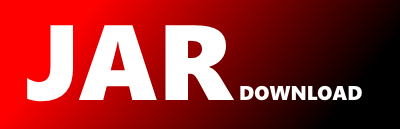
com.amazonaws.services.ecs.model.Service Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ecs Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ecs.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Details on a service within a cluster.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Service implements Serializable, Cloneable, StructuredPojo {
/**
*
* The ARN that identifies the service. For more information about the ARN format, see Amazon Resource Name (ARN) in the Amazon ECS Developer Guide.
*
*/
private String serviceArn;
/**
*
* The name of your service. Up to 255 letters (uppercase and lowercase), numbers, underscores, and hyphens are
* allowed. Service names must be unique within a cluster. However, you can have similarly named services in
* multiple clusters within a Region or across multiple Regions.
*
*/
private String serviceName;
/**
*
* The Amazon Resource Name (ARN) of the cluster that hosts the service.
*
*/
private String clusterArn;
/**
*
* A list of Elastic Load Balancing load balancer objects. It contains the load balancer name, the container name,
* and the container port to access from the load balancer. The container name is as it appears in a container
* definition.
*
*/
private com.amazonaws.internal.SdkInternalList loadBalancers;
/**
*
* The details for the service discovery registries to assign to this service. For more information, see Service Discovery.
*
*/
private com.amazonaws.internal.SdkInternalList serviceRegistries;
/**
*
* The status of the service. The valid values are ACTIVE
, DRAINING
, or
* INACTIVE
.
*
*/
private String status;
/**
*
* The desired number of instantiations of the task definition to keep running on the service. This value is
* specified when the service is created with CreateService, and it can be modified with
* UpdateService.
*
*/
private Integer desiredCount;
/**
*
* The number of tasks in the cluster that are in the RUNNING
state.
*
*/
private Integer runningCount;
/**
*
* The number of tasks in the cluster that are in the PENDING
state.
*
*/
private Integer pendingCount;
/**
*
* The launch type the service is using. When using the DescribeServices API, this field is omitted if the service
* was created using a capacity provider strategy.
*
*/
private String launchType;
/**
*
* The capacity provider strategy the service uses. When using the DescribeServices API, this field is omitted if
* the service was created using a launch type.
*
*/
private com.amazonaws.internal.SdkInternalList capacityProviderStrategy;
/**
*
* The platform version to run your service on. A platform version is only specified for tasks that are hosted on
* Fargate. If one isn't specified, the LATEST
platform version is used. For more information, see Fargate Platform
* Versions in the Amazon Elastic Container Service Developer Guide.
*
*/
private String platformVersion;
/**
*
* The operating system that your tasks in the service run on. A platform family is specified only for tasks using
* the Fargate launch type.
*
*
* All tasks that run as part of this service must use the same platformFamily
value as the service
* (for example, LINUX
).
*
*/
private String platformFamily;
/**
*
* The task definition to use for tasks in the service. This value is specified when the service is created with
* CreateService, and it can be modified with UpdateService.
*
*/
private String taskDefinition;
/**
*
* Optional deployment parameters that control how many tasks run during the deployment and the ordering of stopping
* and starting tasks.
*
*/
private DeploymentConfiguration deploymentConfiguration;
/**
*
* Information about a set of Amazon ECS tasks in either an CodeDeploy or an EXTERNAL
deployment. An
* Amazon ECS task set includes details such as the desired number of tasks, how many tasks are running, and whether
* the task set serves production traffic.
*
*/
private com.amazonaws.internal.SdkInternalList taskSets;
/**
*
* The current state of deployments for the service.
*
*/
private com.amazonaws.internal.SdkInternalList deployments;
/**
*
* The ARN of the IAM role that's associated with the service. It allows the Amazon ECS container agent to register
* container instances with an Elastic Load Balancing load balancer.
*
*/
private String roleArn;
/**
*
* The event stream for your service. A maximum of 100 of the latest events are displayed.
*
*/
private com.amazonaws.internal.SdkInternalList events;
/**
*
* The Unix timestamp for the time when the service was created.
*
*/
private java.util.Date createdAt;
/**
*
* The placement constraints for the tasks in the service.
*
*/
private com.amazonaws.internal.SdkInternalList placementConstraints;
/**
*
* The placement strategy that determines how tasks for the service are placed.
*
*/
private com.amazonaws.internal.SdkInternalList placementStrategy;
/**
*
* The VPC subnet and security group configuration for tasks that receive their own elastic network interface by
* using the awsvpc
networking mode.
*
*/
private NetworkConfiguration networkConfiguration;
/**
*
* The period of time, in seconds, that the Amazon ECS service scheduler ignores unhealthy Elastic Load Balancing
* target health checks after a task has first started.
*
*/
private Integer healthCheckGracePeriodSeconds;
/**
*
* The scheduling strategy to use for the service. For more information, see Services.
*
*
* There are two service scheduler strategies available.
*
*
* -
*
* REPLICA
-The replica scheduling strategy places and maintains the desired number of tasks across your
* cluster. By default, the service scheduler spreads tasks across Availability Zones. You can use task placement
* strategies and constraints to customize task placement decisions.
*
*
* -
*
* DAEMON
-The daemon scheduling strategy deploys exactly one task on each active container instance.
* This task meets all of the task placement constraints that you specify in your cluster. The service scheduler
* also evaluates the task placement constraints for running tasks. It stop tasks that don't meet the placement
* constraints.
*
*
*
* Fargate tasks don't support the DAEMON
scheduling strategy.
*
*
*
*/
private String schedulingStrategy;
/**
*
* The deployment controller type the service is using.
*
*/
private DeploymentController deploymentController;
/**
*
* The metadata that you apply to the service to help you categorize and organize them. Each tag consists of a key
* and an optional value. You define bot the key and value.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* either keys or values as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys or values
* with this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*
*/
private com.amazonaws.internal.SdkInternalList tags;
/**
*
* The principal that created the service.
*
*/
private String createdBy;
/**
*
* Determines whether to use Amazon ECS managed tags for the tasks in the service. For more information, see Tagging Your Amazon ECS
* Resources in the Amazon Elastic Container Service Developer Guide.
*
*/
private Boolean enableECSManagedTags;
/**
*
* Determines whether to propagate the tags from the task definition or the service to the task. If no value is
* specified, the tags aren't propagated.
*
*/
private String propagateTags;
/**
*
* Determines whether the execute command functionality is turned on for the service. If true
, the
* execute command functionality is turned on for all containers in tasks as part of the service.
*
*/
private Boolean enableExecuteCommand;
/**
*
* The ARN that identifies the service. For more information about the ARN format, see Amazon Resource Name (ARN) in the Amazon ECS Developer Guide.
*
*
* @param serviceArn
* The ARN that identifies the service. For more information about the ARN format, see Amazon Resource Name (ARN) in the Amazon ECS Developer Guide.
*/
public void setServiceArn(String serviceArn) {
this.serviceArn = serviceArn;
}
/**
*
* The ARN that identifies the service. For more information about the ARN format, see Amazon Resource Name (ARN) in the Amazon ECS Developer Guide.
*
*
* @return The ARN that identifies the service. For more information about the ARN format, see Amazon Resource Name (ARN) in the Amazon ECS Developer Guide.
*/
public String getServiceArn() {
return this.serviceArn;
}
/**
*
* The ARN that identifies the service. For more information about the ARN format, see Amazon Resource Name (ARN) in the Amazon ECS Developer Guide.
*
*
* @param serviceArn
* The ARN that identifies the service. For more information about the ARN format, see Amazon Resource Name (ARN) in the Amazon ECS Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withServiceArn(String serviceArn) {
setServiceArn(serviceArn);
return this;
}
/**
*
* The name of your service. Up to 255 letters (uppercase and lowercase), numbers, underscores, and hyphens are
* allowed. Service names must be unique within a cluster. However, you can have similarly named services in
* multiple clusters within a Region or across multiple Regions.
*
*
* @param serviceName
* The name of your service. Up to 255 letters (uppercase and lowercase), numbers, underscores, and hyphens
* are allowed. Service names must be unique within a cluster. However, you can have similarly named services
* in multiple clusters within a Region or across multiple Regions.
*/
public void setServiceName(String serviceName) {
this.serviceName = serviceName;
}
/**
*
* The name of your service. Up to 255 letters (uppercase and lowercase), numbers, underscores, and hyphens are
* allowed. Service names must be unique within a cluster. However, you can have similarly named services in
* multiple clusters within a Region or across multiple Regions.
*
*
* @return The name of your service. Up to 255 letters (uppercase and lowercase), numbers, underscores, and hyphens
* are allowed. Service names must be unique within a cluster. However, you can have similarly named
* services in multiple clusters within a Region or across multiple Regions.
