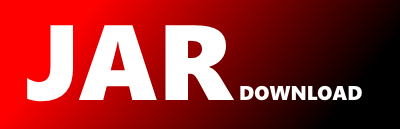
com.amazonaws.services.ecs.model.LinuxParameters Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ecs Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ecs.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The Linux-specific options that are applied to the container, such as Linux KernelCapabilities.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class LinuxParameters implements Serializable, Cloneable, StructuredPojo {
/**
*
* The Linux capabilities for the container that are added to or dropped from the default configuration provided by
* Docker.
*
*
*
* For tasks that use the Fargate launch type, capabilities
is supported for all platform versions but
* the add
parameter is only supported if using platform version 1.4.0 or later.
*
*
*/
private KernelCapabilities capabilities;
/**
*
* Any host devices to expose to the container. This parameter maps to Devices
in the Create a container section of the
* Docker Remote API and the --device
option to
* docker run.
*
*
*
* If you're using tasks that use the Fargate launch type, the devices
parameter isn't supported.
*
*
*/
private com.amazonaws.internal.SdkInternalList devices;
/**
*
* Run an init
process inside the container that forwards signals and reaps processes. This parameter
* maps to the --init
option to docker run. This parameter
* requires version 1.25 of the Docker Remote API or greater on your container instance. To check the Docker Remote
* API version on your container instance, log in to your container instance and run the following command:
* sudo docker version --format '{{.Server.APIVersion}}'
*
*/
private Boolean initProcessEnabled;
/**
*
* The value for the size (in MiB) of the /dev/shm
volume. This parameter maps to the
* --shm-size
option to docker run.
*
*
*
* If you are using tasks that use the Fargate launch type, the sharedMemorySize
parameter is not
* supported.
*
*
*/
private Integer sharedMemorySize;
/**
*
* The container path, mount options, and size (in MiB) of the tmpfs mount. This parameter maps to the
* --tmpfs
option to docker run.
*
*
*
* If you're using tasks that use the Fargate launch type, the tmpfs
parameter isn't supported.
*
*
*/
private com.amazonaws.internal.SdkInternalList tmpfs;
/**
*
* The total amount of swap memory (in MiB) a container can use. This parameter will be translated to the
* --memory-swap
option to docker run where the value would
* be the sum of the container memory plus the maxSwap
value.
*
*
* If a maxSwap
value of 0
is specified, the container will not use swap. Accepted values
* are 0
or any positive integer. If the maxSwap
parameter is omitted, the container will
* use the swap configuration for the container instance it is running on. A maxSwap
value must be set
* for the swappiness
parameter to be used.
*
*
*
* If you're using tasks that use the Fargate launch type, the maxSwap
parameter isn't supported.
*
*
* If you're using tasks on Amazon Linux 2023 the swappiness
parameter isn't supported.
*
*
*/
private Integer maxSwap;
/**
*
* This allows you to tune a container's memory swappiness behavior. A swappiness
value of
* 0
will cause swapping to not happen unless absolutely necessary. A swappiness
value of
* 100
will cause pages to be swapped very aggressively. Accepted values are whole numbers between
* 0
and 100
. If the swappiness
parameter is not specified, a default value
* of 60
is used. If a value is not specified for maxSwap
then this parameter is ignored.
* This parameter maps to the --memory-swappiness
option to docker run.
*
*
*
* If you're using tasks that use the Fargate launch type, the swappiness
parameter isn't supported.
*
*
* If you're using tasks on Amazon Linux 2023 the swappiness
parameter isn't supported.
*
*
*/
private Integer swappiness;
/**
*
* The Linux capabilities for the container that are added to or dropped from the default configuration provided by
* Docker.
*
*
*
* For tasks that use the Fargate launch type, capabilities
is supported for all platform versions but
* the add
parameter is only supported if using platform version 1.4.0 or later.
*
*
*
* @param capabilities
* The Linux capabilities for the container that are added to or dropped from the default configuration
* provided by Docker.
*
* For tasks that use the Fargate launch type, capabilities
is supported for all platform
* versions but the add
parameter is only supported if using platform version 1.4.0 or later.
