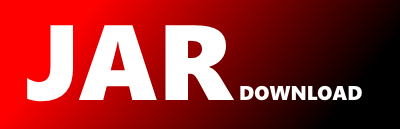
com.amazonaws.services.ecs.model.ServiceConnectConfiguration Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ecs Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ecs.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The Service Connect configuration of your Amazon ECS service. The configuration for this service to discover and
* connect to services, and be discovered by, and connected from, other services within a namespace.
*
*
* Tasks that run in a namespace can use short names to connect to services in the namespace. Tasks can connect to
* services across all of the clusters in the namespace. Tasks connect through a managed proxy container that collects
* logs and metrics for increased visibility. Only the tasks that Amazon ECS services create are supported with Service
* Connect. For more information, see Service Connect in the
* Amazon Elastic Container Service Developer Guide.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ServiceConnectConfiguration implements Serializable, Cloneable, StructuredPojo {
/**
*
* Specifies whether to use Service Connect with this service.
*
*/
private Boolean enabled;
/**
*
* The namespace name or full Amazon Resource Name (ARN) of the Cloud Map namespace for use with Service Connect.
* The namespace must be in the same Amazon Web Services Region as the Amazon ECS service and cluster. The type of
* namespace doesn't affect Service Connect. For more information about Cloud Map, see Working with Services in
* the Cloud Map Developer Guide.
*
*/
private String namespace;
/**
*
* The list of Service Connect service objects. These are names and aliases (also known as endpoints) that are used
* by other Amazon ECS services to connect to this service.
*
*
* This field is not required for a "client" Amazon ECS service that's a member of a namespace only to connect to
* other services within the namespace. An example of this would be a frontend application that accepts incoming
* requests from either a load balancer that's attached to the service or by other means.
*
*
* An object selects a port from the task definition, assigns a name for the Cloud Map service, and a list of
* aliases (endpoints) and ports for client applications to refer to this service.
*
*/
private com.amazonaws.internal.SdkInternalList services;
private LogConfiguration logConfiguration;
/**
*
* Specifies whether to use Service Connect with this service.
*
*
* @param enabled
* Specifies whether to use Service Connect with this service.
*/
public void setEnabled(Boolean enabled) {
this.enabled = enabled;
}
/**
*
* Specifies whether to use Service Connect with this service.
*
*
* @return Specifies whether to use Service Connect with this service.
*/
public Boolean getEnabled() {
return this.enabled;
}
/**
*
* Specifies whether to use Service Connect with this service.
*
*
* @param enabled
* Specifies whether to use Service Connect with this service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceConnectConfiguration withEnabled(Boolean enabled) {
setEnabled(enabled);
return this;
}
/**
*
* Specifies whether to use Service Connect with this service.
*
*
* @return Specifies whether to use Service Connect with this service.
*/
public Boolean isEnabled() {
return this.enabled;
}
/**
*
* The namespace name or full Amazon Resource Name (ARN) of the Cloud Map namespace for use with Service Connect.
* The namespace must be in the same Amazon Web Services Region as the Amazon ECS service and cluster. The type of
* namespace doesn't affect Service Connect. For more information about Cloud Map, see Working with Services in
* the Cloud Map Developer Guide.
*
*
* @param namespace
* The namespace name or full Amazon Resource Name (ARN) of the Cloud Map namespace for use with Service
* Connect. The namespace must be in the same Amazon Web Services Region as the Amazon ECS service and
* cluster. The type of namespace doesn't affect Service Connect. For more information about Cloud Map, see
* Working with
* Services in the Cloud Map Developer Guide.
*/
public void setNamespace(String namespace) {
this.namespace = namespace;
}
/**
*
* The namespace name or full Amazon Resource Name (ARN) of the Cloud Map namespace for use with Service Connect.
* The namespace must be in the same Amazon Web Services Region as the Amazon ECS service and cluster. The type of
* namespace doesn't affect Service Connect. For more information about Cloud Map, see Working with Services in
* the Cloud Map Developer Guide.