*/
public String getServiceName() {
return this.serviceName;
}
/**
*
* The name of your service. Up to 255 letters (uppercase and lowercase), numbers, underscores, and hyphens are
* allowed. Service names must be unique within a cluster. However, you can have similarly named services in
* multiple clusters within a Region or across multiple Regions.
*
*
* @param serviceName
* The name of your service. Up to 255 letters (uppercase and lowercase), numbers, underscores, and hyphens
* are allowed. Service names must be unique within a cluster. However, you can have similarly named services
* in multiple clusters within a Region or across multiple Regions.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withServiceName(String serviceName) {
setServiceName(serviceName);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the cluster that hosts the service.
*
*
* @param clusterArn
* The Amazon Resource Name (ARN) of the cluster that hosts the service.
*/
public void setClusterArn(String clusterArn) {
this.clusterArn = clusterArn;
}
/**
*
* The Amazon Resource Name (ARN) of the cluster that hosts the service.
*
*
* @return The Amazon Resource Name (ARN) of the cluster that hosts the service.
*/
public String getClusterArn() {
return this.clusterArn;
}
/**
*
* The Amazon Resource Name (ARN) of the cluster that hosts the service.
*
*
* @param clusterArn
* The Amazon Resource Name (ARN) of the cluster that hosts the service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withClusterArn(String clusterArn) {
setClusterArn(clusterArn);
return this;
}
/**
*
* A list of Elastic Load Balancing load balancer objects. It contains the load balancer name, the container name,
* and the container port to access from the load balancer. The container name is as it appears in a container
* definition.
*
*
* @return A list of Elastic Load Balancing load balancer objects. It contains the load balancer name, the container
* name, and the container port to access from the load balancer. The container name is as it appears in a
* container definition.
*/
public java.util.List getLoadBalancers() {
if (loadBalancers == null) {
loadBalancers = new com.amazonaws.internal.SdkInternalList();
}
return loadBalancers;
}
/**
*
* A list of Elastic Load Balancing load balancer objects. It contains the load balancer name, the container name,
* and the container port to access from the load balancer. The container name is as it appears in a container
* definition.
*
*
* @param loadBalancers
* A list of Elastic Load Balancing load balancer objects. It contains the load balancer name, the container
* name, and the container port to access from the load balancer. The container name is as it appears in a
* container definition.
*/
public void setLoadBalancers(java.util.Collection loadBalancers) {
if (loadBalancers == null) {
this.loadBalancers = null;
return;
}
this.loadBalancers = new com.amazonaws.internal.SdkInternalList(loadBalancers);
}
/**
*
* A list of Elastic Load Balancing load balancer objects. It contains the load balancer name, the container name,
* and the container port to access from the load balancer. The container name is as it appears in a container
* definition.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setLoadBalancers(java.util.Collection)} or {@link #withLoadBalancers(java.util.Collection)} if you want
* to override the existing values.
*
*
* @param loadBalancers
* A list of Elastic Load Balancing load balancer objects. It contains the load balancer name, the container
* name, and the container port to access from the load balancer. The container name is as it appears in a
* container definition.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withLoadBalancers(LoadBalancer... loadBalancers) {
if (this.loadBalancers == null) {
setLoadBalancers(new com.amazonaws.internal.SdkInternalList(loadBalancers.length));
}
for (LoadBalancer ele : loadBalancers) {
this.loadBalancers.add(ele);
}
return this;
}
/**
*
* A list of Elastic Load Balancing load balancer objects. It contains the load balancer name, the container name,
* and the container port to access from the load balancer. The container name is as it appears in a container
* definition.
*
*
* @param loadBalancers
* A list of Elastic Load Balancing load balancer objects. It contains the load balancer name, the container
* name, and the container port to access from the load balancer. The container name is as it appears in a
* container definition.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withLoadBalancers(java.util.Collection loadBalancers) {
setLoadBalancers(loadBalancers);
return this;
}
/**
*
* The details for the service discovery registries to assign to this service. For more information, see Service Discovery.
*
*
* @return The details for the service discovery registries to assign to this service. For more information, see Service
* Discovery.
*/
public java.util.List getServiceRegistries() {
if (serviceRegistries == null) {
serviceRegistries = new com.amazonaws.internal.SdkInternalList();
}
return serviceRegistries;
}
/**
*
* The details for the service discovery registries to assign to this service. For more information, see Service Discovery.
*
*
* @param serviceRegistries
* The details for the service discovery registries to assign to this service. For more information, see Service
* Discovery.
*/
public void setServiceRegistries(java.util.Collection serviceRegistries) {
if (serviceRegistries == null) {
this.serviceRegistries = null;
return;
}
this.serviceRegistries = new com.amazonaws.internal.SdkInternalList(serviceRegistries);
}
/**
*
* The details for the service discovery registries to assign to this service. For more information, see Service Discovery.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setServiceRegistries(java.util.Collection)} or {@link #withServiceRegistries(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param serviceRegistries
* The details for the service discovery registries to assign to this service. For more information, see Service
* Discovery.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withServiceRegistries(ServiceRegistry... serviceRegistries) {
if (this.serviceRegistries == null) {
setServiceRegistries(new com.amazonaws.internal.SdkInternalList(serviceRegistries.length));
}
for (ServiceRegistry ele : serviceRegistries) {
this.serviceRegistries.add(ele);
}
return this;
}
/**
*
* The details for the service discovery registries to assign to this service. For more information, see Service Discovery.
*
*
* @param serviceRegistries
* The details for the service discovery registries to assign to this service. For more information, see Service
* Discovery.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withServiceRegistries(java.util.Collection serviceRegistries) {
setServiceRegistries(serviceRegistries);
return this;
}
/**
*
* The status of the service. The valid values are ACTIVE
, DRAINING
, or
* INACTIVE
.
*
*
* @param status
* The status of the service. The valid values are ACTIVE
, DRAINING
, or
* INACTIVE
.
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the service. The valid values are ACTIVE
, DRAINING
, or
* INACTIVE
.
*
*
* @return The status of the service. The valid values are ACTIVE
, DRAINING
, or
* INACTIVE
.
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of the service. The valid values are ACTIVE
, DRAINING
, or
* INACTIVE
.
*
*
* @param status
* The status of the service. The valid values are ACTIVE
, DRAINING
, or
* INACTIVE
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The desired number of instantiations of the task definition to keep running on the service. This value is
* specified when the service is created with CreateService, and it can be modified with
* UpdateService.
*
*
* @param desiredCount
* The desired number of instantiations of the task definition to keep running on the service. This value is
* specified when the service is created with CreateService, and it can be modified with
* UpdateService.
*/
public void setDesiredCount(Integer desiredCount) {
this.desiredCount = desiredCount;
}
/**
*
* The desired number of instantiations of the task definition to keep running on the service. This value is
* specified when the service is created with CreateService, and it can be modified with
* UpdateService.
*
*
* @return The desired number of instantiations of the task definition to keep running on the service. This value is
* specified when the service is created with CreateService, and it can be modified with
* UpdateService.
*/
public Integer getDesiredCount() {
return this.desiredCount;
}
/**
*
* The desired number of instantiations of the task definition to keep running on the service. This value is
* specified when the service is created with CreateService, and it can be modified with
* UpdateService.
*
*
* @param desiredCount
* The desired number of instantiations of the task definition to keep running on the service. This value is
* specified when the service is created with CreateService, and it can be modified with
* UpdateService.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withDesiredCount(Integer desiredCount) {
setDesiredCount(desiredCount);
return this;
}
/**
*
* The number of tasks in the cluster that are in the RUNNING
state.
*
*
* @param runningCount
* The number of tasks in the cluster that are in the RUNNING
state.
*/
public void setRunningCount(Integer runningCount) {
this.runningCount = runningCount;
}
/**
*
* The number of tasks in the cluster that are in the RUNNING
state.
*
*
* @return The number of tasks in the cluster that are in the RUNNING
state.
*/
public Integer getRunningCount() {
return this.runningCount;
}
/**
*
* The number of tasks in the cluster that are in the RUNNING
state.
*
*
* @param runningCount
* The number of tasks in the cluster that are in the RUNNING
state.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withRunningCount(Integer runningCount) {
setRunningCount(runningCount);
return this;
}
/**
*
* The number of tasks in the cluster that are in the PENDING
state.
*
*
* @param pendingCount
* The number of tasks in the cluster that are in the PENDING
state.
*/
public void setPendingCount(Integer pendingCount) {
this.pendingCount = pendingCount;
}
/**
*
* The number of tasks in the cluster that are in the PENDING
state.
