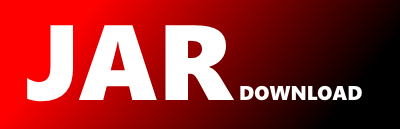
com.amazonaws.services.ecs.model.TaskDefinition Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ecs Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ecs.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The details of a task definition which describes the container and volume definitions of an Amazon Elastic Container
* Service task. You can specify which Docker images to use, the required resources, and other configurations related to
* launching the task definition through an Amazon ECS service or task.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class TaskDefinition implements Serializable, Cloneable, StructuredPojo {
/**
*
* The full Amazon Resource Name (ARN) of the task definition.
*
*/
private String taskDefinitionArn;
/**
*
* A list of container definitions in JSON format that describe the different containers that make up your task. For
* more information about container definition parameters and defaults, see Amazon ECS Task
* Definitions in the Amazon Elastic Container Service Developer Guide.
*
*/
private com.amazonaws.internal.SdkInternalList containerDefinitions;
/**
*
* The name of a family that this task definition is registered to. Up to 255 characters are allowed. Letters (both
* uppercase and lowercase letters), numbers, hyphens (-), and underscores (_) are allowed.
*
*
* A family groups multiple versions of a task definition. Amazon ECS gives the first task definition that you
* registered to a family a revision number of 1. Amazon ECS gives sequential revision numbers to each task
* definition that you add.
*
*/
private String family;
/**
*
* The short name or full Amazon Resource Name (ARN) of the Identity and Access Management role that grants
* containers in the task permission to call Amazon Web Services APIs on your behalf. For more information, see Amazon ECS Task Role
* in the Amazon Elastic Container Service Developer Guide.
*
*
* IAM roles for tasks on Windows require that the -EnableTaskIAMRole
option is set when you launch the
* Amazon ECS-optimized Windows AMI. Your containers must also run some configuration code to use the feature. For
* more information, see Windows IAM roles
* for tasks in the Amazon Elastic Container Service Developer Guide.
*
*/
private String taskRoleArn;
/**
*
* The Amazon Resource Name (ARN) of the task execution role that grants the Amazon ECS container agent permission
* to make Amazon Web Services API calls on your behalf. The task execution IAM role is required depending on the
* requirements of your task. For more information, see Amazon ECS task
* execution IAM role in the Amazon Elastic Container Service Developer Guide.
*
*/
private String executionRoleArn;
/**
*
* The Docker networking mode to use for the containers in the task. The valid values are none
,
* bridge
, awsvpc
, and host
. If no network mode is specified, the default is
* bridge
.
*
*
* For Amazon ECS tasks on Fargate, the awsvpc
network mode is required. For Amazon ECS tasks on Amazon
* EC2 Linux instances, any network mode can be used. For Amazon ECS tasks on Amazon EC2 Windows instances,
* <default>
or awsvpc
can be used. If the network mode is set to none
,
* you cannot specify port mappings in your container definitions, and the tasks containers do not have external
* connectivity. The host
and awsvpc
network modes offer the highest networking
* performance for containers because they use the EC2 network stack instead of the virtualized network stack
* provided by the bridge
mode.
*
*
* With the host
and awsvpc
network modes, exposed container ports are mapped directly to
* the corresponding host port (for the host
network mode) or the attached elastic network interface
* port (for the awsvpc
network mode), so you cannot take advantage of dynamic host port mappings.
*
*
*
* When using the host
network mode, you should not run containers using the root user (UID 0). It is
* considered best practice to use a non-root user.
*
*
*
* If the network mode is awsvpc
, the task is allocated an elastic network interface, and you must
* specify a NetworkConfiguration value when you create a service or run a task with the task definition. For
* more information, see Task Networking in
* the Amazon Elastic Container Service Developer Guide.
*
*
* If the network mode is host
, you cannot run multiple instantiations of the same task on a single
* container instance when port mappings are used.
*
*
* For more information, see Network
* settings in the Docker run reference.
*
*/
private String networkMode;
/**
*
* The revision of the task in a particular family. The revision is a version number of a task definition in a
* family. When you register a task definition for the first time, the revision is 1
. Each time that
* you register a new revision of a task definition in the same family, the revision value always increases by one.
* This is even if you deregistered previous revisions in this family.
*
*/
private Integer revision;
/**
*
* The list of data volume definitions for the task. For more information, see Using data volumes in
* tasks in the Amazon Elastic Container Service Developer Guide.
*
*
*
* The host
and sourcePath
parameters aren't supported for tasks run on Fargate.
*
*
*/
private com.amazonaws.internal.SdkInternalList volumes;
/**
*
* The status of the task definition.
*
*/
private String status;
/**
*
* The container instance attributes required by your task. When an Amazon EC2 instance is registered to your
* cluster, the Amazon ECS container agent assigns some standard attributes to the instance. You can apply custom
* attributes. These are specified as key-value pairs using the Amazon ECS console or the PutAttributes API.
* These attributes are used when determining task placement for tasks hosted on Amazon EC2 instances. For more
* information, see Attributes in the Amazon Elastic Container Service Developer Guide.
*
*
*
* This parameter isn't supported for tasks run on Fargate.
*
*
*/
private com.amazonaws.internal.SdkInternalList requiresAttributes;
/**
*
* An array of placement constraint objects to use for tasks.
*
*
*
* This parameter isn't supported for tasks run on Fargate.
*
*
*/
private com.amazonaws.internal.SdkInternalList placementConstraints;
/**
*
* The task launch types the task definition validated against during task definition registration. For more
* information, see Amazon
* ECS launch types in the Amazon Elastic Container Service Developer Guide.
*
*/
private com.amazonaws.internal.SdkInternalList compatibilities;
/**
*
* The operating system that your task definitions are running on. A platform family is specified only for tasks
* using the Fargate launch type.
*
*
* When you specify a task in a service, this value must match the runtimePlatform
value of the
* service.
*
*/
private RuntimePlatform runtimePlatform;
/**
*
* The task launch types the task definition was validated against. The valid values are EC2
,
* FARGATE
, and EXTERNAL
. For more information, see Amazon ECS launch types
* in the Amazon Elastic Container Service Developer Guide.
*
*/
private com.amazonaws.internal.SdkInternalList requiresCompatibilities;
/**
*
* The number of cpu
units used by the task. If you use the EC2 launch type, this field is optional.
* Any value can be used. If you use the Fargate launch type, this field is required. You must use one of the
* following values. The value that you choose determines your range of valid values for the memory
* parameter.
*
*
* The CPU units cannot be less than 1 vCPU when you use Windows containers on Fargate.
*
*
* -
*
* 256 (.25 vCPU) - Available memory
values: 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB)
*
*
* -
*
* 512 (.5 vCPU) - Available memory
values: 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB)
*
*
* -
*
* 1024 (1 vCPU) - Available memory
values: 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB), 6144 (6
* GB), 7168 (7 GB), 8192 (8 GB)
*
*
* -
*
* 2048 (2 vCPU) - Available memory
values: 4096 (4 GB) and 16384 (16 GB) in increments of 1024 (1 GB)
*
*
* -
*
* 4096 (4 vCPU) - Available memory
values: 8192 (8 GB) and 30720 (30 GB) in increments of 1024 (1 GB)
*
*
* -
*
* 8192 (8 vCPU) - Available memory
values: 16 GB and 60 GB in 4 GB increments
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
* -
*
* 16384 (16vCPU) - Available memory
values: 32GB and 120 GB in 8 GB increments
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
*
*/
private String cpu;
/**
*
* The amount (in MiB) of memory used by the task.
*
*
* If your tasks runs on Amazon EC2 instances, you must specify either a task-level memory value or a
* container-level memory value. This field is optional and any value can be used. If a task-level memory value is
* specified, the container-level memory value is optional. For more information regarding container-level memory
* and memory reservation, see ContainerDefinition.
*
*
* If your tasks runs on Fargate, this field is required. You must use one of the following values. The value you
* choose determines your range of valid values for the cpu
parameter.
*
*
* -
*
* 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB) - Available cpu
values: 256 (.25 vCPU)
*
*
* -
*
* 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB) - Available cpu
values: 512 (.5 vCPU)
*
*
* -
*
* 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB), 6144 (6 GB), 7168 (7 GB), 8192 (8 GB) - Available
* cpu
values: 1024 (1 vCPU)
*
*
* -
*
* Between 4096 (4 GB) and 16384 (16 GB) in increments of 1024 (1 GB) - Available cpu
values: 2048 (2
* vCPU)
*
*
* -
*
* Between 8192 (8 GB) and 30720 (30 GB) in increments of 1024 (1 GB) - Available cpu
values: 4096 (4
* vCPU)
*
*
* -
*
* Between 16 GB and 60 GB in 4 GB increments - Available cpu
values: 8192 (8 vCPU)
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
* -
*
* Between 32GB and 120 GB in 8 GB increments - Available cpu
values: 16384 (16 vCPU)
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
*
*/
private String memory;
/**
*
* The Elastic Inference accelerator that's associated with the task.
*
*/
private com.amazonaws.internal.SdkInternalList inferenceAccelerators;
/**
*
* The process namespace to use for the containers in the task. The valid values are host
or
* task
. On Fargate for Linux containers, the only valid value is task
. For example,
* monitoring sidecars might need pidMode
to access information about other containers running in the
* same task.
*
*
* If host
is specified, all containers within the tasks that specified the host
PID mode
* on the same container instance share the same process namespace with the host Amazon EC2 instance.
*
*
* If task
is specified, all containers within the specified task share the same process namespace.
*
*
* If no value is specified, the default is a private namespace for each container. For more information, see PID settings in the Docker run
* reference.
*
*
* If the host
PID mode is used, there's a heightened risk of undesired process namespace exposure. For
* more information, see Docker security.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* This parameter is only supported for tasks that are hosted on Fargate if the tasks are using platform version
* 1.4.0
or later (Linux). This isn't supported for Windows containers on Fargate.
*
*
*/
private String pidMode;
/**
*
* The IPC resource namespace to use for the containers in the task. The valid values are host
,
* task
, or none
. If host
is specified, then all containers within the tasks
* that specified the host
IPC mode on the same container instance share the same IPC resources with
* the host Amazon EC2 instance. If task
is specified, all containers within the specified task share
* the same IPC resources. If none
is specified, then IPC resources within the containers of a task are
* private and not shared with other containers in a task or on the container instance. If no value is specified,
* then the IPC resource namespace sharing depends on the Docker daemon setting on the container instance. For more
* information, see IPC settings in
* the Docker run reference.
*
*
* If the host
IPC mode is used, be aware that there is a heightened risk of undesired IPC namespace
* expose. For more information, see Docker
* security.
*
*
* If you are setting namespaced kernel parameters using systemControls
for the containers in the task,
* the following will apply to your IPC resource namespace. For more information, see System
* Controls in the Amazon Elastic Container Service Developer Guide.
*
*
* -
*
* For tasks that use the host
IPC mode, IPC namespace related systemControls
are not
* supported.
*
*
* -
*
* For tasks that use the task
IPC mode, IPC namespace related systemControls
will apply
* to all containers within a task.
*
*
*
*
*
* This parameter is not supported for Windows containers or tasks run on Fargate.
*
*
*/
private String ipcMode;
/**
*
* The configuration details for the App Mesh proxy.
*
*
* Your Amazon ECS container instances require at least version 1.26.0 of the container agent and at least version
* 1.26.0-1 of the ecs-init
package to use a proxy configuration. If your container instances are
* launched from the Amazon ECS optimized AMI version 20190301
or later, they contain the required
* versions of the container agent and ecs-init
. For more information, see Amazon ECS-optimized
* Linux AMI in the Amazon Elastic Container Service Developer Guide.
*
*/
private ProxyConfiguration proxyConfiguration;
/**
*
* The Unix timestamp for the time when the task definition was registered.
*
*/
private java.util.Date registeredAt;
/**
*
* The Unix timestamp for the time when the task definition was deregistered.
*
*/
private java.util.Date deregisteredAt;
/**
*
* The principal that registered the task definition.
*
*/
private String registeredBy;
/**
*
* The ephemeral storage settings to use for tasks run with the task definition.
*
*/
private EphemeralStorage ephemeralStorage;
/**
*
* The full Amazon Resource Name (ARN) of the task definition.
*
*
* @param taskDefinitionArn
* The full Amazon Resource Name (ARN) of the task definition.
*/
public void setTaskDefinitionArn(String taskDefinitionArn) {
this.taskDefinitionArn = taskDefinitionArn;
}
/**
*
* The full Amazon Resource Name (ARN) of the task definition.
*
*
* @return The full Amazon Resource Name (ARN) of the task definition.
*/
public String getTaskDefinitionArn() {
return this.taskDefinitionArn;
}
/**
*
* The full Amazon Resource Name (ARN) of the task definition.
*
*
* @param taskDefinitionArn
* The full Amazon Resource Name (ARN) of the task definition.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskDefinition withTaskDefinitionArn(String taskDefinitionArn) {
setTaskDefinitionArn(taskDefinitionArn);
return this;
}
/**
*
* A list of container definitions in JSON format that describe the different containers that make up your task. For
* more information about container definition parameters and defaults, see Amazon ECS Task
* Definitions in the Amazon Elastic Container Service Developer Guide.
*
*
* @return A list of container definitions in JSON format that describe the different containers that make up your
* task. For more information about container definition parameters and defaults, see Amazon ECS Task
* Definitions in the Amazon Elastic Container Service Developer Guide.
*/
public java.util.List getContainerDefinitions() {
if (containerDefinitions == null) {
containerDefinitions = new com.amazonaws.internal.SdkInternalList();
}
return containerDefinitions;
}
/**
*
* A list of container definitions in JSON format that describe the different containers that make up your task. For
* more information about container definition parameters and defaults, see Amazon ECS Task
* Definitions in the Amazon Elastic Container Service Developer Guide.
*
*
* @param containerDefinitions
* A list of container definitions in JSON format that describe the different containers that make up your
* task. For more information about container definition parameters and defaults, see Amazon ECS Task
* Definitions in the Amazon Elastic Container Service Developer Guide.
*/
public void setContainerDefinitions(java.util.Collection containerDefinitions) {
if (containerDefinitions == null) {
this.containerDefinitions = null;
return;
}
this.containerDefinitions = new com.amazonaws.internal.SdkInternalList(containerDefinitions);
}
/**
*
* A list of container definitions in JSON format that describe the different containers that make up your task. For
* more information about container definition parameters and defaults, see Amazon ECS Task
* Definitions in the Amazon Elastic Container Service Developer Guide.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setContainerDefinitions(java.util.Collection)} or {@link #withContainerDefinitions(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param containerDefinitions
* A list of container definitions in JSON format that describe the different containers that make up your
* task. For more information about container definition parameters and defaults, see Amazon ECS Task
* Definitions in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskDefinition withContainerDefinitions(ContainerDefinition... containerDefinitions) {
if (this.containerDefinitions == null) {
setContainerDefinitions(new com.amazonaws.internal.SdkInternalList(containerDefinitions.length));
}
for (ContainerDefinition ele : containerDefinitions) {
this.containerDefinitions.add(ele);
}
return this;
}
/**
*
* A list of container definitions in JSON format that describe the different containers that make up your task. For
* more information about container definition parameters and defaults, see Amazon ECS Task
* Definitions in the Amazon Elastic Container Service Developer Guide.
