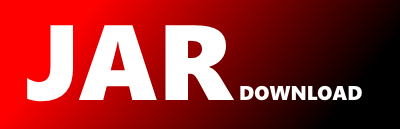
com.amazonaws.services.ecs.model.ContainerInstance Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ecs Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ecs.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* An Amazon EC2 or External instance that's running the Amazon ECS agent and has been registered with a cluster.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ContainerInstance implements Serializable, Cloneable, StructuredPojo {
/**
*
* The Amazon Resource Name (ARN) of the container instance. For more information about the ARN format, see Amazon Resource Name (ARN) in the Amazon ECS Developer Guide.
*
*/
private String containerInstanceArn;
/**
*
* The ID of the container instance. For Amazon EC2 instances, this value is the Amazon EC2 instance ID. For
* external instances, this value is the Amazon Web Services Systems Manager managed instance ID.
*
*/
private String ec2InstanceId;
/**
*
* The capacity provider that's associated with the container instance.
*
*/
private String capacityProviderName;
/**
*
* The version counter for the container instance. Every time a container instance experiences a change that
* triggers a CloudWatch event, the version counter is incremented. If you're replicating your Amazon ECS container
* instance state with CloudWatch Events, you can compare the version of a container instance reported by the Amazon
* ECS APIs with the version reported in CloudWatch Events for the container instance (inside the
* detail
object) to verify that the version in your event stream is current.
*
*/
private Long version;
/**
*
* The version information for the Amazon ECS container agent and Docker daemon running on the container instance.
*
*/
private VersionInfo versionInfo;
/**
*
* For CPU and memory resource types, this parameter describes the remaining CPU and memory that wasn't already
* allocated to tasks and is therefore available for new tasks. For port resource types, this parameter describes
* the ports that were reserved by the Amazon ECS container agent (at instance registration time) and any task
* containers that have reserved port mappings on the host (with the host
or bridge
* network mode). Any port that's not specified here is available for new tasks.
*
*/
private com.amazonaws.internal.SdkInternalList remainingResources;
/**
*
* For CPU and memory resource types, this parameter describes the amount of each resource that was available on the
* container instance when the container agent registered it with Amazon ECS. This value represents the total amount
* of CPU and memory that can be allocated on this container instance to tasks. For port resource types, this
* parameter describes the ports that were reserved by the Amazon ECS container agent when it registered the
* container instance with Amazon ECS.
*
*/
private com.amazonaws.internal.SdkInternalList registeredResources;
/**
*
* The status of the container instance. The valid values are REGISTERING
,
* REGISTRATION_FAILED
, ACTIVE
, INACTIVE
, DEREGISTERING
, or
* DRAINING
.
*
*
* If your account has opted in to the awsvpcTrunking
account setting, then any newly registered
* container instance will transition to a REGISTERING
status while the trunk elastic network interface
* is provisioned for the instance. If the registration fails, the instance will transition to a
* REGISTRATION_FAILED
status. You can describe the container instance and see the reason for failure
* in the statusReason
parameter. Once the container instance is terminated, the instance transitions
* to a DEREGISTERING
status while the trunk elastic network interface is deprovisioned. The instance
* then transitions to an INACTIVE
status.
*
*
* The ACTIVE
status indicates that the container instance can accept tasks. The DRAINING
* indicates that new tasks aren't placed on the container instance and any service tasks running on the container
* instance are removed if possible. For more information, see Container
* instance draining in the Amazon Elastic Container Service Developer Guide.
*
*/
private String status;
/**
*
* The reason that the container instance reached its current status.
*
*/
private String statusReason;
/**
*
* This parameter returns true
if the agent is connected to Amazon ECS. An instance with an agent that
* may be unhealthy or stopped return false
. Only instances connected to an agent can accept task
* placement requests.
*
*/
private Boolean agentConnected;
/**
*
* The number of tasks on the container instance that have a desired status (desiredStatus
) of
* RUNNING
.
*
*/
private Integer runningTasksCount;
/**
*
* The number of tasks on the container instance that are in the PENDING
status.
*
*/
private Integer pendingTasksCount;
/**
*
* The status of the most recent agent update. If an update wasn't ever requested, this value is NULL
.
*
*/
private String agentUpdateStatus;
/**
*
* The attributes set for the container instance, either by the Amazon ECS container agent at instance registration
* or manually with the PutAttributes operation.
*
*/
private com.amazonaws.internal.SdkInternalList attributes;
/**
*
* The Unix timestamp for the time when the container instance was registered.
*
*/
private java.util.Date registeredAt;
/**
*
* The resources attached to a container instance, such as an elastic network interface.
*
*/
private com.amazonaws.internal.SdkInternalList attachments;
/**
*
* The metadata that you apply to the container instance to help you categorize and organize them. Each tag consists
* of a key and an optional value. You define both.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* either keys or values as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys or values
* with this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*
*/
private com.amazonaws.internal.SdkInternalList tags;
/**
*
* An object representing the health status of the container instance.
*
*/
private ContainerInstanceHealthStatus healthStatus;
/**
*
* The Amazon Resource Name (ARN) of the container instance. For more information about the ARN format, see Amazon Resource Name (ARN) in the Amazon ECS Developer Guide.
*
*
* @param containerInstanceArn
* The Amazon Resource Name (ARN) of the container instance. For more information about the ARN format, see
* Amazon Resource Name (ARN) in the Amazon ECS Developer Guide.
*/
public void setContainerInstanceArn(String containerInstanceArn) {
this.containerInstanceArn = containerInstanceArn;
}
/**
*
* The Amazon Resource Name (ARN) of the container instance. For more information about the ARN format, see Amazon Resource Name (ARN) in the Amazon ECS Developer Guide.
