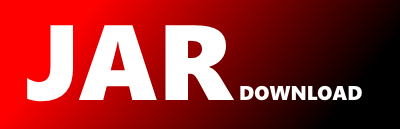
com.amazonaws.services.ecs.model.Deployment Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ecs Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ecs.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The details of an Amazon ECS service deployment. This is used only when a service uses the ECS
* deployment controller type.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Deployment implements Serializable, Cloneable, StructuredPojo {
/**
*
* The ID of the deployment.
*
*/
private String id;
/**
*
* The status of the deployment. The following describes each state.
*
*
* - PRIMARY
* -
*
* The most recent deployment of a service.
*
*
* - ACTIVE
* -
*
* A service deployment that still has running tasks, but are in the process of being replaced with a new
* PRIMARY
deployment.
*
*
* - INACTIVE
* -
*
* A deployment that has been completely replaced.
*
*
*
*/
private String status;
/**
*
* The most recent task definition that was specified for the tasks in the service to use.
*
*/
private String taskDefinition;
/**
*
* The most recent desired count of tasks that was specified for the service to deploy or maintain.
*
*/
private Integer desiredCount;
/**
*
* The number of tasks in the deployment that are in the PENDING
status.
*
*/
private Integer pendingCount;
/**
*
* The number of tasks in the deployment that are in the RUNNING
status.
*
*/
private Integer runningCount;
/**
*
* The number of consecutively failed tasks in the deployment. A task is considered a failure if the service
* scheduler can't launch the task, the task doesn't transition to a RUNNING
state, or if it fails any
* of its defined health checks and is stopped.
*
*
*
* Once a service deployment has one or more successfully running tasks, the failed task count resets to zero and
* stops being evaluated.
*
*
*/
private Integer failedTasks;
/**
*
* The Unix timestamp for the time when the service deployment was created.
*
*/
private java.util.Date createdAt;
/**
*
* The Unix timestamp for the time when the service deployment was last updated.
*
*/
private java.util.Date updatedAt;
/**
*
* The capacity provider strategy that the deployment is using.
*
*/
private com.amazonaws.internal.SdkInternalList capacityProviderStrategy;
/**
*
* The launch type the tasks in the service are using. For more information, see Amazon ECS Launch Types
* in the Amazon Elastic Container Service Developer Guide.
*
*/
private String launchType;
/**
*
* The platform version that your tasks in the service run on. A platform version is only specified for tasks using
* the Fargate launch type. If one isn't specified, the LATEST
platform version is used. For more
* information, see Fargate Platform
* Versions in the Amazon Elastic Container Service Developer Guide.
*
*/
private String platformVersion;
/**
*
* The operating system that your tasks in the service, or tasks are running on. A platform family is specified only
* for tasks using the Fargate launch type.
*
*
* All tasks that run as part of this service must use the same platformFamily
value as the service,
* for example, LINUX.
.
*
*/
private String platformFamily;
/**
*
* The VPC subnet and security group configuration for tasks that receive their own elastic network interface by
* using the awsvpc
networking mode.
*
*/
private NetworkConfiguration networkConfiguration;
/**
*
*
* The rolloutState
of a service is only returned for services that use the rolling update (
* ECS
) deployment type that aren't behind a Classic Load Balancer.
*
*
*
* The rollout state of the deployment. When a service deployment is started, it begins in an
* IN_PROGRESS
state. When the service reaches a steady state, the deployment transitions to a
* COMPLETED
state. If the service fails to reach a steady state and circuit breaker is turned on, the
* deployment transitions to a FAILED
state. A deployment in FAILED
state doesn't launch
* any new tasks. For more information, see DeploymentCircuitBreaker.
*
*/
private String rolloutState;
/**
*
* A description of the rollout state of a deployment.
*
*/
private String rolloutStateReason;
/**
*
* The details of the Service Connect configuration that's used by this deployment. Compare the configuration
* between multiple deployments when troubleshooting issues with new deployments.
*
*
* The configuration for this service to discover and connect to services, and be discovered by, and connected from,
* other services within a namespace.
*
*
* Tasks that run in a namespace can use short names to connect to services in the namespace. Tasks can connect to
* services across all of the clusters in the namespace. Tasks connect through a managed proxy container that
* collects logs and metrics for increased visibility. Only the tasks that Amazon ECS services create are supported
* with Service Connect. For more information, see Service Connect in
* the Amazon Elastic Container Service Developer Guide.
*
*/
private ServiceConnectConfiguration serviceConnectConfiguration;
/**
*
* The list of Service Connect resources that are associated with this deployment. Each list entry maps a discovery
* name to a Cloud Map service name.
*
*/
private com.amazonaws.internal.SdkInternalList serviceConnectResources;
/**
*
* The details of the volume that was configuredAtLaunch
. You can configure different settings like the
* size, throughput, volumeType, and ecryption in ServiceManagedEBSVolumeConfiguration. The name
of the volume must match the name
* from the task definition.
*
*/
private com.amazonaws.internal.SdkInternalList volumeConfigurations;
/**
*
* The Fargate ephemeral storage settings for the deployment.
*
*/
private DeploymentEphemeralStorage fargateEphemeralStorage;
/**
*
* The ID of the deployment.
*
*
* @param id
* The ID of the deployment.
*/
public void setId(String id) {
this.id = id;
}
/**
*
* The ID of the deployment.
*
*
* @return The ID of the deployment.
*/
public String getId() {
return this.id;
}
/**
*
* The ID of the deployment.
*
*
* @param id
* The ID of the deployment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Deployment withId(String id) {
setId(id);
return this;
}
/**
*
* The status of the deployment. The following describes each state.
*
*
* - PRIMARY
* -
*
* The most recent deployment of a service.
*
*
* - ACTIVE
* -
*
* A service deployment that still has running tasks, but are in the process of being replaced with a new
* PRIMARY
deployment.
*
*
* - INACTIVE
* -
*
* A deployment that has been completely replaced.
*
*
*
*
* @param status
* The status of the deployment. The following describes each state.