*
*/
public void setCapabilities(KernelCapabilities capabilities) {
this.capabilities = capabilities;
}
/**
*
* The Linux capabilities for the container that are added to or dropped from the default configuration provided by
* Docker.
*
*
*
* For tasks that use the Fargate launch type, capabilities
is supported for all platform versions but
* the add
parameter is only supported if using platform version 1.4.0 or later.
*
*
*
* @return The Linux capabilities for the container that are added to or dropped from the default configuration
* provided by Docker.
*
* For tasks that use the Fargate launch type, capabilities
is supported for all platform
* versions but the add
parameter is only supported if using platform version 1.4.0 or later.
*
*/
public KernelCapabilities getCapabilities() {
return this.capabilities;
}
/**
*
* The Linux capabilities for the container that are added to or dropped from the default configuration provided by
* Docker.
*
*
*
* For tasks that use the Fargate launch type, capabilities
is supported for all platform versions but
* the add
parameter is only supported if using platform version 1.4.0 or later.
*
*
*
* @param capabilities
* The Linux capabilities for the container that are added to or dropped from the default configuration
* provided by Docker.
*
* For tasks that use the Fargate launch type, capabilities
is supported for all platform
* versions but the add
parameter is only supported if using platform version 1.4.0 or later.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LinuxParameters withCapabilities(KernelCapabilities capabilities) {
setCapabilities(capabilities);
return this;
}
/**
*
* Any host devices to expose to the container. This parameter maps to Devices
in the Create a container section of the
* Docker Remote API and the --device
option to
* docker run.
*
*
*
* If you're using tasks that use the Fargate launch type, the devices
parameter isn't supported.
*
*
*
* @return Any host devices to expose to the container. This parameter maps to Devices
in the Create a container section
* of the Docker Remote API and the
* --device
option to docker run.
*
* If you're using tasks that use the Fargate launch type, the devices
parameter isn't
* supported.
*
*/
public java.util.List getDevices() {
if (devices == null) {
devices = new com.amazonaws.internal.SdkInternalList();
}
return devices;
}
/**
*
* Any host devices to expose to the container. This parameter maps to Devices
in the Create a container section of the
* Docker Remote API and the --device
option to
* docker run.
*
*
*
* If you're using tasks that use the Fargate launch type, the devices
parameter isn't supported.
*
*
*
* @param devices
* Any host devices to expose to the container. This parameter maps to Devices
in the Create a container section
* of the Docker Remote API and the
* --device
option to docker run.
*
* If you're using tasks that use the Fargate launch type, the devices
parameter isn't
* supported.
*
*/
public void setDevices(java.util.Collection devices) {
if (devices == null) {
this.devices = null;
return;
}
this.devices = new com.amazonaws.internal.SdkInternalList(devices);
}
/**
*
* Any host devices to expose to the container. This parameter maps to Devices
in the Create a container section of the
* Docker Remote API and the --device
option to
* docker run.
*
*
*
* If you're using tasks that use the Fargate launch type, the devices
parameter isn't supported.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDevices(java.util.Collection)} or {@link #withDevices(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param devices
* Any host devices to expose to the container. This parameter maps to Devices
in the Create a container section
* of the Docker Remote API and the
* --device
option to docker run.
*
* If you're using tasks that use the Fargate launch type, the devices
parameter isn't
* supported.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LinuxParameters withDevices(Device... devices) {
if (this.devices == null) {
setDevices(new com.amazonaws.internal.SdkInternalList(devices.length));
}
for (Device ele : devices) {
this.devices.add(ele);
}
return this;
}
/**
*
* Any host devices to expose to the container. This parameter maps to Devices
in the Create a container section of the
* Docker Remote API and the --device
option to
* docker run.
*
*
*
* If you're using tasks that use the Fargate launch type, the devices
parameter isn't supported.
*
*
*
* @param devices
* Any host devices to expose to the container. This parameter maps to Devices
in the Create a container section
* of the Docker Remote API and the
* --device
option to docker run.