*
*
* @return The Amazon Resource Name (ARN) of the container instance. For more information about the ARN format, see
* Amazon Resource Name (ARN) in the Amazon ECS Developer Guide.
*/
public String getContainerInstanceArn() {
return this.containerInstanceArn;
}
/**
*
* The Amazon Resource Name (ARN) of the container instance. For more information about the ARN format, see Amazon Resource Name (ARN) in the Amazon ECS Developer Guide.
*
*
* @param containerInstanceArn
* The Amazon Resource Name (ARN) of the container instance. For more information about the ARN format, see
* Amazon Resource Name (ARN) in the Amazon ECS Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerInstance withContainerInstanceArn(String containerInstanceArn) {
setContainerInstanceArn(containerInstanceArn);
return this;
}
/**
*
* The ID of the container instance. For Amazon EC2 instances, this value is the Amazon EC2 instance ID. For
* external instances, this value is the Amazon Web Services Systems Manager managed instance ID.
*
*
* @param ec2InstanceId
* The ID of the container instance. For Amazon EC2 instances, this value is the Amazon EC2 instance ID. For
* external instances, this value is the Amazon Web Services Systems Manager managed instance ID.
*/
public void setEc2InstanceId(String ec2InstanceId) {
this.ec2InstanceId = ec2InstanceId;
}
/**
*
* The ID of the container instance. For Amazon EC2 instances, this value is the Amazon EC2 instance ID. For
* external instances, this value is the Amazon Web Services Systems Manager managed instance ID.
*
*
* @return The ID of the container instance. For Amazon EC2 instances, this value is the Amazon EC2 instance ID. For
* external instances, this value is the Amazon Web Services Systems Manager managed instance ID.
*/
public String getEc2InstanceId() {
return this.ec2InstanceId;
}
/**
*
* The ID of the container instance. For Amazon EC2 instances, this value is the Amazon EC2 instance ID. For
* external instances, this value is the Amazon Web Services Systems Manager managed instance ID.
*
*
* @param ec2InstanceId
* The ID of the container instance. For Amazon EC2 instances, this value is the Amazon EC2 instance ID. For
* external instances, this value is the Amazon Web Services Systems Manager managed instance ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerInstance withEc2InstanceId(String ec2InstanceId) {
setEc2InstanceId(ec2InstanceId);
return this;
}
/**
*
* The capacity provider that's associated with the container instance.
*
*
* @param capacityProviderName
* The capacity provider that's associated with the container instance.
*/
public void setCapacityProviderName(String capacityProviderName) {
this.capacityProviderName = capacityProviderName;
}
/**
*
* The capacity provider that's associated with the container instance.
*
*
* @return The capacity provider that's associated with the container instance.
*/
public String getCapacityProviderName() {
return this.capacityProviderName;
}
/**
*
* The capacity provider that's associated with the container instance.
*
*
* @param capacityProviderName
* The capacity provider that's associated with the container instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerInstance withCapacityProviderName(String capacityProviderName) {
setCapacityProviderName(capacityProviderName);
return this;
}
/**
*
* The version counter for the container instance. Every time a container instance experiences a change that
* triggers a CloudWatch event, the version counter is incremented. If you're replicating your Amazon ECS container
* instance state with CloudWatch Events, you can compare the version of a container instance reported by the Amazon
* ECS APIs with the version reported in CloudWatch Events for the container instance (inside the
* detail
object) to verify that the version in your event stream is current.
*
*
* @param version
* The version counter for the container instance. Every time a container instance experiences a change that
* triggers a CloudWatch event, the version counter is incremented. If you're replicating your Amazon ECS
* container instance state with CloudWatch Events, you can compare the version of a container instance
* reported by the Amazon ECS APIs with the version reported in CloudWatch Events for the container instance
* (inside the detail
object) to verify that the version in your event stream is current.
*/
public void setVersion(Long version) {
this.version = version;
}
/**
*
* The version counter for the container instance. Every time a container instance experiences a change that
* triggers a CloudWatch event, the version counter is incremented. If you're replicating your Amazon ECS container
* instance state with CloudWatch Events, you can compare the version of a container instance reported by the Amazon
* ECS APIs with the version reported in CloudWatch Events for the container instance (inside the
* detail
object) to verify that the version in your event stream is current.
*
*
* @return The version counter for the container instance. Every time a container instance experiences a change that
* triggers a CloudWatch event, the version counter is incremented. If you're replicating your Amazon ECS
* container instance state with CloudWatch Events, you can compare the version of a container instance
* reported by the Amazon ECS APIs with the version reported in CloudWatch Events for the container instance
* (inside the detail
object) to verify that the version in your event stream is current.
*/
public Long getVersion() {
return this.version;
}
/**
*
* The version counter for the container instance. Every time a container instance experiences a change that
* triggers a CloudWatch event, the version counter is incremented. If you're replicating your Amazon ECS container
* instance state with CloudWatch Events, you can compare the version of a container instance reported by the Amazon
* ECS APIs with the version reported in CloudWatch Events for the container instance (inside the
* detail
object) to verify that the version in your event stream is current.
*
*
* @param version
* The version counter for the container instance. Every time a container instance experiences a change that
* triggers a CloudWatch event, the version counter is incremented. If you're replicating your Amazon ECS
* container instance state with CloudWatch Events, you can compare the version of a container instance
* reported by the Amazon ECS APIs with the version reported in CloudWatch Events for the container instance
* (inside the detail
object) to verify that the version in your event stream is current.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerInstance withVersion(Long version) {
setVersion(version);
return this;
}
/**
*
* The version information for the Amazon ECS container agent and Docker daemon running on the container instance.
*
*
* @param versionInfo
* The version information for the Amazon ECS container agent and Docker daemon running on the container
* instance.
*/
public void setVersionInfo(VersionInfo versionInfo) {
this.versionInfo = versionInfo;
}
/**
*
* The version information for the Amazon ECS container agent and Docker daemon running on the container instance.