*
* - PRIMARY
* -
*
* The most recent deployment of a service.
*
*
* - ACTIVE
* -
*
* A service deployment that still has running tasks, but are in the process of being replaced with a new
* PRIMARY
deployment.
*
*
* - INACTIVE
* -
*
* A deployment that has been completely replaced.
*
*
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the deployment. The following describes each state.
*
*
* - PRIMARY
* -
*
* The most recent deployment of a service.
*
*
* - ACTIVE
* -
*
* A service deployment that still has running tasks, but are in the process of being replaced with a new
* PRIMARY
deployment.
*
*
* - INACTIVE
* -
*
* A deployment that has been completely replaced.
*
*
*
*
* @return The status of the deployment. The following describes each state.
*
* - PRIMARY
* -
*
* The most recent deployment of a service.
*
*
* - ACTIVE
* -
*
* A service deployment that still has running tasks, but are in the process of being replaced with a new
* PRIMARY
deployment.
*
*
* - INACTIVE
* -
*
* A deployment that has been completely replaced.
*
*
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of the deployment. The following describes each state.
*
*
* - PRIMARY
* -
*
* The most recent deployment of a service.
*
*
* - ACTIVE
* -
*
* A service deployment that still has running tasks, but are in the process of being replaced with a new
* PRIMARY
deployment.
*
*
* - INACTIVE
* -
*
* A deployment that has been completely replaced.
*
*
*
*
* @param status
* The status of the deployment. The following describes each state.
*
* - PRIMARY
* -
*
* The most recent deployment of a service.
*
*
* - ACTIVE
* -
*
* A service deployment that still has running tasks, but are in the process of being replaced with a new
* PRIMARY
deployment.
*
*
* - INACTIVE
* -
*
* A deployment that has been completely replaced.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Deployment withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The most recent task definition that was specified for the tasks in the service to use.
*
*
* @param taskDefinition
* The most recent task definition that was specified for the tasks in the service to use.
*/
public void setTaskDefinition(String taskDefinition) {
this.taskDefinition = taskDefinition;
}
/**
*
* The most recent task definition that was specified for the tasks in the service to use.
*
*
* @return The most recent task definition that was specified for the tasks in the service to use.
*/
public String getTaskDefinition() {
return this.taskDefinition;
}
/**
*
* The most recent task definition that was specified for the tasks in the service to use.
*
*
* @param taskDefinition
* The most recent task definition that was specified for the tasks in the service to use.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Deployment withTaskDefinition(String taskDefinition) {
setTaskDefinition(taskDefinition);
return this;
}
/**
*
* The most recent desired count of tasks that was specified for the service to deploy or maintain.
*
*
* @param desiredCount
* The most recent desired count of tasks that was specified for the service to deploy or maintain.
*/
public void setDesiredCount(Integer desiredCount) {
this.desiredCount = desiredCount;
}
/**
*
* The most recent desired count of tasks that was specified for the service to deploy or maintain.
*
*
* @return The most recent desired count of tasks that was specified for the service to deploy or maintain.
*/
public Integer getDesiredCount() {
return this.desiredCount;
}
/**
*
* The most recent desired count of tasks that was specified for the service to deploy or maintain.
*
*
* @param desiredCount
* The most recent desired count of tasks that was specified for the service to deploy or maintain.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Deployment withDesiredCount(Integer desiredCount) {
setDesiredCount(desiredCount);
return this;
}
/**
*
* The number of tasks in the deployment that are in the PENDING
status.
*
*
* @param pendingCount
* The number of tasks in the deployment that are in the PENDING
status.
*/
public void setPendingCount(Integer pendingCount) {
this.pendingCount = pendingCount;
}
/**
*
* The number of tasks in the deployment that are in the PENDING
status.
*
*
* @return The number of tasks in the deployment that are in the PENDING
status.
*/
public Integer getPendingCount() {
return this.pendingCount;
}
/**
*
* The number of tasks in the deployment that are in the PENDING
status.
*
*
* @param pendingCount
* The number of tasks in the deployment that are in the PENDING
status.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Deployment withPendingCount(Integer pendingCount) {
setPendingCount(pendingCount);
return this;
}
/**
*
* The number of tasks in the deployment that are in the RUNNING
status.
*
*
* @param runningCount
* The number of tasks in the deployment that are in the RUNNING
status.
*/
public void setRunningCount(Integer runningCount) {
this.runningCount = runningCount;
}
/**
*
* The number of tasks in the deployment that are in the RUNNING
status.
*
*
* @return The number of tasks in the deployment that are in the RUNNING
status.
*/
public Integer getRunningCount() {
return this.runningCount;
}
/**
*
* The number of tasks in the deployment that are in the RUNNING
status.
*
*
* @param runningCount
* The number of tasks in the deployment that are in the RUNNING
status.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Deployment withRunningCount(Integer runningCount) {
setRunningCount(runningCount);
return this;
}
/**
*
* The number of consecutively failed tasks in the deployment. A task is considered a failure if the service
* scheduler can't launch the task, the task doesn't transition to a RUNNING
state, or if it fails any
* of its defined health checks and is stopped.
*
*
*
* Once a service deployment has one or more successfully running tasks, the failed task count resets to zero and
* stops being evaluated.
*
*
*
* @param failedTasks
* The number of consecutively failed tasks in the deployment. A task is considered a failure if the service
* scheduler can't launch the task, the task doesn't transition to a RUNNING
state, or if it
* fails any of its defined health checks and is stopped.
*
* Once a service deployment has one or more successfully running tasks, the failed task count resets to zero
* and stops being evaluated.
*
*/
public void setFailedTasks(Integer failedTasks) {
this.failedTasks = failedTasks;
}
/**
*
* The number of consecutively failed tasks in the deployment. A task is considered a failure if the service
* scheduler can't launch the task, the task doesn't transition to a RUNNING
state, or if it fails any
* of its defined health checks and is stopped.
*
*
*
* Once a service deployment has one or more successfully running tasks, the failed task count resets to zero and
* stops being evaluated.