*
* If you're using tasks that use the Fargate launch type, the devices
parameter isn't
* supported.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LinuxParameters withDevices(java.util.Collection devices) {
setDevices(devices);
return this;
}
/**
*
* Run an init
process inside the container that forwards signals and reaps processes. This parameter
* maps to the --init
option to docker run. This parameter
* requires version 1.25 of the Docker Remote API or greater on your container instance. To check the Docker Remote
* API version on your container instance, log in to your container instance and run the following command:
* sudo docker version --format '{{.Server.APIVersion}}'
*
*
* @param initProcessEnabled
* Run an init
process inside the container that forwards signals and reaps processes. This
* parameter maps to the --init
option to docker run. This parameter
* requires version 1.25 of the Docker Remote API or greater on your container instance. To check the Docker
* Remote API version on your container instance, log in to your container instance and run the following
* command: sudo docker version --format '{{.Server.APIVersion}}'
*/
public void setInitProcessEnabled(Boolean initProcessEnabled) {
this.initProcessEnabled = initProcessEnabled;
}
/**
*
* Run an init
process inside the container that forwards signals and reaps processes. This parameter
* maps to the --init
option to docker run. This parameter
* requires version 1.25 of the Docker Remote API or greater on your container instance. To check the Docker Remote
* API version on your container instance, log in to your container instance and run the following command:
* sudo docker version --format '{{.Server.APIVersion}}'
*
*
* @return Run an init
process inside the container that forwards signals and reaps processes. This
* parameter maps to the --init
option to docker run. This
* parameter requires version 1.25 of the Docker Remote API or greater on your container instance. To check
* the Docker Remote API version on your container instance, log in to your container instance and run the
* following command: sudo docker version --format '{{.Server.APIVersion}}'
*/
public Boolean getInitProcessEnabled() {
return this.initProcessEnabled;
}
/**
*
* Run an init
process inside the container that forwards signals and reaps processes. This parameter
* maps to the --init
option to docker run. This parameter
* requires version 1.25 of the Docker Remote API or greater on your container instance. To check the Docker Remote
* API version on your container instance, log in to your container instance and run the following command:
* sudo docker version --format '{{.Server.APIVersion}}'
*
*
* @param initProcessEnabled
* Run an init
process inside the container that forwards signals and reaps processes. This
* parameter maps to the --init
option to docker run. This parameter
* requires version 1.25 of the Docker Remote API or greater on your container instance. To check the Docker
* Remote API version on your container instance, log in to your container instance and run the following
* command: sudo docker version --format '{{.Server.APIVersion}}'
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LinuxParameters withInitProcessEnabled(Boolean initProcessEnabled) {
setInitProcessEnabled(initProcessEnabled);
return this;
}
/**
*
* Run an init
process inside the container that forwards signals and reaps processes. This parameter
* maps to the --init
option to docker run. This parameter
* requires version 1.25 of the Docker Remote API or greater on your container instance. To check the Docker Remote
* API version on your container instance, log in to your container instance and run the following command:
* sudo docker version --format '{{.Server.APIVersion}}'
*
*
* @return Run an init
process inside the container that forwards signals and reaps processes. This
* parameter maps to the --init
option to docker run. This
* parameter requires version 1.25 of the Docker Remote API or greater on your container instance. To check
* the Docker Remote API version on your container instance, log in to your container instance and run the
* following command: sudo docker version --format '{{.Server.APIVersion}}'
*/
public Boolean isInitProcessEnabled() {
return this.initProcessEnabled;
}
/**
*
* The value for the size (in MiB) of the /dev/shm
volume. This parameter maps to the
* --shm-size
option to docker run.
*
*
*
* If you are using tasks that use the Fargate launch type, the sharedMemorySize
parameter is not
* supported.
*
*
*
* @param sharedMemorySize
* The value for the size (in MiB) of the /dev/shm
volume. This parameter maps to the
* --shm-size
option to docker run.
*
* If you are using tasks that use the Fargate launch type, the sharedMemorySize
parameter is
* not supported.
