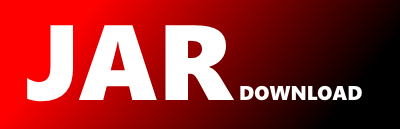
com.amazonaws.services.ecs.AmazonECS Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ecs;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.ecs.model.*;
import com.amazonaws.services.ecs.waiters.AmazonECSWaiters;
/**
* Interface for accessing Amazon ECS.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.ecs.AbstractAmazonECS} instead.
*
*
* Amazon Elastic Container Service
*
* Amazon Elastic Container Service (Amazon ECS) is a highly scalable, fast, container management service. It makes it
* easy to run, stop, and manage Docker containers. You can host your cluster on a serverless infrastructure that's
* managed by Amazon ECS by launching your services or tasks on Fargate. For more control, you can host your tasks on a
* cluster of Amazon Elastic Compute Cloud (Amazon EC2) or External (on-premises) instances that you manage.
*
*
* Amazon ECS makes it easy to launch and stop container-based applications with simple API calls. This makes it easy to
* get the state of your cluster from a centralized service, and gives you access to many familiar Amazon EC2 features.
*
*
* You can use Amazon ECS to schedule the placement of containers across your cluster based on your resource needs,
* isolation policies, and availability requirements. With Amazon ECS, you don't need to operate your own cluster
* management and configuration management systems. You also don't need to worry about scaling your management
* infrastructure.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonECS {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "ecs";
/**
* Overrides the default endpoint for this client ("https://ecs.us-east-1.amazonaws.com"). Callers can use this
* method to control which AWS region they want to work with.
*
* Callers can pass in just the endpoint (ex: "ecs.us-east-1.amazonaws.com") or a full URL, including the protocol
* (ex: "https://ecs.us-east-1.amazonaws.com"). If the protocol is not specified here, the default protocol from
* this client's {@link ClientConfiguration} will be used, which by default is HTTPS.
*
* For more information on using AWS regions with the AWS SDK for Java, and a complete list of all available
* endpoints for all AWS services, see: https://docs.aws.amazon.com/sdk-for-java/v1/developer-guide/java-dg-region-selection.html#region-selection-
* choose-endpoint
*
* This method is not threadsafe. An endpoint should be configured when the client is created and before any
* service requests are made. Changing it afterwards creates inevitable race conditions for any service requests in
* transit or retrying.
*
* @param endpoint
* The endpoint (ex: "ecs.us-east-1.amazonaws.com") or a full URL, including the protocol (ex:
* "https://ecs.us-east-1.amazonaws.com") of the region specific AWS endpoint this client will communicate
* with.
* @deprecated use {@link AwsClientBuilder#setEndpointConfiguration(AwsClientBuilder.EndpointConfiguration)} for
* example:
* {@code builder.setEndpointConfiguration(new EndpointConfiguration(endpoint, signingRegion));}
*/
@Deprecated
void setEndpoint(String endpoint);
/**
* An alternative to {@link AmazonECS#setEndpoint(String)}, sets the regional endpoint for this client's service
* calls. Callers can use this method to control which AWS region they want to work with.
*
* By default, all service endpoints in all regions use the https protocol. To use http instead, specify it in the
* {@link ClientConfiguration} supplied at construction.
*
* This method is not threadsafe. A region should be configured when the client is created and before any service
* requests are made. Changing it afterwards creates inevitable race conditions for any service requests in transit
* or retrying.
*
* @param region
* The region this client will communicate with. See {@link Region#getRegion(com.amazonaws.regions.Regions)}
* for accessing a given region. Must not be null and must be a region where the service is available.
*
* @see Region#getRegion(com.amazonaws.regions.Regions)
* @see Region#createClient(Class, com.amazonaws.auth.AWSCredentialsProvider, ClientConfiguration)
* @see Region#isServiceSupported(String)
* @deprecated use {@link AwsClientBuilder#setRegion(String)}
*/
@Deprecated
void setRegion(Region region);
/**
*
* Creates a new capacity provider. Capacity providers are associated with an Amazon ECS cluster and are used in
* capacity provider strategies to facilitate cluster auto scaling.
*
*
* Only capacity providers that use an Auto Scaling group can be created. Amazon ECS tasks on Fargate use the
* FARGATE
and FARGATE_SPOT
capacity providers. These providers are available to all
* accounts in the Amazon Web Services Regions that Fargate supports.
*
*
* @param createCapacityProviderRequest
* @return Result of the CreateCapacityProvider operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @throws LimitExceededException
* The limit for the resource was exceeded.
* @throws UpdateInProgressException
* There's already a current Amazon ECS container agent update in progress on the container instance that's
* specified. If the container agent becomes disconnected while it's in a transitional stage, such as
* PENDING
or STAGING
, the update process can get stuck in that state. However,
* when the agent reconnects, it resumes where it stopped previously.
* @sample AmazonECS.CreateCapacityProvider
* @see AWS API
* Documentation
*/
CreateCapacityProviderResult createCapacityProvider(CreateCapacityProviderRequest createCapacityProviderRequest);
/**
*
* Creates a new Amazon ECS cluster. By default, your account receives a default
cluster when you
* launch your first container instance. However, you can create your own cluster with a unique name with the
* CreateCluster
action.
*
*
*
* When you call the CreateCluster API operation, Amazon ECS attempts to create the Amazon ECS service-linked
* role for your account. This is so that it can manage required resources in other Amazon Web Services services on
* your behalf. However, if the user that makes the call doesn't have permissions to create the service-linked role,
* it isn't created. For more information, see Using
* service-linked roles for Amazon ECS in the Amazon Elastic Container Service Developer Guide.
*
*
*
* @param createClusterRequest
* @return Result of the CreateCluster operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @throws NamespaceNotFoundException
* The specified namespace wasn't found.
* @sample AmazonECS.CreateCluster
* @see AWS API
* Documentation
*/
CreateClusterResult createCluster(CreateClusterRequest createClusterRequest);
/**
* Simplified method form for invoking the CreateCluster operation.
*
* @see #createCluster(CreateClusterRequest)
*/
CreateClusterResult createCluster();
/**
*
* Runs and maintains your desired number of tasks from a specified task definition. If the number of tasks running
* in a service drops below the desiredCount
, Amazon ECS runs another copy of the task in the specified
* cluster. To update an existing service, see the UpdateService action.
*
*
*
* On March 21, 2024, a change was made to resolve the task definition revision before authorization. When a task
* definition revision is not specified, authorization will occur using the latest revision of a task definition.
*
*
*
* In addition to maintaining the desired count of tasks in your service, you can optionally run your service behind
* one or more load balancers. The load balancers distribute traffic across the tasks that are associated with the
* service. For more information, see Service load
* balancing in the Amazon Elastic Container Service Developer Guide.
*
*
* You can attach Amazon EBS volumes to Amazon ECS tasks by configuring the volume when creating or updating a
* service. volumeConfigurations
is only supported for REPLICA service and not DAEMON service. For more
* infomation, see Amazon EBS
* volumes in the Amazon Elastic Container Service Developer Guide.
*
*
* Tasks for services that don't use a load balancer are considered healthy if they're in the RUNNING
* state. Tasks for services that use a load balancer are considered healthy if they're in the RUNNING
* state and are reported as healthy by the load balancer.
*
*
* There are two service scheduler strategies available:
*
*
* -
*
* REPLICA
- The replica scheduling strategy places and maintains your desired number of tasks across
* your cluster. By default, the service scheduler spreads tasks across Availability Zones. You can use task
* placement strategies and constraints to customize task placement decisions. For more information, see Service scheduler
* concepts in the Amazon Elastic Container Service Developer Guide.
*
*
* -
*
* DAEMON
- The daemon scheduling strategy deploys exactly one task on each active container instance
* that meets all of the task placement constraints that you specify in your cluster. The service scheduler also
* evaluates the task placement constraints for running tasks. It also stops tasks that don't meet the placement
* constraints. When using this strategy, you don't need to specify a desired number of tasks, a task placement
* strategy, or use Service Auto Scaling policies. For more information, see Service scheduler
* concepts in the Amazon Elastic Container Service Developer Guide.
*
*
*
*
* You can optionally specify a deployment configuration for your service. The deployment is initiated by changing
* properties. For example, the deployment might be initiated by the task definition or by your desired count of a
* service. This is done with an UpdateService operation. The default value for a replica service for
* minimumHealthyPercent
is 100%. The default value for a daemon service for
* minimumHealthyPercent
is 0%.
*
*
* If a service uses the ECS
deployment controller, the minimum healthy percent represents a lower
* limit on the number of tasks in a service that must remain in the RUNNING
state during a deployment.