*
*
*
* @return The number of consecutively failed tasks in the deployment. A task is considered a failure if the service
* scheduler can't launch the task, the task doesn't transition to a RUNNING
state, or if it
* fails any of its defined health checks and is stopped.
*
* Once a service deployment has one or more successfully running tasks, the failed task count resets to
* zero and stops being evaluated.
*
*/
public Integer getFailedTasks() {
return this.failedTasks;
}
/**
*
* The number of consecutively failed tasks in the deployment. A task is considered a failure if the service
* scheduler can't launch the task, the task doesn't transition to a RUNNING
state, or if it fails any
* of its defined health checks and is stopped.
*
*
*
* Once a service deployment has one or more successfully running tasks, the failed task count resets to zero and
* stops being evaluated.
*
*
*
* @param failedTasks
* The number of consecutively failed tasks in the deployment. A task is considered a failure if the service
* scheduler can't launch the task, the task doesn't transition to a RUNNING
state, or if it
* fails any of its defined health checks and is stopped.
*
* Once a service deployment has one or more successfully running tasks, the failed task count resets to zero
* and stops being evaluated.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Deployment withFailedTasks(Integer failedTasks) {
setFailedTasks(failedTasks);
return this;
}
/**
*
* The Unix timestamp for the time when the service deployment was created.
*
*
* @param createdAt
* The Unix timestamp for the time when the service deployment was created.
*/
public void setCreatedAt(java.util.Date createdAt) {
this.createdAt = createdAt;
}
/**
*
* The Unix timestamp for the time when the service deployment was created.
*
*
* @return The Unix timestamp for the time when the service deployment was created.
*/
public java.util.Date getCreatedAt() {
return this.createdAt;
}
/**
*
* The Unix timestamp for the time when the service deployment was created.
*
*
* @param createdAt
* The Unix timestamp for the time when the service deployment was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Deployment withCreatedAt(java.util.Date createdAt) {
setCreatedAt(createdAt);
return this;
}
/**
*
* The Unix timestamp for the time when the service deployment was last updated.
*
*
* @param updatedAt
* The Unix timestamp for the time when the service deployment was last updated.
*/
public void setUpdatedAt(java.util.Date updatedAt) {
this.updatedAt = updatedAt;
}
/**
*
* The Unix timestamp for the time when the service deployment was last updated.
*
*
* @return The Unix timestamp for the time when the service deployment was last updated.
*/
public java.util.Date getUpdatedAt() {
return this.updatedAt;
}
/**
*
* The Unix timestamp for the time when the service deployment was last updated.
*
*
* @param updatedAt
* The Unix timestamp for the time when the service deployment was last updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Deployment withUpdatedAt(java.util.Date updatedAt) {
setUpdatedAt(updatedAt);
return this;
}
/**
*
* The capacity provider strategy that the deployment is using.
*
*
* @return The capacity provider strategy that the deployment is using.
*/
public java.util.List getCapacityProviderStrategy() {
if (capacityProviderStrategy == null) {
capacityProviderStrategy = new com.amazonaws.internal.SdkInternalList();
}
return capacityProviderStrategy;
}
/**
*
* The capacity provider strategy that the deployment is using.
*
*
* @param capacityProviderStrategy
* The capacity provider strategy that the deployment is using.
*/
public void setCapacityProviderStrategy(java.util.Collection capacityProviderStrategy) {
if (capacityProviderStrategy == null) {
this.capacityProviderStrategy = null;
return;
}
this.capacityProviderStrategy = new com.amazonaws.internal.SdkInternalList(capacityProviderStrategy);
}
/**
*
* The capacity provider strategy that the deployment is using.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCapacityProviderStrategy(java.util.Collection)} or
* {@link #withCapacityProviderStrategy(java.util.Collection)} if you want to override the existing values.
*
*
* @param capacityProviderStrategy
* The capacity provider strategy that the deployment is using.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Deployment withCapacityProviderStrategy(CapacityProviderStrategyItem... capacityProviderStrategy) {
if (this.capacityProviderStrategy == null) {
setCapacityProviderStrategy(new com.amazonaws.internal.SdkInternalList(capacityProviderStrategy.length));
}
for (CapacityProviderStrategyItem ele : capacityProviderStrategy) {
this.capacityProviderStrategy.add(ele);
}
return this;
}
/**
*
* The capacity provider strategy that the deployment is using.
*
*
* @param capacityProviderStrategy
* The capacity provider strategy that the deployment is using.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Deployment withCapacityProviderStrategy(java.util.Collection capacityProviderStrategy) {
setCapacityProviderStrategy(capacityProviderStrategy);
return this;
}
/**
*
* The launch type the tasks in the service are using. For more information, see Amazon ECS Launch Types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* @param launchType
* The launch type the tasks in the service are using. For more information, see Amazon ECS Launch
* Types in the Amazon Elastic Container Service Developer Guide.
* @see LaunchType
*/
public void setLaunchType(String launchType) {
this.launchType = launchType;
}
/**
*
* The launch type the tasks in the service are using. For more information, see Amazon ECS Launch Types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* @return The launch type the tasks in the service are using. For more information, see Amazon ECS Launch
* Types in the Amazon Elastic Container Service Developer Guide.
* @see LaunchType
*/
public String getLaunchType() {
return this.launchType;
}
/**
*
* The launch type the tasks in the service are using. For more information, see Amazon ECS Launch Types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* @param launchType
* The launch type the tasks in the service are using. For more information, see Amazon ECS Launch
* Types in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LaunchType
*/
public Deployment withLaunchType(String launchType) {
setLaunchType(launchType);
return this;
}
/**
*
* The launch type the tasks in the service are using. For more information, see Amazon ECS Launch Types
* in the Amazon Elastic Container Service Developer Guide.