*
*/
public void setSharedMemorySize(Integer sharedMemorySize) {
this.sharedMemorySize = sharedMemorySize;
}
/**
*
* The value for the size (in MiB) of the /dev/shm
volume. This parameter maps to the
* --shm-size
option to docker run.
*
*
*
* If you are using tasks that use the Fargate launch type, the sharedMemorySize
parameter is not
* supported.
*
*
*
* @return The value for the size (in MiB) of the /dev/shm
volume. This parameter maps to the
* --shm-size
option to docker run.
*
* If you are using tasks that use the Fargate launch type, the sharedMemorySize
parameter is
* not supported.
*
*/
public Integer getSharedMemorySize() {
return this.sharedMemorySize;
}
/**
*
* The value for the size (in MiB) of the /dev/shm
volume. This parameter maps to the
* --shm-size
option to docker run.
*
*
*
* If you are using tasks that use the Fargate launch type, the sharedMemorySize
parameter is not
* supported.
*
*
*
* @param sharedMemorySize
* The value for the size (in MiB) of the /dev/shm
volume. This parameter maps to the
* --shm-size
option to docker run.
*
* If you are using tasks that use the Fargate launch type, the sharedMemorySize
parameter is
* not supported.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LinuxParameters withSharedMemorySize(Integer sharedMemorySize) {
setSharedMemorySize(sharedMemorySize);
return this;
}
/**
*
* The container path, mount options, and size (in MiB) of the tmpfs mount. This parameter maps to the
* --tmpfs
option to docker run.
*
*
*
* If you're using tasks that use the Fargate launch type, the tmpfs
parameter isn't supported.
*
*
*
* @return The container path, mount options, and size (in MiB) of the tmpfs mount. This parameter maps to the
* --tmpfs
option to docker run.
*
* If you're using tasks that use the Fargate launch type, the tmpfs
parameter isn't supported.
*
*/
public java.util.List getTmpfs() {
if (tmpfs == null) {
tmpfs = new com.amazonaws.internal.SdkInternalList();
}
return tmpfs;
}
/**
*
* The container path, mount options, and size (in MiB) of the tmpfs mount. This parameter maps to the
* --tmpfs
option to docker run.
*
*
*
* If you're using tasks that use the Fargate launch type, the tmpfs
parameter isn't supported.
*
*
*
* @param tmpfs
* The container path, mount options, and size (in MiB) of the tmpfs mount. This parameter maps to the
* --tmpfs
option to docker run.
*
* If you're using tasks that use the Fargate launch type, the tmpfs
parameter isn't supported.
*
*/
public void setTmpfs(java.util.Collection tmpfs) {
if (tmpfs == null) {
this.tmpfs = null;
return;
}
this.tmpfs = new com.amazonaws.internal.SdkInternalList(tmpfs);
}
/**
*
* The container path, mount options, and size (in MiB) of the tmpfs mount. This parameter maps to the
* --tmpfs
option to docker run.
*
*
*
* If you're using tasks that use the Fargate launch type, the tmpfs
parameter isn't supported.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTmpfs(java.util.Collection)} or {@link #withTmpfs(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tmpfs
* The container path, mount options, and size (in MiB) of the tmpfs mount. This parameter maps to the
* --tmpfs
option to docker run.
*
* If you're using tasks that use the Fargate launch type, the tmpfs
parameter isn't supported.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LinuxParameters withTmpfs(Tmpfs... tmpfs) {
if (this.tmpfs == null) {
setTmpfs(new com.amazonaws.internal.SdkInternalList(tmpfs.length));
}
for (Tmpfs ele : tmpfs) {
this.tmpfs.add(ele);
}
return this;
}
/**
*
* The container path, mount options, and size (in MiB) of the tmpfs mount. This parameter maps to the
* --tmpfs
option to docker run.
*
*
*
* If you're using tasks that use the Fargate launch type, the tmpfs
parameter isn't supported.
*
*
*
* @param tmpfs
* The container path, mount options, and size (in MiB) of the tmpfs mount. This parameter maps to the
* --tmpfs
option to docker run.