* Specifically, it represents it as a percentage of your desired number of tasks (rounded up to the nearest
* integer). This happens when any of your container instances are in the DRAINING
state if the service
* contains tasks using the EC2 launch type. Using this parameter, you can deploy without using additional cluster
* capacity. For example, if you set your service to have desired number of four tasks and a minimum healthy percent
* of 50%, the scheduler might stop two existing tasks to free up cluster capacity before starting two new tasks. If
* they're in the RUNNING
state, tasks for services that don't use a load balancer are considered
* healthy . If they're in the RUNNING
state and reported as healthy by the load balancer, tasks for
* services that do use a load balancer are considered healthy . The default value for minimum healthy
* percent is 100%.
*
*
* If a service uses the ECS
deployment controller, the maximum percent parameter represents an
* upper limit on the number of tasks in a service that are allowed in the RUNNING
or
* PENDING
state during a deployment. Specifically, it represents it as a percentage of the desired
* number of tasks (rounded down to the nearest integer). This happens when any of your container instances are in
* the DRAINING
state if the service contains tasks using the EC2 launch type. Using this parameter,
* you can define the deployment batch size. For example, if your service has a desired number of four tasks and a
* maximum percent value of 200%, the scheduler may start four new tasks before stopping the four older tasks
* (provided that the cluster resources required to do this are available). The default value for maximum percent is
* 200%.
*
*
* If a service uses either the CODE_DEPLOY
or EXTERNAL
deployment controller types and
* tasks that use the EC2 launch type, the minimum healthy percent and maximum percent values are used
* only to define the lower and upper limit on the number of the tasks in the service that remain in the
* RUNNING
state. This is while the container instances are in the DRAINING
state. If the
* tasks in the service use the Fargate launch type, the minimum healthy percent and maximum percent values aren't
* used. This is the case even if they're currently visible when describing your service.
*
*
* When creating a service that uses the EXTERNAL
deployment controller, you can specify only
* parameters that aren't controlled at the task set level. The only required parameter is the service name. You
* control your services using the CreateTaskSet operation. For more information, see Amazon ECS deployment
* types in the Amazon Elastic Container Service Developer Guide.
*
*
* When the service scheduler launches new tasks, it determines task placement. For information about task placement
* and task placement strategies, see Amazon ECS task
* placement in the Amazon Elastic Container Service Developer Guide
*
*
* Starting April 15, 2023, Amazon Web Services will not onboard new customers to Amazon Elastic Inference (EI), and
* will help current customers migrate their workloads to options that offer better price and performance. After
* April 15, 2023, new customers will not be able to launch instances with Amazon EI accelerators in Amazon
* SageMaker, Amazon ECS, or Amazon EC2. However, customers who have used Amazon EI at least once during the past
* 30-day period are considered current customers and will be able to continue using the service.
*
*
* @param createServiceRequest
* @return Result of the CreateService operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster wasn't found. You can view your available clusters with ListClusters. Amazon
* ECS clusters are Region specific.
* @throws UnsupportedFeatureException
* The specified task isn't supported in this Region.
* @throws PlatformUnknownException
* The specified platform version doesn't exist.
* @throws PlatformTaskDefinitionIncompatibilityException
* The specified platform version doesn't satisfy the required capabilities of the task definition.
* @throws AccessDeniedException
* You don't have authorization to perform the requested action.
* @throws NamespaceNotFoundException
* The specified namespace wasn't found.
* @sample AmazonECS.CreateService
* @see AWS API
* Documentation
*/
CreateServiceResult createService(CreateServiceRequest createServiceRequest);
/**
*
* Create a task set in the specified cluster and service. This is used when a service uses the
* EXTERNAL
deployment controller type. For more information, see Amazon ECS deployment
* types in the Amazon Elastic Container Service Developer Guide.
*
*
*
* On March 21, 2024, a change was made to resolve the task definition revision before authorization. When a task
* definition revision is not specified, authorization will occur using the latest revision of a task definition.
*
*
*
* For information about the maximum number of task sets and otther quotas, see Amazon ECS service
* quotas in the Amazon Elastic Container Service Developer Guide.
*
*
* @param createTaskSetRequest
* @return Result of the CreateTaskSet operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster wasn't found. You can view your available clusters with ListClusters. Amazon
* ECS clusters are Region specific.
* @throws UnsupportedFeatureException
* The specified task isn't supported in this Region.
* @throws PlatformUnknownException
* The specified platform version doesn't exist.
* @throws PlatformTaskDefinitionIncompatibilityException
* The specified platform version doesn't satisfy the required capabilities of the task definition.
* @throws AccessDeniedException
* You don't have authorization to perform the requested action.
* @throws ServiceNotFoundException
* The specified service wasn't found. You can view your available services with ListServices. Amazon
* ECS services are cluster specific and Region specific.
* @throws ServiceNotActiveException
* The specified service isn't active. You can't update a service that's inactive. If you have previously
* deleted a service, you can re-create it with CreateService.
* @throws NamespaceNotFoundException
* The specified namespace wasn't found.
* @sample AmazonECS.CreateTaskSet
* @see AWS API
* Documentation
*/
CreateTaskSetResult createTaskSet(CreateTaskSetRequest createTaskSetRequest);
/**
*
* Disables an account setting for a specified user, role, or the root user for an account.
*
*
* @param deleteAccountSettingRequest
* @return Result of the DeleteAccountSetting operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @sample AmazonECS.DeleteAccountSetting
* @see AWS API
* Documentation
*/
DeleteAccountSettingResult deleteAccountSetting(DeleteAccountSettingRequest deleteAccountSettingRequest);
/**
*
* Deletes one or more custom attributes from an Amazon ECS resource.
*
*
* @param deleteAttributesRequest
* @return Result of the DeleteAttributes operation returned by the service.
* @throws ClusterNotFoundException
* The specified cluster wasn't found. You can view your available clusters with ListClusters. Amazon
* ECS clusters are Region specific.
* @throws TargetNotFoundException
* The specified target wasn't found. You can view your available container instances with
* ListContainerInstances. Amazon ECS container instances are cluster-specific and Region-specific.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @sample AmazonECS.DeleteAttributes
* @see AWS API
* Documentation
*/
DeleteAttributesResult deleteAttributes(DeleteAttributesRequest deleteAttributesRequest);
/**
*
* Deletes the specified capacity provider.
*
*
*
* The FARGATE
and FARGATE_SPOT
capacity providers are reserved and can't be deleted. You
* can disassociate them from a cluster using either the PutClusterCapacityProviders API or by deleting the
* cluster.
*
*
*
* Prior to a capacity provider being deleted, the capacity provider must be removed from the capacity provider
* strategy from all services. The UpdateService API can be used to remove a capacity provider from a
* service's capacity provider strategy. When updating a service, the forceNewDeployment
option can be
* used to ensure that any tasks using the Amazon EC2 instance capacity provided by the capacity provider are
* transitioned to use the capacity from the remaining capacity providers. Only capacity providers that aren't
* associated with a cluster can be deleted. To remove a capacity provider from a cluster, you can either use
* PutClusterCapacityProviders or delete the cluster.
*
*
* @param deleteCapacityProviderRequest
* @return Result of the DeleteCapacityProvider operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @sample AmazonECS.DeleteCapacityProvider
* @see AWS API
* Documentation
*/
DeleteCapacityProviderResult deleteCapacityProvider(DeleteCapacityProviderRequest deleteCapacityProviderRequest);
/**
*
* Deletes the specified cluster. The cluster transitions to the INACTIVE
state. Clusters with an
* INACTIVE
status might remain discoverable in your account for a period of time. However, this
* behavior is subject to change in the future. We don't recommend that you rely on INACTIVE
clusters
* persisting.
*
*
* You must deregister all container instances from this cluster before you may delete it. You can list the
* container instances in a cluster with ListContainerInstances and deregister them with
* DeregisterContainerInstance.
*
*
* @param deleteClusterRequest
* @return Result of the DeleteCluster operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster wasn't found. You can view your available clusters with ListClusters. Amazon
* ECS clusters are Region specific.
* @throws ClusterContainsContainerInstancesException
* You can't delete a cluster that has registered container instances. First, deregister the container
* instances before you can delete the cluster. For more information, see
* DeregisterContainerInstance.
* @throws ClusterContainsServicesException
* You can't delete a cluster that contains services. First, update the service to reduce its desired task
* count to 0, and then delete the service. For more information, see UpdateService and
* DeleteService.
* @throws ClusterContainsTasksException
* You can't delete a cluster that has active tasks.
* @throws UpdateInProgressException
* There's already a current Amazon ECS container agent update in progress on the container instance that's
* specified. If the container agent becomes disconnected while it's in a transitional stage, such as
* PENDING
or STAGING
, the update process can get stuck in that state. However,
* when the agent reconnects, it resumes where it stopped previously.