*
*
* @return The version information for the Amazon ECS container agent and Docker daemon running on the container
* instance.
*/
public VersionInfo getVersionInfo() {
return this.versionInfo;
}
/**
*
* The version information for the Amazon ECS container agent and Docker daemon running on the container instance.
*
*
* @param versionInfo
* The version information for the Amazon ECS container agent and Docker daemon running on the container
* instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerInstance withVersionInfo(VersionInfo versionInfo) {
setVersionInfo(versionInfo);
return this;
}
/**
*
* For CPU and memory resource types, this parameter describes the remaining CPU and memory that wasn't already
* allocated to tasks and is therefore available for new tasks. For port resource types, this parameter describes
* the ports that were reserved by the Amazon ECS container agent (at instance registration time) and any task
* containers that have reserved port mappings on the host (with the host
or bridge
* network mode). Any port that's not specified here is available for new tasks.
*
*
* @return For CPU and memory resource types, this parameter describes the remaining CPU and memory that wasn't
* already allocated to tasks and is therefore available for new tasks. For port resource types, this
* parameter describes the ports that were reserved by the Amazon ECS container agent (at instance
* registration time) and any task containers that have reserved port mappings on the host (with the
* host
or bridge
network mode). Any port that's not specified here is available
* for new tasks.
*/
public java.util.List getRemainingResources() {
if (remainingResources == null) {
remainingResources = new com.amazonaws.internal.SdkInternalList();
}
return remainingResources;
}
/**
*
* For CPU and memory resource types, this parameter describes the remaining CPU and memory that wasn't already
* allocated to tasks and is therefore available for new tasks. For port resource types, this parameter describes
* the ports that were reserved by the Amazon ECS container agent (at instance registration time) and any task
* containers that have reserved port mappings on the host (with the host
or bridge
* network mode). Any port that's not specified here is available for new tasks.
*
*
* @param remainingResources
* For CPU and memory resource types, this parameter describes the remaining CPU and memory that wasn't
* already allocated to tasks and is therefore available for new tasks. For port resource types, this
* parameter describes the ports that were reserved by the Amazon ECS container agent (at instance
* registration time) and any task containers that have reserved port mappings on the host (with the
* host
or bridge
network mode). Any port that's not specified here is available
* for new tasks.
*/
public void setRemainingResources(java.util.Collection remainingResources) {
if (remainingResources == null) {
this.remainingResources = null;
return;
}
this.remainingResources = new com.amazonaws.internal.SdkInternalList(remainingResources);
}
/**
*
* For CPU and memory resource types, this parameter describes the remaining CPU and memory that wasn't already
* allocated to tasks and is therefore available for new tasks. For port resource types, this parameter describes
* the ports that were reserved by the Amazon ECS container agent (at instance registration time) and any task
* containers that have reserved port mappings on the host (with the host
or bridge
* network mode). Any port that's not specified here is available for new tasks.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRemainingResources(java.util.Collection)} or {@link #withRemainingResources(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param remainingResources
* For CPU and memory resource types, this parameter describes the remaining CPU and memory that wasn't
* already allocated to tasks and is therefore available for new tasks. For port resource types, this
* parameter describes the ports that were reserved by the Amazon ECS container agent (at instance
* registration time) and any task containers that have reserved port mappings on the host (with the
* host
or bridge
network mode). Any port that's not specified here is available
* for new tasks.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerInstance withRemainingResources(Resource... remainingResources) {
if (this.remainingResources == null) {
setRemainingResources(new com.amazonaws.internal.SdkInternalList(remainingResources.length));
}
for (Resource ele : remainingResources) {
this.remainingResources.add(ele);
}
return this;
}
/**
*
* For CPU and memory resource types, this parameter describes the remaining CPU and memory that wasn't already
* allocated to tasks and is therefore available for new tasks. For port resource types, this parameter describes
* the ports that were reserved by the Amazon ECS container agent (at instance registration time) and any task
* containers that have reserved port mappings on the host (with the host
or bridge
* network mode). Any port that's not specified here is available for new tasks.
*
*
* @param remainingResources
* For CPU and memory resource types, this parameter describes the remaining CPU and memory that wasn't
* already allocated to tasks and is therefore available for new tasks. For port resource types, this
* parameter describes the ports that were reserved by the Amazon ECS container agent (at instance
* registration time) and any task containers that have reserved port mappings on the host (with the
* host
or bridge
network mode). Any port that's not specified here is available
* for new tasks.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerInstance withRemainingResources(java.util.Collection remainingResources) {
setRemainingResources(remainingResources);
return this;
}
/**
*
* For CPU and memory resource types, this parameter describes the amount of each resource that was available on the
* container instance when the container agent registered it with Amazon ECS. This value represents the total amount
* of CPU and memory that can be allocated on this container instance to tasks. For port resource types, this
* parameter describes the ports that were reserved by the Amazon ECS container agent when it registered the
* container instance with Amazon ECS.
*
*
* @return For CPU and memory resource types, this parameter describes the amount of each resource that was
* available on the container instance when the container agent registered it with Amazon ECS. This value
* represents the total amount of CPU and memory that can be allocated on this container instance to tasks.
* For port resource types, this parameter describes the ports that were reserved by the Amazon ECS
* container agent when it registered the container instance with Amazon ECS.
*/
public java.util.List getRegisteredResources() {
if (registeredResources == null) {
registeredResources = new com.amazonaws.internal.SdkInternalList();
}
return registeredResources;
}
/**
*
* For CPU and memory resource types, this parameter describes the amount of each resource that was available on the
* container instance when the container agent registered it with Amazon ECS. This value represents the total amount
* of CPU and memory that can be allocated on this container instance to tasks. For port resource types, this
* parameter describes the ports that were reserved by the Amazon ECS container agent when it registered the
* container instance with Amazon ECS.
