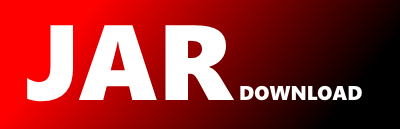
com.amazonaws.services.ecs.model.TaskManagedEBSVolumeConfiguration Maven / Gradle / Ivy
Show all versions of aws-java-sdk-ecs Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.ecs.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* The configuration for the Amazon EBS volume that Amazon ECS creates and manages on your behalf. These settings are
* used to create each Amazon EBS volume, with one volume created for each task.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class TaskManagedEBSVolumeConfiguration implements Serializable, Cloneable, StructuredPojo {
/**
*
* Indicates whether the volume should be encrypted. If no value is specified, encryption is turned on by default.
* This parameter maps 1:1 with the Encrypted
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*/
private Boolean encrypted;
/**
*
* The Amazon Resource Name (ARN) identifier of the Amazon Web Services Key Management Service key to use for Amazon
* EBS encryption. When encryption is turned on and no Amazon Web Services Key Management Service key is specified,
* the default Amazon Web Services managed key for Amazon EBS volumes is used. This parameter maps 1:1 with the
* KmsKeyId
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*
*
* Amazon Web Services authenticates the Amazon Web Services Key Management Service key asynchronously. Therefore,
* if you specify an ID, alias, or ARN that is invalid, the action can appear to complete, but eventually fails.
*
*
*/
private String kmsKeyId;
/**
*
* The volume type. This parameter maps 1:1 with the VolumeType
parameter of the CreateVolume API in the
* Amazon EC2 API Reference. For more information, see Amazon EBS volume types in
* the Amazon EC2 User Guide.
*
*
* The following are the supported volume types.
*
*
* -
*
* General Purpose SSD: gp2
|gp3
*
*
* -
*
* Provisioned IOPS SSD: io1
|io2
*
*
* -
*
* Throughput Optimized HDD: st1
*
*
* -
*
* Cold HDD: sc1
*
*
* -
*
* Magnetic: standard
*
*
*
* The magnetic volume type is not supported on Fargate.
*
*
*
*/
private String volumeType;
/**
*
* The size of the volume in GiB. You must specify either a volume size or a snapshot ID. If you specify a snapshot
* ID, the snapshot size is used for the volume size by default. You can optionally specify a volume size greater
* than or equal to the snapshot size. This parameter maps 1:1 with the Size
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*
* The following are the supported volume size values for each volume type.
*
*
* -
*
* gp2
and gp3
: 1-16,384
*
*
* -
*
* io1
and io2
: 4-16,384
*
*
* -
*
* st1
and sc1
: 125-16,384
*
*
* -
*
* standard
: 1-1,024
*
*
*
*/
private Integer sizeInGiB;
/**
*
* The snapshot that Amazon ECS uses to create the volume. You must specify either a snapshot ID or a volume size.
* This parameter maps 1:1 with the SnapshotId
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*/
private String snapshotId;
/**
*
* The number of I/O operations per second (IOPS). For gp3
, io1
, and io2
* volumes, this represents the number of IOPS that are provisioned for the volume. For gp2
volumes,
* this represents the baseline performance of the volume and the rate at which the volume accumulates I/O credits
* for bursting.
*
*
* The following are the supported values for each volume type.
*
*
* -
*
* gp3
: 3,000 - 16,000 IOPS
*
*
* -
*
* io1
: 100 - 64,000 IOPS
*
*
* -
*
* io2
: 100 - 256,000 IOPS
*
*
*
*
* This parameter is required for io1
and io2
volume types. The default for
* gp3
volumes is 3,000 IOPS
. This parameter is not supported for st1
,
* sc1
, or standard
volume types.
*
*
* This parameter maps 1:1 with the Iops
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*/
private Integer iops;
/**
*
* The throughput to provision for a volume, in MiB/s, with a maximum of 1,000 MiB/s. This parameter maps 1:1 with
* the Throughput
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*
*
* This parameter is only supported for the gp3
volume type.
*
*
*/
private Integer throughput;
/**
*
* The tags to apply to the volume. Amazon ECS applies service-managed tags by default. This parameter maps 1:1 with
* the TagSpecifications.N
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*/
private com.amazonaws.internal.SdkInternalList tagSpecifications;
/**
*
* The ARN of the IAM role to associate with this volume. This is the Amazon ECS infrastructure IAM role that is
* used to manage your Amazon Web Services infrastructure. We recommend using the Amazon ECS-managed
* AmazonECSInfrastructureRolePolicyForVolumes
IAM policy with this role. For more information, see Amazon ECS
* infrastructure IAM role in the Amazon ECS Developer Guide.
*
*/
private String roleArn;
/**
*
* The termination policy for the volume when the task exits. This provides a way to control whether Amazon ECS
* terminates the Amazon EBS volume when the task stops.
*
*/
private TaskManagedEBSVolumeTerminationPolicy terminationPolicy;
/**
*
* The Linux filesystem type for the volume. For volumes created from a snapshot, you must specify the same
* filesystem type that the volume was using when the snapshot was created. If there is a filesystem type mismatch,
* the task will fail to start.
*
*
* The available filesystem types are
ext3
, ext4
, and xfs
. If no
* value is specified, the xfs
filesystem type is used by default.
*
*/
private String filesystemType;
/**
*
* Indicates whether the volume should be encrypted. If no value is specified, encryption is turned on by default.