* @sample AmazonECS.DeleteCluster
* @see AWS API
* Documentation
*/
DeleteClusterResult deleteCluster(DeleteClusterRequest deleteClusterRequest);
/**
*
* Deletes a specified service within a cluster. You can delete a service if you have no running tasks in it and the
* desired task count is zero. If the service is actively maintaining tasks, you can't delete it, and you must
* update the service to a desired task count of zero. For more information, see UpdateService.
*
*
*
* When you delete a service, if there are still running tasks that require cleanup, the service status moves from
* ACTIVE
to DRAINING
, and the service is no longer visible in the console or in the
* ListServices API operation. After all tasks have transitioned to either STOPPING
or
* STOPPED
status, the service status moves from DRAINING
to INACTIVE
.
* Services in the DRAINING
or INACTIVE
status can still be viewed with the
* DescribeServices API operation. However, in the future, INACTIVE
services may be cleaned up
* and purged from Amazon ECS record keeping, and DescribeServices calls on those services return a
* ServiceNotFoundException
error.
*
*
*
* If you attempt to create a new service with the same name as an existing service in either ACTIVE
or
* DRAINING
status, you receive an error.
*
*
*
* @param deleteServiceRequest
* @return Result of the DeleteService operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster wasn't found. You can view your available clusters with ListClusters. Amazon
* ECS clusters are Region specific.
* @throws ServiceNotFoundException
* The specified service wasn't found. You can view your available services with ListServices. Amazon
* ECS services are cluster specific and Region specific.
* @sample AmazonECS.DeleteService
* @see AWS API
* Documentation
*/
DeleteServiceResult deleteService(DeleteServiceRequest deleteServiceRequest);
/**
*
* Deletes one or more task definitions.
*
*
* You must deregister a task definition revision before you delete it. For more information, see DeregisterTaskDefinition.
*
*
* When you delete a task definition revision, it is immediately transitions from the INACTIVE
to
* DELETE_IN_PROGRESS
. Existing tasks and services that reference a DELETE_IN_PROGRESS
* task definition revision continue to run without disruption. Existing services that reference a
* DELETE_IN_PROGRESS
task definition revision can still scale up or down by modifying the service's
* desired count.
*
*
* You can't use a DELETE_IN_PROGRESS
task definition revision to run new tasks or create new services.
* You also can't update an existing service to reference a DELETE_IN_PROGRESS
task definition
* revision.
*
*
* A task definition revision will stay in DELETE_IN_PROGRESS
status until all the associated tasks and
* services have been terminated.
*
*
* When you delete all INACTIVE
task definition revisions, the task definition name is not displayed in
* the console and not returned in the API. If a task definition revisions are in the
* DELETE_IN_PROGRESS
state, the task definition name is displayed in the console and returned in the
* API. The task definition name is retained by Amazon ECS and the revision is incremented the next time you create
* a task definition with that name.
*
*
* @param deleteTaskDefinitionsRequest
* @return Result of the DeleteTaskDefinitions operation returned by the service.
* @throws AccessDeniedException
* You don't have authorization to perform the requested action.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @throws ServerException
* These errors are usually caused by a server issue.
* @sample AmazonECS.DeleteTaskDefinitions
* @see AWS API
* Documentation
*/
DeleteTaskDefinitionsResult deleteTaskDefinitions(DeleteTaskDefinitionsRequest deleteTaskDefinitionsRequest);
/**
*
* Deletes a specified task set within a service. This is used when a service uses the EXTERNAL
* deployment controller type. For more information, see Amazon ECS deployment
* types in the Amazon Elastic Container Service Developer Guide.
*
*
* @param deleteTaskSetRequest
* @return Result of the DeleteTaskSet operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster wasn't found. You can view your available clusters with ListClusters. Amazon
* ECS clusters are Region specific.
* @throws UnsupportedFeatureException
* The specified task isn't supported in this Region.
* @throws AccessDeniedException
* You don't have authorization to perform the requested action.
* @throws ServiceNotFoundException
* The specified service wasn't found. You can view your available services with ListServices. Amazon
* ECS services are cluster specific and Region specific.
* @throws ServiceNotActiveException
* The specified service isn't active. You can't update a service that's inactive. If you have previously
* deleted a service, you can re-create it with CreateService.
* @throws TaskSetNotFoundException
* The specified task set wasn't found. You can view your available task sets with DescribeTaskSets.
* Task sets are specific to each cluster, service and Region.
* @sample AmazonECS.DeleteTaskSet
* @see AWS API
* Documentation
*/
DeleteTaskSetResult deleteTaskSet(DeleteTaskSetRequest deleteTaskSetRequest);
/**
*
* Deregisters an Amazon ECS container instance from the specified cluster. This instance is no longer available to
* run tasks.
*
*
* If you intend to use the container instance for some other purpose after deregistration, we recommend that you
* stop all of the tasks running on the container instance before deregistration. That prevents any orphaned tasks
* from consuming resources.
*
*
* Deregistering a container instance removes the instance from a cluster, but it doesn't terminate the EC2
* instance. If you are finished using the instance, be sure to terminate it in the Amazon EC2 console to stop
* billing.
*
*
*
* If you terminate a running container instance, Amazon ECS automatically deregisters the instance from your
* cluster (stopped container instances or instances with disconnected agents aren't automatically deregistered when
* terminated).
*
*
*
* @param deregisterContainerInstanceRequest
* @return Result of the DeregisterContainerInstance operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster wasn't found. You can view your available clusters with ListClusters. Amazon
* ECS clusters are Region specific.
* @sample AmazonECS.DeregisterContainerInstance
* @see AWS API Documentation
*/
DeregisterContainerInstanceResult deregisterContainerInstance(DeregisterContainerInstanceRequest deregisterContainerInstanceRequest);
/**
*
* Deregisters the specified task definition by family and revision. Upon deregistration, the task definition is
* marked as INACTIVE
. Existing tasks and services that reference an INACTIVE
task
* definition continue to run without disruption. Existing services that reference an INACTIVE
task
* definition can still scale up or down by modifying the service's desired count. If you want to delete a task
* definition revision, you must first deregister the task definition revision.
*
*
* You can't use an INACTIVE
task definition to run new tasks or create new services, and you can't
* update an existing service to reference an INACTIVE
task definition. However, there may be up to a
* 10-minute window following deregistration where these restrictions have not yet taken effect.
*
*
*
* At this time, INACTIVE
task definitions remain discoverable in your account indefinitely. However,
* this behavior is subject to change in the future. We don't recommend that you rely on INACTIVE
task
* definitions persisting beyond the lifecycle of any associated tasks and services.
*
*
*
* You must deregister a task definition revision before you delete it. For more information, see DeleteTaskDefinitions.
*
*
* @param deregisterTaskDefinitionRequest
* @return Result of the DeregisterTaskDefinition operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @sample AmazonECS.DeregisterTaskDefinition
* @see AWS
* API Documentation
*/
DeregisterTaskDefinitionResult deregisterTaskDefinition(DeregisterTaskDefinitionRequest deregisterTaskDefinitionRequest);
/**
*
* Describes one or more of your capacity providers.
*
*
* @param describeCapacityProvidersRequest
* @return Result of the DescribeCapacityProviders operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @sample AmazonECS.DescribeCapacityProviders
* @see AWS
* API Documentation
*/
DescribeCapacityProvidersResult describeCapacityProviders(DescribeCapacityProvidersRequest describeCapacityProvidersRequest);
/**
*
* Describes one or more of your clusters.
*
*
* @param describeClustersRequest
* @return Result of the DescribeClusters operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @sample AmazonECS.DescribeClusters
* @see AWS API
* Documentation
*/
DescribeClustersResult describeClusters(DescribeClustersRequest describeClustersRequest);
/**
* Simplified method form for invoking the DescribeClusters operation.
*
* @see #describeClusters(DescribeClustersRequest)
*/
DescribeClustersResult describeClusters();
/**
*
* Describes one or more container instances. Returns metadata about each container instance requested.
*
*
* @param describeContainerInstancesRequest
* @return Result of the DescribeContainerInstances operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster wasn't found. You can view your available clusters with ListClusters. Amazon
* ECS clusters are Region specific.
* @sample AmazonECS.DescribeContainerInstances
* @see AWS
* API Documentation
*/
DescribeContainerInstancesResult describeContainerInstances(DescribeContainerInstancesRequest describeContainerInstancesRequest);
/**
*
* Describes the specified services running in your cluster.
*
*
* @param describeServicesRequest
* @return Result of the DescribeServices operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster wasn't found. You can view your available clusters with ListClusters. Amazon
* ECS clusters are Region specific.
* @sample AmazonECS.DescribeServices
* @see AWS API
* Documentation
*/
DescribeServicesResult describeServices(DescribeServicesRequest describeServicesRequest);
/**
*
* Describes a task definition. You can specify a family
and revision
to find information
* about a specific task definition, or you can simply specify the family to find the latest ACTIVE
* revision in that family.
*
*
*
* You can only describe INACTIVE
task definitions while an active task or service references them.
