrets to pass to the container. For more information, see Specifying
* Sensitive Data in the Amazon Elastic Container Service Developer Guide.
*
*
* @return The secrets to pass to the container. For more information, see Specifying Sensitive Data in the Amazon Elastic Container Service Developer Guide.
*/
public java.util.List getSecrets() {
if (secrets == null) {
secrets = new com.amazonaws.internal.SdkInternalList();
}
return secrets;
}
/**
*
* The secrets to pass to the container. For more information, see Specifying
* Sensitive Data in the Amazon Elastic Container Service Developer Guide.
*
*
* @param secrets
* The secrets to pass to the container. For more information, see Specifying Sensitive Data in the Amazon Elastic Container Service Developer Guide.
*/
public void setSecrets(java.util.Collection secrets) {
if (secrets == null) {
this.secrets = null;
return;
}
this.secrets = new com.amazonaws.internal.SdkInternalList(secrets);
}
/**
*
* The secrets to pass to the container. For more information, see Specifying
* Sensitive Data in the Amazon Elastic Container Service Developer Guide.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSecrets(java.util.Collection)} or {@link #withSecrets(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param secrets
* The secrets to pass to the container. For more information, see Specifying Sensitive Data in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withSecrets(Secret... secrets) {
if (this.secrets == null) {
setSecrets(new com.amazonaws.internal.SdkInternalList(secrets.length));
}
for (Secret ele : secrets) {
this.secrets.add(ele);
}
return this;
}
/**
*
* The secrets to pass to the container. For more information, see Specifying
* Sensitive Data in the Amazon Elastic Container Service Developer Guide.
*
*
* @param secrets
* The secrets to pass to the container. For more information, see Specifying Sensitive Data in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withSecrets(java.util.Collection secrets) {
setSecrets(secrets);
return this;
}
/**
*
* The dependencies defined for container startup and shutdown. A container can contain multiple dependencies on
* other containers in a task definition. When a dependency is defined for container startup, for container shutdown
* it is reversed.
*
*
* For tasks using the EC2 launch type, the container instances require at least version 1.26.0 of the container
* agent to turn on container dependencies. However, we recommend using the latest container agent version. For
* information about checking your agent version and updating to the latest version, see Updating the Amazon ECS
* Container Agent in the Amazon Elastic Container Service Developer Guide. If you're using an Amazon
* ECS-optimized Linux AMI, your instance needs at least version 1.26.0-1 of the ecs-init
package. If
* your container instances are launched from version 20190301
or later, then they contain the required
* versions of the container agent and ecs-init
. For more information, see Amazon ECS-optimized
* Linux AMI in the Amazon Elastic Container Service Developer Guide.
*
*
* For tasks using the Fargate launch type, the task or service requires the following platforms:
*
*
* -
*
* Linux platform version 1.3.0
or later.
*
*
* -
*
* Windows platform version 1.0.0
or later.
*
*
*
*
* @return The dependencies defined for container startup and shutdown. A container can contain multiple
* dependencies on other containers in a task definition. When a dependency is defined for container
* startup, for container shutdown it is reversed.
*
* For tasks using the EC2 launch type, the container instances require at least version 1.26.0 of the
* container agent to turn on container dependencies. However, we recommend using the latest container agent
* version. For information about checking your agent version and updating to the latest version, see Updating the
* Amazon ECS Container Agent in the Amazon Elastic Container Service Developer Guide. If you're
* using an Amazon ECS-optimized Linux AMI, your instance needs at least version 1.26.0-1 of the
* ecs-init
package. If your container instances are launched from version
* 20190301
or later, then they contain the required versions of the container agent and
* ecs-init
. For more information, see Amazon
* ECS-optimized Linux AMI in the Amazon Elastic Container Service Developer Guide.
*
*
* For tasks using the Fargate launch type, the task or service requires the following platforms:
*
*
* -
*
* Linux platform version 1.3.0
or later.
*
*
* -
*
* Windows platform version 1.0.0
or later.
*
*
*/
public java.util.List getDependsOn() {
if (dependsOn == null) {
dependsOn = new com.amazonaws.internal.SdkInternalList();
}
return dependsOn;
}
/**
*
* The dependencies defined for container startup and shutdown. A container can contain multiple dependencies on
* other containers in a task definition. When a dependency is defined for container startup, for container shutdown
* it is reversed.
*
*
* For tasks using the EC2 launch type, the container instances require at least version 1.26.0 of the container
* agent to turn on container dependencies. However, we recommend using the latest container agent version. For
* information about checking your agent version and updating to the latest version, see Updating the Amazon ECS
* Container Agent in the Amazon Elastic Container Service Developer Guide. If you're using an Amazon
* ECS-optimized Linux AMI, your instance needs at least version 1.26.0-1 of the ecs-init
package. If
* your container instances are launched from version 20190301
or later, then they contain the required
* versions of the container agent and ecs-init
. For more information, see Amazon ECS-optimized
* Linux AMI in the Amazon Elastic Container Service Developer Guide.
*
*
* For tasks using the Fargate launch type, the task or service requires the following platforms:
*
*
* -
*
* Linux platform version 1.3.0
or later.
*
*
* -
*
* Windows platform version 1.0.0
or later.
*
*
*
*
* @param dependsOn
* The dependencies defined for container startup and shutdown. A container can contain multiple dependencies
* on other containers in a task definition. When a dependency is defined for container startup, for
* container shutdown it is reversed.
*
* For tasks using the EC2 launch type, the container instances require at least version 1.26.0 of the
* container agent to turn on container dependencies. However, we recommend using the latest container agent
* version. For information about checking your agent version and updating to the latest version, see Updating the
* Amazon ECS Container Agent in the Amazon Elastic Container Service Developer Guide. If you're
* using an Amazon ECS-optimized Linux AMI, your instance needs at least version 1.26.0-1 of the
* ecs-init
package. If your container instances are launched from version 20190301
* or later, then they contain the required versions of the container agent and ecs-init
. For
* more information, see Amazon
* ECS-optimized Linux AMI in the Amazon Elastic Container Service Developer Guide.
*
*
* For tasks using the Fargate launch type, the task or service requires the following platforms:
*
*
* -
*
* Linux platform version 1.3.0
or later.
*
*
* -
*
* Windows platform version 1.0.0
or later.
*
*
*/
public void setDependsOn(java.util.Collection dependsOn) {
if (dependsOn == null) {
this.dependsOn = null;
return;
}
this.dependsOn = new com.amazonaws.internal.SdkInternalList(dependsOn);
}
/**
*
* The dependencies defined for container startup and shutdown. A container can contain multiple dependencies on
* other containers in a task definition. When a dependency is defined for container startup, for container shutdown
* it is reversed.
*
*
* For tasks using the EC2 launch type, the container instances require at least version 1.26.0 of the container
* agent to turn on container dependencies. However, we recommend using the latest container agent version. For
* information about checking your agent version and updating to the latest version, see Updating the Amazon ECS
* Container Agent in the Amazon Elastic Container Service Developer Guide. If you're using an Amazon
* ECS-optimized Linux AMI, your instance needs at least version 1.26.0-1 of the ecs-init
package. If
* your container instances are launched from version 20190301
or later, then they contain the required
* versions of the container agent and ecs-init
. For more information, see Amazon ECS-optimized
* Linux AMI in the Amazon Elastic Container Service Developer Guide.
*
*
* For tasks using the Fargate launch type, the task or service requires the following platforms:
*
*
* -
*
* Linux platform version 1.3.0
or later.
*
*
* -
*
* Windows platform version 1.0.0
or later.
*
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDependsOn(java.util.Collection)} or {@link #withDependsOn(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param dependsOn
* The dependencies defined for container startup and shutdown. A container can contain multiple dependencies
* on other containers in a task definition. When a dependency is defined for container startup, for
* container shutdown it is reversed.
*
* For tasks using the EC2 launch type, the container instances require at least version 1.26.0 of the
* container agent to turn on container dependencies. However, we recommend using the latest container agent
* version. For information about checking your agent version and updating to the latest version, see Updating the
* Amazon ECS Container Agent in the Amazon Elastic Container Service Developer Guide. If you're
* using an Amazon ECS-optimized Linux AMI, your instance needs at least version 1.26.0-1 of the
* ecs-init
package. If your container instances are launched from version 20190301
* or later, then they contain the required versions of the container agent and ecs-init
. For
* more information, see Amazon
* ECS-optimized Linux AMI in the Amazon Elastic Container Service Developer Guide.
*
*
* For tasks using the Fargate launch type, the task or service requires the following platforms:
*
*
* -
*
* Linux platform version 1.3.0
or later.
*
*
* -
*
* Windows platform version 1.0.0
or later.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withDependsOn(ContainerDependency... dependsOn) {
if (this.dependsOn == null) {
setDependsOn(new com.amazonaws.internal.SdkInternalList(dependsOn.length));
}
for (ContainerDependency ele : dependsOn) {
this.dependsOn.add(ele);
}
return this;
}
/**
*
* The dependencies defined for container startup and shutdown. A container can contain multiple dependencies on
* other containers in a task definition. When a dependency is defined for container startup, for container shutdown
* it is reversed.
*
*
* For tasks using the EC2 launch type, the container instances require at least version 1.26.0 of the container
* agent to turn on container dependencies. However, we recommend using the latest container agent version. For
* information about checking your agent version and updating to the latest version, see Updating the Amazon ECS
* Container Agent in the Amazon Elastic Container Service Developer Guide. If you're using an Amazon
* ECS-optimized Linux AMI, your instance needs at least version 1.26.0-1 of the ecs-init
package. If
* your container instances are launched from version 20190301
or later, then they contain the required
* versions of the container agent and ecs-init
. For more information, see Amazon ECS-optimized
* Linux AMI in the Amazon Elastic Container Service Developer Guide.
*
*
* For tasks using the Fargate launch type, the task or service requires the following platforms:
*
*
* -
*
* Linux platform version 1.3.0
or later.
*
*
* -
*
* Windows platform version 1.0.0
or later.
*
*
*
*
* @param dependsOn
* The dependencies defined for container startup and shutdown. A container can contain multiple dependencies
* on other containers in a task definition. When a dependency is defined for container startup, for
* container shutdown it is reversed.
*
* For tasks using the EC2 launch type, the container instances require at least version 1.26.0 of the
* container agent to turn on container dependencies. However, we recommend using the latest container agent
* version. For information about checking your agent version and updating to the latest version, see Updating the
* Amazon ECS Container Agent in the Amazon Elastic Container Service Developer Guide. If you're
* using an Amazon ECS-optimized Linux AMI, your instance needs at least version 1.26.0-1 of the
* ecs-init
package. If your container instances are launched from version 20190301
* or later, then they contain the required versions of the container agent and ecs-init
. For
* more information, see Amazon
* ECS-optimized Linux AMI in the Amazon Elastic Container Service Developer Guide.
*
*
* For tasks using the Fargate launch type, the task or service requires the following platforms:
*
*
* -
*
* Linux platform version 1.3.0
or later.
*
*
* -
*
* Windows platform version 1.0.0
or later.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withDependsOn(java.util.Collection dependsOn) {
setDependsOn(dependsOn);
return this;
}
/**
*
* Time duration (in seconds) to wait before giving up on resolving dependencies for a container. For example, you
* specify two containers in a task definition with containerA having a dependency on containerB reaching a
* COMPLETE
, SUCCESS
, or HEALTHY
status. If a startTimeout
value
* is specified for containerB and it doesn't reach the desired status within that time then containerA gives up and
* not start. This results in the task transitioning to a STOPPED
state.
*
*
*
* When the ECS_CONTAINER_START_TIMEOUT
container agent configuration variable is used, it's enforced
* independently from this start timeout value.
*
*
*
* For tasks using the Fargate launch type, the task or service requires the following platforms:
*
*
* -
*
* Linux platform version 1.3.0
or later.
*
*
* -
*
* Windows platform version 1.0.0
or later.
*
*
*
*
* For tasks using the EC2 launch type, your container instances require at least version 1.26.0
of the
* container agent to use a container start timeout value. However, we recommend using the latest container agent
* version. For information about checking your agent version and updating to the latest version, see Updating the Amazon ECS
* Container Agent in the Amazon Elastic Container Service Developer Guide. If you're using an Amazon
* ECS-optimized Linux AMI, your instance needs at least version 1.26.0-1
of the ecs-init
* package. If your container instances are launched from version 20190301
or later, then they contain
* the required versions of the container agent and ecs-init
. For more information, see Amazon ECS-optimized
* Linux AMI in the Amazon Elastic Container Service Developer Guide.
*
*
* The valid values are 2-120 seconds.
*
*
* @param startTimeout
* Time duration (in seconds) to wait before giving up on resolving dependencies for a container. For
* example, you specify two containers in a task definition with containerA having a dependency on containerB
* reaching a COMPLETE
, SUCCESS
, or HEALTHY
status. If a
* startTimeout
value is specified for containerB and it doesn't reach the desired status within
* that time then containerA gives up and not start. This results in the task transitioning to a
* STOPPED
state.
*
* When the ECS_CONTAINER_START_TIMEOUT
container agent configuration variable is used, it's
* enforced independently from this start timeout value.
*
*
*
* For tasks using the Fargate launch type, the task or service requires the following platforms:
*
*
* -
*
* Linux platform version 1.3.0
or later.
*
*
* -
*
* Windows platform version 1.0.0
or later.
*
*
*
*
* For tasks using the EC2 launch type, your container instances require at least version 1.26.0
* of the container agent to use a container start timeout value. However, we recommend using the latest
* container agent version. For information about checking your agent version and updating to the latest
* version, see Updating the
* Amazon ECS Container Agent in the Amazon Elastic Container Service Developer Guide. If you're
* using an Amazon ECS-optimized Linux AMI, your instance needs at least version 1.26.0-1
of the
* ecs-init
package. If your container instances are launched from version 20190301
* or later, then they contain the required versions of the container agent and ecs-init
. For
* more information, see Amazon
* ECS-optimized Linux AMI in the Amazon Elastic Container Service Developer Guide.
*
*
* The valid values are 2-120 seconds.
*/
public void setStartTimeout(Integer startTimeout) {
this.startTimeout = startTimeout;
}
/**
*
* Time duration (in seconds) to wait before giving up on resolving dependencies for a container. For example, you
* specify two containers in a task definition with containerA having a dependency on containerB reaching a
* COMPLETE
, SUCCESS
, or HEALTHY
status. If a startTimeout
value
* is specified for containerB and it doesn't reach the desired status within that time then containerA gives up and
* not start. This results in the task transitioning to a STOPPED
state.
*
*
*
* When the ECS_CONTAINER_START_TIMEOUT
container agent configuration variable is used, it's enforced
* independently from this start timeout value.
*
*
*
* For tasks using the Fargate launch type, the task or service requires the following platforms:
*
*
* -
*
* Linux platform version 1.3.0
or later.
*
*
* -
*
* Windows platform version 1.0.0
or later.
*
*
*
*
* For tasks using the EC2 launch type, your container instances require at least version 1.26.0
of the
* container agent to use a container start timeout value. However, we recommend using the latest container agent
* version. For information about checking your agent version and updating to the latest version, see Updating the Amazon ECS
* Container Agent in the Amazon Elastic Container Service Developer Guide. If you're using an Amazon
* ECS-optimized Linux AMI, your instance needs at least version 1.26.0-1
of the ecs-init
* package. If your container instances are launched from version 20190301
or later, then they contain
* the required versions of the container agent and ecs-init
. For more information, see Amazon ECS-optimized
* Linux AMI in the Amazon Elastic Container Service Developer Guide.
*
*
* The valid values are 2-120 seconds.
*
*
* @return Time duration (in seconds) to wait before giving up on resolving dependencies for a container. For
* example, you specify two containers in a task definition with containerA having a dependency on
* containerB reaching a COMPLETE
, SUCCESS
, or HEALTHY
status. If a
* startTimeout
value is specified for containerB and it doesn't reach the desired status
* within that time then containerA gives up and not start. This results in the task transitioning to a
* STOPPED
state.
