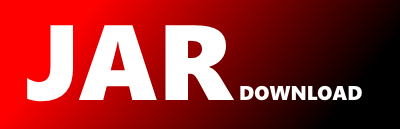
com.amazonaws.services.ecs.model.Container Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ecs Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ecs.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* A Docker container that's part of a task.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Container implements Serializable, Cloneable, StructuredPojo {
/**
*
* The Amazon Resource Name (ARN) of the container.
*
*/
private String containerArn;
/**
*
* The ARN of the task.
*
*/
private String taskArn;
/**
*
* The name of the container.
*
*/
private String name;
/**
*
* The image used for the container.
*
*/
private String image;
/**
*
* The container image manifest digest.
*
*/
private String imageDigest;
/**
*
* The ID of the Docker container.
*
*/
private String runtimeId;
/**
*
* The last known status of the container.
*
*/
private String lastStatus;
/**
*
* The exit code returned from the container.
*
*/
private Integer exitCode;
/**
*
* A short (255 max characters) human-readable string to provide additional details about a running or stopped
* container.
*
*/
private String reason;
/**
*
* The network bindings associated with the container.
*
*/
private com.amazonaws.internal.SdkInternalList networkBindings;
/**
*
* The network interfaces associated with the container.
*
*/
private com.amazonaws.internal.SdkInternalList networkInterfaces;
/**
*
* The health status of the container. If health checks aren't configured for this container in its task definition,
* then it reports the health status as UNKNOWN
.
*
*/
private String healthStatus;
/**
*
* The details of any Amazon ECS managed agents associated with the container.
*
*/
private com.amazonaws.internal.SdkInternalList managedAgents;
/**
*
* The number of CPU units set for the container. The value is 0
if no value was specified in the
* container definition when the task definition was registered.
*
*/
private String cpu;
/**
*
* The hard limit (in MiB) of memory set for the container.
*
*/
private String memory;
/**
*
* The soft limit (in MiB) of memory set for the container.
*
*/
private String memoryReservation;
/**
*
* The IDs of each GPU assigned to the container.
*
*/
private com.amazonaws.internal.SdkInternalList gpuIds;
/**
*
* The Amazon Resource Name (ARN) of the container.
*
*
* @param containerArn
* The Amazon Resource Name (ARN) of the container.
*/
public void setContainerArn(String containerArn) {
this.containerArn = containerArn;
}
/**
*
* The Amazon Resource Name (ARN) of the container.
*
*
* @return The Amazon Resource Name (ARN) of the container.
*/
public String getContainerArn() {
return this.containerArn;
}
/**
*
* The Amazon Resource Name (ARN) of the container.
*
*
* @param containerArn
* The Amazon Resource Name (ARN) of the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Container withContainerArn(String containerArn) {
setContainerArn(containerArn);
return this;
}
/**
*
* The ARN of the task.
*
*
* @param taskArn
* The ARN of the task.
*/
public void setTaskArn(String taskArn) {
this.taskArn = taskArn;
}
/**
*
* The ARN of the task.
*
*
* @return The ARN of the task.
*/
public String getTaskArn() {
return this.taskArn;
}
/**
*
* The ARN of the task.
*
*
* @param taskArn
* The ARN of the task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Container withTaskArn(String taskArn) {
setTaskArn(taskArn);
return this;
}
/**
*
* The name of the container.
*
*
* @param name
* The name of the container.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the container.
*
*
* @return The name of the container.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the container.
*
*
* @param name
* The name of the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Container withName(String name) {
setName(name);
return this;
}
/**
*
* The image used for the container.
*
*
* @param image
* The image used for the container.
*/
public void setImage(String image) {
this.image = image;
}
/**
*
* The image used for the container.
*
*
* @return The image used for the container.
*/
public String getImage() {
return this.image;
}
/**
*
* The image used for the container.
*
*
* @param image
* The image used for the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Container withImage(String image) {
setImage(image);
return this;
}
/**
*
* The container image manifest digest.
*
*
* @param imageDigest
* The container image manifest digest.
*/
public void setImageDigest(String imageDigest) {
this.imageDigest = imageDigest;
}
/**
*
* The container image manifest digest.
*
*
* @return The container image manifest digest.
*/
public String getImageDigest() {
return this.imageDigest;
}
/**
*
* The container image manifest digest.
*
*
* @param imageDigest
* The container image manifest digest.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Container withImageDigest(String imageDigest) {
setImageDigest(imageDigest);
return this;
}
/**
*
* The ID of the Docker container.
*
*
* @param runtimeId
* The ID of the Docker container.