*
* If you're using tasks that use the Fargate launch type, the tmpfs
parameter isn't supported.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LinuxParameters withTmpfs(java.util.Collection tmpfs) {
setTmpfs(tmpfs);
return this;
}
/**
*
* The total amount of swap memory (in MiB) a container can use. This parameter will be translated to the
* --memory-swap
option to docker run where the value would
* be the sum of the container memory plus the maxSwap
value.
*
*
* If a maxSwap
value of 0
is specified, the container will not use swap. Accepted values
* are 0
or any positive integer. If the maxSwap
parameter is omitted, the container will
* use the swap configuration for the container instance it is running on. A maxSwap
value must be set
* for the swappiness
parameter to be used.
*
*
*
* If you're using tasks that use the Fargate launch type, the maxSwap
parameter isn't supported.
*
*
* If you're using tasks on Amazon Linux 2023 the swappiness
parameter isn't supported.
*
*
*
* @param maxSwap
* The total amount of swap memory (in MiB) a container can use. This parameter will be translated to the
* --memory-swap
option to docker run where the value
* would be the sum of the container memory plus the maxSwap
value.
*
* If a maxSwap
value of 0
is specified, the container will not use swap. Accepted
* values are 0
or any positive integer. If the maxSwap
parameter is omitted, the
* container will use the swap configuration for the container instance it is running on. A
* maxSwap
value must be set for the swappiness
parameter to be used.
*
*
*
* If you're using tasks that use the Fargate launch type, the maxSwap
parameter isn't
* supported.
*
*
* If you're using tasks on Amazon Linux 2023 the swappiness
parameter isn't supported.
*
*/
public void setMaxSwap(Integer maxSwap) {
this.maxSwap = maxSwap;
}
/**
*
* The total amount of swap memory (in MiB) a container can use. This parameter will be translated to the
* --memory-swap
option to docker run where the value would
* be the sum of the container memory plus the maxSwap
value.
*
*
* If a maxSwap
value of 0
is specified, the container will not use swap. Accepted values
* are 0
or any positive integer. If the maxSwap
parameter is omitted, the container will
* use the swap configuration for the container instance it is running on. A maxSwap
value must be set
* for the swappiness
parameter to be used.
*
*
*
* If you're using tasks that use the Fargate launch type, the maxSwap
parameter isn't supported.
*
*
* If you're using tasks on Amazon Linux 2023 the swappiness
parameter isn't supported.
*
*
*
* @return The total amount of swap memory (in MiB) a container can use. This parameter will be translated to the
* --memory-swap
option to docker run where the
* value would be the sum of the container memory plus the maxSwap
value.
*
* If a maxSwap
value of 0
is specified, the container will not use swap. Accepted
* values are 0
or any positive integer. If the maxSwap
parameter is omitted, the
* container will use the swap configuration for the container instance it is running on. A
* maxSwap
value must be set for the swappiness
parameter to be used.
*
*
*
* If you're using tasks that use the Fargate launch type, the maxSwap
parameter isn't
* supported.
*
*
* If you're using tasks on Amazon Linux 2023 the swappiness
parameter isn't supported.
*
*/
public Integer getMaxSwap() {
return this.maxSwap;
}
/**
*
* The total amount of swap memory (in MiB) a container can use. This parameter will be translated to the
* --memory-swap
option to docker run where the value would
* be the sum of the container memory plus the maxSwap
value.
*
*
* If a maxSwap
value of 0
is specified, the container will not use swap. Accepted values
* are 0
or any positive integer. If the maxSwap
parameter is omitted, the container will
* use the swap configuration for the container instance it is running on. A maxSwap
value must be set
* for the swappiness
parameter to be used.
*
*
*
* If you're using tasks that use the Fargate launch type, the maxSwap
parameter isn't supported.
*
*
* If you're using tasks on Amazon Linux 2023 the swappiness
parameter isn't supported.
*
*
*
* @param maxSwap
* The total amount of swap memory (in MiB) a container can use. This parameter will be translated to the
* --memory-swap
option to docker run where the value
* would be the sum of the container memory plus the maxSwap
value.