*
* When the ECS_CONTAINER_START_TIMEOUT
container agent configuration variable is used, it's
* enforced independently from this start timeout value.
*
*
*
* For tasks using the Fargate launch type, the task or service requires the following platforms:
*
*
* -
*
* Linux platform version 1.3.0
or later.
*
*
* -
*
* Windows platform version 1.0.0
or later.
*
*
*
*
* For tasks using the EC2 launch type, your container instances require at least version
* 1.26.0
of the container agent to use a container start timeout value. However, we recommend
* using the latest container agent version. For information about checking your agent version and updating
* to the latest version, see Updating the
* Amazon ECS Container Agent in the Amazon Elastic Container Service Developer Guide. If you're
* using an Amazon ECS-optimized Linux AMI, your instance needs at least version 1.26.0-1
of
* the ecs-init
package. If your container instances are launched from version
* 20190301
or later, then they contain the required versions of the container agent and
* ecs-init
. For more information, see Amazon
* ECS-optimized Linux AMI in the Amazon Elastic Container Service Developer Guide.
*
*
* The valid values are 2-120 seconds.
*/
public Integer getStartTimeout() {
return this.startTimeout;
}
/**
*
* Time duration (in seconds) to wait before giving up on resolving dependencies for a container. For example, you
* specify two containers in a task definition with containerA having a dependency on containerB reaching a
* COMPLETE
, SUCCESS
, or HEALTHY
status. If a startTimeout
value
* is specified for containerB and it doesn't reach the desired status within that time then containerA gives up and
* not start. This results in the task transitioning to a STOPPED
state.
*
*
*
* When the ECS_CONTAINER_START_TIMEOUT
container agent configuration variable is used, it's enforced
* independently from this start timeout value.
*
*
*
* For tasks using the Fargate launch type, the task or service requires the following platforms:
*
*
* -
*
* Linux platform version 1.3.0
or later.
*
*
* -
*
* Windows platform version 1.0.0
or later.
*
*
*
*
* For tasks using the EC2 launch type, your container instances require at least version 1.26.0
of the
* container agent to use a container start timeout value. However, we recommend using the latest container agent
* version. For information about checking your agent version and updating to the latest version, see Updating the Amazon ECS
* Container Agent in the Amazon Elastic Container Service Developer Guide. If you're using an Amazon
* ECS-optimized Linux AMI, your instance needs at least version 1.26.0-1
of the ecs-init
* package. If your container instances are launched from version 20190301
or later, then they contain
* the required versions of the container agent and ecs-init
. For more information, see Amazon ECS-optimized
* Linux AMI in the Amazon Elastic Container Service Developer Guide.
*
*
* The valid values are 2-120 seconds.
*
*
* @param startTimeout
* Time duration (in seconds) to wait before giving up on resolving dependencies for a container. For
* example, you specify two containers in a task definition with containerA having a dependency on containerB
* reaching a COMPLETE
, SUCCESS
, or HEALTHY
status. If a
* startTimeout
value is specified for containerB and it doesn't reach the desired status within
* that time then containerA gives up and not start. This results in the task transitioning to a
* STOPPED
state.
*
* When the ECS_CONTAINER_START_TIMEOUT
container agent configuration variable is used, it's
* enforced independently from this start timeout value.
*
*
*
* For tasks using the Fargate launch type, the task or service requires the following platforms:
*
*
* -
*
* Linux platform version 1.3.0
or later.
*
*
* -
*
* Windows platform version 1.0.0
or later.
*
*
*
*
* For tasks using the EC2 launch type, your container instances require at least version 1.26.0
* of the container agent to use a container start timeout value. However, we recommend using the latest
* container agent version. For information about checking your agent version and updating to the latest
* version, see Updating the
* Amazon ECS Container Agent in the Amazon Elastic Container Service Developer Guide. If you're
* using an Amazon ECS-optimized Linux AMI, your instance needs at least version 1.26.0-1
of the
* ecs-init
package. If your container instances are launched from version 20190301
* or later, then they contain the required versions of the container agent and ecs-init
. For
* more information, see Amazon
* ECS-optimized Linux AMI in the Amazon Elastic Container Service Developer Guide.
*
*
* The valid values are 2-120 seconds.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withStartTimeout(Integer startTimeout) {
setStartTimeout(startTimeout);
return this;
}
/**
*
* Time duration (in seconds) to wait before the container is forcefully killed if it doesn't exit normally on its
* own.
*
*
* For tasks using the Fargate launch type, the task or service requires the following platforms:
*
*
* -
*
* Linux platform version 1.3.0
or later.
*
*
* -
*
* Windows platform version 1.0.0
or later.
*
*
*
*
* The max stop timeout value is 120 seconds and if the parameter is not specified, the default value of 30 seconds
* is used.
*
*
* For tasks that use the EC2 launch type, if the stopTimeout
parameter isn't specified, the value set
* for the Amazon ECS container agent configuration variable ECS_CONTAINER_STOP_TIMEOUT
is used. If
* neither the stopTimeout
parameter or the ECS_CONTAINER_STOP_TIMEOUT
agent configuration
* variable are set, then the default values of 30 seconds for Linux containers and 30 seconds on Windows containers
* are used. Your container instances require at least version 1.26.0 of the container agent to use a container stop
* timeout value. However, we recommend using the latest container agent version. For information about checking
* your agent version and updating to the latest version, see Updating the Amazon ECS
* Container Agent in the Amazon Elastic Container Service Developer Guide. If you're using an Amazon
* ECS-optimized Linux AMI, your instance needs at least version 1.26.0-1 of the ecs-init
package. If
* your container instances are launched from version 20190301
or later, then they contain the required
* versions of the container agent and ecs-init
. For more information, see Amazon ECS-optimized
* Linux AMI in the Amazon Elastic Container Service Developer Guide.
*
*
* The valid values are 2-120 seconds.
*
*
* @param stopTimeout
* Time duration (in seconds) to wait before the container is forcefully killed if it doesn't exit normally
* on its own.
*
* For tasks using the Fargate launch type, the task or service requires the following platforms:
*
*
* -
*
* Linux platform version 1.3.0
or later.
*
*
* -
*
* Windows platform version 1.0.0
or later.
*
*
*
*
* The max stop timeout value is 120 seconds and if the parameter is not specified, the default value of 30
* seconds is used.
*
*
* For tasks that use the EC2 launch type, if the stopTimeout
parameter isn't specified, the
* value set for the Amazon ECS container agent configuration variable
* ECS_CONTAINER_STOP_TIMEOUT
is used. If neither the stopTimeout
parameter or the
* ECS_CONTAINER_STOP_TIMEOUT
agent configuration variable are set, then the default values of
* 30 seconds for Linux containers and 30 seconds on Windows containers are used. Your container instances
* require at least version 1.26.0 of the container agent to use a container stop timeout value. However, we
* recommend using the latest container agent version. For information about checking your agent version and
* updating to the latest version, see Updating the
* Amazon ECS Container Agent in the Amazon Elastic Container Service Developer Guide. If you're
* using an Amazon ECS-optimized Linux AMI, your instance needs at least version 1.26.0-1 of the
* ecs-init
package. If your container instances are launched from version 20190301
* or later, then they contain the required versions of the container agent and ecs-init
. For
* more information, see Amazon
* ECS-optimized Linux AMI in the Amazon Elastic Container Service Developer Guide.
*
*
* The valid values are 2-120 seconds.
*/
public void setStopTimeout(Integer stopTimeout) {
this.stopTimeout = stopTimeout;
}
/**
*
* Time duration (in seconds) to wait before the container is forcefully killed if it doesn't exit normally on its
* own.
*
*
* For tasks using the Fargate launch type, the task or service requires the following platforms:
*
*
* -
*
* Linux platform version 1.3.0
or later.
*
*
* -
*
* Windows platform version 1.0.0
or later.
*
*
*
*
* The max stop timeout value is 120 seconds and if the parameter is not specified, the default value of 30 seconds
* is used.
*
*
* For tasks that use the EC2 launch type, if the stopTimeout
parameter isn't specified, the value set
* for the Amazon ECS container agent configuration variable ECS_CONTAINER_STOP_TIMEOUT
is used. If
* neither the stopTimeout
parameter or the ECS_CONTAINER_STOP_TIMEOUT
agent configuration
* variable are set, then the default values of 30 seconds for Linux containers and 30 seconds on Windows containers
* are used. Your container instances require at least version 1.26.0 of the container agent to use a container stop
* timeout value. However, we recommend using the latest container agent version. For information about checking
* your agent version and updating to the latest version, see Updating the Amazon ECS
* Container Agent in the Amazon Elastic Container Service Developer Guide. If you're using an Amazon
* ECS-optimized Linux AMI, your instance needs at least version 1.26.0-1 of the ecs-init
package. If
* your container instances are launched from version 20190301
or later, then they contain the required
* versions of the container agent and ecs-init
. For more information, see Amazon ECS-optimized
* Linux AMI in the Amazon Elastic Container Service Developer Guide.
*
*
* The valid values are 2-120 seconds.
*
*
* @return Time duration (in seconds) to wait before the container is forcefully killed if it doesn't exit normally
* on its own.
*
* For tasks using the Fargate launch type, the task or service requires the following platforms:
*
*
* -
*
* Linux platform version 1.3.0
or later.
*
*
* -
*
* Windows platform version 1.0.0
or later.
*
*
*
*
* The max stop timeout value is 120 seconds and if the parameter is not specified, the default value of 30
* seconds is used.
*
*
* For tasks that use the EC2 launch type, if the stopTimeout
parameter isn't specified, the
* value set for the Amazon ECS container agent configuration variable
* ECS_CONTAINER_STOP_TIMEOUT
is used. If neither the stopTimeout
parameter or the
* ECS_CONTAINER_STOP_TIMEOUT
agent configuration variable are set, then the default values of
* 30 seconds for Linux containers and 30 seconds on Windows containers are used. Your container instances
* require at least version 1.26.0 of the container agent to use a container stop timeout value. However, we
* recommend using the latest container agent version. For information about checking your agent version and
* updating to the latest version, see Updating the
* Amazon ECS Container Agent in the Amazon Elastic Container Service Developer Guide. If you're
* using an Amazon ECS-optimized Linux AMI, your instance needs at least version 1.26.0-1 of the
* ecs-init
package. If your container instances are launched from version
* 20190301
or later, then they contain the required versions of the container agent and
* ecs-init
. For more information, see Amazon
* ECS-optimized Linux AMI in the Amazon Elastic Container Service Developer Guide.
*
*
* The valid values are 2-120 seconds.
*/
public Integer getStopTimeout() {
return this.stopTimeout;
}
/**
*
* Time duration (in seconds) to wait before the container is forcefully killed if it doesn't exit normally on its
* own.
*
*
* For tasks using the Fargate launch type, the task or service requires the following platforms:
*
*
* -
*
* Linux platform version 1.3.0
or later.
*
*
* -
*
* Windows platform version 1.0.0
or later.
*
*
*
*
* The max stop timeout value is 120 seconds and if the parameter is not specified, the default value of 30 seconds
* is used.
*
*
* For tasks that use the EC2 launch type, if the stopTimeout
parameter isn't specified, the value set
* for the Amazon ECS container agent configuration variable ECS_CONTAINER_STOP_TIMEOUT
is used. If
* neither the stopTimeout
parameter or the ECS_CONTAINER_STOP_TIMEOUT
agent configuration
* variable are set, then the default values of 30 seconds for Linux containers and 30 seconds on Windows containers
* are used. Your container instances require at least version 1.26.0 of the container agent to use a container stop
* timeout value. However, we recommend using the latest container agent version. For information about checking
* your agent version and updating to the latest version, see Updating the Amazon ECS
* Container Agent in the Amazon Elastic Container Service Developer Guide. If you're using an Amazon
* ECS-optimized Linux AMI, your instance needs at least version 1.26.0-1 of the ecs-init
package. If
* your container instances are launched from version 20190301
or later, then they contain the required
* versions of the container agent and ecs-init
. For more information, see Amazon ECS-optimized
* Linux AMI in the Amazon Elastic Container Service Developer Guide.
*
*
* The valid values are 2-120 seconds.
*
*
* @param stopTimeout
* Time duration (in seconds) to wait before the container is forcefully killed if it doesn't exit normally
* on its own.
*
* For tasks using the Fargate launch type, the task or service requires the following platforms:
*
*
* -
*
* Linux platform version 1.3.0
or later.
*
*
* -
*
* Windows platform version 1.0.0
or later.
*
*
*
*
* The max stop timeout value is 120 seconds and if the parameter is not specified, the default value of 30
* seconds is used.
*
*
* For tasks that use the EC2 launch type, if the stopTimeout
parameter isn't specified, the
* value set for the Amazon ECS container agent configuration variable
* ECS_CONTAINER_STOP_TIMEOUT
is used. If neither the stopTimeout
parameter or the
* ECS_CONTAINER_STOP_TIMEOUT
agent configuration variable are set, then the default values of
* 30 seconds for Linux containers and 30 seconds on Windows containers are used. Your container instances
* require at least version 1.26.0 of the container agent to use a container stop timeout value. However, we
* recommend using the latest container agent version. For information about checking your agent version and
* updating to the latest version, see Updating the
* Amazon ECS Container Agent in the Amazon Elastic Container Service Developer Guide. If you're
* using an Amazon ECS-optimized Linux AMI, your instance needs at least version 1.26.0-1 of the
* ecs-init
package. If your container instances are launched from version 20190301
* or later, then they contain the required versions of the container agent and ecs-init
. For
* more information, see Amazon
* ECS-optimized Linux AMI in the Amazon Elastic Container Service Developer Guide.
*
*
* The valid values are 2-120 seconds.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withStopTimeout(Integer stopTimeout) {
setStopTimeout(stopTimeout);
return this;
}
/**
*
* The hostname to use for your container. This parameter maps to Hostname
in the Create a container section of the
* Docker Remote API and the --hostname
option
* to docker run.
*
*
*
* The hostname
parameter is not supported if you're using the awsvpc
network mode.
*
*
*
* @param hostname
* The hostname to use for your container. This parameter maps to Hostname
in the Create a container section
* of the Docker Remote API and the
* --hostname
option to docker run.
*
* The hostname
parameter is not supported if you're using the awsvpc
network mode.
*
*/
public void setHostname(String hostname) {
this.hostname = hostname;
}
/**
*
* The hostname to use for your container. This parameter maps to Hostname
in the Create a container section of the
* Docker Remote API and the --hostname
option
* to docker run.
*
*
*
* The hostname
parameter is not supported if you're using the awsvpc
network mode.
*
*
*
* @return The hostname to use for your container. This parameter maps to Hostname
in the Create a container section
* of the Docker Remote API and the
* --hostname
option to docker run.
*
* The hostname
parameter is not supported if you're using the awsvpc
network
* mode.
*
*/
public String getHostname() {
return this.hostname;
}
/**
*
* The hostname to use for your container. This parameter maps to Hostname
in the Create a container section of the
* Docker Remote API and the --hostname
option
* to docker run.
*
*
*
* The hostname
parameter is not supported if you're using the awsvpc
network mode.
*
*
*
* @param hostname
* The hostname to use for your container. This parameter maps to Hostname
in the Create a container section
* of the Docker Remote API and the
* --hostname
option to docker run.
*
* The hostname
parameter is not supported if you're using the awsvpc
network mode.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withHostname(String hostname) {
setHostname(hostname);
return this;
}
/**
*
* The user to use inside the container. This parameter maps to User
in the Create a container section of the
* Docker Remote API and the --user
option to
* docker run.
*
*
*
* When running tasks using the host
network mode, don't run containers using the root user (UID 0). We
* recommend using a non-root user for better security.