*
*
* @param registeredResources
* For CPU and memory resource types, this parameter describes the amount of each resource that was available
* on the container instance when the container agent registered it with Amazon ECS. This value represents
* the total amount of CPU and memory that can be allocated on this container instance to tasks. For port
* resource types, this parameter describes the ports that were reserved by the Amazon ECS container agent
* when it registered the container instance with Amazon ECS.
*/
public void setRegisteredResources(java.util.Collection registeredResources) {
if (registeredResources == null) {
this.registeredResources = null;
return;
}
this.registeredResources = new com.amazonaws.internal.SdkInternalList(registeredResources);
}
/**
*
* For CPU and memory resource types, this parameter describes the amount of each resource that was available on the
* container instance when the container agent registered it with Amazon ECS. This value represents the total amount
* of CPU and memory that can be allocated on this container instance to tasks. For port resource types, this
* parameter describes the ports that were reserved by the Amazon ECS container agent when it registered the
* container instance with Amazon ECS.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRegisteredResources(java.util.Collection)} or {@link #withRegisteredResources(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param registeredResources
* For CPU and memory resource types, this parameter describes the amount of each resource that was available
* on the container instance when the container agent registered it with Amazon ECS. This value represents
* the total amount of CPU and memory that can be allocated on this container instance to tasks. For port
* resource types, this parameter describes the ports that were reserved by the Amazon ECS container agent
* when it registered the container instance with Amazon ECS.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerInstance withRegisteredResources(Resource... registeredResources) {
if (this.registeredResources == null) {
setRegisteredResources(new com.amazonaws.internal.SdkInternalList(registeredResources.length));
}
for (Resource ele : registeredResources) {
this.registeredResources.add(ele);
}
return this;
}
/**
*
* For CPU and memory resource types, this parameter describes the amount of each resource that was available on the
* container instance when the container agent registered it with Amazon ECS. This value represents the total amount
* of CPU and memory that can be allocated on this container instance to tasks. For port resource types, this
* parameter describes the ports that were reserved by the Amazon ECS container agent when it registered the
* container instance with Amazon ECS.
*
*
* @param registeredResources
* For CPU and memory resource types, this parameter describes the amount of each resource that was available
* on the container instance when the container agent registered it with Amazon ECS. This value represents
* the total amount of CPU and memory that can be allocated on this container instance to tasks. For port
* resource types, this parameter describes the ports that were reserved by the Amazon ECS container agent
* when it registered the container instance with Amazon ECS.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerInstance withRegisteredResources(java.util.Collection registeredResources) {
setRegisteredResources(registeredResources);
return this;
}
/**
*
* The status of the container instance. The valid values are REGISTERING
,
* REGISTRATION_FAILED
, ACTIVE
, INACTIVE
, DEREGISTERING
, or
* DRAINING
.
*
*
* If your account has opted in to the awsvpcTrunking
account setting, then any newly registered
* container instance will transition to a REGISTERING
status while the trunk elastic network interface
* is provisioned for the instance. If the registration fails, the instance will transition to a
* REGISTRATION_FAILED
status. You can describe the container instance and see the reason for failure
* in the statusReason
parameter. Once the container instance is terminated, the instance transitions
* to a DEREGISTERING
status while the trunk elastic network interface is deprovisioned. The instance
* then transitions to an INACTIVE
status.
*
*
* The ACTIVE
status indicates that the container instance can accept tasks. The DRAINING
* indicates that new tasks aren't placed on the container instance and any service tasks running on the container
* instance are removed if possible. For more information, see Container
* instance draining in the Amazon Elastic Container Service Developer Guide.
*
*
* @param status
* The status of the container instance. The valid values are REGISTERING
,
* REGISTRATION_FAILED
, ACTIVE
, INACTIVE
, DEREGISTERING
,
* or DRAINING
.
*
* If your account has opted in to the awsvpcTrunking
account setting, then any newly registered
* container instance will transition to a REGISTERING
status while the trunk elastic network
* interface is provisioned for the instance. If the registration fails, the instance will transition to a
* REGISTRATION_FAILED
status. You can describe the container instance and see the reason for
* failure in the statusReason
parameter. Once the container instance is terminated, the
* instance transitions to a DEREGISTERING
status while the trunk elastic network interface is
* deprovisioned. The instance then transitions to an INACTIVE
status.
*
*
* The ACTIVE
status indicates that the container instance can accept tasks. The
* DRAINING
indicates that new tasks aren't placed on the container instance and any service
* tasks running on the container instance are removed if possible. For more information, see Container instance draining in the Amazon Elastic Container Service Developer Guide.
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the container instance. The valid values are REGISTERING
,
* REGISTRATION_FAILED
, ACTIVE
, INACTIVE
, DEREGISTERING
, or
* DRAINING
.
*
*
* If your account has opted in to the awsvpcTrunking
account setting, then any newly registered
* container instance will transition to a REGISTERING
status while the trunk elastic network interface
* is provisioned for the instance. If the registration fails, the instance will transition to a
* REGISTRATION_FAILED
status. You can describe the container instance and see the reason for failure
* in the statusReason
parameter. Once the container instance is terminated, the instance transitions
* to a DEREGISTERING
status while the trunk elastic network interface is deprovisioned. The instance
* then transitions to an INACTIVE
status.
*
*
* The ACTIVE
status indicates that the container instance can accept tasks. The DRAINING
* indicates that new tasks aren't placed on the container instance and any service tasks running on the container
* instance are removed if possible. For more information, see Container
* instance draining in the Amazon Elastic Container Service Developer Guide.
*
*
* @return The status of the container instance. The valid values are REGISTERING
,
* REGISTRATION_FAILED
, ACTIVE
, INACTIVE
, DEREGISTERING
,
* or DRAINING
.