*/
public void setRuntimeId(String runtimeId) {
this.runtimeId = runtimeId;
}
/**
*
* The ID of the Docker container.
*
*
* @return The ID of the Docker container.
*/
public String getRuntimeId() {
return this.runtimeId;
}
/**
*
* The ID of the Docker container.
*
*
* @param runtimeId
* The ID of the Docker container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Container withRuntimeId(String runtimeId) {
setRuntimeId(runtimeId);
return this;
}
/**
*
* The last known status of the container.
*
*
* @param lastStatus
* The last known status of the container.
*/
public void setLastStatus(String lastStatus) {
this.lastStatus = lastStatus;
}
/**
*
* The last known status of the container.
*
*
* @return The last known status of the container.
*/
public String getLastStatus() {
return this.lastStatus;
}
/**
*
* The last known status of the container.
*
*
* @param lastStatus
* The last known status of the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Container withLastStatus(String lastStatus) {
setLastStatus(lastStatus);
return this;
}
/**
*
* The exit code returned from the container.
*
*
* @param exitCode
* The exit code returned from the container.
*/
public void setExitCode(Integer exitCode) {
this.exitCode = exitCode;
}
/**
*
* The exit code returned from the container.
*
*
* @return The exit code returned from the container.
*/
public Integer getExitCode() {
return this.exitCode;
}
/**
*
* The exit code returned from the container.
*
*
* @param exitCode
* The exit code returned from the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Container withExitCode(Integer exitCode) {
setExitCode(exitCode);
return this;
}
/**
*
* A short (255 max characters) human-readable string to provide additional details about a running or stopped
* container.
*
*
* @param reason
* A short (255 max characters) human-readable string to provide additional details about a running or
* stopped container.
*/
public void setReason(String reason) {
this.reason = reason;
}
/**
*
* A short (255 max characters) human-readable string to provide additional details about a running or stopped
* container.
*
*
* @return A short (255 max characters) human-readable string to provide additional details about a running or
* stopped container.
*/
public String getReason() {
return this.reason;
}
/**
*
* A short (255 max characters) human-readable string to provide additional details about a running or stopped
* container.
*
*
* @param reason
* A short (255 max characters) human-readable string to provide additional details about a running or
* stopped container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Container withReason(String reason) {
setReason(reason);
return this;
}
/**
*
* The network bindings associated with the container.
*
*
* @return The network bindings associated with the container.
*/
public java.util.List getNetworkBindings() {
if (networkBindings == null) {
networkBindings = new com.amazonaws.internal.SdkInternalList();
}
return networkBindings;
}
/**
*
* The network bindings associated with the container.
*
*
* @param networkBindings
* The network bindings associated with the container.
*/
public void setNetworkBindings(java.util.Collection networkBindings) {
if (networkBindings == null) {
this.networkBindings = null;
return;
}
this.networkBindings = new com.amazonaws.internal.SdkInternalList(networkBindings);
}
/**
*
* The network bindings associated with the container.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setNetworkBindings(java.util.Collection)} or {@link #withNetworkBindings(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param networkBindings
* The network bindings associated with the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Container withNetworkBindings(NetworkBinding... networkBindings) {
if (this.networkBindings == null) {
setNetworkBindings(new com.amazonaws.internal.SdkInternalList(networkBindings.length));
}
for (NetworkBinding ele : networkBindings) {
this.networkBindings.add(ele);
}
return this;
}
/**
*
* The network bindings associated with the container.
*
*
* @param networkBindings
* The network bindings associated with the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Container withNetworkBindings(java.util.Collection networkBindings) {
setNetworkBindings(networkBindings);
return this;
}
/**
*
* The network interfaces associated with the container.
*
*
* @return The network interfaces associated with the container.
*/
public java.util.List getNetworkInterfaces() {
if (networkInterfaces == null) {
networkInterfaces = new com.amazonaws.internal.SdkInternalList();
}
return networkInterfaces;
}
/**
*
* The network interfaces associated with the container.
*
*
* @param networkInterfaces
* The network interfaces associated with the container.
*/
public void setNetworkInterfaces(java.util.Collection networkInterfaces) {
if (networkInterfaces == null) {
this.networkInterfaces = null;
return;
}
this.networkInterfaces = new com.amazonaws.internal.SdkInternalList(networkInterfaces);
}
/**
*
* The network interfaces associated with the container.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setNetworkInterfaces(java.util.Collection)} or {@link #withNetworkInterfaces(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param networkInterfaces
* The network interfaces associated with the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Container withNetworkInterfaces(NetworkInterface... networkInterfaces) {
if (this.networkInterfaces == null) {
setNetworkInterfaces(new com.amazonaws.internal.SdkInternalList(networkInterfaces.length));
}
for (NetworkInterface ele : networkInterfaces) {
this.networkInterfaces.add(ele);
}
return this;
}
/**
*
* The network interfaces associated with the container.