*
*
* @return The namespace name or full Amazon Resource Name (ARN) of the Cloud Map namespace for use with Service
* Connect. The namespace must be in the same Amazon Web Services Region as the Amazon ECS service and
* cluster. The type of namespace doesn't affect Service Connect. For more information about Cloud Map, see
* Working with
* Services in the Cloud Map Developer Guide.
*/
public String getNamespace() {
return this.namespace;
}
/**
*
* The namespace name or full Amazon Resource Name (ARN) of the Cloud Map namespace for use with Service Connect.
* The namespace must be in the same Amazon Web Services Region as the Amazon ECS service and cluster. The type of
* namespace doesn't affect Service Connect. For more information about Cloud Map, see Working with Services in
* the Cloud Map Developer Guide.
*
*
* @param namespace
* The namespace name or full Amazon Resource Name (ARN) of the Cloud Map namespace for use with Service
* Connect. The namespace must be in the same Amazon Web Services Region as the Amazon ECS service and
* cluster. The type of namespace doesn't affect Service Connect. For more information about Cloud Map, see
* Working with
* Services in the Cloud Map Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceConnectConfiguration withNamespace(String namespace) {
setNamespace(namespace);
return this;
}
/**
*
* The list of Service Connect service objects. These are names and aliases (also known as endpoints) that are used
* by other Amazon ECS services to connect to this service.
*
*
* This field is not required for a "client" Amazon ECS service that's a member of a namespace only to connect to
* other services within the namespace. An example of this would be a frontend application that accepts incoming
* requests from either a load balancer that's attached to the service or by other means.
*
*
* An object selects a port from the task definition, assigns a name for the Cloud Map service, and a list of
* aliases (endpoints) and ports for client applications to refer to this service.
*
*
* @return The list of Service Connect service objects. These are names and aliases (also known as endpoints) that
* are used by other Amazon ECS services to connect to this service.
*
* This field is not required for a "client" Amazon ECS service that's a member of a namespace only to
* connect to other services within the namespace. An example of this would be a frontend application that
* accepts incoming requests from either a load balancer that's attached to the service or by other means.
*
*
* An object selects a port from the task definition, assigns a name for the Cloud Map service, and a list
* of aliases (endpoints) and ports for client applications to refer to this service.
*/
public java.util.List getServices() {
if (services == null) {
services = new com.amazonaws.internal.SdkInternalList();
}
return services;
}
/**
*
* The list of Service Connect service objects. These are names and aliases (also known as endpoints) that are used
* by other Amazon ECS services to connect to this service.
*
*
* This field is not required for a "client" Amazon ECS service that's a member of a namespace only to connect to
* other services within the namespace. An example of this would be a frontend application that accepts incoming
* requests from either a load balancer that's attached to the service or by other means.
*
*
* An object selects a port from the task definition, assigns a name for the Cloud Map service, and a list of
* aliases (endpoints) and ports for client applications to refer to this service.
*
*
* @param services
* The list of Service Connect service objects. These are names and aliases (also known as endpoints) that
* are used by other Amazon ECS services to connect to this service.
*
* This field is not required for a "client" Amazon ECS service that's a member of a namespace only to
* connect to other services within the namespace. An example of this would be a frontend application that
* accepts incoming requests from either a load balancer that's attached to the service or by other means.
*
*
* An object selects a port from the task definition, assigns a name for the Cloud Map service, and a list of
* aliases (endpoints) and ports for client applications to refer to this service.
*/
public void setServices(java.util.Collection services) {
if (services == null) {
this.services = null;
return;
}
this.services = new com.amazonaws.internal.SdkInternalList(services);
}
/**
*
* The list of Service Connect service objects. These are names and aliases (also known as endpoints) that are used
* by other Amazon ECS services to connect to this service.
*
*
* This field is not required for a "client" Amazon ECS service that's a member of a namespace only to connect to
* other services within the namespace. An example of this would be a frontend application that accepts incoming
* requests from either a load balancer that's attached to the service or by other means.
*
*
* An object selects a port from the task definition, assigns a name for the Cloud Map service, and a list of
* aliases (endpoints) and ports for client applications to refer to this service.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setServices(java.util.Collection)} or {@link #withServices(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param services
* The list of Service Connect service objects. These are names and aliases (also known as endpoints) that
* are used by other Amazon ECS services to connect to this service.