*
*
*
* @param describeTaskDefinitionRequest
* @return Result of the DescribeTaskDefinition operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @sample AmazonECS.DescribeTaskDefinition
* @see AWS API
* Documentation
*/
DescribeTaskDefinitionResult describeTaskDefinition(DescribeTaskDefinitionRequest describeTaskDefinitionRequest);
/**
*
* Describes the task sets in the specified cluster and service. This is used when a service uses the
* EXTERNAL
deployment controller type. For more information, see Amazon ECS Deployment
* Types in the Amazon Elastic Container Service Developer Guide.
*
*
* @param describeTaskSetsRequest
* @return Result of the DescribeTaskSets operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster wasn't found. You can view your available clusters with ListClusters. Amazon
* ECS clusters are Region specific.
* @throws UnsupportedFeatureException
* The specified task isn't supported in this Region.
* @throws AccessDeniedException
* You don't have authorization to perform the requested action.
* @throws ServiceNotFoundException
* The specified service wasn't found. You can view your available services with ListServices. Amazon
* ECS services are cluster specific and Region specific.
* @throws ServiceNotActiveException
* The specified service isn't active. You can't update a service that's inactive. If you have previously
* deleted a service, you can re-create it with CreateService.
* @sample AmazonECS.DescribeTaskSets
* @see AWS API
* Documentation
*/
DescribeTaskSetsResult describeTaskSets(DescribeTaskSetsRequest describeTaskSetsRequest);
/**
*
* Describes a specified task or tasks.
*
*
* Currently, stopped tasks appear in the returned results for at least one hour.
*
*
* If you have tasks with tags, and then delete the cluster, the tagged tasks are returned in the response. If you
* create a new cluster with the same name as the deleted cluster, the tagged tasks are not included in the
* response.
*
*
* @param describeTasksRequest
* @return Result of the DescribeTasks operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster wasn't found. You can view your available clusters with ListClusters. Amazon
* ECS clusters are Region specific.
* @sample AmazonECS.DescribeTasks
* @see AWS API
* Documentation
*/
DescribeTasksResult describeTasks(DescribeTasksRequest describeTasksRequest);
/**
*
*
* This action is only used by the Amazon ECS agent, and it is not intended for use outside of the agent.
*
*
*
* Returns an endpoint for the Amazon ECS agent to poll for updates.
*
*
* @param discoverPollEndpointRequest
* @return Result of the DiscoverPollEndpoint operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @sample AmazonECS.DiscoverPollEndpoint
* @see AWS API
* Documentation
*/
DiscoverPollEndpointResult discoverPollEndpoint(DiscoverPollEndpointRequest discoverPollEndpointRequest);
/**
* Simplified method form for invoking the DiscoverPollEndpoint operation.
*
* @see #discoverPollEndpoint(DiscoverPollEndpointRequest)
*/
DiscoverPollEndpointResult discoverPollEndpoint();
/**
*
* Runs a command remotely on a container within a task.
*
*
* If you use a condition key in your IAM policy to refine the conditions for the policy statement, for example
* limit the actions to a specific cluster, you receive an AccessDeniedException
when there is a
* mismatch between the condition key value and the corresponding parameter value.
*
*
* For information about required permissions and considerations, see Using Amazon ECS Exec for
* debugging in the Amazon ECS Developer Guide.
*
*
* @param executeCommandRequest
* @return Result of the ExecuteCommand operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @throws AccessDeniedException
* You don't have authorization to perform the requested action.
* @throws ClusterNotFoundException
* The specified cluster wasn't found. You can view your available clusters with ListClusters. Amazon
* ECS clusters are Region specific.
* @throws TargetNotConnectedException
* The execute command cannot run. This error can be caused by any of the following configuration
* issues:
*
* -
*
* Incorrect IAM permissions
*
*
* -
*
* The SSM agent is not installed or is not running
*
*
* -
*
* There is an interface Amazon VPC endpoint for Amazon ECS, but there is not one for Systems Manager
* Session Manager
*
*
*
*
* For information about how to troubleshoot the issues, see Troubleshooting issues
* with ECS Exec in the Amazon Elastic Container Service Developer Guide.
* @sample AmazonECS.ExecuteCommand
* @see AWS API
* Documentation
*/
ExecuteCommandResult executeCommand(ExecuteCommandRequest executeCommandRequest);
/**
*
* Retrieves the protection status of tasks in an Amazon ECS service.
*
*
* @param getTaskProtectionRequest
* @return Result of the GetTaskProtection operation returned by the service.
* @throws AccessDeniedException
* You don't have authorization to perform the requested action.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws ClusterNotFoundException
* The specified cluster wasn't found. You can view your available clusters with ListClusters. Amazon
* ECS clusters are Region specific.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @throws ResourceNotFoundException
* The specified resource wasn't found.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws UnsupportedFeatureException
* The specified task isn't supported in this Region.
* @sample AmazonECS.GetTaskProtection
* @see AWS API
* Documentation
*/
GetTaskProtectionResult getTaskProtection(GetTaskProtectionRequest getTaskProtectionRequest);
/**
*
* Lists the account settings for a specified principal.
*
*
* @param listAccountSettingsRequest
* @return Result of the ListAccountSettings operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @sample AmazonECS.ListAccountSettings
* @see AWS API
* Documentation
*/
ListAccountSettingsResult listAccountSettings(ListAccountSettingsRequest listAccountSettingsRequest);
/**
*
* Lists the attributes for Amazon ECS resources within a specified target type and cluster. When you specify a
* target type and cluster, ListAttributes
returns a list of attribute objects, one for each attribute
* on each resource. You can filter the list of results to a single attribute name to only return results that have
* that name. You can also filter the results by attribute name and value. You can do this, for example, to see
* which container instances in a cluster are running a Linux AMI (ecs.os-type=linux
).
*
*
* @param listAttributesRequest
* @return Result of the ListAttributes operation returned by the service.
* @throws ClusterNotFoundException
* The specified cluster wasn't found. You can view your available clusters with ListClusters. Amazon
* ECS clusters are Region specific.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @sample AmazonECS.ListAttributes
* @see AWS API
* Documentation
*/
ListAttributesResult listAttributes(ListAttributesRequest listAttributesRequest);
/**
*
* Returns a list of existing clusters.
*
*
* @param listClustersRequest
* @return Result of the ListClusters operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @sample AmazonECS.ListClusters
* @see AWS API
* Documentation
*/
ListClustersResult listClusters(ListClustersRequest listClustersRequest);
/**
* Simplified method form for invoking the ListClusters operation.
*
* @see #listClusters(ListClustersRequest)
*/
ListClustersResult listClusters();
/**
*
* Returns a list of container instances in a specified cluster. You can filter the results of a
* ListContainerInstances
operation with cluster query language statements inside the
* filter
parameter. For more information, see Cluster Query
* Language in the Amazon Elastic Container Service Developer Guide.
*
*
* @param listContainerInstancesRequest
* @return Result of the ListContainerInstances operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster wasn't found. You can view your available clusters with ListClusters. Amazon
* ECS clusters are Region specific.
* @sample AmazonECS.ListContainerInstances
* @see AWS API
* Documentation
*/
ListContainerInstancesResult listContainerInstances(ListContainerInstancesRequest listContainerInstancesRequest);
/**
* Simplified method form for invoking the ListContainerInstances operation.
*
* @see #listContainerInstances(ListContainerInstancesRequest)
*/
ListContainerInstancesResult listContainerInstances();
/**
*
* Returns a list of services. You can filter the results by cluster, launch type, and scheduling strategy.
*
*
* @param listServicesRequest
* @return Result of the ListServices operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster wasn't found. You can view your available clusters with ListClusters. Amazon
* ECS clusters are Region specific.
* @sample AmazonECS.ListServices
* @see AWS API
* Documentation
*/
ListServicesResult listServices(ListServicesRequest listServicesRequest);
/**
* Simplified method form for invoking the ListServices operation.
*
* @see #listServices(ListServicesRequest)
*/
ListServicesResult listServices();
/**
*
* This operation lists all of the services that are associated with a Cloud Map namespace. This list might include
* services in different clusters. In contrast, ListServices
can only list services in one cluster at a
* time. If you need to filter the list of services in a single cluster by various parameters, use
* ListServices
. For more information, see Service Connect in
* the Amazon Elastic Container Service Developer Guide.
*
*
* @param listServicesByNamespaceRequest
* @return Result of the ListServicesByNamespace operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @throws NamespaceNotFoundException
* The specified namespace wasn't found.