*
*
* @param networkInterfaces
* The network interfaces associated with the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Container withNetworkInterfaces(java.util.Collection networkInterfaces) {
setNetworkInterfaces(networkInterfaces);
return this;
}
/**
*
* The health status of the container. If health checks aren't configured for this container in its task definition,
* then it reports the health status as UNKNOWN
.
*
*
* @param healthStatus
* The health status of the container. If health checks aren't configured for this container in its task
* definition, then it reports the health status as UNKNOWN
.
* @see HealthStatus
*/
public void setHealthStatus(String healthStatus) {
this.healthStatus = healthStatus;
}
/**
*
* The health status of the container. If health checks aren't configured for this container in its task definition,
* then it reports the health status as UNKNOWN
.
*
*
* @return The health status of the container. If health checks aren't configured for this container in its task
* definition, then it reports the health status as UNKNOWN
.
* @see HealthStatus
*/
public String getHealthStatus() {
return this.healthStatus;
}
/**
*
* The health status of the container. If health checks aren't configured for this container in its task definition,
* then it reports the health status as UNKNOWN
.
*
*
* @param healthStatus
* The health status of the container. If health checks aren't configured for this container in its task
* definition, then it reports the health status as UNKNOWN
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see HealthStatus
*/
public Container withHealthStatus(String healthStatus) {
setHealthStatus(healthStatus);
return this;
}
/**
*
* The health status of the container. If health checks aren't configured for this container in its task definition,
* then it reports the health status as UNKNOWN
.
*
*
* @param healthStatus
* The health status of the container. If health checks aren't configured for this container in its task
* definition, then it reports the health status as UNKNOWN
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see HealthStatus
*/
public Container withHealthStatus(HealthStatus healthStatus) {
this.healthStatus = healthStatus.toString();
return this;
}
/**
*
* The details of any Amazon ECS managed agents associated with the container.
*
*
* @return The details of any Amazon ECS managed agents associated with the container.
*/
public java.util.List getManagedAgents() {
if (managedAgents == null) {
managedAgents = new com.amazonaws.internal.SdkInternalList();
}
return managedAgents;
}
/**
*
* The details of any Amazon ECS managed agents associated with the container.
*
*
* @param managedAgents
* The details of any Amazon ECS managed agents associated with the container.
*/
public void setManagedAgents(java.util.Collection managedAgents) {
if (managedAgents == null) {
this.managedAgents = null;
return;
}
this.managedAgents = new com.amazonaws.internal.SdkInternalList(managedAgents);
}
/**
*
* The details of any Amazon ECS managed agents associated with the container.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setManagedAgents(java.util.Collection)} or {@link #withManagedAgents(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param managedAgents
* The details of any Amazon ECS managed agents associated with the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Container withManagedAgents(ManagedAgent... managedAgents) {
if (this.managedAgents == null) {
setManagedAgents(new com.amazonaws.internal.SdkInternalList(managedAgents.length));
}
for (ManagedAgent ele : managedAgents) {
this.managedAgents.add(ele);
}
return this;
}
/**
*
* The details of any Amazon ECS managed agents associated with the container.
*
*
* @param managedAgents
* The details of any Amazon ECS managed agents associated with the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Container withManagedAgents(java.util.Collection managedAgents) {
setManagedAgents(managedAgents);
return this;
}
/**
*
* The number of CPU units set for the container. The value is 0
if no value was specified in the
* container definition when the task definition was registered.
*
*
* @param cpu
* The number of CPU units set for the container. The value is 0
if no value was specified in
* the container definition when the task definition was registered.
*/
public void setCpu(String cpu) {
this.cpu = cpu;
}
/**
*
* The number of CPU units set for the container. The value is 0
if no value was specified in the
* container definition when the task definition was registered.
*
*
* @return The number of CPU units set for the container. The value is 0
if no value was specified in
* the container definition when the task definition was registered.
*/
public String getCpu() {
return this.cpu;
}
/**
*
* The number of CPU units set for the container. The value is 0
if no value was specified in the
* container definition when the task definition was registered.
*
*
* @param cpu
* The number of CPU units set for the container. The value is 0
if no value was specified in
* the container definition when the task definition was registered.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Container withCpu(String cpu) {
setCpu(cpu);
return this;
}
/**
*
* The hard limit (in MiB) of memory set for the container.