*
* If a maxSwap
value of 0
is specified, the container will not use swap. Accepted
* values are 0
or any positive integer. If the maxSwap
parameter is omitted, the
* container will use the swap configuration for the container instance it is running on. A
* maxSwap
value must be set for the swappiness
parameter to be used.
*
*
*
* If you're using tasks that use the Fargate launch type, the maxSwap
parameter isn't
* supported.
*
*
* If you're using tasks on Amazon Linux 2023 the swappiness
parameter isn't supported.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LinuxParameters withMaxSwap(Integer maxSwap) {
setMaxSwap(maxSwap);
return this;
}
/**
*
* This allows you to tune a container's memory swappiness behavior. A swappiness
value of
* 0
will cause swapping to not happen unless absolutely necessary. A swappiness
value of
* 100
will cause pages to be swapped very aggressively. Accepted values are whole numbers between
* 0
and 100
. If the swappiness
parameter is not specified, a default value
* of 60
is used. If a value is not specified for maxSwap
then this parameter is ignored.
* This parameter maps to the --memory-swappiness
option to docker run.
*
*
*
* If you're using tasks that use the Fargate launch type, the swappiness
parameter isn't supported.
*
*
* If you're using tasks on Amazon Linux 2023 the swappiness
parameter isn't supported.
*
*
*
* @param swappiness
* This allows you to tune a container's memory swappiness behavior. A swappiness
value of
* 0
will cause swapping to not happen unless absolutely necessary. A swappiness
* value of 100
will cause pages to be swapped very aggressively. Accepted values are whole
* numbers between 0
and 100
. If the swappiness
parameter is not
* specified, a default value of 60
is used. If a value is not specified for
* maxSwap
then this parameter is ignored. This parameter maps to the
* --memory-swappiness
option to docker run.
*
* If you're using tasks that use the Fargate launch type, the swappiness
parameter isn't
* supported.
*
*
* If you're using tasks on Amazon Linux 2023 the swappiness
parameter isn't supported.
*
*/
public void setSwappiness(Integer swappiness) {
this.swappiness = swappiness;
}
/**
*
* This allows you to tune a container's memory swappiness behavior. A swappiness
value of
* 0
will cause swapping to not happen unless absolutely necessary. A swappiness
value of
* 100
will cause pages to be swapped very aggressively. Accepted values are whole numbers between
* 0
and 100
. If the swappiness
parameter is not specified, a default value
* of 60
is used. If a value is not specified for maxSwap
then this parameter is ignored.
* This parameter maps to the --memory-swappiness
option to docker run.
*
*
*
* If you're using tasks that use the Fargate launch type, the swappiness
parameter isn't supported.
*
*
* If you're using tasks on Amazon Linux 2023 the swappiness
parameter isn't supported.
*
*
*
* @return This allows you to tune a container's memory swappiness behavior. A swappiness
value of
* 0
will cause swapping to not happen unless absolutely necessary. A swappiness
* value of 100
will cause pages to be swapped very aggressively. Accepted values are whole
* numbers between 0
and 100
. If the swappiness
parameter is not
* specified, a default value of 60
is used. If a value is not specified for
* maxSwap
then this parameter is ignored. This parameter maps to the
* --memory-swappiness
option to docker run.
*
* If you're using tasks that use the Fargate launch type, the swappiness
parameter isn't
* supported.
*
*
* If you're using tasks on Amazon Linux 2023 the swappiness
parameter isn't supported.
*
*/
public Integer getSwappiness() {
return this.swappiness;
}
/**
*
* This allows you to tune a container's memory swappiness behavior. A swappiness
value of
* 0
will cause swapping to not happen unless absolutely necessary. A swappiness
value of
* 100
will cause pages to be swapped very aggressively. Accepted values are whole numbers between
* 0
and 100
. If the swappiness
parameter is not specified, a default value
* of 60
is used. If a value is not specified for maxSwap
then this parameter is ignored.
* This parameter maps to the --memory-swappiness
option to docker run.
*
*
*
* If you're using tasks that use the Fargate launch type, the swappiness
parameter isn't supported.