*
*
* @param launchType
* The launch type the tasks in the service are using. For more information, see Amazon ECS Launch
* Types in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LaunchType
*/
public Deployment withLaunchType(LaunchType launchType) {
this.launchType = launchType.toString();
return this;
}
/**
*
* The platform version that your tasks in the service run on. A platform version is only specified for tasks using
* the Fargate launch type. If one isn't specified, the LATEST
platform version is used. For more
* information, see Fargate Platform
* Versions in the Amazon Elastic Container Service Developer Guide.
*
*
* @param platformVersion
* The platform version that your tasks in the service run on. A platform version is only specified for tasks
* using the Fargate launch type. If one isn't specified, the LATEST
platform version is used.
* For more information, see Fargate Platform
* Versions in the Amazon Elastic Container Service Developer Guide.
*/
public void setPlatformVersion(String platformVersion) {
this.platformVersion = platformVersion;
}
/**
*
* The platform version that your tasks in the service run on. A platform version is only specified for tasks using
* the Fargate launch type. If one isn't specified, the LATEST
platform version is used. For more
* information, see Fargate Platform
* Versions in the Amazon Elastic Container Service Developer Guide.
*
*
* @return The platform version that your tasks in the service run on. A platform version is only specified for
* tasks using the Fargate launch type. If one isn't specified, the LATEST
platform version is
* used. For more information, see Fargate
* Platform Versions in the Amazon Elastic Container Service Developer Guide.
*/
public String getPlatformVersion() {
return this.platformVersion;
}
/**
*
* The platform version that your tasks in the service run on. A platform version is only specified for tasks using
* the Fargate launch type. If one isn't specified, the LATEST
platform version is used. For more
* information, see Fargate Platform
* Versions in the Amazon Elastic Container Service Developer Guide.
*
*
* @param platformVersion
* The platform version that your tasks in the service run on. A platform version is only specified for tasks
* using the Fargate launch type. If one isn't specified, the LATEST
platform version is used.
* For more information, see Fargate Platform
* Versions in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Deployment withPlatformVersion(String platformVersion) {
setPlatformVersion(platformVersion);
return this;
}
/**
*
* The operating system that your tasks in the service, or tasks are running on. A platform family is specified only
* for tasks using the Fargate launch type.
*
*
* All tasks that run as part of this service must use the same platformFamily
value as the service,
* for example, LINUX.
.
*
*
* @param platformFamily
* The operating system that your tasks in the service, or tasks are running on. A platform family is
* specified only for tasks using the Fargate launch type.
*
* All tasks that run as part of this service must use the same platformFamily
value as the
* service, for example, LINUX.
.
*/
public void setPlatformFamily(String platformFamily) {
this.platformFamily = platformFamily;
}
/**
*
* The operating system that your tasks in the service, or tasks are running on. A platform family is specified only
* for tasks using the Fargate launch type.
*
*
* All tasks that run as part of this service must use the same platformFamily
value as the service,
* for example, LINUX.
.
*
*
* @return The operating system that your tasks in the service, or tasks are running on. A platform family is
* specified only for tasks using the Fargate launch type.
*
* All tasks that run as part of this service must use the same platformFamily
value as the
* service, for example, LINUX.
.
*/
public String getPlatformFamily() {
return this.platformFamily;
}
/**
*
* The operating system that your tasks in the service, or tasks are running on. A platform family is specified only
* for tasks using the Fargate launch type.
*
*
* All tasks that run as part of this service must use the same platformFamily
value as the service,
* for example, LINUX.
.
*
*
* @param platformFamily
* The operating system that your tasks in the service, or tasks are running on. A platform family is
* specified only for tasks using the Fargate launch type.
*
* All tasks that run as part of this service must use the same platformFamily
value as the
* service, for example, LINUX.
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Deployment withPlatformFamily(String platformFamily) {
setPlatformFamily(platformFamily);
return this;
}
/**
*
* The VPC subnet and security group configuration for tasks that receive their own elastic network interface by
* using the awsvpc
networking mode.
*
*
* @param networkConfiguration
* The VPC subnet and security group configuration for tasks that receive their own elastic network interface
* by using the awsvpc
networking mode.
*/
public void setNetworkConfiguration(NetworkConfiguration networkConfiguration) {
this.networkConfiguration = networkConfiguration;
}
/**
*
* The VPC subnet and security group configuration for tasks that receive their own elastic network interface by
* using the awsvpc
networking mode.
*
*
* @return The VPC subnet and security group configuration for tasks that receive their own elastic network
* interface by using the awsvpc
networking mode.
*/
public NetworkConfiguration getNetworkConfiguration() {
return this.networkConfiguration;
}
/**
*
* The VPC subnet and security group configuration for tasks that receive their own elastic network interface by
* using the awsvpc
networking mode.
*
*
* @param networkConfiguration
* The VPC subnet and security group configuration for tasks that receive their own elastic network interface
* by using the awsvpc
networking mode.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Deployment withNetworkConfiguration(NetworkConfiguration networkConfiguration) {
setNetworkConfiguration(networkConfiguration);
return this;
}
/**
*
*
* The rolloutState
of a service is only returned for services that use the rolling update (
* ECS
) deployment type that aren't behind a Classic Load Balancer.
*
*
*
* The rollout state of the deployment. When a service deployment is started, it begins in an
* IN_PROGRESS
state. When the service reaches a steady state, the deployment transitions to a
* COMPLETED
state. If the service fails to reach a steady state and circuit breaker is turned on, the
* deployment transitions to a FAILED
state. A deployment in FAILED
state doesn't launch
* any new tasks. For more information, see DeploymentCircuitBreaker.
*
*
* @param rolloutState
*
* The rolloutState
of a service is only returned for services that use the rolling update (
* ECS
) deployment type that aren't behind a Classic Load Balancer.
*
*
*
* The rollout state of the deployment. When a service deployment is started, it begins in an
* IN_PROGRESS
state. When the service reaches a steady state, the deployment transitions to a
* COMPLETED
state. If the service fails to reach a steady state and circuit breaker is turned
* on, the deployment transitions to a FAILED
state. A deployment in FAILED
state
* doesn't launch any new tasks. For more information, see DeploymentCircuitBreaker.