*
*
* @param containerDefinitions
* A list of container definitions in JSON format that describe the different containers that make up your
* task. For more information about container definition parameters and defaults, see Amazon ECS Task
* Definitions in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskDefinition withContainerDefinitions(java.util.Collection containerDefinitions) {
setContainerDefinitions(containerDefinitions);
return this;
}
/**
*
* The name of a family that this task definition is registered to. Up to 255 characters are allowed. Letters (both
* uppercase and lowercase letters), numbers, hyphens (-), and underscores (_) are allowed.
*
*
* A family groups multiple versions of a task definition. Amazon ECS gives the first task definition that you
* registered to a family a revision number of 1. Amazon ECS gives sequential revision numbers to each task
* definition that you add.
*
*
* @param family
* The name of a family that this task definition is registered to. Up to 255 characters are allowed. Letters
* (both uppercase and lowercase letters), numbers, hyphens (-), and underscores (_) are allowed.
*
* A family groups multiple versions of a task definition. Amazon ECS gives the first task definition that
* you registered to a family a revision number of 1. Amazon ECS gives sequential revision numbers to each
* task definition that you add.
*/
public void setFamily(String family) {
this.family = family;
}
/**
*
* The name of a family that this task definition is registered to. Up to 255 characters are allowed. Letters (both
* uppercase and lowercase letters), numbers, hyphens (-), and underscores (_) are allowed.
*
*
* A family groups multiple versions of a task definition. Amazon ECS gives the first task definition that you
* registered to a family a revision number of 1. Amazon ECS gives sequential revision numbers to each task
* definition that you add.
*
*
* @return The name of a family that this task definition is registered to. Up to 255 characters are allowed.
* Letters (both uppercase and lowercase letters), numbers, hyphens (-), and underscores (_) are
* allowed.
*
* A family groups multiple versions of a task definition. Amazon ECS gives the first task definition that
* you registered to a family a revision number of 1. Amazon ECS gives sequential revision numbers to each
* task definition that you add.
*/
public String getFamily() {
return this.family;
}
/**
*
* The name of a family that this task definition is registered to. Up to 255 characters are allowed. Letters (both
* uppercase and lowercase letters), numbers, hyphens (-), and underscores (_) are allowed.
*
*
* A family groups multiple versions of a task definition. Amazon ECS gives the first task definition that you
* registered to a family a revision number of 1. Amazon ECS gives sequential revision numbers to each task
* definition that you add.
*
*
* @param family
* The name of a family that this task definition is registered to. Up to 255 characters are allowed. Letters
* (both uppercase and lowercase letters), numbers, hyphens (-), and underscores (_) are allowed.
*
* A family groups multiple versions of a task definition. Amazon ECS gives the first task definition that
* you registered to a family a revision number of 1. Amazon ECS gives sequential revision numbers to each
* task definition that you add.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskDefinition withFamily(String family) {
setFamily(family);
return this;
}
/**
*
* The short name or full Amazon Resource Name (ARN) of the Identity and Access Management role that grants
* containers in the task permission to call Amazon Web Services APIs on your behalf. For more information, see Amazon ECS Task Role
* in the Amazon Elastic Container Service Developer Guide.
*
*
* IAM roles for tasks on Windows require that the -EnableTaskIAMRole
option is set when you launch the
* Amazon ECS-optimized Windows AMI. Your containers must also run some configuration code to use the feature. For
* more information, see Windows IAM roles
* for tasks in the Amazon Elastic Container Service Developer Guide.
*
*
* @param taskRoleArn
* The short name or full Amazon Resource Name (ARN) of the Identity and Access Management role that grants
* containers in the task permission to call Amazon Web Services APIs on your behalf. For more information,
* see Amazon ECS
* Task Role in the Amazon Elastic Container Service Developer Guide.
*
* IAM roles for tasks on Windows require that the -EnableTaskIAMRole
option is set when you
* launch the Amazon ECS-optimized Windows AMI. Your containers must also run some configuration code to use
* the feature. For more information, see Windows IAM
* roles for tasks in the Amazon Elastic Container Service Developer Guide.
*/
public void setTaskRoleArn(String taskRoleArn) {
this.taskRoleArn = taskRoleArn;
}
/**
*
* The short name or full Amazon Resource Name (ARN) of the Identity and Access Management role that grants
* containers in the task permission to call Amazon Web Services APIs on your behalf. For more information, see Amazon ECS Task Role
* in the Amazon Elastic Container Service Developer Guide.
*
*
* IAM roles for tasks on Windows require that the -EnableTaskIAMRole
option is set when you launch the
* Amazon ECS-optimized Windows AMI. Your containers must also run some configuration code to use the feature. For
* more information, see Windows IAM roles
* for tasks in the Amazon Elastic Container Service Developer Guide.
*
*
* @return The short name or full Amazon Resource Name (ARN) of the Identity and Access Management role that grants
* containers in the task permission to call Amazon Web Services APIs on your behalf. For more information,
* see Amazon ECS
* Task Role in the Amazon Elastic Container Service Developer Guide.
*
* IAM roles for tasks on Windows require that the -EnableTaskIAMRole
option is set when you
* launch the Amazon ECS-optimized Windows AMI. Your containers must also run some configuration code to use
* the feature. For more information, see Windows
* IAM roles for tasks in the Amazon Elastic Container Service Developer Guide.
*/
public String getTaskRoleArn() {
return this.taskRoleArn;
}
/**
*
* The short name or full Amazon Resource Name (ARN) of the Identity and Access Management role that grants
* containers in the task permission to call Amazon Web Services APIs on your behalf. For more information, see Amazon ECS Task Role
* in the Amazon Elastic Container Service Developer Guide.
*
*
* IAM roles for tasks on Windows require that the -EnableTaskIAMRole
option is set when you launch the
* Amazon ECS-optimized Windows AMI. Your containers must also run some configuration code to use the feature. For
* more information, see Windows IAM roles
* for tasks in the Amazon Elastic Container Service Developer Guide.
*
*
* @param taskRoleArn
* The short name or full Amazon Resource Name (ARN) of the Identity and Access Management role that grants
* containers in the task permission to call Amazon Web Services APIs on your behalf. For more information,
* see Amazon ECS
* Task Role in the Amazon Elastic Container Service Developer Guide.
*
* IAM roles for tasks on Windows require that the -EnableTaskIAMRole
option is set when you
* launch the Amazon ECS-optimized Windows AMI. Your containers must also run some configuration code to use
* the feature. For more information, see Windows IAM
* roles for tasks in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskDefinition withTaskRoleArn(String taskRoleArn) {
setTaskRoleArn(taskRoleArn);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the task execution role that grants the Amazon ECS container agent permission
* to make Amazon Web Services API calls on your behalf. The task execution IAM role is required depending on the
* requirements of your task. For more information, see Amazon ECS task
* execution IAM role in the Amazon Elastic Container Service Developer Guide.
*
*
* @param executionRoleArn
* The Amazon Resource Name (ARN) of the task execution role that grants the Amazon ECS container agent
* permission to make Amazon Web Services API calls on your behalf. The task execution IAM role is required
* depending on the requirements of your task. For more information, see Amazon ECS
* task execution IAM role in the Amazon Elastic Container Service Developer Guide.
*/
public void setExecutionRoleArn(String executionRoleArn) {
this.executionRoleArn = executionRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the task execution role that grants the Amazon ECS container agent permission
* to make Amazon Web Services API calls on your behalf. The task execution IAM role is required depending on the
* requirements of your task. For more information, see Amazon ECS task
* execution IAM role in the Amazon Elastic Container Service Developer Guide.
*
*
* @return The Amazon Resource Name (ARN) of the task execution role that grants the Amazon ECS container agent
* permission to make Amazon Web Services API calls on your behalf. The task execution IAM role is required
* depending on the requirements of your task. For more information, see Amazon
* ECS task execution IAM role in the Amazon Elastic Container Service Developer Guide.
*/
public String getExecutionRoleArn() {
return this.executionRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the task execution role that grants the Amazon ECS container agent permission
* to make Amazon Web Services API calls on your behalf. The task execution IAM role is required depending on the
* requirements of your task. For more information, see Amazon ECS task
* execution IAM role in the Amazon Elastic Container Service Developer Guide.
*
*
* @param executionRoleArn
* The Amazon Resource Name (ARN) of the task execution role that grants the Amazon ECS container agent
* permission to make Amazon Web Services API calls on your behalf. The task execution IAM role is required
* depending on the requirements of your task. For more information, see Amazon ECS
* task execution IAM role in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskDefinition withExecutionRoleArn(String executionRoleArn) {
setExecutionRoleArn(executionRoleArn);
return this;
}
/**
*
* The Docker networking mode to use for the containers in the task. The valid values are none
,
* bridge
, awsvpc
, and host
. If no network mode is specified, the default is
* bridge
.
*
*
* For Amazon ECS tasks on Fargate, the awsvpc
network mode is required. For Amazon ECS tasks on Amazon
* EC2 Linux instances, any network mode can be used. For Amazon ECS tasks on Amazon EC2 Windows instances,
* <default>
or awsvpc
can be used. If the network mode is set to none
,
* you cannot specify port mappings in your container definitions, and the tasks containers do not have external
* connectivity. The host
and awsvpc
network modes offer the highest networking
* performance for containers because they use the EC2 network stack instead of the virtualized network stack
* provided by the bridge
mode.
*
*
* With the host
and awsvpc
network modes, exposed container ports are mapped directly to
* the corresponding host port (for the host
network mode) or the attached elastic network interface
* port (for the awsvpc
network mode), so you cannot take advantage of dynamic host port mappings.
*
*
*
* When using the host
network mode, you should not run containers using the root user (UID 0). It is
* considered best practice to use a non-root user.
*
*
*
* If the network mode is awsvpc
, the task is allocated an elastic network interface, and you must
* specify a NetworkConfiguration value when you create a service or run a task with the task definition. For
* more information, see Task Networking in
* the Amazon Elastic Container Service Developer Guide.
*
*
* If the network mode is host
, you cannot run multiple instantiations of the same task on a single
* container instance when port mappings are used.
*
*
* For more information, see Network
* settings in the Docker run reference.
*
*
* @param networkMode
* The Docker networking mode to use for the containers in the task. The valid values are none
,
* bridge
, awsvpc
, and host
. If no network mode is specified, the
* default is bridge
.
*
* For Amazon ECS tasks on Fargate, the awsvpc
network mode is required. For Amazon ECS tasks on
* Amazon EC2 Linux instances, any network mode can be used. For Amazon ECS tasks on Amazon EC2 Windows
* instances, <default>
or awsvpc
can be used. If the network mode is set to
* none
, you cannot specify port mappings in your container definitions, and the tasks
* containers do not have external connectivity. The host
and awsvpc
network modes
* offer the highest networking performance for containers because they use the EC2 network stack instead of
* the virtualized network stack provided by the bridge
mode.
*
*
* With the host
and awsvpc
network modes, exposed container ports are mapped
* directly to the corresponding host port (for the host
network mode) or the attached elastic
* network interface port (for the awsvpc
network mode), so you cannot take advantage of dynamic
* host port mappings.
*
*
*
* When using the host
network mode, you should not run containers using the root user (UID 0).
* It is considered best practice to use a non-root user.
*
*
*
* If the network mode is awsvpc
, the task is allocated an elastic network interface, and you
* must specify a NetworkConfiguration value when you create a service or run a task with the task
* definition. For more information, see Task
* Networking in the Amazon Elastic Container Service Developer Guide.
*
*
* If the network mode is host
, you cannot run multiple instantiations of the same task on a
* single container instance when port mappings are used.
*
*
* For more information, see Network
* settings in the Docker run reference.
* @see NetworkMode
*/
public void setNetworkMode(String networkMode) {
this.networkMode = networkMode;
}
/**
*
* The Docker networking mode to use for the containers in the task. The valid values are none
,
* bridge
, awsvpc
, and host
. If no network mode is specified, the default is
* bridge
.
*
*
* For Amazon ECS tasks on Fargate, the awsvpc
network mode is required. For Amazon ECS tasks on Amazon
* EC2 Linux instances, any network mode can be used. For Amazon ECS tasks on Amazon EC2 Windows instances,
* <default>
or awsvpc
can be used. If the network mode is set to none
,
* you cannot specify port mappings in your container definitions, and the tasks containers do not have external
* connectivity. The host
and awsvpc
network modes offer the highest networking
* performance for containers because they use the EC2 network stack instead of the virtualized network stack
* provided by the bridge
mode.
*
*
* With the host
and awsvpc
network modes, exposed container ports are mapped directly to
* the corresponding host port (for the host
network mode) or the attached elastic network interface
* port (for the awsvpc
network mode), so you cannot take advantage of dynamic host port mappings.
*
*
*
* When using the host
network mode, you should not run containers using the root user (UID 0). It is
* considered best practice to use a non-root user.
*
*
*
* If the network mode is awsvpc
, the task is allocated an elastic network interface, and you must
* specify a NetworkConfiguration value when you create a service or run a task with the task definition. For
* more information, see Task Networking in
* the Amazon Elastic Container Service Developer Guide.
*
*
* If the network mode is host
, you cannot run multiple instantiations of the same task on a single
* container instance when port mappings are used.
*
*
* For more information, see Network
* settings in the Docker run reference.
*
*
* @return The Docker networking mode to use for the containers in the task. The valid values are none
,
* bridge
, awsvpc
, and host
. If no network mode is specified, the
* default is bridge
.
*
* For Amazon ECS tasks on Fargate, the awsvpc
network mode is required. For Amazon ECS tasks
* on Amazon EC2 Linux instances, any network mode can be used. For Amazon ECS tasks on Amazon EC2 Windows
* instances, <default>
or awsvpc
can be used. If the network mode is set to
* none
, you cannot specify port mappings in your container definitions, and the tasks
* containers do not have external connectivity. The host
and awsvpc
network modes
* offer the highest networking performance for containers because they use the EC2 network stack instead of
* the virtualized network stack provided by the bridge
mode.