*
*
*
* You can specify the user
using the following formats. If specifying a UID or GID, you must specify
* it as a positive integer.
*
*
* -
*
* user
*
*
* -
*
* user:group
*
*
* -
*
* uid
*
*
* -
*
* uid:gid
*
*
* -
*
* user:gid
*
*
* -
*
* uid:group
*
*
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* @param user
* The user to use inside the container. This parameter maps to User
in the Create a container section
* of the Docker Remote API and the
* --user
option to docker run.
*
*
* When running tasks using the host
network mode, don't run containers using the root user (UID
* 0). We recommend using a non-root user for better security.
*
*
*
* You can specify the user
using the following formats. If specifying a UID or GID, you must
* specify it as a positive integer.
*
*
* -
*
* user
*
*
* -
*
* user:group
*
*
* -
*
* uid
*
*
* -
*
* uid:gid
*
*
* -
*
* user:gid
*
*
* -
*
* uid:group
*
*
*
*
*
* This parameter is not supported for Windows containers.
*
*/
public void setUser(String user) {
this.user = user;
}
/**
*
* The user to use inside the container. This parameter maps to User
in the Create a container section of the
* Docker Remote API and the --user
option to
* docker run.
*
*
*
* When running tasks using the host
network mode, don't run containers using the root user (UID 0). We
* recommend using a non-root user for better security.
*
*
*
* You can specify the user
using the following formats. If specifying a UID or GID, you must specify
* it as a positive integer.
*
*
* -
*
* user
*
*
* -
*
* user:group
*
*
* -
*
* uid
*
*
* -
*
* uid:gid
*
*
* -
*
* user:gid
*
*
* -
*
* uid:group
*
*
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* @return The user to use inside the container. This parameter maps to User
in the Create a container section
* of the Docker Remote API and the
* --user
option to docker run.
*
*
* When running tasks using the host
network mode, don't run containers using the root user
* (UID 0). We recommend using a non-root user for better security.
*
*
*
* You can specify the user
using the following formats. If specifying a UID or GID, you must
* specify it as a positive integer.
*
*
* -
*
* user
*
*
* -
*
* user:group
*
*
* -
*
* uid
*
*
* -
*
* uid:gid
*
*
* -
*
* user:gid
*
*
* -
*
* uid:group
*
*
*
*
*
* This parameter is not supported for Windows containers.
*
*/
public String getUser() {
return this.user;
}
/**
*
* The user to use inside the container. This parameter maps to User
in the Create a container section of the
* Docker Remote API and the --user
option to
* docker run.
*
*
*
* When running tasks using the host
network mode, don't run containers using the root user (UID 0). We
* recommend using a non-root user for better security.
*
*
*
* You can specify the user
using the following formats. If specifying a UID or GID, you must specify
* it as a positive integer.
*
*
* -
*
* user
*
*
* -
*
* user:group
*
*
* -
*
* uid
*
*
* -
*
* uid:gid
*
*
* -
*
* user:gid
*
*
* -
*
* uid:group
*
*
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* @param user
* The user to use inside the container. This parameter maps to User
in the Create a container section
* of the Docker Remote API and the
* --user
option to docker run.
*
*
* When running tasks using the host
network mode, don't run containers using the root user (UID
* 0). We recommend using a non-root user for better security.
*
*
*
* You can specify the user
using the following formats. If specifying a UID or GID, you must
* specify it as a positive integer.
*
*
* -
*
* user
*
*
* -
*
* user:group
*
*
* -
*
* uid
*
*
* -
*
* uid:gid
*
*
* -
*
* user:gid
*
*
* -
*
* uid:group
*
*
*
*
*
* This parameter is not supported for Windows containers.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withUser(String user) {
setUser(user);
return this;
}
/**
*
* The working directory to run commands inside the container in. This parameter maps to WorkingDir
in
* the Create a container section
* of the Docker Remote API and the --workdir
* option to docker run.
*
*
* @param workingDirectory
* The working directory to run commands inside the container in. This parameter maps to
* WorkingDir
in the Create a container section
* of the Docker Remote API and the
* --workdir
option to docker run.
*/
public void setWorkingDirectory(String workingDirectory) {
this.workingDirectory = workingDirectory;
}
/**
*
* The working directory to run commands inside the container in. This parameter maps to WorkingDir
in
* the Create a container section
* of the Docker Remote API and the --workdir
* option to docker run.
*
*
* @return The working directory to run commands inside the container in. This parameter maps to
* WorkingDir
in the Create a container section
* of the Docker Remote API and the
* --workdir
option to docker run.
*/
public String getWorkingDirectory() {
return this.workingDirectory;
}
/**
*
* The working directory to run commands inside the container in. This parameter maps to WorkingDir
in
* the Create a container section
* of the Docker Remote API and the --workdir
* option to docker run.
*
*
* @param workingDirectory
* The working directory to run commands inside the container in. This parameter maps to
* WorkingDir
in the Create a container section
* of the Docker Remote API and the
* --workdir
option to docker run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withWorkingDirectory(String workingDirectory) {
setWorkingDirectory(workingDirectory);
return this;
}
/**
*
* When this parameter is true, networking is off within the container. This parameter maps to
* NetworkDisabled
in the Create a container section of the
* Docker Remote API.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* @param disableNetworking
* When this parameter is true, networking is off within the container. This parameter maps to
* NetworkDisabled
in the Create a container section
* of the Docker Remote API.
*
* This parameter is not supported for Windows containers.
*
*/
public void setDisableNetworking(Boolean disableNetworking) {
this.disableNetworking = disableNetworking;
}
/**
*
* When this parameter is true, networking is off within the container. This parameter maps to
* NetworkDisabled
in the Create a container section of the
* Docker Remote API.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* @return When this parameter is true, networking is off within the container. This parameter maps to
* NetworkDisabled
in the Create a container section
* of the Docker Remote API.
*
* This parameter is not supported for Windows containers.
*
*/
public Boolean getDisableNetworking() {
return this.disableNetworking;
}
/**
*
* When this parameter is true, networking is off within the container. This parameter maps to
* NetworkDisabled
in the Create a container section of the
* Docker Remote API.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* @param disableNetworking
* When this parameter is true, networking is off within the container. This parameter maps to
* NetworkDisabled
in the Create a container section
* of the Docker Remote API.
*
* This parameter is not supported for Windows containers.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withDisableNetworking(Boolean disableNetworking) {
setDisableNetworking(disableNetworking);
return this;
}
/**
*
* When this parameter is true, networking is off within the container. This parameter maps to
* NetworkDisabled
in the Create a container section of the
* Docker Remote API.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* @return When this parameter is true, networking is off within the container. This parameter maps to
* NetworkDisabled
in the Create a container section
* of the Docker Remote API.
*
* This parameter is not supported for Windows containers.
*
*/
public Boolean isDisableNetworking() {
return this.disableNetworking;
}
/**
*
* When this parameter is true, the container is given elevated privileges on the host container instance (similar
* to the root
user). This parameter maps to Privileged
in the Create a container section of the
* Docker Remote API and the --privileged
* option to docker run.
*
*
*
* This parameter is not supported for Windows containers or tasks run on Fargate.
*
*
*
* @param privileged
* When this parameter is true, the container is given elevated privileges on the host container instance
* (similar to the root
user). This parameter maps to Privileged
in the Create a container section
* of the Docker Remote API and the
* --privileged
option to docker run.
*
* This parameter is not supported for Windows containers or tasks run on Fargate.
*
*/
public void setPrivileged(Boolean privileged) {
this.privileged = privileged;
}
/**
*
* When this parameter is true, the container is given elevated privileges on the host container instance (similar
* to the root
user). This parameter maps to Privileged
in the Create a container section of the
* Docker Remote API and the --privileged
* option to docker run.
*
*
*
* This parameter is not supported for Windows containers or tasks run on Fargate.
*
*
*
* @return When this parameter is true, the container is given elevated privileges on the host container instance
* (similar to the root
user). This parameter maps to Privileged
in the Create a container section
* of the Docker Remote API and the
* --privileged
option to docker run.
*
* This parameter is not supported for Windows containers or tasks run on Fargate.
*
*/
public Boolean getPrivileged() {
return this.privileged;
}
/**
*
* When this parameter is true, the container is given elevated privileges on the host container instance (similar
* to the root
user). This parameter maps to Privileged
in the Create a container section of the
* Docker Remote API and the --privileged
* option to docker run.
*
*
*
* This parameter is not supported for Windows containers or tasks run on Fargate.
*
*
*
* @param privileged
* When this parameter is true, the container is given elevated privileges on the host container instance
* (similar to the root
user). This parameter maps to Privileged
in the Create a container section
* of the Docker Remote API and the
* --privileged
option to docker run.
*
* This parameter is not supported for Windows containers or tasks run on Fargate.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withPrivileged(Boolean privileged) {
setPrivileged(privileged);
return this;
}
/**
*
* When this parameter is true, the container is given elevated privileges on the host container instance (similar
* to the root
user). This parameter maps to Privileged
in the Create a container section of the
* Docker Remote API and the --privileged
* option to docker run.
*
*
*
* This parameter is not supported for Windows containers or tasks run on Fargate.
*
*
*
* @return When this parameter is true, the container is given elevated privileges on the host container instance
* (similar to the root
user). This parameter maps to Privileged
in the Create a container section
* of the Docker Remote API and the
* --privileged
option to docker run.
*
* This parameter is not supported for Windows containers or tasks run on Fargate.
*
*/
public Boolean isPrivileged() {
return this.privileged;
}
/**
*
* When this parameter is true, the container is given read-only access to its root file system. This parameter maps
* to ReadonlyRootfs
in the Create a container section of the
* Docker Remote API and the --read-only
option
* to docker run.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* @param readonlyRootFilesystem
* When this parameter is true, the container is given read-only access to its root file system. This
* parameter maps to ReadonlyRootfs
in the Create a container section
* of the Docker Remote API and the
* --read-only
option to docker run.
*
* This parameter is not supported for Windows containers.
*
*/
public void setReadonlyRootFilesystem(Boolean readonlyRootFilesystem) {
this.readonlyRootFilesystem = readonlyRootFilesystem;
}
/**
*
* When this parameter is true, the container is given read-only access to its root file system. This parameter maps
* to ReadonlyRootfs
in the Create a container section of the
* Docker Remote API and the --read-only
option
* to docker run.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* @return When this parameter is true, the container is given read-only access to its root file system. This
* parameter maps to ReadonlyRootfs
in the Create a container section
* of the Docker Remote API and the
* --read-only
option to docker run.
*
* This parameter is not supported for Windows containers.
*
*/
public Boolean getReadonlyRootFilesystem() {
return this.readonlyRootFilesystem;
}
/**
*
* When this parameter is true, the container is given read-only access to its root file system. This parameter maps
* to ReadonlyRootfs
in the Create a container section of the
* Docker Remote API and the --read-only
option
* to docker run.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* @param readonlyRootFilesystem
* When this parameter is true, the container is given read-only access to its root file system. This
* parameter maps to ReadonlyRootfs
in the Create a container section
* of the Docker Remote API and the
* --read-only
option to docker run.
*
* This parameter is not supported for Windows containers.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withReadonlyRootFilesystem(Boolean readonlyRootFilesystem) {
setReadonlyRootFilesystem(readonlyRootFilesystem);
return this;
}
/**
*
* When this parameter is true, the container is given read-only access to its root file system. This parameter maps
* to ReadonlyRootfs
in the Create a container section of the
* Docker Remote API and the --read-only
option
* to docker run.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* @return When this parameter is true, the container is given read-only access to its root file system. This
* parameter maps to ReadonlyRootfs
in the Create a container section
* of the Docker Remote API and the
* --read-only
option to docker run.
*
* This parameter is not supported for Windows containers.
*
*/
public Boolean isReadonlyRootFilesystem() {
return this.readonlyRootFilesystem;
}
/**
*
* A list of DNS servers that are presented to the container. This parameter maps to Dns
in the Create a container section of the
* Docker Remote API and the --dns
option to docker run.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* @return A list of DNS servers that are presented to the container. This parameter maps to Dns
in the
* Create a container
* section of the Docker Remote API and the
* --dns
option to docker run.
*
* This parameter is not supported for Windows containers.
*
*/
public java.util.List getDnsServers() {
if (dnsServers == null) {
dnsServers = new com.amazonaws.internal.SdkInternalList();
}
return dnsServers;
}
/**
*
* A list of DNS servers that are presented to the container. This parameter maps to Dns
in the Create a container section of the
* Docker Remote API and the --dns
option to docker run.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* @param dnsServers
* A list of DNS servers that are presented to the container. This parameter maps to Dns
in the
* Create a container
* section of the Docker Remote API and the
* --dns
option to docker run.
*
* This parameter is not supported for Windows containers.
*
*/
public void setDnsServers(java.util.Collection dnsServers) {
if (dnsServers == null) {
this.dnsServers = null;
return;
}
this.dnsServers = new com.amazonaws.internal.SdkInternalList(dnsServers);
}
/**
*
* A list of DNS servers that are presented to the container. This parameter maps to Dns
in the Create a container section of the
* Docker Remote API and the --dns
option to docker run.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDnsServers(java.util.Collection)} or {@link #withDnsServers(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param dnsServers
* A list of DNS servers that are presented to the container. This parameter maps to Dns
in the
* Create a container
* section of the Docker Remote API and the
* --dns
option to docker run.
*
* This parameter is not supported for Windows containers.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withDnsServers(String... dnsServers) {
if (this.dnsServers == null) {
setDnsServers(new com.amazonaws.internal.SdkInternalList(dnsServers.length));
}
for (String ele : dnsServers) {
this.dnsServers.add(ele);
}
return this;
}
/**
*
* A list of DNS servers that are presented to the container. This parameter maps to Dns
in the Create a container section of the
* Docker Remote API and the --dns
option to docker run.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* @param dnsServers
* A list of DNS servers that are presented to the container. This parameter maps to Dns
in the
* Create a container
* section of the Docker Remote API and the
* --dns
option to docker run.
*
* This parameter is not supported for Windows containers.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withDnsServers(java.util.Collection dnsServers) {
setDnsServers(dnsServers);
return this;
}
/**
*
* A list of DNS search domains that are presented to the container. This parameter maps to DnsSearch
* in the Create a container
* section of the Docker Remote API and the
* --dns-search
option to docker run.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* @return A list of DNS search domains that are presented to the container. This parameter maps to
* DnsSearch
in the Create a container section
* of the Docker Remote API and the
* --dns-search
option to docker run.
*
* This parameter is not supported for Windows containers.
*
*/
public java.util.List getDnsSearchDomains() {
if (dnsSearchDomains == null) {
dnsSearchDomains = new com.amazonaws.internal.SdkInternalList();
}
return dnsSearchDomains;
}
/**
*
* A list of DNS search domains that are presented to the container. This parameter maps to DnsSearch
* in the Create a container
* section of the Docker Remote API and the
* --dns-search
option to docker run.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* @param dnsSearchDomains
* A list of DNS search domains that are presented to the container. This parameter maps to
* DnsSearch
in the Create a container section
* of the Docker Remote API and the
* --dns-search
option to docker run.
*
* This parameter is not supported for Windows containers.
*
*/
public void setDnsSearchDomains(java.util.Collection dnsSearchDomains) {
if (dnsSearchDomains == null) {
this.dnsSearchDomains = null;
return;
}
this.dnsSearchDomains = new com.amazonaws.internal.SdkInternalList(dnsSearchDomains);
}
/**
*
* A list of DNS search domains that are presented to the container. This parameter maps to DnsSearch
* in the Create a container
* section of the Docker Remote API and the
* --dns-search
option to docker run.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDnsSearchDomains(java.util.Collection)} or {@link #withDnsSearchDomains(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param dnsSearchDomains
* A list of DNS search domains that are presented to the container. This parameter maps to
* DnsSearch
in the Create a container section
* of the Docker Remote API and the
* --dns-search
option to docker run.