* @see DeploymentRolloutState
*/
public void setRolloutState(String rolloutState) {
this.rolloutState = rolloutState;
}
/**
*
*
* The rolloutState
of a service is only returned for services that use the rolling update (
* ECS
) deployment type that aren't behind a Classic Load Balancer.
*
*
*
* The rollout state of the deployment. When a service deployment is started, it begins in an
* IN_PROGRESS
state. When the service reaches a steady state, the deployment transitions to a
* COMPLETED
state. If the service fails to reach a steady state and circuit breaker is turned on, the
* deployment transitions to a FAILED
state. A deployment in FAILED
state doesn't launch
* any new tasks. For more information, see DeploymentCircuitBreaker.
*
*
* @return
* The rolloutState
of a service is only returned for services that use the rolling update (
* ECS
) deployment type that aren't behind a Classic Load Balancer.
*
*
*
* The rollout state of the deployment. When a service deployment is started, it begins in an
* IN_PROGRESS
state. When the service reaches a steady state, the deployment transitions to a
* COMPLETED
state. If the service fails to reach a steady state and circuit breaker is turned
* on, the deployment transitions to a FAILED
state. A deployment in FAILED
state
* doesn't launch any new tasks. For more information, see DeploymentCircuitBreaker.
* @see DeploymentRolloutState
*/
public String getRolloutState() {
return this.rolloutState;
}
/**
*
*
* The rolloutState
of a service is only returned for services that use the rolling update (
* ECS
) deployment type that aren't behind a Classic Load Balancer.
*
*
*
* The rollout state of the deployment. When a service deployment is started, it begins in an
* IN_PROGRESS
state. When the service reaches a steady state, the deployment transitions to a
* COMPLETED
state. If the service fails to reach a steady state and circuit breaker is turned on, the
* deployment transitions to a FAILED
state. A deployment in FAILED
state doesn't launch
* any new tasks. For more information, see DeploymentCircuitBreaker.
*
*
* @param rolloutState
*
* The rolloutState
of a service is only returned for services that use the rolling update (
* ECS
) deployment type that aren't behind a Classic Load Balancer.
*
*
*
* The rollout state of the deployment. When a service deployment is started, it begins in an
* IN_PROGRESS
state. When the service reaches a steady state, the deployment transitions to a
* COMPLETED
state. If the service fails to reach a steady state and circuit breaker is turned
* on, the deployment transitions to a FAILED
state. A deployment in FAILED
state
* doesn't launch any new tasks. For more information, see DeploymentCircuitBreaker.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DeploymentRolloutState
*/
public Deployment withRolloutState(String rolloutState) {
setRolloutState(rolloutState);
return this;
}
/**
*
*
* The rolloutState
of a service is only returned for services that use the rolling update (
* ECS
) deployment type that aren't behind a Classic Load Balancer.
*
*
*
* The rollout state of the deployment. When a service deployment is started, it begins in an
* IN_PROGRESS
state. When the service reaches a steady state, the deployment transitions to a
* COMPLETED
state. If the service fails to reach a steady state and circuit breaker is turned on, the
* deployment transitions to a FAILED
state. A deployment in FAILED
state doesn't launch
* any new tasks. For more information, see DeploymentCircuitBreaker.
*
*
* @param rolloutState
*
* The rolloutState
of a service is only returned for services that use the rolling update (
* ECS
) deployment type that aren't behind a Classic Load Balancer.
*
*
*
* The rollout state of the deployment. When a service deployment is started, it begins in an
* IN_PROGRESS
state. When the service reaches a steady state, the deployment transitions to a
* COMPLETED
state. If the service fails to reach a steady state and circuit breaker is turned
* on, the deployment transitions to a FAILED
state. A deployment in FAILED
state
* doesn't launch any new tasks. For more information, see DeploymentCircuitBreaker.
* @return Returns a reference to this object so that method calls can be chained together.
* @see DeploymentRolloutState
*/
public Deployment withRolloutState(DeploymentRolloutState rolloutState) {
this.rolloutState = rolloutState.toString();
return this;
}
/**
*
* A description of the rollout state of a deployment.
*
*
* @param rolloutStateReason
* A description of the rollout state of a deployment.
*/
public void setRolloutStateReason(String rolloutStateReason) {
this.rolloutStateReason = rolloutStateReason;
}
/**
*
* A description of the rollout state of a deployment.
*
*
* @return A description of the rollout state of a deployment.
*/
public String getRolloutStateReason() {
return this.rolloutStateReason;
}
/**
*
* A description of the rollout state of a deployment.
*
*
* @param rolloutStateReason
* A description of the rollout state of a deployment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Deployment withRolloutStateReason(String rolloutStateReason) {
setRolloutStateReason(rolloutStateReason);
return this;
}
/**
*
* The details of the Service Connect configuration that's used by this deployment. Compare the configuration
* between multiple deployments when troubleshooting issues with new deployments.
*
*
* The configuration for this service to discover and connect to services, and be discovered by, and connected from,
* other services within a namespace.
*
*
* Tasks that run in a namespace can use short names to connect to services in the namespace. Tasks can connect to
* services across all of the clusters in the namespace. Tasks connect through a managed proxy container that
* collects logs and metrics for increased visibility. Only the tasks that Amazon ECS services create are supported
* with Service Connect. For more information, see Service Connect in
* the Amazon Elastic Container Service Developer Guide.
*
*
* @param serviceConnectConfiguration
* The details of the Service Connect configuration that's used by this deployment. Compare the configuration
* between multiple deployments when troubleshooting issues with new deployments.
*
* The configuration for this service to discover and connect to services, and be discovered by, and
* connected from, other services within a namespace.
*
*
* Tasks that run in a namespace can use short names to connect to services in the namespace. Tasks can
* connect to services across all of the clusters in the namespace. Tasks connect through a managed proxy
* container that collects logs and metrics for increased visibility. Only the tasks that Amazon ECS services
* create are supported with Service Connect. For more information, see Service
* Connect in the Amazon Elastic Container Service Developer Guide.