* This parameter maps 1:1 with the Encrypted
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*
* @param encrypted
* Indicates whether the volume should be encrypted. If no value is specified, encryption is turned on by
* default. This parameter maps 1:1 with the Encrypted
parameter of the CreateVolume API
* in the Amazon EC2 API Reference.
*/
public void setEncrypted(Boolean encrypted) {
this.encrypted = encrypted;
}
/**
*
* Indicates whether the volume should be encrypted. If no value is specified, encryption is turned on by default.
* This parameter maps 1:1 with the Encrypted
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*
* @return Indicates whether the volume should be encrypted. If no value is specified, encryption is turned on by
* default. This parameter maps 1:1 with the Encrypted
parameter of the CreateVolume API
* in the Amazon EC2 API Reference.
*/
public Boolean getEncrypted() {
return this.encrypted;
}
/**
*
* Indicates whether the volume should be encrypted. If no value is specified, encryption is turned on by default.
* This parameter maps 1:1 with the Encrypted
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*
* @param encrypted
* Indicates whether the volume should be encrypted. If no value is specified, encryption is turned on by
* default. This parameter maps 1:1 with the Encrypted
parameter of the CreateVolume API
* in the Amazon EC2 API Reference.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskManagedEBSVolumeConfiguration withEncrypted(Boolean encrypted) {
setEncrypted(encrypted);
return this;
}
/**
*
* Indicates whether the volume should be encrypted. If no value is specified, encryption is turned on by default.
* This parameter maps 1:1 with the Encrypted
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*
* @return Indicates whether the volume should be encrypted. If no value is specified, encryption is turned on by
* default. This parameter maps 1:1 with the Encrypted
parameter of the CreateVolume API
* in the Amazon EC2 API Reference.
*/
public Boolean isEncrypted() {
return this.encrypted;
}
/**
*
* The Amazon Resource Name (ARN) identifier of the Amazon Web Services Key Management Service key to use for Amazon
* EBS encryption. When encryption is turned on and no Amazon Web Services Key Management Service key is specified,
* the default Amazon Web Services managed key for Amazon EBS volumes is used. This parameter maps 1:1 with the
* KmsKeyId
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*
*
* Amazon Web Services authenticates the Amazon Web Services Key Management Service key asynchronously. Therefore,
* if you specify an ID, alias, or ARN that is invalid, the action can appear to complete, but eventually fails.
*
*
*
* @param kmsKeyId
* The Amazon Resource Name (ARN) identifier of the Amazon Web Services Key Management Service key to use for
* Amazon EBS encryption. When encryption is turned on and no Amazon Web Services Key Management Service key
* is specified, the default Amazon Web Services managed key for Amazon EBS volumes is used. This parameter
* maps 1:1 with the KmsKeyId
parameter of the CreateVolume API
* in the Amazon EC2 API Reference.
*
* Amazon Web Services authenticates the Amazon Web Services Key Management Service key asynchronously.
* Therefore, if you specify an ID, alias, or ARN that is invalid, the action can appear to complete, but
* eventually fails.
*
*/
public void setKmsKeyId(String kmsKeyId) {
this.kmsKeyId = kmsKeyId;
}
/**
*
* The Amazon Resource Name (ARN) identifier of the Amazon Web Services Key Management Service key to use for Amazon
* EBS encryption. When encryption is turned on and no Amazon Web Services Key Management Service key is specified,
* the default Amazon Web Services managed key for Amazon EBS volumes is used. This parameter maps 1:1 with the
* KmsKeyId
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*
*
* Amazon Web Services authenticates the Amazon Web Services Key Management Service key asynchronously. Therefore,
* if you specify an ID, alias, or ARN that is invalid, the action can appear to complete, but eventually fails.
*
*
*
* @return The Amazon Resource Name (ARN) identifier of the Amazon Web Services Key Management Service key to use
* for Amazon EBS encryption. When encryption is turned on and no Amazon Web Services Key Management Service
* key is specified, the default Amazon Web Services managed key for Amazon EBS volumes is used. This
* parameter maps 1:1 with the KmsKeyId
parameter of the CreateVolume API
* in the Amazon EC2 API Reference.
*
* Amazon Web Services authenticates the Amazon Web Services Key Management Service key asynchronously.
* Therefore, if you specify an ID, alias, or ARN that is invalid, the action can appear to complete, but
* eventually fails.
*
*/
public String getKmsKeyId() {
return this.kmsKeyId;
}
/**
*
* The Amazon Resource Name (ARN) identifier of the Amazon Web Services Key Management Service key to use for Amazon
* EBS encryption. When encryption is turned on and no Amazon Web Services Key Management Service key is specified,
* the default Amazon Web Services managed key for Amazon EBS volumes is used. This parameter maps 1:1 with the
* KmsKeyId
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*
*
* Amazon Web Services authenticates the Amazon Web Services Key Management Service key asynchronously. Therefore,
* if you specify an ID, alias, or ARN that is invalid, the action can appear to complete, but eventually fails.
*
*
*
* @param kmsKeyId
* The Amazon Resource Name (ARN) identifier of the Amazon Web Services Key Management Service key to use for
* Amazon EBS encryption. When encryption is turned on and no Amazon Web Services Key Management Service key
* is specified, the default Amazon Web Services managed key for Amazon EBS volumes is used. This parameter
* maps 1:1 with the KmsKeyId
parameter of the CreateVolume API
* in the Amazon EC2 API Reference.