*
* This parameter is not supported for Windows containers.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withDnsSearchDomains(String... dnsSearchDomains) {
if (this.dnsSearchDomains == null) {
setDnsSearchDomains(new com.amazonaws.internal.SdkInternalList(dnsSearchDomains.length));
}
for (String ele : dnsSearchDomains) {
this.dnsSearchDomains.add(ele);
}
return this;
}
/**
*
* A list of DNS search domains that are presented to the container. This parameter maps to DnsSearch
* in the Create a container
* section of the Docker Remote API and the
* --dns-search
option to docker run.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* @param dnsSearchDomains
* A list of DNS search domains that are presented to the container. This parameter maps to
* DnsSearch
in the Create a container section
* of the Docker Remote API and the
* --dns-search
option to docker run.
*
* This parameter is not supported for Windows containers.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withDnsSearchDomains(java.util.Collection dnsSearchDomains) {
setDnsSearchDomains(dnsSearchDomains);
return this;
}
/**
*
* A list of hostnames and IP address mappings to append to the /etc/hosts
file on the container. This
* parameter maps to ExtraHosts
in the Create a container section of the
* Docker Remote API and the --add-host
option
* to docker run.
*
*
*
* This parameter isn't supported for Windows containers or tasks that use the awsvpc
network mode.
*
*
*
* @return A list of hostnames and IP address mappings to append to the /etc/hosts
file on the
* container. This parameter maps to ExtraHosts
in the Create a container section
* of the Docker Remote API and the
* --add-host
option to docker run.
*
* This parameter isn't supported for Windows containers or tasks that use the awsvpc
network
* mode.
*
*/
public java.util.List getExtraHosts() {
if (extraHosts == null) {
extraHosts = new com.amazonaws.internal.SdkInternalList();
}
return extraHosts;
}
/**
*
* A list of hostnames and IP address mappings to append to the /etc/hosts
file on the container. This
* parameter maps to ExtraHosts
in the Create a container section of the
* Docker Remote API and the --add-host
option
* to docker run.
*
*
*
* This parameter isn't supported for Windows containers or tasks that use the awsvpc
network mode.
*
*
*
* @param extraHosts
* A list of hostnames and IP address mappings to append to the /etc/hosts
file on the
* container. This parameter maps to ExtraHosts
in the Create a container section
* of the Docker Remote API and the
* --add-host
option to docker run.
*
* This parameter isn't supported for Windows containers or tasks that use the awsvpc
network
* mode.
*
*/
public void setExtraHosts(java.util.Collection extraHosts) {
if (extraHosts == null) {
this.extraHosts = null;
return;
}
this.extraHosts = new com.amazonaws.internal.SdkInternalList(extraHosts);
}
/**
*
* A list of hostnames and IP address mappings to append to the /etc/hosts
file on the container. This
* parameter maps to ExtraHosts
in the Create a container section of the
* Docker Remote API and the --add-host
option
* to docker run.
*
*
*
* This parameter isn't supported for Windows containers or tasks that use the awsvpc
network mode.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setExtraHosts(java.util.Collection)} or {@link #withExtraHosts(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param extraHosts
* A list of hostnames and IP address mappings to append to the /etc/hosts
file on the
* container. This parameter maps to ExtraHosts
in the Create a container section
* of the Docker Remote API and the
* --add-host
option to docker run.
*
* This parameter isn't supported for Windows containers or tasks that use the awsvpc
network
* mode.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withExtraHosts(HostEntry... extraHosts) {
if (this.extraHosts == null) {
setExtraHosts(new com.amazonaws.internal.SdkInternalList(extraHosts.length));
}
for (HostEntry ele : extraHosts) {
this.extraHosts.add(ele);
}
return this;
}
/**
*
* A list of hostnames and IP address mappings to append to the /etc/hosts
file on the container. This
* parameter maps to ExtraHosts
in the Create a container section of the
* Docker Remote API and the --add-host
option
* to docker run.
*
*
*
* This parameter isn't supported for Windows containers or tasks that use the awsvpc
network mode.
*
*
*
* @param extraHosts
* A list of hostnames and IP address mappings to append to the /etc/hosts
file on the
* container. This parameter maps to ExtraHosts
in the Create a container section
* of the Docker Remote API and the
* --add-host
option to docker run.
*
* This parameter isn't supported for Windows containers or tasks that use the awsvpc
network
* mode.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withExtraHosts(java.util.Collection extraHosts) {
setExtraHosts(extraHosts);
return this;
}
/**
*
* A list of strings to provide custom configuration for multiple security systems. For more information about valid
* values, see Docker Run Security
* Configuration. This field isn't valid for containers in tasks using the Fargate launch type.
*
*
* For Linux tasks on EC2, this parameter can be used to reference custom labels for SELinux and AppArmor
* multi-level security systems.
*
*
* For any tasks on EC2, this parameter can be used to reference a credential spec file that configures a container
* for Active Directory authentication. For more information, see Using gMSAs for Windows
* Containers and Using
* gMSAs for Linux Containers in the Amazon Elastic Container Service Developer Guide.
*
*
* This parameter maps to SecurityOpt
in the Create a container section of the
* Docker Remote API and the --security-opt
* option to docker run.
*
*
*
* The Amazon ECS container agent running on a container instance must register with the
* ECS_SELINUX_CAPABLE=true
or ECS_APPARMOR_CAPABLE=true
environment variables before
* containers placed on that instance can use these security options. For more information, see Amazon ECS Container
* Agent Configuration in the Amazon Elastic Container Service Developer Guide.
*
*
*
* For more information about valid values, see Docker Run Security
* Configuration.
*
*
* Valid values: "no-new-privileges" | "apparmor:PROFILE" | "label:value" | "credentialspec:CredentialSpecFilePath"
*
*
* @return A list of strings to provide custom configuration for multiple security systems. For more information
* about valid values, see Docker Run Security
* Configuration. This field isn't valid for containers in tasks using the Fargate launch type.
*
* For Linux tasks on EC2, this parameter can be used to reference custom labels for SELinux and AppArmor
* multi-level security systems.
*
*
* For any tasks on EC2, this parameter can be used to reference a credential spec file that configures a
* container for Active Directory authentication. For more information, see Using gMSAs for
* Windows Containers and Using gMSAs for Linux
* Containers in the Amazon Elastic Container Service Developer Guide.
*
*
* This parameter maps to SecurityOpt
in the Create a container section
* of the Docker Remote API and the
* --security-opt
option to docker run.
*
*
*
* The Amazon ECS container agent running on a container instance must register with the
* ECS_SELINUX_CAPABLE=true
or ECS_APPARMOR_CAPABLE=true
environment variables
* before containers placed on that instance can use these security options. For more information, see Amazon ECS
* Container Agent Configuration in the Amazon Elastic Container Service Developer Guide.
*
*
*
* For more information about valid values, see Docker Run Security
* Configuration.
*
*
* Valid values: "no-new-privileges" | "apparmor:PROFILE" | "label:value" |
* "credentialspec:CredentialSpecFilePath"
*/
public java.util.List getDockerSecurityOptions() {
if (dockerSecurityOptions == null) {
dockerSecurityOptions = new com.amazonaws.internal.SdkInternalList();
}
return dockerSecurityOptions;
}
/**
*
* A list of strings to provide custom configuration for multiple security systems. For more information about valid
* values, see Docker Run Security
* Configuration. This field isn't valid for containers in tasks using the Fargate launch type.
*
*
* For Linux tasks on EC2, this parameter can be used to reference custom labels for SELinux and AppArmor
* multi-level security systems.
*
*
* For any tasks on EC2, this parameter can be used to reference a credential spec file that configures a container
* for Active Directory authentication. For more information, see Using gMSAs for Windows
* Containers and Using
* gMSAs for Linux Containers in the Amazon Elastic Container Service Developer Guide.
*
*
* This parameter maps to SecurityOpt
in the Create a container section of the
* Docker Remote API and the --security-opt
* option to docker run.
*
*
*
* The Amazon ECS container agent running on a container instance must register with the
* ECS_SELINUX_CAPABLE=true
or ECS_APPARMOR_CAPABLE=true
environment variables before
* containers placed on that instance can use these security options. For more information, see Amazon ECS Container
* Agent Configuration in the Amazon Elastic Container Service Developer Guide.
*
*
*
* For more information about valid values, see Docker Run Security
* Configuration.
*
*
* Valid values: "no-new-privileges" | "apparmor:PROFILE" | "label:value" | "credentialspec:CredentialSpecFilePath"
*
*
* @param dockerSecurityOptions
* A list of strings to provide custom configuration for multiple security systems. For more information
* about valid values, see Docker Run Security
* Configuration. This field isn't valid for containers in tasks using the Fargate launch type.
*
* For Linux tasks on EC2, this parameter can be used to reference custom labels for SELinux and AppArmor
* multi-level security systems.
*
*
* For any tasks on EC2, this parameter can be used to reference a credential spec file that configures a
* container for Active Directory authentication. For more information, see Using gMSAs for
* Windows Containers and Using gMSAs for Linux
* Containers in the Amazon Elastic Container Service Developer Guide.
*
*
* This parameter maps to SecurityOpt
in the Create a container section
* of the Docker Remote API and the
* --security-opt
option to docker run.
*
*
*
* The Amazon ECS container agent running on a container instance must register with the
* ECS_SELINUX_CAPABLE=true
or ECS_APPARMOR_CAPABLE=true
environment variables
* before containers placed on that instance can use these security options. For more information, see Amazon ECS
* Container Agent Configuration in the Amazon Elastic Container Service Developer Guide.
*
*
*
* For more information about valid values, see Docker Run Security
* Configuration.
*
*
* Valid values: "no-new-privileges" | "apparmor:PROFILE" | "label:value" |
* "credentialspec:CredentialSpecFilePath"
*/
public void setDockerSecurityOptions(java.util.Collection dockerSecurityOptions) {
if (dockerSecurityOptions == null) {
this.dockerSecurityOptions = null;
return;
}
this.dockerSecurityOptions = new com.amazonaws.internal.SdkInternalList(dockerSecurityOptions);
}
/**
*
* A list of strings to provide custom configuration for multiple security systems. For more information about valid
* values, see Docker Run Security
* Configuration. This field isn't valid for containers in tasks using the Fargate launch type.
*
*
* For Linux tasks on EC2, this parameter can be used to reference custom labels for SELinux and AppArmor
* multi-level security systems.
*
*
* For any tasks on EC2, this parameter can be used to reference a credential spec file that configures a container
* for Active Directory authentication. For more information, see Using gMSAs for Windows
* Containers and Using
* gMSAs for Linux Containers in the Amazon Elastic Container Service Developer Guide.
*
*
* This parameter maps to SecurityOpt
in the Create a container section of the
* Docker Remote API and the --security-opt
* option to docker run.
*
*
*
* The Amazon ECS container agent running on a container instance must register with the
* ECS_SELINUX_CAPABLE=true
or ECS_APPARMOR_CAPABLE=true
environment variables before
* containers placed on that instance can use these security options. For more information, see Amazon ECS Container
* Agent Configuration in the Amazon Elastic Container Service Developer Guide.
*
*
*
* For more information about valid values, see Docker Run Security
* Configuration.
*
*
* Valid values: "no-new-privileges" | "apparmor:PROFILE" | "label:value" | "credentialspec:CredentialSpecFilePath"
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDockerSecurityOptions(java.util.Collection)} or
* {@link #withDockerSecurityOptions(java.util.Collection)} if you want to override the existing values.
*
*
* @param dockerSecurityOptions
* A list of strings to provide custom configuration for multiple security systems. For more information
* about valid values, see Docker Run Security
* Configuration. This field isn't valid for containers in tasks using the Fargate launch type.
*
* For Linux tasks on EC2, this parameter can be used to reference custom labels for SELinux and AppArmor
* multi-level security systems.
*
*
* For any tasks on EC2, this parameter can be used to reference a credential spec file that configures a
* container for Active Directory authentication. For more information, see Using gMSAs for
* Windows Containers and Using gMSAs for Linux
* Containers in the Amazon Elastic Container Service Developer Guide.
*
*
* This parameter maps to SecurityOpt
in the Create a container section
* of the Docker Remote API and the
* --security-opt
option to docker run.
*
*
*
* The Amazon ECS container agent running on a container instance must register with the
* ECS_SELINUX_CAPABLE=true
or ECS_APPARMOR_CAPABLE=true
environment variables
* before containers placed on that instance can use these security options. For more information, see Amazon ECS
* Container Agent Configuration in the Amazon Elastic Container Service Developer Guide.
*
*
*
* For more information about valid values, see Docker Run Security
* Configuration.
*
*
* Valid values: "no-new-privileges" | "apparmor:PROFILE" | "label:value" |
* "credentialspec:CredentialSpecFilePath"
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withDockerSecurityOptions(String... dockerSecurityOptions) {
if (this.dockerSecurityOptions == null) {
setDockerSecurityOptions(new com.amazonaws.internal.SdkInternalList(dockerSecurityOptions.length));
}
for (String ele : dockerSecurityOptions) {
this.dockerSecurityOptions.add(ele);
}
return this;
}
/**
*
* A list of strings to provide custom configuration for multiple security systems. For more information about valid
* values, see Docker Run Security
* Configuration. This field isn't valid for containers in tasks using the Fargate launch type.
*
*
* For Linux tasks on EC2, this parameter can be used to reference custom labels for SELinux and AppArmor
* multi-level security systems.
*
*
* For any tasks on EC2, this parameter can be used to reference a credential spec file that configures a container
* for Active Directory authentication. For more information, see Using gMSAs for Windows
* Containers and Using
* gMSAs for Linux Containers in the Amazon Elastic Container Service Developer Guide.
*
*
* This parameter maps to SecurityOpt
in the Create a container section of the
* Docker Remote API and the --security-opt
* option to docker run.
*
*
*
* The Amazon ECS container agent running on a container instance must register with the
* ECS_SELINUX_CAPABLE=true
or ECS_APPARMOR_CAPABLE=true
environment variables before
* containers placed on that instance can use these security options. For more information, see Amazon ECS Container
* Agent Configuration in the Amazon Elastic Container Service Developer Guide.
*
*
*
* For more information about valid values, see Docker Run Security
* Configuration.
*
*
* Valid values: "no-new-privileges" | "apparmor:PROFILE" | "label:value" | "credentialspec:CredentialSpecFilePath"
*
*
* @param dockerSecurityOptions
* A list of strings to provide custom configuration for multiple security systems. For more information
* about valid values, see Docker Run Security
* Configuration. This field isn't valid for containers in tasks using the Fargate launch type.
*
* For Linux tasks on EC2, this parameter can be used to reference custom labels for SELinux and AppArmor
* multi-level security systems.
*
*
* For any tasks on EC2, this parameter can be used to reference a credential spec file that configures a
* container for Active Directory authentication. For more information, see Using gMSAs for
* Windows Containers and Using gMSAs for Linux
* Containers in the Amazon Elastic Container Service Developer Guide.
*
*
* This parameter maps to SecurityOpt
in the Create a container section
* of the Docker Remote API and the
* --security-opt
option to docker run.
*
*
*
* The Amazon ECS container agent running on a container instance must register with the
* ECS_SELINUX_CAPABLE=true
or ECS_APPARMOR_CAPABLE=true
environment variables
* before containers placed on that instance can use these security options. For more information, see Amazon ECS
* Container Agent Configuration in the Amazon Elastic Container Service Developer Guide.
*
*
*
* For more information about valid values, see Docker Run Security
* Configuration.
*
*
* Valid values: "no-new-privileges" | "apparmor:PROFILE" | "label:value" |
* "credentialspec:CredentialSpecFilePath"
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withDockerSecurityOptions(java.util.Collection dockerSecurityOptions) {
setDockerSecurityOptions(dockerSecurityOptions);
return this;
}
/**
*
* When this parameter is true
, you can deploy containerized applications that require
* stdin
or a tty
to be allocated. This parameter maps to OpenStdin
in the Create a container section of the
* Docker Remote API and the --interactive
* option to docker run.