*
*
* @return The number of tasks in the cluster that are in the PENDING
state.
*/
public Integer getPendingCount() {
return this.pendingCount;
}
/**
*
* The number of tasks in the cluster that are in the PENDING
state.
*
*
* @param pendingCount
* The number of tasks in the cluster that are in the PENDING
state.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withPendingCount(Integer pendingCount) {
setPendingCount(pendingCount);
return this;
}
/**
*
* The launch type the service is using. When using the DescribeServices API, this field is omitted if the service
* was created using a capacity provider strategy.
*
*
* @param launchType
* The launch type the service is using. When using the DescribeServices API, this field is omitted if the
* service was created using a capacity provider strategy.
* @see LaunchType
*/
public void setLaunchType(String launchType) {
this.launchType = launchType;
}
/**
*
* The launch type the service is using. When using the DescribeServices API, this field is omitted if the service
* was created using a capacity provider strategy.
*
*
* @return The launch type the service is using. When using the DescribeServices API, this field is omitted if the
* service was created using a capacity provider strategy.
* @see LaunchType
*/
public String getLaunchType() {
return this.launchType;
}
/**
*
* The launch type the service is using. When using the DescribeServices API, this field is omitted if the service
* was created using a capacity provider strategy.
*
*
* @param launchType
* The launch type the service is using. When using the DescribeServices API, this field is omitted if the
* service was created using a capacity provider strategy.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LaunchType
*/
public Service withLaunchType(String launchType) {
setLaunchType(launchType);
return this;
}
/**
*
* The launch type the service is using. When using the DescribeServices API, this field is omitted if the service
* was created using a capacity provider strategy.
*
*
* @param launchType
* The launch type the service is using. When using the DescribeServices API, this field is omitted if the
* service was created using a capacity provider strategy.
* @return Returns a reference to this object so that method calls can be chained together.
* @see LaunchType
*/
public Service withLaunchType(LaunchType launchType) {
this.launchType = launchType.toString();
return this;
}
/**
*
* The capacity provider strategy the service uses. When using the DescribeServices API, this field is omitted if
* the service was created using a launch type.
*
*
* @return The capacity provider strategy the service uses. When using the DescribeServices API, this field is
* omitted if the service was created using a launch type.
*/
public java.util.List getCapacityProviderStrategy() {
if (capacityProviderStrategy == null) {
capacityProviderStrategy = new com.amazonaws.internal.SdkInternalList();
}
return capacityProviderStrategy;
}
/**
*
* The capacity provider strategy the service uses. When using the DescribeServices API, this field is omitted if
* the service was created using a launch type.
*
*
* @param capacityProviderStrategy
* The capacity provider strategy the service uses. When using the DescribeServices API, this field is
* omitted if the service was created using a launch type.
*/
public void setCapacityProviderStrategy(java.util.Collection capacityProviderStrategy) {
if (capacityProviderStrategy == null) {
this.capacityProviderStrategy = null;
return;
}
this.capacityProviderStrategy = new com.amazonaws.internal.SdkInternalList(capacityProviderStrategy);
}
/**
*
* The capacity provider strategy the service uses. When using the DescribeServices API, this field is omitted if
* the service was created using a launch type.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCapacityProviderStrategy(java.util.Collection)} or
* {@link #withCapacityProviderStrategy(java.util.Collection)} if you want to override the existing values.
*
*
* @param capacityProviderStrategy
* The capacity provider strategy the service uses. When using the DescribeServices API, this field is
* omitted if the service was created using a launch type.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withCapacityProviderStrategy(CapacityProviderStrategyItem... capacityProviderStrategy) {
if (this.capacityProviderStrategy == null) {
setCapacityProviderStrategy(new com.amazonaws.internal.SdkInternalList(capacityProviderStrategy.length));
}
for (CapacityProviderStrategyItem ele : capacityProviderStrategy) {
this.capacityProviderStrategy.add(ele);
}
return this;
}
/**
*
* The capacity provider strategy the service uses. When using the DescribeServices API, this field is omitted if
* the service was created using a launch type.
*
*
* @param capacityProviderStrategy
* The capacity provider strategy the service uses. When using the DescribeServices API, this field is
* omitted if the service was created using a launch type.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withCapacityProviderStrategy(java.util.Collection capacityProviderStrategy) {
setCapacityProviderStrategy(capacityProviderStrategy);
return this;
}
/**
*
* The platform version to run your service on. A platform version is only specified for tasks that are hosted on
* Fargate. If one isn't specified, the LATEST
platform version is used. For more information, see Fargate Platform
* Versions in the Amazon Elastic Container Service Developer Guide.
*
*
* @param platformVersion
* The platform version to run your service on. A platform version is only specified for tasks that are
* hosted on Fargate. If one isn't specified, the LATEST
platform version is used. For more
* information, see Fargate Platform
* Versions in the Amazon Elastic Container Service Developer Guide.
*/
public void setPlatformVersion(String platformVersion) {
this.platformVersion = platformVersion;
}
/**
*
* The platform version to run your service on. A platform version is only specified for tasks that are hosted on
* Fargate. If one isn't specified, the LATEST
platform version is used. For more information, see Fargate Platform
* Versions in the Amazon Elastic Container Service Developer Guide.
*
*
* @return The platform version to run your service on. A platform version is only specified for tasks that are
* hosted on Fargate. If one isn't specified, the LATEST
platform version is used. For more
* information, see Fargate
* Platform Versions in the Amazon Elastic Container Service Developer Guide.
*/
public String getPlatformVersion() {
return this.platformVersion;
}
/**
*
* The platform version to run your service on. A platform version is only specified for tasks that are hosted on
* Fargate. If one isn't specified, the LATEST
platform version is used. For more information, see Fargate Platform
* Versions in the Amazon Elastic Container Service Developer Guide.
*
*
* @param platformVersion
* The platform version to run your service on. A platform version is only specified for tasks that are
* hosted on Fargate. If one isn't specified, the LATEST
platform version is used. For more
* information, see Fargate Platform
* Versions in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withPlatformVersion(String platformVersion) {
setPlatformVersion(platformVersion);
return this;
}
/**
*
* The operating system that your tasks in the service run on. A platform family is specified only for tasks using
* the Fargate launch type.
*
*
* All tasks that run as part of this service must use the same platformFamily
value as the service
* (for example, LINUX
).
*
*
* @param platformFamily
* The operating system that your tasks in the service run on. A platform family is specified only for tasks
* using the Fargate launch type.
*
* All tasks that run as part of this service must use the same platformFamily
value as the
* service (for example, LINUX
).
*/
public void setPlatformFamily(String platformFamily) {
this.platformFamily = platformFamily;
}
/**
*
* The operating system that your tasks in the service run on. A platform family is specified only for tasks using
* the Fargate launch type.
*
*
* All tasks that run as part of this service must use the same platformFamily
value as the service
* (for example, LINUX
).
*
*
* @return The operating system that your tasks in the service run on. A platform family is specified only for tasks
* using the Fargate launch type.
*
* All tasks that run as part of this service must use the same platformFamily
value as the
* service (for example, LINUX
).
*/
public String getPlatformFamily() {
return this.platformFamily;
}
/**
*
* The operating system that your tasks in the service run on. A platform family is specified only for tasks using
* the Fargate launch type.
*
*
* All tasks that run as part of this service must use the same platformFamily
value as the service
* (for example, LINUX
).
*
*
* @param platformFamily
* The operating system that your tasks in the service run on. A platform family is specified only for tasks
* using the Fargate launch type.
*
* All tasks that run as part of this service must use the same platformFamily
value as the
* service (for example, LINUX
).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withPlatformFamily(String platformFamily) {
setPlatformFamily(platformFamily);
return this;
}
/**
*
* The task definition to use for tasks in the service. This value is specified when the service is created with
* CreateService, and it can be modified with UpdateService.
*
*
* @param taskDefinition
* The task definition to use for tasks in the service. This value is specified when the service is created
* with CreateService, and it can be modified with UpdateService.
*/
public void setTaskDefinition(String taskDefinition) {
this.taskDefinition = taskDefinition;
}
/**
*
* The task definition to use for tasks in the service. This value is specified when the service is created with
* CreateService, and it can be modified with UpdateService.
*
*
* @return The task definition to use for tasks in the service. This value is specified when the service is created
* with CreateService, and it can be modified with UpdateService.
*/
public String getTaskDefinition() {
return this.taskDefinition;
}
/**
*
* The task definition to use for tasks in the service. This value is specified when the service is created with
* CreateService, and it can be modified with UpdateService.