*
* Amazon Web Services authenticates the Amazon Web Services Key Management Service key asynchronously.
* Therefore, if you specify an ID, alias, or ARN that is invalid, the action can appear to complete, but
* eventually fails.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskManagedEBSVolumeConfiguration withKmsKeyId(String kmsKeyId) {
setKmsKeyId(kmsKeyId);
return this;
}
/**
*
* The volume type. This parameter maps 1:1 with the VolumeType
parameter of the CreateVolume API in the
* Amazon EC2 API Reference. For more information, see Amazon EBS volume types in
* the Amazon EC2 User Guide.
*
*
* The following are the supported volume types.
*
*
* -
*
* General Purpose SSD: gp2
|gp3
*
*
* -
*
* Provisioned IOPS SSD: io1
|io2
*
*
* -
*
* Throughput Optimized HDD: st1
*
*
* -
*
* Cold HDD: sc1
*
*
* -
*
* Magnetic: standard
*
*
*
* The magnetic volume type is not supported on Fargate.
*
*
*
*
* @param volumeType
* The volume type. This parameter maps 1:1 with the VolumeType
parameter of the CreateVolume API
* in the Amazon EC2 API Reference. For more information, see Amazon EBS volume
* types in the Amazon EC2 User Guide.
*
* The following are the supported volume types.
*
*
* -
*
* General Purpose SSD: gp2
|gp3
*
*
* -
*
* Provisioned IOPS SSD: io1
|io2
*
*
* -
*
* Throughput Optimized HDD: st1
*
*
* -
*
* Cold HDD: sc1
*
*
* -
*
* Magnetic: standard
*
*
*
* The magnetic volume type is not supported on Fargate.
*
*
*/
public void setVolumeType(String volumeType) {
this.volumeType = volumeType;
}
/**
*
* The volume type. This parameter maps 1:1 with the VolumeType
parameter of the CreateVolume API in the
* Amazon EC2 API Reference. For more information, see Amazon EBS volume types in
* the Amazon EC2 User Guide.
*
*
* The following are the supported volume types.
*
*
* -
*
* General Purpose SSD: gp2
|gp3
*
*
* -
*
* Provisioned IOPS SSD: io1
|io2
*
*
* -
*
* Throughput Optimized HDD: st1
*
*
* -
*
* Cold HDD: sc1
*
*
* -
*
* Magnetic: standard
*
*
*
* The magnetic volume type is not supported on Fargate.
*
*
*
*
* @return The volume type. This parameter maps 1:1 with the VolumeType
parameter of the CreateVolume API
* in the Amazon EC2 API Reference. For more information, see Amazon EBS volume
* types in the Amazon EC2 User Guide.
*
* The following are the supported volume types.
*
*
* -
*
* General Purpose SSD: gp2
|gp3
*
*
* -
*
* Provisioned IOPS SSD: io1
|io2
*
*
* -
*
* Throughput Optimized HDD: st1
*
*
* -
*
* Cold HDD: sc1
*
*
* -
*
* Magnetic: standard
*
*
*
* The magnetic volume type is not supported on Fargate.
*
*
*/
public String getVolumeType() {
return this.volumeType;
}
/**
*
* The volume type. This parameter maps 1:1 with the VolumeType
parameter of the CreateVolume API in the
* Amazon EC2 API Reference. For more information, see Amazon EBS volume types in
* the Amazon EC2 User Guide.
*
*
* The following are the supported volume types.
*
*
* -
*
* General Purpose SSD: gp2
|gp3
*
*
* -
*
* Provisioned IOPS SSD: io1
|io2
*
*
* -
*
* Throughput Optimized HDD: st1
*
*
* -
*
* Cold HDD: sc1
*
*
* -
*
* Magnetic: standard
*
*
*
* The magnetic volume type is not supported on Fargate.
*
*
*
*
* @param volumeType
* The volume type. This parameter maps 1:1 with the VolumeType
parameter of the CreateVolume API
* in the Amazon EC2 API Reference. For more information, see Amazon EBS volume
* types in the Amazon EC2 User Guide.
*
* The following are the supported volume types.
*
*
* -
*
* General Purpose SSD: gp2
|gp3
*
*
* -
*
* Provisioned IOPS SSD: io1
|io2
*
*
* -
*
* Throughput Optimized HDD: st1
*
*
* -
*
* Cold HDD: sc1
*
*
* -
*
* Magnetic: standard
*
*
*
* The magnetic volume type is not supported on Fargate.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskManagedEBSVolumeConfiguration withVolumeType(String volumeType) {
setVolumeType(volumeType);
return this;
}
/**
*
* The size of the volume in GiB. You must specify either a volume size or a snapshot ID. If you specify a snapshot
* ID, the snapshot size is used for the volume size by default. You can optionally specify a volume size greater
* than or equal to the snapshot size. This parameter maps 1:1 with the Size
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*
* The following are the supported volume size values for each volume type.
*
*
* -
*
* gp2
and gp3
: 1-16,384
*
*
* -
*
* io1
and io2
: 4-16,384
*
*
* -
*
* st1
and sc1
: 125-16,384
*
*
* -
*
* standard
: 1-1,024
*
*
*
*
* @param sizeInGiB
* The size of the volume in GiB. You must specify either a volume size or a snapshot ID. If you specify a
* snapshot ID, the snapshot size is used for the volume size by default. You can optionally specify a volume
* size greater than or equal to the snapshot size. This parameter maps 1:1 with the Size
* parameter of the CreateVolume API
* in the Amazon EC2 API Reference.