*
*
* @param interactive
* When this parameter is true
, you can deploy containerized applications that require
* stdin
or a tty
to be allocated. This parameter maps to OpenStdin
in
* the Create a container
* section of the Docker Remote API and the
* --interactive
option to docker run.
*/
public void setInteractive(Boolean interactive) {
this.interactive = interactive;
}
/**
*
* When this parameter is true
, you can deploy containerized applications that require
* stdin
or a tty
to be allocated. This parameter maps to OpenStdin
in the Create a container section of the
* Docker Remote API and the --interactive
* option to docker run.
*
*
* @return When this parameter is true
, you can deploy containerized applications that require
* stdin
or a tty
to be allocated. This parameter maps to OpenStdin
* in the Create a
* container section of the Docker Remote API
* and the --interactive
option to docker run.
*/
public Boolean getInteractive() {
return this.interactive;
}
/**
*
* When this parameter is true
, you can deploy containerized applications that require
* stdin
or a tty
to be allocated. This parameter maps to OpenStdin
in the Create a container section of the
* Docker Remote API and the --interactive
* option to docker run.
*
*
* @param interactive
* When this parameter is true
, you can deploy containerized applications that require
* stdin
or a tty
to be allocated. This parameter maps to OpenStdin
in
* the Create a container
* section of the Docker Remote API and the
* --interactive
option to docker run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withInteractive(Boolean interactive) {
setInteractive(interactive);
return this;
}
/**
*
* When this parameter is true
, you can deploy containerized applications that require
* stdin
or a tty
to be allocated. This parameter maps to OpenStdin
in the Create a container section of the
* Docker Remote API and the --interactive
* option to docker run.
*
*
* @return When this parameter is true
, you can deploy containerized applications that require
* stdin
or a tty
to be allocated. This parameter maps to OpenStdin
* in the Create a
* container section of the Docker Remote API
* and the --interactive
option to docker run.
*/
public Boolean isInteractive() {
return this.interactive;
}
/**
*
* When this parameter is true
, a TTY is allocated. This parameter maps to Tty
in the Create a container section of the
* Docker Remote API and the --tty
option to docker run.
*
*
* @param pseudoTerminal
* When this parameter is true
, a TTY is allocated. This parameter maps to Tty
in
* the Create a container
* section of the Docker Remote API and the
* --tty
option to docker run.
*/
public void setPseudoTerminal(Boolean pseudoTerminal) {
this.pseudoTerminal = pseudoTerminal;
}
/**
*
* When this parameter is true
, a TTY is allocated. This parameter maps to Tty
in the Create a container section of the
* Docker Remote API and the --tty
option to docker run.
*
*
* @return When this parameter is true
, a TTY is allocated. This parameter maps to Tty
in
* the Create a container
* section of the Docker Remote API and the
* --tty
option to docker run.
*/
public Boolean getPseudoTerminal() {
return this.pseudoTerminal;
}
/**
*
* When this parameter is true
, a TTY is allocated. This parameter maps to Tty
in the Create a container section of the
* Docker Remote API and the --tty
option to docker run.
*
*
* @param pseudoTerminal
* When this parameter is true
, a TTY is allocated. This parameter maps to Tty
in
* the Create a container
* section of the Docker Remote API and the
* --tty
option to docker run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withPseudoTerminal(Boolean pseudoTerminal) {
setPseudoTerminal(pseudoTerminal);
return this;
}
/**
*
* When this parameter is true
, a TTY is allocated. This parameter maps to Tty
in the Create a container section of the
* Docker Remote API and the --tty
option to docker run.
*
*
* @return When this parameter is true
, a TTY is allocated. This parameter maps to Tty
in
* the Create a container
* section of the Docker Remote API and the
* --tty
option to docker run.
*/
public Boolean isPseudoTerminal() {
return this.pseudoTerminal;
}
/**
*
* A key/value map of labels to add to the container. This parameter maps to Labels
in the Create a container section of the
* Docker Remote API and the --label
option to
* docker run. This parameter
* requires version 1.18 of the Docker Remote API or greater on your container instance. To check the Docker Remote
* API version on your container instance, log in to your container instance and run the following command:
* sudo docker version --format '{{.Server.APIVersion}}'
*
*
* @return A key/value map of labels to add to the container. This parameter maps to Labels
in the Create a container section
* of the Docker Remote API and the
* --label
option to docker run. This
* parameter requires version 1.18 of the Docker Remote API or greater on your container instance. To check
* the Docker Remote API version on your container instance, log in to your container instance and run the
* following command: sudo docker version --format '{{.Server.APIVersion}}'
*/
public java.util.Map getDockerLabels() {
return dockerLabels;
}
/**
*
* A key/value map of labels to add to the container. This parameter maps to Labels
in the Create a container section of the
* Docker Remote API and the --label
option to
* docker run. This parameter
* requires version 1.18 of the Docker Remote API or greater on your container instance. To check the Docker Remote
* API version on your container instance, log in to your container instance and run the following command:
* sudo docker version --format '{{.Server.APIVersion}}'
*
*
* @param dockerLabels
* A key/value map of labels to add to the container. This parameter maps to Labels
in the Create a container section
* of the Docker Remote API and the
* --label
option to docker run. This parameter
* requires version 1.18 of the Docker Remote API or greater on your container instance. To check the Docker
* Remote API version on your container instance, log in to your container instance and run the following
* command: sudo docker version --format '{{.Server.APIVersion}}'
*/
public void setDockerLabels(java.util.Map dockerLabels) {
this.dockerLabels = dockerLabels;
}
/**
*
* A key/value map of labels to add to the container. This parameter maps to Labels
in the Create a container section of the
* Docker Remote API and the --label
option to
* docker run. This parameter
* requires version 1.18 of the Docker Remote API or greater on your container instance. To check the Docker Remote
* API version on your container instance, log in to your container instance and run the following command:
* sudo docker version --format '{{.Server.APIVersion}}'
*
*
* @param dockerLabels
* A key/value map of labels to add to the container. This parameter maps to Labels
in the Create a container section
* of the Docker Remote API and the
* --label
option to docker run. This parameter
* requires version 1.18 of the Docker Remote API or greater on your container instance. To check the Docker
* Remote API version on your container instance, log in to your container instance and run the following
* command: sudo docker version --format '{{.Server.APIVersion}}'
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withDockerLabels(java.util.Map dockerLabels) {
setDockerLabels(dockerLabels);
return this;
}
/**
* Add a single DockerLabels entry
*
* @see ContainerDefinition#withDockerLabels
* @returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition addDockerLabelsEntry(String key, String value) {
if (null == this.dockerLabels) {
this.dockerLabels = new java.util.HashMap();
}
if (this.dockerLabels.containsKey(key))
throw new IllegalArgumentException("Duplicated keys (" + key.toString() + ") are provided.");
this.dockerLabels.put(key, value);
return this;
}
/**
* Removes all the entries added into DockerLabels.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition clearDockerLabelsEntries() {
this.dockerLabels = null;
return this;
}
/**
*
* A list of ulimits
to set in the container. If a ulimit
value is specified in a task
* definition, it overrides the default values set by Docker. This parameter maps to Ulimits
in the Create a container section of the
* Docker Remote API and the --ulimit
option to
* docker run. Valid naming
* values are displayed in the Ulimit data type.
*
*
* Amazon ECS tasks hosted on Fargate use the default resource limit values set by the operating system with the
* exception of the nofile
resource limit parameter which Fargate overrides. The nofile
* resource limit sets a restriction on the number of open files that a container can use. The default
* nofile
soft limit is 1024
and the default hard limit is 4096
.
*
*
* This parameter requires version 1.18 of the Docker Remote API or greater on your container instance. To check the
* Docker Remote API version on your container instance, log in to your container instance and run the following
* command: sudo docker version --format '{{.Server.APIVersion}}'
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* @return A list of ulimits
to set in the container. If a ulimit
value is specified in a
* task definition, it overrides the default values set by Docker. This parameter maps to
* Ulimits
in the Create a container section
* of the Docker Remote API and the
* --ulimit
option to docker run. Valid naming
* values are displayed in the Ulimit data type.
*
* Amazon ECS tasks hosted on Fargate use the default resource limit values set by the operating system with
* the exception of the nofile
resource limit parameter which Fargate overrides. The
* nofile
resource limit sets a restriction on the number of open files that a container can
* use. The default nofile
soft limit is 1024
and the default hard limit is
* 4096
.
*
*
* This parameter requires version 1.18 of the Docker Remote API or greater on your container instance. To
* check the Docker Remote API version on your container instance, log in to your container instance and run
* the following command: sudo docker version --format '{{.Server.APIVersion}}'
*
*
*
* This parameter is not supported for Windows containers.
*
*/
public java.util.List getUlimits() {
if (ulimits == null) {
ulimits = new com.amazonaws.internal.SdkInternalList();
}
return ulimits;
}
/**
*
* A list of ulimits
to set in the container. If a ulimit
value is specified in a task
* definition, it overrides the default values set by Docker. This parameter maps to Ulimits
in the Create a container section of the
* Docker Remote API and the --ulimit
option to
* docker run. Valid naming
* values are displayed in the Ulimit data type.
*
*
* Amazon ECS tasks hosted on Fargate use the default resource limit values set by the operating system with the
* exception of the nofile
resource limit parameter which Fargate overrides. The nofile
* resource limit sets a restriction on the number of open files that a container can use. The default
* nofile
soft limit is 1024
and the default hard limit is 4096
.
*
*
* This parameter requires version 1.18 of the Docker Remote API or greater on your container instance. To check the
* Docker Remote API version on your container instance, log in to your container instance and run the following
* command: sudo docker version --format '{{.Server.APIVersion}}'
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* @param ulimits
* A list of ulimits
to set in the container. If a ulimit
value is specified in a
* task definition, it overrides the default values set by Docker. This parameter maps to
* Ulimits
in the Create a container section
* of the Docker Remote API and the
* --ulimit
option to docker run. Valid naming
* values are displayed in the Ulimit data type.
*
* Amazon ECS tasks hosted on Fargate use the default resource limit values set by the operating system with
* the exception of the nofile
resource limit parameter which Fargate overrides. The
* nofile
resource limit sets a restriction on the number of open files that a container can
* use. The default nofile
soft limit is 1024
and the default hard limit is
* 4096
.
*
*
* This parameter requires version 1.18 of the Docker Remote API or greater on your container instance. To
* check the Docker Remote API version on your container instance, log in to your container instance and run
* the following command: sudo docker version --format '{{.Server.APIVersion}}'
*
*
*
* This parameter is not supported for Windows containers.
*
*/
public void setUlimits(java.util.Collection ulimits) {
if (ulimits == null) {
this.ulimits = null;
return;
}
this.ulimits = new com.amazonaws.internal.SdkInternalList(ulimits);
}
/**
*
* A list of ulimits
to set in the container. If a ulimit
value is specified in a task
* definition, it overrides the default values set by Docker. This parameter maps to Ulimits
in the Create a container section of the
* Docker Remote API and the --ulimit
option to
* docker run. Valid naming
* values are displayed in the Ulimit data type.
*
*
* Amazon ECS tasks hosted on Fargate use the default resource limit values set by the operating system with the
* exception of the nofile
resource limit parameter which Fargate overrides. The nofile
* resource limit sets a restriction on the number of open files that a container can use. The default
* nofile
soft limit is 1024
and the default hard limit is 4096
.
*
*
* This parameter requires version 1.18 of the Docker Remote API or greater on your container instance. To check the
* Docker Remote API version on your container instance, log in to your container instance and run the following
* command: sudo docker version --format '{{.Server.APIVersion}}'
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setUlimits(java.util.Collection)} or {@link #withUlimits(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param ulimits
* A list of ulimits
to set in the container. If a ulimit
value is specified in a
* task definition, it overrides the default values set by Docker. This parameter maps to
* Ulimits
in the Create a container section
* of the Docker Remote API and the
* --ulimit
option to docker run. Valid naming
* values are displayed in the Ulimit data type.
*
* Amazon ECS tasks hosted on Fargate use the default resource limit values set by the operating system with
* the exception of the nofile
resource limit parameter which Fargate overrides. The
* nofile
resource limit sets a restriction on the number of open files that a container can
* use. The default nofile
soft limit is 1024
and the default hard limit is
* 4096
.
*
*
* This parameter requires version 1.18 of the Docker Remote API or greater on your container instance. To
* check the Docker Remote API version on your container instance, log in to your container instance and run
* the following command: sudo docker version --format '{{.Server.APIVersion}}'
*
*
*
* This parameter is not supported for Windows containers.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withUlimits(Ulimit... ulimits) {
if (this.ulimits == null) {
setUlimits(new com.amazonaws.internal.SdkInternalList(ulimits.length));
}
for (Ulimit ele : ulimits) {
this.ulimits.add(ele);
}
return this;
}
/**
*
* A list of ulimits
to set in the container. If a ulimit
value is specified in a task
* definition, it overrides the default values set by Docker. This parameter maps to Ulimits
in the Create a container section of the
* Docker Remote API and the --ulimit
option to
* docker run. Valid naming
* values are displayed in the Ulimit data type.
*
*
* Amazon ECS tasks hosted on Fargate use the default resource limit values set by the operating system with the
* exception of the nofile
resource limit parameter which Fargate overrides. The nofile
* resource limit sets a restriction on the number of open files that a container can use. The default
* nofile
soft limit is 1024
and the default hard limit is 4096
.
*
*
* This parameter requires version 1.18 of the Docker Remote API or greater on your container instance. To check the
* Docker Remote API version on your container instance, log in to your container instance and run the following
* command: sudo docker version --format '{{.Server.APIVersion}}'
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* @param ulimits
* A list of ulimits
to set in the container. If a ulimit
value is specified in a
* task definition, it overrides the default values set by Docker. This parameter maps to
* Ulimits
in the Create a container section
* of the Docker Remote API and the
* --ulimit
option to docker run. Valid naming
* values are displayed in the Ulimit data type.
*
* Amazon ECS tasks hosted on Fargate use the default resource limit values set by the operating system with
* the exception of the nofile
resource limit parameter which Fargate overrides. The
* nofile
resource limit sets a restriction on the number of open files that a container can
* use. The default nofile
soft limit is 1024
and the default hard limit is
* 4096
.
*
*
* This parameter requires version 1.18 of the Docker Remote API or greater on your container instance. To
* check the Docker Remote API version on your container instance, log in to your container instance and run
* the following command: sudo docker version --format '{{.Server.APIVersion}}'
*
*
*
* This parameter is not supported for Windows containers.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withUlimits(java.util.Collection ulimits) {
setUlimits(ulimits);
return this;
}
/**
*
* The log configuration specification for the container.
*
*
* This parameter maps to LogConfig
in the Create a container section of the
* Docker Remote API and the --log-driver
* option to docker run. By
* default, containers use the same logging driver that the Docker daemon uses. However the container can use a
* different logging driver than the Docker daemon by specifying a log driver with this parameter in the container
* definition. To use a different logging driver for a container, the log system must be configured properly on the
* container instance (or on a different log server for remote logging options). For more information about the
* options for different supported log drivers, see Configure logging drivers in the Docker
* documentation.
*
*
*
* Amazon ECS currently supports a subset of the logging drivers available to the Docker daemon (shown in the
* LogConfiguration data type). Additional log drivers may be available in future releases of the Amazon ECS
* container agent.
*
*
*
* This parameter requires version 1.18 of the Docker Remote API or greater on your container instance. To check the
* Docker Remote API version on your container instance, log in to your container instance and run the following
* command: sudo docker version --format '{{.Server.APIVersion}}'
*
*
*
* The Amazon ECS container agent running on a container instance must register the logging drivers available on
* that instance with the ECS_AVAILABLE_LOGGING_DRIVERS
environment variable before containers placed
* on that instance can use these log configuration options. For more information, see Amazon ECS Container
* Agent Configuration in the Amazon Elastic Container Service Developer Guide.