*
*
* @param taskDefinition
* The task definition to use for tasks in the service. This value is specified when the service is created
* with CreateService, and it can be modified with UpdateService.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withTaskDefinition(String taskDefinition) {
setTaskDefinition(taskDefinition);
return this;
}
/**
*
* Optional deployment parameters that control how many tasks run during the deployment and the ordering of stopping
* and starting tasks.
*
*
* @param deploymentConfiguration
* Optional deployment parameters that control how many tasks run during the deployment and the ordering of
* stopping and starting tasks.
*/
public void setDeploymentConfiguration(DeploymentConfiguration deploymentConfiguration) {
this.deploymentConfiguration = deploymentConfiguration;
}
/**
*
* Optional deployment parameters that control how many tasks run during the deployment and the ordering of stopping
* and starting tasks.
*
*
* @return Optional deployment parameters that control how many tasks run during the deployment and the ordering of
* stopping and starting tasks.
*/
public DeploymentConfiguration getDeploymentConfiguration() {
return this.deploymentConfiguration;
}
/**
*
* Optional deployment parameters that control how many tasks run during the deployment and the ordering of stopping
* and starting tasks.
*
*
* @param deploymentConfiguration
* Optional deployment parameters that control how many tasks run during the deployment and the ordering of
* stopping and starting tasks.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withDeploymentConfiguration(DeploymentConfiguration deploymentConfiguration) {
setDeploymentConfiguration(deploymentConfiguration);
return this;
}
/**
*
* Information about a set of Amazon ECS tasks in either an CodeDeploy or an EXTERNAL
deployment. An
* Amazon ECS task set includes details such as the desired number of tasks, how many tasks are running, and whether
* the task set serves production traffic.
*
*
* @return Information about a set of Amazon ECS tasks in either an CodeDeploy or an EXTERNAL
* deployment. An Amazon ECS task set includes details such as the desired number of tasks, how many tasks
* are running, and whether the task set serves production traffic.
*/
public java.util.List getTaskSets() {
if (taskSets == null) {
taskSets = new com.amazonaws.internal.SdkInternalList();
}
return taskSets;
}
/**
*
* Information about a set of Amazon ECS tasks in either an CodeDeploy or an EXTERNAL
deployment. An
* Amazon ECS task set includes details such as the desired number of tasks, how many tasks are running, and whether
* the task set serves production traffic.
*
*
* @param taskSets
* Information about a set of Amazon ECS tasks in either an CodeDeploy or an EXTERNAL
* deployment. An Amazon ECS task set includes details such as the desired number of tasks, how many tasks
* are running, and whether the task set serves production traffic.
*/
public void setTaskSets(java.util.Collection taskSets) {
if (taskSets == null) {
this.taskSets = null;
return;
}
this.taskSets = new com.amazonaws.internal.SdkInternalList(taskSets);
}
/**
*
* Information about a set of Amazon ECS tasks in either an CodeDeploy or an EXTERNAL
deployment. An
* Amazon ECS task set includes details such as the desired number of tasks, how many tasks are running, and whether
* the task set serves production traffic.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTaskSets(java.util.Collection)} or {@link #withTaskSets(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param taskSets
* Information about a set of Amazon ECS tasks in either an CodeDeploy or an EXTERNAL
* deployment. An Amazon ECS task set includes details such as the desired number of tasks, how many tasks
* are running, and whether the task set serves production traffic.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withTaskSets(TaskSet... taskSets) {
if (this.taskSets == null) {
setTaskSets(new com.amazonaws.internal.SdkInternalList(taskSets.length));
}
for (TaskSet ele : taskSets) {
this.taskSets.add(ele);
}
return this;
}
/**
*
* Information about a set of Amazon ECS tasks in either an CodeDeploy or an EXTERNAL
deployment. An
* Amazon ECS task set includes details such as the desired number of tasks, how many tasks are running, and whether
* the task set serves production traffic.
*
*
* @param taskSets
* Information about a set of Amazon ECS tasks in either an CodeDeploy or an EXTERNAL
* deployment. An Amazon ECS task set includes details such as the desired number of tasks, how many tasks
* are running, and whether the task set serves production traffic.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withTaskSets(java.util.Collection taskSets) {
setTaskSets(taskSets);
return this;
}
/**
*
* The current state of deployments for the service.
*
*
* @return The current state of deployments for the service.
*/
public java.util.List getDeployments() {
if (deployments == null) {
deployments = new com.amazonaws.internal.SdkInternalList();
}
return deployments;
}
/**
*
* The current state of deployments for the service.
*
*
* @param deployments
* The current state of deployments for the service.
*/
public void setDeployments(java.util.Collection deployments) {
if (deployments == null) {
this.deployments = null;
return;
}
this.deployments = new com.amazonaws.internal.SdkInternalList(deployments);
}
/**
*
* The current state of deployments for the service.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDeployments(java.util.Collection)} or {@link #withDeployments(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param deployments
* The current state of deployments for the service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withDeployments(Deployment... deployments) {
if (this.deployments == null) {
setDeployments(new com.amazonaws.internal.SdkInternalList(deployments.length));
}
for (Deployment ele : deployments) {
this.deployments.add(ele);
}
return this;
}
/**
*
* The current state of deployments for the service.
*
*
* @param deployments
* The current state of deployments for the service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withDeployments(java.util.Collection deployments) {
setDeployments(deployments);
return this;
}
/**
*
* The ARN of the IAM role that's associated with the service. It allows the Amazon ECS container agent to register
* container instances with an Elastic Load Balancing load balancer.
*
*
* @param roleArn
* The ARN of the IAM role that's associated with the service. It allows the Amazon ECS container agent to
* register container instances with an Elastic Load Balancing load balancer.
*/
public void setRoleArn(String roleArn) {
this.roleArn = roleArn;
}
/**
*
* The ARN of the IAM role that's associated with the service. It allows the Amazon ECS container agent to register
* container instances with an Elastic Load Balancing load balancer.
*
*
* @return The ARN of the IAM role that's associated with the service. It allows the Amazon ECS container agent to
* register container instances with an Elastic Load Balancing load balancer.
*/
public String getRoleArn() {
return this.roleArn;
}
/**
*
* The ARN of the IAM role that's associated with the service. It allows the Amazon ECS container agent to register
* container instances with an Elastic Load Balancing load balancer.
*
*
* @param roleArn
* The ARN of the IAM role that's associated with the service. It allows the Amazon ECS container agent to
* register container instances with an Elastic Load Balancing load balancer.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withRoleArn(String roleArn) {
setRoleArn(roleArn);
return this;
}
/**
*
* The event stream for your service. A maximum of 100 of the latest events are displayed.
*
*
* @return The event stream for your service. A maximum of 100 of the latest events are displayed.
*/
public java.util.List getEvents() {
if (events == null) {
events = new com.amazonaws.internal.SdkInternalList();
}
return events;
}
/**
*
* The event stream for your service. A maximum of 100 of the latest events are displayed.
*
*
* @param events
* The event stream for your service. A maximum of 100 of the latest events are displayed.
*/
public void setEvents(java.util.Collection events) {
if (events == null) {
this.events = null;
return;
}
this.events = new com.amazonaws.internal.SdkInternalList(events);
}
/**
*
* The event stream for your service. A maximum of 100 of the latest events are displayed.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setEvents(java.util.Collection)} or {@link #withEvents(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param events
* The event stream for your service. A maximum of 100 of the latest events are displayed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withEvents(ServiceEvent... events) {
if (this.events == null) {
setEvents(new com.amazonaws.internal.SdkInternalList(events.length));
}
for (ServiceEvent ele : events) {
this.events.add(ele);
}
return this;
}
/**
*
* The event stream for your service. A maximum of 100 of the latest events are displayed.
*
*
* @param events
* The event stream for your service. A maximum of 100 of the latest events are displayed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withEvents(java.util.Collection events) {
setEvents(events);
return this;
}
/**
*
* The Unix timestamp for the time when the service was created.
*
*
* @param createdAt
* The Unix timestamp for the time when the service was created.
*/
public void setCreatedAt(java.util.Date createdAt) {
this.createdAt = createdAt;
}
/**
*
* The Unix timestamp for the time when the service was created.
*
*
* @return The Unix timestamp for the time when the service was created.
*/
public java.util.Date getCreatedAt() {
return this.createdAt;
}
/**
*
* The Unix timestamp for the time when the service was created.
*
*
* @param createdAt
* The Unix timestamp for the time when the service was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withCreatedAt(java.util.Date createdAt) {
setCreatedAt(createdAt);
return this;
}
/**
*
* The placement constraints for the tasks in the service.
*
*
* @return The placement constraints for the tasks in the service.