*
*
* @param memory
* The hard limit (in MiB) of memory set for the container.
*/
public void setMemory(String memory) {
this.memory = memory;
}
/**
*
* The hard limit (in MiB) of memory set for the container.
*
*
* @return The hard limit (in MiB) of memory set for the container.
*/
public String getMemory() {
return this.memory;
}
/**
*
* The hard limit (in MiB) of memory set for the container.
*
*
* @param memory
* The hard limit (in MiB) of memory set for the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Container withMemory(String memory) {
setMemory(memory);
return this;
}
/**
*
* The soft limit (in MiB) of memory set for the container.
*
*
* @param memoryReservation
* The soft limit (in MiB) of memory set for the container.
*/
public void setMemoryReservation(String memoryReservation) {
this.memoryReservation = memoryReservation;
}
/**
*
* The soft limit (in MiB) of memory set for the container.
*
*
* @return The soft limit (in MiB) of memory set for the container.
*/
public String getMemoryReservation() {
return this.memoryReservation;
}
/**
*
* The soft limit (in MiB) of memory set for the container.
*
*
* @param memoryReservation
* The soft limit (in MiB) of memory set for the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Container withMemoryReservation(String memoryReservation) {
setMemoryReservation(memoryReservation);
return this;
}
/**
*
* The IDs of each GPU assigned to the container.
*
*
* @return The IDs of each GPU assigned to the container.
*/
public java.util.List getGpuIds() {
if (gpuIds == null) {
gpuIds = new com.amazonaws.internal.SdkInternalList();
}
return gpuIds;
}
/**
*
* The IDs of each GPU assigned to the container.
*
*
* @param gpuIds
* The IDs of each GPU assigned to the container.
*/
public void setGpuIds(java.util.Collection gpuIds) {
if (gpuIds == null) {
this.gpuIds = null;
return;
}
this.gpuIds = new com.amazonaws.internal.SdkInternalList(gpuIds);
}
/**
*
* The IDs of each GPU assigned to the container.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setGpuIds(java.util.Collection)} or {@link #withGpuIds(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param gpuIds
* The IDs of each GPU assigned to the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Container withGpuIds(String... gpuIds) {
if (this.gpuIds == null) {
setGpuIds(new com.amazonaws.internal.SdkInternalList(gpuIds.length));
}
for (String ele : gpuIds) {
this.gpuIds.add(ele);
}
return this;
}
/**
*
* The IDs of each GPU assigned to the container.
*
*
* @param gpuIds
* The IDs of each GPU assigned to the container.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Container withGpuIds(java.util.Collection gpuIds) {
setGpuIds(gpuIds);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getContainerArn() != null)
sb.append("ContainerArn: ").append(getContainerArn()).append(",");
if (getTaskArn() != null)
sb.append("TaskArn: ").append(getTaskArn()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getImage() != null)
sb.append("Image: ").append(getImage()).append(",");
if (getImageDigest() != null)
sb.append("ImageDigest: ").append(getImageDigest()).append(",");
if (getRuntimeId() != null)
sb.append("RuntimeId: ").append(getRuntimeId()).append(",");
if (getLastStatus() != null)
sb.append("LastStatus: ").append(getLastStatus()).append(",");
if (getExitCode() != null)
sb.append("ExitCode: ").append(getExitCode()).append(",");
if (getReason() != null)
sb.append("Reason: ").append(getReason()).append(",");
if (getNetworkBindings() != null)
sb.append("NetworkBindings: ").append(getNetworkBindings()).append(",");
if (getNetworkInterfaces() != null)
sb.append("NetworkInterfaces: ").append(getNetworkInterfaces()).append(",");
if (getHealthStatus() != null)
sb.append("HealthStatus: ").append(getHealthStatus()).append(",");
if (getManagedAgents() != null)
sb.append("ManagedAgents: ").append(getManagedAgents()).append(",");
if (getCpu() != null)
sb.append("Cpu: ").append(getCpu()).append(",");
if (getMemory() != null)
sb.append("Memory: ").append(getMemory()).append(",");
if (getMemoryReservation() != null)
sb.append("MemoryReservation: ").append(getMemoryReservation()).append(",");
if (getGpuIds() != null)
sb.append("GpuIds: ").append(getGpuIds());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Container == false)
return false;
Container other = (Container) obj;
if (other.getContainerArn() == null ^ this.getContainerArn() == null)
return false;
if (other.getContainerArn() != null && other.getContainerArn().equals(this.getContainerArn()) == false)
return false;