*
*
*
* @param logConfiguration
* The log configuration specification for the container.
*
* This parameter maps to LogConfig
in the Create a container section
* of the Docker Remote API and the
* --log-driver
option to docker run. By default,
* containers use the same logging driver that the Docker daemon uses. However the container can use a
* different logging driver than the Docker daemon by specifying a log driver with this parameter in the
* container definition. To use a different logging driver for a container, the log system must be configured
* properly on the container instance (or on a different log server for remote logging options). For more
* information about the options for different supported log drivers, see Configure logging drivers in the Docker
* documentation.
*
*
*
* Amazon ECS currently supports a subset of the logging drivers available to the Docker daemon (shown in the
* LogConfiguration data type). Additional log drivers may be available in future releases of the
* Amazon ECS container agent.
*
*
*
* This parameter requires version 1.18 of the Docker Remote API or greater on your container instance. To
* check the Docker Remote API version on your container instance, log in to your container instance and run
* the following command: sudo docker version --format '{{.Server.APIVersion}}'
*
*
*
* The Amazon ECS container agent running on a container instance must register the logging drivers available
* on that instance with the ECS_AVAILABLE_LOGGING_DRIVERS
environment variable before
* containers placed on that instance can use these log configuration options. For more information, see Amazon ECS
* Container Agent Configuration in the Amazon Elastic Container Service Developer Guide.
*
*/
public void setLogConfiguration(LogConfiguration logConfiguration) {
this.logConfiguration = logConfiguration;
}
/**
*
* The log configuration specification for the container.
*
*
* This parameter maps to LogConfig
in the Create a container section of the
* Docker Remote API and the --log-driver
* option to docker run. By
* default, containers use the same logging driver that the Docker daemon uses. However the container can use a
* different logging driver than the Docker daemon by specifying a log driver with this parameter in the container
* definition. To use a different logging driver for a container, the log system must be configured properly on the
* container instance (or on a different log server for remote logging options). For more information about the
* options for different supported log drivers, see Configure logging drivers in the Docker
* documentation.
*
*
*
* Amazon ECS currently supports a subset of the logging drivers available to the Docker daemon (shown in the
* LogConfiguration data type). Additional log drivers may be available in future releases of the Amazon ECS
* container agent.
*
*
*
* This parameter requires version 1.18 of the Docker Remote API or greater on your container instance. To check the
* Docker Remote API version on your container instance, log in to your container instance and run the following
* command: sudo docker version --format '{{.Server.APIVersion}}'
*
*
*
* The Amazon ECS container agent running on a container instance must register the logging drivers available on
* that instance with the ECS_AVAILABLE_LOGGING_DRIVERS
environment variable before containers placed
* on that instance can use these log configuration options. For more information, see Amazon ECS Container
* Agent Configuration in the Amazon Elastic Container Service Developer Guide.
*
*
*
* @return The log configuration specification for the container.
*
* This parameter maps to LogConfig
in the Create a container section
* of the Docker Remote API and the
* --log-driver
option to docker run. By default,
* containers use the same logging driver that the Docker daemon uses. However the container can use a
* different logging driver than the Docker daemon by specifying a log driver with this parameter in the
* container definition. To use a different logging driver for a container, the log system must be
* configured properly on the container instance (or on a different log server for remote logging options).
* For more information about the options for different supported log drivers, see Configure logging drivers in the Docker
* documentation.
*
*
*
* Amazon ECS currently supports a subset of the logging drivers available to the Docker daemon (shown in
* the LogConfiguration data type). Additional log drivers may be available in future releases of the
* Amazon ECS container agent.
*
*
*
* This parameter requires version 1.18 of the Docker Remote API or greater on your container instance. To
* check the Docker Remote API version on your container instance, log in to your container instance and run
* the following command: sudo docker version --format '{{.Server.APIVersion}}'
*
*
*
* The Amazon ECS container agent running on a container instance must register the logging drivers
* available on that instance with the ECS_AVAILABLE_LOGGING_DRIVERS
environment variable
* before containers placed on that instance can use these log configuration options. For more information,
* see Amazon
* ECS Container Agent Configuration in the Amazon Elastic Container Service Developer Guide.
*
*/
public LogConfiguration getLogConfiguration() {
return this.logConfiguration;
}
/**
*
* The log configuration specification for the container.
*
*
* This parameter maps to LogConfig
in the Create a container section of the
* Docker Remote API and the --log-driver
* option to docker run. By
* default, containers use the same logging driver that the Docker daemon uses. However the container can use a
* different logging driver than the Docker daemon by specifying a log driver with this parameter in the container
* definition. To use a different logging driver for a container, the log system must be configured properly on the
* container instance (or on a different log server for remote logging options). For more information about the
* options for different supported log drivers, see Configure logging drivers in the Docker
* documentation.
*
*
*
* Amazon ECS currently supports a subset of the logging drivers available to the Docker daemon (shown in the
* LogConfiguration data type). Additional log drivers may be available in future releases of the Amazon ECS
* container agent.
*
*
*
* This parameter requires version 1.18 of the Docker Remote API or greater on your container instance. To check the
* Docker Remote API version on your container instance, log in to your container instance and run the following
* command: sudo docker version --format '{{.Server.APIVersion}}'
*
*
*
* The Amazon ECS container agent running on a container instance must register the logging drivers available on
* that instance with the ECS_AVAILABLE_LOGGING_DRIVERS
environment variable before containers placed
* on that instance can use these log configuration options. For more information, see Amazon ECS Container
* Agent Configuration in the Amazon Elastic Container Service Developer Guide.
*
*
*
* @param logConfiguration
* The log configuration specification for the container.
*
* This parameter maps to LogConfig
in the Create a container section
* of the Docker Remote API and the
* --log-driver
option to docker run. By default,
* containers use the same logging driver that the Docker daemon uses. However the container can use a
* different logging driver than the Docker daemon by specifying a log driver with this parameter in the
* container definition. To use a different logging driver for a container, the log system must be configured
* properly on the container instance (or on a different log server for remote logging options). For more
* information about the options for different supported log drivers, see Configure logging drivers in the Docker
* documentation.
*
*
*
* Amazon ECS currently supports a subset of the logging drivers available to the Docker daemon (shown in the
* LogConfiguration data type). Additional log drivers may be available in future releases of the
* Amazon ECS container agent.
*
*
*
* This parameter requires version 1.18 of the Docker Remote API or greater on your container instance. To
* check the Docker Remote API version on your container instance, log in to your container instance and run
* the following command: sudo docker version --format '{{.Server.APIVersion}}'
*
*
*
* The Amazon ECS container agent running on a container instance must register the logging drivers available
* on that instance with the ECS_AVAILABLE_LOGGING_DRIVERS
environment variable before
* containers placed on that instance can use these log configuration options. For more information, see Amazon ECS
* Container Agent Configuration in the Amazon Elastic Container Service Developer Guide.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withLogConfiguration(LogConfiguration logConfiguration) {
setLogConfiguration(logConfiguration);
return this;
}
/**
*
* The container health check command and associated configuration parameters for the container. This parameter maps
* to HealthCheck
in the Create a container section of the
* Docker Remote API and the HEALTHCHECK
* parameter of docker run.
*
*
* @param healthCheck
* The container health check command and associated configuration parameters for the container. This
* parameter maps to HealthCheck
in the Create a container section
* of the Docker Remote API and the
* HEALTHCHECK
parameter of docker run.
*/
public void setHealthCheck(HealthCheck healthCheck) {
this.healthCheck = healthCheck;
}
/**
*
* The container health check command and associated configuration parameters for the container. This parameter maps
* to HealthCheck
in the Create a container section of the
* Docker Remote API and the HEALTHCHECK
* parameter of docker run.
*
*
* @return The container health check command and associated configuration parameters for the container. This
* parameter maps to HealthCheck
in the Create a container section
* of the Docker Remote API and the
* HEALTHCHECK
parameter of docker run.
*/
public HealthCheck getHealthCheck() {
return this.healthCheck;
}
/**
*
* The container health check command and associated configuration parameters for the container. This parameter maps
* to HealthCheck
in the Create a container section of the
* Docker Remote API and the HEALTHCHECK
* parameter of docker run.
*
*
* @param healthCheck
* The container health check command and associated configuration parameters for the container. This
* parameter maps to HealthCheck
in the Create a container section
* of the Docker Remote API and the
* HEALTHCHECK
parameter of docker run.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withHealthCheck(HealthCheck healthCheck) {
setHealthCheck(healthCheck);
return this;
}
/**
*
* A list of namespaced kernel parameters to set in the container. This parameter maps to Sysctls
in
* the Create a container section
* of the Docker Remote API and the --sysctl
* option to docker run. For
* example, you can configure net.ipv4.tcp_keepalive_time
setting to maintain longer lived connections.
*
*
*
* We don't recommended that you specify network-related systemControls
parameters for multiple
* containers in a single task that also uses either the awsvpc
or host
network modes. For
* tasks that use the awsvpc
network mode, the container that's started last determines which
* systemControls
parameters take effect. For tasks that use the host
network mode, it
* changes the container instance's namespaced kernel parameters as well as the containers.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* This parameter is only supported for tasks that are hosted on Fargate if the tasks are using platform version
* 1.4.0
or later (Linux). This isn't supported for Windows containers on Fargate.
*
*
*
* @return A list of namespaced kernel parameters to set in the container. This parameter maps to
* Sysctls
in the Create a container section
* of the Docker Remote API and the
* --sysctl
option to docker run. For example,
* you can configure net.ipv4.tcp_keepalive_time
setting to maintain longer lived
* connections.
*
* We don't recommended that you specify network-related systemControls
parameters for multiple
* containers in a single task that also uses either the awsvpc
or host
network
* modes. For tasks that use the awsvpc
network mode, the container that's started last
* determines which systemControls
parameters take effect. For tasks that use the
* host
network mode, it changes the container instance's namespaced kernel parameters as well
* as the containers.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* This parameter is only supported for tasks that are hosted on Fargate if the tasks are using platform
* version 1.4.0
or later (Linux). This isn't supported for Windows containers on Fargate.
*
*/
public java.util.List getSystemControls() {
if (systemControls == null) {
systemControls = new com.amazonaws.internal.SdkInternalList();
}
return systemControls;
}
/**
*
* A list of namespaced kernel parameters to set in the container. This parameter maps to Sysctls
in
* the Create a container section
* of the Docker Remote API and the --sysctl
* option to docker run. For
* example, you can configure net.ipv4.tcp_keepalive_time
setting to maintain longer lived connections.
*
*
*
* We don't recommended that you specify network-related systemControls
parameters for multiple
* containers in a single task that also uses either the awsvpc
or host
network modes. For
* tasks that use the awsvpc
network mode, the container that's started last determines which
* systemControls
parameters take effect. For tasks that use the host
network mode, it
* changes the container instance's namespaced kernel parameters as well as the containers.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* This parameter is only supported for tasks that are hosted on Fargate if the tasks are using platform version
* 1.4.0
or later (Linux). This isn't supported for Windows containers on Fargate.
*
*
*
* @param systemControls
* A list of namespaced kernel parameters to set in the container. This parameter maps to
* Sysctls
in the Create a container section
* of the Docker Remote API and the
* --sysctl
option to docker run. For example,
* you can configure net.ipv4.tcp_keepalive_time
setting to maintain longer lived
* connections.
*
* We don't recommended that you specify network-related systemControls
parameters for multiple
* containers in a single task that also uses either the awsvpc
or host
network
* modes. For tasks that use the awsvpc
network mode, the container that's started last
* determines which systemControls
parameters take effect. For tasks that use the
* host
network mode, it changes the container instance's namespaced kernel parameters as well
* as the containers.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* This parameter is only supported for tasks that are hosted on Fargate if the tasks are using platform
* version 1.4.0
or later (Linux). This isn't supported for Windows containers on Fargate.
*
*/
public void setSystemControls(java.util.Collection systemControls) {
if (systemControls == null) {
this.systemControls = null;
return;
}
this.systemControls = new com.amazonaws.internal.SdkInternalList(systemControls);
}
/**
*
* A list of namespaced kernel parameters to set in the container. This parameter maps to Sysctls
in
* the Create a container section
* of the Docker Remote API and the --sysctl
* option to docker run. For
* example, you can configure net.ipv4.tcp_keepalive_time
setting to maintain longer lived connections.
*
*
*
* We don't recommended that you specify network-related systemControls
parameters for multiple
* containers in a single task that also uses either the awsvpc
or host
network modes. For
* tasks that use the awsvpc
network mode, the container that's started last determines which
* systemControls
parameters take effect. For tasks that use the host
network mode, it
* changes the container instance's namespaced kernel parameters as well as the containers.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* This parameter is only supported for tasks that are hosted on Fargate if the tasks are using platform version
* 1.4.0
or later (Linux). This isn't supported for Windows containers on Fargate.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setSystemControls(java.util.Collection)} or {@link #withSystemControls(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param systemControls
* A list of namespaced kernel parameters to set in the container. This parameter maps to
* Sysctls
in the Create a container section
* of the Docker Remote API and the
* --sysctl
option to docker run. For example,
* you can configure net.ipv4.tcp_keepalive_time
setting to maintain longer lived
* connections.
*
* We don't recommended that you specify network-related systemControls
parameters for multiple
* containers in a single task that also uses either the awsvpc
or host
network
* modes. For tasks that use the awsvpc
network mode, the container that's started last
* determines which systemControls
parameters take effect. For tasks that use the
* host
network mode, it changes the container instance's namespaced kernel parameters as well
* as the containers.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* This parameter is only supported for tasks that are hosted on Fargate if the tasks are using platform
* version 1.4.0
or later (Linux). This isn't supported for Windows containers on Fargate.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withSystemControls(SystemControl... systemControls) {
if (this.systemControls == null) {
setSystemControls(new com.amazonaws.internal.SdkInternalList(systemControls.length));
}
for (SystemControl ele : systemControls) {
this.systemControls.add(ele);
}
return this;
}
/**
*
* A list of namespaced kernel parameters to set in the container. This parameter maps to Sysctls
in
* the Create a container section
* of the Docker Remote API and the --sysctl
* option to docker run. For
* example, you can configure net.ipv4.tcp_keepalive_time
setting to maintain longer lived connections.
*
*
*
* We don't recommended that you specify network-related systemControls
parameters for multiple
* containers in a single task that also uses either the awsvpc
or host
network modes. For
* tasks that use the awsvpc
network mode, the container that's started last determines which
* systemControls
parameters take effect. For tasks that use the host
network mode, it
* changes the container instance's namespaced kernel parameters as well as the containers.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* This parameter is only supported for tasks that are hosted on Fargate if the tasks are using platform version
* 1.4.0
or later (Linux). This isn't supported for Windows containers on Fargate.
*
*
*
* @param systemControls
* A list of namespaced kernel parameters to set in the container. This parameter maps to
* Sysctls
in the Create a container section
* of the Docker Remote API and the
* --sysctl
option to docker run. For example,
* you can configure net.ipv4.tcp_keepalive_time
setting to maintain longer lived
* connections.
*
* We don't recommended that you specify network-related systemControls
parameters for multiple
* containers in a single task that also uses either the awsvpc
or host
network
* modes. For tasks that use the awsvpc
network mode, the container that's started last
* determines which systemControls
parameters take effect. For tasks that use the
* host
network mode, it changes the container instance's namespaced kernel parameters as well
* as the containers.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* This parameter is only supported for tasks that are hosted on Fargate if the tasks are using platform
* version 1.4.0
or later (Linux). This isn't supported for Windows containers on Fargate.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withSystemControls(java.util.Collection systemControls) {
setSystemControls(systemControls);
return this;
}
/**
*
* The type and amount of a resource to assign to a container. The only supported resource is a GPU.