*/
public java.util.List getPlacementConstraints() {
if (placementConstraints == null) {
placementConstraints = new com.amazonaws.internal.SdkInternalList();
}
return placementConstraints;
}
/**
*
* The placement constraints for the tasks in the service.
*
*
* @param placementConstraints
* The placement constraints for the tasks in the service.
*/
public void setPlacementConstraints(java.util.Collection placementConstraints) {
if (placementConstraints == null) {
this.placementConstraints = null;
return;
}
this.placementConstraints = new com.amazonaws.internal.SdkInternalList(placementConstraints);
}
/**
*
* The placement constraints for the tasks in the service.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPlacementConstraints(java.util.Collection)} or {@link #withPlacementConstraints(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param placementConstraints
* The placement constraints for the tasks in the service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withPlacementConstraints(PlacementConstraint... placementConstraints) {
if (this.placementConstraints == null) {
setPlacementConstraints(new com.amazonaws.internal.SdkInternalList(placementConstraints.length));
}
for (PlacementConstraint ele : placementConstraints) {
this.placementConstraints.add(ele);
}
return this;
}
/**
*
* The placement constraints for the tasks in the service.
*
*
* @param placementConstraints
* The placement constraints for the tasks in the service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withPlacementConstraints(java.util.Collection placementConstraints) {
setPlacementConstraints(placementConstraints);
return this;
}
/**
*
* The placement strategy that determines how tasks for the service are placed.
*
*
* @return The placement strategy that determines how tasks for the service are placed.
*/
public java.util.List getPlacementStrategy() {
if (placementStrategy == null) {
placementStrategy = new com.amazonaws.internal.SdkInternalList();
}
return placementStrategy;
}
/**
*
* The placement strategy that determines how tasks for the service are placed.
*
*
* @param placementStrategy
* The placement strategy that determines how tasks for the service are placed.
*/
public void setPlacementStrategy(java.util.Collection placementStrategy) {
if (placementStrategy == null) {
this.placementStrategy = null;
return;
}
this.placementStrategy = new com.amazonaws.internal.SdkInternalList(placementStrategy);
}
/**
*
* The placement strategy that determines how tasks for the service are placed.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPlacementStrategy(java.util.Collection)} or {@link #withPlacementStrategy(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param placementStrategy
* The placement strategy that determines how tasks for the service are placed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withPlacementStrategy(PlacementStrategy... placementStrategy) {
if (this.placementStrategy == null) {
setPlacementStrategy(new com.amazonaws.internal.SdkInternalList(placementStrategy.length));
}
for (PlacementStrategy ele : placementStrategy) {
this.placementStrategy.add(ele);
}
return this;
}
/**
*
* The placement strategy that determines how tasks for the service are placed.
*
*
* @param placementStrategy
* The placement strategy that determines how tasks for the service are placed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withPlacementStrategy(java.util.Collection placementStrategy) {
setPlacementStrategy(placementStrategy);
return this;
}
/**
*
* The VPC subnet and security group configuration for tasks that receive their own elastic network interface by
* using the awsvpc
networking mode.
*
*
* @param networkConfiguration
* The VPC subnet and security group configuration for tasks that receive their own elastic network interface
* by using the awsvpc
networking mode.
*/
public void setNetworkConfiguration(NetworkConfiguration networkConfiguration) {
this.networkConfiguration = networkConfiguration;
}
/**
*
* The VPC subnet and security group configuration for tasks that receive their own elastic network interface by
* using the awsvpc
networking mode.
*
*
* @return The VPC subnet and security group configuration for tasks that receive their own elastic network
* interface by using the awsvpc
networking mode.
*/
public NetworkConfiguration getNetworkConfiguration() {
return this.networkConfiguration;
}
/**
*
* The VPC subnet and security group configuration for tasks that receive their own elastic network interface by
* using the awsvpc
networking mode.
*
*
* @param networkConfiguration
* The VPC subnet and security group configuration for tasks that receive their own elastic network interface
* by using the awsvpc
networking mode.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withNetworkConfiguration(NetworkConfiguration networkConfiguration) {
setNetworkConfiguration(networkConfiguration);
return this;
}
/**
*
* The period of time, in seconds, that the Amazon ECS service scheduler ignores unhealthy Elastic Load Balancing
* target health checks after a task has first started.
*
*
* @param healthCheckGracePeriodSeconds
* The period of time, in seconds, that the Amazon ECS service scheduler ignores unhealthy Elastic Load
* Balancing target health checks after a task has first started.
*/
public void setHealthCheckGracePeriodSeconds(Integer healthCheckGracePeriodSeconds) {
this.healthCheckGracePeriodSeconds = healthCheckGracePeriodSeconds;
}
/**
*
* The period of time, in seconds, that the Amazon ECS service scheduler ignores unhealthy Elastic Load Balancing
* target health checks after a task has first started.
*
*
* @return The period of time, in seconds, that the Amazon ECS service scheduler ignores unhealthy Elastic Load
* Balancing target health checks after a task has first started.
*/
public Integer getHealthCheckGracePeriodSeconds() {
return this.healthCheckGracePeriodSeconds;
}
/**
*
* The period of time, in seconds, that the Amazon ECS service scheduler ignores unhealthy Elastic Load Balancing
* target health checks after a task has first started.
*
*
* @param healthCheckGracePeriodSeconds
* The period of time, in seconds, that the Amazon ECS service scheduler ignores unhealthy Elastic Load
* Balancing target health checks after a task has first started.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withHealthCheckGracePeriodSeconds(Integer healthCheckGracePeriodSeconds) {
setHealthCheckGracePeriodSeconds(healthCheckGracePeriodSeconds);
return this;
}
/**
*
* The scheduling strategy to use for the service. For more information, see Services.
*
*
* There are two service scheduler strategies available.
*
*
* -
*
* REPLICA
-The replica scheduling strategy places and maintains the desired number of tasks across your
* cluster. By default, the service scheduler spreads tasks across Availability Zones. You can use task placement
* strategies and constraints to customize task placement decisions.
*
*
* -
*
* DAEMON
-The daemon scheduling strategy deploys exactly one task on each active container instance.
* This task meets all of the task placement constraints that you specify in your cluster. The service scheduler
* also evaluates the task placement constraints for running tasks. It stop tasks that don't meet the placement
* constraints.
*
*
*
* Fargate tasks don't support the DAEMON
scheduling strategy.
*
*
*
*
* @param schedulingStrategy
* The scheduling strategy to use for the service. For more information, see Services.
*
* There are two service scheduler strategies available.
*
*
* -
*
* REPLICA
-The replica scheduling strategy places and maintains the desired number of tasks
* across your cluster. By default, the service scheduler spreads tasks across Availability Zones. You can
* use task placement strategies and constraints to customize task placement decisions.
*
*
* -
*
* DAEMON
-The daemon scheduling strategy deploys exactly one task on each active container
* instance. This task meets all of the task placement constraints that you specify in your cluster. The
* service scheduler also evaluates the task placement constraints for running tasks. It stop tasks that
* don't meet the placement constraints.
*
*
*
* Fargate tasks don't support the DAEMON
scheduling strategy.
*
*
* @see SchedulingStrategy
*/
public void setSchedulingStrategy(String schedulingStrategy) {
this.schedulingStrategy = schedulingStrategy;
}
/**
*
* The scheduling strategy to use for the service. For more information, see Services.
*
*
* There are two service scheduler strategies available.
*
*
* -
*
* REPLICA
-The replica scheduling strategy places and maintains the desired number of tasks across your
* cluster. By default, the service scheduler spreads tasks across Availability Zones. You can use task placement
* strategies and constraints to customize task placement decisions.
*
*
* -
*
* DAEMON
-The daemon scheduling strategy deploys exactly one task on each active container instance.
* This task meets all of the task placement constraints that you specify in your cluster. The service scheduler
* also evaluates the task placement constraints for running tasks. It stop tasks that don't meet the placement
* constraints.
*
*
*
* Fargate tasks don't support the DAEMON
scheduling strategy.
*
*
*
*
* @return The scheduling strategy to use for the service. For more information, see Services.
*
* There are two service scheduler strategies available.
*
*
* -
*
* REPLICA
-The replica scheduling strategy places and maintains the desired number of tasks
* across your cluster. By default, the service scheduler spreads tasks across Availability Zones. You can
* use task placement strategies and constraints to customize task placement decisions.
*
*
* -
*
* DAEMON
-The daemon scheduling strategy deploys exactly one task on each active container
* instance. This task meets all of the task placement constraints that you specify in your cluster. The
* service scheduler also evaluates the task placement constraints for running tasks. It stop tasks that
* don't meet the placement constraints.
*
*
*
* Fargate tasks don't support the DAEMON
scheduling strategy.
