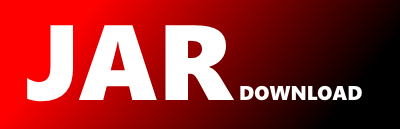
com.amazonaws.services.ecs.model.Volume Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ecs Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ecs.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The data volume configuration for tasks launched using this task definition. Specifying a volume configuration in a
* task definition is optional. The volume configuration may contain multiple volumes but only one volume configured at
* launch is supported. Each volume defined in the volume configuration may only specify a name
and one of
* either configuredAtLaunch
, dockerVolumeConfiguration
, efsVolumeConfiguration
,
* fsxWindowsFileServerVolumeConfiguration
, or host
. If an empty volume configuration is
* specified, by default Amazon ECS uses a host volume. For more information, see Using data volumes in
* tasks.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Volume implements Serializable, Cloneable, StructuredPojo {
/**
*
* The name of the volume. Up to 255 letters (uppercase and lowercase), numbers, underscores, and hyphens are
* allowed.
*
*
* When using a volume configured at launch, the name
is required and must also be specified as the
* volume name in the ServiceVolumeConfiguration
or TaskVolumeConfiguration
parameter when
* creating your service or standalone task.
*
*
* For all other types of volumes, this name is referenced in the sourceVolume
parameter of the
* mountPoints
object in the container definition.
*
*
* When a volume is using the efsVolumeConfiguration
, the name is required.
*
*/
private String name;
/**
*
* This parameter is specified when you use bind mount host volumes. The contents of the host
parameter
* determine whether your bind mount host volume persists on the host container instance and where it's stored. If
* the host
parameter is empty, then the Docker daemon assigns a host path for your data volume.
* However, the data isn't guaranteed to persist after the containers that are associated with it stop running.
*
*
* Windows containers can mount whole directories on the same drive as $env:ProgramData
. Windows
* containers can't mount directories on a different drive, and mount point can't be across drives. For example, you
* can mount C:\my\path:C:\my\path
and D:\:D:\
, but not D:\my\path:C:\my\path
* or D:\:C:\my\path
.
*
*/
private HostVolumeProperties host;
/**
*
* This parameter is specified when you use Docker volumes.
*
*
* Windows containers only support the use of the local
driver. To use bind mounts, specify the
* host
parameter instead.
*
*
*
* Docker volumes aren't supported by tasks run on Fargate.
*
*
*/
private DockerVolumeConfiguration dockerVolumeConfiguration;
/**
*
* This parameter is specified when you use an Amazon Elastic File System file system for task storage.
*
*/
private EFSVolumeConfiguration efsVolumeConfiguration;
/**
*
* This parameter is specified when you use Amazon FSx for Windows File Server file system for task storage.
*
*/
private FSxWindowsFileServerVolumeConfiguration fsxWindowsFileServerVolumeConfiguration;
/**
*
* Indicates whether the volume should be configured at launch time. This is used to create Amazon EBS volumes for
* standalone tasks or tasks created as part of a service. Each task definition revision may only have one volume
* configured at launch in the volume configuration.
*
*
* To configure a volume at launch time, use this task definition revision and specify a
* volumeConfigurations
object when calling the CreateService
, UpdateService
,
* RunTask
or StartTask
APIs.
*
*/
private Boolean configuredAtLaunch;
/**
*
* The name of the volume. Up to 255 letters (uppercase and lowercase), numbers, underscores, and hyphens are
* allowed.
*
*
* When using a volume configured at launch, the name
is required and must also be specified as the
* volume name in the ServiceVolumeConfiguration
or TaskVolumeConfiguration
parameter when
* creating your service or standalone task.
*
*
* For all other types of volumes, this name is referenced in the sourceVolume
parameter of the
* mountPoints
object in the container definition.
*
*
* When a volume is using the efsVolumeConfiguration
, the name is required.
*
*
* @param name
* The name of the volume. Up to 255 letters (uppercase and lowercase), numbers, underscores, and hyphens are
* allowed.
*
* When using a volume configured at launch, the name
is required and must also be specified as
* the volume name in the ServiceVolumeConfiguration
or TaskVolumeConfiguration
* parameter when creating your service or standalone task.
*
*
* For all other types of volumes, this name is referenced in the sourceVolume
parameter of the
* mountPoints
object in the container definition.
*
*
* When a volume is using the efsVolumeConfiguration
, the name is required.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the volume. Up to 255 letters (uppercase and lowercase), numbers, underscores, and hyphens are
* allowed.