*
*
* @return The type and amount of a resource to assign to a container. The only supported resource is a GPU.
*/
public java.util.List getResourceRequirements() {
if (resourceRequirements == null) {
resourceRequirements = new com.amazonaws.internal.SdkInternalList();
}
return resourceRequirements;
}
/**
*
* The type and amount of a resource to assign to a container. The only supported resource is a GPU.
*
*
* @param resourceRequirements
* The type and amount of a resource to assign to a container. The only supported resource is a GPU.
*/
public void setResourceRequirements(java.util.Collection resourceRequirements) {
if (resourceRequirements == null) {
this.resourceRequirements = null;
return;
}
this.resourceRequirements = new com.amazonaws.internal.SdkInternalList(resourceRequirements);
}
/**
*
* The type and amount of a resource to assign to a container. The only supported resource is a GPU.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setResourceRequirements(java.util.Collection)} or {@link #withResourceRequirements(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param resourceRequirements
* The type and amount of a resource to assign to a container. The only supported resource is a GPU.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withResourceRequirements(ResourceRequirement... resourceRequirements) {
if (this.resourceRequirements == null) {
setResourceRequirements(new com.amazonaws.internal.SdkInternalList(resourceRequirements.length));
}
for (ResourceRequirement ele : resourceRequirements) {
this.resourceRequirements.add(ele);
}
return this;
}
/**
*
* The type and amount of a resource to assign to a container. The only supported resource is a GPU.
*
*
* @param resourceRequirements
* The type and amount of a resource to assign to a container. The only supported resource is a GPU.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withResourceRequirements(java.util.Collection resourceRequirements) {
setResourceRequirements(resourceRequirements);
return this;
}
/**
*
* The FireLens configuration for the container. This is used to specify and configure a log router for container
* logs. For more information, see Custom Log Routing in
* the Amazon Elastic Container Service Developer Guide.
*
*
* @param firelensConfiguration
* The FireLens configuration for the container. This is used to specify and configure a log router for
* container logs. For more information, see Custom Log
* Routing in the Amazon Elastic Container Service Developer Guide.
*/
public void setFirelensConfiguration(FirelensConfiguration firelensConfiguration) {
this.firelensConfiguration = firelensConfiguration;
}
/**
*
* The FireLens configuration for the container. This is used to specify and configure a log router for container
* logs. For more information, see Custom Log Routing in
* the Amazon Elastic Container Service Developer Guide.
*
*
* @return The FireLens configuration for the container. This is used to specify and configure a log router for
* container logs. For more information, see Custom Log
* Routing in the Amazon Elastic Container Service Developer Guide.
*/
public FirelensConfiguration getFirelensConfiguration() {
return this.firelensConfiguration;
}
/**
*
* The FireLens configuration for the container. This is used to specify and configure a log router for container
* logs. For more information, see Custom Log Routing in
* the Amazon Elastic Container Service Developer Guide.
*
*
* @param firelensConfiguration
* The FireLens configuration for the container. This is used to specify and configure a log router for
* container logs. For more information, see Custom Log
* Routing in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withFirelensConfiguration(FirelensConfiguration firelensConfiguration) {
setFirelensConfiguration(firelensConfiguration);
return this;
}
/**
*
* A list of ARNs in SSM or Amazon S3 to a credential spec (CredSpec
) file that configures the
* container for Active Directory authentication. We recommend that you use this parameter instead of the
* dockerSecurityOptions
. The maximum number of ARNs is 1.
*
*
* There are two formats for each ARN.
*
*
* - credentialspecdomainless:MyARN
* -
*
* You use credentialspecdomainless:MyARN
to provide a CredSpec
with an additional section
* for a secret in Secrets Manager. You provide the login credentials to the domain in the secret.
*
*
* Each task that runs on any container instance can join different domains.
*
*
* You can use this format without joining the container instance to a domain.
*
*
* - credentialspec:MyARN
* -
*
* You use credentialspec:MyARN
to provide a CredSpec
for a single domain.
*
*
* You must join the container instance to the domain before you start any tasks that use this task definition.
*
*
*
*
* In both formats, replace MyARN
with the ARN in SSM or Amazon S3.
*
*
* If you provide a credentialspecdomainless:MyARN
, the credspec
must provide a ARN in
* Secrets Manager for a secret containing the username, password, and the domain to connect to. For better
* security, the instance isn't joined to the domain for domainless authentication. Other applications on the
* instance can't use the domainless credentials. You can use this parameter to run tasks on the same instance, even
* it the tasks need to join different domains. For more information, see Using gMSAs for Windows
* Containers and Using
* gMSAs for Linux Containers.
*
*
* @return A list of ARNs in SSM or Amazon S3 to a credential spec (CredSpec
) file that configures the
* container for Active Directory authentication. We recommend that you use this parameter instead of the
* dockerSecurityOptions
. The maximum number of ARNs is 1.
*
* There are two formats for each ARN.
*
*
* - credentialspecdomainless:MyARN
* -
*
* You use credentialspecdomainless:MyARN
to provide a CredSpec
with an additional
* section for a secret in Secrets Manager. You provide the login credentials to the domain in the secret.
*
*
* Each task that runs on any container instance can join different domains.
*
*
* You can use this format without joining the container instance to a domain.
*
*
* - credentialspec:MyARN
* -
*
* You use credentialspec:MyARN
to provide a CredSpec
for a single domain.
*
*
* You must join the container instance to the domain before you start any tasks that use this task
* definition.
*
*
*
*
* In both formats, replace MyARN
with the ARN in SSM or Amazon S3.
*
*
* If you provide a credentialspecdomainless:MyARN
, the credspec
must provide a
* ARN in Secrets Manager for a secret containing the username, password, and the domain to connect to. For
* better security, the instance isn't joined to the domain for domainless authentication. Other
* applications on the instance can't use the domainless credentials. You can use this parameter to run
* tasks on the same instance, even it the tasks need to join different domains. For more information, see
* Using gMSAs for
* Windows Containers and Using gMSAs for Linux
* Containers.
*/
public java.util.List getCredentialSpecs() {
if (credentialSpecs == null) {
credentialSpecs = new com.amazonaws.internal.SdkInternalList();
}
return credentialSpecs;
}
/**
*
* A list of ARNs in SSM or Amazon S3 to a credential spec (CredSpec
) file that configures the
* container for Active Directory authentication. We recommend that you use this parameter instead of the
* dockerSecurityOptions
. The maximum number of ARNs is 1.
*
*
* There are two formats for each ARN.
*
*
* - credentialspecdomainless:MyARN
* -
*
* You use credentialspecdomainless:MyARN
to provide a CredSpec
with an additional section
* for a secret in Secrets Manager. You provide the login credentials to the domain in the secret.
*
*
* Each task that runs on any container instance can join different domains.
*
*
* You can use this format without joining the container instance to a domain.
*
*
* - credentialspec:MyARN
* -
*
* You use credentialspec:MyARN
to provide a CredSpec
for a single domain.
*
*
* You must join the container instance to the domain before you start any tasks that use this task definition.
*
*
*
*
* In both formats, replace MyARN
with the ARN in SSM or Amazon S3.
*
*
* If you provide a credentialspecdomainless:MyARN
, the credspec
must provide a ARN in
* Secrets Manager for a secret containing the username, password, and the domain to connect to. For better
* security, the instance isn't joined to the domain for domainless authentication. Other applications on the
* instance can't use the domainless credentials. You can use this parameter to run tasks on the same instance, even
* it the tasks need to join different domains. For more information, see Using gMSAs for Windows
* Containers and Using
* gMSAs for Linux Containers.
*
*
* @param credentialSpecs
* A list of ARNs in SSM or Amazon S3 to a credential spec (CredSpec
) file that configures the
* container for Active Directory authentication. We recommend that you use this parameter instead of the
* dockerSecurityOptions
. The maximum number of ARNs is 1.
*
* There are two formats for each ARN.
*
*
* - credentialspecdomainless:MyARN
* -
*
* You use credentialspecdomainless:MyARN
to provide a CredSpec
with an additional
* section for a secret in Secrets Manager. You provide the login credentials to the domain in the secret.
*
*
* Each task that runs on any container instance can join different domains.
*
*
* You can use this format without joining the container instance to a domain.
*
*
* - credentialspec:MyARN
* -
*
* You use credentialspec:MyARN
to provide a CredSpec
for a single domain.
*
*
* You must join the container instance to the domain before you start any tasks that use this task
* definition.
*
*
*
*
* In both formats, replace MyARN
with the ARN in SSM or Amazon S3.
*
*
* If you provide a credentialspecdomainless:MyARN
, the credspec
must provide a ARN
* in Secrets Manager for a secret containing the username, password, and the domain to connect to. For
* better security, the instance isn't joined to the domain for domainless authentication. Other applications
* on the instance can't use the domainless credentials. You can use this parameter to run tasks on the same
* instance, even it the tasks need to join different domains. For more information, see Using gMSAs for
* Windows Containers and Using gMSAs for Linux
* Containers.
*/
public void setCredentialSpecs(java.util.Collection credentialSpecs) {
if (credentialSpecs == null) {
this.credentialSpecs = null;
return;
}
this.credentialSpecs = new com.amazonaws.internal.SdkInternalList(credentialSpecs);
}
/**
*
* A list of ARNs in SSM or Amazon S3 to a credential spec (CredSpec
) file that configures the
* container for Active Directory authentication. We recommend that you use this parameter instead of the
* dockerSecurityOptions
. The maximum number of ARNs is 1.
*
*
* There are two formats for each ARN.
*
*
* - credentialspecdomainless:MyARN
* -
*
* You use credentialspecdomainless:MyARN
to provide a CredSpec
with an additional section
* for a secret in Secrets Manager. You provide the login credentials to the domain in the secret.
*
*
* Each task that runs on any container instance can join different domains.
*
*
* You can use this format without joining the container instance to a domain.
*
*
* - credentialspec:MyARN
* -
*
* You use credentialspec:MyARN
to provide a CredSpec
for a single domain.
*
*
* You must join the container instance to the domain before you start any tasks that use this task definition.
*
*
*
*
* In both formats, replace MyARN
with the ARN in SSM or Amazon S3.
*
*
* If you provide a credentialspecdomainless:MyARN
, the credspec
must provide a ARN in
* Secrets Manager for a secret containing the username, password, and the domain to connect to. For better
* security, the instance isn't joined to the domain for domainless authentication. Other applications on the
* instance can't use the domainless credentials. You can use this parameter to run tasks on the same instance, even
* it the tasks need to join different domains. For more information, see Using gMSAs for Windows
* Containers and Using
* gMSAs for Linux Containers.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCredentialSpecs(java.util.Collection)} or {@link #withCredentialSpecs(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param credentialSpecs
* A list of ARNs in SSM or Amazon S3 to a credential spec (CredSpec
) file that configures the
* container for Active Directory authentication. We recommend that you use this parameter instead of the
* dockerSecurityOptions
. The maximum number of ARNs is 1.
*
* There are two formats for each ARN.
*
*
* - credentialspecdomainless:MyARN
* -
*
* You use credentialspecdomainless:MyARN
to provide a CredSpec
with an additional
* section for a secret in Secrets Manager. You provide the login credentials to the domain in the secret.
*
*
* Each task that runs on any container instance can join different domains.
*
*
* You can use this format without joining the container instance to a domain.
*
*
* - credentialspec:MyARN
* -
*
* You use credentialspec:MyARN
to provide a CredSpec
for a single domain.
*
*
* You must join the container instance to the domain before you start any tasks that use this task
* definition.
*
*
*
*
* In both formats, replace MyARN
with the ARN in SSM or Amazon S3.
*
*
* If you provide a credentialspecdomainless:MyARN
, the credspec
must provide a ARN
* in Secrets Manager for a secret containing the username, password, and the domain to connect to. For
* better security, the instance isn't joined to the domain for domainless authentication. Other applications
* on the instance can't use the domainless credentials. You can use this parameter to run tasks on the same
* instance, even it the tasks need to join different domains. For more information, see Using gMSAs for
* Windows Containers and Using gMSAs for Linux
* Containers.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withCredentialSpecs(String... credentialSpecs) {
if (this.credentialSpecs == null) {
setCredentialSpecs(new com.amazonaws.internal.SdkInternalList(credentialSpecs.length));
}
for (String ele : credentialSpecs) {
this.credentialSpecs.add(ele);
}
return this;
}
/**
*
* A list of ARNs in SSM or Amazon S3 to a credential spec (CredSpec
) file that configures the
* container for Active Directory authentication. We recommend that you use this parameter instead of the
* dockerSecurityOptions
. The maximum number of ARNs is 1.
*
*
* There are two formats for each ARN.
*
*
* - credentialspecdomainless:MyARN
* -
*
* You use credentialspecdomainless:MyARN
to provide a CredSpec
with an additional section
* for a secret in Secrets Manager. You provide the login credentials to the domain in the secret.
*
*
* Each task that runs on any container instance can join different domains.
*
*
* You can use this format without joining the container instance to a domain.
*
*
* - credentialspec:MyARN
* -
*
* You use credentialspec:MyARN
to provide a CredSpec
for a single domain.
*
*
* You must join the container instance to the domain before you start any tasks that use this task definition.
*
*
*
*
* In both formats, replace MyARN
with the ARN in SSM or Amazon S3.
*
*
* If you provide a credentialspecdomainless:MyARN
, the credspec
must provide a ARN in
* Secrets Manager for a secret containing the username, password, and the domain to connect to. For better
* security, the instance isn't joined to the domain for domainless authentication. Other applications on the
* instance can't use the domainless credentials. You can use this parameter to run tasks on the same instance, even
* it the tasks need to join different domains. For more information, see Using gMSAs for Windows
* Containers and Using
* gMSAs for Linux Containers.
*
*
* @param credentialSpecs
* A list of ARNs in SSM or Amazon S3 to a credential spec (CredSpec
) file that configures the
* container for Active Directory authentication. We recommend that you use this parameter instead of the
* dockerSecurityOptions
. The maximum number of ARNs is 1.
*
* There are two formats for each ARN.
*
*
* - credentialspecdomainless:MyARN
* -
*
* You use credentialspecdomainless:MyARN
to provide a CredSpec
with an additional
* section for a secret in Secrets Manager. You provide the login credentials to the domain in the secret.
*
*
* Each task that runs on any container instance can join different domains.
*
*
* You can use this format without joining the container instance to a domain.
*
*
* - credentialspec:MyARN
* -
*
* You use credentialspec:MyARN
to provide a CredSpec
for a single domain.
*
*
* You must join the container instance to the domain before you start any tasks that use this task
* definition.
*
*
*
*
* In both formats, replace MyARN
with the ARN in SSM or Amazon S3.