*
* If your account has opted in to the awsvpcTrunking
account setting, then any newly
* registered container instance will transition to a REGISTERING
status while the trunk
* elastic network interface is provisioned for the instance. If the registration fails, the instance will
* transition to a REGISTRATION_FAILED
status. You can describe the container instance and see
* the reason for failure in the statusReason
parameter. Once the container instance is
* terminated, the instance transitions to a DEREGISTERING
status while the trunk elastic
* network interface is deprovisioned. The instance then transitions to an INACTIVE
status.
*
*
* The ACTIVE
status indicates that the container instance can accept tasks. The
* DRAINING
indicates that new tasks aren't placed on the container instance and any service
* tasks running on the container instance are removed if possible. For more information, see Container instance draining in the Amazon Elastic Container Service Developer Guide.
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of the container instance. The valid values are REGISTERING
,
* REGISTRATION_FAILED
, ACTIVE
, INACTIVE
, DEREGISTERING
, or
* DRAINING
.
*
*
* If your account has opted in to the awsvpcTrunking
account setting, then any newly registered
* container instance will transition to a REGISTERING
status while the trunk elastic network interface
* is provisioned for the instance. If the registration fails, the instance will transition to a
* REGISTRATION_FAILED
status. You can describe the container instance and see the reason for failure
* in the statusReason
parameter. Once the container instance is terminated, the instance transitions
* to a DEREGISTERING
status while the trunk elastic network interface is deprovisioned. The instance
* then transitions to an INACTIVE
status.
*
*
* The ACTIVE
status indicates that the container instance can accept tasks. The DRAINING
* indicates that new tasks aren't placed on the container instance and any service tasks running on the container
* instance are removed if possible. For more information, see Container
* instance draining in the Amazon Elastic Container Service Developer Guide.
*
*
* @param status
* The status of the container instance. The valid values are REGISTERING
,
* REGISTRATION_FAILED
, ACTIVE
, INACTIVE
, DEREGISTERING
,
* or DRAINING
.
*
* If your account has opted in to the awsvpcTrunking
account setting, then any newly registered
* container instance will transition to a REGISTERING
status while the trunk elastic network
* interface is provisioned for the instance. If the registration fails, the instance will transition to a
* REGISTRATION_FAILED
status. You can describe the container instance and see the reason for
* failure in the statusReason
parameter. Once the container instance is terminated, the
* instance transitions to a DEREGISTERING
status while the trunk elastic network interface is
* deprovisioned. The instance then transitions to an INACTIVE
status.
*
*
* The ACTIVE
status indicates that the container instance can accept tasks. The
* DRAINING
indicates that new tasks aren't placed on the container instance and any service
* tasks running on the container instance are removed if possible. For more information, see Container instance draining in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerInstance withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The reason that the container instance reached its current status.
*
*
* @param statusReason
* The reason that the container instance reached its current status.
*/
public void setStatusReason(String statusReason) {
this.statusReason = statusReason;
}
/**
*
* The reason that the container instance reached its current status.
*
*
* @return The reason that the container instance reached its current status.
*/
public String getStatusReason() {
return this.statusReason;
}
/**
*
* The reason that the container instance reached its current status.
*
*
* @param statusReason
* The reason that the container instance reached its current status.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerInstance withStatusReason(String statusReason) {
setStatusReason(statusReason);
return this;
}
/**
*
* This parameter returns true
if the agent is connected to Amazon ECS. An instance with an agent that
* may be unhealthy or stopped return false
. Only instances connected to an agent can accept task
* placement requests.
*
*
* @param agentConnected
* This parameter returns true
if the agent is connected to Amazon ECS. An instance with an
* agent that may be unhealthy or stopped return false
. Only instances connected to an agent can
* accept task placement requests.
*/
public void setAgentConnected(Boolean agentConnected) {
this.agentConnected = agentConnected;
}
/**
*
* This parameter returns true
if the agent is connected to Amazon ECS. An instance with an agent that
* may be unhealthy or stopped return false
. Only instances connected to an agent can accept task
* placement requests.
*
*
* @return This parameter returns true
if the agent is connected to Amazon ECS. An instance with an
* agent that may be unhealthy or stopped return false
. Only instances connected to an agent
* can accept task placement requests.
*/
public Boolean getAgentConnected() {
return this.agentConnected;
}
/**
*
* This parameter returns true
if the agent is connected to Amazon ECS. An instance with an agent that
* may be unhealthy or stopped return false
. Only instances connected to an agent can accept task
* placement requests.
*
*
* @param agentConnected
* This parameter returns true
if the agent is connected to Amazon ECS. An instance with an
* agent that may be unhealthy or stopped return false
. Only instances connected to an agent can
* accept task placement requests.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerInstance withAgentConnected(Boolean agentConnected) {
setAgentConnected(agentConnected);
return this;
}
/**
*
* This parameter returns true
if the agent is connected to Amazon ECS. An instance with an agent that
* may be unhealthy or stopped return false
. Only instances connected to an agent can accept task
* placement requests.
*
*
* @return This parameter returns true
if the agent is connected to Amazon ECS. An instance with an
* agent that may be unhealthy or stopped return false
. Only instances connected to an agent
* can accept task placement requests.
*/
public Boolean isAgentConnected() {
return this.agentConnected;
}
/**
*
* The number of tasks on the container instance that have a desired status (desiredStatus
) of
* RUNNING
.
*
*
* @param runningTasksCount
* The number of tasks on the container instance that have a desired status (desiredStatus
) of
* RUNNING
.
*/
public void setRunningTasksCount(Integer runningTasksCount) {
this.runningTasksCount = runningTasksCount;
}
/**
*
* The number of tasks on the container instance that have a desired status (desiredStatus
) of
* RUNNING
.