*
*
* When using a volume configured at launch, the name
is required and must also be specified as the
* volume name in the ServiceVolumeConfiguration
or TaskVolumeConfiguration
parameter when
* creating your service or standalone task.
*
*
* For all other types of volumes, this name is referenced in the sourceVolume
parameter of the
* mountPoints
object in the container definition.
*
*
* When a volume is using the efsVolumeConfiguration
, the name is required.
*
*
* @return The name of the volume. Up to 255 letters (uppercase and lowercase), numbers, underscores, and hyphens
* are allowed.
*
* When using a volume configured at launch, the name
is required and must also be specified as
* the volume name in the ServiceVolumeConfiguration
or TaskVolumeConfiguration
* parameter when creating your service or standalone task.
*
*
* For all other types of volumes, this name is referenced in the sourceVolume
parameter of the
* mountPoints
object in the container definition.
*
*
* When a volume is using the efsVolumeConfiguration
, the name is required.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the volume. Up to 255 letters (uppercase and lowercase), numbers, underscores, and hyphens are
* allowed.
*
*
* When using a volume configured at launch, the name
is required and must also be specified as the
* volume name in the ServiceVolumeConfiguration
or TaskVolumeConfiguration
parameter when
* creating your service or standalone task.
*
*
* For all other types of volumes, this name is referenced in the sourceVolume
parameter of the
* mountPoints
object in the container definition.
*
*
* When a volume is using the efsVolumeConfiguration
, the name is required.
*
*
* @param name
* The name of the volume. Up to 255 letters (uppercase and lowercase), numbers, underscores, and hyphens are
* allowed.
*
* When using a volume configured at launch, the name
is required and must also be specified as
* the volume name in the ServiceVolumeConfiguration
or TaskVolumeConfiguration
* parameter when creating your service or standalone task.
*
*
* For all other types of volumes, this name is referenced in the sourceVolume
parameter of the
* mountPoints
object in the container definition.
*
*
* When a volume is using the efsVolumeConfiguration
, the name is required.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Volume withName(String name) {
setName(name);
return this;
}
/**
*
* This parameter is specified when you use bind mount host volumes. The contents of the host
parameter
* determine whether your bind mount host volume persists on the host container instance and where it's stored. If
* the host
parameter is empty, then the Docker daemon assigns a host path for your data volume.
* However, the data isn't guaranteed to persist after the containers that are associated with it stop running.
*
*
* Windows containers can mount whole directories on the same drive as $env:ProgramData
. Windows
* containers can't mount directories on a different drive, and mount point can't be across drives. For example, you
* can mount C:\my\path:C:\my\path
and D:\:D:\
, but not D:\my\path:C:\my\path
* or D:\:C:\my\path
.
*
*
* @param host
* This parameter is specified when you use bind mount host volumes. The contents of the host
* parameter determine whether your bind mount host volume persists on the host container instance and where
* it's stored. If the host
parameter is empty, then the Docker daemon assigns a host path for
* your data volume. However, the data isn't guaranteed to persist after the containers that are associated
* with it stop running.
*
* Windows containers can mount whole directories on the same drive as $env:ProgramData
. Windows
* containers can't mount directories on a different drive, and mount point can't be across drives. For
* example, you can mount C:\my\path:C:\my\path
and D:\:D:\
, but not
* D:\my\path:C:\my\path
or D:\:C:\my\path
.
*/
public void setHost(HostVolumeProperties host) {
this.host = host;
}
/**
*
* This parameter is specified when you use bind mount host volumes. The contents of the host
parameter
* determine whether your bind mount host volume persists on the host container instance and where it's stored. If
* the host
parameter is empty, then the Docker daemon assigns a host path for your data volume.
* However, the data isn't guaranteed to persist after the containers that are associated with it stop running.
*
*
* Windows containers can mount whole directories on the same drive as $env:ProgramData
. Windows
* containers can't mount directories on a different drive, and mount point can't be across drives. For example, you
* can mount C:\my\path:C:\my\path
and D:\:D:\
, but not D:\my\path:C:\my\path
* or D:\:C:\my\path
.
*
*
* @return This parameter is specified when you use bind mount host volumes. The contents of the host
* parameter determine whether your bind mount host volume persists on the host container instance and where
* it's stored. If the host
parameter is empty, then the Docker daemon assigns a host path for
* your data volume. However, the data isn't guaranteed to persist after the containers that are associated
* with it stop running.