*
*
* @see SchedulingStrategy
*/
public String getSchedulingStrategy() {
return this.schedulingStrategy;
}
/**
*
* The scheduling strategy to use for the service. For more information, see Services.
*
*
* There are two service scheduler strategies available.
*
*
* -
*
* REPLICA
-The replica scheduling strategy places and maintains the desired number of tasks across your
* cluster. By default, the service scheduler spreads tasks across Availability Zones. You can use task placement
* strategies and constraints to customize task placement decisions.
*
*
* -
*
* DAEMON
-The daemon scheduling strategy deploys exactly one task on each active container instance.
* This task meets all of the task placement constraints that you specify in your cluster. The service scheduler
* also evaluates the task placement constraints for running tasks. It stop tasks that don't meet the placement
* constraints.
*
*
*
* Fargate tasks don't support the DAEMON
scheduling strategy.
*
*
*
*
* @param schedulingStrategy
* The scheduling strategy to use for the service. For more information, see Services.
*
* There are two service scheduler strategies available.
*
*
* -
*
* REPLICA
-The replica scheduling strategy places and maintains the desired number of tasks
* across your cluster. By default, the service scheduler spreads tasks across Availability Zones. You can
* use task placement strategies and constraints to customize task placement decisions.
*
*
* -
*
* DAEMON
-The daemon scheduling strategy deploys exactly one task on each active container
* instance. This task meets all of the task placement constraints that you specify in your cluster. The
* service scheduler also evaluates the task placement constraints for running tasks. It stop tasks that
* don't meet the placement constraints.
*
*
*
* Fargate tasks don't support the DAEMON
scheduling strategy.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see SchedulingStrategy
*/
public Service withSchedulingStrategy(String schedulingStrategy) {
setSchedulingStrategy(schedulingStrategy);
return this;
}
/**
*
* The scheduling strategy to use for the service. For more information, see Services.
*
*
* There are two service scheduler strategies available.
*
*
* -
*
* REPLICA
-The replica scheduling strategy places and maintains the desired number of tasks across your
* cluster. By default, the service scheduler spreads tasks across Availability Zones. You can use task placement
* strategies and constraints to customize task placement decisions.
*
*
* -
*
* DAEMON
-The daemon scheduling strategy deploys exactly one task on each active container instance.
* This task meets all of the task placement constraints that you specify in your cluster. The service scheduler
* also evaluates the task placement constraints for running tasks. It stop tasks that don't meet the placement
* constraints.
*
*
*
* Fargate tasks don't support the DAEMON
scheduling strategy.
*
*
*
*
* @param schedulingStrategy
* The scheduling strategy to use for the service. For more information, see Services.
*
* There are two service scheduler strategies available.
*
*
* -
*
* REPLICA
-The replica scheduling strategy places and maintains the desired number of tasks
* across your cluster. By default, the service scheduler spreads tasks across Availability Zones. You can
* use task placement strategies and constraints to customize task placement decisions.
*
*
* -
*
* DAEMON
-The daemon scheduling strategy deploys exactly one task on each active container
* instance. This task meets all of the task placement constraints that you specify in your cluster. The
* service scheduler also evaluates the task placement constraints for running tasks. It stop tasks that
* don't meet the placement constraints.
*
*
*
* Fargate tasks don't support the DAEMON
scheduling strategy.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see SchedulingStrategy
*/
public Service withSchedulingStrategy(SchedulingStrategy schedulingStrategy) {
this.schedulingStrategy = schedulingStrategy.toString();
return this;
}
/**
*
* The deployment controller type the service is using.
*
*
* @param deploymentController
* The deployment controller type the service is using.
*/
public void setDeploymentController(DeploymentController deploymentController) {
this.deploymentController = deploymentController;
}
/**
*
* The deployment controller type the service is using.
*
*
* @return The deployment controller type the service is using.
*/
public DeploymentController getDeploymentController() {
return this.deploymentController;
}
/**
*
* The deployment controller type the service is using.
*
*
* @param deploymentController
* The deployment controller type the service is using.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withDeploymentController(DeploymentController deploymentController) {
setDeploymentController(deploymentController);
return this;
}
/**
*
* The metadata that you apply to the service to help you categorize and organize them. Each tag consists of a key
* and an optional value. You define bot the key and value.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* either keys or values as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys or values
* with this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*
*
* @return The metadata that you apply to the service to help you categorize and organize them. Each tag consists of
* a key and an optional value. You define bot the key and value.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may
* have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a
* prefix for either keys or values as it is reserved for Amazon Web Services use. You cannot edit or delete
* tag keys or values with this prefix. Tags with this prefix do not count against your tags per resource
* limit.
*
*
*/
public java.util.List getTags() {
if (tags == null) {
tags = new com.amazonaws.internal.SdkInternalList();
}
return tags;
}
/**
*
* The metadata that you apply to the service to help you categorize and organize them. Each tag consists of a key
* and an optional value. You define bot the key and value.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* either keys or values as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys or values
* with this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*
*
* @param tags
* The metadata that you apply to the service to help you categorize and organize them. Each tag consists of
* a key and an optional value. You define bot the key and value.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may
* have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix
* for either keys or values as it is reserved for Amazon Web Services use. You cannot edit or delete tag
* keys or values with this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new com.amazonaws.internal.SdkInternalList(tags);
}
/**
*
* The metadata that you apply to the service to help you categorize and organize them. Each tag consists of a key
* and an optional value. You define bot the key and value.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* either keys or values as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys or values
* with this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* The metadata that you apply to the service to help you categorize and organize them. Each tag consists of
* a key and an optional value. You define bot the key and value.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may
* have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix
* for either keys or values as it is reserved for Amazon Web Services use. You cannot edit or delete tag
* keys or values with this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withTags(Tag... tags) {
if (this.tags == null) {
setTags(new com.amazonaws.internal.SdkInternalList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* The metadata that you apply to the service to help you categorize and organize them. Each tag consists of a key
* and an optional value. You define bot the key and value.
*
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may have
* restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces representable
* in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix for
* either keys or values as it is reserved for Amazon Web Services use. You cannot edit or delete tag keys or values
* with this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
*
*
* @param tags
* The metadata that you apply to the service to help you categorize and organize them. Each tag consists of
* a key and an optional value. You define bot the key and value.
*
* The following basic restrictions apply to tags:
*
*
* -
*
* Maximum number of tags per resource - 50
*
*
* -
*
* For each resource, each tag key must be unique, and each tag key can have only one value.
*
*
* -
*
* Maximum key length - 128 Unicode characters in UTF-8
*
*
* -
*
* Maximum value length - 256 Unicode characters in UTF-8
*
*
* -
*
* If your tagging schema is used across multiple services and resources, remember that other services may
* have restrictions on allowed characters. Generally allowed characters are: letters, numbers, and spaces
* representable in UTF-8, and the following characters: + - = . _ : / @.
*
*
* -
*
* Tag keys and values are case-sensitive.
*
*
* -
*
* Do not use aws:
, AWS:
, or any upper or lowercase combination of such as a prefix
* for either keys or values as it is reserved for Amazon Web Services use. You cannot edit or delete tag
* keys or values with this prefix. Tags with this prefix do not count against your tags per resource limit.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* The principal that created the service.
*
*
* @param createdBy
* The principal that created the service.
*/
public void setCreatedBy(String createdBy) {
this.createdBy = createdBy;
}
/**
*
* The principal that created the service.
*
*
* @return The principal that created the service.
*/
public String getCreatedBy() {
return this.createdBy;
}
/**
*
* The principal that created the service.
*
*
* @param createdBy
* The principal that created the service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withCreatedBy(String createdBy) {
setCreatedBy(createdBy);
return this;
}
/**
*
* Determines whether to use Amazon ECS managed tags for the tasks in the service. For more information, see Tagging Your Amazon ECS
* Resources in the Amazon Elastic Container Service Developer Guide.
*
*
* @param enableECSManagedTags
* Determines whether to use Amazon ECS managed tags for the tasks in the service. For more information, see
* Tagging Your
* Amazon ECS Resources in the Amazon Elastic Container Service Developer Guide.
*/
public void setEnableECSManagedTags(Boolean enableECSManagedTags) {
this.enableECSManagedTags = enableECSManagedTags;
}
/**
*
* Determines whether to use Amazon ECS managed tags for the tasks in the service. For more information, see Tagging Your Amazon ECS
* Resources in the Amazon Elastic Container Service Developer Guide.
*
*
* @return Determines whether to use Amazon ECS managed tags for the tasks in the service. For more information, see
* Tagging Your
* Amazon ECS Resources in the Amazon Elastic Container Service Developer Guide.