* @sample AmazonECS.ListServicesByNamespace
* @see AWS
* API Documentation
*/
ListServicesByNamespaceResult listServicesByNamespace(ListServicesByNamespaceRequest listServicesByNamespaceRequest);
/**
*
* List the tags for an Amazon ECS resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws ClusterNotFoundException
* The specified cluster wasn't found. You can view your available clusters with ListClusters. Amazon
* ECS clusters are Region specific.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @sample AmazonECS.ListTagsForResource
* @see AWS API
* Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Returns a list of task definition families that are registered to your account. This list includes task
* definition families that no longer have any ACTIVE
task definition revisions.
*
*
* You can filter out task definition families that don't contain any ACTIVE
task definition revisions
* by setting the status
parameter to ACTIVE
. You can also filter the results with the
* familyPrefix
parameter.
*
*
* @param listTaskDefinitionFamiliesRequest
* @return Result of the ListTaskDefinitionFamilies operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @sample AmazonECS.ListTaskDefinitionFamilies
* @see AWS
* API Documentation
*/
ListTaskDefinitionFamiliesResult listTaskDefinitionFamilies(ListTaskDefinitionFamiliesRequest listTaskDefinitionFamiliesRequest);
/**
* Simplified method form for invoking the ListTaskDefinitionFamilies operation.
*
* @see #listTaskDefinitionFamilies(ListTaskDefinitionFamiliesRequest)
*/
ListTaskDefinitionFamiliesResult listTaskDefinitionFamilies();
/**
*
* Returns a list of task definitions that are registered to your account. You can filter the results by family name
* with the familyPrefix
parameter or by status with the status
parameter.
*
*
* @param listTaskDefinitionsRequest
* @return Result of the ListTaskDefinitions operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @sample AmazonECS.ListTaskDefinitions
* @see AWS API
* Documentation
*/
ListTaskDefinitionsResult listTaskDefinitions(ListTaskDefinitionsRequest listTaskDefinitionsRequest);
/**
* Simplified method form for invoking the ListTaskDefinitions operation.
*
* @see #listTaskDefinitions(ListTaskDefinitionsRequest)
*/
ListTaskDefinitionsResult listTaskDefinitions();
/**
*
* Returns a list of tasks. You can filter the results by cluster, task definition family, container instance,
* launch type, what IAM principal started the task, or by the desired status of the task.
*
*
* Recently stopped tasks might appear in the returned results.
*
*
* @param listTasksRequest
* @return Result of the ListTasks operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster wasn't found. You can view your available clusters with ListClusters. Amazon
* ECS clusters are Region specific.
* @throws ServiceNotFoundException
* The specified service wasn't found. You can view your available services with ListServices. Amazon
* ECS services are cluster specific and Region specific.
* @sample AmazonECS.ListTasks
* @see AWS API
* Documentation
*/
ListTasksResult listTasks(ListTasksRequest listTasksRequest);
/**
* Simplified method form for invoking the ListTasks operation.
*
* @see #listTasks(ListTasksRequest)
*/
ListTasksResult listTasks();
/**
*
* Modifies an account setting. Account settings are set on a per-Region basis.
*
*
* If you change the root user account setting, the default settings are reset for users and roles that do not have
* specified individual account settings. For more information, see Account Settings
* in the Amazon Elastic Container Service Developer Guide.
*
*
* @param putAccountSettingRequest
* @return Result of the PutAccountSetting operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @sample AmazonECS.PutAccountSetting
* @see AWS API
* Documentation
*/
PutAccountSettingResult putAccountSetting(PutAccountSettingRequest putAccountSettingRequest);
/**
*
* Modifies an account setting for all users on an account for whom no individual account setting has been
* specified. Account settings are set on a per-Region basis.
*
*
* @param putAccountSettingDefaultRequest
* @return Result of the PutAccountSettingDefault operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @sample AmazonECS.PutAccountSettingDefault
* @see AWS
* API Documentation
*/
PutAccountSettingDefaultResult putAccountSettingDefault(PutAccountSettingDefaultRequest putAccountSettingDefaultRequest);
/**
*
* Create or update an attribute on an Amazon ECS resource. If the attribute doesn't exist, it's created. If the
* attribute exists, its value is replaced with the specified value. To delete an attribute, use
* DeleteAttributes. For more information, see Attributes in the Amazon Elastic Container Service Developer Guide.
*
*
* @param putAttributesRequest
* @return Result of the PutAttributes operation returned by the service.
* @throws ClusterNotFoundException
* The specified cluster wasn't found. You can view your available clusters with ListClusters. Amazon
* ECS clusters are Region specific.
* @throws TargetNotFoundException
* The specified target wasn't found. You can view your available container instances with
* ListContainerInstances. Amazon ECS container instances are cluster-specific and Region-specific.
* @throws AttributeLimitExceededException
* You can apply up to 10 custom attributes for each resource. You can view the attributes of a resource
* with ListAttributes. You can remove existing attributes on a resource with
* DeleteAttributes.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @sample AmazonECS.PutAttributes
* @see AWS API
* Documentation
*/
PutAttributesResult putAttributes(PutAttributesRequest putAttributesRequest);
/**
*
* Modifies the available capacity providers and the default capacity provider strategy for a cluster.
*
*
* You must specify both the available capacity providers and a default capacity provider strategy for the cluster.
* If the specified cluster has existing capacity providers associated with it, you must specify all existing
* capacity providers in addition to any new ones you want to add. Any existing capacity providers that are
* associated with a cluster that are omitted from a PutClusterCapacityProviders API call will be
* disassociated with the cluster. You can only disassociate an existing capacity provider from a cluster if it's
* not being used by any existing tasks.
*
*
* When creating a service or running a task on a cluster, if no capacity provider or launch type is specified, then
* the cluster's default capacity provider strategy is used. We recommend that you define a default capacity
* provider strategy for your cluster. However, you must specify an empty array ([]
) to bypass defining
* a default strategy.
*
*
* @param putClusterCapacityProvidersRequest
* @return Result of the PutClusterCapacityProviders operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster wasn't found. You can view your available clusters with ListClusters. Amazon
* ECS clusters are Region specific.
* @throws ResourceInUseException
* The specified resource is in-use and can't be removed.
* @throws UpdateInProgressException
* There's already a current Amazon ECS container agent update in progress on the container instance that's
* specified. If the container agent becomes disconnected while it's in a transitional stage, such as
* PENDING
or STAGING
, the update process can get stuck in that state. However,
* when the agent reconnects, it resumes where it stopped previously.
* @sample AmazonECS.PutClusterCapacityProviders
* @see AWS API Documentation
*/
PutClusterCapacityProvidersResult putClusterCapacityProviders(PutClusterCapacityProvidersRequest putClusterCapacityProvidersRequest);
/**
*
*
* This action is only used by the Amazon ECS agent, and it is not intended for use outside of the agent.
*
*
*
* Registers an EC2 instance into the specified cluster. This instance becomes available to place containers on.
*
*
* @param registerContainerInstanceRequest
* @return Result of the RegisterContainerInstance operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @sample AmazonECS.RegisterContainerInstance
* @see AWS
* API Documentation
*/
RegisterContainerInstanceResult registerContainerInstance(RegisterContainerInstanceRequest registerContainerInstanceRequest);
/**
*
* Registers a new task definition from the supplied family
and containerDefinitions
.
* Optionally, you can add data volumes to your containers with the volumes
parameter. For more
* information about task definition parameters and defaults, see Amazon ECS Task
* Definitions in the Amazon Elastic Container Service Developer Guide.
*
*
* You can specify a role for your task with the taskRoleArn
parameter. When you specify a role for a
* task, its containers can then use the latest versions of the CLI or SDKs to make API requests to the Amazon Web
* Services services that are specified in the policy that's associated with the role. For more information, see IAM Roles for Tasks in
* the Amazon Elastic Container Service Developer Guide.
*
*
* You can specify a Docker networking mode for the containers in your task definition with the
* networkMode
parameter. The available network modes correspond to those described in Network settings in the Docker run
* reference. If you specify the awsvpc
network mode, the task is allocated an elastic network
* interface, and you must specify a NetworkConfiguration when you create a service or run a task with the
* task definition. For more information, see Task Networking in
* the Amazon Elastic Container Service Developer Guide.
*
*
* @param registerTaskDefinitionRequest
* @return Result of the RegisterTaskDefinition operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @sample AmazonECS.RegisterTaskDefinition
* @see AWS API
* Documentation
*/
RegisterTaskDefinitionResult registerTaskDefinition(RegisterTaskDefinitionRequest registerTaskDefinitionRequest);
/**
*
* Starts a new task using the specified task definition.
*
*
*
* On March 21, 2024, a change was made to resolve the task definition revision before authorization. When a task
* definition revision is not specified, authorization will occur using the latest revision of a task definition.
*
*
*
* You can allow Amazon ECS to place tasks for you, or you can customize how Amazon ECS places tasks using placement
* constraints and placement strategies. For more information, see Scheduling Tasks in
* the Amazon Elastic Container Service Developer Guide.
*
*
* Alternatively, you can use StartTask to use your own scheduler or place tasks manually on specific
* container instances.