*
* The following are the supported volume size values for each volume type.
*
*
* -
*
* gp2
and gp3
: 1-16,384
*
*
* -
*
* io1
and io2
: 4-16,384
*
*
* -
*
* st1
and sc1
: 125-16,384
*
*
* -
*
* standard
: 1-1,024
*
*
*/
public void setSizeInGiB(Integer sizeInGiB) {
this.sizeInGiB = sizeInGiB;
}
/**
*
* The size of the volume in GiB. You must specify either a volume size or a snapshot ID. If you specify a snapshot
* ID, the snapshot size is used for the volume size by default. You can optionally specify a volume size greater
* than or equal to the snapshot size. This parameter maps 1:1 with the Size
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*
* The following are the supported volume size values for each volume type.
*
*
* -
*
* gp2
and gp3
: 1-16,384
*
*
* -
*
* io1
and io2
: 4-16,384
*
*
* -
*
* st1
and sc1
: 125-16,384
*
*
* -
*
* standard
: 1-1,024
*
*
*
*
* @return The size of the volume in GiB. You must specify either a volume size or a snapshot ID. If you specify a
* snapshot ID, the snapshot size is used for the volume size by default. You can optionally specify a
* volume size greater than or equal to the snapshot size. This parameter maps 1:1 with the
* Size
parameter of the CreateVolume API
* in the Amazon EC2 API Reference.
*
* The following are the supported volume size values for each volume type.
*
*
* -
*
* gp2
and gp3
: 1-16,384
*
*
* -
*
* io1
and io2
: 4-16,384
*
*
* -
*
* st1
and sc1
: 125-16,384
*
*
* -
*
* standard
: 1-1,024
*
*
*/
public Integer getSizeInGiB() {
return this.sizeInGiB;
}
/**
*
* The size of the volume in GiB. You must specify either a volume size or a snapshot ID. If you specify a snapshot
* ID, the snapshot size is used for the volume size by default. You can optionally specify a volume size greater
* than or equal to the snapshot size. This parameter maps 1:1 with the Size
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*
* The following are the supported volume size values for each volume type.
*
*
* -
*
* gp2
and gp3
: 1-16,384
*
*
* -
*
* io1
and io2
: 4-16,384
*
*
* -
*
* st1
and sc1
: 125-16,384
*
*
* -
*
* standard
: 1-1,024
*
*
*
*
* @param sizeInGiB
* The size of the volume in GiB. You must specify either a volume size or a snapshot ID. If you specify a
* snapshot ID, the snapshot size is used for the volume size by default. You can optionally specify a volume
* size greater than or equal to the snapshot size. This parameter maps 1:1 with the Size
* parameter of the CreateVolume API
* in the Amazon EC2 API Reference.
*
* The following are the supported volume size values for each volume type.
*
*
* -
*
* gp2
and gp3
: 1-16,384
*
*
* -
*
* io1
and io2
: 4-16,384
*
*
* -
*
* st1
and sc1
: 125-16,384
*
*
* -
*
* standard
: 1-1,024
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskManagedEBSVolumeConfiguration withSizeInGiB(Integer sizeInGiB) {
setSizeInGiB(sizeInGiB);
return this;
}
/**
*
* The snapshot that Amazon ECS uses to create the volume. You must specify either a snapshot ID or a volume size.
* This parameter maps 1:1 with the SnapshotId
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*
* @param snapshotId
* The snapshot that Amazon ECS uses to create the volume. You must specify either a snapshot ID or a volume
* size. This parameter maps 1:1 with the SnapshotId
parameter of the CreateVolume API
* in the Amazon EC2 API Reference.
*/
public void setSnapshotId(String snapshotId) {
this.snapshotId = snapshotId;
}
/**
*
* The snapshot that Amazon ECS uses to create the volume. You must specify either a snapshot ID or a volume size.
* This parameter maps 1:1 with the SnapshotId
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*
* @return The snapshot that Amazon ECS uses to create the volume. You must specify either a snapshot ID or a volume
* size. This parameter maps 1:1 with the SnapshotId
parameter of the CreateVolume API
* in the Amazon EC2 API Reference.
*/
public String getSnapshotId() {
return this.snapshotId;
}
/**
*
* The snapshot that Amazon ECS uses to create the volume. You must specify either a snapshot ID or a volume size.
* This parameter maps 1:1 with the SnapshotId
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*
* @param snapshotId
* The snapshot that Amazon ECS uses to create the volume. You must specify either a snapshot ID or a volume
* size. This parameter maps 1:1 with the SnapshotId
parameter of the CreateVolume API
* in the Amazon EC2 API Reference.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskManagedEBSVolumeConfiguration withSnapshotId(String snapshotId) {
setSnapshotId(snapshotId);
return this;
}
/**
*
* The number of I/O operations per second (IOPS). For gp3
, io1
, and io2
* volumes, this represents the number of IOPS that are provisioned for the volume. For gp2
volumes,
* this represents the baseline performance of the volume and the rate at which the volume accumulates I/O credits
* for bursting.
*
*
* The following are the supported values for each volume type.
*
*
* -
*
* gp3
: 3,000 - 16,000 IOPS
*
*
* -
*
* io1
: 100 - 64,000 IOPS
*
*
* -
*
* io2
: 100 - 256,000 IOPS
*
*
*
*
* This parameter is required for io1
and io2
volume types. The default for
* gp3
volumes is 3,000 IOPS
. This parameter is not supported for st1
,
* sc1
, or standard
volume types.