*/
public Boolean getEnableECSManagedTags() {
return this.enableECSManagedTags;
}
/**
*
* Determines whether to use Amazon ECS managed tags for the tasks in the service. For more information, see Tagging Your Amazon ECS
* Resources in the Amazon Elastic Container Service Developer Guide.
*
*
* @param enableECSManagedTags
* Determines whether to use Amazon ECS managed tags for the tasks in the service. For more information, see
* Tagging Your
* Amazon ECS Resources in the Amazon Elastic Container Service Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withEnableECSManagedTags(Boolean enableECSManagedTags) {
setEnableECSManagedTags(enableECSManagedTags);
return this;
}
/**
*
* Determines whether to use Amazon ECS managed tags for the tasks in the service. For more information, see Tagging Your Amazon ECS
* Resources in the Amazon Elastic Container Service Developer Guide.
*
*
* @return Determines whether to use Amazon ECS managed tags for the tasks in the service. For more information, see
* Tagging Your
* Amazon ECS Resources in the Amazon Elastic Container Service Developer Guide.
*/
public Boolean isEnableECSManagedTags() {
return this.enableECSManagedTags;
}
/**
*
* Determines whether to propagate the tags from the task definition or the service to the task. If no value is
* specified, the tags aren't propagated.
*
*
* @param propagateTags
* Determines whether to propagate the tags from the task definition or the service to the task. If no value
* is specified, the tags aren't propagated.
* @see PropagateTags
*/
public void setPropagateTags(String propagateTags) {
this.propagateTags = propagateTags;
}
/**
*
* Determines whether to propagate the tags from the task definition or the service to the task. If no value is
* specified, the tags aren't propagated.
*
*
* @return Determines whether to propagate the tags from the task definition or the service to the task. If no value
* is specified, the tags aren't propagated.
* @see PropagateTags
*/
public String getPropagateTags() {
return this.propagateTags;
}
/**
*
* Determines whether to propagate the tags from the task definition or the service to the task. If no value is
* specified, the tags aren't propagated.
*
*
* @param propagateTags
* Determines whether to propagate the tags from the task definition or the service to the task. If no value
* is specified, the tags aren't propagated.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PropagateTags
*/
public Service withPropagateTags(String propagateTags) {
setPropagateTags(propagateTags);
return this;
}
/**
*
* Determines whether to propagate the tags from the task definition or the service to the task. If no value is
* specified, the tags aren't propagated.
*
*
* @param propagateTags
* Determines whether to propagate the tags from the task definition or the service to the task. If no value
* is specified, the tags aren't propagated.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PropagateTags
*/
public Service withPropagateTags(PropagateTags propagateTags) {
this.propagateTags = propagateTags.toString();
return this;
}
/**
*
* Determines whether the execute command functionality is turned on for the service. If true
, the
* execute command functionality is turned on for all containers in tasks as part of the service.
*
*
* @param enableExecuteCommand
* Determines whether the execute command functionality is turned on for the service. If true
,
* the execute command functionality is turned on for all containers in tasks as part of the service.
*/
public void setEnableExecuteCommand(Boolean enableExecuteCommand) {
this.enableExecuteCommand = enableExecuteCommand;
}
/**
*
* Determines whether the execute command functionality is turned on for the service. If true
, the
* execute command functionality is turned on for all containers in tasks as part of the service.
*
*
* @return Determines whether the execute command functionality is turned on for the service. If true
,
* the execute command functionality is turned on for all containers in tasks as part of the service.
*/
public Boolean getEnableExecuteCommand() {
return this.enableExecuteCommand;
}
/**
*
* Determines whether the execute command functionality is turned on for the service. If true
, the
* execute command functionality is turned on for all containers in tasks as part of the service.
*
*
* @param enableExecuteCommand
* Determines whether the execute command functionality is turned on for the service. If true
,
* the execute command functionality is turned on for all containers in tasks as part of the service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Service withEnableExecuteCommand(Boolean enableExecuteCommand) {
setEnableExecuteCommand(enableExecuteCommand);
return this;
}
/**
*
* Determines whether the execute command functionality is turned on for the service. If true
, the
* execute command functionality is turned on for all containers in tasks as part of the service.
*
*
* @return Determines whether the execute command functionality is turned on for the service. If true
,
* the execute command functionality is turned on for all containers in tasks as part of the service.
*/
public Boolean isEnableExecuteCommand() {
return this.enableExecuteCommand;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getServiceArn() != null)
sb.append("ServiceArn: ").append(getServiceArn()).append(",");
if (getServiceName() != null)
sb.append("ServiceName: ").append(getServiceName()).append(",");
if (getClusterArn() != null)
sb.append("ClusterArn: ").append(getClusterArn()).append(",");
if (getLoadBalancers() != null)
sb.append("LoadBalancers: ").append(getLoadBalancers()).append(",");
if (getServiceRegistries() != null)
sb.append("ServiceRegistries: ").append(getServiceRegistries()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getDesiredCount() != null)
sb.append("DesiredCount: ").append(getDesiredCount()).append(",");
if (getRunningCount() != null)
sb.append("RunningCount: ").append(getRunningCount()).append(",");
if (getPendingCount() != null)
sb.append("PendingCount: ").append(getPendingCount()).append(",");
if (getLaunchType() != null)
sb.append("LaunchType: ").append(getLaunchType()).append(",");
if (getCapacityProviderStrategy() != null)
sb.append("CapacityProviderStrategy: ").append(getCapacityProviderStrategy()).append(",");
if (getPlatformVersion() != null)
sb.append("PlatformVersion: ").append(getPlatformVersion()).append(",");
if (getPlatformFamily() != null)
sb.append("PlatformFamily: ").append(getPlatformFamily()).append(",");
if (getTaskDefinition() != null)
sb.append("TaskDefinition: ").append(getTaskDefinition()).append(",");
if (getDeploymentConfiguration() != null)
sb.append("DeploymentConfiguration: ").append(getDeploymentConfiguration()).append(",");
if (getTaskSets() != null)
sb.append("TaskSets: ").append(getTaskSets()).append(",");
if (getDeployments() != null)
sb.append("Deployments: ").append(getDeployments()).append(",");
if (getRoleArn() != null)
sb.append("RoleArn: ").append(getRoleArn()).append(",");
if (getEvents() != null)
sb.append("Events: ").append(getEvents()).append(",");
if (getCreatedAt() != null)
sb.append("CreatedAt: ").append(getCreatedAt()).append(",");
if (getPlacementConstraints() != null)
sb.append("PlacementConstraints: ").append(getPlacementConstraints()).append(",");
if (getPlacementStrategy() != null)
sb.append("PlacementStrategy: ").append(getPlacementStrategy()).append(",");
if (getNetworkConfiguration() != null)
sb.append("NetworkConfiguration: ").append(getNetworkConfiguration()).append(",");
if (getHealthCheckGracePeriodSeconds() != null)
sb.append("HealthCheckGracePeriodSeconds: ").append(getHealthCheckGracePeriodSeconds()).append(",");
if (getSchedulingStrategy() != null)
sb.append("SchedulingStrategy: ").append(getSchedulingStrategy()).append(",");
if (getDeploymentController() != null)
sb.append("DeploymentController: ").append(getDeploymentController()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getCreatedBy() != null)
sb.append("CreatedBy: ").append(getCreatedBy()).append(",");
if (getEnableECSManagedTags() != null)
sb.append("EnableECSManagedTags: ").append(getEnableECSManagedTags()).append(",");
if (getPropagateTags() != null)
sb.append("PropagateTags: ").append(getPropagateTags()).append(",");
if (getEnableExecuteCommand() != null)
sb.append("EnableExecuteCommand: ").append(getEnableExecuteCommand());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Service == false)
return false;
Service other = (Service) obj;
if (other.getServiceArn() == null ^ this.getServiceArn() == null)
return false;
if (other.getServiceArn() != null && other.getServiceArn().equals(this.getServiceArn()) == false)
return false;
if (other.getServiceName() == null ^ this.getServiceName() == null)
return false;
if (other.getServiceName() != null && other.getServiceName().equals(this.getServiceName()) == false)
return false;
if (other.getClusterArn() == null ^ this.getClusterArn() == null)