*
*
* Starting April 15, 2023, Amazon Web Services will not onboard new customers to Amazon Elastic Inference (EI), and
* will help current customers migrate their workloads to options that offer better price and performance. After
* April 15, 2023, new customers will not be able to launch instances with Amazon EI accelerators in Amazon
* SageMaker, Amazon ECS, or Amazon EC2. However, customers who have used Amazon EI at least once during the past
* 30-day period are considered current customers and will be able to continue using the service.
*
*
* You can attach Amazon EBS volumes to Amazon ECS tasks by configuring the volume when creating or updating a
* service. For more infomation, see Amazon EBS
* volumes in the Amazon Elastic Container Service Developer Guide.
*
*
* The Amazon ECS API follows an eventual consistency model. This is because of the distributed nature of the system
* supporting the API. This means that the result of an API command you run that affects your Amazon ECS resources
* might not be immediately visible to all subsequent commands you run. Keep this in mind when you carry out an API
* command that immediately follows a previous API command.
*
*
* To manage eventual consistency, you can do the following:
*
*
* -
*
* Confirm the state of the resource before you run a command to modify it. Run the DescribeTasks command using an
* exponential backoff algorithm to ensure that you allow enough time for the previous command to propagate through
* the system. To do this, run the DescribeTasks command repeatedly, starting with a couple of seconds of wait time
* and increasing gradually up to five minutes of wait time.
*
*
* -
*
* Add wait time between subsequent commands, even if the DescribeTasks command returns an accurate response. Apply
* an exponential backoff algorithm starting with a couple of seconds of wait time, and increase gradually up to
* about five minutes of wait time.
*
*
*
*
* @param runTaskRequest
* @return Result of the RunTask operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster wasn't found. You can view your available clusters with ListClusters. Amazon
* ECS clusters are Region specific.
* @throws UnsupportedFeatureException
* The specified task isn't supported in this Region.
* @throws PlatformUnknownException
* The specified platform version doesn't exist.
* @throws PlatformTaskDefinitionIncompatibilityException
* The specified platform version doesn't satisfy the required capabilities of the task definition.
* @throws AccessDeniedException
* You don't have authorization to perform the requested action.
* @throws BlockedException
* Your Amazon Web Services account was blocked. For more information, contact Amazon Web Services Support.
* @throws ConflictException
* The RunTask
request could not be processed due to conflicts. The provided
* clientToken
is already in use with a different RunTask
request. The
* resourceIds
are the existing task ARNs which are already associated with the
* clientToken
.
*
* To fix this issue:
*
*
* -
*
* Run RunTask
with a unique clientToken
.
*
*
* -
*
* Run RunTask
with the clientToken
and the original set of parameters
*
*
* @sample AmazonECS.RunTask
* @see AWS API
* Documentation
*/
RunTaskResult runTask(RunTaskRequest runTaskRequest);
/**
*
* Starts a new task from the specified task definition on the specified container instance or instances.
*
*
*
* On March 21, 2024, a change was made to resolve the task definition revision before authorization. When a task
* definition revision is not specified, authorization will occur using the latest revision of a task definition.
*
*
*
* Starting April 15, 2023, Amazon Web Services will not onboard new customers to Amazon Elastic Inference (EI), and
* will help current customers migrate their workloads to options that offer better price and performance. After
* April 15, 2023, new customers will not be able to launch instances with Amazon EI accelerators in Amazon
* SageMaker, Amazon ECS, or Amazon EC2. However, customers who have used Amazon EI at least once during the past
* 30-day period are considered current customers and will be able to continue using the service.
*
*
* Alternatively, you can use RunTask to place tasks for you. For more information, see Scheduling Tasks in
* the Amazon Elastic Container Service Developer Guide.
*
*
* You can attach Amazon EBS volumes to Amazon ECS tasks by configuring the volume when creating or updating a
* service. For more infomation, see Amazon EBS
* volumes in the Amazon Elastic Container Service Developer Guide.
*
*
* @param startTaskRequest
* @return Result of the StartTask operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster wasn't found. You can view your available clusters with ListClusters. Amazon
* ECS clusters are Region specific.
* @throws UnsupportedFeatureException
* The specified task isn't supported in this Region.
* @sample AmazonECS.StartTask
* @see AWS API
* Documentation
*/
StartTaskResult startTask(StartTaskRequest startTaskRequest);
/**
*
* Stops a running task. Any tags associated with the task will be deleted.
*
*
* When StopTask is called on a task, the equivalent of docker stop
is issued to the containers
* running in the task. This results in a SIGTERM
value and a default 30-second timeout, after which
* the SIGKILL
value is sent and the containers are forcibly stopped. If the container handles the
* SIGTERM
value gracefully and exits within 30 seconds from receiving it, no SIGKILL
* value is sent.
*
*
* For Windows containers, POSIX signals do not work and runtime stops the container by sending a
* CTRL_SHUTDOWN_EVENT
. For more information, see Unable to react to graceful shutdown of (Windows) container
* #25982 on GitHub.
*
*
*
* The default 30-second timeout can be configured on the Amazon ECS container agent with the
* ECS_CONTAINER_STOP_TIMEOUT
variable. For more information, see Amazon ECS Container
* Agent Configuration in the Amazon Elastic Container Service Developer Guide.
*
*
*
* @param stopTaskRequest
* @return Result of the StopTask operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster wasn't found. You can view your available clusters with ListClusters. Amazon
* ECS clusters are Region specific.
* @sample AmazonECS.StopTask
* @see AWS API
* Documentation
*/
StopTaskResult stopTask(StopTaskRequest stopTaskRequest);
/**
*
*
* This action is only used by the Amazon ECS agent, and it is not intended for use outside of the agent.
*
*
*
* Sent to acknowledge that an attachment changed states.
*
*
* @param submitAttachmentStateChangesRequest
* @return Result of the SubmitAttachmentStateChanges operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws AccessDeniedException
* You don't have authorization to perform the requested action.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @sample AmazonECS.SubmitAttachmentStateChanges
* @see AWS API Documentation
*/
SubmitAttachmentStateChangesResult submitAttachmentStateChanges(SubmitAttachmentStateChangesRequest submitAttachmentStateChangesRequest);
/**
*
*
* This action is only used by the Amazon ECS agent, and it is not intended for use outside of the agent.
*
*
*
* Sent to acknowledge that a container changed states.
*
*
* @param submitContainerStateChangeRequest
* @return Result of the SubmitContainerStateChange operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws AccessDeniedException
* You don't have authorization to perform the requested action.
* @sample AmazonECS.SubmitContainerStateChange
* @see AWS
* API Documentation
*/
SubmitContainerStateChangeResult submitContainerStateChange(SubmitContainerStateChangeRequest submitContainerStateChangeRequest);
/**
* Simplified method form for invoking the SubmitContainerStateChange operation.
*
* @see #submitContainerStateChange(SubmitContainerStateChangeRequest)
*/
SubmitContainerStateChangeResult submitContainerStateChange();
/**
*
*
* This action is only used by the Amazon ECS agent, and it is not intended for use outside of the agent.
*
*
*
* Sent to acknowledge that a task changed states.
*
*
* @param submitTaskStateChangeRequest
* @return Result of the SubmitTaskStateChange operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws AccessDeniedException
* You don't have authorization to perform the requested action.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @sample AmazonECS.SubmitTaskStateChange
* @see AWS API
* Documentation
*/
SubmitTaskStateChangeResult submitTaskStateChange(SubmitTaskStateChangeRequest submitTaskStateChangeRequest);
/**
*
* Associates the specified tags to a resource with the specified resourceArn
. If existing tags on a
* resource aren't specified in the request parameters, they aren't changed. When a resource is deleted, the tags
* that are associated with that resource are deleted as well.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws ClusterNotFoundException
* The specified cluster wasn't found. You can view your available clusters with ListClusters. Amazon
* ECS clusters are Region specific.
* @throws ResourceNotFoundException
* The specified resource wasn't found.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @sample AmazonECS.TagResource
* @see AWS API
* Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Deletes specified tags from a resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws ClusterNotFoundException
* The specified cluster wasn't found. You can view your available clusters with ListClusters. Amazon
* ECS clusters are Region specific.
* @throws ResourceNotFoundException
* The specified resource wasn't found.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @sample AmazonECS.UntagResource
* @see AWS API
* Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Modifies the parameters for a capacity provider.
*
*
* @param updateCapacityProviderRequest
* @return Result of the UpdateCapacityProvider operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @sample AmazonECS.UpdateCapacityProvider
* @see AWS API
* Documentation
*/
UpdateCapacityProviderResult updateCapacityProvider(UpdateCapacityProviderRequest updateCapacityProviderRequest);
/**
*
* Updates the cluster.