*
*
* With the host
and awsvpc
network modes, exposed container ports are mapped
* directly to the corresponding host port (for the host
network mode) or the attached elastic
* network interface port (for the awsvpc
network mode), so you cannot take advantage of
* dynamic host port mappings.
*
*
*
* When using the host
network mode, you should not run containers using the root user (UID 0).
* It is considered best practice to use a non-root user.
*
*
*
* If the network mode is awsvpc
, the task is allocated an elastic network interface, and you
* must specify a NetworkConfiguration value when you create a service or run a task with the task
* definition. For more information, see Task
* Networking in the Amazon Elastic Container Service Developer Guide.
*
*
* If the network mode is host
, you cannot run multiple instantiations of the same task on a
* single container instance when port mappings are used.
*
*
* For more information, see Network settings in the
* Docker run reference.
* @see NetworkMode
*/
public String getNetworkMode() {
return this.networkMode;
}
/**
*
* The Docker networking mode to use for the containers in the task. The valid values are none
,
* bridge
, awsvpc
, and host
. If no network mode is specified, the default is
* bridge
.
*
*
* For Amazon ECS tasks on Fargate, the awsvpc
network mode is required. For Amazon ECS tasks on Amazon
* EC2 Linux instances, any network mode can be used. For Amazon ECS tasks on Amazon EC2 Windows instances,
* <default>
or awsvpc
can be used. If the network mode is set to none
,
* you cannot specify port mappings in your container definitions, and the tasks containers do not have external
* connectivity. The host
and awsvpc
network modes offer the highest networking
* performance for containers because they use the EC2 network stack instead of the virtualized network stack
* provided by the bridge
mode.
*
*
* With the host
and awsvpc
network modes, exposed container ports are mapped directly to
* the corresponding host port (for the host
network mode) or the attached elastic network interface
* port (for the awsvpc
network mode), so you cannot take advantage of dynamic host port mappings.
*
*
*
* When using the host
network mode, you should not run containers using the root user (UID 0). It is
* considered best practice to use a non-root user.
*
*
*
* If the network mode is awsvpc
, the task is allocated an elastic network interface, and you must
* specify a NetworkConfiguration value when you create a service or run a task with the task definition. For
* more information, see Task Networking in
* the Amazon Elastic Container Service Developer Guide.
*
*
* If the network mode is host
, you cannot run multiple instantiations of the same task on a single
* container instance when port mappings are used.
*
*
* For more information, see Network
* settings in the Docker run reference.
*
*
* @param networkMode
* The Docker networking mode to use for the containers in the task. The valid values are none
,
* bridge
, awsvpc
, and host
. If no network mode is specified, the
* default is bridge
.
*
* For Amazon ECS tasks on Fargate, the awsvpc
network mode is required. For Amazon ECS tasks on
* Amazon EC2 Linux instances, any network mode can be used. For Amazon ECS tasks on Amazon EC2 Windows
* instances, <default>
or awsvpc
can be used. If the network mode is set to
* none
, you cannot specify port mappings in your container definitions, and the tasks
* containers do not have external connectivity. The host
and awsvpc
network modes
* offer the highest networking performance for containers because they use the EC2 network stack instead of
* the virtualized network stack provided by the bridge
mode.
*
*
* With the host
and awsvpc
network modes, exposed container ports are mapped
* directly to the corresponding host port (for the host
network mode) or the attached elastic
* network interface port (for the awsvpc
network mode), so you cannot take advantage of dynamic
* host port mappings.
*
*
*
* When using the host
network mode, you should not run containers using the root user (UID 0).
* It is considered best practice to use a non-root user.
*
*
*
* If the network mode is awsvpc
, the task is allocated an elastic network interface, and you
* must specify a NetworkConfiguration value when you create a service or run a task with the task
* definition. For more information, see Task
* Networking in the Amazon Elastic Container Service Developer Guide.
*
*
* If the network mode is host
, you cannot run multiple instantiations of the same task on a
* single container instance when port mappings are used.
*
*
* For more information, see Network
* settings in the Docker run reference.
* @return Returns a reference to this object so that method calls can be chained together.
* @see NetworkMode
*/
public TaskDefinition withNetworkMode(String networkMode) {
setNetworkMode(networkMode);
return this;
}
/**
*
* The Docker networking mode to use for the containers in the task. The valid values are none
,
* bridge
, awsvpc
, and host
. If no network mode is specified, the default is
* bridge
.
*
*
* For Amazon ECS tasks on Fargate, the awsvpc
network mode is required. For Amazon ECS tasks on Amazon
* EC2 Linux instances, any network mode can be used. For Amazon ECS tasks on Amazon EC2 Windows instances,
* <default>
or awsvpc
can be used. If the network mode is set to none
,
* you cannot specify port mappings in your container definitions, and the tasks containers do not have external
* connectivity. The host
and awsvpc
network modes offer the highest networking
* performance for containers because they use the EC2 network stack instead of the virtualized network stack
* provided by the bridge
mode.
*
*
* With the host
and awsvpc
network modes, exposed container ports are mapped directly to
* the corresponding host port (for the host
network mode) or the attached elastic network interface
* port (for the awsvpc
network mode), so you cannot take advantage of dynamic host port mappings.
*
*
*
* When using the host
network mode, you should not run containers using the root user (UID 0). It is
* considered best practice to use a non-root user.
*
*
*
* If the network mode is awsvpc
, the task is allocated an elastic network interface, and you must
* specify a NetworkConfiguration value when you create a service or run a task with the task definition. For
* more information, see Task Networking in
* the Amazon Elastic Container Service Developer Guide.
*
*
* If the network mode is host
, you cannot run multiple instantiations of the same task on a single
* container instance when port mappings are used.
*
*
* For more information, see Network
* settings in the Docker run reference.
*
*
* @param networkMode
* The Docker networking mode to use for the containers in the task. The valid values are none
,
* bridge
, awsvpc
, and host
. If no network mode is specified, the
* default is bridge
.
*
* For Amazon ECS tasks on Fargate, the awsvpc
network mode is required. For Amazon ECS tasks on
* Amazon EC2 Linux instances, any network mode can be used. For Amazon ECS tasks on Amazon EC2 Windows
* instances, <default>
or awsvpc
can be used. If the network mode is set to
* none
, you cannot specify port mappings in your container definitions, and the tasks
* containers do not have external connectivity. The host
and awsvpc
network modes
* offer the highest networking performance for containers because they use the EC2 network stack instead of
* the virtualized network stack provided by the bridge
mode.
*
*
* With the host
and awsvpc
network modes, exposed container ports are mapped
* directly to the corresponding host port (for the host
network mode) or the attached elastic
* network interface port (for the awsvpc
network mode), so you cannot take advantage of dynamic
* host port mappings.
*
*
*
* When using the host
network mode, you should not run containers using the root user (UID 0).
* It is considered best practice to use a non-root user.
*
*
*
* If the network mode is awsvpc
, the task is allocated an elastic network interface, and you
* must specify a NetworkConfiguration value when you create a service or run a task with the task
* definition. For more information, see Task
* Networking in the Amazon Elastic Container Service Developer Guide.
*
*
* If the network mode is host
, you cannot run multiple instantiations of the same task on a
* single container instance when port mappings are used.
*
*
* For more information, see Network
* settings in the Docker run reference.
* @see NetworkMode
*/
public void setNetworkMode(NetworkMode networkMode) {
withNetworkMode(networkMode);
}
/**
*
* The Docker networking mode to use for the containers in the task. The valid values are none
,
* bridge
, awsvpc
, and host
. If no network mode is specified, the default is
* bridge
.
*
*
* For Amazon ECS tasks on Fargate, the awsvpc
network mode is required. For Amazon ECS tasks on Amazon
* EC2 Linux instances, any network mode can be used. For Amazon ECS tasks on Amazon EC2 Windows instances,
* <default>
or awsvpc
can be used. If the network mode is set to none
,
* you cannot specify port mappings in your container definitions, and the tasks containers do not have external
* connectivity. The host
and awsvpc
network modes offer the highest networking
* performance for containers because they use the EC2 network stack instead of the virtualized network stack
* provided by the bridge
mode.
*
*
* With the host
and awsvpc
network modes, exposed container ports are mapped directly to
* the corresponding host port (for the host
network mode) or the attached elastic network interface
* port (for the awsvpc
network mode), so you cannot take advantage of dynamic host port mappings.
*
*
*
* When using the host
network mode, you should not run containers using the root user (UID 0). It is
* considered best practice to use a non-root user.
*
*
*
* If the network mode is awsvpc
, the task is allocated an elastic network interface, and you must
* specify a NetworkConfiguration value when you create a service or run a task with the task definition. For
* more information, see Task Networking in
* the Amazon Elastic Container Service Developer Guide.
*
*
* If the network mode is host
, you cannot run multiple instantiations of the same task on a single
* container instance when port mappings are used.
*
*
* For more information, see Network
* settings in the Docker run reference.
*
*
* @param networkMode
* The Docker networking mode to use for the containers in the task. The valid values are none
,
* bridge
, awsvpc
, and host
. If no network mode is specified, the
* default is bridge
.
*
* For Amazon ECS tasks on Fargate, the awsvpc
network mode is required. For Amazon ECS tasks on
* Amazon EC2 Linux instances, any network mode can be used. For Amazon ECS tasks on Amazon EC2 Windows
* instances, <default>
or awsvpc
can be used. If the network mode is set to
* none
, you cannot specify port mappings in your container definitions, and the tasks
* containers do not have external connectivity. The host
and awsvpc
network modes
* offer the highest networking performance for containers because they use the EC2 network stack instead of
* the virtualized network stack provided by the bridge
mode.
*
*
* With the host
and awsvpc
network modes, exposed container ports are mapped
* directly to the corresponding host port (for the host
network mode) or the attached elastic
* network interface port (for the awsvpc
network mode), so you cannot take advantage of dynamic
* host port mappings.
*
*
*
* When using the host
network mode, you should not run containers using the root user (UID 0).
* It is considered best practice to use a non-root user.
*
*
*
* If the network mode is awsvpc
, the task is allocated an elastic network interface, and you
* must specify a NetworkConfiguration value when you create a service or run a task with the task
* definition. For more information, see Task
* Networking in the Amazon Elastic Container Service Developer Guide.
*
*
* If the network mode is host
, you cannot run multiple instantiations of the same task on a
* single container instance when port mappings are used.
*
*
* For more information, see Network
* settings in the Docker run reference.
* @return Returns a reference to this object so that method calls can be chained together.
* @see NetworkMode
*/
public TaskDefinition withNetworkMode(NetworkMode networkMode) {
this.networkMode = networkMode.toString();
return this;
}
/**
*
* The revision of the task in a particular family. The revision is a version number of a task definition in a
* family. When you register a task definition for the first time, the revision is 1
. Each time that
* you register a new revision of a task definition in the same family, the revision value always increases by one.
* This is even if you deregistered previous revisions in this family.
*
*
* @param revision
* The revision of the task in a particular family. The revision is a version number of a task definition in
* a family. When you register a task definition for the first time, the revision is 1
. Each
* time that you register a new revision of a task definition in the same family, the revision value always
* increases by one. This is even if you deregistered previous revisions in this family.
*/
public void setRevision(Integer revision) {
this.revision = revision;
}
/**
*
* The revision of the task in a particular family. The revision is a version number of a task definition in a
* family. When you register a task definition for the first time, the revision is 1
. Each time that
* you register a new revision of a task definition in the same family, the revision value always increases by one.
* This is even if you deregistered previous revisions in this family.
*
*
* @return The revision of the task in a particular family. The revision is a version number of a task definition in
* a family. When you register a task definition for the first time, the revision is 1
. Each
* time that you register a new revision of a task definition in the same family, the revision value always
* increases by one. This is even if you deregistered previous revisions in this family.
*/
public Integer getRevision() {
return this.revision;
}
/**
*
* The revision of the task in a particular family. The revision is a version number of a task definition in a
* family. When you register a task definition for the first time, the revision is 1
. Each time that
* you register a new revision of a task definition in the same family, the revision value always increases by one.
* This is even if you deregistered previous revisions in this family.
*
*
* @param revision
* The revision of the task in a particular family. The revision is a version number of a task definition in
* a family. When you register a task definition for the first time, the revision is 1
. Each
* time that you register a new revision of a task definition in the same family, the revision value always
* increases by one. This is even if you deregistered previous revisions in this family.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskDefinition withRevision(Integer revision) {
setRevision(revision);
return this;
}
/**
*
* The list of data volume definitions for the task. For more information, see Using data volumes in
* tasks in the Amazon Elastic Container Service Developer Guide.
*
*
*
* The host
and sourcePath
parameters aren't supported for tasks run on Fargate.
*
*
*
* @return The list of data volume definitions for the task. For more information, see Using data
* volumes in tasks in the Amazon Elastic Container Service Developer Guide.
*
* The host
and sourcePath
parameters aren't supported for tasks run on Fargate.
*
*/
public java.util.List getVolumes() {
if (volumes == null) {
volumes = new com.amazonaws.internal.SdkInternalList();
}
return volumes;
}
/**
*
* The list of data volume definitions for the task. For more information, see Using data volumes in
* tasks in the Amazon Elastic Container Service Developer Guide.
*
*
*
* The host
and sourcePath
parameters aren't supported for tasks run on Fargate.
*
*
*
* @param volumes
* The list of data volume definitions for the task. For more information, see Using data
* volumes in tasks in the Amazon Elastic Container Service Developer Guide.
*
* The host
and sourcePath
parameters aren't supported for tasks run on Fargate.
*
*/
public void setVolumes(java.util.Collection volumes) {
if (volumes == null) {
this.volumes = null;
return;
}
this.volumes = new com.amazonaws.internal.SdkInternalList(volumes);
}
/**
*
* The list of data volume definitions for the task. For more information, see Using data volumes in
* tasks in the Amazon Elastic Container Service Developer Guide.
*
*
*
* The host
and sourcePath
parameters aren't supported for tasks run on Fargate.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setVolumes(java.util.Collection)} or {@link #withVolumes(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param volumes
* The list of data volume definitions for the task. For more information, see Using data
* volumes in tasks in the Amazon Elastic Container Service Developer Guide.
*
* The host
and sourcePath
parameters aren't supported for tasks run on Fargate.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskDefinition withVolumes(Volume... volumes) {
if (this.volumes == null) {
setVolumes(new com.amazonaws.internal.SdkInternalList(volumes.length));
}
for (Volume ele : volumes) {
this.volumes.add(ele);
}
return this;
}
/**
*
* The list of data volume definitions for the task. For more information, see Using data volumes in
* tasks in the Amazon Elastic Container Service Developer Guide.
*
*
*
* The host
and sourcePath
parameters aren't supported for tasks run on Fargate.
*
*
*
* @param volumes
* The list of data volume definitions for the task. For more information, see Using data
* volumes in tasks in the Amazon Elastic Container Service Developer Guide.