return false;
if (other.getClusterArn() != null && other.getClusterArn().equals(this.getClusterArn()) == false)
return false;
if (other.getLoadBalancers() == null ^ this.getLoadBalancers() == null)
return false;
if (other.getLoadBalancers() != null && other.getLoadBalancers().equals(this.getLoadBalancers()) == false)
return false;
if (other.getServiceRegistries() == null ^ this.getServiceRegistries() == null)
return false;
if (other.getServiceRegistries() != null && other.getServiceRegistries().equals(this.getServiceRegistries()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getDesiredCount() == null ^ this.getDesiredCount() == null)
return false;
if (other.getDesiredCount() != null && other.getDesiredCount().equals(this.getDesiredCount()) == false)
return false;
if (other.getRunningCount() == null ^ this.getRunningCount() == null)
return false;
if (other.getRunningCount() != null && other.getRunningCount().equals(this.getRunningCount()) == false)
return false;
if (other.getPendingCount() == null ^ this.getPendingCount() == null)
return false;
if (other.getPendingCount() != null && other.getPendingCount().equals(this.getPendingCount()) == false)
return false;
if (other.getLaunchType() == null ^ this.getLaunchType() == null)
return false;
if (other.getLaunchType() != null && other.getLaunchType().equals(this.getLaunchType()) == false)
return false;
if (other.getCapacityProviderStrategy() == null ^ this.getCapacityProviderStrategy() == null)
return false;
if (other.getCapacityProviderStrategy() != null && other.getCapacityProviderStrategy().equals(this.getCapacityProviderStrategy()) == false)
return false;
if (other.getPlatformVersion() == null ^ this.getPlatformVersion() == null)
return false;
if (other.getPlatformVersion() != null && other.getPlatformVersion().equals(this.getPlatformVersion()) == false)
return false;
if (other.getPlatformFamily() == null ^ this.getPlatformFamily() == null)
return false;
if (other.getPlatformFamily() != null && other.getPlatformFamily().equals(this.getPlatformFamily()) == false)
return false;
if (other.getTaskDefinition() == null ^ this.getTaskDefinition() == null)
return false;
if (other.getTaskDefinition() != null && other.getTaskDefinition().equals(this.getTaskDefinition()) == false)
return false;
if (other.getDeploymentConfiguration() == null ^ this.getDeploymentConfiguration() == null)
return false;
if (other.getDeploymentConfiguration() != null && other.getDeploymentConfiguration().equals(this.getDeploymentConfiguration()) == false)
return false;
if (other.getTaskSets() == null ^ this.getTaskSets() == null)
return false;
if (other.getTaskSets() != null && other.getTaskSets().equals(this.getTaskSets()) == false)
return false;
if (other.getDeployments() == null ^ this.getDeployments() == null)
return false;
if (other.getDeployments() != null && other.getDeployments().equals(this.getDeployments()) == false)
return false;
if (other.getRoleArn() == null ^ this.getRoleArn() == null)
return false;
if (other.getRoleArn() != null && other.getRoleArn().equals(this.getRoleArn()) == false)
return false;
if (other.getEvents() == null ^ this.getEvents() == null)
return false;
if (other.getEvents() != null && other.getEvents().equals(this.getEvents()) == false)
return false;
if (other.getCreatedAt() == null ^ this.getCreatedAt() == null)
return false;
if (other.getCreatedAt() != null && other.getCreatedAt().equals(this.getCreatedAt()) == false)
return false;
if (other.getPlacementConstraints() == null ^ this.getPlacementConstraints() == null)
return false;
if (other.getPlacementConstraints() != null && other.getPlacementConstraints().equals(this.getPlacementConstraints()) == false)
return false;
if (other.getPlacementStrategy() == null ^ this.getPlacementStrategy() == null)
return false;
if (other.getPlacementStrategy() != null && other.getPlacementStrategy().equals(this.getPlacementStrategy()) == false)
return false;
if (other.getNetworkConfiguration() == null ^ this.getNetworkConfiguration() == null)
return false;
if (other.getNetworkConfiguration() != null && other.getNetworkConfiguration().equals(this.getNetworkConfiguration()) == false)
return false;
if (other.getHealthCheckGracePeriodSeconds() == null ^ this.getHealthCheckGracePeriodSeconds() == null)
return false;
if (other.getHealthCheckGracePeriodSeconds() != null
&& other.getHealthCheckGracePeriodSeconds().equals(this.getHealthCheckGracePeriodSeconds()) == false)
return false;
if (other.getSchedulingStrategy() == null ^ this.getSchedulingStrategy() == null)
return false;
if (other.getSchedulingStrategy() != null && other.getSchedulingStrategy().equals(this.getSchedulingStrategy()) == false)
return false;
if (other.getDeploymentController() == null ^ this.getDeploymentController() == null)
return false;
if (other.getDeploymentController() != null && other.getDeploymentController().equals(this.getDeploymentController()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getCreatedBy() == null ^ this.getCreatedBy() == null)
return false;
if (other.getCreatedBy() != null && other.getCreatedBy().equals(this.getCreatedBy()) == false)
return false;
if (other.getEnableECSManagedTags() == null ^ this.getEnableECSManagedTags() == null)
return false;
if (other.getEnableECSManagedTags() != null && other.getEnableECSManagedTags().equals(this.getEnableECSManagedTags()) == false)
return false;
if (other.getPropagateTags() == null ^ this.getPropagateTags() == null)
return false;
if (other.getPropagateTags() != null && other.getPropagateTags().equals(this.getPropagateTags()) == false)
return false;
if (other.getEnableExecuteCommand() == null ^ this.getEnableExecuteCommand() == null)
return false;
if (other.getEnableExecuteCommand() != null && other.getEnableExecuteCommand().equals(this.getEnableExecuteCommand()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getServiceArn() == null) ? 0 : getServiceArn().hashCode());
hashCode = prime * hashCode + ((getServiceName() == null) ? 0 : getServiceName().hashCode());
hashCode = prime * hashCode + ((getClusterArn() == null) ? 0 : getClusterArn().hashCode());
hashCode = prime * hashCode + ((getLoadBalancers() == null) ? 0 : getLoadBalancers().hashCode());
hashCode = prime * hashCode + ((getServiceRegistries() == null) ? 0 : getServiceRegistries().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getDesiredCount() == null) ? 0 : getDesiredCount().hashCode());
hashCode = prime * hashCode + ((getRunningCount() == null) ? 0 : getRunningCount().hashCode());
hashCode = prime * hashCode + ((getPendingCount() == null) ? 0 : getPendingCount().hashCode());
hashCode = prime * hashCode + ((getLaunchType() == null) ? 0 : getLaunchType().hashCode());
hashCode = prime * hashCode + ((getCapacityProviderStrategy() == null) ? 0 : getCapacityProviderStrategy().hashCode());
hashCode = prime * hashCode + ((getPlatformVersion() == null) ? 0 : getPlatformVersion().hashCode());
hashCode = prime * hashCode + ((getPlatformFamily() == null) ? 0 : getPlatformFamily().hashCode());
hashCode = prime * hashCode + ((getTaskDefinition() == null) ? 0 : getTaskDefinition().hashCode());
hashCode = prime * hashCode + ((getDeploymentConfiguration() == null) ? 0 : getDeploymentConfiguration().hashCode());
hashCode = prime * hashCode + ((getTaskSets() == null) ? 0 : getTaskSets().hashCode());
hashCode = prime * hashCode + ((getDeployments() == null) ? 0 : getDeployments().hashCode());
hashCode = prime * hashCode + ((getRoleArn() == null) ? 0 : getRoleArn().hashCode());
hashCode = prime * hashCode + ((getEvents() == null) ? 0 : getEvents().hashCode());
hashCode = prime * hashCode + ((getCreatedAt() == null) ? 0 : getCreatedAt().hashCode());
hashCode = prime * hashCode + ((getPlacementConstraints() == null) ? 0 : getPlacementConstraints().hashCode());
hashCode = prime * hashCode + ((getPlacementStrategy() == null) ? 0 : getPlacementStrategy().hashCode());
hashCode = prime * hashCode + ((getNetworkConfiguration() == null) ? 0 : getNetworkConfiguration().hashCode());
hashCode = prime * hashCode + ((getHealthCheckGracePeriodSeconds() == null) ? 0 : getHealthCheckGracePeriodSeconds().hashCode());
hashCode = prime * hashCode + ((getSchedulingStrategy() == null) ? 0 : getSchedulingStrategy().hashCode());
hashCode = prime * hashCode + ((getDeploymentController() == null) ? 0 : getDeploymentController().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getCreatedBy() == null) ? 0 : getCreatedBy().hashCode());
hashCode = prime * hashCode + ((getEnableECSManagedTags() == null) ? 0 : getEnableECSManagedTags().hashCode());
hashCode = prime * hashCode + ((getPropagateTags() == null) ? 0 : getPropagateTags().hashCode());
hashCode = prime * hashCode + ((getEnableExecuteCommand() == null) ? 0 : getEnableExecuteCommand().hashCode());
return hashCode;
}
@Override
public Service clone() {
try {
return (Service) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.ecs.model.transform.ServiceMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}