*
* Windows containers can mount whole directories on the same drive as $env:ProgramData
.
* Windows containers can't mount directories on a different drive, and mount point can't be across drives.
* For example, you can mount C:\my\path:C:\my\path
and D:\:D:\
, but not
* D:\my\path:C:\my\path
or D:\:C:\my\path
.
*/
public HostVolumeProperties getHost() {
return this.host;
}
/**
*
* This parameter is specified when you use bind mount host volumes. The contents of the host
parameter
* determine whether your bind mount host volume persists on the host container instance and where it's stored. If
* the host
parameter is empty, then the Docker daemon assigns a host path for your data volume.
* However, the data isn't guaranteed to persist after the containers that are associated with it stop running.
*
*
* Windows containers can mount whole directories on the same drive as $env:ProgramData
. Windows
* containers can't mount directories on a different drive, and mount point can't be across drives. For example, you
* can mount C:\my\path:C:\my\path
and D:\:D:\
, but not D:\my\path:C:\my\path
* or D:\:C:\my\path
.
*
*
* @param host
* This parameter is specified when you use bind mount host volumes. The contents of the host
* parameter determine whether your bind mount host volume persists on the host container instance and where
* it's stored. If the host
parameter is empty, then the Docker daemon assigns a host path for
* your data volume. However, the data isn't guaranteed to persist after the containers that are associated
* with it stop running.
*
* Windows containers can mount whole directories on the same drive as $env:ProgramData
. Windows
* containers can't mount directories on a different drive, and mount point can't be across drives. For
* example, you can mount C:\my\path:C:\my\path
and D:\:D:\
, but not
* D:\my\path:C:\my\path
or D:\:C:\my\path
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Volume withHost(HostVolumeProperties host) {
setHost(host);
return this;
}
/**
*
* This parameter is specified when you use Docker volumes.
*
*
* Windows containers only support the use of the local
driver. To use bind mounts, specify the
* host
parameter instead.
*
*
*
* Docker volumes aren't supported by tasks run on Fargate.
*
*
*
* @param dockerVolumeConfiguration
* This parameter is specified when you use Docker volumes.
*
* Windows containers only support the use of the local
driver. To use bind mounts, specify the
* host
parameter instead.
*
*
*
* Docker volumes aren't supported by tasks run on Fargate.
*
*/
public void setDockerVolumeConfiguration(DockerVolumeConfiguration dockerVolumeConfiguration) {
this.dockerVolumeConfiguration = dockerVolumeConfiguration;
}
/**
*
* This parameter is specified when you use Docker volumes.
*
*
* Windows containers only support the use of the local
driver. To use bind mounts, specify the
* host
parameter instead.
*
*
*
* Docker volumes aren't supported by tasks run on Fargate.
*
*
*
* @return This parameter is specified when you use Docker volumes.
*
* Windows containers only support the use of the local
driver. To use bind mounts, specify the
* host
parameter instead.
*
*
*
* Docker volumes aren't supported by tasks run on Fargate.
*
*/
public DockerVolumeConfiguration getDockerVolumeConfiguration() {
return this.dockerVolumeConfiguration;
}
/**
*
* This parameter is specified when you use Docker volumes.
*
*
* Windows containers only support the use of the local
driver. To use bind mounts, specify the
* host
parameter instead.
*
*
*
* Docker volumes aren't supported by tasks run on Fargate.
*
*
*
* @param dockerVolumeConfiguration
* This parameter is specified when you use Docker volumes.
*
* Windows containers only support the use of the local
driver. To use bind mounts, specify the
* host
parameter instead.
*
*
*
* Docker volumes aren't supported by tasks run on Fargate.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Volume withDockerVolumeConfiguration(DockerVolumeConfiguration dockerVolumeConfiguration) {
setDockerVolumeConfiguration(dockerVolumeConfiguration);
return this;
}
/**
*
* This parameter is specified when you use an Amazon Elastic File System file system for task storage.
*
*
* @param efsVolumeConfiguration
* This parameter is specified when you use an Amazon Elastic File System file system for task storage.
*/
public void setEfsVolumeConfiguration(EFSVolumeConfiguration efsVolumeConfiguration) {
this.efsVolumeConfiguration = efsVolumeConfiguration;
}
/**
*
* This parameter is specified when you use an Amazon Elastic File System file system for task storage.