*
* The host
and sourcePath
parameters aren't supported for tasks run on Fargate.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskDefinition withVolumes(java.util.Collection volumes) {
setVolumes(volumes);
return this;
}
/**
*
* The status of the task definition.
*
*
* @param status
* The status of the task definition.
* @see TaskDefinitionStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the task definition.
*
*
* @return The status of the task definition.
* @see TaskDefinitionStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of the task definition.
*
*
* @param status
* The status of the task definition.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TaskDefinitionStatus
*/
public TaskDefinition withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The status of the task definition.
*
*
* @param status
* The status of the task definition.
* @see TaskDefinitionStatus
*/
public void setStatus(TaskDefinitionStatus status) {
withStatus(status);
}
/**
*
* The status of the task definition.
*
*
* @param status
* The status of the task definition.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TaskDefinitionStatus
*/
public TaskDefinition withStatus(TaskDefinitionStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The container instance attributes required by your task. When an Amazon EC2 instance is registered to your
* cluster, the Amazon ECS container agent assigns some standard attributes to the instance. You can apply custom
* attributes. These are specified as key-value pairs using the Amazon ECS console or the PutAttributes API.
* These attributes are used when determining task placement for tasks hosted on Amazon EC2 instances. For more
* information, see Attributes in the Amazon Elastic Container Service Developer Guide.
*
*
*
* This parameter isn't supported for tasks run on Fargate.
*
*
*
* @return The container instance attributes required by your task. When an Amazon EC2 instance is registered to
* your cluster, the Amazon ECS container agent assigns some standard attributes to the instance. You can
* apply custom attributes. These are specified as key-value pairs using the Amazon ECS console or the
* PutAttributes API. These attributes are used when determining task placement for tasks hosted on
* Amazon EC2 instances. For more information, see Attributes in the Amazon Elastic Container Service Developer Guide.
*
* This parameter isn't supported for tasks run on Fargate.
*
*/
public java.util.List getRequiresAttributes() {
if (requiresAttributes == null) {
requiresAttributes = new com.amazonaws.internal.SdkInternalList();
}
return requiresAttributes;
}
/**
*
* The container instance attributes required by your task. When an Amazon EC2 instance is registered to your
* cluster, the Amazon ECS container agent assigns some standard attributes to the instance. You can apply custom
* attributes. These are specified as key-value pairs using the Amazon ECS console or the PutAttributes API.
* These attributes are used when determining task placement for tasks hosted on Amazon EC2 instances. For more
* information, see Attributes in the Amazon Elastic Container Service Developer Guide.
*
*
*
* This parameter isn't supported for tasks run on Fargate.
*
*
*
* @param requiresAttributes
* The container instance attributes required by your task. When an Amazon EC2 instance is registered to your
* cluster, the Amazon ECS container agent assigns some standard attributes to the instance. You can apply
* custom attributes. These are specified as key-value pairs using the Amazon ECS console or the
* PutAttributes API. These attributes are used when determining task placement for tasks hosted on
* Amazon EC2 instances. For more information, see Attributes in the Amazon Elastic Container Service Developer Guide.
*
* This parameter isn't supported for tasks run on Fargate.
*
*/
public void setRequiresAttributes(java.util.Collection requiresAttributes) {
if (requiresAttributes == null) {
this.requiresAttributes = null;
return;
}
this.requiresAttributes = new com.amazonaws.internal.SdkInternalList(requiresAttributes);
}
/**
*
* The container instance attributes required by your task. When an Amazon EC2 instance is registered to your
* cluster, the Amazon ECS container agent assigns some standard attributes to the instance. You can apply custom
* attributes. These are specified as key-value pairs using the Amazon ECS console or the PutAttributes API.
* These attributes are used when determining task placement for tasks hosted on Amazon EC2 instances. For more
* information, see Attributes in the Amazon Elastic Container Service Developer Guide.
*
*
*
* This parameter isn't supported for tasks run on Fargate.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRequiresAttributes(java.util.Collection)} or {@link #withRequiresAttributes(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param requiresAttributes
* The container instance attributes required by your task. When an Amazon EC2 instance is registered to your
* cluster, the Amazon ECS container agent assigns some standard attributes to the instance. You can apply
* custom attributes. These are specified as key-value pairs using the Amazon ECS console or the
* PutAttributes API. These attributes are used when determining task placement for tasks hosted on
* Amazon EC2 instances. For more information, see Attributes in the Amazon Elastic Container Service Developer Guide.
*
* This parameter isn't supported for tasks run on Fargate.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskDefinition withRequiresAttributes(Attribute... requiresAttributes) {
if (this.requiresAttributes == null) {
setRequiresAttributes(new com.amazonaws.internal.SdkInternalList(requiresAttributes.length));
}
for (Attribute ele : requiresAttributes) {
this.requiresAttributes.add(ele);
}
return this;
}
/**
*
* The container instance attributes required by your task. When an Amazon EC2 instance is registered to your
* cluster, the Amazon ECS container agent assigns some standard attributes to the instance. You can apply custom
* attributes. These are specified as key-value pairs using the Amazon ECS console or the PutAttributes API.
* These attributes are used when determining task placement for tasks hosted on Amazon EC2 instances. For more
* information, see Attributes in the Amazon Elastic Container Service Developer Guide.
*
*
*
* This parameter isn't supported for tasks run on Fargate.
*
*
*
* @param requiresAttributes
* The container instance attributes required by your task. When an Amazon EC2 instance is registered to your
* cluster, the Amazon ECS container agent assigns some standard attributes to the instance. You can apply
* custom attributes. These are specified as key-value pairs using the Amazon ECS console or the
* PutAttributes API. These attributes are used when determining task placement for tasks hosted on
* Amazon EC2 instances. For more information, see Attributes in the Amazon Elastic Container Service Developer Guide.
*
* This parameter isn't supported for tasks run on Fargate.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskDefinition withRequiresAttributes(java.util.Collection requiresAttributes) {
setRequiresAttributes(requiresAttributes);
return this;
}
/**
*
* An array of placement constraint objects to use for tasks.
*
*
*
* This parameter isn't supported for tasks run on Fargate.
*
*
*
* @return An array of placement constraint objects to use for tasks.
*
* This parameter isn't supported for tasks run on Fargate.
*
*/
public java.util.List getPlacementConstraints() {
if (placementConstraints == null) {
placementConstraints = new com.amazonaws.internal.SdkInternalList();
}
return placementConstraints;
}
/**
*
* An array of placement constraint objects to use for tasks.
*
*
*
* This parameter isn't supported for tasks run on Fargate.
*
*
*
* @param placementConstraints
* An array of placement constraint objects to use for tasks.
*
* This parameter isn't supported for tasks run on Fargate.
*
*/
public void setPlacementConstraints(java.util.Collection placementConstraints) {
if (placementConstraints == null) {
this.placementConstraints = null;
return;
}
this.placementConstraints = new com.amazonaws.internal.SdkInternalList(placementConstraints);
}
/**
*
* An array of placement constraint objects to use for tasks.
*
*
*
* This parameter isn't supported for tasks run on Fargate.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPlacementConstraints(java.util.Collection)} or {@link #withPlacementConstraints(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param placementConstraints
* An array of placement constraint objects to use for tasks.
*
* This parameter isn't supported for tasks run on Fargate.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskDefinition withPlacementConstraints(TaskDefinitionPlacementConstraint... placementConstraints) {
if (this.placementConstraints == null) {
setPlacementConstraints(new com.amazonaws.internal.SdkInternalList(placementConstraints.length));
}
for (TaskDefinitionPlacementConstraint ele : placementConstraints) {
this.placementConstraints.add(ele);
}
return this;
}
/**
*
* An array of placement constraint objects to use for tasks.
*
*
*
* This parameter isn't supported for tasks run on Fargate.
*
*
*
* @param placementConstraints
* An array of placement constraint objects to use for tasks.
*
* This parameter isn't supported for tasks run on Fargate.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskDefinition withPlacementConstraints(java.util.Collection placementConstraints) {
setPlacementConstraints(placementConstraints);
return this;
}
/**
*
* The task launch types the task definition validated against during task definition registration. For more
* information, see Amazon
* ECS launch types in the Amazon Elastic Container Service Developer Guide.
*
*
* @return The task launch types the task definition validated against during task definition registration. For more
* information, see Amazon ECS launch
* types in the Amazon Elastic Container Service Developer Guide.
* @see Compatibility
*/
public java.util.List getCompatibilities() {
if (compatibilities == null) {
compatibilities = new com.amazonaws.internal.SdkInternalList();
}
return compatibilities;
}
/**
*
* The task launch types the task definition validated against during task definition registration. For more
* information, see Amazon
* ECS launch types in the Amazon Elastic Container Service Developer Guide.
*
*
* @param compatibilities
* The task launch types the task definition validated against during task definition registration. For more
* information, see Amazon ECS launch
* types in the Amazon Elastic Container Service Developer Guide.
* @see Compatibility
*/
public void setCompatibilities(java.util.Collection compatibilities) {
if (compatibilities == null) {
this.compatibilities = null;
return;
}
this.compatibilities = new com.amazonaws.internal.SdkInternalList(compatibilities);
}
/**
*
* The task launch types the task definition validated against during task definition registration. For more
* information, see Amazon
* ECS launch types in the Amazon Elastic Container Service Developer Guide.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCompatibilities(java.util.Collection)} or {@link #withCompatibilities(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param compatibilities
* The task launch types the task definition validated against during task definition registration. For more
* information, see Amazon ECS launch
* types in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Compatibility
*/
public TaskDefinition withCompatibilities(String... compatibilities) {
if (this.compatibilities == null) {
setCompatibilities(new com.amazonaws.internal.SdkInternalList(compatibilities.length));
}
for (String ele : compatibilities) {
this.compatibilities.add(ele);
}
return this;
}
/**
*
* The task launch types the task definition validated against during task definition registration. For more
* information, see Amazon
* ECS launch types in the Amazon Elastic Container Service Developer Guide.
*
*
* @param compatibilities
* The task launch types the task definition validated against during task definition registration. For more
* information, see Amazon ECS launch
* types in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Compatibility
*/
public TaskDefinition withCompatibilities(java.util.Collection compatibilities) {
setCompatibilities(compatibilities);
return this;
}
/**
*
* The task launch types the task definition validated against during task definition registration. For more
* information, see Amazon
* ECS launch types in the Amazon Elastic Container Service Developer Guide.
*
*
* @param compatibilities
* The task launch types the task definition validated against during task definition registration. For more
* information, see Amazon ECS launch
* types in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Compatibility
*/
public TaskDefinition withCompatibilities(Compatibility... compatibilities) {
com.amazonaws.internal.SdkInternalList compatibilitiesCopy = new com.amazonaws.internal.SdkInternalList(compatibilities.length);
for (Compatibility value : compatibilities) {
compatibilitiesCopy.add(value.toString());
}
if (getCompatibilities() == null) {
setCompatibilities(compatibilitiesCopy);
} else {
getCompatibilities().addAll(compatibilitiesCopy);
}
return this;
}
/**
*
* The operating system that your task definitions are running on. A platform family is specified only for tasks
* using the Fargate launch type.
*
*
* When you specify a task in a service, this value must match the runtimePlatform
value of the
* service.
*
*
* @param runtimePlatform
* The operating system that your task definitions are running on. A platform family is specified only for
* tasks using the Fargate launch type.
*
* When you specify a task in a service, this value must match the runtimePlatform
value of the
* service.
*/
public void setRuntimePlatform(RuntimePlatform runtimePlatform) {
this.runtimePlatform = runtimePlatform;
}
/**
*
* The operating system that your task definitions are running on. A platform family is specified only for tasks
* using the Fargate launch type.
*
*
* When you specify a task in a service, this value must match the runtimePlatform
value of the
* service.
*
*
* @return The operating system that your task definitions are running on. A platform family is specified only for
* tasks using the Fargate launch type.
*
* When you specify a task in a service, this value must match the runtimePlatform
value of the
* service.
*/
public RuntimePlatform getRuntimePlatform() {
return this.runtimePlatform;
}
/**
*
* The operating system that your task definitions are running on. A platform family is specified only for tasks
* using the Fargate launch type.
*
*
* When you specify a task in a service, this value must match the runtimePlatform
value of the
* service.
*
*
* @param runtimePlatform
* The operating system that your task definitions are running on. A platform family is specified only for
* tasks using the Fargate launch type.
*
* When you specify a task in a service, this value must match the runtimePlatform
value of the
* service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskDefinition withRuntimePlatform(RuntimePlatform runtimePlatform) {
setRuntimePlatform(runtimePlatform);
return this;
}
/**
*
* The task launch types the task definition was validated against. The valid values are EC2
,
* FARGATE
, and EXTERNAL
. For more information, see Amazon ECS launch types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* @return The task launch types the task definition was validated against. The valid values are EC2
,
* FARGATE
, and EXTERNAL
. For more information, see Amazon ECS launch
* types in the Amazon Elastic Container Service Developer Guide.
* @see Compatibility
*/
public java.util.List getRequiresCompatibilities() {
if (requiresCompatibilities == null) {
requiresCompatibilities = new com.amazonaws.internal.SdkInternalList();
}
return requiresCompatibilities;
}
/**
*
* The task launch types the task definition was validated against. The valid values are EC2
,
* FARGATE
, and EXTERNAL
. For more information, see Amazon ECS launch types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* @param requiresCompatibilities
* The task launch types the task definition was validated against. The valid values are EC2
,
* FARGATE
, and EXTERNAL
. For more information, see Amazon ECS launch
* types in the Amazon Elastic Container Service Developer Guide.
* @see Compatibility
*/
public void setRequiresCompatibilities(java.util.Collection requiresCompatibilities) {
if (requiresCompatibilities == null) {
this.requiresCompatibilities = null;
return;
}
this.requiresCompatibilities = new com.amazonaws.internal.SdkInternalList(requiresCompatibilities);
}
/**
*
* The task launch types the task definition was validated against. The valid values are EC2
,
* FARGATE
, and EXTERNAL
. For more information, see Amazon ECS launch types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRequiresCompatibilities(java.util.Collection)} or
* {@link #withRequiresCompatibilities(java.util.Collection)} if you want to override the existing values.