*
*
* This parameter maps 1:1 with the Iops
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*
* @param iops
* The number of I/O operations per second (IOPS). For gp3
, io1
, and
* io2
volumes, this represents the number of IOPS that are provisioned for the volume. For
* gp2
volumes, this represents the baseline performance of the volume and the rate at which the
* volume accumulates I/O credits for bursting.
*
* The following are the supported values for each volume type.
*
*
* -
*
* gp3
: 3,000 - 16,000 IOPS
*
*
* -
*
* io1
: 100 - 64,000 IOPS
*
*
* -
*
* io2
: 100 - 256,000 IOPS
*
*
*
*
* This parameter is required for io1
and io2
volume types. The default for
* gp3
volumes is 3,000 IOPS
. This parameter is not supported for st1
,
* sc1
, or standard
volume types.
*
*
* This parameter maps 1:1 with the Iops
parameter of the CreateVolume API
* in the Amazon EC2 API Reference.
*/
public void setIops(Integer iops) {
this.iops = iops;
}
/**
*
* The number of I/O operations per second (IOPS). For gp3
, io1
, and io2
* volumes, this represents the number of IOPS that are provisioned for the volume. For gp2
volumes,
* this represents the baseline performance of the volume and the rate at which the volume accumulates I/O credits
* for bursting.
*
*
* The following are the supported values for each volume type.
*
*
* -
*
* gp3
: 3,000 - 16,000 IOPS
*
*
* -
*
* io1
: 100 - 64,000 IOPS
*
*
* -
*
* io2
: 100 - 256,000 IOPS
*
*
*
*
* This parameter is required for io1
and io2
volume types. The default for
* gp3
volumes is 3,000 IOPS
. This parameter is not supported for st1
,
* sc1
, or standard
volume types.
*
*
* This parameter maps 1:1 with the Iops
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*
* @return The number of I/O operations per second (IOPS). For gp3
, io1
, and
* io2
volumes, this represents the number of IOPS that are provisioned for the volume. For
* gp2
volumes, this represents the baseline performance of the volume and the rate at which
* the volume accumulates I/O credits for bursting.
*
* The following are the supported values for each volume type.
*
*
* -
*
* gp3
: 3,000 - 16,000 IOPS
*
*
* -
*
* io1
: 100 - 64,000 IOPS
*
*
* -
*
* io2
: 100 - 256,000 IOPS
*
*
*
*
* This parameter is required for io1
and io2
volume types. The default for
* gp3
volumes is 3,000 IOPS
. This parameter is not supported for st1
, sc1
, or standard
volume types.
*
*
* This parameter maps 1:1 with the Iops
parameter of the CreateVolume API
* in the Amazon EC2 API Reference.
*/
public Integer getIops() {
return this.iops;
}
/**
*
* The number of I/O operations per second (IOPS). For gp3
, io1
, and io2
* volumes, this represents the number of IOPS that are provisioned for the volume. For gp2
volumes,
* this represents the baseline performance of the volume and the rate at which the volume accumulates I/O credits
* for bursting.
*
*
* The following are the supported values for each volume type.
*
*
* -
*
* gp3
: 3,000 - 16,000 IOPS
*
*
* -
*
* io1
: 100 - 64,000 IOPS
*
*
* -
*
* io2
: 100 - 256,000 IOPS
*
*
*
*
* This parameter is required for io1
and io2
volume types. The default for
* gp3
volumes is 3,000 IOPS
. This parameter is not supported for st1
,
* sc1
, or standard
volume types.
*
*
* This parameter maps 1:1 with the Iops
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*
* @param iops
* The number of I/O operations per second (IOPS). For gp3
, io1
, and
* io2
volumes, this represents the number of IOPS that are provisioned for the volume. For
* gp2
volumes, this represents the baseline performance of the volume and the rate at which the
* volume accumulates I/O credits for bursting.
*
* The following are the supported values for each volume type.
*
*
* -
*
* gp3
: 3,000 - 16,000 IOPS
*
*
* -
*
* io1
: 100 - 64,000 IOPS
*
*
* -
*
* io2
: 100 - 256,000 IOPS
*
*
*
*
* This parameter is required for io1
and io2
volume types. The default for
* gp3
volumes is 3,000 IOPS
. This parameter is not supported for st1
,
* sc1
, or standard
volume types.
*
*
* This parameter maps 1:1 with the Iops
parameter of the CreateVolume API
* in the Amazon EC2 API Reference.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskManagedEBSVolumeConfiguration withIops(Integer iops) {
setIops(iops);
return this;
}
/**
*
* The throughput to provision for a volume, in MiB/s, with a maximum of 1,000 MiB/s. This parameter maps 1:1 with
* the Throughput
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*
*
* This parameter is only supported for the gp3
volume type.
*
*
*
* @param throughput
* The throughput to provision for a volume, in MiB/s, with a maximum of 1,000 MiB/s. This parameter maps 1:1
* with the Throughput
parameter of the CreateVolume API
* in the Amazon EC2 API Reference.
*
* This parameter is only supported for the gp3
volume type.
*
*/
public void setThroughput(Integer throughput) {
this.throughput = throughput;
}
/**
*
* The throughput to provision for a volume, in MiB/s, with a maximum of 1,000 MiB/s. This parameter maps 1:1 with
* the Throughput
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*
*
* This parameter is only supported for the gp3
volume type.