*
* This field is not required for a "client" Amazon ECS service that's a member of a namespace only to
* connect to other services within the namespace. An example of this would be a frontend application that
* accepts incoming requests from either a load balancer that's attached to the service or by other means.
*
*
* An object selects a port from the task definition, assigns a name for the Cloud Map service, and a list of
* aliases (endpoints) and ports for client applications to refer to this service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceConnectConfiguration withServices(ServiceConnectService... services) {
if (this.services == null) {
setServices(new com.amazonaws.internal.SdkInternalList(services.length));
}
for (ServiceConnectService ele : services) {
this.services.add(ele);
}
return this;
}
/**
*
* The list of Service Connect service objects. These are names and aliases (also known as endpoints) that are used
* by other Amazon ECS services to connect to this service.
*
*
* This field is not required for a "client" Amazon ECS service that's a member of a namespace only to connect to
* other services within the namespace. An example of this would be a frontend application that accepts incoming
* requests from either a load balancer that's attached to the service or by other means.
*
*
* An object selects a port from the task definition, assigns a name for the Cloud Map service, and a list of
* aliases (endpoints) and ports for client applications to refer to this service.
*
*
* @param services
* The list of Service Connect service objects. These are names and aliases (also known as endpoints) that
* are used by other Amazon ECS services to connect to this service.
*
* This field is not required for a "client" Amazon ECS service that's a member of a namespace only to
* connect to other services within the namespace. An example of this would be a frontend application that
* accepts incoming requests from either a load balancer that's attached to the service or by other means.
*
*
* An object selects a port from the task definition, assigns a name for the Cloud Map service, and a list of
* aliases (endpoints) and ports for client applications to refer to this service.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceConnectConfiguration withServices(java.util.Collection services) {
setServices(services);
return this;
}
/**
* @param logConfiguration
*/
public void setLogConfiguration(LogConfiguration logConfiguration) {
this.logConfiguration = logConfiguration;
}
/**
* @return
*/
public LogConfiguration getLogConfiguration() {
return this.logConfiguration;
}
/**
* @param logConfiguration
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ServiceConnectConfiguration withLogConfiguration(LogConfiguration logConfiguration) {
setLogConfiguration(logConfiguration);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getEnabled() != null)
sb.append("Enabled: ").append(getEnabled()).append(",");
if (getNamespace() != null)
sb.append("Namespace: ").append(getNamespace()).append(",");
if (getServices() != null)
sb.append("Services: ").append(getServices()).append(",");
if (getLogConfiguration() != null)
sb.append("LogConfiguration: ").append(getLogConfiguration());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ServiceConnectConfiguration == false)
return false;
ServiceConnectConfiguration other = (ServiceConnectConfiguration) obj;
if (other.getEnabled() == null ^ this.getEnabled() == null)
return false;
if (other.getEnabled() != null && other.getEnabled().equals(this.getEnabled()) == false)
return false;
if (other.getNamespace() == null ^ this.getNamespace() == null)
return false;
if (other.getNamespace() != null && other.getNamespace().equals(this.getNamespace()) == false)
return false;
if (other.getServices() == null ^ this.getServices() == null)
return false;
if (other.getServices() != null && other.getServices().equals(this.getServices()) == false)
return false;
if (other.getLogConfiguration() == null ^ this.getLogConfiguration() == null)
return false;
if (other.getLogConfiguration() != null && other.getLogConfiguration().equals(this.getLogConfiguration()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getEnabled() == null) ? 0 : getEnabled().hashCode());
hashCode = prime * hashCode + ((getNamespace() == null) ? 0 : getNamespace().hashCode());
hashCode = prime * hashCode + ((getServices() == null) ? 0 : getServices().hashCode());
hashCode = prime * hashCode + ((getLogConfiguration() == null) ? 0 : getLogConfiguration().hashCode());
return hashCode;
}
@Override
public ServiceConnectConfiguration clone() {
try {
return (ServiceConnectConfiguration) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.ecs.model.transform.ServiceConnectConfigurationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}