*
*
* @param requiresCompatibilities
* The task launch types the task definition was validated against. The valid values are EC2
,
* FARGATE
, and EXTERNAL
. For more information, see Amazon ECS launch
* types in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Compatibility
*/
public TaskDefinition withRequiresCompatibilities(String... requiresCompatibilities) {
if (this.requiresCompatibilities == null) {
setRequiresCompatibilities(new com.amazonaws.internal.SdkInternalList(requiresCompatibilities.length));
}
for (String ele : requiresCompatibilities) {
this.requiresCompatibilities.add(ele);
}
return this;
}
/**
*
* The task launch types the task definition was validated against. The valid values are EC2
,
* FARGATE
, and EXTERNAL
. For more information, see Amazon ECS launch types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* @param requiresCompatibilities
* The task launch types the task definition was validated against. The valid values are EC2
,
* FARGATE
, and EXTERNAL
. For more information, see Amazon ECS launch
* types in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Compatibility
*/
public TaskDefinition withRequiresCompatibilities(java.util.Collection requiresCompatibilities) {
setRequiresCompatibilities(requiresCompatibilities);
return this;
}
/**
*
* The task launch types the task definition was validated against. The valid values are EC2
,
* FARGATE
, and EXTERNAL
. For more information, see Amazon ECS launch types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* @param requiresCompatibilities
* The task launch types the task definition was validated against. The valid values are EC2
,
* FARGATE
, and EXTERNAL
. For more information, see Amazon ECS launch
* types in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Compatibility
*/
public TaskDefinition withRequiresCompatibilities(Compatibility... requiresCompatibilities) {
com.amazonaws.internal.SdkInternalList requiresCompatibilitiesCopy = new com.amazonaws.internal.SdkInternalList(
requiresCompatibilities.length);
for (Compatibility value : requiresCompatibilities) {
requiresCompatibilitiesCopy.add(value.toString());
}
if (getRequiresCompatibilities() == null) {
setRequiresCompatibilities(requiresCompatibilitiesCopy);
} else {
getRequiresCompatibilities().addAll(requiresCompatibilitiesCopy);
}
return this;
}
/**
*
* The number of cpu
units used by the task. If you use the EC2 launch type, this field is optional.
* Any value can be used. If you use the Fargate launch type, this field is required. You must use one of the
* following values. The value that you choose determines your range of valid values for the memory
* parameter.
*
*
* The CPU units cannot be less than 1 vCPU when you use Windows containers on Fargate.
*
*
* -
*
* 256 (.25 vCPU) - Available memory
values: 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB)
*
*
* -
*
* 512 (.5 vCPU) - Available memory
values: 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB)
*
*
* -
*
* 1024 (1 vCPU) - Available memory
values: 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB), 6144 (6
* GB), 7168 (7 GB), 8192 (8 GB)
*
*
* -
*
* 2048 (2 vCPU) - Available memory
values: 4096 (4 GB) and 16384 (16 GB) in increments of 1024 (1 GB)
*
*
* -
*
* 4096 (4 vCPU) - Available memory
values: 8192 (8 GB) and 30720 (30 GB) in increments of 1024 (1 GB)
*
*
* -
*
* 8192 (8 vCPU) - Available memory
values: 16 GB and 60 GB in 4 GB increments
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
* -
*
* 16384 (16vCPU) - Available memory
values: 32GB and 120 GB in 8 GB increments
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
*
*
* @param cpu
* The number of cpu
units used by the task. If you use the EC2 launch type, this field is
* optional. Any value can be used. If you use the Fargate launch type, this field is required. You must use
* one of the following values. The value that you choose determines your range of valid values for the
* memory
parameter.
*
* The CPU units cannot be less than 1 vCPU when you use Windows containers on Fargate.
*
*
* -
*
* 256 (.25 vCPU) - Available memory
values: 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB)
*
*
* -
*
* 512 (.5 vCPU) - Available memory
values: 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB)
*
*
* -
*
* 1024 (1 vCPU) - Available memory
values: 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB),
* 6144 (6 GB), 7168 (7 GB), 8192 (8 GB)
*
*
* -
*
* 2048 (2 vCPU) - Available memory
values: 4096 (4 GB) and 16384 (16 GB) in increments of 1024
* (1 GB)
*
*
* -
*
* 4096 (4 vCPU) - Available memory
values: 8192 (8 GB) and 30720 (30 GB) in increments of 1024
* (1 GB)
*
*
* -
*
* 8192 (8 vCPU) - Available memory
values: 16 GB and 60 GB in 4 GB increments
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
* -
*
* 16384 (16vCPU) - Available memory
values: 32GB and 120 GB in 8 GB increments
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
*/
public void setCpu(String cpu) {
this.cpu = cpu;
}
/**
*
* The number of cpu
units used by the task. If you use the EC2 launch type, this field is optional.
* Any value can be used. If you use the Fargate launch type, this field is required. You must use one of the
* following values. The value that you choose determines your range of valid values for the memory
* parameter.
*
*
* The CPU units cannot be less than 1 vCPU when you use Windows containers on Fargate.
*
*
* -
*
* 256 (.25 vCPU) - Available memory
values: 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB)
*
*
* -
*
* 512 (.5 vCPU) - Available memory
values: 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB)
*
*
* -
*
* 1024 (1 vCPU) - Available memory
values: 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB), 6144 (6
* GB), 7168 (7 GB), 8192 (8 GB)
*
*
* -
*
* 2048 (2 vCPU) - Available memory
values: 4096 (4 GB) and 16384 (16 GB) in increments of 1024 (1 GB)
*
*
* -
*
* 4096 (4 vCPU) - Available memory
values: 8192 (8 GB) and 30720 (30 GB) in increments of 1024 (1 GB)
*
*
* -
*
* 8192 (8 vCPU) - Available memory
values: 16 GB and 60 GB in 4 GB increments
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
* -
*
* 16384 (16vCPU) - Available memory
values: 32GB and 120 GB in 8 GB increments
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
*
*
* @return The number of cpu
units used by the task. If you use the EC2 launch type, this field is
* optional. Any value can be used. If you use the Fargate launch type, this field is required. You must use
* one of the following values. The value that you choose determines your range of valid values for the
* memory
parameter.
*
* The CPU units cannot be less than 1 vCPU when you use Windows containers on Fargate.
*
*
* -
*
* 256 (.25 vCPU) - Available memory
values: 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB)
*
*
* -
*
* 512 (.5 vCPU) - Available memory
values: 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB)
*
*
* -
*
* 1024 (1 vCPU) - Available memory
values: 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB),
* 6144 (6 GB), 7168 (7 GB), 8192 (8 GB)
*
*
* -
*
* 2048 (2 vCPU) - Available memory
values: 4096 (4 GB) and 16384 (16 GB) in increments of 1024
* (1 GB)
*
*
* -
*
* 4096 (4 vCPU) - Available memory
values: 8192 (8 GB) and 30720 (30 GB) in increments of 1024
* (1 GB)
*
*
* -
*
* 8192 (8 vCPU) - Available memory
values: 16 GB and 60 GB in 4 GB increments
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
* -
*
* 16384 (16vCPU) - Available memory
values: 32GB and 120 GB in 8 GB increments
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
*/
public String getCpu() {
return this.cpu;
}
/**
*
* The number of cpu
units used by the task. If you use the EC2 launch type, this field is optional.
* Any value can be used. If you use the Fargate launch type, this field is required. You must use one of the
* following values. The value that you choose determines your range of valid values for the memory
* parameter.
*
*
* The CPU units cannot be less than 1 vCPU when you use Windows containers on Fargate.
*
*
* -
*
* 256 (.25 vCPU) - Available memory
values: 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB)
*
*
* -
*
* 512 (.5 vCPU) - Available memory
values: 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB)
*
*
* -
*
* 1024 (1 vCPU) - Available memory
values: 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB), 6144 (6
* GB), 7168 (7 GB), 8192 (8 GB)
*
*
* -
*
* 2048 (2 vCPU) - Available memory
values: 4096 (4 GB) and 16384 (16 GB) in increments of 1024 (1 GB)
*
*
* -
*
* 4096 (4 vCPU) - Available memory
values: 8192 (8 GB) and 30720 (30 GB) in increments of 1024 (1 GB)
*
*
* -
*
* 8192 (8 vCPU) - Available memory
values: 16 GB and 60 GB in 4 GB increments
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
* -
*
* 16384 (16vCPU) - Available memory
values: 32GB and 120 GB in 8 GB increments
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
*
*
* @param cpu
* The number of cpu
units used by the task. If you use the EC2 launch type, this field is
* optional. Any value can be used. If you use the Fargate launch type, this field is required. You must use
* one of the following values. The value that you choose determines your range of valid values for the
* memory
parameter.
*
* The CPU units cannot be less than 1 vCPU when you use Windows containers on Fargate.
*
*
* -
*
* 256 (.25 vCPU) - Available memory
values: 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB)
*
*
* -
*
* 512 (.5 vCPU) - Available memory
values: 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB)
*
*
* -
*
* 1024 (1 vCPU) - Available memory
values: 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB),
* 6144 (6 GB), 7168 (7 GB), 8192 (8 GB)
*
*
* -
*
* 2048 (2 vCPU) - Available memory
values: 4096 (4 GB) and 16384 (16 GB) in increments of 1024
* (1 GB)
*
*
* -
*
* 4096 (4 vCPU) - Available memory
values: 8192 (8 GB) and 30720 (30 GB) in increments of 1024
* (1 GB)
*
*
* -
*
* 8192 (8 vCPU) - Available memory
values: 16 GB and 60 GB in 4 GB increments
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
* -
*
* 16384 (16vCPU) - Available memory
values: 32GB and 120 GB in 8 GB increments
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskDefinition withCpu(String cpu) {
setCpu(cpu);
return this;
}
/**
*
* The amount (in MiB) of memory used by the task.
*
*
* If your tasks runs on Amazon EC2 instances, you must specify either a task-level memory value or a
* container-level memory value. This field is optional and any value can be used. If a task-level memory value is
* specified, the container-level memory value is optional. For more information regarding container-level memory
* and memory reservation, see ContainerDefinition.
*
*
* If your tasks runs on Fargate, this field is required. You must use one of the following values. The value you
* choose determines your range of valid values for the cpu
parameter.
*
*
* -
*
* 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB) - Available cpu
values: 256 (.25 vCPU)
*
*
* -
*
* 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB) - Available cpu
values: 512 (.5 vCPU)
*
*
* -
*
* 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB), 6144 (6 GB), 7168 (7 GB), 8192 (8 GB) - Available
* cpu
values: 1024 (1 vCPU)
*
*
* -
*
* Between 4096 (4 GB) and 16384 (16 GB) in increments of 1024 (1 GB) - Available cpu
values: 2048 (2
* vCPU)
*
*
* -
*
* Between 8192 (8 GB) and 30720 (30 GB) in increments of 1024 (1 GB) - Available cpu
values: 4096 (4
* vCPU)
*
*
* -
*
* Between 16 GB and 60 GB in 4 GB increments - Available cpu
values: 8192 (8 vCPU)
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
* -
*
* Between 32GB and 120 GB in 8 GB increments - Available cpu
values: 16384 (16 vCPU)
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
*
*
* @param memory
* The amount (in MiB) of memory used by the task.
*
* If your tasks runs on Amazon EC2 instances, you must specify either a task-level memory value or a
* container-level memory value. This field is optional and any value can be used. If a task-level memory
* value is specified, the container-level memory value is optional. For more information regarding
* container-level memory and memory reservation, see ContainerDefinition.
*
*
* If your tasks runs on Fargate, this field is required. You must use one of the following values. The value
* you choose determines your range of valid values for the cpu
parameter.
*
*
* -
*
* 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB) - Available cpu
values: 256 (.25 vCPU)
*
*
* -
*
* 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB) - Available cpu
values: 512 (.5 vCPU)
*
*
* -
*
* 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB), 6144 (6 GB), 7168 (7 GB), 8192 (8 GB) - Available
* cpu
values: 1024 (1 vCPU)
*
*
* -
*
* Between 4096 (4 GB) and 16384 (16 GB) in increments of 1024 (1 GB) - Available cpu
values:
* 2048 (2 vCPU)
*
*
* -
*
* Between 8192 (8 GB) and 30720 (30 GB) in increments of 1024 (1 GB) - Available cpu
values:
* 4096 (4 vCPU)
*
*
* -
*
* Between 16 GB and 60 GB in 4 GB increments - Available cpu
values: 8192 (8 vCPU)
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
* -
*
* Between 32GB and 120 GB in 8 GB increments - Available cpu
values: 16384 (16 vCPU)
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
*/
public void setMemory(String memory) {
this.memory = memory;
}
/**
*
* The amount (in MiB) of memory used by the task.
*
*
* If your tasks runs on Amazon EC2 instances, you must specify either a task-level memory value or a
* container-level memory value. This field is optional and any value can be used. If a task-level memory value is
* specified, the container-level memory value is optional. For more information regarding container-level memory
* and memory reservation, see ContainerDefinition.
*
*
* If your tasks runs on Fargate, this field is required. You must use one of the following values. The value you
* choose determines your range of valid values for the cpu
parameter.
*
*
* -
*
* 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB) - Available cpu
values: 256 (.25 vCPU)
*
*
* -
*
* 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB) - Available cpu
values: 512 (.5 vCPU)
*
*
* -
*
* 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB), 6144 (6 GB), 7168 (7 GB), 8192 (8 GB) - Available
* cpu
values: 1024 (1 vCPU)
*
*
* -
*
* Between 4096 (4 GB) and 16384 (16 GB) in increments of 1024 (1 GB) - Available cpu
values: 2048 (2
* vCPU)
*
*
* -
*
* Between 8192 (8 GB) and 30720 (30 GB) in increments of 1024 (1 GB) - Available cpu
values: 4096 (4
* vCPU)
*
*
* -
*
* Between 16 GB and 60 GB in 4 GB increments - Available cpu
values: 8192 (8 vCPU)
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
* -
*
* Between 32GB and 120 GB in 8 GB increments - Available cpu
values: 16384 (16 vCPU)
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
*
*
* @return The amount (in MiB) of memory used by the task.
*
* If your tasks runs on Amazon EC2 instances, you must specify either a task-level memory value or a
* container-level memory value. This field is optional and any value can be used. If a task-level memory
* value is specified, the container-level memory value is optional. For more information regarding
* container-level memory and memory reservation, see ContainerDefinition.
*
*
* If your tasks runs on Fargate, this field is required. You must use one of the following values. The
* value you choose determines your range of valid values for the cpu
parameter.
*
*
* -
*
* 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB) - Available cpu
values: 256 (.25 vCPU)
*
*
* -
*
* 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB) - Available cpu
values: 512 (.5 vCPU)
*
*
* -
*
* 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB), 6144 (6 GB), 7168 (7 GB), 8192 (8 GB) - Available
* cpu
values: 1024 (1 vCPU)
*
*
* -
*
* Between 4096 (4 GB) and 16384 (16 GB) in increments of 1024 (1 GB) - Available cpu
values:
* 2048 (2 vCPU)
*
*
* -
*
* Between 8192 (8 GB) and 30720 (30 GB) in increments of 1024 (1 GB) - Available cpu
values:
* 4096 (4 vCPU)
*
*
* -
*
* Between 16 GB and 60 GB in 4 GB increments - Available cpu
values: 8192 (8 vCPU)
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
* -
*
* Between 32GB and 120 GB in 8 GB increments - Available cpu
values: 16384 (16 vCPU)
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
*/
public String getMemory() {
return this.memory;
}
/**
*
* The amount (in MiB) of memory used by the task.