*
*
* @return The number of tasks on the container instance that have a desired status (desiredStatus
) of
* RUNNING
.
*/
public Integer getRunningTasksCount() {
return this.runningTasksCount;
}
/**
*
* The number of tasks on the container instance that have a desired status (desiredStatus
) of
* RUNNING
.
*
*
* @param runningTasksCount
* The number of tasks on the container instance that have a desired status (desiredStatus
) of
* RUNNING
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerInstance withRunningTasksCount(Integer runningTasksCount) {
setRunningTasksCount(runningTasksCount);
return this;
}
/**
*
* The number of tasks on the container instance that are in the PENDING
status.
*
*
* @param pendingTasksCount
* The number of tasks on the container instance that are in the PENDING
status.
*/
public void setPendingTasksCount(Integer pendingTasksCount) {
this.pendingTasksCount = pendingTasksCount;
}
/**
*
* The number of tasks on the container instance that are in the PENDING
status.
*
*
* @return The number of tasks on the container instance that are in the PENDING
status.
*/
public Integer getPendingTasksCount() {
return this.pendingTasksCount;
}
/**
*
* The number of tasks on the container instance that are in the PENDING
status.
*
*
* @param pendingTasksCount
* The number of tasks on the container instance that are in the PENDING
status.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerInstance withPendingTasksCount(Integer pendingTasksCount) {
setPendingTasksCount(pendingTasksCount);
return this;
}
/**
*
* The status of the most recent agent update. If an update wasn't ever requested, this value is NULL
.
*
*
* @param agentUpdateStatus
* The status of the most recent agent update. If an update wasn't ever requested, this value is
* NULL
.
* @see AgentUpdateStatus
*/
public void setAgentUpdateStatus(String agentUpdateStatus) {
this.agentUpdateStatus = agentUpdateStatus;
}
/**
*
* The status of the most recent agent update. If an update wasn't ever requested, this value is NULL
.
*
*
* @return The status of the most recent agent update. If an update wasn't ever requested, this value is
* NULL
.
* @see AgentUpdateStatus
*/
public String getAgentUpdateStatus() {
return this.agentUpdateStatus;
}
/**
*
* The status of the most recent agent update. If an update wasn't ever requested, this value is NULL
.
*
*
* @param agentUpdateStatus
* The status of the most recent agent update. If an update wasn't ever requested, this value is
* NULL
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AgentUpdateStatus
*/
public ContainerInstance withAgentUpdateStatus(String agentUpdateStatus) {
setAgentUpdateStatus(agentUpdateStatus);
return this;
}
/**
*
* The status of the most recent agent update. If an update wasn't ever requested, this value is NULL
.
*
*
* @param agentUpdateStatus
* The status of the most recent agent update. If an update wasn't ever requested, this value is
* NULL
.
* @see AgentUpdateStatus
*/
public void setAgentUpdateStatus(AgentUpdateStatus agentUpdateStatus) {
withAgentUpdateStatus(agentUpdateStatus);
}
/**
*
* The status of the most recent agent update. If an update wasn't ever requested, this value is NULL
.
*
*
* @param agentUpdateStatus
* The status of the most recent agent update. If an update wasn't ever requested, this value is
* NULL
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see AgentUpdateStatus
*/
public ContainerInstance withAgentUpdateStatus(AgentUpdateStatus agentUpdateStatus) {
this.agentUpdateStatus = agentUpdateStatus.toString();
return this;
}
/**
*
* The attributes set for the container instance, either by the Amazon ECS container agent at instance registration
* or manually with the PutAttributes operation.
*
*
* @return The attributes set for the container instance, either by the Amazon ECS container agent at instance
* registration or manually with the PutAttributes operation.
*/
public java.util.List getAttributes() {
if (attributes == null) {
attributes = new com.amazonaws.internal.SdkInternalList();
}
return attributes;
}
/**
*
* The attributes set for the container instance, either by the Amazon ECS container agent at instance registration
* or manually with the PutAttributes operation.
*
*
* @param attributes
* The attributes set for the container instance, either by the Amazon ECS container agent at instance
* registration or manually with the PutAttributes operation.
*/
public void setAttributes(java.util.Collection attributes) {
if (attributes == null) {
this.attributes = null;
return;
}
this.attributes = new com.amazonaws.internal.SdkInternalList(attributes);
}
/**
*
* The attributes set for the container instance, either by the Amazon ECS container agent at instance registration
* or manually with the PutAttributes operation.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAttributes(java.util.Collection)} or {@link #withAttributes(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param attributes
* The attributes set for the container instance, either by the Amazon ECS container agent at instance
* registration or manually with the PutAttributes operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerInstance withAttributes(Attribute... attributes) {
if (this.attributes == null) {
setAttributes(new com.amazonaws.internal.SdkInternalList(attributes.length));
}
for (Attribute ele : attributes) {
this.attributes.add(ele);
}
return this;
}
/**
*
* The attributes set for the container instance, either by the Amazon ECS container agent at instance registration
* or manually with the PutAttributes operation.
*
*
* @param attributes
* The attributes set for the container instance, either by the Amazon ECS container agent at instance
* registration or manually with the PutAttributes operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerInstance withAttributes(java.util.Collection attributes) {
setAttributes(attributes);
return this;
}
/**
*
* The Unix timestamp for the time when the container instance was registered.
*
*
* @param registeredAt
* The Unix timestamp for the time when the container instance was registered.
*/
public void setRegisteredAt(java.util.Date registeredAt) {
this.registeredAt = registeredAt;
}
/**
*
* The Unix timestamp for the time when the container instance was registered.
*
*
* @return The Unix timestamp for the time when the container instance was registered.
*/
public java.util.Date getRegisteredAt() {
return this.registeredAt;
}
/**
*
* The Unix timestamp for the time when the container instance was registered.