*
*
* If you provide a credentialspecdomainless:MyARN
, the credspec
must provide a ARN
* in Secrets Manager for a secret containing the username, password, and the domain to connect to. For
* better security, the instance isn't joined to the domain for domainless authentication. Other applications
* on the instance can't use the domainless credentials. You can use this parameter to run tasks on the same
* instance, even it the tasks need to join different domains. For more information, see Using gMSAs for
* Windows Containers and Using gMSAs for Linux
* Containers.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerDefinition withCredentialSpecs(java.util.Collection credentialSpecs) {
setCredentialSpecs(credentialSpecs);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getImage() != null)
sb.append("Image: ").append(getImage()).append(",");
if (getRepositoryCredentials() != null)
sb.append("RepositoryCredentials: ").append(getRepositoryCredentials()).append(",");
if (getCpu() != null)
sb.append("Cpu: ").append(getCpu()).append(",");
if (getMemory() != null)
sb.append("Memory: ").append(getMemory()).append(",");
if (getMemoryReservation() != null)
sb.append("MemoryReservation: ").append(getMemoryReservation()).append(",");
if (getLinks() != null)
sb.append("Links: ").append(getLinks()).append(",");
if (getPortMappings() != null)
sb.append("PortMappings: ").append(getPortMappings()).append(",");
if (getEssential() != null)
sb.append("Essential: ").append(getEssential()).append(",");
if (getEntryPoint() != null)
sb.append("EntryPoint: ").append(getEntryPoint()).append(",");
if (getCommand() != null)
sb.append("Command: ").append(getCommand()).append(",");
if (getEnvironment() != null)
sb.append("Environment: ").append(getEnvironment()).append(",");
if (getEnvironmentFiles() != null)
sb.append("EnvironmentFiles: ").append(getEnvironmentFiles()).append(",");
if (getMountPoints() != null)
sb.append("MountPoints: ").append(getMountPoints()).append(",");
if (getVolumesFrom() != null)
sb.append("VolumesFrom: ").append(getVolumesFrom()).append(",");
if (getLinuxParameters() != null)
sb.append("LinuxParameters: ").append(getLinuxParameters()).append(",");
if (getSecrets() != null)
sb.append("Secrets: ").append(getSecrets()).append(",");
if (getDependsOn() != null)
sb.append("DependsOn: ").append(getDependsOn()).append(",");
if (getStartTimeout() != null)
sb.append("StartTimeout: ").append(getStartTimeout()).append(",");
if (getStopTimeout() != null)
sb.append("StopTimeout: ").append(getStopTimeout()).append(",");
if (getHostname() != null)
sb.append("Hostname: ").append(getHostname()).append(",");
if (getUser() != null)
sb.append("User: ").append(getUser()).append(",");
if (getWorkingDirectory() != null)
sb.append("WorkingDirectory: ").append(getWorkingDirectory()).append(",");
if (getDisableNetworking() != null)
sb.append("DisableNetworking: ").append(getDisableNetworking()).append(",");
if (getPrivileged() != null)
sb.append("Privileged: ").append(getPrivileged()).append(",");
if (getReadonlyRootFilesystem() != null)
sb.append("ReadonlyRootFilesystem: ").append(getReadonlyRootFilesystem()).append(",");
if (getDnsServers() != null)
sb.append("DnsServers: ").append(getDnsServers()).append(",");
if (getDnsSearchDomains() != null)
sb.append("DnsSearchDomains: ").append(getDnsSearchDomains()).append(",");
if (getExtraHosts() != null)
sb.append("ExtraHosts: ").append(getExtraHosts()).append(",");
if (getDockerSecurityOptions() != null)
sb.append("DockerSecurityOptions: ").append(getDockerSecurityOptions()).append(",");
if (getInteractive() != null)
sb.append("Interactive: ").append(getInteractive()).append(",");
if (getPseudoTerminal() != null)
sb.append("PseudoTerminal: ").append(getPseudoTerminal()).append(",");
if (getDockerLabels() != null)
sb.append("DockerLabels: ").append(getDockerLabels()).append(",");
if (getUlimits() != null)
sb.append("Ulimits: ").append(getUlimits()).append(",");
if (getLogConfiguration() != null)
sb.append("LogConfiguration: ").append(getLogConfiguration()).append(",");
if (getHealthCheck() != null)
sb.append("HealthCheck: ").append(getHealthCheck()).append(",");
if (getSystemControls() != null)
sb.append("SystemControls: ").append(getSystemControls()).append(",");
if (getResourceRequirements() != null)
sb.append("ResourceRequirements: ").append(getResourceRequirements()).append(",");
if (getFirelensConfiguration() != null)
sb.append("FirelensConfiguration: ").append(getFirelensConfiguration()).append(",");
if (getCredentialSpecs() != null)
sb.append("CredentialSpecs: ").append(getCredentialSpecs());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ContainerDefinition == false)
return false;
ContainerDefinition other = (ContainerDefinition) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getImage() == null ^ this.getImage() == null)
return false;
if (other.getImage() != null && other.getImage().equals(this.getImage()) == false)
return false;
if (other.getRepositoryCredentials() == null ^ this.getRepositoryCredentials() == null)
return false;
if (other.getRepositoryCredentials() != null && other.getRepositoryCredentials().equals(this.getRepositoryCredentials()) == false)
return false;
if (other.getCpu() == null ^ this.getCpu() == null)
return false;
if (other.getCpu() != null && other.getCpu().equals(this.getCpu()) == false)
return false;
if (other.getMemory() == null ^ this.getMemory() == null)
return false;
if (other.getMemory() != null && other.getMemory().equals(this.getMemory()) == false)
return false;
if (other.getMemoryReservation() == null ^ this.getMemoryReservation() == null)
return false;
if (other.getMemoryReservation() != null && other.getMemoryReservation().equals(this.getMemoryReservation()) == false)
return false;
if (other.getLinks() == null ^ this.getLinks() == null)
return false;
if (other.getLinks() != null && other.getLinks().equals(this.getLinks()) == false)
return false;
if (other.getPortMappings() == null ^ this.getPortMappings() == null)
return false;
if (other.getPortMappings() != null && other.getPortMappings().equals(this.getPortMappings()) == false)
return false;
if (other.getEssential() == null ^ this.getEssential() == null)
return false;
if (other.getEssential() != null && other.getEssential().equals(this.getEssential()) == false)
return false;
if (other.getEntryPoint() == null ^ this.getEntryPoint() == null)
return false;
if (other.getEntryPoint() != null && other.getEntryPoint().equals(this.getEntryPoint()) == false)
return false;
if (other.getCommand() == null ^ this.getCommand() == null)
return false;
if (other.getCommand() != null && other.getCommand().equals(this.getCommand()) == false)
return false;
if (other.getEnvironment() == null ^ this.getEnvironment() == null)
return false;
if (other.getEnvironment() != null && other.getEnvironment().equals(this.getEnvironment()) == false)
return false;
if (other.getEnvironmentFiles() == null ^ this.getEnvironmentFiles() == null)
return false;
if (other.getEnvironmentFiles() != null && other.getEnvironmentFiles().equals(this.getEnvironmentFiles()) == false)
return false;
if (other.getMountPoints() == null ^ this.getMountPoints() == null)
return false;
if (other.getMountPoints() != null && other.getMountPoints().equals(this.getMountPoints()) == false)
return false;
if (other.getVolumesFrom() == null ^ this.getVolumesFrom() == null)
return false;
if (other.getVolumesFrom() != null && other.getVolumesFrom().equals(this.getVolumesFrom()) == false)
return false;
if (other.getLinuxParameters() == null ^ this.getLinuxParameters() == null)
return false;
if (other.getLinuxParameters() != null && other.getLinuxParameters().equals(this.getLinuxParameters()) == false)
return false;
if (other.getSecrets() == null ^ this.getSecrets() == null)
return false;
if (other.getSecrets() != null && other.getSecrets().equals(this.getSecrets()) == false)
return false;
if (other.getDependsOn() == null ^ this.getDependsOn() == null)
return false;
if (other.getDependsOn() != null && other.getDependsOn().equals(this.getDependsOn()) == false)
return false;
if (other.getStartTimeout() == null ^ this.getStartTimeout() == null)
return false;
if (other.getStartTimeout() != null && other.getStartTimeout().equals(this.getStartTimeout()) == false)
return false;
if (other.getStopTimeout() == null ^ this.getStopTimeout() == null)
return false;
if (other.getStopTimeout() != null && other.getStopTimeout().equals(this.getStopTimeout()) == false)
return false;
if (other.getHostname() == null ^ this.getHostname() == null)
return false;
if (other.getHostname() != null && other.getHostname().equals(this.getHostname()) == false)
return false;
if (other.getUser() == null ^ this.getUser() == null)
return false;
if (other.getUser() != null && other.getUser().equals(this.getUser()) == false)
return false;
if (other.getWorkingDirectory() == null ^ this.getWorkingDirectory() == null)
return false;
if (other.getWorkingDirectory() != null && other.getWorkingDirectory().equals(this.getWorkingDirectory()) == false)
return false;
if (other.getDisableNetworking() == null ^ this.getDisableNetworking() == null)
return false;
if (other.getDisableNetworking() != null && other.getDisableNetworking().equals(this.getDisableNetworking()) == false)
return false;
if (other.getPrivileged() == null ^ this.getPrivileged() == null)
return false;
if (other.getPrivileged() != null && other.getPrivileged().equals(this.getPrivileged()) == false)
return false;
if (other.getReadonlyRootFilesystem() == null ^ this.getReadonlyRootFilesystem() == null)
return false;
if (other.getReadonlyRootFilesystem() != null && other.getReadonlyRootFilesystem().equals(this.getReadonlyRootFilesystem()) == false)
return false;
if (other.getDnsServers() == null ^ this.getDnsServers() == null)
return false;
if (other.getDnsServers() != null && other.getDnsServers().equals(this.getDnsServers()) == false)
return false;
if (other.getDnsSearchDomains() == null ^ this.getDnsSearchDomains() == null)
return false;
if (other.getDnsSearchDomains() != null && other.getDnsSearchDomains().equals(this.getDnsSearchDomains()) == false)
return false;
if (other.getExtraHosts() == null ^ this.getExtraHosts() == null)
return false;
if (other.getExtraHosts() != null && other.getExtraHosts().equals(this.getExtraHosts()) == false)
return false;
if (other.getDockerSecurityOptions() == null ^ this.getDockerSecurityOptions() == null)
return false;
if (other.getDockerSecurityOptions() != null && other.getDockerSecurityOptions().equals(this.getDockerSecurityOptions()) == false)
return false;
if (other.getInteractive() == null ^ this.getInteractive() == null)
return false;
if (other.getInteractive() != null && other.getInteractive().equals(this.getInteractive()) == false)
return false;
if (other.getPseudoTerminal() == null ^ this.getPseudoTerminal() == null)
return false;
if (other.getPseudoTerminal() != null && other.getPseudoTerminal().equals(this.getPseudoTerminal()) == false)
return false;
if (other.getDockerLabels() == null ^ this.getDockerLabels() == null)
return false;
if (other.getDockerLabels() != null && other.getDockerLabels().equals(this.getDockerLabels()) == false)
return false;
if (other.getUlimits() == null ^ this.getUlimits() == null)
return false;
if (other.getUlimits() != null && other.getUlimits().equals(this.getUlimits()) == false)
return false;
if (other.getLogConfiguration() == null ^ this.getLogConfiguration() == null)
return false;
if (other.getLogConfiguration() != null && other.getLogConfiguration().equals(this.getLogConfiguration()) == false)
return false;
if (other.getHealthCheck() == null ^ this.getHealthCheck() == null)
return false;
if (other.getHealthCheck() != null && other.getHealthCheck().equals(this.getHealthCheck()) == false)
return false;
if (other.getSystemControls() == null ^ this.getSystemControls() == null)
return false;
if (other.getSystemControls() != null && other.getSystemControls().equals(this.getSystemControls()) == false)
return false;
if (other.getResourceRequirements() == null ^ this.getResourceRequirements() == null)
return false;
if (other.getResourceRequirements() != null && other.getResourceRequirements().equals(this.getResourceRequirements()) == false)
return false;
if (other.getFirelensConfiguration() == null ^ this.getFirelensConfiguration() == null)
return false;
if (other.getFirelensConfiguration() != null && other.getFirelensConfiguration().equals(this.getFirelensConfiguration()) == false)
return false;
if (other.getCredentialSpecs() == null ^ this.getCredentialSpecs() == null)
return false;
if (other.getCredentialSpecs() != null && other.getCredentialSpecs().equals(this.getCredentialSpecs()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getImage() == null) ? 0 : getImage().hashCode());
hashCode = prime * hashCode + ((getRepositoryCredentials() == null) ? 0 : getRepositoryCredentials().hashCode());
hashCode = prime * hashCode + ((getCpu() == null) ? 0 : getCpu().hashCode());
hashCode = prime * hashCode + ((getMemory() == null) ? 0 : getMemory().hashCode());
hashCode = prime * hashCode + ((getMemoryReservation() == null) ? 0 : getMemoryReservation().hashCode());
hashCode = prime * hashCode + ((getLinks() == null) ? 0 : getLinks().hashCode());
hashCode = prime * hashCode + ((getPortMappings() == null) ? 0 : getPortMappings().hashCode());
hashCode = prime * hashCode + ((getEssential() == null) ? 0 : getEssential().hashCode());
hashCode = prime * hashCode + ((getEntryPoint() == null) ? 0 : getEntryPoint().hashCode());
hashCode = prime * hashCode + ((getCommand() == null) ? 0 : getCommand().hashCode());
hashCode = prime * hashCode + ((getEnvironment() == null) ? 0 : getEnvironment().hashCode());
hashCode = prime * hashCode + ((getEnvironmentFiles() == null) ? 0 : getEnvironmentFiles().hashCode());
hashCode = prime * hashCode + ((getMountPoints() == null) ? 0 : getMountPoints().hashCode());
hashCode = prime * hashCode + ((getVolumesFrom() == null) ? 0 : getVolumesFrom().hashCode());
hashCode = prime * hashCode + ((getLinuxParameters() == null) ? 0 : getLinuxParameters().hashCode());
hashCode = prime * hashCode + ((getSecrets() == null) ? 0 : getSecrets().hashCode());
hashCode = prime * hashCode + ((getDependsOn() == null) ? 0 : getDependsOn().hashCode());
hashCode = prime * hashCode + ((getStartTimeout() == null) ? 0 : getStartTimeout().hashCode());
hashCode = prime * hashCode + ((getStopTimeout() == null) ? 0 : getStopTimeout().hashCode());
hashCode = prime * hashCode + ((getHostname() == null) ? 0 : getHostname().hashCode());
hashCode = prime * hashCode + ((getUser() == null) ? 0 : getUser().hashCode());
hashCode = prime * hashCode + ((getWorkingDirectory() == null) ? 0 : getWorkingDirectory().hashCode());
hashCode = prime * hashCode + ((getDisableNetworking() == null) ? 0 : getDisableNetworking().hashCode());
hashCode = prime * hashCode + ((getPrivileged() == null) ? 0 : getPrivileged().hashCode());
hashCode = prime * hashCode + ((getReadonlyRootFilesystem() == null) ? 0 : getReadonlyRootFilesystem().hashCode());
hashCode = prime * hashCode + ((getDnsServers() == null) ? 0 : getDnsServers().hashCode());
hashCode = prime * hashCode + ((getDnsSearchDomains() == null) ? 0 : getDnsSearchDomains().hashCode());
hashCode = prime * hashCode + ((getExtraHosts() == null) ? 0 : getExtraHosts().hashCode());
hashCode = prime * hashCode + ((getDockerSecurityOptions() == null) ? 0 : getDockerSecurityOptions().hashCode());
hashCode = prime * hashCode + ((getInteractive() == null) ? 0 : getInteractive().hashCode());
hashCode = prime * hashCode + ((getPseudoTerminal() == null) ? 0 : getPseudoTerminal().hashCode());
hashCode = prime * hashCode + ((getDockerLabels() == null) ? 0 : getDockerLabels().hashCode());
hashCode = prime * hashCode + ((getUlimits() == null) ? 0 : getUlimits().hashCode());
hashCode = prime * hashCode + ((getLogConfiguration() == null) ? 0 : getLogConfiguration().hashCode());
hashCode = prime * hashCode + ((getHealthCheck() == null) ? 0 : getHealthCheck().hashCode());
hashCode = prime * hashCode + ((getSystemControls() == null) ? 0 : getSystemControls().hashCode());
hashCode = prime * hashCode + ((getResourceRequirements() == null) ? 0 : getResourceRequirements().hashCode());
hashCode = prime * hashCode + ((getFirelensConfiguration() == null) ? 0 : getFirelensConfiguration().hashCode());
hashCode = prime * hashCode + ((getCredentialSpecs() == null) ? 0 : getCredentialSpecs().hashCode());
return hashCode;
}
@Override
public ContainerDefinition clone() {
try {
return (ContainerDefinition) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.ecs.model.transform.ContainerDefinitionMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}