*
*
* If your tasks runs on Amazon EC2 instances, you must specify either a task-level memory value or a
* container-level memory value. This field is optional and any value can be used. If a task-level memory value is
* specified, the container-level memory value is optional. For more information regarding container-level memory
* and memory reservation, see ContainerDefinition.
*
*
* If your tasks runs on Fargate, this field is required. You must use one of the following values. The value you
* choose determines your range of valid values for the cpu
parameter.
*
*
* -
*
* 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB) - Available cpu
values: 256 (.25 vCPU)
*
*
* -
*
* 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB) - Available cpu
values: 512 (.5 vCPU)
*
*
* -
*
* 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB), 6144 (6 GB), 7168 (7 GB), 8192 (8 GB) - Available
* cpu
values: 1024 (1 vCPU)
*
*
* -
*
* Between 4096 (4 GB) and 16384 (16 GB) in increments of 1024 (1 GB) - Available cpu
values: 2048 (2
* vCPU)
*
*
* -
*
* Between 8192 (8 GB) and 30720 (30 GB) in increments of 1024 (1 GB) - Available cpu
values: 4096 (4
* vCPU)
*
*
* -
*
* Between 16 GB and 60 GB in 4 GB increments - Available cpu
values: 8192 (8 vCPU)
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
* -
*
* Between 32GB and 120 GB in 8 GB increments - Available cpu
values: 16384 (16 vCPU)
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
*
*
* @param memory
* The amount (in MiB) of memory used by the task.
*
* If your tasks runs on Amazon EC2 instances, you must specify either a task-level memory value or a
* container-level memory value. This field is optional and any value can be used. If a task-level memory
* value is specified, the container-level memory value is optional. For more information regarding
* container-level memory and memory reservation, see ContainerDefinition.
*
*
* If your tasks runs on Fargate, this field is required. You must use one of the following values. The value
* you choose determines your range of valid values for the cpu
parameter.
*
*
* -
*
* 512 (0.5 GB), 1024 (1 GB), 2048 (2 GB) - Available cpu
values: 256 (.25 vCPU)
*
*
* -
*
* 1024 (1 GB), 2048 (2 GB), 3072 (3 GB), 4096 (4 GB) - Available cpu
values: 512 (.5 vCPU)
*
*
* -
*
* 2048 (2 GB), 3072 (3 GB), 4096 (4 GB), 5120 (5 GB), 6144 (6 GB), 7168 (7 GB), 8192 (8 GB) - Available
* cpu
values: 1024 (1 vCPU)
*
*
* -
*
* Between 4096 (4 GB) and 16384 (16 GB) in increments of 1024 (1 GB) - Available cpu
values:
* 2048 (2 vCPU)
*
*
* -
*
* Between 8192 (8 GB) and 30720 (30 GB) in increments of 1024 (1 GB) - Available cpu
values:
* 4096 (4 vCPU)
*
*
* -
*
* Between 16 GB and 60 GB in 4 GB increments - Available cpu
values: 8192 (8 vCPU)
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
* -
*
* Between 32GB and 120 GB in 8 GB increments - Available cpu
values: 16384 (16 vCPU)
*
*
* This option requires Linux platform 1.4.0
or later.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskDefinition withMemory(String memory) {
setMemory(memory);
return this;
}
/**
*
* The Elastic Inference accelerator that's associated with the task.
*
*
* @return The Elastic Inference accelerator that's associated with the task.
*/
public java.util.List getInferenceAccelerators() {
if (inferenceAccelerators == null) {
inferenceAccelerators = new com.amazonaws.internal.SdkInternalList();
}
return inferenceAccelerators;
}
/**
*
* The Elastic Inference accelerator that's associated with the task.
*
*
* @param inferenceAccelerators
* The Elastic Inference accelerator that's associated with the task.
*/
public void setInferenceAccelerators(java.util.Collection inferenceAccelerators) {
if (inferenceAccelerators == null) {
this.inferenceAccelerators = null;
return;
}
this.inferenceAccelerators = new com.amazonaws.internal.SdkInternalList(inferenceAccelerators);
}
/**
*
* The Elastic Inference accelerator that's associated with the task.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setInferenceAccelerators(java.util.Collection)} or
* {@link #withInferenceAccelerators(java.util.Collection)} if you want to override the existing values.
*
*
* @param inferenceAccelerators
* The Elastic Inference accelerator that's associated with the task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskDefinition withInferenceAccelerators(InferenceAccelerator... inferenceAccelerators) {
if (this.inferenceAccelerators == null) {
setInferenceAccelerators(new com.amazonaws.internal.SdkInternalList(inferenceAccelerators.length));
}
for (InferenceAccelerator ele : inferenceAccelerators) {
this.inferenceAccelerators.add(ele);
}
return this;
}
/**
*
* The Elastic Inference accelerator that's associated with the task.
*
*
* @param inferenceAccelerators
* The Elastic Inference accelerator that's associated with the task.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskDefinition withInferenceAccelerators(java.util.Collection inferenceAccelerators) {
setInferenceAccelerators(inferenceAccelerators);
return this;
}
/**
*
* The process namespace to use for the containers in the task. The valid values are host
or
* task
. On Fargate for Linux containers, the only valid value is task
. For example,
* monitoring sidecars might need pidMode
to access information about other containers running in the
* same task.
*
*
* If host
is specified, all containers within the tasks that specified the host
PID mode
* on the same container instance share the same process namespace with the host Amazon EC2 instance.
*
*
* If task
is specified, all containers within the specified task share the same process namespace.
*
*
* If no value is specified, the default is a private namespace for each container. For more information, see PID settings in the Docker run
* reference.
*
*
* If the host
PID mode is used, there's a heightened risk of undesired process namespace exposure. For
* more information, see Docker security.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* This parameter is only supported for tasks that are hosted on Fargate if the tasks are using platform version
* 1.4.0
or later (Linux). This isn't supported for Windows containers on Fargate.
*
*
*
* @param pidMode
* The process namespace to use for the containers in the task. The valid values are host
or
* task
. On Fargate for Linux containers, the only valid value is task
. For
* example, monitoring sidecars might need pidMode
to access information about other containers
* running in the same task.
*
* If host
is specified, all containers within the tasks that specified the host
* PID mode on the same container instance share the same process namespace with the host Amazon EC2
* instance.
*
*
* If task
is specified, all containers within the specified task share the same process
* namespace.
*
*
* If no value is specified, the default is a private namespace for each container. For more information, see
* PID settings in the
* Docker run reference.
*
*
* If the host
PID mode is used, there's a heightened risk of undesired process namespace
* exposure. For more information, see Docker
* security.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* This parameter is only supported for tasks that are hosted on Fargate if the tasks are using platform
* version 1.4.0
or later (Linux). This isn't supported for Windows containers on Fargate.
*
* @see PidMode
*/
public void setPidMode(String pidMode) {
this.pidMode = pidMode;
}
/**
*
* The process namespace to use for the containers in the task. The valid values are host
or
* task
. On Fargate for Linux containers, the only valid value is task
. For example,
* monitoring sidecars might need pidMode
to access information about other containers running in the
* same task.
*
*
* If host
is specified, all containers within the tasks that specified the host
PID mode
* on the same container instance share the same process namespace with the host Amazon EC2 instance.
*
*
* If task
is specified, all containers within the specified task share the same process namespace.
*
*
* If no value is specified, the default is a private namespace for each container. For more information, see PID settings in the Docker run
* reference.
*
*
* If the host
PID mode is used, there's a heightened risk of undesired process namespace exposure. For
* more information, see Docker security.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* This parameter is only supported for tasks that are hosted on Fargate if the tasks are using platform version
* 1.4.0
or later (Linux). This isn't supported for Windows containers on Fargate.
*
*
*
* @return The process namespace to use for the containers in the task. The valid values are host
or
* task
. On Fargate for Linux containers, the only valid value is task
. For
* example, monitoring sidecars might need pidMode
to access information about other containers
* running in the same task.
*
* If host
is specified, all containers within the tasks that specified the host
* PID mode on the same container instance share the same process namespace with the host Amazon EC2
* instance.
*
*
* If task
is specified, all containers within the specified task share the same process
* namespace.
*
*
* If no value is specified, the default is a private namespace for each container. For more information,
* see PID settings in the
* Docker run reference.
*
*
* If the host
PID mode is used, there's a heightened risk of undesired process namespace
* exposure. For more information, see Docker
* security.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* This parameter is only supported for tasks that are hosted on Fargate if the tasks are using platform
* version 1.4.0
or later (Linux). This isn't supported for Windows containers on Fargate.
*
* @see PidMode
*/
public String getPidMode() {
return this.pidMode;
}
/**
*
* The process namespace to use for the containers in the task. The valid values are host
or
* task
. On Fargate for Linux containers, the only valid value is task
. For example,
* monitoring sidecars might need pidMode
to access information about other containers running in the
* same task.
*
*
* If host
is specified, all containers within the tasks that specified the host
PID mode
* on the same container instance share the same process namespace with the host Amazon EC2 instance.
*
*
* If task
is specified, all containers within the specified task share the same process namespace.
*
*
* If no value is specified, the default is a private namespace for each container. For more information, see PID settings in the Docker run
* reference.
*
*
* If the host
PID mode is used, there's a heightened risk of undesired process namespace exposure. For
* more information, see Docker security.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* This parameter is only supported for tasks that are hosted on Fargate if the tasks are using platform version
* 1.4.0
or later (Linux). This isn't supported for Windows containers on Fargate.
*
*
*
* @param pidMode
* The process namespace to use for the containers in the task. The valid values are host
or
* task
. On Fargate for Linux containers, the only valid value is task
. For
* example, monitoring sidecars might need pidMode
to access information about other containers
* running in the same task.
*
* If host
is specified, all containers within the tasks that specified the host
* PID mode on the same container instance share the same process namespace with the host Amazon EC2
* instance.
*
*
* If task
is specified, all containers within the specified task share the same process
* namespace.
*
*
* If no value is specified, the default is a private namespace for each container. For more information, see
* PID settings in the
* Docker run reference.
*
*
* If the host
PID mode is used, there's a heightened risk of undesired process namespace
* exposure. For more information, see Docker
* security.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* This parameter is only supported for tasks that are hosted on Fargate if the tasks are using platform
* version 1.4.0
or later (Linux). This isn't supported for Windows containers on Fargate.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see PidMode
*/
public TaskDefinition withPidMode(String pidMode) {
setPidMode(pidMode);
return this;
}
/**
*
* The process namespace to use for the containers in the task. The valid values are host
or
* task
. On Fargate for Linux containers, the only valid value is task
. For example,
* monitoring sidecars might need pidMode
to access information about other containers running in the
* same task.
*
*
* If host
is specified, all containers within the tasks that specified the host
PID mode
* on the same container instance share the same process namespace with the host Amazon EC2 instance.
*
*
* If task
is specified, all containers within the specified task share the same process namespace.
*
*
* If no value is specified, the default is a private namespace for each container. For more information, see PID settings in the Docker run
* reference.
*
*
* If the host
PID mode is used, there's a heightened risk of undesired process namespace exposure. For
* more information, see Docker security.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* This parameter is only supported for tasks that are hosted on Fargate if the tasks are using platform version
* 1.4.0
or later (Linux). This isn't supported for Windows containers on Fargate.
*
*
*
* @param pidMode
* The process namespace to use for the containers in the task. The valid values are host
or
* task
. On Fargate for Linux containers, the only valid value is task
. For
* example, monitoring sidecars might need pidMode
to access information about other containers
* running in the same task.
*
* If host
is specified, all containers within the tasks that specified the host
* PID mode on the same container instance share the same process namespace with the host Amazon EC2
* instance.
*
*
* If task
is specified, all containers within the specified task share the same process
* namespace.
*
*
* If no value is specified, the default is a private namespace for each container. For more information, see
* PID settings in the
* Docker run reference.
*
*
* If the host
PID mode is used, there's a heightened risk of undesired process namespace
* exposure. For more information, see Docker
* security.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* This parameter is only supported for tasks that are hosted on Fargate if the tasks are using platform
* version 1.4.0
or later (Linux). This isn't supported for Windows containers on Fargate.
*
* @see PidMode
*/
public void setPidMode(PidMode pidMode) {
withPidMode(pidMode);
}
/**
*
* The process namespace to use for the containers in the task. The valid values are host
or
* task
. On Fargate for Linux containers, the only valid value is task
. For example,
* monitoring sidecars might need pidMode
to access information about other containers running in the
* same task.
*
*
* If host
is specified, all containers within the tasks that specified the host
PID mode
* on the same container instance share the same process namespace with the host Amazon EC2 instance.
*
*
* If task
is specified, all containers within the specified task share the same process namespace.
*
*
* If no value is specified, the default is a private namespace for each container. For more information, see PID settings in the Docker run
* reference.
*
*
* If the host
PID mode is used, there's a heightened risk of undesired process namespace exposure. For
* more information, see Docker security.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* This parameter is only supported for tasks that are hosted on Fargate if the tasks are using platform version
* 1.4.0
or later (Linux). This isn't supported for Windows containers on Fargate.
*
*
*
* @param pidMode
* The process namespace to use for the containers in the task. The valid values are host
or
* task
. On Fargate for Linux containers, the only valid value is task
. For
* example, monitoring sidecars might need pidMode
to access information about other containers
* running in the same task.
*
* If host
is specified, all containers within the tasks that specified the host
* PID mode on the same container instance share the same process namespace with the host Amazon EC2
* instance.
*
*
* If task
is specified, all containers within the specified task share the same process
* namespace.
*
*
* If no value is specified, the default is a private namespace for each container. For more information, see
* PID settings in the
* Docker run reference.
*
*
* If the host
PID mode is used, there's a heightened risk of undesired process namespace
* exposure. For more information, see Docker
* security.
*
*
*
* This parameter is not supported for Windows containers.