*
*
* @param registeredAt
* The Unix timestamp for the time when the container instance was registered.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerInstance withRegisteredAt(java.util.Date registeredAt) {
setRegisteredAt(registeredAt);
return this;
}
/**
*
* The resources attached to a container instance, such as an elastic network interface.
*
*
* @return The resources attached to a container instance, such as an elastic network interface.
*/
public java.util.List getAttachments() {
if (attachments == null) {
attachments = new com.amazonaws.internal.SdkInternalList();
}
return attachments;
}
/**
*
* The resources attached to a container instance, such as an elastic network interface.
*
*
* @param attachments
* The resources attached to a container instance, such as an elastic network interface.
*/
public void setAttachments(java.util.Collection attachments) {
if (attachments == null) {
this.attachments = null;
return;
}
this.attachments = new com.amazonaws.internal.SdkInternalList(attachments);
}
/**
*
* The resources attached to a container instance, such as an elastic network interface.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAttachments(java.util.Collection)} or {@link #withAttachments(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param attachments
* The resources attached to a container instance, such as an elastic network interface.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerInstance withAttachments(Attachment... attachments) {
if (this.attachments == null) {
setAttachments(new com.amazonaws.internal.SdkInternalList(attachments.length));
}
for (Attachment ele : attachments) {
this.attachments.add(ele);
}
return this;
}
/**
*
* The resources attached to a container instance, such as an elastic network interface.
*
*
* @param attachments
* The resources attached to a container instance, such as an elastic network interface.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerInstance withAttachments(java.util.Collection attachments) {
setAttachments(attachments);
return this;
}
/**
*
* The metadata that you apply to the container instance to help you categorize and organize them. Each tag consists
* of a key and an optional value. You define both.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* either keys or values as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys or values
* with this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*
*
* @return The metadata that you apply to the container instance to help you categorize and organize them. Each tag
* consists of a key and an optional value. You define both.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may
* have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a
* prefix for either keys or values as it is reserved for Amazon Web Services use. You cannot edit or delete
* tag keys or values with this prefix. Tags with this prefix do not count against your tags per resource
* limit.
*
*
*/
public java.util.List getTags() {
if (tags == null) {
tags = new com.amazonaws.internal.SdkInternalList();
}
return tags;
}
/**
*
* The metadata that you apply to the container instance to help you categorize and organize them. Each tag consists
* of a key and an optional value. You define both.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* either keys or values as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys or values
* with this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*
*
* @param tags
* The metadata that you apply to the container instance to help you categorize and organize them. Each tag
* consists of a key and an optional value. You define both.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may
* have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix
* for either keys or values as it is reserved for Amazon Web Services use. You cannot edit or delete tag
* keys or values with this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new com.amazonaws.internal.SdkInternalList(tags);
}
/**
*
* The metadata that you apply to the container instance to help you categorize and organize them. Each tag consists
* of a key and an optional value. You define both.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* either keys or values as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys or values
* with this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* The metadata that you apply to the container instance to help you categorize and organize them. Each tag
* consists of a key and an optional value. You define both.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may
* have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix
* for either keys or values as it is reserved for Amazon Web Services use. You cannot edit or delete tag
* keys or values with this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerInstance withTags(Tag... tags) {
if (this.tags == null) {
setTags(new com.amazonaws.internal.SdkInternalList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* The metadata that you apply to the container instance to help you categorize and organize them. Each tag consists
* of a key and an optional value. You define both.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* either keys or values as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys or values
* with this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*
*
* @param tags
* The metadata that you apply to the container instance to help you categorize and organize them. Each tag
* consists of a key and an optional value. You define both.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may
* have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix
* for either keys or values as it is reserved for Amazon Web Services use. You cannot edit or delete tag
* keys or values with this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerInstance withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* An object representing the health status of the container instance.
*
*
* @param healthStatus
* An object representing the health status of the container instance.
*/
public void setHealthStatus(ContainerInstanceHealthStatus healthStatus) {
this.healthStatus = healthStatus;
}
/**
*
* An object representing the health status of the container instance.
*
*
* @return An object representing the health status of the container instance.
*/
public ContainerInstanceHealthStatus getHealthStatus() {
return this.healthStatus;
}
/**
*
* An object representing the health status of the container instance.