*
*
* @param updateClusterRequest
* @return Result of the UpdateCluster operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws ClusterNotFoundException
* The specified cluster wasn't found. You can view your available clusters with ListClusters. Amazon
* ECS clusters are Region specific.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @throws NamespaceNotFoundException
* The specified namespace wasn't found.
* @sample AmazonECS.UpdateCluster
* @see AWS API
* Documentation
*/
UpdateClusterResult updateCluster(UpdateClusterRequest updateClusterRequest);
/**
*
* Modifies the settings to use for a cluster.
*
*
* @param updateClusterSettingsRequest
* @return Result of the UpdateClusterSettings operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws ClusterNotFoundException
* The specified cluster wasn't found. You can view your available clusters with ListClusters. Amazon
* ECS clusters are Region specific.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @sample AmazonECS.UpdateClusterSettings
* @see AWS API
* Documentation
*/
UpdateClusterSettingsResult updateClusterSettings(UpdateClusterSettingsRequest updateClusterSettingsRequest);
/**
*
* Updates the Amazon ECS container agent on a specified container instance. Updating the Amazon ECS container agent
* doesn't interrupt running tasks or services on the container instance. The process for updating the agent differs
* depending on whether your container instance was launched with the Amazon ECS-optimized AMI or another operating
* system.
*
*
*
* The UpdateContainerAgent
API isn't supported for container instances using the Amazon ECS-optimized
* Amazon Linux 2 (arm64) AMI. To update the container agent, you can update the ecs-init
package. This
* updates the agent. For more information, see Updating the Amazon
* ECS container agent in the Amazon Elastic Container Service Developer Guide.
*
*
*
* Agent updates with the UpdateContainerAgent
API operation do not apply to Windows container
* instances. We recommend that you launch new container instances to update the agent version in your Windows
* clusters.
*
*
*
* The UpdateContainerAgent
API requires an Amazon ECS-optimized AMI or Amazon Linux AMI with the
* ecs-init
service installed and running. For help updating the Amazon ECS container agent on other
* operating systems, see Manually updating the Amazon ECS container agent in the Amazon Elastic Container Service Developer
* Guide.
*
*
* @param updateContainerAgentRequest
* @return Result of the UpdateContainerAgent operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster wasn't found. You can view your available clusters with ListClusters. Amazon
* ECS clusters are Region specific.
* @throws UpdateInProgressException
* There's already a current Amazon ECS container agent update in progress on the container instance that's
* specified. If the container agent becomes disconnected while it's in a transitional stage, such as
* PENDING
or STAGING
, the update process can get stuck in that state. However,
* when the agent reconnects, it resumes where it stopped previously.
* @throws NoUpdateAvailableException
* There's no update available for this Amazon ECS container agent. This might be because the agent is
* already running the latest version or because it's so old that there's no update path to the current
* version.
* @throws MissingVersionException
* Amazon ECS can't determine the current version of the Amazon ECS container agent on the container
* instance and doesn't have enough information to proceed with an update. This could be because the agent
* running on the container instance is a previous or custom version that doesn't use our version
* information.
* @sample AmazonECS.UpdateContainerAgent
* @see AWS API
* Documentation
*/
UpdateContainerAgentResult updateContainerAgent(UpdateContainerAgentRequest updateContainerAgentRequest);
/**
*
* Modifies the status of an Amazon ECS container instance.
*
*
* Once a container instance has reached an ACTIVE
state, you can change the status of a container
* instance to DRAINING
to manually remove an instance from a cluster, for example to perform system
* updates, update the Docker daemon, or scale down the cluster size.
*
*
*
* A container instance can't be changed to DRAINING
until it has reached an ACTIVE
* status. If the instance is in any other status, an error will be received.
*
*
*
* When you set a container instance to DRAINING
, Amazon ECS prevents new tasks from being scheduled
* for placement on the container instance and replacement service tasks are started on other container instances in
* the cluster if the resources are available. Service tasks on the container instance that are in the
* PENDING
state are stopped immediately.
*
*
* Service tasks on the container instance that are in the RUNNING
state are stopped and replaced
* according to the service's deployment configuration parameters, minimumHealthyPercent
and
* maximumPercent
. You can change the deployment configuration of your service using
* UpdateService.
*
*
* -
*
* If minimumHealthyPercent
is below 100%, the scheduler can ignore desiredCount
* temporarily during task replacement. For example, desiredCount
is four tasks, a minimum of 50%
* allows the scheduler to stop two existing tasks before starting two new tasks. If the minimum is 100%, the
* service scheduler can't remove existing tasks until the replacement tasks are considered healthy. Tasks for
* services that do not use a load balancer are considered healthy if they're in the RUNNING
state.
* Tasks for services that use a load balancer are considered healthy if they're in the RUNNING
state
* and are reported as healthy by the load balancer.
*
*
* -
*
* The maximumPercent
parameter represents an upper limit on the number of running tasks during task
* replacement. You can use this to define the replacement batch size. For example, if desiredCount
is
* four tasks, a maximum of 200% starts four new tasks before stopping the four tasks to be drained, provided that
* the cluster resources required to do this are available. If the maximum is 100%, then replacement tasks can't
* start until the draining tasks have stopped.
*
*
*
*
* Any PENDING
or RUNNING
tasks that do not belong to a service aren't affected. You must
* wait for them to finish or stop them manually.
*
*
* A container instance has completed draining when it has no more RUNNING
tasks. You can verify this
* using ListTasks.
*
*
* When a container instance has been drained, you can set a container instance to ACTIVE
status and
* once it has reached that status the Amazon ECS scheduler can begin scheduling tasks on the instance again.
*
*
* @param updateContainerInstancesStateRequest
* @return Result of the UpdateContainerInstancesState operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster wasn't found. You can view your available clusters with ListClusters. Amazon
* ECS clusters are Region specific.
* @sample AmazonECS.UpdateContainerInstancesState
* @see AWS API Documentation
*/
UpdateContainerInstancesStateResult updateContainerInstancesState(UpdateContainerInstancesStateRequest updateContainerInstancesStateRequest);
/**
*
* Modifies the parameters of a service.
*
*
*
* On March 21, 2024, a change was made to resolve the task definition revision before authorization. When a task
* definition revision is not specified, authorization will occur using the latest revision of a task definition.
*
*
*
* For services using the rolling update (ECS
) you can update the desired count, deployment
* configuration, network configuration, load balancers, service registries, enable ECS managed tags option,
* propagate tags option, task placement constraints and strategies, and task definition. When you update any of
* these parameters, Amazon ECS starts new tasks with the new configuration.
*
*
* You can attach Amazon EBS volumes to Amazon ECS tasks by configuring the volume when starting or running a task,
* or when creating or updating a service. For more infomation, see Amazon EBS
* volumes in the Amazon Elastic Container Service Developer Guide. You can update your volume
* configurations and trigger a new deployment. volumeConfigurations
is only supported for REPLICA
* service and not DAEMON service. If you leave volumeConfigurations
null
, it doesn't
* trigger a new deployment. For more infomation on volumes, see Amazon EBS
* volumes in the Amazon Elastic Container Service Developer Guide.
*
*
* For services using the blue/green (CODE_DEPLOY
) deployment controller, only the desired count,
* deployment configuration, health check grace period, task placement constraints and strategies, enable ECS
* managed tags option, and propagate tags can be updated using this API. If the network configuration, platform
* version, task definition, or load balancer need to be updated, create a new CodeDeploy deployment. For more
* information, see CreateDeployment
* in the CodeDeploy API Reference.
*
*
* For services using an external deployment controller, you can update only the desired count, task placement
* constraints and strategies, health check grace period, enable ECS managed tags option, and propagate tags option,
* using this API. If the launch type, load balancer, network configuration, platform version, or task definition
* need to be updated, create a new task set For more information, see CreateTaskSet.
*
*
* You can add to or subtract from the number of instantiations of a task definition in a service by specifying the
* cluster that the service is running in and a new desiredCount
parameter.
*
*
* You can attach Amazon EBS volumes to Amazon ECS tasks by configuring the volume when starting or running a task,
* or when creating or updating a service. For more infomation, see Amazon EBS
* volumes in the Amazon Elastic Container Service Developer Guide.
*
*
* If you have updated the container image of your application, you can create a new task definition with that image
* and deploy it to your service. The service scheduler uses the minimum healthy percent and maximum percent
* parameters (in the service's deployment configuration) to determine the deployment strategy.
*
*
*
* If your updated Docker image uses the same tag as what is in the existing task definition for your service (for
* example, my_image:latest
), you don't need to create a new revision of your task definition. You can
* update the service using the forceNewDeployment
option. The new tasks launched by the deployment
* pull the current image/tag combination from your repository when they start.
*
*
*
* You can also update the deployment configuration of a service. When a deployment is triggered by updating the
* task definition of a service, the service scheduler uses the deployment configuration parameters,
* minimumHealthyPercent
and maximumPercent
, to determine the deployment strategy.