*
*
* @return This parameter is specified when you use an Amazon Elastic File System file system for task storage.
*/
public EFSVolumeConfiguration getEfsVolumeConfiguration() {
return this.efsVolumeConfiguration;
}
/**
*
* This parameter is specified when you use an Amazon Elastic File System file system for task storage.
*
*
* @param efsVolumeConfiguration
* This parameter is specified when you use an Amazon Elastic File System file system for task storage.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Volume withEfsVolumeConfiguration(EFSVolumeConfiguration efsVolumeConfiguration) {
setEfsVolumeConfiguration(efsVolumeConfiguration);
return this;
}
/**
*
* This parameter is specified when you use Amazon FSx for Windows File Server file system for task storage.
*
*
* @param fsxWindowsFileServerVolumeConfiguration
* This parameter is specified when you use Amazon FSx for Windows File Server file system for task storage.
*/
public void setFsxWindowsFileServerVolumeConfiguration(FSxWindowsFileServerVolumeConfiguration fsxWindowsFileServerVolumeConfiguration) {
this.fsxWindowsFileServerVolumeConfiguration = fsxWindowsFileServerVolumeConfiguration;
}
/**
*
* This parameter is specified when you use Amazon FSx for Windows File Server file system for task storage.
*
*
* @return This parameter is specified when you use Amazon FSx for Windows File Server file system for task storage.
*/
public FSxWindowsFileServerVolumeConfiguration getFsxWindowsFileServerVolumeConfiguration() {
return this.fsxWindowsFileServerVolumeConfiguration;
}
/**
*
* This parameter is specified when you use Amazon FSx for Windows File Server file system for task storage.
*
*
* @param fsxWindowsFileServerVolumeConfiguration
* This parameter is specified when you use Amazon FSx for Windows File Server file system for task storage.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Volume withFsxWindowsFileServerVolumeConfiguration(FSxWindowsFileServerVolumeConfiguration fsxWindowsFileServerVolumeConfiguration) {
setFsxWindowsFileServerVolumeConfiguration(fsxWindowsFileServerVolumeConfiguration);
return this;
}
/**
*
* Indicates whether the volume should be configured at launch time. This is used to create Amazon EBS volumes for
* standalone tasks or tasks created as part of a service. Each task definition revision may only have one volume
* configured at launch in the volume configuration.
*
*
* To configure a volume at launch time, use this task definition revision and specify a
* volumeConfigurations
object when calling the CreateService
, UpdateService
,
* RunTask
or StartTask
APIs.
*
*
* @param configuredAtLaunch
* Indicates whether the volume should be configured at launch time. This is used to create Amazon EBS
* volumes for standalone tasks or tasks created as part of a service. Each task definition revision may only
* have one volume configured at launch in the volume configuration.
*
* To configure a volume at launch time, use this task definition revision and specify a
* volumeConfigurations
object when calling the CreateService
,
* UpdateService
, RunTask
or StartTask
APIs.
*/
public void setConfiguredAtLaunch(Boolean configuredAtLaunch) {
this.configuredAtLaunch = configuredAtLaunch;
}
/**
*
* Indicates whether the volume should be configured at launch time. This is used to create Amazon EBS volumes for
* standalone tasks or tasks created as part of a service. Each task definition revision may only have one volume
* configured at launch in the volume configuration.
*
*
* To configure a volume at launch time, use this task definition revision and specify a
* volumeConfigurations
object when calling the CreateService
, UpdateService
,
* RunTask
or StartTask
APIs.
*
*
* @return Indicates whether the volume should be configured at launch time. This is used to create Amazon EBS
* volumes for standalone tasks or tasks created as part of a service. Each task definition revision may
* only have one volume configured at launch in the volume configuration.
*
* To configure a volume at launch time, use this task definition revision and specify a
* volumeConfigurations
object when calling the CreateService
,
* UpdateService
, RunTask
or StartTask
APIs.
*/
public Boolean getConfiguredAtLaunch() {
return this.configuredAtLaunch;
}
/**
*
* Indicates whether the volume should be configured at launch time. This is used to create Amazon EBS volumes for
* standalone tasks or tasks created as part of a service. Each task definition revision may only have one volume
* configured at launch in the volume configuration.
*
*
* To configure a volume at launch time, use this task definition revision and specify a
* volumeConfigurations
object when calling the CreateService
, UpdateService
,
* RunTask
or StartTask
APIs.