*
*
*
* @return The throughput to provision for a volume, in MiB/s, with a maximum of 1,000 MiB/s. This parameter maps
* 1:1 with the Throughput
parameter of the CreateVolume API
* in the Amazon EC2 API Reference.
*
* This parameter is only supported for the gp3
volume type.
*
*/
public Integer getThroughput() {
return this.throughput;
}
/**
*
* The throughput to provision for a volume, in MiB/s, with a maximum of 1,000 MiB/s. This parameter maps 1:1 with
* the Throughput
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*
*
* This parameter is only supported for the gp3
volume type.
*
*
*
* @param throughput
* The throughput to provision for a volume, in MiB/s, with a maximum of 1,000 MiB/s. This parameter maps 1:1
* with the Throughput
parameter of the CreateVolume API
* in the Amazon EC2 API Reference.
*
* This parameter is only supported for the gp3
volume type.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskManagedEBSVolumeConfiguration withThroughput(Integer throughput) {
setThroughput(throughput);
return this;
}
/**
*
* The tags to apply to the volume. Amazon ECS applies service-managed tags by default. This parameter maps 1:1 with
* the TagSpecifications.N
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*
* @return The tags to apply to the volume. Amazon ECS applies service-managed tags by default. This parameter maps
* 1:1 with the TagSpecifications.N
parameter of the CreateVolume API
* in the Amazon EC2 API Reference.
*/
public java.util.List getTagSpecifications() {
if (tagSpecifications == null) {
tagSpecifications = new com.amazonaws.internal.SdkInternalList();
}
return tagSpecifications;
}
/**
*
* The tags to apply to the volume. Amazon ECS applies service-managed tags by default. This parameter maps 1:1 with
* the TagSpecifications.N
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*
* @param tagSpecifications
* The tags to apply to the volume. Amazon ECS applies service-managed tags by default. This parameter maps
* 1:1 with the TagSpecifications.N
parameter of the CreateVolume API
* in the Amazon EC2 API Reference.
*/
public void setTagSpecifications(java.util.Collection tagSpecifications) {
if (tagSpecifications == null) {
this.tagSpecifications = null;
return;
}
this.tagSpecifications = new com.amazonaws.internal.SdkInternalList(tagSpecifications);
}
/**
*
* The tags to apply to the volume. Amazon ECS applies service-managed tags by default. This parameter maps 1:1 with
* the TagSpecifications.N
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTagSpecifications(java.util.Collection)} or {@link #withTagSpecifications(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param tagSpecifications
* The tags to apply to the volume. Amazon ECS applies service-managed tags by default. This parameter maps
* 1:1 with the TagSpecifications.N
parameter of the CreateVolume API
* in the Amazon EC2 API Reference.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskManagedEBSVolumeConfiguration withTagSpecifications(EBSTagSpecification... tagSpecifications) {
if (this.tagSpecifications == null) {
setTagSpecifications(new com.amazonaws.internal.SdkInternalList(tagSpecifications.length));
}
for (EBSTagSpecification ele : tagSpecifications) {
this.tagSpecifications.add(ele);
}
return this;
}
/**
*
* The tags to apply to the volume. Amazon ECS applies service-managed tags by default. This parameter maps 1:1 with
* the TagSpecifications.N
parameter of the CreateVolume API in the
* Amazon EC2 API Reference.
*
*
* @param tagSpecifications
* The tags to apply to the volume. Amazon ECS applies service-managed tags by default. This parameter maps
* 1:1 with the TagSpecifications.N
parameter of the CreateVolume API
* in the Amazon EC2 API Reference.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskManagedEBSVolumeConfiguration withTagSpecifications(java.util.Collection tagSpecifications) {
setTagSpecifications(tagSpecifications);
return this;
}
/**
*
* The ARN of the IAM role to associate with this volume. This is the Amazon ECS infrastructure IAM role that is
* used to manage your Amazon Web Services infrastructure. We recommend using the Amazon ECS-managed
* AmazonECSInfrastructureRolePolicyForVolumes
IAM policy with this role. For more information, see Amazon ECS
* infrastructure IAM role in the Amazon ECS Developer Guide.
*
*
* @param roleArn
* The ARN of the IAM role to associate with this volume. This is the Amazon ECS infrastructure IAM role that
* is used to manage your Amazon Web Services infrastructure. We recommend using the Amazon ECS-managed
* AmazonECSInfrastructureRolePolicyForVolumes
IAM policy with this role. For more information,
* see Amazon ECS
* infrastructure IAM role in the Amazon ECS Developer Guide.
*/
public void setRoleArn(String roleArn) {
this.roleArn = roleArn;
}
/**
*
* The ARN of the IAM role to associate with this volume. This is the Amazon ECS infrastructure IAM role that is
* used to manage your Amazon Web Services infrastructure. We recommend using the Amazon ECS-managed
* AmazonECSInfrastructureRolePolicyForVolumes
IAM policy with this role. For more information, see Amazon ECS
* infrastructure IAM role in the Amazon ECS Developer Guide.
*
*
* @return The ARN of the IAM role to associate with this volume. This is the Amazon ECS infrastructure IAM role
* that is used to manage your Amazon Web Services infrastructure. We recommend using the Amazon ECS-managed
* AmazonECSInfrastructureRolePolicyForVolumes
IAM policy with this role. For more information,
* see Amazon
* ECS infrastructure IAM role in the Amazon ECS Developer Guide.