*
*
* -
*
* If minimumHealthyPercent
is below 100%, the scheduler can ignore desiredCount
* temporarily during a deployment. For example, if desiredCount
is four tasks, a minimum of 50% allows
* the scheduler to stop two existing tasks before starting two new tasks. Tasks for services that don't use a load
* balancer are considered healthy if they're in the RUNNING
state. Tasks for services that use a load
* balancer are considered healthy if they're in the RUNNING
state and are reported as healthy by the
* load balancer.
*
*
* -
*
* The maximumPercent
parameter represents an upper limit on the number of running tasks during a
* deployment. You can use it to define the deployment batch size. For example, if desiredCount
is four
* tasks, a maximum of 200% starts four new tasks before stopping the four older tasks (provided that the cluster
* resources required to do this are available).
*
*
*
*
* When UpdateService stops a task during a deployment, the equivalent of docker stop
is issued
* to the containers running in the task. This results in a SIGTERM
and a 30-second timeout. After
* this, SIGKILL
is sent and the containers are forcibly stopped. If the container handles the
* SIGTERM
gracefully and exits within 30 seconds from receiving it, no SIGKILL
is sent.
*
*
* When the service scheduler launches new tasks, it determines task placement in your cluster with the following
* logic.
*
*
* -
*
* Determine which of the container instances in your cluster can support your service's task definition. For
* example, they have the required CPU, memory, ports, and container instance attributes.
*
*
* -
*
* By default, the service scheduler attempts to balance tasks across Availability Zones in this manner even though
* you can choose a different placement strategy.
*
*
* -
*
* Sort the valid container instances by the fewest number of running tasks for this service in the same
* Availability Zone as the instance. For example, if zone A has one running service task and zones B and C each
* have zero, valid container instances in either zone B or C are considered optimal for placement.
*
*
* -
*
* Place the new service task on a valid container instance in an optimal Availability Zone (based on the previous
* steps), favoring container instances with the fewest number of running tasks for this service.
*
*
*
*
*
*
* When the service scheduler stops running tasks, it attempts to maintain balance across the Availability Zones in
* your cluster using the following logic:
*
*
* -
*
* Sort the container instances by the largest number of running tasks for this service in the same Availability
* Zone as the instance. For example, if zone A has one running service task and zones B and C each have two,
* container instances in either zone B or C are considered optimal for termination.
*
*
* -
*
* Stop the task on a container instance in an optimal Availability Zone (based on the previous steps), favoring
* container instances with the largest number of running tasks for this service.
*
*
*
*
*
* You must have a service-linked role when you update any of the following service properties:
*
*
* -
*
* loadBalancers
,
*
*
* -
*
* serviceRegistries
*
*
*
*
* For more information about the role see the CreateService
request parameter role
.
*
*
*
* @param updateServiceRequest
* @return Result of the UpdateService operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster wasn't found. You can view your available clusters with ListClusters. Amazon
* ECS clusters are Region specific.
* @throws ServiceNotFoundException
* The specified service wasn't found. You can view your available services with ListServices. Amazon
* ECS services are cluster specific and Region specific.
* @throws ServiceNotActiveException
* The specified service isn't active. You can't update a service that's inactive. If you have previously
* deleted a service, you can re-create it with CreateService.
* @throws PlatformUnknownException
* The specified platform version doesn't exist.
* @throws PlatformTaskDefinitionIncompatibilityException
* The specified platform version doesn't satisfy the required capabilities of the task definition.
* @throws AccessDeniedException
* You don't have authorization to perform the requested action.
* @throws NamespaceNotFoundException
* The specified namespace wasn't found.
* @throws UnsupportedFeatureException
* The specified task isn't supported in this Region.
* @sample AmazonECS.UpdateService
* @see AWS API
* Documentation
*/
UpdateServiceResult updateService(UpdateServiceRequest updateServiceRequest);
/**
*
* Modifies which task set in a service is the primary task set. Any parameters that are updated on the primary task
* set in a service will transition to the service. This is used when a service uses the EXTERNAL
* deployment controller type. For more information, see Amazon ECS Deployment
* Types in the Amazon Elastic Container Service Developer Guide.
*
*
* @param updateServicePrimaryTaskSetRequest
* @return Result of the UpdateServicePrimaryTaskSet operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster wasn't found. You can view your available clusters with ListClusters. Amazon
* ECS clusters are Region specific.
* @throws UnsupportedFeatureException
* The specified task isn't supported in this Region.
* @throws ServiceNotFoundException
* The specified service wasn't found. You can view your available services with ListServices. Amazon
* ECS services are cluster specific and Region specific.
* @throws ServiceNotActiveException
* The specified service isn't active. You can't update a service that's inactive. If you have previously
* deleted a service, you can re-create it with CreateService.
* @throws TaskSetNotFoundException
* The specified task set wasn't found. You can view your available task sets with DescribeTaskSets.
* Task sets are specific to each cluster, service and Region.
* @throws AccessDeniedException
* You don't have authorization to perform the requested action.
* @sample AmazonECS.UpdateServicePrimaryTaskSet
* @see AWS API Documentation
*/
UpdateServicePrimaryTaskSetResult updateServicePrimaryTaskSet(UpdateServicePrimaryTaskSetRequest updateServicePrimaryTaskSetRequest);
/**
*
* Updates the protection status of a task. You can set protectionEnabled
to true
to
* protect your task from termination during scale-in events from Service
* Autoscaling or deployments.
*
*
* Task-protection, by default, expires after 2 hours at which point Amazon ECS clears the
* protectionEnabled
property making the task eligible for termination by a subsequent scale-in event.
*
*
* You can specify a custom expiration period for task protection from 1 minute to up to 2,880 minutes (48 hours).
* To specify the custom expiration period, set the expiresInMinutes
property. The
* expiresInMinutes
property is always reset when you invoke this operation for a task that already has
* protectionEnabled
set to true
. You can keep extending the protection expiration period
* of a task by invoking this operation repeatedly.
*
*
* To learn more about Amazon ECS task protection, see Task scale-in
* protection in the Amazon Elastic Container Service Developer Guide .
*
*
*
* This operation is only supported for tasks belonging to an Amazon ECS service. Invoking this operation for a
* standalone task will result in an TASK_NOT_VALID
failure. For more information, see API failure
* reasons.
*
*
*
* If you prefer to set task protection from within the container, we recommend using the Task
* scale-in protection endpoint.
*
*
*
* @param updateTaskProtectionRequest
* @return Result of the UpdateTaskProtection operation returned by the service.
* @throws AccessDeniedException
* You don't have authorization to perform the requested action.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws ClusterNotFoundException
* The specified cluster wasn't found. You can view your available clusters with ListClusters. Amazon
* ECS clusters are Region specific.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @throws ResourceNotFoundException
* The specified resource wasn't found.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws UnsupportedFeatureException
* The specified task isn't supported in this Region.
* @sample AmazonECS.UpdateTaskProtection
* @see AWS API
* Documentation
*/
UpdateTaskProtectionResult updateTaskProtection(UpdateTaskProtectionRequest updateTaskProtectionRequest);
/**
*
* Modifies a task set. This is used when a service uses the EXTERNAL
deployment controller type. For
* more information, see Amazon ECS Deployment
* Types in the Amazon Elastic Container Service Developer Guide.
*
*
* @param updateTaskSetRequest
* @return Result of the UpdateTaskSet operation returned by the service.
* @throws ServerException
* These errors are usually caused by a server issue.
* @throws ClientException
* These errors are usually caused by a client action. This client action might be using an action or
* resource on behalf of a user that doesn't have permissions to use the action or resource. Or, it might be
* specifying an identifier that isn't valid.
* @throws InvalidParameterException
* The specified parameter isn't valid. Review the available parameters for the API request.
* @throws ClusterNotFoundException
* The specified cluster wasn't found. You can view your available clusters with ListClusters. Amazon
* ECS clusters are Region specific.
* @throws UnsupportedFeatureException
* The specified task isn't supported in this Region.
* @throws AccessDeniedException
* You don't have authorization to perform the requested action.
* @throws ServiceNotFoundException
* The specified service wasn't found. You can view your available services with ListServices. Amazon
* ECS services are cluster specific and Region specific.
* @throws ServiceNotActiveException
* The specified service isn't active. You can't update a service that's inactive. If you have previously
* deleted a service, you can re-create it with CreateService.
* @throws TaskSetNotFoundException
* The specified task set wasn't found. You can view your available task sets with DescribeTaskSets.
* Task sets are specific to each cluster, service and Region.
* @sample AmazonECS.UpdateTaskSet
* @see AWS API
* Documentation
*/
UpdateTaskSetResult updateTaskSet(UpdateTaskSetRequest updateTaskSetRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
AmazonECSWaiters waiters();
}