if (other.getTaskArn() == null ^ this.getTaskArn() == null)
return false;
if (other.getTaskArn() != null && other.getTaskArn().equals(this.getTaskArn()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getImage() == null ^ this.getImage() == null)
return false;
if (other.getImage() != null && other.getImage().equals(this.getImage()) == false)
return false;
if (other.getImageDigest() == null ^ this.getImageDigest() == null)
return false;
if (other.getImageDigest() != null && other.getImageDigest().equals(this.getImageDigest()) == false)
return false;
if (other.getRuntimeId() == null ^ this.getRuntimeId() == null)
return false;
if (other.getRuntimeId() != null && other.getRuntimeId().equals(this.getRuntimeId()) == false)
return false;
if (other.getLastStatus() == null ^ this.getLastStatus() == null)
return false;
if (other.getLastStatus() != null && other.getLastStatus().equals(this.getLastStatus()) == false)
return false;
if (other.getExitCode() == null ^ this.getExitCode() == null)
return false;
if (other.getExitCode() != null && other.getExitCode().equals(this.getExitCode()) == false)
return false;
if (other.getReason() == null ^ this.getReason() == null)
return false;
if (other.getReason() != null && other.getReason().equals(this.getReason()) == false)
return false;
if (other.getNetworkBindings() == null ^ this.getNetworkBindings() == null)
return false;
if (other.getNetworkBindings() != null && other.getNetworkBindings().equals(this.getNetworkBindings()) == false)
return false;
if (other.getNetworkInterfaces() == null ^ this.getNetworkInterfaces() == null)
return false;
if (other.getNetworkInterfaces() != null && other.getNetworkInterfaces().equals(this.getNetworkInterfaces()) == false)
return false;
if (other.getHealthStatus() == null ^ this.getHealthStatus() == null)
return false;
if (other.getHealthStatus() != null && other.getHealthStatus().equals(this.getHealthStatus()) == false)
return false;
if (other.getManagedAgents() == null ^ this.getManagedAgents() == null)
return false;
if (other.getManagedAgents() != null && other.getManagedAgents().equals(this.getManagedAgents()) == false)
return false;
if (other.getCpu() == null ^ this.getCpu() == null)
return false;
if (other.getCpu() != null && other.getCpu().equals(this.getCpu()) == false)
return false;
if (other.getMemory() == null ^ this.getMemory() == null)
return false;
if (other.getMemory() != null && other.getMemory().equals(this.getMemory()) == false)
return false;
if (other.getMemoryReservation() == null ^ this.getMemoryReservation() == null)
return false;
if (other.getMemoryReservation() != null && other.getMemoryReservation().equals(this.getMemoryReservation()) == false)
return false;
if (other.getGpuIds() == null ^ this.getGpuIds() == null)
return false;
if (other.getGpuIds() != null && other.getGpuIds().equals(this.getGpuIds()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getContainerArn() == null) ? 0 : getContainerArn().hashCode());
hashCode = prime * hashCode + ((getTaskArn() == null) ? 0 : getTaskArn().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getImage() == null) ? 0 : getImage().hashCode());
hashCode = prime * hashCode + ((getImageDigest() == null) ? 0 : getImageDigest().hashCode());
hashCode = prime * hashCode + ((getRuntimeId() == null) ? 0 : getRuntimeId().hashCode());
hashCode = prime * hashCode + ((getLastStatus() == null) ? 0 : getLastStatus().hashCode());
hashCode = prime * hashCode + ((getExitCode() == null) ? 0 : getExitCode().hashCode());
hashCode = prime * hashCode + ((getReason() == null) ? 0 : getReason().hashCode());
hashCode = prime * hashCode + ((getNetworkBindings() == null) ? 0 : getNetworkBindings().hashCode());
hashCode = prime * hashCode + ((getNetworkInterfaces() == null) ? 0 : getNetworkInterfaces().hashCode());
hashCode = prime * hashCode + ((getHealthStatus() == null) ? 0 : getHealthStatus().hashCode());
hashCode = prime * hashCode + ((getManagedAgents() == null) ? 0 : getManagedAgents().hashCode());
hashCode = prime * hashCode + ((getCpu() == null) ? 0 : getCpu().hashCode());
hashCode = prime * hashCode + ((getMemory() == null) ? 0 : getMemory().hashCode());
hashCode = prime * hashCode + ((getMemoryReservation() == null) ? 0 : getMemoryReservation().hashCode());
hashCode = prime * hashCode + ((getGpuIds() == null) ? 0 : getGpuIds().hashCode());
return hashCode;
}
@Override
public Container clone() {
try {
return (Container) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.ecs.model.transform.ContainerMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}