*/
public String getRoleArn() {
return this.roleArn;
}
/**
*
* The ARN of the IAM role to associate with this volume. This is the Amazon ECS infrastructure IAM role that is
* used to manage your Amazon Web Services infrastructure. We recommend using the Amazon ECS-managed
* AmazonECSInfrastructureRolePolicyForVolumes
IAM policy with this role. For more information, see Amazon ECS
* infrastructure IAM role in the Amazon ECS Developer Guide.
*
*
* @param roleArn
* The ARN of the IAM role to associate with this volume. This is the Amazon ECS infrastructure IAM role that
* is used to manage your Amazon Web Services infrastructure. We recommend using the Amazon ECS-managed
* AmazonECSInfrastructureRolePolicyForVolumes
IAM policy with this role. For more information,
* see Amazon ECS
* infrastructure IAM role in the Amazon ECS Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskManagedEBSVolumeConfiguration withRoleArn(String roleArn) {
setRoleArn(roleArn);
return this;
}
/**
*
* The termination policy for the volume when the task exits. This provides a way to control whether Amazon ECS
* terminates the Amazon EBS volume when the task stops.
*
*
* @param terminationPolicy
* The termination policy for the volume when the task exits. This provides a way to control whether Amazon
* ECS terminates the Amazon EBS volume when the task stops.
*/
public void setTerminationPolicy(TaskManagedEBSVolumeTerminationPolicy terminationPolicy) {
this.terminationPolicy = terminationPolicy;
}
/**
*
* The termination policy for the volume when the task exits. This provides a way to control whether Amazon ECS
* terminates the Amazon EBS volume when the task stops.
*
*
* @return The termination policy for the volume when the task exits. This provides a way to control whether Amazon
* ECS terminates the Amazon EBS volume when the task stops.
*/
public TaskManagedEBSVolumeTerminationPolicy getTerminationPolicy() {
return this.terminationPolicy;
}
/**
*
* The termination policy for the volume when the task exits. This provides a way to control whether Amazon ECS
* terminates the Amazon EBS volume when the task stops.
*
*
* @param terminationPolicy
* The termination policy for the volume when the task exits. This provides a way to control whether Amazon
* ECS terminates the Amazon EBS volume when the task stops.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public TaskManagedEBSVolumeConfiguration withTerminationPolicy(TaskManagedEBSVolumeTerminationPolicy terminationPolicy) {
setTerminationPolicy(terminationPolicy);
return this;
}
/**
*
* The Linux filesystem type for the volume. For volumes created from a snapshot, you must specify the same
* filesystem type that the volume was using when the snapshot was created. If there is a filesystem type mismatch,
* the task will fail to start.
*
*
* The available filesystem types are
ext3
, ext4
, and xfs
. If no
* value is specified, the xfs
filesystem type is used by default.
*
*
* @param filesystemType
* The Linux filesystem type for the volume. For volumes created from a snapshot, you must specify the same
* filesystem type that the volume was using when the snapshot was created. If there is a filesystem type
* mismatch, the task will fail to start.
*
* The available filesystem types are
ext3
, ext4
, and xfs
. If
* no value is specified, the xfs
filesystem type is used by default.
* @see TaskFilesystemType
*/
public void setFilesystemType(String filesystemType) {
this.filesystemType = filesystemType;
}
/**
*
* The Linux filesystem type for the volume. For volumes created from a snapshot, you must specify the same
* filesystem type that the volume was using when the snapshot was created. If there is a filesystem type mismatch,
* the task will fail to start.
*
*
* The available filesystem types are
ext3
, ext4
, and xfs
. If no
* value is specified, the xfs
filesystem type is used by default.
*
*
* @return The Linux filesystem type for the volume. For volumes created from a snapshot, you must specify the same
* filesystem type that the volume was using when the snapshot was created. If there is a filesystem type
* mismatch, the task will fail to start.
*
* The available filesystem types are
ext3
, ext4
, and xfs
. If
* no value is specified, the xfs
filesystem type is used by default.
* @see TaskFilesystemType
*/
public String getFilesystemType() {
return this.filesystemType;
}
/**
*
* The Linux filesystem type for the volume. For volumes created from a snapshot, you must specify the same
* filesystem type that the volume was using when the snapshot was created. If there is a filesystem type mismatch,
* the task will fail to start.
*
*
* The available filesystem types are
ext3
, ext4
, and xfs
. If no
* value is specified, the xfs
filesystem type is used by default.
*
*
* @param filesystemType
* The Linux filesystem type for the volume. For volumes created from a snapshot, you must specify the same
* filesystem type that the volume was using when the snapshot was created. If there is a filesystem type
* mismatch, the task will fail to start.
*
* The available filesystem types are
ext3
, ext4
, and xfs
. If
* no value is specified, the xfs
filesystem type is used by default.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TaskFilesystemType
*/
public TaskManagedEBSVolumeConfiguration withFilesystemType(String filesystemType) {
setFilesystemType(filesystemType);
return this;
}
/**
*
* The Linux filesystem type for the volume. For volumes created from a snapshot, you must specify the same
* filesystem type that the volume was using when the snapshot was created. If there is a filesystem type mismatch,
* the task will fail to start.
*
*
* The available filesystem types are
ext3
, ext4
, and xfs
. If no
* value is specified, the xfs
filesystem type is used by default.