*/
public void setServiceConnectConfiguration(ServiceConnectConfiguration serviceConnectConfiguration) {
this.serviceConnectConfiguration = serviceConnectConfiguration;
}
/**
*
* The details of the Service Connect configuration that's used by this deployment. Compare the configuration
* between multiple deployments when troubleshooting issues with new deployments.
*
*
* The configuration for this service to discover and connect to services, and be discovered by, and connected from,
* other services within a namespace.
*
*
* Tasks that run in a namespace can use short names to connect to services in the namespace. Tasks can connect to
* services across all of the clusters in the namespace. Tasks connect through a managed proxy container that
* collects logs and metrics for increased visibility. Only the tasks that Amazon ECS services create are supported
* with Service Connect. For more information, see Service Connect in
* the Amazon Elastic Container Service Developer Guide.
*
*
* @return The details of the Service Connect configuration that's used by this deployment. Compare the
* configuration between multiple deployments when troubleshooting issues with new deployments.
*
* The configuration for this service to discover and connect to services, and be discovered by, and
* connected from, other services within a namespace.
*
*
* Tasks that run in a namespace can use short names to connect to services in the namespace. Tasks can
* connect to services across all of the clusters in the namespace. Tasks connect through a managed proxy
* container that collects logs and metrics for increased visibility. Only the tasks that Amazon ECS
* services create are supported with Service Connect. For more information, see Service
* Connect in the Amazon Elastic Container Service Developer Guide.
*/
public ServiceConnectConfiguration getServiceConnectConfiguration() {
return this.serviceConnectConfiguration;
}
/**
*
* The details of the Service Connect configuration that's used by this deployment. Compare the configuration
* between multiple deployments when troubleshooting issues with new deployments.
*
*
* The configuration for this service to discover and connect to services, and be discovered by, and connected from,
* other services within a namespace.
*
*
* Tasks that run in a namespace can use short names to connect to services in the namespace. Tasks can connect to
* services across all of the clusters in the namespace. Tasks connect through a managed proxy container that
* collects logs and metrics for increased visibility. Only the tasks that Amazon ECS services create are supported
* with Service Connect. For more information, see Service Connect in
* the Amazon Elastic Container Service Developer Guide.
*
*
* @param serviceConnectConfiguration
* The details of the Service Connect configuration that's used by this deployment. Compare the configuration
* between multiple deployments when troubleshooting issues with new deployments.
*
* The configuration for this service to discover and connect to services, and be discovered by, and
* connected from, other services within a namespace.
*
*
* Tasks that run in a namespace can use short names to connect to services in the namespace. Tasks can
* connect to services across all of the clusters in the namespace. Tasks connect through a managed proxy
* container that collects logs and metrics for increased visibility. Only the tasks that Amazon ECS services
* create are supported with Service Connect. For more information, see Service
* Connect in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Deployment withServiceConnectConfiguration(ServiceConnectConfiguration serviceConnectConfiguration) {
setServiceConnectConfiguration(serviceConnectConfiguration);
return this;
}
/**
*
* The list of Service Connect resources that are associated with this deployment. Each list entry maps a discovery
* name to a Cloud Map service name.
*
*
* @return The list of Service Connect resources that are associated with this deployment. Each list entry maps a
* discovery name to a Cloud Map service name.
*/
public java.util.List getServiceConnectResources() {
if (serviceConnectResources == null) {
serviceConnectResources = new com.amazonaws.internal.SdkInternalList();
}
return serviceConnectResources;
}
/**
*
* The list of Service Connect resources that are associated with this deployment. Each list entry maps a discovery
* name to a Cloud Map service name.
*
*
* @param serviceConnectResources
* The list of Service Connect resources that are associated with this deployment. Each list entry maps a
* discovery name to a Cloud Map service name.
*/
public void setServiceConnectResources(java.util.Collection serviceConnectResources) {
if (serviceConnectResources == null) {
this.serviceConnectResources = null;
return;
}
this.serviceConnectResources = new com.amazonaws.internal.SdkInternalList(serviceConnectResources);
}
/**
*
* The list of Service Connect resources that are associated with this deployment. Each list entry maps a discovery
* name to a Cloud Map service name.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setServiceConnectResources(java.util.Collection)} or
* {@link #withServiceConnectResources(java.util.Collection)} if you want to override the existing values.
*
*
* @param serviceConnectResources
* The list of Service Connect resources that are associated with this deployment. Each list entry maps a
* discovery name to a Cloud Map service name.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Deployment withServiceConnectResources(ServiceConnectServiceResource... serviceConnectResources) {
if (this.serviceConnectResources == null) {
setServiceConnectResources(new com.amazonaws.internal.SdkInternalList(serviceConnectResources.length));
}
for (ServiceConnectServiceResource ele : serviceConnectResources) {
this.serviceConnectResources.add(ele);
}
return this;
}
/**
*
* The list of Service Connect resources that are associated with this deployment. Each list entry maps a discovery
* name to a Cloud Map service name.
*
*
* @param serviceConnectResources
* The list of Service Connect resources that are associated with this deployment. Each list entry maps a
* discovery name to a Cloud Map service name.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Deployment withServiceConnectResources(java.util.Collection serviceConnectResources) {
setServiceConnectResources(serviceConnectResources);
return this;
}
/**
*
* The details of the volume that was configuredAtLaunch
. You can configure different settings like the
* size, throughput, volumeType, and ecryption in ServiceManagedEBSVolumeConfiguration. The name
of the volume must match the name
* from the task definition.
*
*
* @return The details of the volume that was configuredAtLaunch
. You can configure different settings
* like the size, throughput, volumeType, and ecryption in ServiceManagedEBSVolumeConfiguration. The name
of the volume must match the
* name
from the task definition.
*/
public java.util.List getVolumeConfigurations() {
if (volumeConfigurations == null) {
volumeConfigurations = new com.amazonaws.internal.SdkInternalList();
}
return volumeConfigurations;
}
/**
*
* The details of the volume that was configuredAtLaunch
. You can configure different settings like the
* size, throughput, volumeType, and ecryption in ServiceManagedEBSVolumeConfiguration. The name
of the volume must match the name
* from the task definition.