*
*
*
* This parameter is only supported for tasks that are hosted on Fargate if the tasks are using platform
* version 1.4.0
or later (Linux). This isn't supported for Windows containers on Fargate.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see PidMode
*/
public TaskDefinition withPidMode(PidMode pidMode) {
this.pidMode = pidMode.toString();
return this;
}
/**
*
* The IPC resource namespace to use for the containers in the task. The valid values are host
,
* task
, or none
. If host
is specified, then all containers within the tasks
* that specified the host
IPC mode on the same container instance share the same IPC resources with
* the host Amazon EC2 instance. If task
is specified, all containers within the specified task share
* the same IPC resources. If none
is specified, then IPC resources within the containers of a task are
* private and not shared with other containers in a task or on the container instance. If no value is specified,
* then the IPC resource namespace sharing depends on the Docker daemon setting on the container instance. For more
* information, see IPC settings in
* the Docker run reference.
*
*
* If the host
IPC mode is used, be aware that there is a heightened risk of undesired IPC namespace
* expose. For more information, see Docker
* security.
*
*
* If you are setting namespaced kernel parameters using systemControls
for the containers in the task,
* the following will apply to your IPC resource namespace. For more information, see System
* Controls in the Amazon Elastic Container Service Developer Guide.
*
*
* -
*
* For tasks that use the host
IPC mode, IPC namespace related systemControls
are not
* supported.
*
*
* -
*
* For tasks that use the task
IPC mode, IPC namespace related systemControls
will apply
* to all containers within a task.
*
*
*
*
*
* This parameter is not supported for Windows containers or tasks run on Fargate.
*
*
*
* @param ipcMode
* The IPC resource namespace to use for the containers in the task. The valid values are host
,
* task
, or none
. If host
is specified, then all containers within the
* tasks that specified the host
IPC mode on the same container instance share the same IPC
* resources with the host Amazon EC2 instance. If task
is specified, all containers within the
* specified task share the same IPC resources. If none
is specified, then IPC resources within
* the containers of a task are private and not shared with other containers in a task or on the container
* instance. If no value is specified, then the IPC resource namespace sharing depends on the Docker daemon
* setting on the container instance. For more information, see IPC settings in the Docker
* run reference.
*
* If the host
IPC mode is used, be aware that there is a heightened risk of undesired IPC
* namespace expose. For more information, see Docker security.
*
*
* If you are setting namespaced kernel parameters using systemControls
for the containers in
* the task, the following will apply to your IPC resource namespace. For more information, see System
* Controls in the Amazon Elastic Container Service Developer Guide.
*
*
* -
*
* For tasks that use the host
IPC mode, IPC namespace related systemControls
are
* not supported.
*
*
* -
*
* For tasks that use the task
IPC mode, IPC namespace related systemControls
will
* apply to all containers within a task.
*
*
*
*
*
* This parameter is not supported for Windows containers or tasks run on Fargate.
*
* @see IpcMode
*/
public void setIpcMode(String ipcMode) {
this.ipcMode = ipcMode;
}
/**
*
* The IPC resource namespace to use for the containers in the task. The valid values are host
,
* task
, or none
. If host
is specified, then all containers within the tasks
* that specified the host
IPC mode on the same container instance share the same IPC resources with
* the host Amazon EC2 instance. If task
is specified, all containers within the specified task share
* the same IPC resources. If none
is specified, then IPC resources within the containers of a task are
* private and not shared with other containers in a task or on the container instance. If no value is specified,
* then the IPC resource namespace sharing depends on the Docker daemon setting on the container instance. For more
* information, see IPC settings in
* the Docker run reference.
*
*
* If the host
IPC mode is used, be aware that there is a heightened risk of undesired IPC namespace
* expose. For more information, see Docker
* security.
*
*
* If you are setting namespaced kernel parameters using systemControls
for the containers in the task,
* the following will apply to your IPC resource namespace. For more information, see System
* Controls in the Amazon Elastic Container Service Developer Guide.
*
*
* -
*
* For tasks that use the host
IPC mode, IPC namespace related systemControls
are not
* supported.
*
*
* -
*
* For tasks that use the task
IPC mode, IPC namespace related systemControls
will apply
* to all containers within a task.
*
*
*
*
*
* This parameter is not supported for Windows containers or tasks run on Fargate.
*
*
*
* @return The IPC resource namespace to use for the containers in the task. The valid values are host
,
* task
, or none
. If host
is specified, then all containers within
* the tasks that specified the host
IPC mode on the same container instance share the same IPC
* resources with the host Amazon EC2 instance. If task
is specified, all containers within the
* specified task share the same IPC resources. If none
is specified, then IPC resources within
* the containers of a task are private and not shared with other containers in a task or on the container
* instance. If no value is specified, then the IPC resource namespace sharing depends on the Docker daemon
* setting on the container instance. For more information, see IPC settings in the Docker
* run reference.
*
* If the host
IPC mode is used, be aware that there is a heightened risk of undesired IPC
* namespace expose. For more information, see Docker security.
*
*
* If you are setting namespaced kernel parameters using systemControls
for the containers in
* the task, the following will apply to your IPC resource namespace. For more information, see System
* Controls in the Amazon Elastic Container Service Developer Guide.
*
*
* -
*
* For tasks that use the host
IPC mode, IPC namespace related systemControls
are
* not supported.
*
*
* -
*
* For tasks that use the task
IPC mode, IPC namespace related systemControls
will
* apply to all containers within a task.
*
*
*
*
*
* This parameter is not supported for Windows containers or tasks run on Fargate.
*
* @see IpcMode
*/
public String getIpcMode() {
return this.ipcMode;
}
/**
*
* The IPC resource namespace to use for the containers in the task. The valid values are host
,
* task
, or none
. If host
is specified, then all containers within the tasks
* that specified the host
IPC mode on the same container instance share the same IPC resources with
* the host Amazon EC2 instance. If task
is specified, all containers within the specified task share
* the same IPC resources. If none
is specified, then IPC resources within the containers of a task are
* private and not shared with other containers in a task or on the container instance. If no value is specified,
* then the IPC resource namespace sharing depends on the Docker daemon setting on the container instance. For more
* information, see IPC settings in
* the Docker run reference.
*
*
* If the host
IPC mode is used, be aware that there is a heightened risk of undesired IPC namespace
* expose. For more information, see Docker
* security.
*
*
* If you are setting namespaced kernel parameters using systemControls
for the containers in the task,
* the following will apply to your IPC resource namespace. For more information, see System
* Controls in the Amazon Elastic Container Service Developer Guide.
*
*
* -
*
* For tasks that use the host
IPC mode, IPC namespace related systemControls
are not
* supported.
*
*
* -
*
* For tasks that use the task
IPC mode, IPC namespace related systemControls
will apply
* to all containers within a task.
*
*
*
*
*
* This parameter is not supported for Windows containers or tasks run on Fargate.
*
*
*
* @param ipcMode
* The IPC resource namespace to use for the containers in the task. The valid values are host
,
* task
, or none
. If host
is specified, then all containers within the
* tasks that specified the host
IPC mode on the same container instance share the same IPC
* resources with the host Amazon EC2 instance. If task
is specified, all containers within the
* specified task share the same IPC resources. If none
is specified, then IPC resources within
* the containers of a task are private and not shared with other containers in a task or on the container
* instance. If no value is specified, then the IPC resource namespace sharing depends on the Docker daemon
* setting on the container instance. For more information, see IPC settings in the Docker
* run reference.
*
* If the host
IPC mode is used, be aware that there is a heightened risk of undesired IPC
* namespace expose. For more information, see Docker security.
*
*
* If you are setting namespaced kernel parameters using systemControls
for the containers in
* the task, the following will apply to your IPC resource namespace. For more information, see System
* Controls in the Amazon Elastic Container Service Developer Guide.
*
*
* -
*
* For tasks that use the host
IPC mode, IPC namespace related systemControls
are
* not supported.
*
*
* -
*
* For tasks that use the task
IPC mode, IPC namespace related systemControls
will
* apply to all containers within a task.
*
*
*
*
*
* This parameter is not supported for Windows containers or tasks run on Fargate.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see IpcMode
*/
public TaskDefinition withIpcMode(String ipcMode) {
setIpcMode(ipcMode);
return this;
}
/**
*
* The IPC resource namespace to use for the containers in the task. The valid values are host
,
* task
, or none
. If host
is specified, then all containers within the tasks
* that specified the host
IPC mode on the same container instance share the same IPC resources with
* the host Amazon EC2 instance. If task
is specified, all containers within the specified task share
* the same IPC resources. If none
is specified, then IPC resources within the containers of a task are
* private and not shared with other containers in a task or on the container instance. If no value is specified,
* then the IPC resource namespace sharing depends on the Docker daemon setting on the container instance. For more
* information, see IPC settings in
* the Docker run reference.
*
*
* If the host
IPC mode is used, be aware that there is a heightened risk of undesired IPC namespace
* expose. For more information, see Docker
* security.
*
*
* If you are setting namespaced kernel parameters using systemControls
for the containers in the task,
* the following will apply to your IPC resource namespace. For more information, see System
* Controls in the Amazon Elastic Container Service Developer Guide.
*
*
* -
*
* For tasks that use the host
IPC mode, IPC namespace related systemControls
are not
* supported.
*
*
* -
*
* For tasks that use the task
IPC mode, IPC namespace related systemControls
will apply
* to all containers within a task.
*
*
*
*
*
* This parameter is not supported for Windows containers or tasks run on Fargate.
*
*
*
* @param ipcMode
* The IPC resource namespace to use for the containers in the task. The valid values are host
,
* task
, or none
. If host
is specified, then all containers within the
* tasks that specified the host
IPC mode on the same container instance share the same IPC
* resources with the host Amazon EC2 instance. If task
is specified, all containers within the
* specified task share the same IPC resources. If none
is specified, then IPC resources within
* the containers of a task are private and not shared with other containers in a task or on the container
* instance. If no value is specified, then the IPC resource namespace sharing depends on the Docker daemon
* setting on the container instance. For more information, see IPC settings in the Docker
* run reference.
*
* If the host
IPC mode is used, be aware that there is a heightened risk of undesired IPC
* namespace expose. For more information, see Docker security.
*
*
* If you are setting namespaced kernel parameters using systemControls
for the containers in
* the task, the following will apply to your IPC resource namespace. For more information, see System
* Controls in the Amazon Elastic Container Service Developer Guide.
*
*
* -
*
* For tasks that use the host
IPC mode, IPC namespace related systemControls
are
* not supported.
*
*
* -
*
* For tasks that use the task
IPC mode, IPC namespace related systemControls
will
* apply to all containers within a task.
*
*
*
*
*
* This parameter is not supported for Windows containers or tasks run on Fargate.
*
* @see IpcMode
*/
public void setIpcMode(IpcMode ipcMode) {
withIpcMode(ipcMode);
}
/**
*
* The IPC resource namespace to use for the containers in the task. The valid values are host
,
* task
, or none
. If host
is specified, then all containers within the tasks
* that specified the host
IPC mode on the same container instance share the same IPC resources with
* the host Amazon EC2 instance. If task
is specified, all containers within the specified task share
* the same IPC resources. If none
is specified, then IPC resources within the containers of a task are
* private and not shared with other containers in a task or on the container instance. If no value is specified,
* then the IPC resource namespace sharing depends on the Docker daemon setting on the container instance. For more
* information, see IPC settings in
* the Docker run reference.
*
*
* If the host
IPC mode is used, be aware that there is a heightened risk of undesired IPC namespace
* expose. For more information, see Docker
* security.
*
*
* If you are setting namespaced kernel parameters using systemControls
for the containers in the task,
* the following will apply to your IPC resource namespace. For more information, see System
* Controls in the Amazon Elastic Container Service Developer Guide.
*
*
* -
*
* For tasks that use the host
IPC mode, IPC namespace related systemControls
are not
* supported.
*
*
* -
*
* For tasks that use the task
IPC mode, IPC namespace related systemControls
will apply
* to all containers within a task.
*
*
*
*
*
* This parameter is not supported for Windows containers or tasks run on Fargate.
*
*
*
* @param ipcMode
* The IPC resource namespace to use for the containers in the task. The valid values are host
,
* task
, or none
. If host
is specified, then all containers within the
* tasks that specified the host
IPC mode on the same container instance share the same IPC
* resources with the host Amazon EC2 instance. If task
is specified, all containers within the
* specified task share the same IPC resources. If none
is specified, then IPC resources within
* the containers of a task are private and not shared with other containers in a task or on the container
* instance. If no value is specified, then the IPC resource namespace sharing depends on the Docker daemon
* setting on the container instance. For more information, see IPC settings in the Docker
* run reference.
*
* If the host
IPC mode is used, be aware that there is a heightened risk of undesired IPC
* namespace expose. For more information, see Docker security.
*
*
* If you are setting namespaced kernel parameters using systemControls
for the containers in
* the task, the following will apply to your IPC resource namespace. For more information, see System
* Controls in the Amazon Elastic Container Service Developer Guide.
*
*
* -
*
* For tasks that use the host
IPC mode, IPC namespace related systemControls
are
* not supported.
*
*
* -
*
* For tasks that use the task
IPC mode, IPC namespace related systemControls
will
* apply to all containers within a task.
*
*
*
*
*
* This parameter is not supported for Windows containers or tasks run on Fargate.
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see IpcMode
*/
public TaskDefinition withIpcMode(IpcMode ipcMode) {
this.ipcMode = ipcMode.toString();
return this;
}
/**
*
* The configuration details for the App Mesh proxy.
*
*
* Your Amazon ECS container instances require at least version 1.26.0 of the container agent and at least version
* 1.26.0-1 of the ecs-init
package to use a proxy configuration. If your container instances are
* launched from the Amazon ECS optimized AMI version 20190301
or later, they contain the required
* versions of the container agent and ecs-init
. For more information, see Amazon ECS-optimized
* Linux AMI in the Amazon Elastic Container Service Developer Guide.
*
*
* @param proxyConfiguration
* The configuration details for the App Mesh proxy.
*
* Your Amazon ECS container instances require at least version 1.26.0 of the container agent and at least
* version 1.26.0-1 of the ecs-init
package to use a proxy configuration. If your container
* instances are launched from the Amazon ECS optimized AMI version 20190301
or later, they
* contain the required versions of the container agent and ecs-init
. For more information, see
* Amazon
* ECS-optimized Linux AMI in the Amazon Elastic Container Service Developer Guide.
*/
public void setProxyConfiguration(ProxyConfiguration proxyConfiguration) {
this.proxyConfiguration = proxyConfiguration;
}
/**
*
* The configuration details for the App Mesh proxy.
*
*
* Your Amazon ECS container instances require at least version 1.26.0 of the container agent and at least version
* 1.26.0-1 of the ecs-init
package to use a proxy configuration. If your container instances are
* launched from the Amazon ECS optimized AMI version 20190301
or later, they contain the required
* versions of the container agent and ecs-init
. For more information, see Amazon ECS-optimized
* Linux AMI in the Amazon Elastic Container Service Developer Guide.
*
*
* @return The configuration details for the App Mesh proxy.