*
*
* @param filesystemType
* The Linux filesystem type for the volume. For volumes created from a snapshot, you must specify the same
* filesystem type that the volume was using when the snapshot was created. If there is a filesystem type
* mismatch, the task will fail to start.
*
* The available filesystem types are
ext3
, ext4
, and xfs
. If
* no value is specified, the xfs
filesystem type is used by default.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TaskFilesystemType
*/
public TaskManagedEBSVolumeConfiguration withFilesystemType(TaskFilesystemType filesystemType) {
this.filesystemType = filesystemType.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getEncrypted() != null)
sb.append("Encrypted: ").append(getEncrypted()).append(",");
if (getKmsKeyId() != null)
sb.append("KmsKeyId: ").append(getKmsKeyId()).append(",");
if (getVolumeType() != null)
sb.append("VolumeType: ").append(getVolumeType()).append(",");
if (getSizeInGiB() != null)
sb.append("SizeInGiB: ").append(getSizeInGiB()).append(",");
if (getSnapshotId() != null)
sb.append("SnapshotId: ").append(getSnapshotId()).append(",");
if (getIops() != null)
sb.append("Iops: ").append(getIops()).append(",");
if (getThroughput() != null)
sb.append("Throughput: ").append(getThroughput()).append(",");
if (getTagSpecifications() != null)
sb.append("TagSpecifications: ").append(getTagSpecifications()).append(",");
if (getRoleArn() != null)
sb.append("RoleArn: ").append(getRoleArn()).append(",");
if (getTerminationPolicy() != null)
sb.append("TerminationPolicy: ").append(getTerminationPolicy()).append(",");
if (getFilesystemType() != null)
sb.append("FilesystemType: ").append(getFilesystemType());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof TaskManagedEBSVolumeConfiguration == false)
return false;
TaskManagedEBSVolumeConfiguration other = (TaskManagedEBSVolumeConfiguration) obj;
if (other.getEncrypted() == null ^ this.getEncrypted() == null)
return false;
if (other.getEncrypted() != null && other.getEncrypted().equals(this.getEncrypted()) == false)
return false;
if (other.getKmsKeyId() == null ^ this.getKmsKeyId() == null)
return false;
if (other.getKmsKeyId() != null && other.getKmsKeyId().equals(this.getKmsKeyId()) == false)
return false;
if (other.getVolumeType() == null ^ this.getVolumeType() == null)
return false;
if (other.getVolumeType() != null && other.getVolumeType().equals(this.getVolumeType()) == false)
return false;
if (other.getSizeInGiB() == null ^ this.getSizeInGiB() == null)
return false;
if (other.getSizeInGiB() != null && other.getSizeInGiB().equals(this.getSizeInGiB()) == false)
return false;
if (other.getSnapshotId() == null ^ this.getSnapshotId() == null)
return false;
if (other.getSnapshotId() != null && other.getSnapshotId().equals(this.getSnapshotId()) == false)
return false;
if (other.getIops() == null ^ this.getIops() == null)
return false;
if (other.getIops() != null && other.getIops().equals(this.getIops()) == false)
return false;
if (other.getThroughput() == null ^ this.getThroughput() == null)
return false;
if (other.getThroughput() != null && other.getThroughput().equals(this.getThroughput()) == false)
return false;
if (other.getTagSpecifications() == null ^ this.getTagSpecifications() == null)
return false;
if (other.getTagSpecifications() != null && other.getTagSpecifications().equals(this.getTagSpecifications()) == false)
return false;
if (other.getRoleArn() == null ^ this.getRoleArn() == null)
return false;
if (other.getRoleArn() != null && other.getRoleArn().equals(this.getRoleArn()) == false)
return false;
if (other.getTerminationPolicy() == null ^ this.getTerminationPolicy() == null)
return false;
if (other.getTerminationPolicy() != null && other.getTerminationPolicy().equals(this.getTerminationPolicy()) == false)
return false;
if (other.getFilesystemType() == null ^ this.getFilesystemType() == null)
return false;
if (other.getFilesystemType() != null && other.getFilesystemType().equals(this.getFilesystemType()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getEncrypted() == null) ? 0 : getEncrypted().hashCode());
hashCode = prime * hashCode + ((getKmsKeyId() == null) ? 0 : getKmsKeyId().hashCode());
hashCode = prime * hashCode + ((getVolumeType() == null) ? 0 : getVolumeType().hashCode());
hashCode = prime * hashCode + ((getSizeInGiB() == null) ? 0 : getSizeInGiB().hashCode());
hashCode = prime * hashCode + ((getSnapshotId() == null) ? 0 : getSnapshotId().hashCode());
hashCode = prime * hashCode + ((getIops() == null) ? 0 : getIops().hashCode());
hashCode = prime * hashCode + ((getThroughput() == null) ? 0 : getThroughput().hashCode());
hashCode = prime * hashCode + ((getTagSpecifications() == null) ? 0 : getTagSpecifications().hashCode());
hashCode = prime * hashCode + ((getRoleArn() == null) ? 0 : getRoleArn().hashCode());
hashCode = prime * hashCode + ((getTerminationPolicy() == null) ? 0 : getTerminationPolicy().hashCode());
hashCode = prime * hashCode + ((getFilesystemType() == null) ? 0 : getFilesystemType().hashCode());
return hashCode;
}
@Override
public TaskManagedEBSVolumeConfiguration clone() {
try {
return (TaskManagedEBSVolumeConfiguration) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.ecs.model.transform.TaskManagedEBSVolumeConfigurationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}