*
*
* If you're using tasks on Amazon Linux 2023 the swappiness
parameter isn't supported.
*
*
*
* @param swappiness
* This allows you to tune a container's memory swappiness behavior. A swappiness
value of
* 0
will cause swapping to not happen unless absolutely necessary. A swappiness
* value of 100
will cause pages to be swapped very aggressively. Accepted values are whole
* numbers between 0
and 100
. If the swappiness
parameter is not
* specified, a default value of 60
is used. If a value is not specified for
* maxSwap
then this parameter is ignored. This parameter maps to the
* --memory-swappiness
option to docker run.
*
* If you're using tasks that use the Fargate launch type, the swappiness
parameter isn't
* supported.
*
*
* If you're using tasks on Amazon Linux 2023 the swappiness
parameter isn't supported.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public LinuxParameters withSwappiness(Integer swappiness) {
setSwappiness(swappiness);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getCapabilities() != null)
sb.append("Capabilities: ").append(getCapabilities()).append(",");
if (getDevices() != null)
sb.append("Devices: ").append(getDevices()).append(",");
if (getInitProcessEnabled() != null)
sb.append("InitProcessEnabled: ").append(getInitProcessEnabled()).append(",");
if (getSharedMemorySize() != null)
sb.append("SharedMemorySize: ").append(getSharedMemorySize()).append(",");
if (getTmpfs() != null)
sb.append("Tmpfs: ").append(getTmpfs()).append(",");
if (getMaxSwap() != null)
sb.append("MaxSwap: ").append(getMaxSwap()).append(",");
if (getSwappiness() != null)
sb.append("Swappiness: ").append(getSwappiness());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof LinuxParameters == false)
return false;
LinuxParameters other = (LinuxParameters) obj;
if (other.getCapabilities() == null ^ this.getCapabilities() == null)
return false;
if (other.getCapabilities() != null && other.getCapabilities().equals(this.getCapabilities()) == false)
return false;
if (other.getDevices() == null ^ this.getDevices() == null)
return false;
if (other.getDevices() != null && other.getDevices().equals(this.getDevices()) == false)
return false;
if (other.getInitProcessEnabled() == null ^ this.getInitProcessEnabled() == null)
return false;
if (other.getInitProcessEnabled() != null && other.getInitProcessEnabled().equals(this.getInitProcessEnabled()) == false)
return false;
if (other.getSharedMemorySize() == null ^ this.getSharedMemorySize() == null)
return false;
if (other.getSharedMemorySize() != null && other.getSharedMemorySize().equals(this.getSharedMemorySize()) == false)
return false;
if (other.getTmpfs() == null ^ this.getTmpfs() == null)
return false;
if (other.getTmpfs() != null && other.getTmpfs().equals(this.getTmpfs()) == false)
return false;
if (other.getMaxSwap() == null ^ this.getMaxSwap() == null)
return false;
if (other.getMaxSwap() != null && other.getMaxSwap().equals(this.getMaxSwap()) == false)
return false;
if (other.getSwappiness() == null ^ this.getSwappiness() == null)
return false;
if (other.getSwappiness() != null && other.getSwappiness().equals(this.getSwappiness()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getCapabilities() == null) ? 0 : getCapabilities().hashCode());
hashCode = prime * hashCode + ((getDevices() == null) ? 0 : getDevices().hashCode());
hashCode = prime * hashCode + ((getInitProcessEnabled() == null) ? 0 : getInitProcessEnabled().hashCode());
hashCode = prime * hashCode + ((getSharedMemorySize() == null) ? 0 : getSharedMemorySize().hashCode());
hashCode = prime * hashCode + ((getTmpfs() == null) ? 0 : getTmpfs().hashCode());
hashCode = prime * hashCode + ((getMaxSwap() == null) ? 0 : getMaxSwap().hashCode());
hashCode = prime * hashCode + ((getSwappiness() == null) ? 0 : getSwappiness().hashCode());
return hashCode;
}
@Override
public LinuxParameters clone() {
try {
return (LinuxParameters) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.ecs.model.transform.LinuxParametersMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}