*
* Your Amazon ECS container instances require at least version 1.26.0 of the container agent and at least
* version 1.26.0-1 of the ecs-init
package to use a proxy configuration. If your container
* instances are launched from the Amazon ECS optimized AMI version 20190301
or later, they
* contain the required versions of the container agent and ecs-init
. For more information, see
* Amazon
* ECS-optimized Linux AMI in the Amazon Elastic Container Service Developer Guide.
*/
public ProxyConfiguration getProxyConfiguration() {
return this.proxyConfiguration;
}
/**
*
* The configuration details for the App Mesh proxy.
*
*
* Your Amazon ECS container instances require at least version 1.26.0 of the container agent and at least version
* 1.26.0-1 of the ecs-init
package to use a proxy configuration. If your container instances are
* launched from the Amazon ECS optimized AMI version 20190301
or later, they contain the required
* versions of the container agent and ecs-init
. For more information, see Amazon ECS-optimized
* Linux AMI in the Amazon Elastic Container Service Developer Guide.
*
*
* @param proxyConfiguration
* The configuration details for the App Mesh proxy.
*
* Your Amazon ECS container instances require at least version 1.26.0 of the container agent and at least
* version 1.26.0-1 of the ecs-init
package to use a proxy configuration. If your container
* instances are launched from the Amazon ECS optimized AMI version 20190301
or later, they
* contain the required versions of the container agent and ecs-init
. For more information, see
* Amazon
* ECS-optimized Linux AMI in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskDefinition withProxyConfiguration(ProxyConfiguration proxyConfiguration) {
setProxyConfiguration(proxyConfiguration);
return this;
}
/**
*
* The Unix timestamp for the time when the task definition was registered.
*
*
* @param registeredAt
* The Unix timestamp for the time when the task definition was registered.
*/
public void setRegisteredAt(java.util.Date registeredAt) {
this.registeredAt = registeredAt;
}
/**
*
* The Unix timestamp for the time when the task definition was registered.
*
*
* @return The Unix timestamp for the time when the task definition was registered.
*/
public java.util.Date getRegisteredAt() {
return this.registeredAt;
}
/**
*
* The Unix timestamp for the time when the task definition was registered.
*
*
* @param registeredAt
* The Unix timestamp for the time when the task definition was registered.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskDefinition withRegisteredAt(java.util.Date registeredAt) {
setRegisteredAt(registeredAt);
return this;
}
/**
*
* The Unix timestamp for the time when the task definition was deregistered.
*
*
* @param deregisteredAt
* The Unix timestamp for the time when the task definition was deregistered.
*/
public void setDeregisteredAt(java.util.Date deregisteredAt) {
this.deregisteredAt = deregisteredAt;
}
/**
*
* The Unix timestamp for the time when the task definition was deregistered.
*
*
* @return The Unix timestamp for the time when the task definition was deregistered.
*/
public java.util.Date getDeregisteredAt() {
return this.deregisteredAt;
}
/**
*
* The Unix timestamp for the time when the task definition was deregistered.
*
*
* @param deregisteredAt
* The Unix timestamp for the time when the task definition was deregistered.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskDefinition withDeregisteredAt(java.util.Date deregisteredAt) {
setDeregisteredAt(deregisteredAt);
return this;
}
/**
*
* The principal that registered the task definition.
*
*
* @param registeredBy
* The principal that registered the task definition.
*/
public void setRegisteredBy(String registeredBy) {
this.registeredBy = registeredBy;
}
/**
*
* The principal that registered the task definition.
*
*
* @return The principal that registered the task definition.
*/
public String getRegisteredBy() {
return this.registeredBy;
}
/**
*
* The principal that registered the task definition.
*
*
* @param registeredBy
* The principal that registered the task definition.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskDefinition withRegisteredBy(String registeredBy) {
setRegisteredBy(registeredBy);
return this;
}
/**
*
* The ephemeral storage settings to use for tasks run with the task definition.
*
*
* @param ephemeralStorage
* The ephemeral storage settings to use for tasks run with the task definition.
*/
public void setEphemeralStorage(EphemeralStorage ephemeralStorage) {
this.ephemeralStorage = ephemeralStorage;
}
/**
*
* The ephemeral storage settings to use for tasks run with the task definition.
*
*
* @return The ephemeral storage settings to use for tasks run with the task definition.
*/
public EphemeralStorage getEphemeralStorage() {
return this.ephemeralStorage;
}
/**
*
* The ephemeral storage settings to use for tasks run with the task definition.
*
*
* @param ephemeralStorage
* The ephemeral storage settings to use for tasks run with the task definition.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskDefinition withEphemeralStorage(EphemeralStorage ephemeralStorage) {
setEphemeralStorage(ephemeralStorage);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getTaskDefinitionArn() != null)
sb.append("TaskDefinitionArn: ").append(getTaskDefinitionArn()).append(",");
if (getContainerDefinitions() != null)
sb.append("ContainerDefinitions: ").append(getContainerDefinitions()).append(",");
if (getFamily() != null)
sb.append("Family: ").append(getFamily()).append(",");
if (getTaskRoleArn() != null)
sb.append("TaskRoleArn: ").append(getTaskRoleArn()).append(",");
if (getExecutionRoleArn() != null)
sb.append("ExecutionRoleArn: ").append(getExecutionRoleArn()).append(",");
if (getNetworkMode() != null)
sb.append("NetworkMode: ").append(getNetworkMode()).append(",");
if (getRevision() != null)
sb.append("Revision: ").append(getRevision()).append(",");
if (getVolumes() != null)
sb.append("Volumes: ").append(getVolumes()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getRequiresAttributes() != null)
sb.append("RequiresAttributes: ").append(getRequiresAttributes()).append(",");
if (getPlacementConstraints() != null)
sb.append("PlacementConstraints: ").append(getPlacementConstraints()).append(",");
if (getCompatibilities() != null)
sb.append("Compatibilities: ").append(getCompatibilities()).append(",");
if (getRuntimePlatform() != null)
sb.append("RuntimePlatform: ").append(getRuntimePlatform()).append(",");
if (getRequiresCompatibilities() != null)
sb.append("RequiresCompatibilities: ").append(getRequiresCompatibilities()).append(",");
if (getCpu() != null)
sb.append("Cpu: ").append(getCpu()).append(",");
if (getMemory() != null)
sb.append("Memory: ").append(getMemory()).append(",");
if (getInferenceAccelerators() != null)
sb.append("InferenceAccelerators: ").append(getInferenceAccelerators()).append(",");
if (getPidMode() != null)
sb.append("PidMode: ").append(getPidMode()).append(",");
if (getIpcMode() != null)
sb.append("IpcMode: ").append(getIpcMode()).append(",");
if (getProxyConfiguration() != null)
sb.append("ProxyConfiguration: ").append(getProxyConfiguration()).append(",");
if (getRegisteredAt() != null)
sb.append("RegisteredAt: ").append(getRegisteredAt()).append(",");
if (getDeregisteredAt() != null)
sb.append("DeregisteredAt: ").append(getDeregisteredAt()).append(",");
if (getRegisteredBy() != null)
sb.append("RegisteredBy: ").append(getRegisteredBy()).append(",");
if (getEphemeralStorage() != null)
sb.append("EphemeralStorage: ").append(getEphemeralStorage());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof TaskDefinition == false)
return false;
TaskDefinition other = (TaskDefinition) obj;
if (other.getTaskDefinitionArn() == null ^ this.getTaskDefinitionArn() == null)
return false;
if (other.getTaskDefinitionArn() != null && other.getTaskDefinitionArn().equals(this.getTaskDefinitionArn()) == false)
return false;
if (other.getContainerDefinitions() == null ^ this.getContainerDefinitions() == null)
return false;
if (other.getContainerDefinitions() != null && other.getContainerDefinitions().equals(this.getContainerDefinitions()) == false)
return false;
if (other.getFamily() == null ^ this.getFamily() == null)
return false;
if (other.getFamily() != null && other.getFamily().equals(this.getFamily()) == false)
return false;
if (other.getTaskRoleArn() == null ^ this.getTaskRoleArn() == null)
return false;
if (other.getTaskRoleArn() != null && other.getTaskRoleArn().equals(this.getTaskRoleArn()) == false)
return false;
if (other.getExecutionRoleArn() == null ^ this.getExecutionRoleArn() == null)
return false;
if (other.getExecutionRoleArn() != null && other.getExecutionRoleArn().equals(this.getExecutionRoleArn()) == false)
return false;
if (other.getNetworkMode() == null ^ this.getNetworkMode() == null)
return false;
if (other.getNetworkMode() != null && other.getNetworkMode().equals(this.getNetworkMode()) == false)
return false;
if (other.getRevision() == null ^ this.getRevision() == null)
return false;
if (other.getRevision() != null && other.getRevision().equals(this.getRevision()) == false)
return false;
if (other.getVolumes() == null ^ this.getVolumes() == null)
return false;
if (other.getVolumes() != null && other.getVolumes().equals(this.getVolumes()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getRequiresAttributes() == null ^ this.getRequiresAttributes() == null)
return false;
if (other.getRequiresAttributes() != null && other.getRequiresAttributes().equals(this.getRequiresAttributes()) == false)
return false;
if (other.getPlacementConstraints() == null ^ this.getPlacementConstraints() == null)
return false;
if (other.getPlacementConstraints() != null && other.getPlacementConstraints().equals(this.getPlacementConstraints()) == false)
return false;
if (other.getCompatibilities() == null ^ this.getCompatibilities() == null)
return false;
if (other.getCompatibilities() != null && other.getCompatibilities().equals(this.getCompatibilities()) == false)
return false;
if (other.getRuntimePlatform() == null ^ this.getRuntimePlatform() == null)
return false;
if (other.getRuntimePlatform() != null && other.getRuntimePlatform().equals(this.getRuntimePlatform()) == false)
return false;
if (other.getRequiresCompatibilities() == null ^ this.getRequiresCompatibilities() == null)
return false;
if (other.getRequiresCompatibilities() != null && other.getRequiresCompatibilities().equals(this.getRequiresCompatibilities()) == false)
return false;
if (other.getCpu() == null ^ this.getCpu() == null)
return false;
if (other.getCpu() != null && other.getCpu().equals(this.getCpu()) == false)
return false;
if (other.getMemory() == null ^ this.getMemory() == null)
return false;
if (other.getMemory() != null && other.getMemory().equals(this.getMemory()) == false)
return false;
if (other.getInferenceAccelerators() == null ^ this.getInferenceAccelerators() == null)
return false;
if (other.getInferenceAccelerators() != null && other.getInferenceAccelerators().equals(this.getInferenceAccelerators()) == false)
return false;
if (other.getPidMode() == null ^ this.getPidMode() == null)
return false;
if (other.getPidMode() != null && other.getPidMode().equals(this.getPidMode()) == false)
return false;
if (other.getIpcMode() == null ^ this.getIpcMode() == null)
return false;
if (other.getIpcMode() != null && other.getIpcMode().equals(this.getIpcMode()) == false)
return false;
if (other.getProxyConfiguration() == null ^ this.getProxyConfiguration() == null)
return false;
if (other.getProxyConfiguration() != null && other.getProxyConfiguration().equals(this.getProxyConfiguration()) == false)
return false;
if (other.getRegisteredAt() == null ^ this.getRegisteredAt() == null)
return false;
if (other.getRegisteredAt() != null && other.getRegisteredAt().equals(this.getRegisteredAt()) == false)
return false;
if (other.getDeregisteredAt() == null ^ this.getDeregisteredAt() == null)
return false;
if (other.getDeregisteredAt() != null && other.getDeregisteredAt().equals(this.getDeregisteredAt()) == false)
return false;
if (other.getRegisteredBy() == null ^ this.getRegisteredBy() == null)
return false;
if (other.getRegisteredBy() != null && other.getRegisteredBy().equals(this.getRegisteredBy()) == false)
return false;
if (other.getEphemeralStorage() == null ^ this.getEphemeralStorage() == null)
return false;
if (other.getEphemeralStorage() != null && other.getEphemeralStorage().equals(this.getEphemeralStorage()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getTaskDefinitionArn() == null) ? 0 : getTaskDefinitionArn().hashCode());
hashCode = prime * hashCode + ((getContainerDefinitions() == null) ? 0 : getContainerDefinitions().hashCode());
hashCode = prime * hashCode + ((getFamily() == null) ? 0 : getFamily().hashCode());
hashCode = prime * hashCode + ((getTaskRoleArn() == null) ? 0 : getTaskRoleArn().hashCode());
hashCode = prime * hashCode + ((getExecutionRoleArn() == null) ? 0 : getExecutionRoleArn().hashCode());
hashCode = prime * hashCode + ((getNetworkMode() == null) ? 0 : getNetworkMode().hashCode());
hashCode = prime * hashCode + ((getRevision() == null) ? 0 : getRevision().hashCode());
hashCode = prime * hashCode + ((getVolumes() == null) ? 0 : getVolumes().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getRequiresAttributes() == null) ? 0 : getRequiresAttributes().hashCode());
hashCode = prime * hashCode + ((getPlacementConstraints() == null) ? 0 : getPlacementConstraints().hashCode());
hashCode = prime * hashCode + ((getCompatibilities() == null) ? 0 : getCompatibilities().hashCode());
hashCode = prime * hashCode + ((getRuntimePlatform() == null) ? 0 : getRuntimePlatform().hashCode());
hashCode = prime * hashCode + ((getRequiresCompatibilities() == null) ? 0 : getRequiresCompatibilities().hashCode());
hashCode = prime * hashCode + ((getCpu() == null) ? 0 : getCpu().hashCode());
hashCode = prime * hashCode + ((getMemory() == null) ? 0 : getMemory().hashCode());
hashCode = prime * hashCode + ((getInferenceAccelerators() == null) ? 0 : getInferenceAccelerators().hashCode());
hashCode = prime * hashCode + ((getPidMode() == null) ? 0 : getPidMode().hashCode());
hashCode = prime * hashCode + ((getIpcMode() == null) ? 0 : getIpcMode().hashCode());
hashCode = prime * hashCode + ((getProxyConfiguration() == null) ? 0 : getProxyConfiguration().hashCode());
hashCode = prime * hashCode + ((getRegisteredAt() == null) ? 0 : getRegisteredAt().hashCode());
hashCode = prime * hashCode + ((getDeregisteredAt() == null) ? 0 : getDeregisteredAt().hashCode());
hashCode = prime * hashCode + ((getRegisteredBy() == null) ? 0 : getRegisteredBy().hashCode());
hashCode = prime * hashCode + ((getEphemeralStorage() == null) ? 0 : getEphemeralStorage().hashCode());
return hashCode;
}
@Override
public TaskDefinition clone() {
try {
return (TaskDefinition) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.ecs.model.transform.TaskDefinitionMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}