*
*
* @param volumeConfigurations
* The details of the volume that was configuredAtLaunch
. You can configure different settings
* like the size, throughput, volumeType, and ecryption in ServiceManagedEBSVolumeConfiguration. The name
of the volume must match the
* name
from the task definition.
*/
public void setVolumeConfigurations(java.util.Collection volumeConfigurations) {
if (volumeConfigurations == null) {
this.volumeConfigurations = null;
return;
}
this.volumeConfigurations = new com.amazonaws.internal.SdkInternalList(volumeConfigurations);
}
/**
*
* The details of the volume that was configuredAtLaunch
. You can configure different settings like the
* size, throughput, volumeType, and ecryption in ServiceManagedEBSVolumeConfiguration. The name
of the volume must match the name
* from the task definition.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setVolumeConfigurations(java.util.Collection)} or {@link #withVolumeConfigurations(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param volumeConfigurations
* The details of the volume that was configuredAtLaunch
. You can configure different settings
* like the size, throughput, volumeType, and ecryption in ServiceManagedEBSVolumeConfiguration. The name
of the volume must match the
* name
from the task definition.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Deployment withVolumeConfigurations(ServiceVolumeConfiguration... volumeConfigurations) {
if (this.volumeConfigurations == null) {
setVolumeConfigurations(new com.amazonaws.internal.SdkInternalList(volumeConfigurations.length));
}
for (ServiceVolumeConfiguration ele : volumeConfigurations) {
this.volumeConfigurations.add(ele);
}
return this;
}
/**
*
* The details of the volume that was configuredAtLaunch
. You can configure different settings like the
* size, throughput, volumeType, and ecryption in ServiceManagedEBSVolumeConfiguration. The name
of the volume must match the name
* from the task definition.
*
*
* @param volumeConfigurations
* The details of the volume that was configuredAtLaunch
. You can configure different settings
* like the size, throughput, volumeType, and ecryption in ServiceManagedEBSVolumeConfiguration. The name
of the volume must match the
* name
from the task definition.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Deployment withVolumeConfigurations(java.util.Collection volumeConfigurations) {
setVolumeConfigurations(volumeConfigurations);
return this;
}
/**
*
* The Fargate ephemeral storage settings for the deployment.
*
*
* @param fargateEphemeralStorage
* The Fargate ephemeral storage settings for the deployment.
*/
public void setFargateEphemeralStorage(DeploymentEphemeralStorage fargateEphemeralStorage) {
this.fargateEphemeralStorage = fargateEphemeralStorage;
}
/**
*
* The Fargate ephemeral storage settings for the deployment.
*
*
* @return The Fargate ephemeral storage settings for the deployment.
*/
public DeploymentEphemeralStorage getFargateEphemeralStorage() {
return this.fargateEphemeralStorage;
}
/**
*
* The Fargate ephemeral storage settings for the deployment.
*
*
* @param fargateEphemeralStorage
* The Fargate ephemeral storage settings for the deployment.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Deployment withFargateEphemeralStorage(DeploymentEphemeralStorage fargateEphemeralStorage) {
setFargateEphemeralStorage(fargateEphemeralStorage);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getId() != null)
sb.append("Id: ").append(getId()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getTaskDefinition() != null)
sb.append("TaskDefinition: ").append(getTaskDefinition()).append(",");
if (getDesiredCount() != null)
sb.append("DesiredCount: ").append(getDesiredCount()).append(",");
if (getPendingCount() != null)
sb.append("PendingCount: ").append(getPendingCount()).append(",");
if (getRunningCount() != null)
sb.append("RunningCount: ").append(getRunningCount()).append(",");
if (getFailedTasks() != null)
sb.append("FailedTasks: ").append(getFailedTasks()).append(",");
if (getCreatedAt() != null)
sb.append("CreatedAt: ").append(getCreatedAt()).append(",");
if (getUpdatedAt() != null)
sb.append("UpdatedAt: ").append(getUpdatedAt()).append(",");
if (getCapacityProviderStrategy() != null)
sb.append("CapacityProviderStrategy: ").append(getCapacityProviderStrategy()).append(",");
if (getLaunchType() != null)
sb.append("LaunchType: ").append(getLaunchType()).append(",");
if (getPlatformVersion() != null)
sb.append("PlatformVersion: ").append(getPlatformVersion()).append(",");
if (getPlatformFamily() != null)
sb.append("PlatformFamily: ").append(getPlatformFamily()).append(",");
if (getNetworkConfiguration() != null)
sb.append("NetworkConfiguration: ").append(getNetworkConfiguration()).append(",");
if (getRolloutState() != null)
sb.append("RolloutState: ").append(getRolloutState()).append(",");
if (getRolloutStateReason() != null)
sb.append("RolloutStateReason: ").append(getRolloutStateReason()).append(",");
if (getServiceConnectConfiguration() != null)
sb.append("ServiceConnectConfiguration: ").append(getServiceConnectConfiguration()).append(",");
if (getServiceConnectResources() != null)
sb.append("ServiceConnectResources: ").append(getServiceConnectResources()).append(",");
if (getVolumeConfigurations() != null)
sb.append("VolumeConfigurations: ").append(getVolumeConfigurations()).append(",");
if (getFargateEphemeralStorage() != null)
sb.append("FargateEphemeralStorage: ").append(getFargateEphemeralStorage());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Deployment == false)
return false;
Deployment other = (Deployment) obj;
if (other.getId() == null ^ this.getId() == null)
return false;
if (other.getId() != null && other.getId().equals(this.getId()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getTaskDefinition() == null ^ this.getTaskDefinition() == null)
return false;
if (other.getTaskDefinition() != null && other.getTaskDefinition().equals(this.getTaskDefinition()) == false)