*
*
* @param healthStatus
* An object representing the health status of the container instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ContainerInstance withHealthStatus(ContainerInstanceHealthStatus healthStatus) {
setHealthStatus(healthStatus);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getContainerInstanceArn() != null)
sb.append("ContainerInstanceArn: ").append(getContainerInstanceArn()).append(",");
if (getEc2InstanceId() != null)
sb.append("Ec2InstanceId: ").append(getEc2InstanceId()).append(",");
if (getCapacityProviderName() != null)
sb.append("CapacityProviderName: ").append(getCapacityProviderName()).append(",");
if (getVersion() != null)
sb.append("Version: ").append(getVersion()).append(",");
if (getVersionInfo() != null)
sb.append("VersionInfo: ").append(getVersionInfo()).append(",");
if (getRemainingResources() != null)
sb.append("RemainingResources: ").append(getRemainingResources()).append(",");
if (getRegisteredResources() != null)
sb.append("RegisteredResources: ").append(getRegisteredResources()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getStatusReason() != null)
sb.append("StatusReason: ").append(getStatusReason()).append(",");
if (getAgentConnected() != null)
sb.append("AgentConnected: ").append(getAgentConnected()).append(",");
if (getRunningTasksCount() != null)
sb.append("RunningTasksCount: ").append(getRunningTasksCount()).append(",");
if (getPendingTasksCount() != null)
sb.append("PendingTasksCount: ").append(getPendingTasksCount()).append(",");
if (getAgentUpdateStatus() != null)
sb.append("AgentUpdateStatus: ").append(getAgentUpdateStatus()).append(",");
if (getAttributes() != null)
sb.append("Attributes: ").append(getAttributes()).append(",");
if (getRegisteredAt() != null)
sb.append("RegisteredAt: ").append(getRegisteredAt()).append(",");
if (getAttachments() != null)
sb.append("Attachments: ").append(getAttachments()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getHealthStatus() != null)
sb.append("HealthStatus: ").append(getHealthStatus());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ContainerInstance == false)
return false;
ContainerInstance other = (ContainerInstance) obj;
if (other.getContainerInstanceArn() == null ^ this.getContainerInstanceArn() == null)
return false;
if (other.getContainerInstanceArn() != null && other.getContainerInstanceArn().equals(this.getContainerInstanceArn()) == false)
return false;
if (other.getEc2InstanceId() == null ^ this.getEc2InstanceId() == null)
return false;
if (other.getEc2InstanceId() != null && other.getEc2InstanceId().equals(this.getEc2InstanceId()) == false)
return false;
if (other.getCapacityProviderName() == null ^ this.getCapacityProviderName() == null)
return false;
if (other.getCapacityProviderName() != null && other.getCapacityProviderName().equals(this.getCapacityProviderName()) == false)
return false;
if (other.getVersion() == null ^ this.getVersion() == null)
return false;
if (other.getVersion() != null && other.getVersion().equals(this.getVersion()) == false)
return false;
if (other.getVersionInfo() == null ^ this.getVersionInfo() == null)
return false;
if (other.getVersionInfo() != null && other.getVersionInfo().equals(this.getVersionInfo()) == false)
return false;
if (other.getRemainingResources() == null ^ this.getRemainingResources() == null)
return false;
if (other.getRemainingResources() != null && other.getRemainingResources().equals(this.getRemainingResources()) == false)
return false;
if (other.getRegisteredResources() == null ^ this.getRegisteredResources() == null)
return false;
if (other.getRegisteredResources() != null && other.getRegisteredResources().equals(this.getRegisteredResources()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getStatusReason() == null ^ this.getStatusReason() == null)
return false;
if (other.getStatusReason() != null && other.getStatusReason().equals(this.getStatusReason()) == false)
return false;
if (other.getAgentConnected() == null ^ this.getAgentConnected() == null)
return false;
if (other.getAgentConnected() != null && other.getAgentConnected().equals(this.getAgentConnected()) == false)
return false;
if (other.getRunningTasksCount() == null ^ this.getRunningTasksCount() == null)
return false;
if (other.getRunningTasksCount() != null && other.getRunningTasksCount().equals(this.getRunningTasksCount()) == false)
return false;
if (other.getPendingTasksCount() == null ^ this.getPendingTasksCount() == null)
return false;
if (other.getPendingTasksCount() != null && other.getPendingTasksCount().equals(this.getPendingTasksCount()) == false)
return false;
if (other.getAgentUpdateStatus() == null ^ this.getAgentUpdateStatus() == null)
return false;
if (other.getAgentUpdateStatus() != null && other.getAgentUpdateStatus().equals(this.getAgentUpdateStatus()) == false)
return false;
if (other.getAttributes() == null ^ this.getAttributes() == null)
return false;
if (other.getAttributes() != null && other.getAttributes().equals(this.getAttributes()) == false)
return false;
if (other.getRegisteredAt() == null ^ this.getRegisteredAt() == null)
return false;
if (other.getRegisteredAt() != null && other.getRegisteredAt().equals(this.getRegisteredAt()) == false)
return false;
if (other.getAttachments() == null ^ this.getAttachments() == null)
return false;
if (other.getAttachments() != null && other.getAttachments().equals(this.getAttachments()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getHealthStatus() == null ^ this.getHealthStatus() == null)
return false;
if (other.getHealthStatus() != null && other.getHealthStatus().equals(this.getHealthStatus()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getContainerInstanceArn() == null) ? 0 : getContainerInstanceArn().hashCode());
hashCode = prime * hashCode + ((getEc2InstanceId() == null) ? 0 : getEc2InstanceId().hashCode());
hashCode = prime * hashCode + ((getCapacityProviderName() == null) ? 0 : getCapacityProviderName().hashCode());
hashCode = prime * hashCode + ((getVersion() == null) ? 0 : getVersion().hashCode());
hashCode = prime * hashCode + ((getVersionInfo() == null) ? 0 : getVersionInfo().hashCode());
hashCode = prime * hashCode + ((getRemainingResources() == null) ? 0 : getRemainingResources().hashCode());
hashCode = prime * hashCode + ((getRegisteredResources() == null) ? 0 : getRegisteredResources().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getStatusReason() == null) ? 0 : getStatusReason().hashCode());
hashCode = prime * hashCode + ((getAgentConnected() == null) ? 0 : getAgentConnected().hashCode());
hashCode = prime * hashCode + ((getRunningTasksCount() == null) ? 0 : getRunningTasksCount().hashCode());
hashCode = prime * hashCode + ((getPendingTasksCount() == null) ? 0 : getPendingTasksCount().hashCode());
hashCode = prime * hashCode + ((getAgentUpdateStatus() == null) ? 0 : getAgentUpdateStatus().hashCode());
hashCode = prime * hashCode + ((getAttributes() == null) ? 0 : getAttributes().hashCode());
hashCode = prime * hashCode + ((getRegisteredAt() == null) ? 0 : getRegisteredAt().hashCode());
hashCode = prime * hashCode + ((getAttachments() == null) ? 0 : getAttachments().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getHealthStatus() == null) ? 0 : getHealthStatus().hashCode());
return hashCode;
}
@Override
public ContainerInstance clone() {
try {
return (ContainerInstance) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.ecs.model.transform.ContainerInstanceMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}