*
*
* @param configuredAtLaunch
* Indicates whether the volume should be configured at launch time. This is used to create Amazon EBS
* volumes for standalone tasks or tasks created as part of a service. Each task definition revision may only
* have one volume configured at launch in the volume configuration.
*
* To configure a volume at launch time, use this task definition revision and specify a
* volumeConfigurations
object when calling the CreateService
,
* UpdateService
, RunTask
or StartTask
APIs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Volume withConfiguredAtLaunch(Boolean configuredAtLaunch) {
setConfiguredAtLaunch(configuredAtLaunch);
return this;
}
/**
*
* Indicates whether the volume should be configured at launch time. This is used to create Amazon EBS volumes for
* standalone tasks or tasks created as part of a service. Each task definition revision may only have one volume
* configured at launch in the volume configuration.
*
*
* To configure a volume at launch time, use this task definition revision and specify a
* volumeConfigurations
object when calling the CreateService
, UpdateService
,
* RunTask
or StartTask
APIs.
*
*
* @return Indicates whether the volume should be configured at launch time. This is used to create Amazon EBS
* volumes for standalone tasks or tasks created as part of a service. Each task definition revision may
* only have one volume configured at launch in the volume configuration.
*
* To configure a volume at launch time, use this task definition revision and specify a
* volumeConfigurations
object when calling the CreateService
,
* UpdateService
, RunTask
or StartTask
APIs.
*/
public Boolean isConfiguredAtLaunch() {
return this.configuredAtLaunch;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getHost() != null)
sb.append("Host: ").append(getHost()).append(",");
if (getDockerVolumeConfiguration() != null)
sb.append("DockerVolumeConfiguration: ").append(getDockerVolumeConfiguration()).append(",");
if (getEfsVolumeConfiguration() != null)
sb.append("EfsVolumeConfiguration: ").append(getEfsVolumeConfiguration()).append(",");
if (getFsxWindowsFileServerVolumeConfiguration() != null)
sb.append("FsxWindowsFileServerVolumeConfiguration: ").append(getFsxWindowsFileServerVolumeConfiguration()).append(",");
if (getConfiguredAtLaunch() != null)
sb.append("ConfiguredAtLaunch: ").append(getConfiguredAtLaunch());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Volume == false)
return false;
Volume other = (Volume) obj;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getHost() == null ^ this.getHost() == null)
return false;
if (other.getHost() != null && other.getHost().equals(this.getHost()) == false)
return false;
if (other.getDockerVolumeConfiguration() == null ^ this.getDockerVolumeConfiguration() == null)
return false;
if (other.getDockerVolumeConfiguration() != null && other.getDockerVolumeConfiguration().equals(this.getDockerVolumeConfiguration()) == false)
return false;
if (other.getEfsVolumeConfiguration() == null ^ this.getEfsVolumeConfiguration() == null)
return false;
if (other.getEfsVolumeConfiguration() != null && other.getEfsVolumeConfiguration().equals(this.getEfsVolumeConfiguration()) == false)
return false;
if (other.getFsxWindowsFileServerVolumeConfiguration() == null ^ this.getFsxWindowsFileServerVolumeConfiguration() == null)
return false;
if (other.getFsxWindowsFileServerVolumeConfiguration() != null
&& other.getFsxWindowsFileServerVolumeConfiguration().equals(this.getFsxWindowsFileServerVolumeConfiguration()) == false)
return false;
if (other.getConfiguredAtLaunch() == null ^ this.getConfiguredAtLaunch() == null)
return false;
if (other.getConfiguredAtLaunch() != null && other.getConfiguredAtLaunch().equals(this.getConfiguredAtLaunch()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getHost() == null) ? 0 : getHost().hashCode());
hashCode = prime * hashCode + ((getDockerVolumeConfiguration() == null) ? 0 : getDockerVolumeConfiguration().hashCode());
hashCode = prime * hashCode + ((getEfsVolumeConfiguration() == null) ? 0 : getEfsVolumeConfiguration().hashCode());
hashCode = prime * hashCode + ((getFsxWindowsFileServerVolumeConfiguration() == null) ? 0 : getFsxWindowsFileServerVolumeConfiguration().hashCode());
hashCode = prime * hashCode + ((getConfiguredAtLaunch() == null) ? 0 : getConfiguredAtLaunch().hashCode());
return hashCode;
}
@Override
public Volume clone() {
try {
return (Volume) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.ecs.model.transform.VolumeMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}