return false;
if (other.getDesiredCount() == null ^ this.getDesiredCount() == null)
return false;
if (other.getDesiredCount() != null && other.getDesiredCount().equals(this.getDesiredCount()) == false)
return false;
if (other.getPendingCount() == null ^ this.getPendingCount() == null)
return false;
if (other.getPendingCount() != null && other.getPendingCount().equals(this.getPendingCount()) == false)
return false;
if (other.getRunningCount() == null ^ this.getRunningCount() == null)
return false;
if (other.getRunningCount() != null && other.getRunningCount().equals(this.getRunningCount()) == false)
return false;
if (other.getFailedTasks() == null ^ this.getFailedTasks() == null)
return false;
if (other.getFailedTasks() != null && other.getFailedTasks().equals(this.getFailedTasks()) == false)
return false;
if (other.getCreatedAt() == null ^ this.getCreatedAt() == null)
return false;
if (other.getCreatedAt() != null && other.getCreatedAt().equals(this.getCreatedAt()) == false)
return false;
if (other.getUpdatedAt() == null ^ this.getUpdatedAt() == null)
return false;
if (other.getUpdatedAt() != null && other.getUpdatedAt().equals(this.getUpdatedAt()) == false)
return false;
if (other.getCapacityProviderStrategy() == null ^ this.getCapacityProviderStrategy() == null)
return false;
if (other.getCapacityProviderStrategy() != null && other.getCapacityProviderStrategy().equals(this.getCapacityProviderStrategy()) == false)
return false;
if (other.getLaunchType() == null ^ this.getLaunchType() == null)
return false;
if (other.getLaunchType() != null && other.getLaunchType().equals(this.getLaunchType()) == false)
return false;
if (other.getPlatformVersion() == null ^ this.getPlatformVersion() == null)
return false;
if (other.getPlatformVersion() != null && other.getPlatformVersion().equals(this.getPlatformVersion()) == false)
return false;
if (other.getPlatformFamily() == null ^ this.getPlatformFamily() == null)
return false;
if (other.getPlatformFamily() != null && other.getPlatformFamily().equals(this.getPlatformFamily()) == false)
return false;
if (other.getNetworkConfiguration() == null ^ this.getNetworkConfiguration() == null)
return false;
if (other.getNetworkConfiguration() != null && other.getNetworkConfiguration().equals(this.getNetworkConfiguration()) == false)
return false;
if (other.getRolloutState() == null ^ this.getRolloutState() == null)
return false;
if (other.getRolloutState() != null && other.getRolloutState().equals(this.getRolloutState()) == false)
return false;
if (other.getRolloutStateReason() == null ^ this.getRolloutStateReason() == null)
return false;
if (other.getRolloutStateReason() != null && other.getRolloutStateReason().equals(this.getRolloutStateReason()) == false)
return false;
if (other.getServiceConnectConfiguration() == null ^ this.getServiceConnectConfiguration() == null)
return false;
if (other.getServiceConnectConfiguration() != null && other.getServiceConnectConfiguration().equals(this.getServiceConnectConfiguration()) == false)
return false;
if (other.getServiceConnectResources() == null ^ this.getServiceConnectResources() == null)
return false;
if (other.getServiceConnectResources() != null && other.getServiceConnectResources().equals(this.getServiceConnectResources()) == false)
return false;
if (other.getVolumeConfigurations() == null ^ this.getVolumeConfigurations() == null)
return false;
if (other.getVolumeConfigurations() != null && other.getVolumeConfigurations().equals(this.getVolumeConfigurations()) == false)
return false;
if (other.getFargateEphemeralStorage() == null ^ this.getFargateEphemeralStorage() == null)
return false;
if (other.getFargateEphemeralStorage() != null && other.getFargateEphemeralStorage().equals(this.getFargateEphemeralStorage()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getId() == null) ? 0 : getId().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getTaskDefinition() == null) ? 0 : getTaskDefinition().hashCode());
hashCode = prime * hashCode + ((getDesiredCount() == null) ? 0 : getDesiredCount().hashCode());
hashCode = prime * hashCode + ((getPendingCount() == null) ? 0 : getPendingCount().hashCode());
hashCode = prime * hashCode + ((getRunningCount() == null) ? 0 : getRunningCount().hashCode());
hashCode = prime * hashCode + ((getFailedTasks() == null) ? 0 : getFailedTasks().hashCode());
hashCode = prime * hashCode + ((getCreatedAt() == null) ? 0 : getCreatedAt().hashCode());
hashCode = prime * hashCode + ((getUpdatedAt() == null) ? 0 : getUpdatedAt().hashCode());
hashCode = prime * hashCode + ((getCapacityProviderStrategy() == null) ? 0 : getCapacityProviderStrategy().hashCode());
hashCode = prime * hashCode + ((getLaunchType() == null) ? 0 : getLaunchType().hashCode());
hashCode = prime * hashCode + ((getPlatformVersion() == null) ? 0 : getPlatformVersion().hashCode());
hashCode = prime * hashCode + ((getPlatformFamily() == null) ? 0 : getPlatformFamily().hashCode());
hashCode = prime * hashCode + ((getNetworkConfiguration() == null) ? 0 : getNetworkConfiguration().hashCode());
hashCode = prime * hashCode + ((getRolloutState() == null) ? 0 : getRolloutState().hashCode());
hashCode = prime * hashCode + ((getRolloutStateReason() == null) ? 0 : getRolloutStateReason().hashCode());
hashCode = prime * hashCode + ((getServiceConnectConfiguration() == null) ? 0 : getServiceConnectConfiguration().hashCode());
hashCode = prime * hashCode + ((getServiceConnectResources() == null) ? 0 : getServiceConnectResources().hashCode());
hashCode = prime * hashCode + ((getVolumeConfigurations() == null) ? 0 : getVolumeConfigurations().hashCode());
hashCode = prime * hashCode + ((getFargateEphemeralStorage() == null) ? 0 : getFargateEphemeralStorage().hashCode());
return hashCode;
}
@Override
public Deployment clone() {
try {
return (Deployment) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.ecs.model.transform.DeploymentMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}